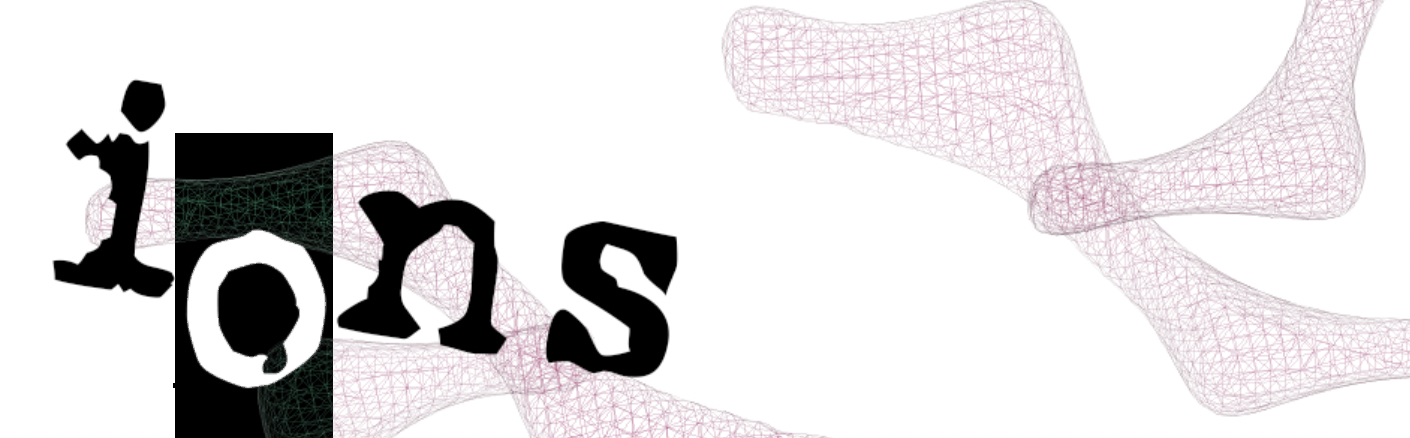
### About
ions is a python library made for studying crystalline ionic conductors
### Installation
```bash
pip install ions
```
or
```bash
git clone https://github.com/dembart/ions
cd ions
pip install .
```
### Functionality examples:
- [Percolation radius and dimensionality](#percolation-radius-and-dimensionality)
- [Inequivalent ionic hops forming percolating network](#inequivalent-ionic-hops-forming-percolating-network)
- [Nudged elastic band (NEB) calculation of a mobile ion activation barrier employing bond valence force field calculator and Atomic Simulation Environment optimizers](#nudged-elastic-band-calculations)
- [Compare NEB with bond valence energy landscape (BVEL) method](#compare-with-bvse-meshgrid-approach-ie-empty-lattice)
- [Decorate Atoms with oxidation states](#how-to-decorate-ases-atoms)
- [Available data](#available-data)
Note:
The library is under active development. Errors are expected. Most of the features are not well documented for now.
#### Percolation radius and dimensionality
```python
from ase.io import read, write
from ions.tools import Percolator
file = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'
atoms = read(file)
specie = 3
pr = Percolator(
atoms,
specie, # atomic number
10.0, # upper bound for Li-Li hops search
)
tr = 0.5 # Minimum allowed distance between the Li-Li edge and the framework
cutoff, dim = pr.mincut_maxdim(tr)
print(f'Maximum percolation dimensionality: {dim}')
print(f'Jump distance cutoff: {cutoff} angstrom', '\n')
for i in range(1, 4):
percolation_radius = pr.percolation_threshold(i)
print(f'{i}D percolation radius: {round(percolation_radius, 2)} angstrom')
```
Maximum percolation dimensionality: 3
Jump distance cutoff: 5.7421875 angstrom
1D percolation radius: 1.58 angstrom
2D percolation radius: 1.46 angstrom
3D percolation radius: 0.97 angstrom
#### Inequivalent ionic hops forming percolating network
```python
edges, _ = pr.unique_edges(cutoff, tr) # list of (source, target, offset_x, offset_y, offset_z)
edges
```
[Edge(0,1,[0 0 0], d=5.74, wrapped_target=1, info = {'multiplicity': 36, 'index': 0}'),
Edge(0,3,[1 0 0], d=5.64, wrapped_target=3, info = {'multiplicity': 24, 'index': 4}'),
Edge(0,3,[0 0 0], d=3.05, wrapped_target=3, info = {'multiplicity': 24, 'index': 1}'),
Edge(0,0,[1 0 0], d=4.75, wrapped_target=0, info = {'multiplicity': 16, 'index': 3}')]
#### Nudged elastic band calculations
```python
from ase.io import read
from ions import Decorator
from ase.optimize import FIRE
from ions.tools import Percolator, SaddleFinder
from ions.utils import collect_bvse_params
def optimize(neb, fmax = 0.1, steps = 100, logfile = 'log'):
images = neb.images
# relax source
optim = FIRE(images[0], logfile = logfile)
optim.run(fmax = fmax, steps = steps)
# relax target
optim = FIRE(images[-1], logfile = logfile)
optim.run(fmax = fmax, steps = steps)
# relax band
optim = FIRE(neb, logfile = logfile)
optim.run(fmax = fmax, steps = steps)
# we perturb the structure before the calculations
if 'perturbation' in images[0].info.keys():
