Name | hypothesis-auto JSON |
Version |
1.1.5
JSON |
| download |
home_page | |
Summary | Extends Hypothesis to add fully automatic testing of type annotated functions |
upload_time | 2023-01-19 05:22:16 |
maintainer | |
docs_url | None |
author | Timothy Crosley |
requires_python | >=3.6 |
license | MIT |
keywords |
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
[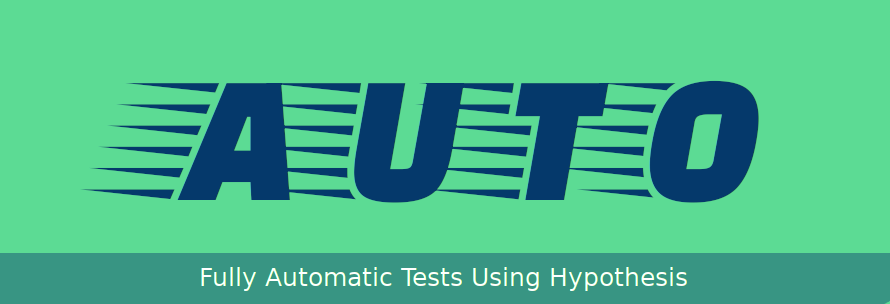](https://timothycrosley.github.io/hypothesis-auto/)
_________________
[](http://badge.fury.io/py/hypothesis-auto)
[](https://travis-ci.org/timothycrosley/hypothesis-auto)
[](https://codecov.io/gh/timothycrosley/hypothesis-auto)
[](https://gitter.im/timothycrosley/hypothesis-auto?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](https://pypi.python.org/pypi/hypothesis-auto/)
[](https://pepy.tech/project/hypothesis-auto)
_________________
[Read Latest Documentation](https://timothycrosley.github.io/hypothesis-auto/) - [Browse GitHub Code Repository](https://github.com/timothycrosley/hypothesis-auto/)
_________________
**hypothesis-auto** is an extension for the [Hypothesis](https://hypothesis.readthedocs.io/en/latest/) project that enables fully automatic tests for type annotated functions.
[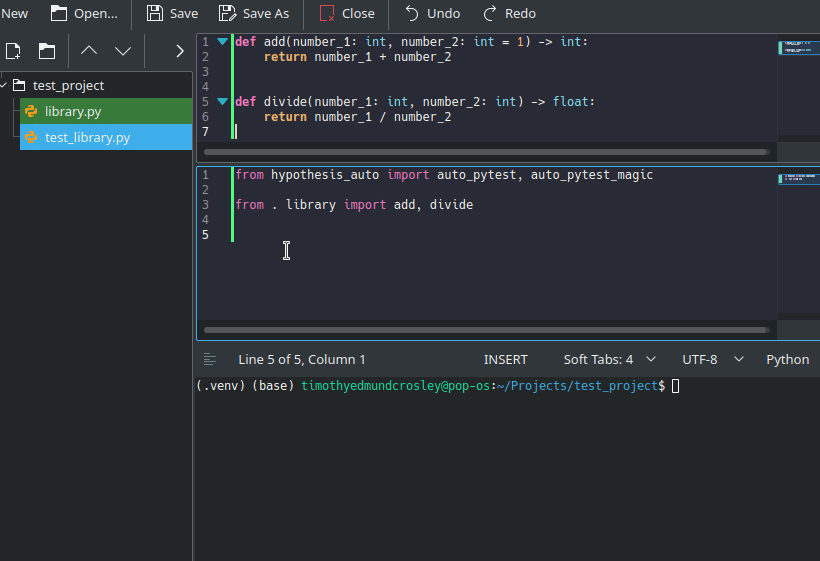](https://github.com/timothycrosley/hypothesis-auto/blob/master/art/demo.gif)
Key Features:
* **Type Annotation Powered**: Utilize your function's existing type annotations to build dozens of test cases automatically.
* **Low Barrier**: Start utilizing property-based testing in the lowest barrier way possible. Just run `auto_test(FUNCTION)` to run dozens of test.
