# ITKColorNormalization
[](https://github.com/InsightSoftwareConsortium/ITKColorNormalization/blob/master/LICENSE) [](https://zenodo.org/badge/latestdoi/263648073) [](https://github.com/InsightSoftwareConsortium/ITKColorNormalization/actions?query=workflow%3A%22Build%2C+test%2C+package%22)
## Overview
This [Insight Toolkit (ITK)](https://itk.org/) module performs Structure Preserving Color Normalization on an [H & E](https://en.wikipedia.org/wiki/H%26E_stain) image using a reference image. The module is in C++ and is also packaged for [Python](https://www.python.org/).
H & E ([hematoxylin](https://en.wikipedia.org/wiki/Haematoxylin) and [eosin](https://en.wikipedia.org/wiki/Eosin)) are stains used to color parts of cells in a histological image, often for medical diagnosis. Hematoxylin is a compound that stains cell nuclei a purple-blue color. Eosin is a compound that stains extracellular matrix and cytoplasm pink. However, the exact color of purple-blue or pink can vary from image to image, and this can make comparison of images difficult. This routine addresses the issue by re-coloring one image (the first image supplied to the routine) using the color scheme of a reference image (the second image supplied to the routine). The technique requires that the images have at least 3 colors, such as red, green, and blue (RGB).
Structure Preserving Color Normalization is a technique described in [Vahadane et al., 2016](https://doi.org/10.1109/TMI.2016.2529665) and modified in [Ramakrishnan et al., 2019](https://arxiv.org/abs/1901.03088). The idea is to model the color of an image pixel as something close to pure white, which is reduced in intensity in a color-specific way via an optical absorption model that depends upon the amounts of hematoxylin and eosin that are present. [Non-negative matrix factorization](https://en.wikipedia.org/wiki/Non-negative_matrix_factorization) is used on each analyzed image to simultaneously derive the amount of hematoxylin and eosin stain at each pixel and the image-wide effective colors of each stain.