for image in images:
image.positions -= image.info['perturbation']
image.info['perturbation'] = 0.0 * image.info['perturbation']
optim = FIRE(neb, logfile = logfile)
optim.run(fmax = fmax, steps = steps)
```
```python
file = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'
atoms = read(file)
Decorator().decorate(atoms)
collect_bvse_params(atoms, 'Li', 1, self_interaction=True)
pl = Percolator(atoms, 3, 10.0)
tr = 0.5
cutoff, dim = pl.mincut_maxdim(tr = tr)
edges, _ = pl.unique_edges(cutoff, tr = 0.5)
relaxed_images = []
for edge in edges:
images = edge.superedge(8.0).interpolate(spacing = .75) # we create a supercell with 8.0 Angstrom size
sf = SaddleFinder()
neb = sf.bvse_neb(images, distort = True, gm = False)
optimize(neb)
barrier = sf.get_barrier(images)
relaxed_images.append(images)
print(edge)
print(f'Ea: {round(barrier, 2)} eV', '\n')
```
Edge(0,1,[0 0 0], d=5.74, wrapped_target=1, info = {'multiplicity': 36, 'index': 0}')
Ea: 3.27 eV
Edge(0,3,[1 0 0], d=5.64, wrapped_target=3, info = {'multiplicity': 24, 'index': 4}')
Ea: 3.56 eV
Edge(0,3,[0 0 0], d=3.05, wrapped_target=3, info = {'multiplicity': 24, 'index': 1}')
Ea: 0.33 eV
Edge(0,0,[1 0 0], d=4.75, wrapped_target=0, info = {'multiplicity': 16, 'index': 3}')
Ea: 3.3 eV
#### Compare with BVSE meshgrid approach (i.e. empty lattice)
- For more details see [BVlain](https://github.com/dembart/BVlain) library
```python
from bvlain import Lain
calc = Lain(verbose = False)
atoms = calc.read_file(file)
_ = calc.bvse_distribution(mobile_ion = 'Li1+') # Li-Li interaction is omitted
calc.percolation_barriers()
```
{'E_1D': 0.4395, 'E_2D': 3.3301, 'E_3D': 3.3594}
### How to decorate ase's Atoms
```python
import numpy as np
from ase.io import read
from ions import Decorator
file = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'
atoms = read(file)
calc = Decorator()
atoms = calc.decorate(atoms)
oxi_states = atoms.get_array('oxi_states')
np.unique(list(zip(atoms.symbols, oxi_states)), axis = 0)
```
array([['Fe', '2'],
['Li', '1'],
['O', '-2'],
['P', '5']], dtype='<U21')
#### Available data
- bv_data - bond valence parameters [1]
- bvse_data - bond valence site energy parameters[2]
- ionic_radii - Shannon ionic radii [3, 4]
- crystal_radii - Shannon crystal radii [3, 4]
- elneg_pauling - Pauling's elenctronegativities [5]
[1]. https://www.iucr.org/resources/data/datasets/bond-valence-parameters (bvparam2020.cif)
[2]. He, B., Chi, S., Ye, A. et al. High-throughput screening platform for solid electrolytes combining hierarchical ion-transport prediction algorithms. Sci Data 7, 151 (2020). https://doi.org/10.1038/s41597-020-0474-y
[3] http://abulafia.mt.ic.ac.uk/shannon/ptable.php
[4] https://github.com/prtkm/ionic-radii
[5] https://mendeleev.readthedocs.io/en/stable/
```python
from ions.data import ionic_radii, crystal_radii, bv_data, bvse_data
#ionic radius
symbol, valence = 'V', 4
r_ionic = ionic_radii[symbol][valence]
#crystal radius
symbol, valence = 'F', -1
r_crystal = crystal_radii[symbol][valence]
# bond valence parameters
source, source_valence = 'Li', 1
