<img src="https://iili.io/HYBJFA7.png" width="64" height="64" align="right" />
# HomeSetup Python Library
>
> Because your Python code is not JUST a script !
[](https://pypi.org/project/hspylib)
[](https://gitter.im/hspylib/community)
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=J5CDEFLF6M3H4)
[](LICENSE.md)
[](docs/CHANGELOG.md#unreleased)
[](https://github.com/yorevs/hspylib/actions/workflows/build-and-test.yml)
HsPyLib is not just a Python library; it's a gateway to elevating your programming experience to new heights. Built on
established principles like **SOLID**, **DRY** (Don't Repeat Yourself), **KISS** (Keep It Simple, Stupid), and **YAGNI**
(You Ain’t Gonna Need It), HsPyLib offers a wealth of frameworks and features. It empowers you to craft sophisticated
Python3 applications, adhering to the philosophy that code should not merely be a simple script - it should be a part
of the 'PYCSNBASS' (Python Code Should Not Be A Simple Script) mindset.
> This project is a part of the [HomeSetup](https://github.com/yorevs/homesetup) project.
## Key Features
- Seamless installation process.
- Application manager offering a helpful scaffold for Python applications.
- Widgets manager for running both 'built-in' and custom Python widgets.
- Improved TUI (Text User Interface) helpers and input methods to enhance your User Experience with terminal applications.
- CRUD (Create, Read, Update, Delete) framework aiding with databases, repositories, and services.
- HTTP request helpers for simplified communication.
- Support for enabling Properties and AppConfigs using popular extensions like .properties, toml, yml, and more.
- Code rigorously tested and consistently adhering to Pylint standards.
- Utilizes the Gradle build system with numerous extensions.
- Diverse set of demos to facilitate a deeper understanding of the library.
- AI model integrations (currently supporting OpenAI).
> Create beautiful menu-select inputs
```python
class SelectableItem:
def __init__(self, name: str, value: str):
self.name = name
self.value = value
def __str__(self):
return f"Name: {self.name} Value: {self.value}"
def __repr__(self):
return str(self)
if __name__ == "__main__":
quantity = 22
digits = len(str(quantity))
it = [SelectableItem(f"Item-{n:>0{digits}}", f"Value-{n:>0{digits}}") for n in range(1, quantity)]
sel = mselect(it)
print(str(sel))