The implementation in ITK accelerates the non-negative matrix factorization by choosing the initial estimate for the color absorption characteristics using a technique mimicking that presented in [Arora et al., 2013](https://proceedings.mlr.press/v28/arora13.html) and modified in [Newberg et al., 2018](https://doi.org/10.1371/journal.pone.0193067). This approach finds a good solution for a non-negative matrix factorization by first transforming it to the problem of finding a convex hull for a set of points in a cloud.
The lead developer of InsightSoftwareConsortium/ITKColorNormalization is [Lee Newberg](https://github.com/Leengit/).
## Try it online
We have set up a demonstration of this using [Binder](www.mybinder.org) that anyone can try. Click here: [](https://mybinder.org/v2/gh/InsightSoftwareConsortium/ITKColorNormalization/master?filepath=examples%2FITKColorNormalization.ipynb)
## Installation for Python
[](https://pypi.python.org/pypi/itk-spcn)
ITKColorNormalization and all its dependencies can be easily installed with [Python wheels](https://blog.kitware.com/itk-is-on-pypi-pip-install-itk-is-here/). Wheels have been generated for macOS, Linux, and Windows and several versions of Python, 3.6, 3.7, 3.8, and 3.9. If you do not want the installation to be to your current Python environment, you should first create and activate a [Python virtual environment (venv)](https://docs.python.org/3/tutorial/venv.html) to work in. Then, run the following from the command-line:
```shell-script
pip install itk-spcn
```
Launch `python`, import the itk package, and set variable names for the input images
```python
import itk
input_image_filename = "path/to/image_to_be_normalized"
reference_image_filename = "path/to/image_to_be_used_as_color_reference"
```
## Usage in Python
The following example transforms this input image
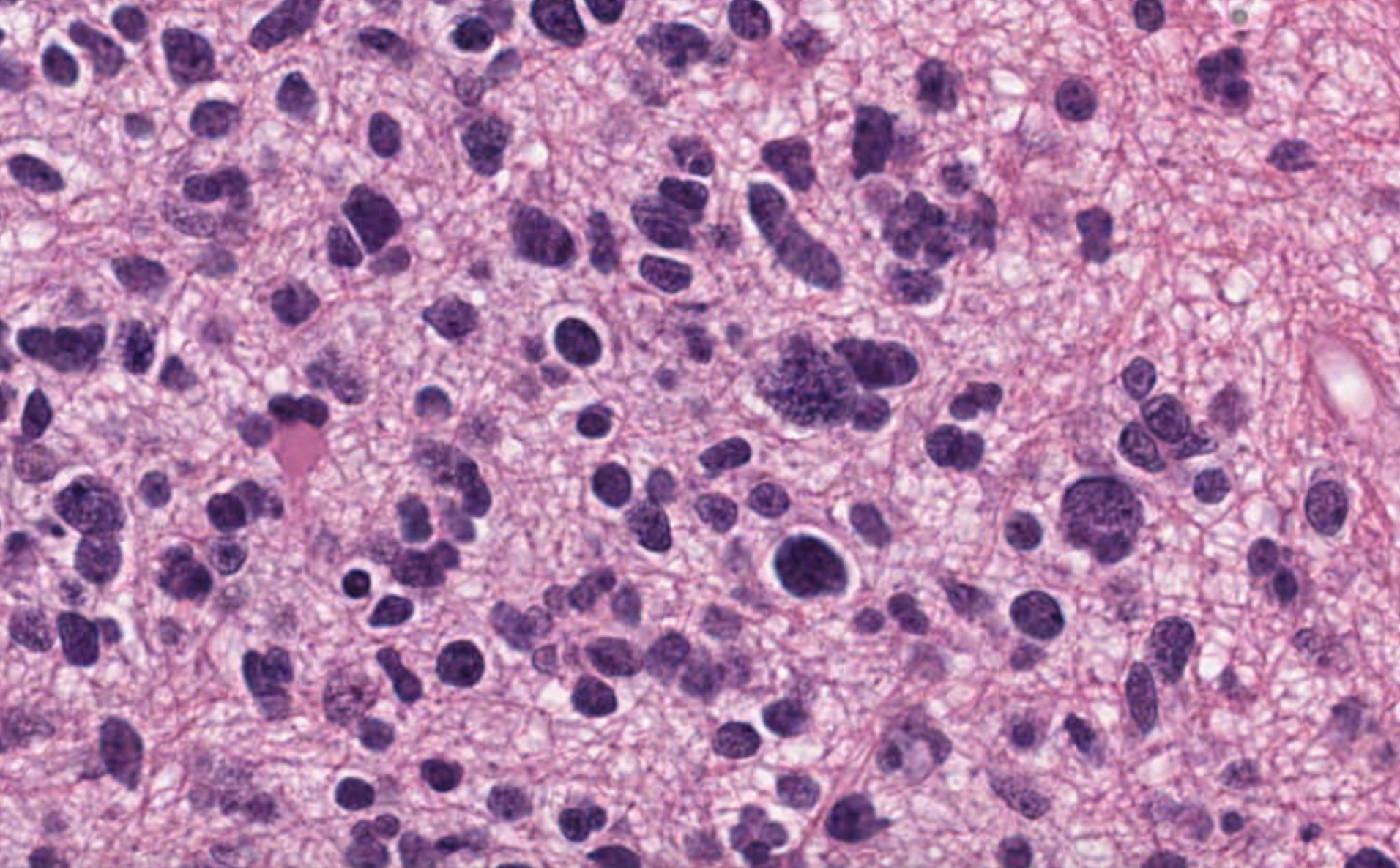
using the color scheme of this reference image
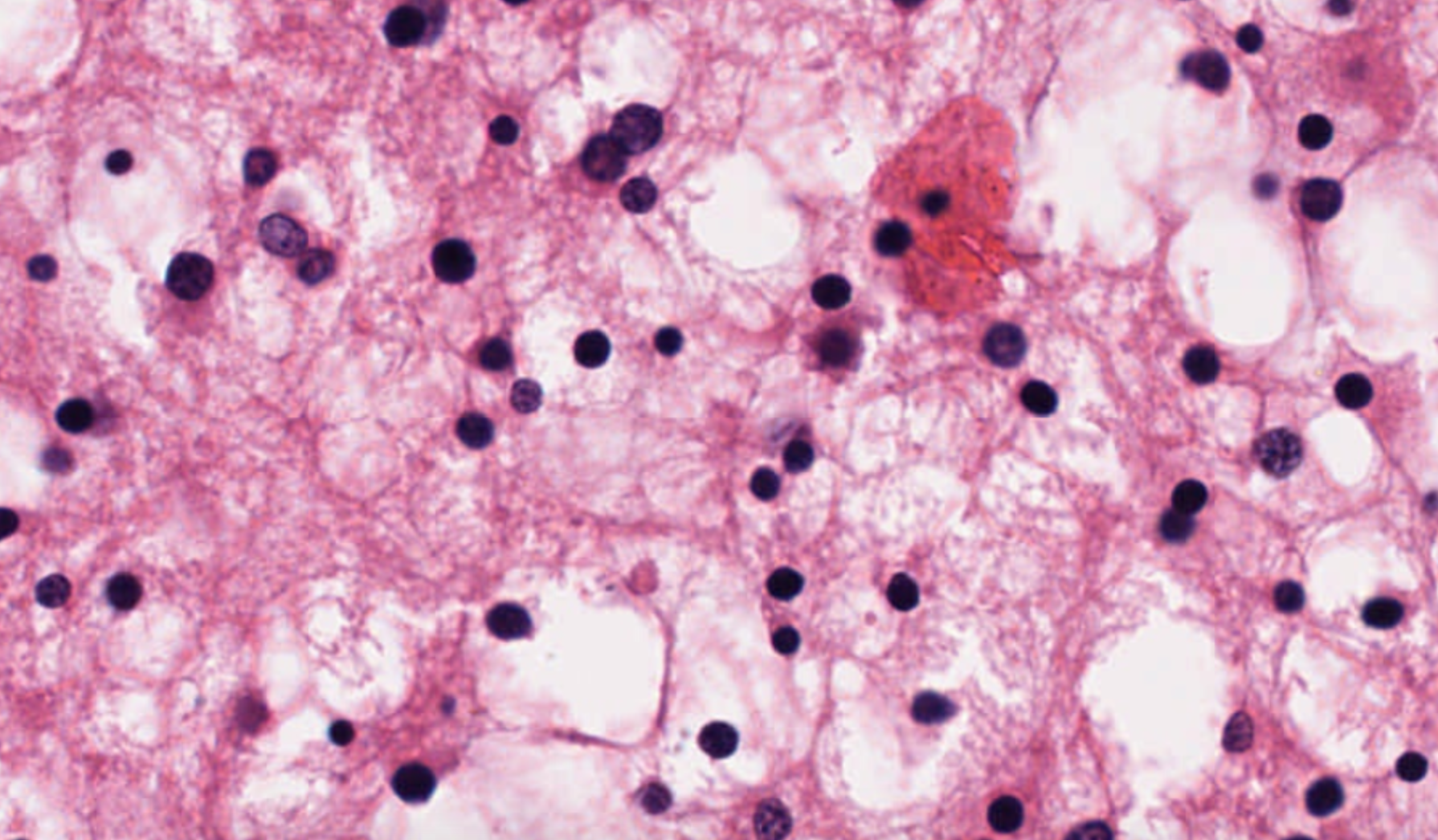
to produce this output image

### Functional interface to ITK
You can use the functional, eager interface to ITK to choose when each step will be executed as follows. The `input_image` and `reference_image` are processed to produce `normalized_image`, which is the `input_image` with the color scheme of the `reference_image`. The `color_index_suppressed_by_hematoxylin` and `color_index_suppressed_by_eosin` arguments are optional if the `input_image` pixel type is RGB or RGBA. Here you are indicating that the color channel most suppressed by hematoxylin is 0 (which is red for RGB and RGBA pixels) and that the color most suppressed by eosin is 1 (which is green for RGB and RGBA pixels)\; these are the defaults for RGB and RGBA pixels.
