Name | json-stream JSON |
Version |
2.3.2
JSON |
| download |
home_page | |
Summary | Streaming JSON encoder and decoder |
upload_time | 2023-06-30 09:43:16 |
maintainer | |
docs_url | None |
author | |
requires_python | <4,>=3.5 |
license | Copyright (c) 2020 Jamie Cockburn Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
json
stream
decoder
encoder
parsing
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# json-stream
[](https://github.com/daggaz/json-stream/actions/workflows/tests.yml)
[](https://pypi.org/project/json-stream)
[](https://pypi.org/project/json-stream/)
[](https://www.buymeacoffee.com/daggaz)
Simple streaming JSON parser and encoder.
When [reading](#reading) JSON data, `json-stream` can decode JSON data in
a streaming manner, providing a pythonic dict/list-like interface, or a
[visitor-based interfeace](#visitor). Can stream from files, [URLs](#urls)
or [iterators](#iterators).
When [writing](#writing) JSON data, `json-stream` can stream JSON objects
as you generate them.
These techniques allow you to [reduce memory consumption and
latency](#standard-json-problems).
# <a id="reading"></a> Reading
`json-stream` is a JSON parser just like the standard library's
[`json.load()`](https://docs.python.org/3/library/json.html#json.load). It
will read a JSON document and convert it into native python types.
```python
import json_stream
data = json_stream.load(f)
```
Features:
* stream all JSON data types (objects, lists and simple types)
* stream nested data
* simple pythonic `list`-like/`dict`-like interface
* stream truncated or malformed JSON data (up to the first error)
* [native code parsing speedups](#rust-tokenizer) for most common platforms
* pure python fallback if native extensions not available
Unlike `json.load()`, `json-stream` can _stream_ JSON data from any file-like or
[iterable](#iterators) object. This has the following benefits:
* it does not require the whole json document to be read into memory up-front
* it can start producing data before the entire document has finished loading
* it only requires enough memory to hold the data currently being parsed
There are specific integrations for streaming JSON data from [URLs](#urls) using the
[`requests`](#requests), [`httpx`](#httpx), or [`urllib`](#urllib).
The objects that `json-stream` produces can be [re-output](#encoding-json-stream-objects)
using `json.dump()` with a little work.
## Usage
### `json_stream.load()`
`json_stream.load()` has two modes of operation, controlled by
the `persistent` argument (default false).
It is also possible to "mix" the modes as you consume the data.
#### Transient mode (default)
This mode is appropriate if you can consume the data iteratively. You cannot
move backwards through the stream to read data that has already been skipped
over. It is the mode you **must** use if you want to process large amounts of
JSON data without consuming large amounts of memory.
In transient mode, only the data currently being read is stored in memory. Any
data previously read from the stream is discarded (it's up to you what to do
with it) and attempting to access this data results in a
`TransientAccessException`.
```python
import json_stream
# JSON: {"count": 3, "results": ["a", "b", "c"]}
data = json_stream.load(f) # data is a transient dict-like object
# stream has been read up to "{"
# use data like a dict
results = data["results"] # results is a transient list-like object
# stream has been read up to "[", we now cannot read "count"
# iterate transient list
for result in results:
print(result) # prints a, b, c
# stream has been read up to "]"
# attempt to read "count" from earlier in stream
count = data["count"] # will raise exception
# stream is now exhausted
# attempt to read from list that has already been iterated
for result in results: # will raise exception
pass
```
#### Persistent mode
In persistent mode all previously read data is stored in memory as
it is parsed. The returned `dict`-like or `list`-like objects
can be used just like normal data structures.
If you request an index or key that has already been read from the stream
then it is retrieved from memory. If you request an index or key that has
not yet been read from the stream, then the request blocks until that item
is found in the stream.
```python
import json_stream
# JSON: {"count": 1, "results": ["a", "b", "c"]}
data = json_stream.load(f, persistent=True)
# data is a streaming dict-like object
# stream has been read up to "{"
# use data like a dict
results = data["results"] # results is a streaming list-like object
# stream has been read up to "["
# count has been stored data
# use results like a list
a_result = results[1] # a_result = "b"
# stream has been read up to the middle of list
# "a" and "b" have been stored in results
# read earlier data from memory
count = data["count"] # count = 1
# consume rest of list
results.read_all()
# stream has been read up to "}"
# "c" is now stored in results too
# results.is_streaming() == False
# consume everything
data.read_all()
# stream is now exhausted
# data.is_streaming() == False
```
Persistent mode is not appropriate if you care about memory consumption, but
provides an identical experience compared to `json.load()`.
#### Mixed mode
In some cases you will need to be able to randomly access some part of the
data, but still only have that specific data taking up memory resources.
For example, you might have a very long list of objects, but you cannot always
access the keys of the objects in stream order. You want to be able to iterate
the list transiently, but access the result objects persistently.
This can be achieved using the `persistent()` method of all the `list` or
`dict`-like objects json_stream produces. Calling `persistent()` causes the existing
transient object to produce persistent child objects.
Note that the `persistent()` method makes the children of the object it
is called on persistent, not the object it is called on.
```python
import json_stream
# JSON: {"results": [{"x": 1, "y": 3}, {"y": 4, "x": 2}]}
# note that the keys of the inner objects are not ordered
data = json_stream.load(f) # data is a transient dict-like object
# iterate transient list, but produce persistent items
for result in data['results'].persistent():
# result is a persistent dict-like object
print(result['x']) # print x
print(result['y']) # print y (error on second result without .persistent())
print(result['x']) # print x again (error without .persistent())
```
The opposite is also possible, going from persistent mode to transient mode, though
the use cases for this are more esoteric.
