# ID Analyzer Python SDK
This is a python SDK library for [ID Analyzer Identity Verification APIs](https://www.idanalyzer.com), though all the APIs can be called with without the SDK using simple HTTP requests as outlined in the [documentation](https://id-analyzer-v2.readme.io), you can use this SDK to accelerate server-side development.
We strongly discourage users to connect to ID Analyzer API endpoint directly from client-side applications that will be distributed to end user, such as mobile app, or in-browser JavaScript. Your API key could be easily compromised, and if you are storing your customer's information inside Vault they could use your API key to fetch all your user details. Therefore, the best practice is always to implement a client side connection to your server, and call our APIs from the server-side.
## Installation
Install through PIP
```shell
pip install idanalyzer
```
## Scanner
This category supports all scanning-related functions specifically used to initiate a new identity document scan & ID face verification transaction by uploading based64-encoded images.
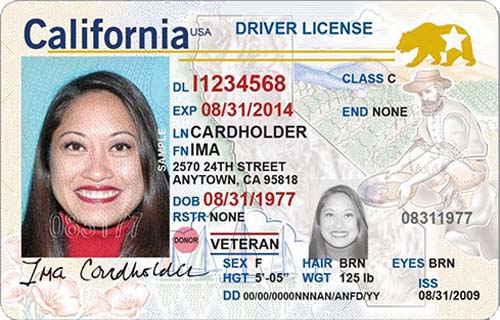
```python
from idanalyzer2 import *
import traceback
import json
try:
profile = Profile(Profile.SECURITY_MEDIUM)
s = Scanner('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')
s.throwApiException(True)
resp = s.quickScan('05.png', "", True)
with open('quickScan.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
s.setProfile(profile)
resp = s.scan("05.png")
with open('scan.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
except APIError as e:
print(traceback.format_exc())
print(e.args[0])
except InvalidArgumentException as e:
print(traceback.format_exc())
print(e.args[0])
except Exception as e:
print(traceback.format_exc())
print(e.args[0])
```
## Biometric
There are two primary functions within this class. The first one is verifyFace and the second is verifyLiveness.
```python
from idanalyzer2 import *
import traceback
import json
try:
profile = Profile(Profile.SECURITY_MEDIUM)
b = Biometric('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')
b.throwApiException(True)
b.setProfile(profile)
resp = b.verifyFace('05.png', '05.png')
with open('verifyFace.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = b.verifyLiveness('05.png', '05.png')
with open('verifyLiveness.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
except APIError as e:
print(traceback.format_exc())
print(e.args[0])
except InvalidArgumentException as e:
print(traceback.format_exc())
print(e.args[0])
except Exception as e:
print(traceback.format_exc())
print(e.args[0])
```
## Contract
All contract-related feature sets are available in Contract class. There are three primary functions in this class.
```python
from idanalyzer2 import *
import traceback
import json
try:
c = Contract('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')
c.throwApiException(True)
temp = c.createTemplate("tempName", "<p>%{fullName}</p>")
with open('createTemplate.json', 'w') as f:
f.write(json.dumps(temp, indent=4))
tempId = temp['templateId']
resp = c.updateTemplate(tempId, "oldTemp", "<p>%{fullName}</p><p>Hello!!</p>")
with open('updateTemplate.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = c.getTemplate(tempId)
with open('getTemplate.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = c.listTemplate()
with open('listTemplate.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = c.generate(tempId, "PDF", "", {
'fullName': "Tian",
})
with open('generate.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = c.deleteTemplate(tempId)
with open('deleteTemplate.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
except APIError as e:
print(traceback.format_exc())
print(e.args[0])
except InvalidArgumentException as e:
print(traceback.format_exc())
print(e.args[0])
except Exception as e:
print(traceback.format_exc())
print(e.args[0])
```
## Docupass
This category supports all rapid user verification based on the ids and the face images provided.