```python
input_image = itk.imread(input_image_filename)
reference_image = itk.imread(reference_image_filename)
eager_normalized_image = itk.structure_preserving_color_normalization_filter(
input_image,
reference_image,
color_index_suppressed_by_hematoxylin=0,
color_index_suppressed_by_eosin=1,
)
itk.imwrite(eager_normalized_image, output_image_filename)
```
### ITK pipeline interface
Alternatively, you can use the ITK pipeline infrastructure that waits until a call to `Update()` or `Write()` before executing the pipeline. The function `itk.StructurePreservingColorNormalizationFilter.New()` uses its argument to determine the pixel type for the filter\; the actual image is not used there but is supplied with the `spcn_filter.SetInput(0, input_reader.GetOutput())` call. As above, the calls to `SetColorIndexSuppressedByHematoxylin` and `SetColorIndexSuppressedByEosin` are optional if the pixel type is RGB or RGBA.
```python
input_reader = itk.ImageFileReader.New(FileName=input_image_filename)
reference_reader = itk.ImageFileReader.New(FileName=reference_image_filename)
spcn_filter = itk.StructurePreservingColorNormalizationFilter.New(
Input=input_reader.GetOutput()
)
spcn_filter.SetColorIndexSuppressedByHematoxylin(0)
spcn_filter.SetColorIndexSuppressedByEosin(1)
spcn_filter.SetInput(0, input_reader.GetOutput())
spcn_filter.SetInput(1, reference_reader.GetOutput())
output_writer = itk.ImageFileWriter.New(spcn_filter.GetOutput())
output_writer.SetInput(spcn_filter.GetOutput())
output_writer.SetFileName(output_image_filename)
output_writer.Write()
```
Note that if `spcn_filter` is used again with a different `input_image`, for example from a different reader,
```python
spcn_filter.SetInput(0, input_reader2.GetOutput())
```
but the `reference_image` is unchanged then the filter will use its cached analysis of the `reference_image`, which saves about half the processing time.