```
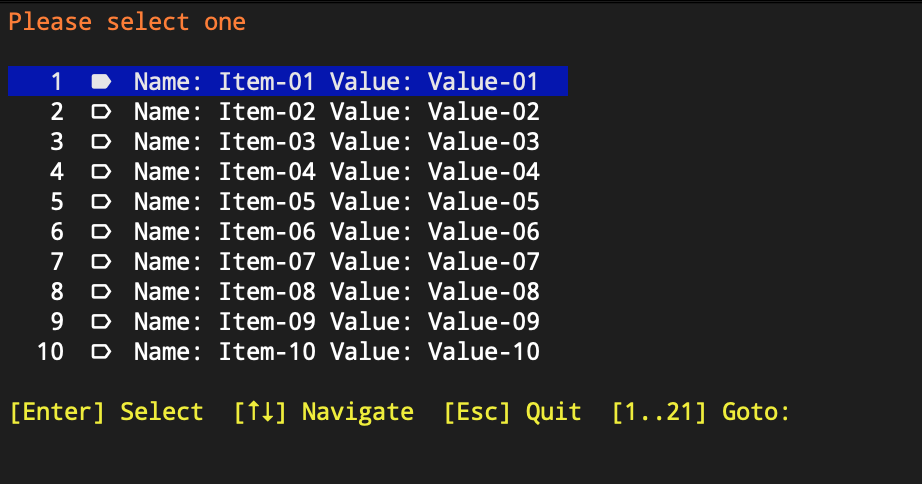
> And create beautiful menu-choose inputs
```python
class ChooseableItem:
def __init__(self, name: str, value: str):
self.name = name
self.value = value
def __str__(self):
return f"Name: {self.name} Value: {self.value}"
def __repr__(self):
return str(self)
if __name__ == "__main__":
quantity = 22
digits = len(str(quantity))
it = [ChooseableItem(f"Item-{n:>0{digits}}", f"Value-{n:>0{digits}}") for n in range(1, quantity)]
sel = mchoose(it, [n % 2 == 0 for n in range(1, quantity)])
print(str(sel))
```
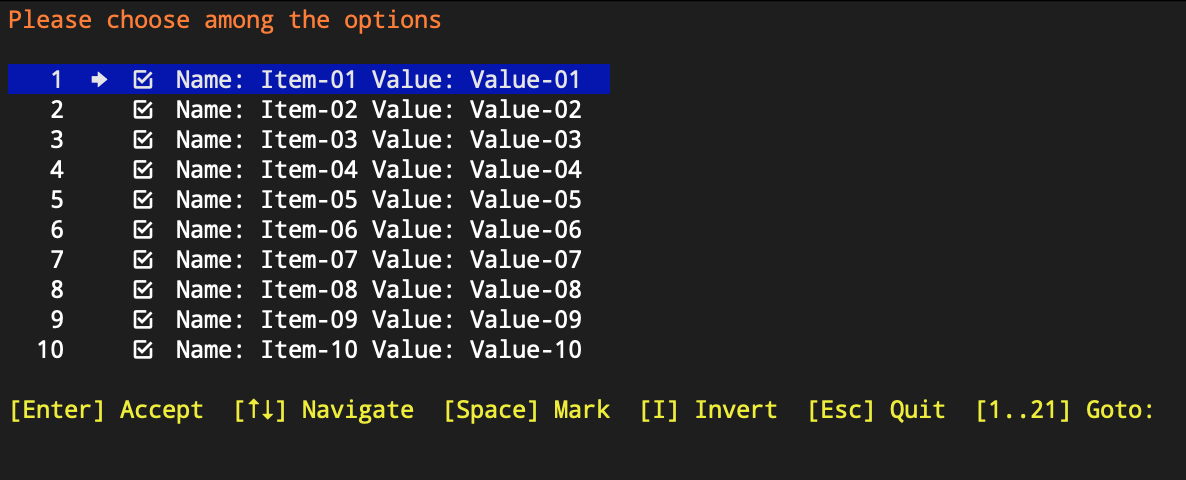
> And also, create beautiful form inputs
```python
from clitt.core.tui.minput.input_validator import InputValidator
from clitt.core.tui.minput.minput import MenuInput, minput
if __name__ == "__main__":
# fmt: off
form_fields = MenuInput.builder() \
.field() \
.label('letters') \
.validator(InputValidator.letters()) \
.build() \
.field() \
.label('word') \
.validator(InputValidator.words()) \
.build() \
.field() \
.label('number') \
.validator(InputValidator.numbers()) \
.min_max_length(1, 4) \
.build() \
.field() \
.label('masked') \
.itype('masked') \
.value('|##::##::## @@') \
.build() \
.field() \
.label('selectable') \
.itype('select') \
.value('one|two|three') \
.build() \
.field() \
.label('checkbox') \
.itype('checkbox') \
.build() \
.field() \
.label('password') \
.itype('password') \
.validator(InputValidator.anything()) \
.min_max_length(4, 8) \
.build() \
.field() \
.label('read-only') \
.access_type('read-only') \
.value('READ-ONLY') \
.build() \
.build()
# fmt: on
result = minput(form_fields)
print(result.__dict__ if result else "None")
```
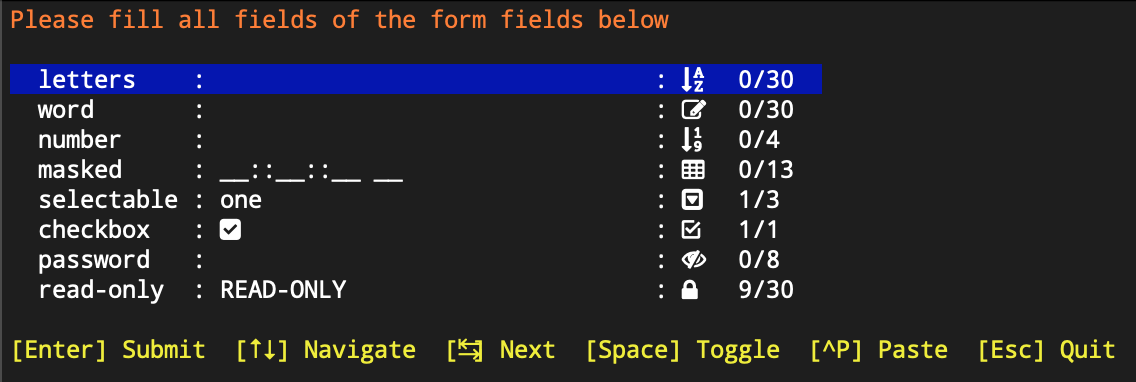
> Or even, create nice dashboards:
```python
if __name__ == "__main__":
# fmt: off
dashboard_items = MenuDashBoard.builder() \
.item() \
.icon(DashboardIcons.POWER) \
.tooltip('Do something') \
.on_trigger(lambda: print('Something')) \
.build() \
.item() \
.icon(DashboardIcons.MOVIE) \
.tooltip('Another something') \
.on_trigger(lambda: print('Another')) \
.build() \
.item() \
.icon(DashboardIcons.NOTIFICATION) \
.tooltip('Notify something') \
.on_trigger(lambda: print('Notification')) \
.build() \
.item() \
.icon(DashboardIcons.LIST) \
.tooltip('List everything') \
.on_trigger(lambda: print('List')) \
.build() \
.item() \
.icon(DashboardIcons.DATABASE) \