target, target_valence = 'O', -2
params = bv_data[source][source_valence][target][target_valence]
r0, b = params['r0'], params['b']
# bond valence site energy parameters
source, source_valence = 'Li', 1
target, target_valence = 'O', -2
params = bvse_data[source][source_valence][target][target_valence]
r0, r_min, alpha, d0 = params['r0'], params['r_min'], params['alpha'], params['d0']
```
### Bond valence sum calculation
```python
import numpy as np
from ions import Decorator
from ase.io import read
from ase.neighborlist import neighbor_list
from ions.data import bv_data
file = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'
atoms = read(file)
calc = Decorator()
atoms = calc.decorate(atoms)
ii, jj, dd = neighbor_list('ijd', atoms, 5.0)
symbols = atoms.symbols
valences = atoms.get_array('oxi_states')
for i in np.unique(ii):
source = symbols[i]
source_valence = valences[i]
neighbors = jj[ii == i]
distances = dd[ii == i]
if source_valence > 0:
bvs = 0
for n, d in zip(neighbors, distances):
target = symbols[n]
target_valence = valences[n]
if source_valence * target_valence < 0:
params = bv_data[source][source_valence][target][target_valence]
r0, b = params['r0'], params['b']
bvs += np.exp((r0 - d) / b)
print(f'Bond valence sum for {source} is {round(bvs, 4)}')
```
Bond valence sum for Li is 1.0775
Bond valence sum for Li is 1.0775
Bond valence sum for Li is 1.0775
Bond valence sum for Li is 1.0775
Bond valence sum for Fe is 1.8394
Bond valence sum for Fe is 1.8394
Bond valence sum for Fe is 1.8394
Bond valence sum for Fe is 1.8394
Bond valence sum for P is 4.6745
Bond valence sum for P is 4.6745
Bond valence sum for P is 4.6745
Bond valence sum for P is 4.6745
Raw data
{
"_id": null,
"home_page": "https://github.com/dembart/ions",
"name": "ions",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "Li-ion, barrier, activation, percolation, ion, ions, crystal, bvse, bvel, neb",
"author": "Artem Dembitskiy",
"author_email": "art.dembitskiy@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/58/e8/dd329d2ffc05c22ad7c5e38b6f372a9251be9afd7d4a5984e424cffe5ff2/ions-0.4.tar.gz",
"platform": null,
"description": "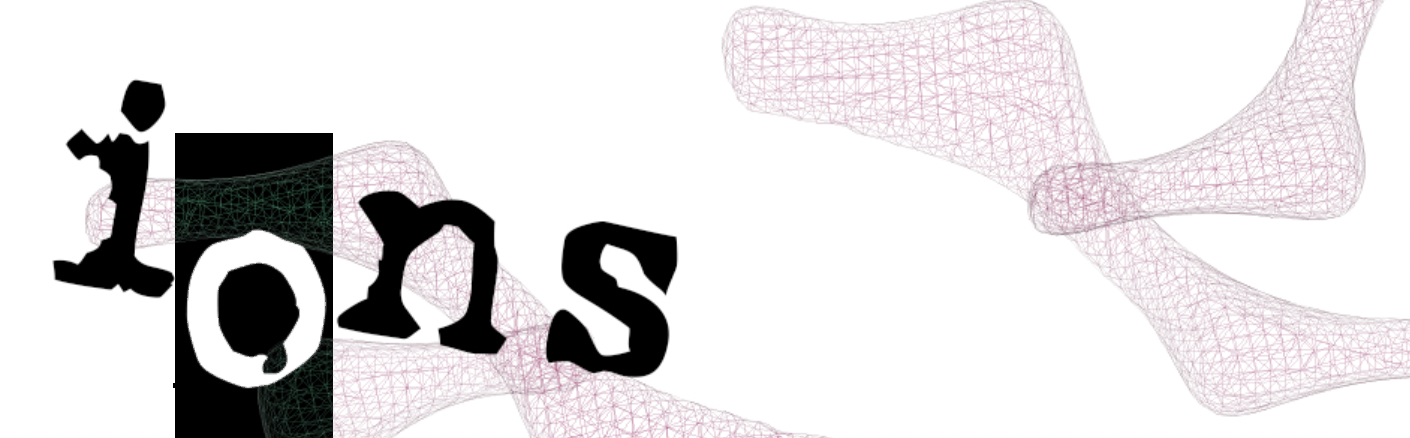\n\n\n### About\n\nions is a python library made for studying crystalline ionic conductors\n\n### Installation\n\n```bash\npip install ions\n```\n\nor \n\n```bash\ngit clone https://github.com/dembart/ions\ncd ions\npip install .\n```\n\n### Functionality examples:\n\n- [Percolation radius and dimensionality](#percolation-radius-and-dimensionality)\n\n- [Inequivalent ionic hops forming percolating network](#inequivalent-ionic-hops-forming-percolating-network)\n\n- [Nudged elastic band (NEB) calculation of a mobile ion activation barrier employing bond valence force field calculator and Atomic Simulation Environment optimizers](#nudged-elastic-band-calculations)\n- [Compare NEB with bond valence energy landscape (BVEL) method](#compare-with-bvse-meshgrid-approach-ie-empty-lattice)\n\n- [Decorate Atoms with oxidation states](#how-to-decorate-ases-atoms)\n\n- [Available data](#available-data)\n\n\nNote:\nThe library is under active development. Errors are expected. Most of the features are not well documented for now.