Raw data
{
"_id": null,
"home_page": "https://github.com/InsightSoftwareConsortium/ITKColorNormalization/",
"name": "itk-spcn",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "ITK InsightToolkit",
"author": "Lee Newberg",
"author_email": "lee.newberg@kitware.com",
"download_url": "",
"platform": null,
"description": "# ITKColorNormalization\n\n[](https://github.com/InsightSoftwareConsortium/ITKColorNormalization/blob/master/LICENSE) [](https://zenodo.org/badge/latestdoi/263648073) [](https://github.com/InsightSoftwareConsortium/ITKColorNormalization/actions?query=workflow%3A%22Build%2C+test%2C+package%22)\n\n## Overview\n\nThis [Insight Toolkit (ITK)](https://itk.org/) module performs Structure Preserving Color Normalization on an [H & E](https://en.wikipedia.org/wiki/H%26E_stain) image using a reference image. The module is in C++ and is also packaged for [Python](https://www.python.org/).\n\nH & E ([hematoxylin](https://en.wikipedia.org/wiki/Haematoxylin) and [eosin](https://en.wikipedia.org/wiki/Eosin)) are stains used to color parts of cells in a histological image, often for medical diagnosis. Hematoxylin is a compound that stains cell nuclei a purple-blue color. Eosin is a compound that stains extracellular matrix and cytoplasm pink. However, the exact color of purple-blue or pink can vary from image to image, and this can make comparison of images difficult. This routine addresses the issue by re-coloring one image (the first image supplied to the routine) using the color scheme of a reference image (the second image supplied to the routine). The technique requires that the images have at least 3 colors, such as red, green, and blue (RGB).\n\nStructure Preserving Color Normalization is a technique described in [Vahadane et al., 2016](https://doi.org/10.1109/TMI.2016.2529665) and modified in [Ramakrishnan et al., 2019](https://arxiv.org/abs/1901.03088). The idea is to model the color of an image pixel as something close to pure white, which is reduced in intensity in a color-specific way via an optical absorption model that depends upon the amounts of hematoxylin and eosin that are present. [Non-negative matrix factorization](https://en.wikipedia.org/wiki/Non-negative_matrix_factorization) is used on each analyzed image to simultaneously derive the amount of hematoxylin and eosin stain at each pixel and the image-wide effective colors of each stain.\n\nThe implementation in ITK accelerates the non-negative matrix factorization by choosing the initial estimate for the color absorption characteristics using a technique mimicking that presented in [Arora et al., 2013](https://proceedings.mlr.press/v28/arora13.html) and modified in [Newberg et al., 2018](https://doi.org/10.1371/journal.pone.0193067). This approach finds a good solution for a non-negative matrix factorization by first transforming it to the problem of finding a convex hull for a set of points in a cloud.\n\nThe lead developer of InsightSoftwareConsortium/ITKColorNormalization is [Lee Newberg](https://github.com/Leengit/).\n\n## Try it online\n\nWe have set up a demonstration of this using [Binder](www.mybinder.org) that anyone can try. Click here: [](https://mybinder.org/v2/gh/InsightSoftwareConsortium/ITKColorNormalization/master?filepath=examples%2FITKColorNormalization.ipynb)\n\n## Installation for Python\n\n[](https://pypi.python.org/pypi/itk-spcn)\n\nITKColorNormalization and all its dependencies can be easily installed with [Python wheels](https://blog.kitware.com/itk-is-on-pypi-pip-install-itk-is-here/). Wheels have been generated for macOS, Linux, and Windows and several versions of Python, 3.6, 3.7, 3.8, and 3.9. If you do not want the installation to be to your current Python environment, you should first create and activate a [Python virtual environment (venv)](https://docs.python.org/3/tutorial/venv.html) to