.tooltip('Database console') \
.on_trigger(lambda: print('Database')) \
.build() \
.item() \
.icon(DashboardIcons.EXIT) \
.tooltip('Exit application') \
.on_trigger(lambda: print('Exit')) \
.build() \
.build()
# fmt: on
result = mdashboard(dashboard_items)
```
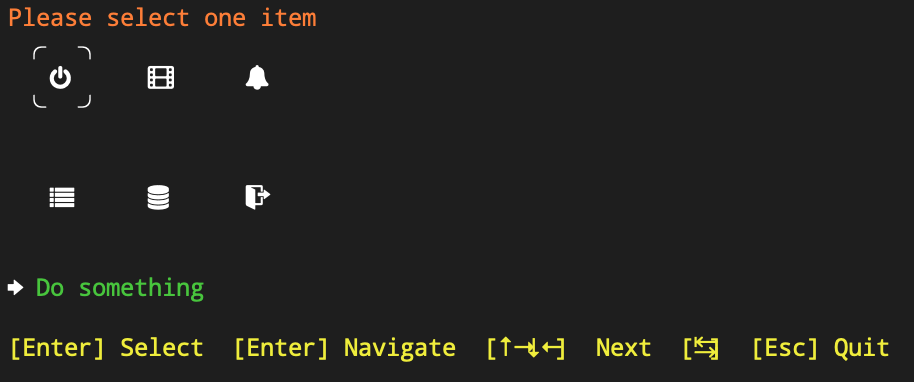
> And even more, create beautiful menus !
```bash
if __name__ == "__main__":
# fmt: off
main_menu = TUIMenuFactory \
.create_main_menu('TUI Main Menu', tooltip='Test Terminal UI Menus') \
.with_item('Sub-Menu-1') \
.with_action("DO IT 1", "Let's do it") \
.on_trigger(lambda x: print("ACTION 1", x)) \
.with_view("Just a View 1", "Show the view 1") \
.on_render("MY BEAUTIFUL VIEW 1") \
.with_action("Back", "Back to the previous menu") \
.on_trigger(TUIMenuUi.back) \
.then() \
.with_item('Sub-Menu-2') \
.with_action("DO IT 2", "Let's do it too") \
.on_trigger(lambda x: print("ACTION 2", x)) \
.with_view("Just a View 2", "Show the view 2") \
.on_render("MY BEAUTIFUL VIEW 2") \
.with_action("Back", "Back to the previous menu") \
.on_trigger(TUIMenuUi.back) \
.then() \
.then() \
.build()
# fmt: on
TUIMenuUi(main_menu, "TUI Main Menu").execute()
```
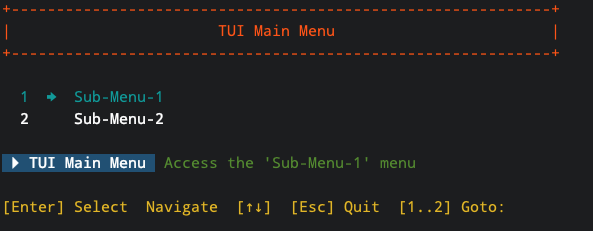
## PyPi Modules
- [A CLI Terminal](https://pypi.org/project/hspylib-clitt) tools.
- [Datasource](https://pypi.org/project/hspylib-datasource) framework.
- [A Firebase](https://pypi.org/project/hspylib-firebase) integration tool.
- [A PyQt](https://pypi.org/project/hspylib-hqt) application framework.
- [Core](https://pypi.org/project/hspylib) HomeSetup library.
- [A Kafka manager](https://pypi.org/project/hspylib-kafman) application tool.
- [A System Settings](https://pypi.org/project/hspylib-kafman) application tool.
- [A Vault](https://pypi.org/project/hspylib-vault) application tool.
## Installation
### Requirements
#### Python
- Python 3.10 and higher
#### Operating Systems
- Darwin
+ High Sierra and higher
- Linux
+ Ubuntu 16 and higher
+ CentOS 7 and higher
+ Fedora 31 and higher
You may want to install HsPyLib on other OS's and it will probably work, but there are no guarantees that it
**WILL ACTUALLY WORK**.
#### Required software
The following software are required:
- Git (To clone the github repository)
- Gradle (To build the HsPyLib project)
There are some python dependencies, but they will be automatically downloaded when the build runs.