```python
# JSON: {"a": 1, "x": ["long", "list", "I", "don't", "want", "in", "memory"], "b": 2}
data = load(StringIO(json), persistent=True).transient()
# data is a persistent dict-list object that produces transient children
print(data["a"]) # prints 1
x = data["x"] # x is a transient list, you can use it accordingly
print(x[0]) # prints long
# access earlier data from memory
print(data["a"]) # this would have raised an exception if data was transient
print(data["b"]) # prints 2
# we have now moved past all the data in the transient list
print(x[0]) # will raise exception
```
### <a id="visitor"></a>visitor pattern
You can also parse using a visitor-style approach where a function you supply
is called for each data item as it is parsed (depth-first).
This uses a transient parser under the hood, so does not consume memory for
the whole document.
```python
import json_stream
# JSON: {"x": 1, "y": {}, "xxxx": [1,2, {"yyyy": 1}, "z", 1, []]}
def visitor(item, path):
print(f"{item} at path {path}")
json_stream.visit(f, visitor)
```
Output:
```
1 at path ('x',)
{} at path ('y',)
1 at path ('xxxx', 0)
2 at path ('xxxx', 1)
1 at path ('xxxx', 2, 'yyyy')
z at path ('xxxx', 3)
1 at path ('xxxx', 4)
[] at path ('xxxx', 5)
```
### <a id="urls"></a> Stream a URL
`json_stream` knows how to stream directly from a URL using a variety of packages.
Supported packages include:
- Python's batteries-included [`urllib`](#urllib) package
- The popular [`requests`](#requests) library
- The newer [`httpx`](#httpx) library
#### <a id="urllib"></a> urllib
[`urllib`](https://docs.python.org/3/library/urllib.html)'s response objects are already
file-like objects, so we can just pass them directly to `json-stream`.
```python
import urllib.request
import json_stream
with urllib.request.urlopen('http://example.com/data.json') as response:
data = json_stream.load(response)
```
#### <a id="requests"></a>requests
To stream JSON data from [`requests`](https://requests.readthedocs.io/en/latest/), you must
pass `stream=True` when making a request, and call `json_stream.requests.load()` passing the response.
```python
import requests
import json_stream.requests
with requests.get('http://example.com/data.json', stream=True) as response:
data = json_stream.requests.load(response)
```
<a id="requests-chunk-size"></a>
Note: these functions use
[`response.iter_content()`](https://requests.readthedocs.io/en/latest/api/#requests.Response.iter_content) under the
hood with a `chunk_size` of 10k bytes. This default allows us to perform effective reads from the response stream and
lower CPU usage. The drawback to this is that `requests` will buffer each read until up to 10k bytes have been read
before passing the data back to `json_stream`. If you need to consume data more responsively the only option is to tune
`chunk_size` back to 1 to disable buffering.
#### <a id="httpx"></a> httpx
To stream JSON data from [`httpx`](https://www.python-httpx.org/), you must call
[`stream()`](https://www.python-httpx.org/quickstart/#streaming-responses) when
making your request, and call `json_stream.httpx.load()` passing the response.
```python
import httpx
import json_stream.httpx
with httpx.Client() as client, client.stream('GET', 'http://example.com/data.json') as response:
data = json_stream.httpx.load(response)
```
Under the hood, this works similarly to the [`requests`](#requests) version above, including
the caveat about [`chunk_size`](#requests-chunk-size).
### Stream a URL (with visitor)
The visitor pattern also works with URL streams.
#### urllib
```python
import urllib.request
import json_stream
def visitor(item, path):
print(f"{item} at path {path}")
with urllib.request.urlopen('http://example.com/data.json') as response:
json_stream.visit(response, visitor)
```
#### requests
```python
import requests
import json_stream.requests
def visitor(item, path):
print(f"{item} at path {path}")
with requests.get('http://example.com/data.json', stream=True) as response:
json_stream.requests.visit(response, visitor)
```
The [`chunk_size`](#requests-chunk-size) note also applies to `visit()`.
#### httpx
```python
import httpx
import json_stream.httpx
def visitor(item, path):
print(f"{item} at path {path}")
with httpx.Client() as client, client.stream('GET', 'http://example.com/data.json') as response:
json_stream.httpx.visit(response, visitor)
```
### <a id="iterators"></a> Stream an iterable
`json-stream`'s parsing functions can take any iterable object that produces encoded JSON as
`byte` objects.
```python
import json_stream
def some_iterator():
yield b'{"some":'
yield b' "JSON"}'
data = json_stream.load(some_iterator())
assert data['some'] == "JSON"
```
This is actually how the [`requests`](#requests) and [`httpx`](#httpx) extensions work, as
both libraries provide methods to iterate over the response content.
### <a id="encoding-json-stream-objects"></a> Encoding json-stream objects
You can re-output (encode) _persistent_ json-stream `dict`-like and `list`-like object back to JSON using the built-in
`json.dump()` or `json.dumps()` functions, but with a little additional work:
```python
import json
import json_stream
from json_stream.dump import JSONStreamEncoder, default
data = json_stream.load(f, persistent=True)
# Option 1: supply json_stream.encoding.default as the default argument
print(json.dumps(data, default=default))
# Option 2: supply json_stream.encoding.JSONStreamEncoder as the cls argument
# This allows you to create your own subclass to further customise encoding
print(json.dumps(data, cls=JSONStreamEncoder))
```
If you are using a library that internally takes data you pass it and encodes
it using `json.dump()`. You can also use JSONStreamEncoder() as a context manager.