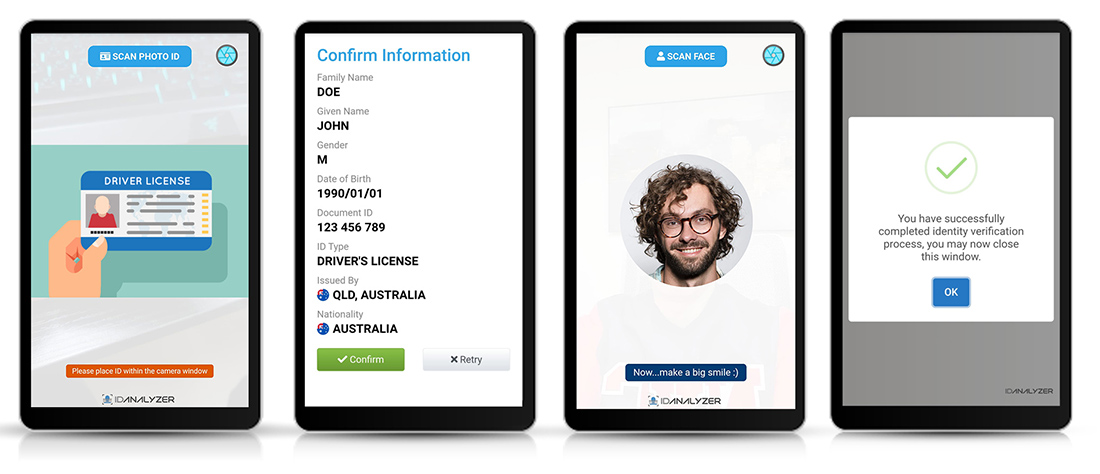
```python
from idanalyzer2 import *
import traceback
import json
try:
d = Docupass('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')
d.throwApiException(True)
doc = d.createDocupass("bbd8436953ef426e98d078953f258835")
with open('createDocupass.json', 'w') as outfile:
json.dump(doc, outfile)
resp = d.listDocupass()
with open('listDocupass.json', 'w') as outfile:
json.dump(resp, outfile)
resp = d.deleteDocupass(doc['reference'])
with open('deleteDocupass.json', 'w') as outfile:
json.dump(resp, outfile)
except APIError as e:
print(traceback.format_exc())
print(e.args[0])
except InvalidArgumentException as e:
print(traceback.format_exc())
print(e.args[0])
except Exception as e:
print(traceback.format_exc())
print(e.args[0])
```
## Transaction
This function enables the developer to retrieve a single transaction record based on the provided transactionId.
```python
from idanalyzer2 import *
import traceback
import json
try:
t = Transaction('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')
t.throwApiException(True)
tid = "da3124d09173474cabc86f3a648c9084"
resp = t.getTransaction(tid)
with open('getTransaction.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = t.listTransaction()
with open('listTransaction.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = t.updateTransaction(tid, "review")
with open('updateTransaction.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
resp = t.deleteTransaction(tid)
with open('deleteTransaction.json', 'w') as f:
f.write(json.dumps(resp, indent=4))
t.saveImage("fb2079b309c116b025408b2b79f90b9c196b02a27827ba98ea1ddc2af63f111c", "test.jpg")
t.saveFile("testsign_3smMjY66x4y7CVPNrbBRGyKoePrlW8oi.pdf", "test.pdf")
t.exportTransaction("./test.zip", [
"305e9fcb7b7a48dbab7c87d3a752b5e1",
], "json")
except APIError as e:
print(traceback.format_exc())
print(e.args[0])
except InvalidArgumentException as e:
print(traceback.format_exc())
print(e.args[0])
except Exception as e:
print(traceback.format_exc())
print(e.args[0])
```
## Api Document
[ID Analyzer Document](https://id-analyzer-v2.readme.io/docs/python)
## Demo
Check out **/demo** folder for more Python demos.
## SDK Reference
Check out [ID Analyzer Python Reference](https://idanalyzer.github.io/id-analyzer-nodejs/)
Raw data
{
"_id": null,
"home_page": "https://www.idanalyzer.com",
"name": "idanalyzer2",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "id card,driver license,passport,id verification,identification card,identity document,mrz,pdf417,aamva,aml,pep,sign document",
"author": "ID Analyzer",
"author_email": "<support@idanalyzer.com>",
"download_url": "https://files.pythonhosted.org/packages/ac/d5/f27af05b2b04591a2308736dc1a332f2ee3363488b28daa2bf69eaf27515/idanalyzer2-1.0.2.tar.gz",
"platform": null,
"description": "\r\n# ID Analyzer Python SDK\r\nThis is a python SDK library for [ID Analyzer Identity Verification APIs](https://www.idanalyzer.com), though all the APIs can be called with without the SDK using simple HTTP requests as outlined in the [documentation](https://id-analyzer-v2.readme.io), you can use this SDK to accelerate server-side development.\r\n\r\nWe strongly discourage users to connect to ID Analyzer API endpoint directly from client-side applications that will be distributed to end user, such as mobile app, or in-browser JavaScript. Your API key could be easily compromised, and if you are storing your customer's information inside Vault they could use your API key to fetch all your user details. Therefore, the best practice is always to implement a client side connection to your server, and call our APIs from the server-side.\r\n\r\n## Installation\r\nInstall through PIP\r\n\r\n```shell\r\npip install idanalyzer\r\n```\r\n\r\n## Scanner\r\nThis category supports all scanning-related functions specifically used to initiate a new identity document scan & ID face verification transaction by uploading based64-encoded images.