* **pytest Compatible**: Like Hypothesis itself, hypothesis-auto has built-in compatibility with the popular [pytest](https://docs.pytest.org/en/latest/) testing framework. This means that you can turn your automatically generated tests into individual pytest test cases with one line.
* **Scales Up**: As you find your self needing to customize your auto_test cases, you can easily utilize all the features of [Hypothesis](https://hypothesis.readthedocs.io/en/latest/), including custom strategies per a parameter.
## Installation:
To get started - install `hypothesis-auto` into your projects virtual environment:
`pip3 install hypothesis-auto`
OR
`poetry add hypothesis-auto`
OR
`pipenv install hypothesis-auto`
## Usage Examples:
!!! warning
In old usage examples you will see `_` prefixed parameters like `_auto_verify=`. This was done to avoid conflicting with existing function parameters.
Based on community feedback the project switched to `_` suffixes, such as `auto_verify_=` to keep the likely hood of conflicting low while
avoiding the connotation of private parameters.
### Framework independent usage
#### Basic `auto_test` usage:
```python3
from hypothesis_auto import auto_test
def add(number_1: int, number_2: int = 1) -> int:
return number_1 + number_2
auto_test(add) # 50 property based scenarios are generated and ran against add
auto_test(add, auto_runs_=1_000) # Let's make that 1,000
```
#### Adding an allowed exception:
```python3
from hypothesis_auto import auto_test
def divide(number_1: int, number_2: int) -> int:
return number_1 / number_2
auto_test(divide)
-> 1012 raise the_error_hypothesis_found
1013
1014 for attrib in dir(test):
<ipython-input-2-65a3aa66e9f9> in divide(number_1, number_2)
1 def divide(number_1: int, number_2: int) -> int:
----> 2 return number_1 / number_2
3
0/0
ZeroDivisionError: division by zero
auto_test(divide, auto_allow_exceptions_=(ZeroDivisionError, ))
```
#### Using `auto_test` with a custom verification method:
```python3
from hypothesis_auto import Scenario, auto_test
def add(number_1: int, number_2: int = 1) -> int:
return number_1 + number_2
def my_custom_verifier(scenario: Scenario):
if scenario.kwargs["number_1"] > 0 and scenario.kwargs["number_2"] > 0:
assert scenario.result > scenario.kwargs["number_1"]
assert scenario.result > scenario.kwargs["number_2"]
elif scenario.kwargs["number_1"] < 0 and scenario.kwargs["number_2"] < 0:
assert scenario.result < scenario.kwargs["number_1"]
assert scenario.result < scenario.kwargs["number_2"]
else:
assert scenario.result >= min(scenario.kwargs.values())
assert scenario.result <= max(scenario.kwargs.values())
auto_test(add, auto_verify_=my_custom_verifier)
```
Custom verification methods should take a single [Scenario](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/#scenario) and raise an exception to signify errors.
For the full set of parameters, you can pass into auto_test see its [API reference documentation](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/).
### pytest usage
#### Using `auto_pytest_magic` to auto-generate dozens of pytest test cases:
```python3
from hypothesis_auto import auto_pytest_magic
def add(number_1: int, number_2: int = 1) -> int:
return number_1 + number_2
auto_pytest_magic(add)
```
#### Using `auto_pytest` to run dozens of test case within a temporary directory:
```python3
from hypothesis_auto import auto_pytest
def add(number_1: int, number_2: int = 1) -> int:
return number_1 + number_2
@auto_pytest()
def test_add(test_case, tmpdir):
tmpdir.mkdir().chdir()
test_case()
```
#### Using `auto_pytest_magic` with a custom verification method:
```python3
from hypothesis_auto import Scenario, auto_pytest
def add(number_1: int, number_2: int = 1) -> int:
return number_1 + number_2
def my_custom_verifier(scenario: Scenario):
if scenario.kwargs["number_1"] > 0 and scenario.kwargs["number_2"] > 0:
assert scenario.result > scenario.kwargs["number_1"]
assert scenario.result > scenario.kwargs["number_2"]
elif scenario.kwargs["number_1"] < 0 and scenario.kwargs["number_2"] < 0:
assert scenario.result < scenario.kwargs["number_1"]
assert scenario.result < scenario.kwargs["number_2"]
else:
assert scenario.result >= min(scenario.kwargs.values())
assert scenario.result <= max(scenario.kwargs.values())
auto_pytest_magic(add, auto_verify_=my_custom_verifier)
```
Custom verification methods should take a single [Scenario](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/#scenario) and raise an exception to signify errors.