It works by monkey-patching the built-in `JSONEncoder.default` method during the
scope of the `with` statement.
```python
# library code
def some_library_function_out_of_your_control(arg):
json.dumps(arg)
# your code
with JSONStreamEncoder():
some_library_function_out_of_your_control(data)
```
### Converting to standard Python types
To convert a json-stream `dict`-like or `list`-like object and all its
descendants to a standard `list` and `dict`, you can use the
`json_stream.to_standard_types` utility:
```python
# JSON: {"round": 1, "results": [1, 2, 3]}
data = json_stream.load(f)
results = data["results"]
print(results) # prints <TransientStreamingJSONList: TRANSIENT, STREAMING>
converted = json_stream.to_standard_types(results)
print(converted) # prints [1, 2, 3]
```
#### Thread safety (experimental)
There is also a thread-safe version of the `json.dump` context manager:
```python
from json_stream.dump.threading import ThreadSafeJSONStreamEncoder
# your code
with ThreadSafeJSONStreamEncoder():
some_library_function_out_of_your_control(data)
```
The thread-safe implementation will ensure that concurrent uses of the
context manager will only apply the patch for the first thread entering
the patched section(s) and will only remove the patch when the last
thread exits the patched sections(s)
Additionally, if the patch is somehow called by a thread that is _not_
currently in a patched section (i.e. some other thread calling
`json.dump`) then that thread will block until the patch has been
removed. While such an un-patched thread is active, any thread attempting
to apply the patch is blocked.
### <a id="rust-tokenizer"></a> Rust tokenizer speedups
By default `json-stream` uses the
[`json-stream-rs-tokenizer`](https://pypi.org/project/json-stream-rs-tokenizer/)
native extension.
This is a 3rd party Rust-based tokenizer implementations that provides
significant parsing speedup compared to pure python implementation.
`json-stream` will fallback to its pure python tokenizer implementation
if `json-stream-rs-tokenizer` is not available.
### Custom tokenizer
You can supply an alternative JSON tokenizer implementation. Simply pass
a tokenizer to the `load()` or `visit()` methods.
```python
json_stream.load(f, tokenizer=some_tokenizer)
```
The requests methods also accept a customer tokenizer parameter.
# Writing
The standard library's `json.dump()` function can only accept standard
python types such as `dict`, `list`, `str`.
`json-stream` allows you to write streaming JSON output based on python
generators instead.
For actually encoding and writing to the stream, `json-stream`
still uses the standard library's `json.dump()` function, but provides
wrappers that adapt python generators into `dict`/`list` subclasses
that `json.dump()` can use.
The means that you do not have to generate all of your data upfront
before calling `json.dump()`.
## Usage
To use `json-stream` to generate JSON data iteratively, you must first
write python generators (or use any other iterable).
To output JSON objects, the iterable must yield key/value pairs.
To output JSON lists, the iterable must yield individual items.
The values yielded can be either be standard python types or another other
`Streamable` object, allowing lists and object to be arbitrarily nested.
`streamable_list`/`streamable_dict` can be used to wrap an existing
iterable:
```python
import sys
import json
from json_stream import streamable_list
# wrap existing iterable
data = streamable_list(range(10))
# consume iterable with standard json.dump()
json.dump(data, sys.stdout)
```
Or they can be used as decorators on generator functions:
```python
import json
import sys
from json_stream import streamable_dict
# declare a new streamable dict generator function
@streamable_dict
def generate_dict_of_squares(n):
for i in range(n):
# this could be some memory intensive operation
# or just a really large value of n
yield i, i ** 2
# data is will already be Streamable because
# of the decorator
data = generate_dict_of_squares(10)
json.dump(data, sys.stdout)
```
## Example
The following example generates a JSON object with a nested JSON list.
It uses `time.sleep()` to slow down the generation and show that the
output is indeed written as the data is created.
```python
import sys
import json
import time
from json_stream.writer import streamable_dict, streamable_list
# define a list data generator that (slowly) yields
# items that will be written as a JSON list
@streamable_list
def generate_list(n):
# output n numbers and their squares
for i in range(n): # range is itself a generator
yield i
time.sleep(1)
# define a dictionary data generator that (slowly) yields
# key/value pairs that will be written as a JSON dict
@streamable_dict
def generate_dict(n):
# output n numbers and their squares
for i in range(n): # range is itself a generator
yield i, i ** 2
time.sleep(1)
# yield another dictionary item key, with the value
# being a streamed nested list
yield "a list", generate_list(n)
# get a streamable generator
data = generate_dict(5)
# use json.dump() to write dict generator to stdout
json.dump(data, sys.stdout, indent=2)
# if you already have an iterable object, you can just
# call streamable_* on it to make it writable
data = streamable_list(range(10))
json.dump(data, sys.stdout)
```
Output:
```json
{
"0": 0,
"1": 1,
"2": 4,
"3": 9,
"4": 16,
"a list": [
0,
1,
2,
3,
4
]
}
```
# <a id="standard-json-problems"></a> What are the problems with the standard `json` package?
## Reading with `json.load()`
The problem with the `json.load()` stem from the fact that it must read
the whole JSON document into memory before parsing it.
### Memory usage
`json.load()` first reads the whole document into memory as a string. It
then starts parsing that string and converting the whole document into python
types again stored in memory. For a very large document, this could be more
memory than you have available to your system.