\r\n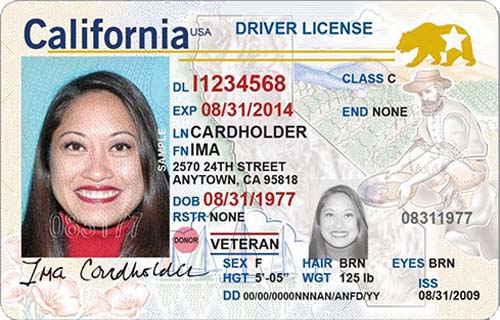\r\n```python\r\nfrom idanalyzer2 import *\r\nimport traceback\r\nimport json\r\n\r\n\r\ntry:\r\n profile = Profile(Profile.SECURITY_MEDIUM)\r\n s = Scanner('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')\r\n s.throwApiException(True)\r\n resp = s.quickScan('05.png', \"\", True)\r\n with open('quickScan.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n s.setProfile(profile)\r\n resp = s.scan(\"05.png\")\r\n with open('scan.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\nexcept APIError as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept InvalidArgumentException as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept Exception as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\n\r\n\r\n```\r\n\r\n## Biometric\r\nThere are two primary functions within this class. The first one is verifyFace and the second is verifyLiveness.\r\n```python\r\nfrom idanalyzer2 import *\r\nimport traceback\r\nimport json\r\n\r\ntry:\r\n profile = Profile(Profile.SECURITY_MEDIUM)\r\n b = Biometric('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')\r\n b.throwApiException(True)\r\n b.setProfile(profile)\r\n resp = b.verifyFace('05.png', '05.png')\r\n with open('verifyFace.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = b.verifyLiveness('05.png', '05.png')\r\n with open('verifyLiveness.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\nexcept APIError as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept InvalidArgumentException as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept Exception as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\n```\r\n\r\n## Contract\r\nAll contract-related feature sets are available in Contract class. There are three primary functions in this class.\r\n```python\r\nfrom idanalyzer2 import *\r\nimport traceback\r\nimport json\r\n\r\ntry:\r\n c = Contract('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')\r\n c.throwApiException(True)\r\n temp = c.createTemplate(\"tempName\", \"<p>%{fullName}</p>\")\r\n with open('createTemplate.json', 'w') as f:\r\n f.write(json.dumps(temp, indent=4))\r\n tempId = temp['templateId']\r\n resp = c.updateTemplate(tempId, \"oldTemp\", \"<p>%{fullName}</p><p>Hello!!</p>\")\r\n with open('updateTemplate.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = c.getTemplate(tempId)\r\n with open('getTemplate.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = c.listTemplate()\r\n with open('listTemplate.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = c.generate(tempId, \"PDF\", \"\", {\r\n 'fullName': \"Tian\",\r\n })\r\n with open('generate.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = c.deleteTemplate(tempId)\r\n with open('deleteTemplate.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\nexcept APIError as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept InvalidArgumentException as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept Exception as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\n\r\n```\r\n\r\n## Docupass\r\nThis category supports all rapid user verification based on the ids and the face images provided.\r\n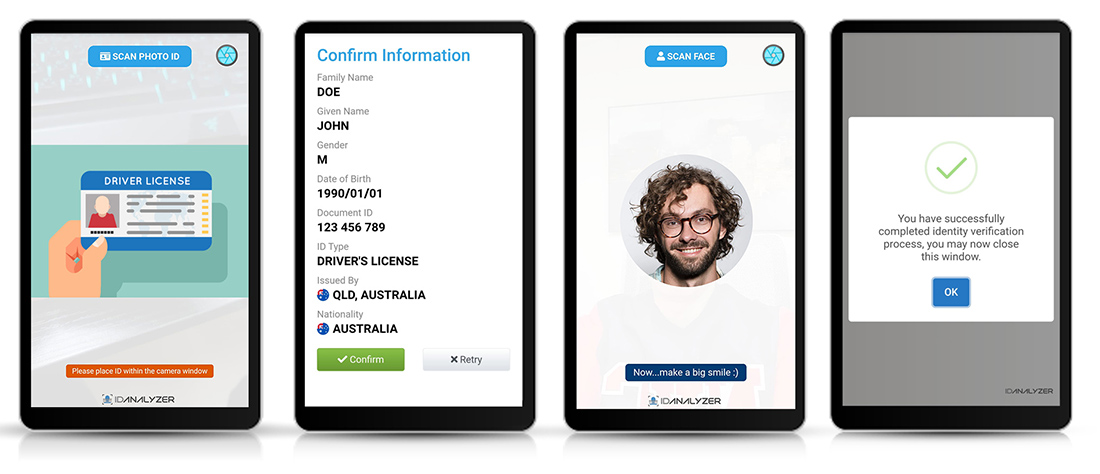\r\n```python\r\nfrom idanalyzer2 import *\r\nimport traceback\r\nimport json\r\n\r\ntry:\r\n d = Docupass('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')\r\n d.throwApiException(True)\r\n doc = d.createDocupass(\"bbd8436953ef426e98d078953f258835\")\r\n with open('createDocupass.json', 'w') as outfile:\r\n json.dump(doc, outfile)\r\n resp = d.listDocupass()\r\n with open('listDocupass.json', 'w') as outfile:\r\n json.dump(resp, outfile)\r\n resp = d.deleteDocupass(doc['reference'])\r\n with open('deleteDocupass.json', 'w') as outfile:\r\n json.dump(resp, outfile)\r\nexcept APIError as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept InvalidArgumentException as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept Exception as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\n\r\n```\r\n\r\n## Transaction\r\nThis function enables the developer to retrieve a single transaction record based on the provided transactionId.