work in. Then, run the following from the command-line:\n\n```shell-script\npip install itk-spcn\n```\n\nLaunch `python`, import the itk package, and set variable names for the input images\n\n```python\nimport itk\n\ninput_image_filename = \"path/to/image_to_be_normalized\"\nreference_image_filename = \"path/to/image_to_be_used_as_color_reference\"\n```\n\n## Usage in Python\n\nThe following example transforms this input image\n\n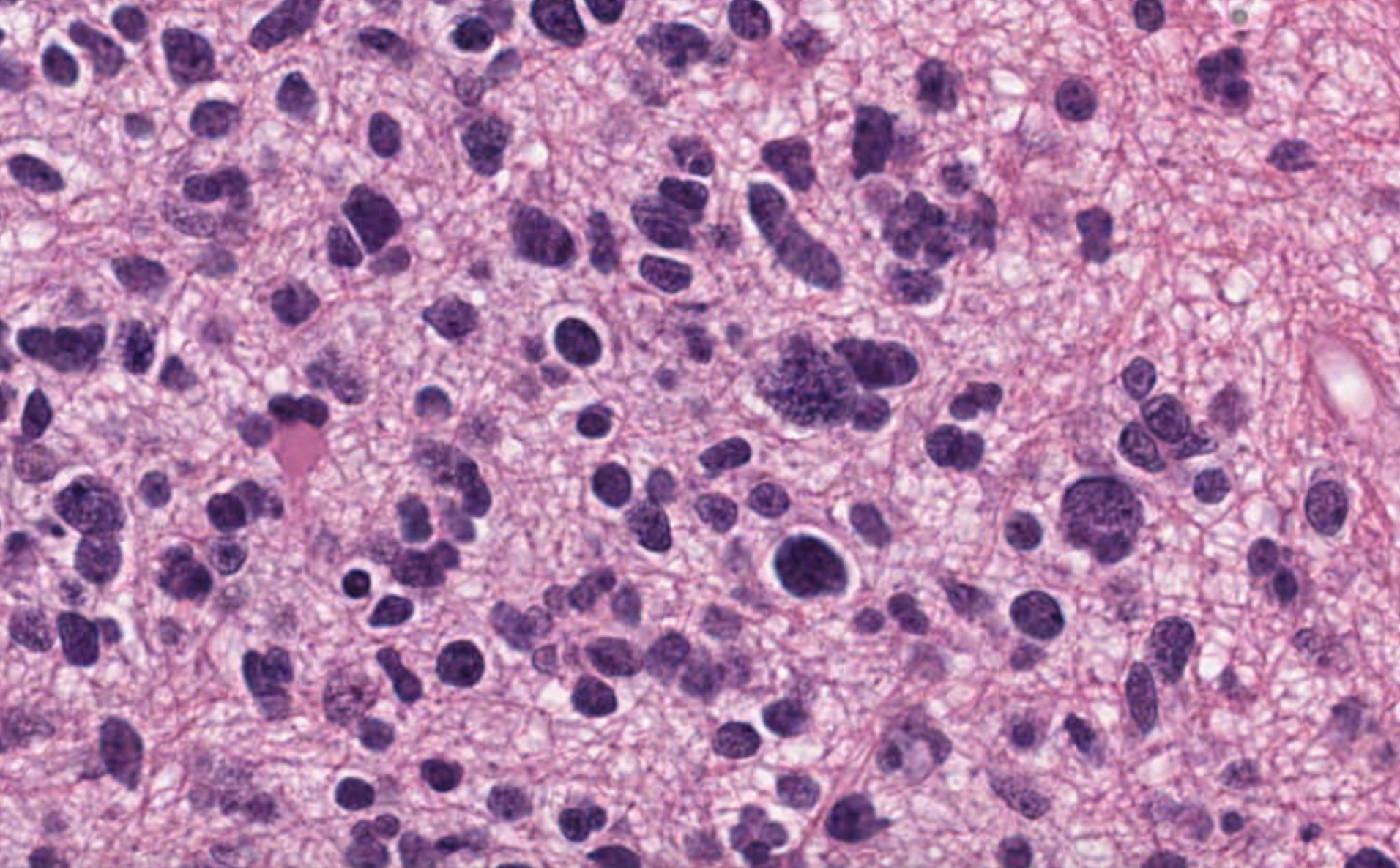\n\nusing the color scheme of this reference image\n\n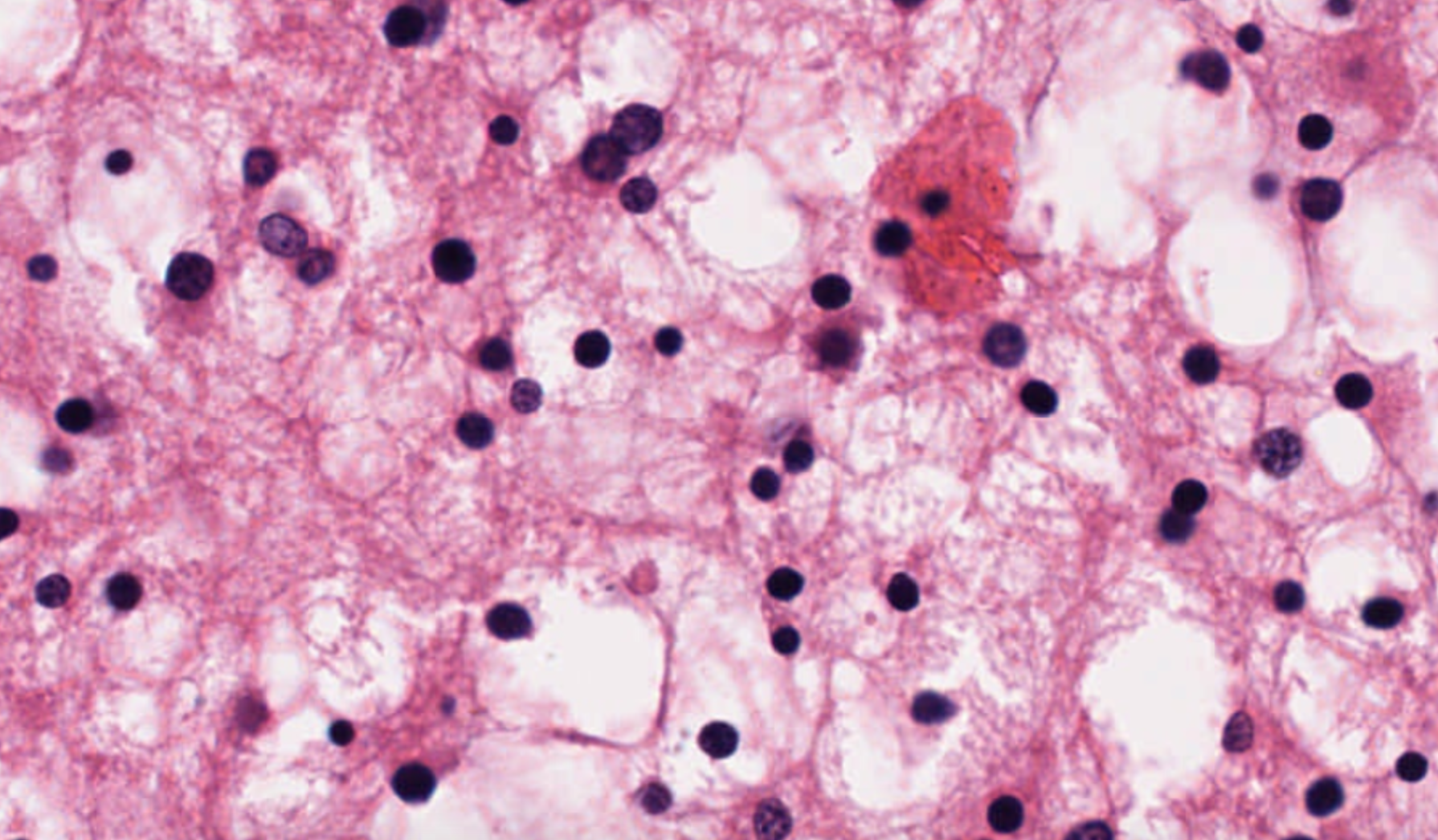\n\nto produce this output image\n\n\n\n### Functional interface to ITK\n\nYou can use the functional, eager interface to ITK to choose when each step will be executed as follows. The `input_image` and `reference_image` are processed to produce `normalized_image`, which is the `input_image` with the color scheme of the `reference_image`. The `color_index_suppressed_by_hematoxylin` and `color_index_suppressed_by_eosin` arguments are optional if the `input_image` pixel type is RGB or RGBA. Here you are indicating that the color channel most suppressed by hematoxylin is 0 (which is red for RGB and RGBA pixels) and that the color most suppressed by eosin is 1 (which is green for RGB and RGBA pixels)\\; these are the defaults for RGB and RGBA pixels.\n\n```python\ninput_image = itk.imread(input_image_filename)\nreference_image = itk.imread(reference_image_filename)\n\neager_normalized_image = itk.structure_preserving_color_normalization_filter(\n input_image,\n reference_image,\n color_index_suppressed_by_hematoxylin=0,\n color_index_suppressed_by_eosin=1,\n)\n\nitk.imwrite(eager_normalized_image, output_image_filename)\n```\n\n### ITK pipeline interface\n\nAlternatively, you can use the ITK pipeline infrastructure that waits until a call to `Update()` or `Write()` before executing the pipeline. The function `itk.StructurePreservingColorNormalizationFilter.New()` uses its argument to determine the pixel type for the filter\\; the actual image is not used there but is supplied with the `spcn_filter.SetInput(0, input_reader.GetOutput())` call. As above, the calls to `SetColorIndexSuppressedByHematoxylin` and `SetColorIndexSuppressedByEosin` are optional if the pixel type is RGB or RGBA.\n\n```python\ninput_reader = itk.ImageFileReader.New(FileName=input_image_filename)\nreference_reader = itk.ImageFileReader.New(FileName=reference_image_filename)\n\nspcn_filter = itk.StructurePreservingColorNormalizationFilter.New(\n Input=input_reader.GetOutput()\n)\nspcn_filter.SetColorIndexSuppressedByHematoxylin(0)\nspcn_filter.SetColorIndexSuppressedByEosin(1)\nspcn_filter.SetInput(0, input_reader.GetOutput())\nspcn_filter.SetInput(1, reference_reader.GetOutput())\n\noutput_writer = itk.ImageFileWriter.New(spcn_filter.GetOutput())\noutput_writer.SetInput(spcn_filter.GetOutput())\noutput_writer.SetFileName(output_image_filename)\noutput_writer.Write()\n```\n\nNote that if `spcn_filter` is used again with a different `input_image`, for example from a different reader,\n\n```python\nspcn_filter.SetInput(0, input_reader2.GetOutput())\n```\n\nbut the `reference_image` is unchanged then the filter will use its cached analysis of the `reference_image`, which saves about half the processing time.\n",
"bugtrack_url": null,
"license": "Apache",
"summary": "This performs structure preserving color normalization on an image using a reference image.",
"version": "0.2.0",
"split_keywords": [
"itk",
"insighttoolkit"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "058f27b8743f2d57b378d43e43f783a7",
"sha256": "9fcfc4571b7e851965c47a95f74dc43f5de025b926cb6e360dc9e2feb86b0a05"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "058f27b8743f2d57b378d43e43f783a7",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 2327415,