### PyPi
To install HsPyLib from PyPi issue the command:
`# python3 -m pip install hspylib`
To upgrade HsPyLib use the command:
`# python3 -m pip install hspylib --upgrade`
Additional modules that can also be installed:
- [CLItt](https://pypi.org/project/hspylib-clitt) : `# python3 -m pip install hspylib-clitt` to install HsPyLib CLI terminal tools.
- [Firebase](https://pypi.org/project/hspylib-firebase) : `# python3 -m pip install hspylib-firebase` to install HsPyLib Firebase application.
- [Vault](https://pypi.org/project/hspylib-vault) : `# python3 -m pip install hspylib-vault` to install HsPyLib Vault application.
- [Kafman](https://pypi.org/project/hspylib-kafman) : `# python3 -m pip install hspylib-kafman` to install Kafka manager.
- [Datasource](https://pypi.org/project/hspylib-datasource) : `# python3 -m pip install hspylib-datasource` to install datasource helpers.
- [HQT](https://pypi.org/project/hspylib-hqt) : `# python3 -m pip install hspylib-hqt` to install HsPyLib PyQt framework.
### GitHub
To clone HsPyLib into your local machine type the command:
`# git clone https://github.com/yorevs/hspylib.git`
## Documentation
The API documentation can be found [here](docs/api/index.html)
## Support
> Your support and contributions are greatly appreciated in helping us improve and enhance HomeSetup. Together, we can
make it even better!
You can support HomeSetup by [donating](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=J5CDEFLF6M3H4)
or contributing code. Feel free to contact me for further details. When making code contributions, please make sure to
review our [guidelines](docs/CONTRIBUTING.md) and adhere to our [code of conduct](docs/CODE_OF_CONDUCT.md).
[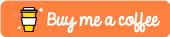](https://www.buymeacoffee.com/yorevs)
You can also sponsor it by using our [GitHub Sponsors](https://github.com/sponsors/yorevs) page.
This project is already supported by:
<a href="https://www.jetbrains.com/community/opensource/?utm_campaign=opensource&utm_content=approved&utm_medium=email&utm_source=newsletter&utm_term=jblogo#support">
<img src="https://resources.jetbrains.com/storage/products/company/brand/logos/jb_beam.png" width="120" height="120">
</a>
Thank you <3 !!
## Known Issues
- [In-Progress] We are aware that there is a problems when using python@3.12 and we are already working on a fix.
## Contacts
- Documentation: [API](docs/api/index.html)
- License: [MIT](LICENSE.md)
- Issue tracker: [HSPyLib-Issues](https://github.com/yorevs/hspylib/issues)
- Official chat: [HSPyLib](https://gitter.im/hspylib/community)
- Contact: [yorevs](https://www.reddit.com/user/yorevs)
- Mailto: [yorevs](mailto:yorevs@hotmail.com)
Raw data
{
"_id": null,
"home_page": "https://github.com/yorevs/hspylib",
"name": "hspylib-hqt",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": null,
"keywords": "qt, ui, extensions, application, pyqt",
"author": "Hugo Saporetti Junior",
"author_email": "yorevs@hotmail.com",
"download_url": "https://files.pythonhosted.org/packages/57/12/2b970f6a8f574fff05541cf879c4459b953e730374dace663f73d7e722d8/hspylib_hqt-0.9.43.tar.gz",