For the full reference of the pytest integration API see the [API reference documentation](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/pytest/).
## Why Create hypothesis-auto?
I wanted a no/low resistance way to start incorporating property-based tests across my projects. Such a solution that also encouraged the use of type hints was a win/win for me.
I hope you too find `hypothesis-auto` useful!
~Timothy Crosley
Raw data
{
"_id": null,
"home_page": "",
"name": "hypothesis-auto",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "",
"author": "Timothy Crosley",
"author_email": "timothy.crosley@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/14/9d/a491aaf55e61b4fee99508d8d2ede409c760ced3e0156d78bcbd34a8e4df/hypothesis_auto-1.1.5.tar.gz",
"platform": null,
"description": "[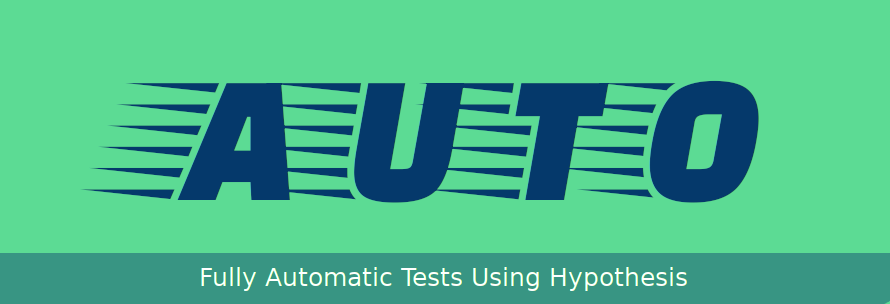](https://timothycrosley.github.io/hypothesis-auto/)\n_________________\n\n[](http://badge.fury.io/py/hypothesis-auto)\n[](https://travis-ci.org/timothycrosley/hypothesis-auto)\n[](https://codecov.io/gh/timothycrosley/hypothesis-auto)\n[](https://gitter.im/timothycrosley/hypothesis-auto?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)\n[](https://pypi.python.org/pypi/hypothesis-auto/)\n[](https://pepy.tech/project/hypothesis-auto)\n_________________\n\n[Read Latest Documentation](https://timothycrosley.github.io/hypothesis-auto/) - [Browse GitHub Code Repository](https://github.com/timothycrosley/hypothesis-auto/)\n_________________\n\n**hypothesis-auto** is an extension for the [Hypothesis](https://hypothesis.readthedocs.io/en/latest/) project that enables fully automatic tests for type annotated functions.\n\n[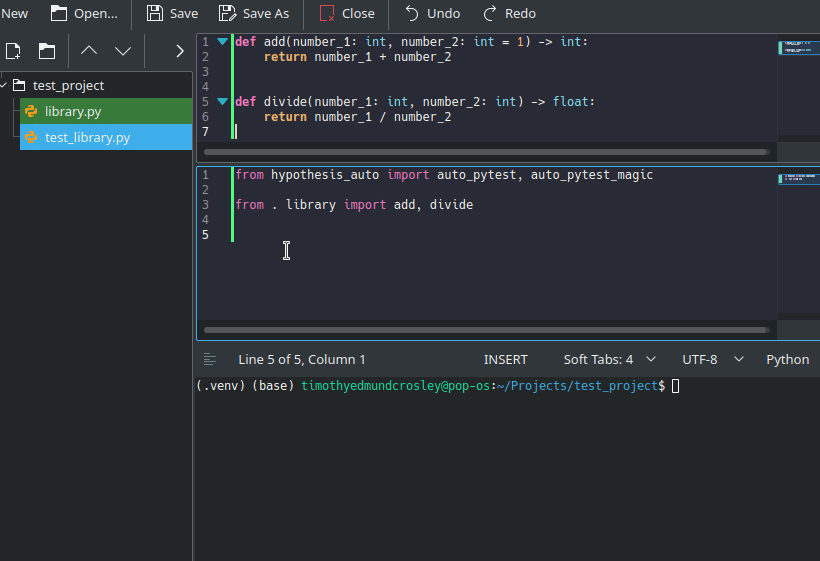](https://github.com/timothycrosley/hypothesis-auto/blob/master/art/demo.gif)\n\nKey Features:\n\n* **Type Annotation Powered**: Utilize your function's existing type annotations to build dozens of test cases automatically.\n* **Low Barrier**: Start utilizing property-based testing in the lowest barrier way possible. Just run `auto_test(FUNCTION)` to run dozens of test.\n* **pytest Compatible**: Like Hypothesis itself, hypothesis-auto has built-in compatibility with the popular [pytest](https://docs.pytest.org/en/latest/) testing framework. This means that you can turn your automatically generated tests into individual pytest test cases with one line.\n* **Scales Up**: As you find your self needing to customize your auto_test cases, you can easily utilize all the features of [Hypothesis](https://hypothesis.readthedocs.io/en/latest/), including custom strategies per a parameter.\n\n## Installation:\n\nTo get started - install `hypothesis-auto` into your projects virtual environment:\n\n`pip3 install hypothesis-auto`\n\nOR\n\n`poetry add hypothesis-auto`\n\nOR\n\n`pipenv install hypothesis-auto`\n\n## Usage Examples:\n\n!!! warning\n In old usage examples you will see `_` prefixed parameters like `_auto_verify=`. This was done to avoid conflicting with existing function parameters.