`json_stream.load()` does not read the whole document into memory, it only
buffers enough from the stream to produce the next item of data.
Additionally, in the default transient mode (see below) `json-stream` doesn't store
up all of the parsed data in memory.
### Latency
`json.load()` produces all the data after parsing the whole document. If you
only care about the first 10 items in a list of 2 million items, then you
have wait until all 2 million items have been parsed first.
`json_stream.load()` produces data as soon as it is available in the stream.
## <a id="writing"></a> Writing
### Memory usage
While `json.dump()` does iteratively write JSON data to the given
file-like object, you must first produce the entire document to be
written as standard python types (`dict`, `list`, etc). For a very
large document, this could be more memory than you have available
to your system.
`json-stream` allows you iteratively generate your data one item at
a time, and thus consumes only the memory required to generate that
one item.
### Latency
`json.dump()` can only start writing to the output file once all the
data has been generated up front at standard python types.
The iterative generation of JSON items provided by `json-stream`
allows the data to be written as it is produced.
# Future improvements
* Allow long strings in the JSON to be read as streams themselves
* Allow transient mode on seekable streams to seek to data earlier in
the stream instead of raising a `TransientAccessException`
* A more efficient tokenizer?
# Alternatives
## NAYA
[NAYA](https://github.com/danielyule/naya) is a pure python JSON parser for
parsing a simple JSON list as a stream.
### Why not NAYA?
* It can only stream JSON containing a top-level list
* It does not provide a pythonic `dict`/`list`-like interface
## Yajl-Py
[Yajl-Py](https://pykler.github.io/yajl-py/) is a wrapper around the C YAJL JSON library that can be used to
generate SAX style events while parsing JSON.
### Why not Yajl-Py?
* No pure python implementation
* It does not provide a pythonic `dict`/`list`-like interface
## jsonslicer
[jsonslicer](https://github.com/AMDmi3/jsonslicer) is another wrapper around the YAJL C library with a
path lookup based interface.
### Why not jsonslicer?
* No pure python implementation
* It does not provide a pythonic `dict`/`list`-like interface
* Must know all data paths lookup in advance (or make multiple passes)
# Contributing
See the project [contribution guide](https://github.com/daggaz/json-stream/blob/master/CONTRIBUTING.md).
# Donations
[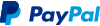](https://paypal.me/JCockburn307?country.x=GB&locale.x=en_GB)
OR
[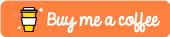](https://www.buymeacoffee.com/daggaz)
# Acknowledgements
The JSON tokenizer used in the project was taken from the
[NAYA](https://github.com/danielyule/naya) project.
Raw data
{
"_id": null,
"home_page": "",
"name": "json-stream",
"maintainer": "",
"docs_url": null,
"requires_python": "<4,>=3.5",
"maintainer_email": "",
"keywords": "json,stream,decoder,encoder,parsing",
"author": "",
"author_email": "Jamie Cockburn <jamie_cockburn@hotmail.co.uk>",
"download_url": "https://files.pythonhosted.org/packages/6a/0c/8d654a510a43e4a5ec8a8ac523a1d225daa81afaccd69e974577c9cfb18b/json-stream-2.3.2.tar.gz",
"platform": null,
"description": "# json-stream\n\n[](https://github.com/daggaz/json-stream/actions/workflows/tests.yml)\n[](https://pypi.org/project/json-stream)\n[](https://pypi.org/project/json-stream/)\n[](https://www.buymeacoffee.com/daggaz)\n\nSimple streaming JSON parser and encoder.\n\nWhen [reading](#reading) JSON data, `json-stream` can decode JSON data in \na streaming manner, providing a pythonic dict/list-like interface, or a\n[visitor-based interfeace](#visitor). Can stream from files, [URLs](#urls) \nor [iterators](#iterators).\n\nWhen [writing](#writing) JSON data, `json-stream` can stream JSON objects \nas you generate them.\n\nThese techniques allow you to [reduce memory consumption and \nlatency](#standard-json-problems).\n\n# <a id=\"reading\"></a> Reading\n\n`json-stream` is a JSON parser just like the standard library's\n [`json.load()`](https://docs.python.org/3/library/json.html#json.load). It \n will read a JSON document and convert it into native python types.\n\n```python\nimport json_stream\ndata = json_stream.load(f)\n```\n\nFeatures:\n* stream all JSON data types (objects, lists and simple types)\n* stream nested data\n* simple pythonic `list`-like/`dict`-like interface\n* stream truncated or malformed JSON data (up to the first error)\n* [native code parsing speedups](#rust-tokenizer) for most common platforms \n* pure python fallback if native extensions not available\n\nUnlike `json.load()`, `json-stream` can _stream_ JSON data from any file-like or\n[iterable](#iterators) object. This has the following benefits:\n\n* it does not require the whole json document to be read into memory up-front\n* it can start producing data before the entire document has finished loading\n* it only requires enough memory to hold the data currently being parsed\n\nThere are specific integrations for streaming JSON data from [URLs](#urls) using the \n[`requests`](#requests), [`httpx`](#httpx), or [`urllib`](#urllib).\n\nThe objects that `json-stream` produces can be [re-output](#encoding-json-stream-objects)\nusing `json.dump()` with a little work.