"platform": "Darwin",
"description": "<img src=\"https://iili.io/HYBJFA7.png\" width=\"64\" height=\"64\" align=\"right\" />\n\n# HomeSetup Python Library\n>\n> Because your Python code is not JUST a script !\n\n[](https://pypi.org/project/hspylib)\n[](https://gitter.im/hspylib/community)\n[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=J5CDEFLF6M3H4)\n[](LICENSE.md)\n[](docs/CHANGELOG.md#unreleased)\n[](https://github.com/yorevs/hspylib/actions/workflows/build-and-test.yml)\n\nHsPyLib is not just a Python library; it's a gateway to elevating your programming experience to new heights. Built on\nestablished principles like **SOLID**, **DRY** (Don't Repeat Yourself), **KISS** (Keep It Simple, Stupid), and **YAGNI**\n(You Ain\u2019t Gonna Need It), HsPyLib offers a wealth of frameworks and features. It empowers you to craft sophisticated\nPython3 applications, adhering to the philosophy that code should not merely be a simple script - it should be a part\nof the 'PYCSNBASS' (Python Code Should Not Be A Simple Script) mindset.\n\n> This project is a part of the [HomeSetup](https://github.com/yorevs/homesetup) project.\n\n## Key Features\n\n- Seamless installation process.\n- Application manager offering a helpful scaffold for Python applications.\n- Widgets manager for running both 'built-in' and custom Python widgets.\n- Improved TUI (Text User Interface) helpers and input methods to enhance your User Experience with terminal applications.\n- CRUD (Create, Read, Update, Delete) framework aiding with databases, repositories, and services.\n- HTTP request helpers for simplified communication.\n- Support for enabling Properties and AppConfigs using popular extensions like .properties, toml, yml, and more.\n- Code rigorously tested and consistently adhering to Pylint standards.\n- Utilizes the Gradle build system with numerous extensions.\n- Diverse set of demos to facilitate a deeper understanding of the library.\n- AI model integrations (currently supporting OpenAI).\n\n> Create beautiful menu-select inputs\n\n```python\nclass SelectableItem:\n def __init__(self, name: str, value: str):\n self.name = name\n self.value = value\n\n def __str__(self):\n return f\"Name: {self.name} Value: {self.value}\"\n\n def __repr__(self):\n return str(self)\n\n\nif __name__ == \"__main__\":\n quantity = 22\n digits = len(str(quantity))\n it = [SelectableItem(f\"Item-{n:>0{digits}}\", f\"Value-{n:>0{digits}}\") for n in range(1, quantity)]\n sel = mselect(it)\n print(str(sel))\n```\n\n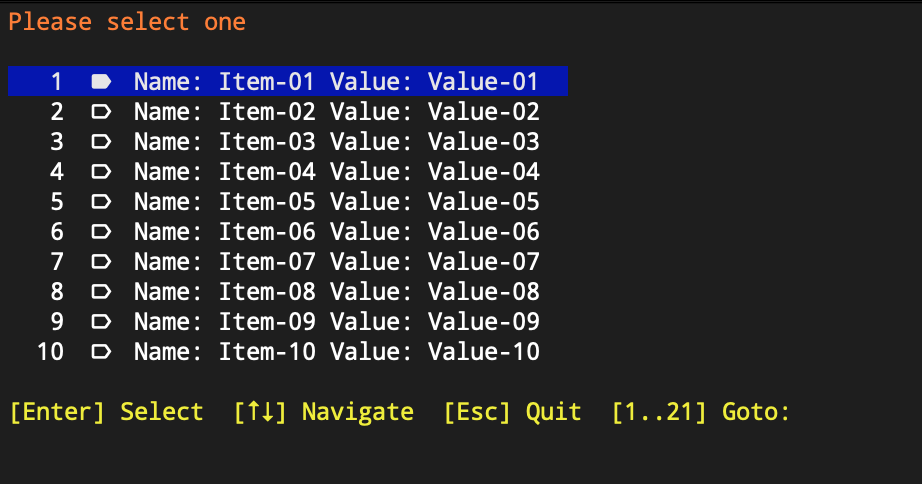\n\n> And create beautiful menu-choose inputs\n\n```python\nclass ChooseableItem:\n def __init__(self, name: str, value: str):\n self.name = name\n self.value = value\n\n def __str__(self):\n return f\"Name: {self.name} Value: {self.value}\"\n\n def __repr__(self):\n return str(self)\n\n\nif __name__ == \"__main__\":\n quantity = 22\n digits = len(str(quantity))\n it = [ChooseableItem(f\"Item-{n:>0{digits}}\", f\"Value-{n:>0{digits}}\") for n in range(1, quantity)]\n sel = mchoose(it, [n % 2 == 0 for n in range(1, quantity)])\n