\n Based on community feedback the project switched to `_` suffixes, such as `auto_verify_=` to keep the likely hood of conflicting low while\n avoiding the connotation of private parameters.\n\n### Framework independent usage\n\n#### Basic `auto_test` usage:\n\n```python3\nfrom hypothesis_auto import auto_test\n\n\ndef add(number_1: int, number_2: int = 1) -> int:\n return number_1 + number_2\n\n\nauto_test(add) # 50 property based scenarios are generated and ran against add\nauto_test(add, auto_runs_=1_000) # Let's make that 1,000\n```\n\n#### Adding an allowed exception:\n\n```python3\nfrom hypothesis_auto import auto_test\n\n\ndef divide(number_1: int, number_2: int) -> int:\n return number_1 / number_2\n\nauto_test(divide)\n\n-> 1012 raise the_error_hypothesis_found\n 1013\n 1014 for attrib in dir(test):\n\n<ipython-input-2-65a3aa66e9f9> in divide(number_1, number_2)\n 1 def divide(number_1: int, number_2: int) -> int:\n----> 2 return number_1 / number_2\n 3\n\n0/0\n\nZeroDivisionError: division by zero\n\n\nauto_test(divide, auto_allow_exceptions_=(ZeroDivisionError, ))\n```\n\n#### Using `auto_test` with a custom verification method:\n\n```python3\nfrom hypothesis_auto import Scenario, auto_test\n\n\ndef add(number_1: int, number_2: int = 1) -> int:\n return number_1 + number_2\n\n\ndef my_custom_verifier(scenario: Scenario):\n if scenario.kwargs[\"number_1\"] > 0 and scenario.kwargs[\"number_2\"] > 0:\n assert scenario.result > scenario.kwargs[\"number_1\"]\n assert scenario.result > scenario.kwargs[\"number_2\"]\n elif scenario.kwargs[\"number_1\"] < 0 and scenario.kwargs[\"number_2\"] < 0:\n assert scenario.result < scenario.kwargs[\"number_1\"]\n assert scenario.result < scenario.kwargs[\"number_2\"]\n else:\n assert scenario.result >= min(scenario.kwargs.values())\n assert scenario.result <= max(scenario.kwargs.values())\n\n\nauto_test(add, auto_verify_=my_custom_verifier)\n```\n\nCustom verification methods should take a single [Scenario](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/#scenario) and raise an exception to signify errors.\n\nFor the full set of parameters, you can pass into auto_test see its [API reference documentation](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/).\n\n### pytest usage\n\n#### Using `auto_pytest_magic` to auto-generate dozens of pytest test cases:\n\n```python3\nfrom hypothesis_auto import auto_pytest_magic\n\n\ndef add(number_1: int, number_2: int = 1) -> int:\n return number_1 + number_2\n\n\nauto_pytest_magic(add)\n```\n\n#### Using `auto_pytest` to run dozens of test case within a temporary directory:\n\n```python3\nfrom hypothesis_auto import auto_pytest\n\n\ndef add(number_1: int, number_2: int = 1) -> int:\n return number_1 + number_2\n\n\n@auto_pytest()\ndef test_add(test_case, tmpdir):\n tmpdir.mkdir().chdir()\n test_case()\n```\n\n#### Using `auto_pytest_magic` with a custom verification method:\n\n```python3\nfrom hypothesis_auto import