"upload_time": "2023-01-02T14:00:32",
"upload_time_iso_8601": "2023-01-02T14:00:32.455009Z",
"url": "https://files.pythonhosted.org/packages/e0/1b/328890efe1fe8e6c95f7a6386ad75f696ddc006efd052b5a4af42ec28a7c/itk_spcn-0.2.0-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "a33754688628df6d45edbf07b482aa1a",
"sha256": "443d700ef78fdecf4107f9da7ecd368ec2f62afd9d4442e6e097432dc6547b6a"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "a33754688628df6d45edbf07b482aa1a",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1766144,
"upload_time": "2023-01-02T14:00:34",
"upload_time_iso_8601": "2023-01-02T14:00:34.675473Z",
"url": "https://files.pythonhosted.org/packages/d2/4f/c86d20be3431edf7c79ed29cf32347e1407bec1e67a3b201e068636ab69e/itk_spcn-0.2.0-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "ac69a9ce1f39f4c54c48108a3442b775",
"sha256": "4a99d061a8577123a107266eaab416e543f8c9ecc8dc5c9e67131cb5c883ab14"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp310-cp310-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "ac69a9ce1f39f4c54c48108a3442b775",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 1689132,
"upload_time": "2023-01-02T14:00:36",
"upload_time_iso_8601": "2023-01-02T14:00:36.311509Z",
"url": "https://files.pythonhosted.org/packages/ac/5f/78c3a0c136ea19ae1ca7d395163b0771415589f5c20809986021e4d26c31/itk_spcn-0.2.0-cp310-cp310-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "c9e53b9c14f058f3fd2de06311ac3691",
"sha256": "de2f51a4c89e029d4d807b4eb755d4846bec1e06ca9d627386113616c60b9613"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "c9e53b9c14f058f3fd2de06311ac3691",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": null,
"size": 692357,
"upload_time": "2023-01-02T14:00:37",
"upload_time_iso_8601": "2023-01-02T14:00:37.475912Z",
"url": "https://files.pythonhosted.org/packages/f6/fc/0bb7e49f1d5c3ad129bc482ebffa38a8d40e6fb7e13a94b9684b245740d8/itk_spcn-0.2.0-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "50724b8900a06f461d29caa54509496d",
"sha256": "88e4951533a7c3526375a02e72841df4dce91579665b4381f523fff8a95e4776"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "50724b8900a06f461d29caa54509496d",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 2327409,
"upload_time": "2023-01-02T14:00:39",
"upload_time_iso_8601": "2023-01-02T14:00:39.157400Z",
"url": "https://files.pythonhosted.org/packages/84/33/a29d414e4bb299a686d271f9a7f5befce669a8425bd27a29d06c431dbf5d/itk_spcn-0.2.0-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "81a21312d5070793bb1776e2a20440bb",
"sha256": "6b85d395fd3e4a0281ce8c610f89fcd5a5781c08d5061c4f670eb44a5502e8f7"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "81a21312d5070793bb1776e2a20440bb",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1766147,
"upload_time": "2023-01-02T14:00:41",
"upload_time_iso_8601": "2023-01-02T14:00:41.428358Z",
"url": "https://files.pythonhosted.org/packages/6c/cc/63bcc3076b919f35e168a856c5e20522586cec47527633f8f2d560d1b6af/itk_spcn-0.2.0-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "a610764c7c5bd42993c1fb201d6ec447",
"sha256": "9e61974759c5143a227a29f167d4038681d15d27d8e4652bdec0efcfcf1d5761"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp311-cp311-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "a610764c7c5bd42993c1fb201d6ec447",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 1689094,
"upload_time": "2023-01-02T14:00:43",
"upload_time_iso_8601": "2023-01-02T14:00:43.407573Z",
"url": "https://files.pythonhosted.org/packages/92/e3/0a46e8aff6651c28856fa776b25f3f4417a2254632efd7a50cf331b0a060/itk_spcn-0.2.0-cp311-cp311-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "0e8e0361721bd2e1171c4282d0bf2aab",
"sha256": "1347a763ac2b1e99af081dd4dee9e765b0edcda00e106b41f784a76910eea4e5"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "0e8e0361721bd2e1171c4282d0bf2aab",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": null,