\r\n```python\r\nfrom idanalyzer2 import *\r\nimport traceback\r\nimport json\r\n\r\ntry:\r\n t = Transaction('CBoQpSfkRcPvUhstucIPfiGNLPVuwB23')\r\n t.throwApiException(True)\r\n tid = \"da3124d09173474cabc86f3a648c9084\"\r\n resp = t.getTransaction(tid)\r\n with open('getTransaction.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = t.listTransaction()\r\n with open('listTransaction.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = t.updateTransaction(tid, \"review\")\r\n with open('updateTransaction.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n resp = t.deleteTransaction(tid)\r\n with open('deleteTransaction.json', 'w') as f:\r\n f.write(json.dumps(resp, indent=4))\r\n\r\n t.saveImage(\"fb2079b309c116b025408b2b79f90b9c196b02a27827ba98ea1ddc2af63f111c\", \"test.jpg\")\r\n t.saveFile(\"testsign_3smMjY66x4y7CVPNrbBRGyKoePrlW8oi.pdf\", \"test.pdf\")\r\n t.exportTransaction(\"./test.zip\", [\r\n \"305e9fcb7b7a48dbab7c87d3a752b5e1\",\r\n ], \"json\")\r\n\r\nexcept APIError as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept InvalidArgumentException as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\nexcept Exception as e:\r\n print(traceback.format_exc())\r\n print(e.args[0])\r\n\r\n```\r\n\r\n## Api Document\r\n[ID Analyzer Document](https://id-analyzer-v2.readme.io/docs/python)\r\n\r\n## Demo\r\nCheck out **/demo** folder for more Python demos.\r\n\r\n## SDK Reference\r\nCheck out [ID Analyzer Python Reference](https://idanalyzer.github.io/id-analyzer-nodejs/)\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "ID Analyzer API V2 client library, scan and verify global passport, driver license and identification card.",
"version": "1.0.2",
"project_urls": {
"Homepage": "https://www.idanalyzer.com"
},
"split_keywords": [
"id card",
"driver license",
"passport",
"id verification",
"identification card",
"identity document",
"mrz",
"pdf417",
"aamva",
"aml",
"pep",
"sign document"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fa4265fe3bd84cb013f3bba8ff8c60cf5807759d85f0bc92a859b1f135f3bad6",
"md5": "9bd0b88097c0e762960a7c5863048163",
"sha256": "af36c34771cd360e55593875922019afe827ae012c9be94576e8583d58678659"
},
"downloads": -1,
"filename": "idanalyzer2-1.0.2-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "9bd0b88097c0e762960a7c5863048163",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": null,
"size": 13152,
"upload_time": "2023-12-05T09:22:48",
"upload_time_iso_8601": "2023-12-05T09:22:48.425691Z",
"url": "https://files.pythonhosted.org/packages/fa/42/65fe3bd84cb013f3bba8ff8c60cf5807759d85f0bc92a859b1f135f3bad6/idanalyzer2-1.0.2-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "acd5f27af05b2b04591a2308736dc1a332f2ee3363488b28daa2bf69eaf27515",
"md5": "4e5f83c46b7a67b204da8b0f6445c31a",
"sha256": "4c7cc868e48054df45e59708e10f9c0da665e1447f3bfddef2db1d08788a94eb"
},
"downloads": -1,
"filename": "idanalyzer2-1.0.2.tar.gz",
"has_sig": false,
"md5_digest": "4e5f83c46b7a67b204da8b0f6445c31a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 14515,
"upload_time": "2023-12-05T09:22:50",
"upload_time_iso_8601": "2023-12-05T09:22:50.464511Z",
"url": "https://files.pythonhosted.org/packages/ac/d5/f27af05b2b04591a2308736dc1a332f2ee3363488b28daa2bf69eaf27515/idanalyzer2-1.0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-05 09:22:50",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "idanalyzer2"
}