\n\n## Usage\n\n### `json_stream.load()`\n\n`json_stream.load()` has two modes of operation, controlled by\nthe `persistent` argument (default false).\n\nIt is also possible to \"mix\" the modes as you consume the data.\n\n#### Transient mode (default)\n\nThis mode is appropriate if you can consume the data iteratively. You cannot \nmove backwards through the stream to read data that has already been skipped\nover. It is the mode you **must** use if you want to process large amounts of\nJSON data without consuming large amounts of memory.\n\nIn transient mode, only the data currently being read is stored in memory. Any\ndata previously read from the stream is discarded (it's up to you what to do \nwith it) and attempting to access this data results in a\n`TransientAccessException`.\n\n```python\nimport json_stream\n\n# JSON: {\"count\": 3, \"results\": [\"a\", \"b\", \"c\"]}\ndata = json_stream.load(f) # data is a transient dict-like object \n# stream has been read up to \"{\"\n\n# use data like a dict\nresults = data[\"results\"] # results is a transient list-like object\n# stream has been read up to \"[\", we now cannot read \"count\"\n\n# iterate transient list\nfor result in results:\n print(result) # prints a, b, c\n# stream has been read up to \"]\"\n\n# attempt to read \"count\" from earlier in stream\ncount = data[\"count\"] # will raise exception\n# stream is now exhausted\n\n# attempt to read from list that has already been iterated\nfor result in results: # will raise exception\n pass\n```\n\n#### Persistent mode\n\nIn persistent mode all previously read data is stored in memory as\nit is parsed. The returned `dict`-like or `list`-like objects\ncan be used just like normal data structures.\n\nIf you request an index or key that has already been read from the stream\nthen it is retrieved from memory. If you request an index or key that has\nnot yet been read from the stream, then the request blocks until that item\nis found in the stream.\n\n```python\nimport json_stream\n\n# JSON: {\"count\": 1, \"results\": [\"a\", \"b\", \"c\"]}\ndata = json_stream.load(f, persistent=True)\n# data is a streaming dict-like object \n# stream has been read up to \"{\"\n\n# use data like a dict\nresults = data[\"results\"] # results is a streaming list-like object\n# stream has been read up to \"[\"\n# count has been stored data\n\n# use results like a list\na_result = results[1] # a_result = \"b\"\n# stream has been read up to the middle of list\n# \"a\" and \"b\" have been stored in results\n\n# read earlier data from memory\ncount = data[\"count\"] # count = 1\n\n# consume rest of list\nresults.read_all()\n# stream has been read up to \"}\"\n# \"c\" is now stored in results too\n# results.is_streaming() == False\n\n# consume everything\ndata.read_all()\n# stream is now exhausted\n# data.is_streaming() == False\n```\n\nPersistent mode is not appropriate if you care about memory consumption, but\nprovides an identical experience compared to `json.load()`.\n\n#### Mixed mode\n\nIn some cases you will need to be able to randomly access some part of the \ndata, but still only have that specific data taking up memory resources.\n\nFor example, you might have a very long list of objects, but you cannot always \naccess the keys of the objects in stream order. You want to be able to iterate\nthe list transiently, but access the result objects persistently.\n\nThis can be achieved using the `persistent()` method of all the `list` or\n`dict`-like objects json_stream produces. Calling `persistent()` causes the existing\ntransient object to produce persistent child objects.\n\nNote that the `persistent()` method makes the children of the object it\nis called on persistent, not the object it is called on.\n\n```python\nimport json_stream\n\n# JSON: {\"results\": [{\"x\": 1, \"y\": 3}, {\"y\": 4, \"x\": 2}]}\n# note that the keys of the inner objects are not ordered \ndata = json_stream.load(f) # data is a transient dict-like object \n\n# iterate transient list, but produce persistent items\nfor result in data['results'].persistent():\n # result is a persistent dict-like object\n print(result['x']) # print x\n print(result['y']) # print y (error on second result without .persistent())\n print(result['x']) # print x again (error without .persistent())\n```\n\nThe opposite is also possible, going from persistent mode to transient mode, though \nthe use cases for this are more esoteric.\n\n```python\n# JSON: {\"a\": 1, \"x\": [\"long\", \"list\", \"I\", \"don't\", \"want\", \"in\", \"memory\"], \"b\": 2}\ndata = load(StringIO(json), persistent=True).transient()\n# data is a persistent dict-list object that produces transient children\n\nprint(data[\"a\"]) # prints 1\nx = data[\"x\"] # x is a transient list, you can use it accordingly\nprint(x[0]) # prints long\n\n# access earlier data from memory\nprint(data[\"a\"]) # this would have raised an exception if data was transient\n\nprint(data[\"b\"]) # prints 2\n\n# we have now moved past all the data in the transient list\nprint(x[0]) # will raise exception\n```\n\n### <a id=\"visitor\"></a>visitor pattern\n\nYou can also parse using a visitor-style approach where a function you supply\nis called for each data item as it is parsed (depth-first).\n\nThis uses a transient parser under the hood, so does not consume memory for\nthe whole document.