\n\n\n#### Percolation radius and dimensionality\n\n\n```python\nfrom ase.io import read, write\nfrom ions.tools import Percolator\n\nfile = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'\natoms = read(file) \n\nspecie = 3 \npr = Percolator(\n atoms, \n specie, # atomic number\n 10.0, # upper bound for Li-Li hops search \n )\n\ntr = 0.5 # Minimum allowed distance between the Li-Li edge and the framework\ncutoff, dim = pr.mincut_maxdim(tr)\n\nprint(f'Maximum percolation dimensionality: {dim}')\nprint(f'Jump distance cutoff: {cutoff} angstrom', '\\n')\n\nfor i in range(1, 4):\n percolation_radius = pr.percolation_threshold(i)\n print(f'{i}D percolation radius: {round(percolation_radius, 2)} angstrom')\n```\n\n Maximum percolation dimensionality: 3\n Jump distance cutoff: 5.7421875 angstrom \n \n 1D percolation radius: 1.58 angstrom\n 2D percolation radius: 1.46 angstrom\n 3D percolation radius: 0.97 angstrom\n\n\n#### Inequivalent ionic hops forming percolating network\n\n\n```python\nedges, _ = pr.unique_edges(cutoff, tr) # list of (source, target, offset_x, offset_y, offset_z)\nedges\n```\n\n\n\n\n [Edge(0,1,[0 0 0], d=5.74, wrapped_target=1, info = {'multiplicity': 36, 'index': 0}'),\n Edge(0,3,[1 0 0], d=5.64, wrapped_target=3, info = {'multiplicity': 24, 'index': 4}'),\n Edge(0,3,[0 0 0], d=3.05, wrapped_target=3, info = {'multiplicity': 24, 'index': 1}'),\n Edge(0,0,[1 0 0], d=4.75, wrapped_target=0, info = {'multiplicity': 16, 'index': 3}')]\n\n\n\n#### Nudged elastic band calculations\n\n\n```python\nfrom ase.io import read\nfrom ions import Decorator\nfrom ase.optimize import FIRE\nfrom ions.tools import Percolator, SaddleFinder\nfrom ions.utils import collect_bvse_params\n\n\ndef optimize(neb, fmax = 0.1, steps = 100, logfile = 'log'):\n images = neb.images\n \n # relax source\n optim = FIRE(images[0], logfile = logfile)\n optim.run(fmax = fmax, steps = steps)\n\n # relax target\n optim = FIRE(images[-1], logfile = logfile)\n optim.run(fmax = fmax, steps = steps)\n \n # relax band\n optim = FIRE(neb, logfile = logfile)\n optim.run(fmax = fmax, steps = steps)\n \n # we perturb the structure before the calculations \n if 'perturbation' in images[0].info.keys():\n for image in images:\n image.positions -= image.info['perturbation']\n image.info['perturbation'] = 0.0 * image.info['perturbation'] \n\n optim = FIRE(neb, logfile = logfile)\n optim.run(fmax = fmax, steps = steps)\n```\n\n\n```python\nfile = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'\natoms = read(file)\nDecorator().decorate(atoms)\ncollect_bvse_params(atoms, 'Li', 1, self_interaction=True)\npl = Percolator(atoms, 3, 10.0)\ntr = 0.5\ncutoff, dim = pl.mincut_maxdim(tr = tr)\nedges, _ = pl.unique_edges(cutoff, tr = 0.5)\n\nrelaxed_images = []\nfor edge in edges:\n images = edge.superedge(8.0).interpolate(spacing = .75) # we create a supercell with 8.0 Angstrom size\n sf = SaddleFinder()\n neb = sf.bvse_neb(images, distort = True, gm = False)\n optimize(neb)\n barrier = sf.get_barrier(images)\n relaxed_images.append(images)\n print(edge)\n print(f'Ea: {round(barrier, 2)} eV', '\\n')\n```\n\n Edge(0,1,[0 0 0], d=5.74, wrapped_target=1, info = {'multiplicity': 36, 'index': 0}')\n Ea: 3.27 eV \n \n Edge(0,3,[1 0 0], d=5.64, wrapped_target=3, info = {'multiplicity': 24, 'index': 4}')\n Ea: 3.56 eV \n \n Edge(0,3,[0 0 0], d=3.05, wrapped_target=3, info = {'multiplicity': 24, 'index': 1}')\n Ea: 0.33 eV \n \n Edge(0,0,[1 0 0], d=4.75, wrapped_target=0, info = {'multiplicity': 16, 'index': 3}')\n Ea: 3.3 eV \n \n\n\n#### Compare with BVSE meshgrid approach (i.e. empty lattice)\n - For more details see [BVlain](https://github.com/dembart/BVlain) library\n\n\n```python\nfrom bvlain import Lain\n\ncalc = Lain(verbose = False)\natoms = calc.read_file(file)\n_ = calc.bvse_distribution(mobile_ion = 'Li1+') # Li-Li interaction is omitted\ncalc.percolation_barriers()\n```\n\n\n\n\n {'E_1D': 0.4395, 'E_2D': 3.3301, 'E_3D': 3.3594}\n\n\n\n### How to decorate ase's Atoms\n\n\n```python\nimport numpy as np\nfrom ase.io import read\nfrom ions import Decorator\n\n\nfile = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'\natoms = read(file)\ncalc = Decorator()\natoms = calc.decorate(atoms)\noxi_states = atoms.get_array('oxi_states')\nnp.unique(list(zip(atoms.symbols, oxi_states)), axis = 0)\n\n```\n\n\n\n\n array([['Fe', '2'],\n ['Li', '1'],\n ['O', '-2'],\n ['P', '5']], dtype='<U21')\n\n\n\n#### Available data\n\n- bv_data - bond valence parameters [1]\n\n- bvse_data - bond valence site energy parameters[2]\n\n- ionic_radii - Shannon ionic radii [3, 4]\n\n- crystal_radii - Shannon crystal radii [3, 4]\n\n- elneg_pauling - Pauling's elenctronegativities [5]\n\n\n\n[1]. https://www.iucr.org/resources/data/datasets/bond-valence-parameters (bvparam2020.cif)\n\n[2]. He, B., Chi, S., Ye, A. et al. High-throughput screening platform for solid electrolytes combining hierarchical ion-transport prediction algorithms. Sci Data 7, 151 (2020). https://doi.org/10.1038/s41597-020-0474-y\n\n[3] http://abulafia.mt.ic.ac.uk/shannon/ptable.php\n\n[4] https://github.com/prtkm/ionic-radii\n\n[5] https://mendeleev.readthedocs.io/en/stable/\n\n\n\n\n```python\nfrom ions.data import ionic_radii, crystal_radii, bv_data, bvse_data\n\n#ionic radius\nsymbol, valence = 'V', 4\nr_ionic = ionic_radii[symbol][valence] \n\n\n#crystal radius\nsymbol, valence = 'F', -1\nr_crystal = crystal_radii[symbol][valence]\n\n\n# bond valence parameters\nsource, source_valence = 'Li', 1\ntarget, target_valence = 'O', -2\nparams = bv_data[source][source_valence][target][target_valence]\nr0, b = params['r0'], params['b']\n\n\n# bond valence site energy parameters\nsource, source_valence = 'Li', 1\ntarget, target_valence = 'O', -2\nparams = bvse_data[source][source_valence][target][target_valence]\nr0, r_min, alpha, d0 = params['r0'], params['r_min'], params['alpha'], params['d0']\n```\n\n### Bond valence sum calculation\n\n\n```python\nimport numpy as np\nfrom ions import Decorator\nfrom ase.io