print(str(sel))\n```\n\n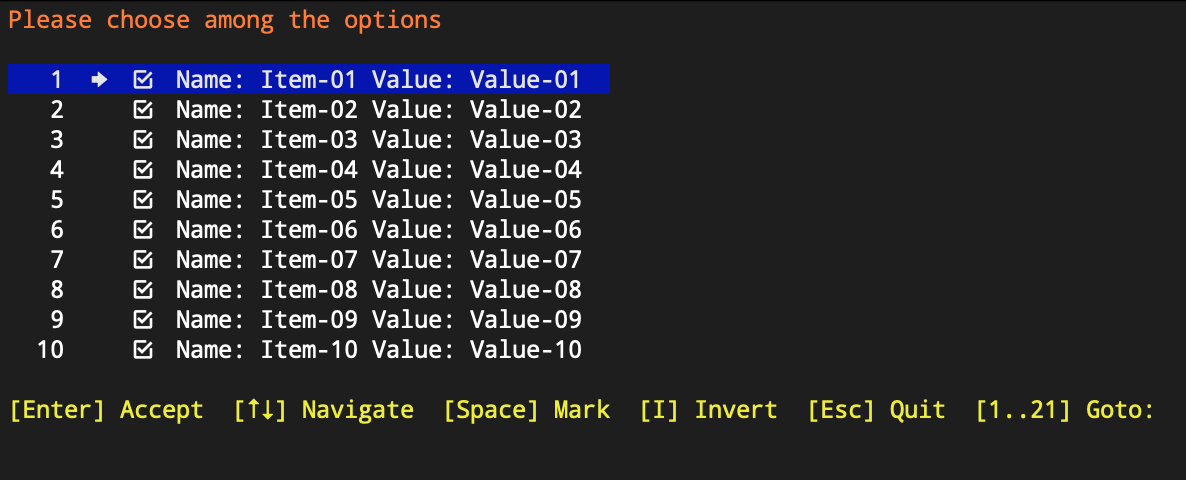\n\n> And also, create beautiful form inputs\n\n```python\nfrom clitt.core.tui.minput.input_validator import InputValidator\nfrom clitt.core.tui.minput.minput import MenuInput, minput\n\nif __name__ == \"__main__\":\n # fmt: off\n form_fields = MenuInput.builder() \\\n .field() \\\n .label('letters') \\\n .validator(InputValidator.letters()) \\\n .build() \\\n .field() \\\n .label('word') \\\n .validator(InputValidator.words()) \\\n .build() \\\n .field() \\\n .label('number') \\\n .validator(InputValidator.numbers()) \\\n .min_max_length(1, 4) \\\n .build() \\\n .field() \\\n .label('masked') \\\n .itype('masked') \\\n .value('|##::##::## @@') \\\n .build() \\\n .field() \\\n .label('selectable') \\\n .itype('select') \\\n .value('one|two|three') \\\n .build() \\\n .field() \\\n .label('checkbox') \\\n .itype('checkbox') \\\n .build() \\\n .field() \\\n .label('password') \\\n .itype('password') \\\n .validator(InputValidator.anything()) \\\n .min_max_length(4, 8) \\\n .build() \\\n .field() \\\n .label('read-only') \\\n .access_type('read-only') \\\n .value('READ-ONLY') \\\n .build() \\\n .build()\n # fmt: on\n\n result = minput(form_fields)\n print(result.__dict__ if result else \"None\")\n```\n\n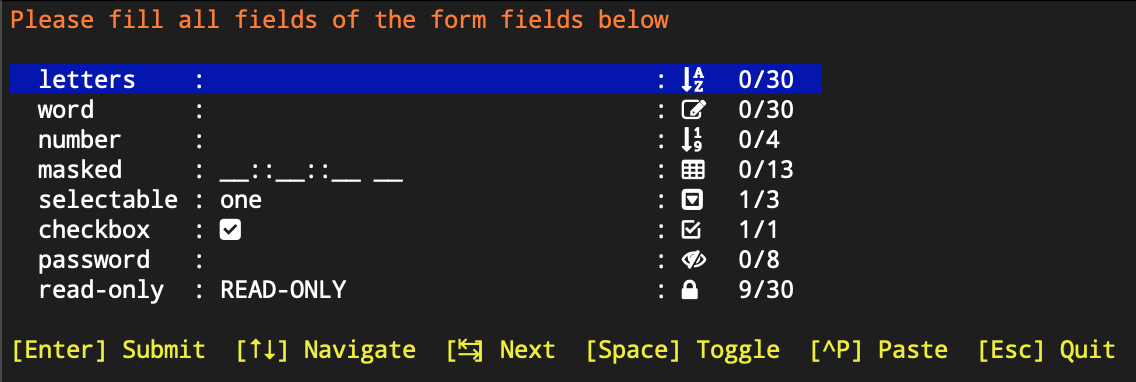\n\n> Or even, create nice dashboards:\n\n```python\nif __name__ == \"__main__\":\n # fmt: off\n dashboard_items = MenuDashBoard.builder() \\\n .item() \\\n .icon(DashboardIcons.POWER) \\\n .tooltip('Do something') \\\n .on_trigger(lambda: print('Something')) \\\n .build() \\\n .item() \\\n .icon(DashboardIcons.MOVIE) \\\n .tooltip('Another something') \\\n .on_trigger(lambda: print('Another')) \\\n .build() \\\n .item() \\\n .icon(DashboardIcons.NOTIFICATION) \\\n .tooltip('Notify something') \\\n .on_trigger(lambda: print('Notification')) \\\n .build() \\\n .item() \\\n .icon(DashboardIcons.LIST) \\\n .tooltip('List everything') \\\n .on_trigger(lambda: print('List')) \\\n .build() \\\n .item() \\\n .icon(DashboardIcons.DATABASE) \\\n .tooltip('Database console') \\\n .on_trigger(lambda: print('Database')) \\\n .build() \\\n .item() \\\n .icon(DashboardIcons.EXIT) \\\n .tooltip('Exit application') \\\n .on_trigger(lambda: print('Exit')) \\\n .build() \\\n .build()\n # fmt: on\n\n result = mdashboard(dashboard_items)\n```\n\n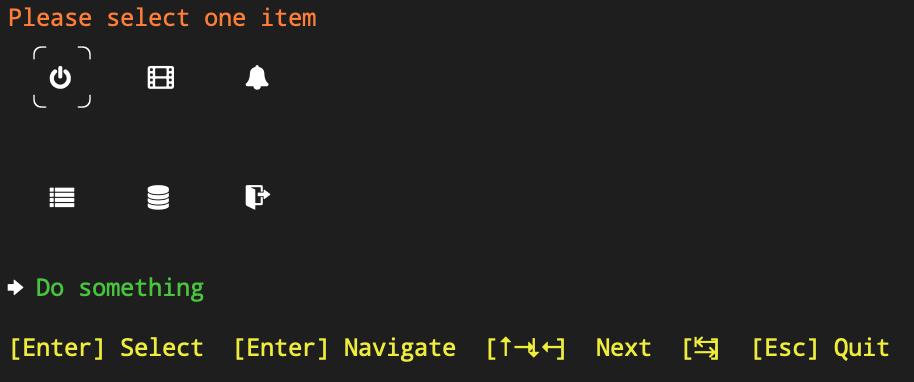\n\n> And even more, create beautiful menus !\n\n```bash\nif __name__ == \"__main__\":\n # fmt: off\n main_menu = TUIMenuFactory \\\n .create_main_menu('TUI Main Menu', tooltip='Test Terminal UI Menus') \\\n .with_item('Sub-Menu-1') \\\n .with_action(\"DO IT 1\", \"Let's do it\") \\\n .on_trigger(lambda x: print(\"ACTION 1\", x)) \\\n .with_view(\"Just a View 1\", \"Show the view 1\") \\\n .on_render(\"MY BEAUTIFUL VIEW 1\") \\\n .with_action(\"Back\", \"Back to the previous menu\") \\\n .on_trigger(TUIMenuUi.back) \\\n .then() \\\n .with_item('Sub-Menu-2') \\\n .with_action(\"DO IT 2\", \"Let's do it too\") \\\n .on_trigger(lambda x: print(\"ACTION 2\", x)) \\\n .with_view(\"Just a View 2\", \"Show the view 2\") \\\n .on_render(\"MY BEAUTIFUL VIEW 2\") \\\n .with_action(\"Back\", \"Back to the previous menu\") \\\n .on_trigger(TUIMenuUi.back) \\\n .then() \\\n .then() \\\n .build()\n # fmt: on\n\n TUIMenuUi(main_menu, \"TUI Main Menu\").execute()\n```\n\n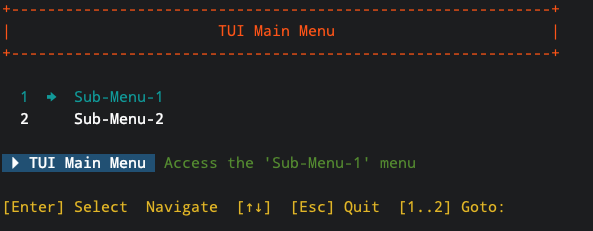\n\n## PyPi Modules\n\n- [A CLI Terminal](https://pypi.org/project/hspylib-clitt) tools.