\n\n```python\nimport json_stream\n\n# JSON: {\"x\": 1, \"y\": {}, \"xxxx\": [1,2, {\"yyyy\": 1}, \"z\", 1, []]}\n\ndef visitor(item, path):\n print(f\"{item} at path {path}\")\n\njson_stream.visit(f, visitor)\n```\n\nOutput:\n```\n1 at path ('x',)\n{} at path ('y',)\n1 at path ('xxxx', 0)\n2 at path ('xxxx', 1)\n1 at path ('xxxx', 2, 'yyyy')\nz at path ('xxxx', 3)\n1 at path ('xxxx', 4)\n[] at path ('xxxx', 5)\n```\n\n### <a id=\"urls\"></a> Stream a URL\n\n`json_stream` knows how to stream directly from a URL using a variety of packages.\nSupported packages include:\n- Python's batteries-included [`urllib`](#urllib) package\n- The popular [`requests`](#requests) library\n- The newer [`httpx`](#httpx) library\n\n#### <a id=\"urllib\"></a> urllib\n\n[`urllib`](https://docs.python.org/3/library/urllib.html)'s response objects are already\nfile-like objects, so we can just pass them directly to `json-stream`.\n\n```python\nimport urllib.request\nimport json_stream\n\nwith urllib.request.urlopen('http://example.com/data.json') as response:\n data = json_stream.load(response)\n```\n\n#### <a id=\"requests\"></a>requests\n\nTo stream JSON data from [`requests`](https://requests.readthedocs.io/en/latest/), you must\npass `stream=True` when making a request, and call `json_stream.requests.load()` passing the response. \n\n```python\nimport requests\nimport json_stream.requests\n\nwith requests.get('http://example.com/data.json', stream=True) as response:\n data = json_stream.requests.load(response)\n```\n\n<a id=\"requests-chunk-size\"></a>\nNote: these functions use\n[`response.iter_content()`](https://requests.readthedocs.io/en/latest/api/#requests.Response.iter_content) under the\nhood with a `chunk_size` of 10k bytes. This default allows us to perform effective reads from the response stream and \nlower CPU usage. The drawback to this is that `requests` will buffer each read until up to 10k bytes have been read \nbefore passing the data back to `json_stream`. If you need to consume data more responsively the only option is to tune\n`chunk_size` back to 1 to disable buffering.\n\n#### <a id=\"httpx\"></a> httpx\n\nTo stream JSON data from [`httpx`](https://www.python-httpx.org/), you must call\n[`stream()`](https://www.python-httpx.org/quickstart/#streaming-responses) when\nmaking your request, and call `json_stream.httpx.load()` passing the response.\n\n```python\nimport httpx\nimport json_stream.httpx\n\nwith httpx.Client() as client, client.stream('GET', 'http://example.com/data.json') as response:\n data = json_stream.httpx.load(response)\n```\n\nUnder the hood, this works similarly to the [`requests`](#requests) version above, including \nthe caveat about [`chunk_size`](#requests-chunk-size).\n\n### Stream a URL (with visitor)\n\nThe visitor pattern also works with URL streams.\n\n#### urllib\n\n```python\nimport urllib.request\nimport json_stream\n\ndef visitor(item, path):\n print(f\"{item} at path {path}\")\n \nwith urllib.request.urlopen('http://example.com/data.json') as response:\n json_stream.visit(response, visitor)\n```\n\n#### requests\n\n```python\nimport requests\nimport json_stream.requests\n\ndef visitor(item, path):\n print(f\"{item} at path {path}\")\n \nwith requests.get('http://example.com/data.json', stream=True) as response:\n json_stream.requests.visit(response, visitor)\n```\n\nThe [`chunk_size`](#requests-chunk-size) note also applies to `visit()`.\n\n#### httpx\n\n```python\nimport httpx\nimport json_stream.httpx\n\ndef visitor(item, path):\n print(f\"{item} at path {path}\")\n \nwith httpx.Client() as client, client.stream('GET', 'http://example.com/data.json') as response:\n json_stream.httpx.visit(response, visitor)\n```\n\n### <a id=\"iterators\"></a> Stream an iterable\n\n`json-stream`'s parsing functions can take any iterable object that produces encoded JSON as\n`byte` objects.\n\n```python\nimport json_stream\n\ndef some_iterator():\n yield b'{\"some\":'\n yield b' \"JSON\"}'\n\ndata = json_stream.load(some_iterator())\nassert data['some'] == \"JSON\"\n```\n\nThis is actually how the [`requests`](#requests) and [`httpx`](#httpx) extensions work, as\nboth libraries provide methods to iterate over the response content.\n\n### <a id=\"encoding-json-stream-objects\"></a> Encoding json-stream objects\n\nYou can re-output (encode) _persistent_ json-stream `dict`-like and `list`-like object back to JSON using the built-in\n`json.dump()` or `json.dumps()` functions, but with a little additional work:\n\n```python\nimport json\n\nimport json_stream\nfrom json_stream.dump import JSONStreamEncoder, default\n\ndata = json_stream.load(f, persistent=True)\n\n# Option 1: supply json_stream.encoding.default as the default argument\nprint(json.dumps(data, default=default))\n\n# Option 2: supply json_stream.encoding.JSONStreamEncoder as the cls argument\n# This allows you to create your own subclass to further customise encoding\nprint(json.dumps(data, cls=JSONStreamEncoder))\n```\n\nIf you are using a library that internally takes data you pass it and encodes\nit using `json.dump()`. You can also use JSONStreamEncoder() as a context manager.\n\nIt works by monkey-patching the built-in `JSONEncoder.default` method during the\nscope of the `with` statement.