import read\nfrom ase.neighborlist import neighbor_list\nfrom ions.data import bv_data\n\nfile = '/Users/artemdembitskiy/Downloads/LiFePO4.cif'\natoms = read(file)\ncalc = Decorator()\natoms = calc.decorate(atoms)\nii, jj, dd = neighbor_list('ijd', atoms, 5.0) \n\nsymbols = atoms.symbols\nvalences = atoms.get_array('oxi_states')\nfor i in np.unique(ii):\n source = symbols[i]\n source_valence = valences[i]\n neighbors = jj[ii == i]\n distances = dd[ii == i]\n if source_valence > 0:\n bvs = 0\n for n, d in zip(neighbors, distances):\n target = symbols[n]\n target_valence = valences[n]\n if source_valence * target_valence < 0:\n params = bv_data[source][source_valence][target][target_valence]\n r0, b = params['r0'], params['b']\n bvs += np.exp((r0 - d) / b)\n print(f'Bond valence sum for {source} is {round(bvs, 4)}')\n\n```\n\n Bond valence sum for Li is 1.0775\n Bond valence sum for Li is 1.0775\n Bond valence sum for Li is 1.0775\n Bond valence sum for Li is 1.0775\n Bond valence sum for Fe is 1.8394\n Bond valence sum for Fe is 1.8394\n Bond valence sum for Fe is 1.8394\n Bond valence sum for Fe is 1.8394\n Bond valence sum for P is 4.6745\n Bond valence sum for P is 4.6745\n Bond valence sum for P is 4.6745\n Bond valence sum for P is 4.6745\n\n\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A python library for studying ionic conductors",
"version": "0.4",
"project_urls": {
"Homepage": "https://github.com/dembart/ions"
},
"split_keywords": [
"li-ion",
" barrier",
" activation",
" percolation",
" ion",
" ions",
" crystal",
" bvse",
" bvel",
" neb"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fe3c90c14a4d12ccbcf2d62799af88af909badb4e2c11d88297bc12bbcc5794c",
"md5": "e03ce0f12509401eed05577a8d37f920",
"sha256": "5631af81313479d26ae2843687251eb327fc6866bceabb0ec654ca72f6f64d11"
},
"downloads": -1,
"filename": "ions-0.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e03ce0f12509401eed05577a8d37f920",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 142032,
"upload_time": "2024-08-29T09:03:48",
"upload_time_iso_8601": "2024-08-29T09:03:48.571051Z",
"url": "https://files.pythonhosted.org/packages/fe/3c/90c14a4d12ccbcf2d62799af88af909badb4e2c11d88297bc12bbcc5794c/ions-0.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "58e8dd329d2ffc05c22ad7c5e38b6f372a9251be9afd7d4a5984e424cffe5ff2",
"md5": "d5170cd9224e236c59cd7a0b1464e775",
"sha256": "092803d5e673eea95b74d19fe560c08cd21edc94e46830e240743fae27299ec1"
},
"downloads": -1,
"filename": "ions-0.4.tar.gz",
"has_sig": false,
"md5_digest": "d5170cd9224e236c59cd7a0b1464e775",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 140440,
"upload_time": "2024-08-29T09:03:50",
"upload_time_iso_8601": "2024-08-29T09:03:50.568520Z",
"url": "https://files.pythonhosted.org/packages/58/e8/dd329d2ffc05c22ad7c5e38b6f372a9251be9afd7d4a5984e424cffe5ff2/ions-0.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-29 09:03:50",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "dembart",
"github_project": "ions",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ions"
}