\n- [Datasource](https://pypi.org/project/hspylib-datasource) framework.\n- [A Firebase](https://pypi.org/project/hspylib-firebase) integration tool.\n- [A PyQt](https://pypi.org/project/hspylib-hqt) application framework.\n- [Core](https://pypi.org/project/hspylib) HomeSetup library.\n- [A Kafka manager](https://pypi.org/project/hspylib-kafman) application tool.\n- [A System Settings](https://pypi.org/project/hspylib-kafman) application tool.\n- [A Vault](https://pypi.org/project/hspylib-vault) application tool.\n\n## Installation\n\n### Requirements\n\n#### Python\n\n- Python 3.10 and higher\n\n#### Operating Systems\n\n- Darwin\n + High Sierra and higher\n- Linux\n + Ubuntu 16 and higher\n + CentOS 7 and higher\n + Fedora 31 and higher\n\nYou may want to install HsPyLib on other OS's and it will probably work, but there are no guarantees that it\n**WILL ACTUALLY WORK**.\n\n#### Required software\n\nThe following software are required:\n\n- Git (To clone the github repository)\n- Gradle (To build the HsPyLib project)\n\nThere are some python dependencies, but they will be automatically downloaded when the build runs.\n\n### PyPi\n\nTo install HsPyLib from PyPi issue the command:\n\n`# python3 -m pip install hspylib`\n\nTo upgrade HsPyLib use the command:\n\n`# python3 -m pip install hspylib --upgrade`\n\nAdditional modules that can also be installed:\n\n- [CLItt](https://pypi.org/project/hspylib-clitt) : `# python3 -m pip install hspylib-clitt` to install HsPyLib CLI terminal tools.\n- [Firebase](https://pypi.org/project/hspylib-firebase) : `# python3 -m pip install hspylib-firebase` to install HsPyLib Firebase application.\n- [Vault](https://pypi.org/project/hspylib-vault) : `# python3 -m pip install hspylib-vault` to install HsPyLib Vault application.\n- [Kafman](https://pypi.org/project/hspylib-kafman) : `# python3 -m pip install hspylib-kafman` to install Kafka manager.\n- [Datasource](https://pypi.org/project/hspylib-datasource) : `# python3 -m pip install hspylib-datasource` to install datasource helpers.\n- [HQT](https://pypi.org/project/hspylib-hqt) : `# python3 -m pip install hspylib-hqt` to install HsPyLib PyQt framework.\n\n### GitHub\n\nTo clone HsPyLib into your local machine type the command:\n\n`# git clone https://github.com/yorevs/hspylib.git`\n\n## Documentation\n\nThe API documentation can be found [here](docs/api/index.html)\n\n## Support\n\n> Your support and contributions are greatly appreciated in helping us improve and enhance HomeSetup. Together, we can\nmake it even better!