\n\n```python \n# library code\ndef some_library_function_out_of_your_control(arg):\n json.dumps(arg)\n\n# your code\nwith JSONStreamEncoder():\n some_library_function_out_of_your_control(data)\n```\n\n### Converting to standard Python types\n\nTo convert a json-stream `dict`-like or `list`-like object and all its\ndescendants to a standard `list` and `dict`, you can use the\n`json_stream.to_standard_types` utility:\n\n```python\n# JSON: {\"round\": 1, \"results\": [1, 2, 3]}\ndata = json_stream.load(f)\nresults = data[\"results\"]\nprint(results) # prints <TransientStreamingJSONList: TRANSIENT, STREAMING>\nconverted = json_stream.to_standard_types(results)\nprint(converted) # prints [1, 2, 3]\n```\n\n#### Thread safety (experimental)\n\nThere is also a thread-safe version of the `json.dump` context manager:\n\n```python\nfrom json_stream.dump.threading import ThreadSafeJSONStreamEncoder\n\n# your code\nwith ThreadSafeJSONStreamEncoder():\n some_library_function_out_of_your_control(data)\n```\n\nThe thread-safe implementation will ensure that concurrent uses of the \ncontext manager will only apply the patch for the first thread entering\nthe patched section(s) and will only remove the patch when the last\nthread exits the patched sections(s)\n\nAdditionally, if the patch is somehow called by a thread that is _not_\ncurrently in a patched section (i.e. some other thread calling \n`json.dump`) then that thread will block until the patch has been\nremoved. While such an un-patched thread is active, any thread attempting\nto apply the patch is blocked.\n\n### <a id=\"rust-tokenizer\"></a> Rust tokenizer speedups\n\nBy default `json-stream` uses the \n[`json-stream-rs-tokenizer`](https://pypi.org/project/json-stream-rs-tokenizer/)\nnative extension.\n\nThis is a 3rd party Rust-based tokenizer implementations that provides\nsignificant parsing speedup compared to pure python implementation.\n\n`json-stream` will fallback to its pure python tokenizer implementation\nif `json-stream-rs-tokenizer` is not available.\n\n### Custom tokenizer\n\nYou can supply an alternative JSON tokenizer implementation. Simply pass \na tokenizer to the `load()` or `visit()` methods.\n\n```python\njson_stream.load(f, tokenizer=some_tokenizer)\n```\n\nThe requests methods also accept a customer tokenizer parameter.\n\n\n# Writing\n\nThe standard library's `json.dump()` function can only accept standard\npython types such as `dict`, `list`, `str`.\n\n`json-stream` allows you to write streaming JSON output based on python\ngenerators instead.\n\nFor actually encoding and writing to the stream, `json-stream` \nstill uses the standard library's `json.dump()` function, but provides\nwrappers that adapt python generators into `dict`/`list` subclasses \nthat `json.dump()` can use.\n\nThe means that you do not have to generate all of your data upfront\nbefore calling `json.dump()`.\n\n## Usage\n\nTo use `json-stream` to generate JSON data iteratively, you must first \nwrite python generators (or use any other iterable).\n\nTo output JSON objects, the iterable must yield key/value pairs.\n\nTo output JSON lists, the iterable must yield individual items.\n\nThe values yielded can be either be standard python types or another other\n`Streamable` object, allowing lists and object to be arbitrarily nested.\n\n`streamable_list`/`streamable_dict` can be used to wrap an existing\niterable:\n```python\nimport sys\nimport json\n\nfrom json_stream import streamable_list\n\n# wrap existing iterable\ndata = streamable_list(range(10))\n\n# consume iterable with standard json.dump()\njson.dump(data, sys.stdout)\n```\n\nOr they can be used as decorators on generator functions:\n```python\nimport json\nimport sys\n\nfrom json_stream import streamable_dict\n\n# declare a new streamable dict generator function\n@streamable_dict\ndef generate_dict_of_squares(n):\n for i in range(n):\n # this could be some memory intensive operation\n # or just a really large value of n\n yield i, i ** 2\n\n# data is will already be Streamable because\n# of the decorator\ndata = generate_dict_of_squares(10)\njson.dump(data, sys.stdout)\n```\n\n## Example\n\nThe following example generates a JSON object with a nested JSON list.\nIt uses `time.sleep()` to slow down the generation and show that the\noutput is indeed written as the data is created.\n\n```python\nimport sys\nimport json\nimport time\n\nfrom json_stream.writer import streamable_dict, streamable_list\n\n\n# define a list data generator that (slowly) yields \n# items that will be written as a JSON list\n@streamable_list\ndef generate_list(n):\n # output n numbers and their squares\n for i in range(n): # range is itself a generator\n yield i\n time.sleep(1)\n\n\n# define a dictionary data generator that (slowly) yields \n# key/value pairs that will be written as a JSON dict\n@streamable_dict\ndef generate_dict(n):\n # output n numbers and their squares\n for i in range(n): # range is itself a generator\n yield i, i ** 2\n time.sleep(1)\n\n # yield another dictionary item key, with the value\n # being a streamed nested list\n yield \"a list\", generate_list(n)\n\n\n# get a streamable generator\ndata = generate_dict(5)\n\n# use json.dump() to write dict generator to stdout\njson.dump(data, sys.stdout, indent=2)\n\n# if you already have an iterable object, you can just\n# call streamable_* on it to make it writable\ndata = streamable_list(range(10))\njson.dump(data, sys.stdout)\n\n```\n\nOutput:\n```json\n{\n \"0\": 0,\n \"1\": 1,\n \"2\": 4,\n \"3\": 9,\n \"4\": 16,\n \"a list\": [\n 0,\n 1,\n 2,\n 3,\n 4\n ]\n}\n```\n\n# <a id=\"standard-json-problems\"></a> What are the problems with the standard `json` package?\n\n## Reading with `json.load()`\nThe problem with the `json.load()` stem from the fact that it must read\nthe whole JSON document into memory before parsing it.