"size": 692370,
"upload_time": "2023-01-02T14:00:44",
"upload_time_iso_8601": "2023-01-02T14:00:44.955560Z",
"url": "https://files.pythonhosted.org/packages/e0/11/60b98b90937700e40e908e256da2966d0b873930013f8fd66b0be321cf06/itk_spcn-0.2.0-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "02185d8682230e166f959a3f65762c50",
"sha256": "25e1ec4fbcacf26b9933a7d67e56a999615b1b63307376131794e2b3b1e1e5da"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp37-cp37m-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "02185d8682230e166f959a3f65762c50",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": null,
"size": 2327844,
"upload_time": "2023-01-02T14:00:46",
"upload_time_iso_8601": "2023-01-02T14:00:46.656636Z",
"url": "https://files.pythonhosted.org/packages/e0/59/a020e3339e1ab7add3204287834881ad403055e84306a286f390078831f6/itk_spcn-0.2.0-cp37-cp37m-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "89502208015adcb5a2e845c08ea97473",
"sha256": "61eeea77ab96a2d86de680f8f1bf8d9ba8f111e75e1130c6214771b8eff7a2e3"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "89502208015adcb5a2e845c08ea97473",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": null,
"size": 1766241,
"upload_time": "2023-01-02T14:00:48",
"upload_time_iso_8601": "2023-01-02T14:00:48.435467Z",
"url": "https://files.pythonhosted.org/packages/f2/d4/8572cd5802aa6887ef2a5c6b3bac6350bd53d125078eefef7953220ff427/itk_spcn-0.2.0-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "660ba9730ae616ab7a1afd5837bffd3d",
"sha256": "52067887188f2f2e401bed018bc3a2749b1599c45c78be80de16109722574d66"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp37-cp37m-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "660ba9730ae616ab7a1afd5837bffd3d",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": null,
"size": 1690138,
"upload_time": "2023-01-02T14:00:49",
"upload_time_iso_8601": "2023-01-02T14:00:49.872140Z",
"url": "https://files.pythonhosted.org/packages/2a/08/414066b7831de428a83c419cfcfd93b0cf05d6adc822b143dd5ab834f860/itk_spcn-0.2.0-cp37-cp37m-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "35c43ed06c9f437db746e535798358d1",
"sha256": "8efde28ccd186846d397b4422ab4ee2e8da6210b57c188387aae14d4a9a1145f"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp37-cp37m-win_amd64.whl",
"has_sig": false,
"md5_digest": "35c43ed06c9f437db746e535798358d1",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": null,
"size": 717201,
"upload_time": "2023-01-02T14:00:51",
"upload_time_iso_8601": "2023-01-02T14:00:51.484027Z",
"url": "https://files.pythonhosted.org/packages/d4/74/d333117661079e1d9bf11f1024051bc11793571281b9784b096d40a5b5b8/itk_spcn-0.2.0-cp37-cp37m-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "73171eb176e4011f499acaec0c0cdcdd",
"sha256": "1ee0631cd178906c963048c358c482e391ff39181f2f854f0ac072dc94ef9a4b"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp38-cp38-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "73171eb176e4011f499acaec0c0cdcdd",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 2327598,
"upload_time": "2023-01-02T14:00:52",
"upload_time_iso_8601": "2023-01-02T14:00:52.841483Z",
"url": "https://files.pythonhosted.org/packages/4c/83/06a93a412eaebb994d34ae4e5f88585587bc8575ee1f1d201ead6a7c137d/itk_spcn-0.2.0-cp38-cp38-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "c1c1fa245585af3f52146c7a74b2da15",
"sha256": "ef776077c2ce33027150d1ebc6be1860287ad585f49b28f1e4f81ee9c797d138"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "c1c1fa245585af3f52146c7a74b2da15",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1765938,
"upload_time": "2023-01-02T14:00:54",
"upload_time_iso_8601": "2023-01-02T14:00:54.330567Z",