\n\nYou can support HomeSetup by [donating](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=J5CDEFLF6M3H4)\nor contributing code. Feel free to contact me for further details. When making code contributions, please make sure to\nreview our [guidelines](docs/CONTRIBUTING.md) and adhere to our [code of conduct](docs/CODE_OF_CONDUCT.md).\n\n[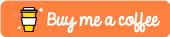](https://www.buymeacoffee.com/yorevs)\n\nYou can also sponsor it by using our [GitHub Sponsors](https://github.com/sponsors/yorevs) page.\n\nThis project is already supported by:\n\n<a href=\"https://www.jetbrains.com/community/opensource/?utm_campaign=opensource&utm_content=approved&utm_medium=email&utm_source=newsletter&utm_term=jblogo#support\">\n <img src=\"https://resources.jetbrains.com/storage/products/company/brand/logos/jb_beam.png\" width=\"120\" height=\"120\">\n</a>\n\nThank you <3 !!\n\n## Known Issues\n\n- [In-Progress] We are aware that there is a problems when using python@3.12 and we are already working on a fix.\n\n## Contacts\n\n- Documentation: [API](docs/api/index.html)\n- License: [MIT](LICENSE.md)\n- Issue tracker: [HSPyLib-Issues](https://github.com/yorevs/hspylib/issues)\n- Official chat: [HSPyLib](https://gitter.im/hspylib/community)\n- Contact: [yorevs](https://www.reddit.com/user/yorevs)\n- Mailto: [yorevs](mailto:yorevs@hotmail.com)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "HsPyLib - QT framework extensions",
"version": "0.9.43",
"project_urls": {
"GitHub": "https://github.com/yorevs/hspylib",
"Homepage": "https://github.com/yorevs/hspylib",
"PyPi": "https://pypi.org/project/hspylib-hqt/"
},
"split_keywords": [
"qt",
" ui",
" extensions",
" application",
" pyqt"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "dbdbb8f74582b0a26ecce0bc6a9533948c55e6892d7645151677789bf3c7fd20",
"md5": "966651c4e85a95b4c97070858d0f5aa5",
"sha256": "964b1c4576c15a14ffb01a8a09a820151cbc454f57bf7abd58cb4448087234d0"
},
"downloads": -1,
"filename": "hspylib_hqt-0.9.43-py3-none-any.whl",
"has_sig": false,
"md5_digest": "966651c4e85a95b4c97070858d0f5aa5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 25855,
"upload_time": "2024-10-03T18:38:43",
"upload_time_iso_8601": "2024-10-03T18:38:43.603629Z",
"url": "https://files.pythonhosted.org/packages/db/db/b8f74582b0a26ecce0bc6a9533948c55e6892d7645151677789bf3c7fd20/hspylib_hqt-0.9.43-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "57122b970f6a8f574fff05541cf879c4459b953e730374dace663f73d7e722d8",
"md5": "401abffa69b78b5f4729dbafccf78ea6",
"sha256": "d89a3754bf4e46b205611c823bfff98832c46c834fa3b6b5fb47752647f33812"
},
"downloads": -1,
"filename": "hspylib_hqt-0.9.43.tar.gz",
"has_sig": false,
"md5_digest": "401abffa69b78b5f4729dbafccf78ea6",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 22344,
"upload_time": "2024-10-03T18:38:45",
"upload_time_iso_8601": "2024-10-03T18:38:45.353251Z",
"url": "https://files.pythonhosted.org/packages/57/12/2b970f6a8f574fff05541cf879c4459b953e730374dace663f73d7e722d8/hspylib_hqt-0.9.43.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-03 18:38:45",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "yorevs",
"github_project": "hspylib",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "hspylib-hqt"
}