\n\n### Memory usage\n\n`json.load()` first reads the whole document into memory as a string. It\nthen starts parsing that string and converting the whole document into python\ntypes again stored in memory. For a very large document, this could be more\nmemory than you have available to your system.\n\n`json_stream.load()` does not read the whole document into memory, it only\nbuffers enough from the stream to produce the next item of data.\n\nAdditionally, in the default transient mode (see below) `json-stream` doesn't store \nup all of the parsed data in memory.\n\n### Latency\n\n`json.load()` produces all the data after parsing the whole document. If you\nonly care about the first 10 items in a list of 2 million items, then you\nhave wait until all 2 million items have been parsed first.\n\n`json_stream.load()` produces data as soon as it is available in the stream.\n\n## <a id=\"writing\"></a> Writing\n\n### Memory usage\n\nWhile `json.dump()` does iteratively write JSON data to the given\nfile-like object, you must first produce the entire document to be \nwritten as standard python types (`dict`, `list`, etc). For a very\nlarge document, this could be more memory than you have available \nto your system.\n\n`json-stream` allows you iteratively generate your data one item at\na time, and thus consumes only the memory required to generate that\none item.\n\n### Latency\n\n`json.dump()` can only start writing to the output file once all the\ndata has been generated up front at standard python types.\n\nThe iterative generation of JSON items provided by `json-stream`\nallows the data to be written as it is produced.\n\n# Future improvements\n\n* Allow long strings in the JSON to be read as streams themselves\n* Allow transient mode on seekable streams to seek to data earlier in\nthe stream instead of raising a `TransientAccessException`\n* A more efficient tokenizer?\n\n# Alternatives\n\n## NAYA\n\n[NAYA](https://github.com/danielyule/naya) is a pure python JSON parser for\nparsing a simple JSON list as a stream.\n\n### Why not NAYA?\n\n* It can only stream JSON containing a top-level list \n* It does not provide a pythonic `dict`/`list`-like interface \n\n## Yajl-Py\n\n[Yajl-Py](https://pykler.github.io/yajl-py/) is a wrapper around the C YAJL JSON library that can be used to \ngenerate SAX style events while parsing JSON.\n\n### Why not Yajl-Py?\n\n* No pure python implementation\n* It does not provide a pythonic `dict`/`list`-like interface \n\n## jsonslicer\n\n[jsonslicer](https://github.com/AMDmi3/jsonslicer) is another wrapper around the YAJL C library with a\npath lookup based interface.\n\n### Why not jsonslicer?\n\n* No pure python implementation\n* It does not provide a pythonic `dict`/`list`-like interface\n* Must know all data paths lookup in advance (or make multiple passes)\n\n# Contributing\n\nSee the project [contribution guide](https://github.com/daggaz/json-stream/blob/master/CONTRIBUTING.md).\n\n# Donations\n\n[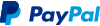](https://paypal.me/JCockburn307?country.x=GB&locale.x=en_GB)\n\nOR\n\n[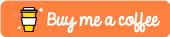](https://www.buymeacoffee.com/daggaz)\n\n# Acknowledgements\n\nThe JSON tokenizer used in the project was taken from the\n[NAYA](https://github.com/danielyule/naya) project.\n",
"bugtrack_url": null,
"license": "Copyright (c) 2020 Jamie Cockburn Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Streaming JSON encoder and decoder",
"version": "2.3.2",
"project_urls": {
"Funding": "https://www.buymeacoffee.com/daggaz",
"Homepage": "https://github.com/daggaz/json-stream",
"Repository": "https://github.com/daggaz/json-stream",
"Tracker": "https://github.com/daggaz/json-stream/issues"
},
"split_keywords": [
"json",
"stream",
"decoder",
"encoder",
"parsing"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "da11861be54b293856d054a1129202d6393801190b5fd6c599c70a47d4c174b2",
"md5": "5510a35b1f352abf65eaeacc3bb4c29c",
"sha256": "236b8e08e2761b209816452a3527355e757913d833e6802b68a034a13b8bd3ac"
},
"downloads": -1,
"filename": "json_stream-2.3.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "5510a35b1f352abf65eaeacc3bb4c29c",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4,>=3.5",
"size": 30687,
"upload_time": "2023-06-30T09:43:14",
"upload_time_iso_8601": "2023-06-30T09:43:14.708508Z",
"url": "https://files.pythonhosted.org/packages/da/11/861be54b293856d054a1129202d6393801190b5fd6c599c70a47d4c174b2/json_stream-2.3.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6a0c8d654a510a43e4a5ec8a8ac523a1d225daa81afaccd69e974577c9cfb18b",
"md5": "71cbb1197380c6a16591cf5dc587f394",
"sha256": "b8b450ea8e8e3c239e9e7e38d12fed934e77a353c14b297f8ee345a5ceb25b91"
},
"downloads": -1,
"filename": "json-stream-2.3.2.tar.gz",
"has_sig": false,
"md5_digest": "71cbb1197380c6a16591cf5dc587f394",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4,>=3.5",
"size": 31485,
"upload_time": "2023-06-30T09:43:16",
"upload_time_iso_8601": "2023-06-30T09:43:16.684516Z",
"url": "https://files.pythonhosted.org/packages/6a/0c/8d654a510a43e4a5ec8a8ac523a1d225daa81afaccd69e974577c9cfb18b/json-stream-2.3.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-06-30 09:43:16",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "daggaz",
"github_project": "json-stream",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "json-stream"
}