Scenario, auto_pytest\n\n\ndef add(number_1: int, number_2: int = 1) -> int:\n return number_1 + number_2\n\n\ndef my_custom_verifier(scenario: Scenario):\n if scenario.kwargs[\"number_1\"] > 0 and scenario.kwargs[\"number_2\"] > 0:\n assert scenario.result > scenario.kwargs[\"number_1\"]\n assert scenario.result > scenario.kwargs[\"number_2\"]\n elif scenario.kwargs[\"number_1\"] < 0 and scenario.kwargs[\"number_2\"] < 0:\n assert scenario.result < scenario.kwargs[\"number_1\"]\n assert scenario.result < scenario.kwargs[\"number_2\"]\n else:\n assert scenario.result >= min(scenario.kwargs.values())\n assert scenario.result <= max(scenario.kwargs.values())\n\n\nauto_pytest_magic(add, auto_verify_=my_custom_verifier)\n```\n\nCustom verification methods should take a single [Scenario](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/tester/#scenario) and raise an exception to signify errors.\n\nFor the full reference of the pytest integration API see the [API reference documentation](https://timothycrosley.github.io/hypothesis-auto/reference/hypothesis_auto/pytest/).\n\n## Why Create hypothesis-auto?\n\nI wanted a no/low resistance way to start incorporating property-based tests across my projects. Such a solution that also encouraged the use of type hints was a win/win for me.\n\nI hope you too find `hypothesis-auto` useful!\n\n~Timothy Crosley\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Extends Hypothesis to add fully automatic testing of type annotated functions",
"version": "1.1.5",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "7bce70744537742b0a6fd155a9b1e6edcfd824a687c2234a1d78c09dd473acb1",
"md5": "9715b08370b7437091605e6c0f2c15a7",
"sha256": "7e962789a9ac691d4d9b6996f06f7afa1708013b67fd2df93a8857a92d6d7854"
},
"downloads": -1,
"filename": "hypothesis_auto-1.1.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "9715b08370b7437091605e6c0f2c15a7",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 7912,
"upload_time": "2023-01-19T05:22:14",
"upload_time_iso_8601": "2023-01-19T05:22:14.541058Z",
"url": "https://files.pythonhosted.org/packages/7b/ce/70744537742b0a6fd155a9b1e6edcfd824a687c2234a1d78c09dd473acb1/hypothesis_auto-1.1.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "149da491aaf55e61b4fee99508d8d2ede409c760ced3e0156d78bcbd34a8e4df",
"md5": "ed3688878c30ebc727cae3e668d8a00f",
"sha256": "534bdc381f635e6515e6fd88c326d5bb66ab6351693e72a43fb9b691b0f5911c"
},
"downloads": -1,
"filename": "hypothesis_auto-1.1.5.tar.gz",
"has_sig": false,
"md5_digest": "ed3688878c30ebc727cae3e668d8a00f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 9423,
"upload_time": "2023-01-19T05:22:16",
"upload_time_iso_8601": "2023-01-19T05:22:16.311743Z",
"url": "https://files.pythonhosted.org/packages/14/9d/a491aaf55e61b4fee99508d8d2ede409c760ced3e0156d78bcbd34a8e4df/hypothesis_auto-1.1.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-01-19 05:22:16",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "hypothesis-auto"
}