"url": "https://files.pythonhosted.org/packages/3b/64/dc849ee874600046dec2fd95d8febdd3dfa303dd5d10190498d22155eebe/itk_spcn-0.2.0-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "2d13cdfeabcf8574e5d203d711f40927",
"sha256": "753ba5eba828c727ad81911fb5717b5eb3afd34163d64dabb671514c01e53bea"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp38-cp38-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "2d13cdfeabcf8574e5d203d711f40927",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 1692227,
"upload_time": "2023-01-02T14:00:55",
"upload_time_iso_8601": "2023-01-02T14:00:55.991251Z",
"url": "https://files.pythonhosted.org/packages/cc/cc/97b20cc4da14ebb45fd0c7160b7102d7dee018352ac534744b37ac7e81e7/itk_spcn-0.2.0-cp38-cp38-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "6e9cfb6e591759af5ee3224da3cdcfb2",
"sha256": "c2f6c32e6e751fad8964afd2e115878f2d9b2a1b7e79f415707883fe2fcda3a6"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp38-cp38-win_amd64.whl",
"has_sig": false,
"md5_digest": "6e9cfb6e591759af5ee3224da3cdcfb2",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": null,
"size": 718149,
"upload_time": "2023-01-02T14:00:57",
"upload_time_iso_8601": "2023-01-02T14:00:57.438920Z",
"url": "https://files.pythonhosted.org/packages/f0/a3/6b5931d81b18c7a10820ecf8637947e199e8352a24269acc7007ff3a83bb/itk_spcn-0.2.0-cp38-cp38-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "07a04bef046b672a1c69dd96c1fa108e",
"sha256": "8903f705e4703116c3ba27f14776fb053e75b245992fac9237879c3ae128fcc2"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "07a04bef046b672a1c69dd96c1fa108e",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 2327442,
"upload_time": "2023-01-02T14:00:59",
"upload_time_iso_8601": "2023-01-02T14:00:59.058176Z",
"url": "https://files.pythonhosted.org/packages/1e/fc/50be9fc56322203e8032d74241159eeaba984915b5dcfc982cd05717f6c2/itk_spcn-0.2.0-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "03168042def6e40fa594d21d7adac050",
"sha256": "6256608b443485e068036147f372533c688c884e58c35f397040138e15257c23"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "03168042def6e40fa594d21d7adac050",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1765933,
"upload_time": "2023-01-02T14:01:00",
"upload_time_iso_8601": "2023-01-02T14:01:00.907121Z",
"url": "https://files.pythonhosted.org/packages/6f/50/9b3b1a34b70e398a7fc69cec30c05225cd4ec4a859e31c5d952dfc70b105/itk_spcn-0.2.0-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "6e6e301a5ddccfff359aff083c1c01a3",
"sha256": "18e3c99db96cd2e75b768bf10d37b2931d0dd21a565a999356297568efb7b971"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp39-cp39-manylinux_2_28_x86_64.whl",
"has_sig": false,
"md5_digest": "6e6e301a5ddccfff359aff083c1c01a3",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 1689206,
"upload_time": "2023-01-02T14:01:02",
"upload_time_iso_8601": "2023-01-02T14:01:02.747422Z",
"url": "https://files.pythonhosted.org/packages/4a/5c/b15f5c83cf828e111c73ea18a2298ebbbfa496aa644d48aa055b0ae037d5/itk_spcn-0.2.0-cp39-cp39-manylinux_2_28_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "38f92ef8da580164503593b22bd876fa",
"sha256": "526895d0ef8c08ec4076344cec7927efba7915d2e079f8693754233af1bf4b98"
},
"downloads": -1,
"filename": "itk_spcn-0.2.0-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "38f92ef8da580164503593b22bd876fa",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": null,
"size": 692857,
"upload_time": "2023-01-02T14:01:04",
"upload_time_iso_8601": "2023-01-02T14:01:04.371659Z",
"url": "https://files.pythonhosted.org/packages/c0/19/9111688b1acba06fbb6a85b9093352836011bfe46eb20ab764bddf4bb19b/itk_spcn-0.2.0-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-01-02 14:00:32",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "InsightSoftwareConsortium",
"github_project": "ITKColorNormalization",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "itk-spcn"
}