# k2000
[](https://github.com/psobot/k2000/actions/workflows/test.yaml)
A Python package for communicating with the Kurzweil K2000/K2500/K2600 family of synthesizers over MIDI.
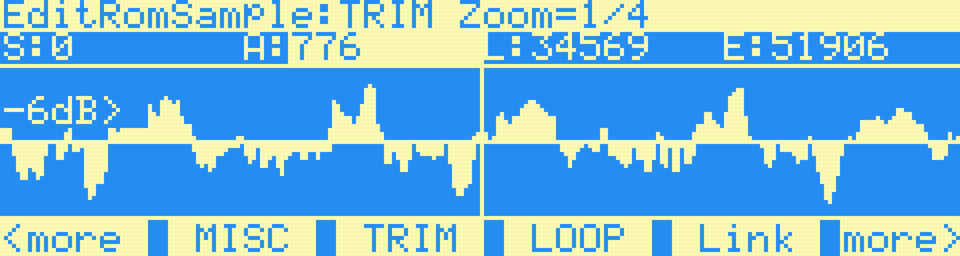
To use, just:
```
pip install k2000
```
Make sure you're on Python 3.8, 3.9, or 3.10. (Other versions may work too.)
### _What?_
Back in the 1990s, [Kurzweil Music Systems](https://en.wikipedia.org/wiki/Kurzweil_Music_Systems) - founded by Raymond Kurzweil and Stevie Wonder - released an extremely advanced line of synthesizers known as [the _K2_ series](https://en.wikipedia.org/wiki/Kurzweil_Music_Systems#K2xxx_synthesizers). Compared to the music technology we have today in the mid-2020s, these synthesizers were... actually not bad. They still hold up today.
This library contains code for communicating with an attached K2-series synthesizer (specifically the **K2000, K2500, or K2600**) via MIDI, implementing its entire SysEx protocol, allowing for full interface control and full object read and write support.
### _How?_
A quick-start example:
```python
from k2000.client import K2500Client
c = K2500Client("My MIDI Interface Name")
assert c.is_connected
print(c.get_screen_text())
# ProgramMode Xpose:0ST <>Channel:1
# 998 Choral Sleigh
# KeyMap Info 999 Pad Nine
# Grand Piano 1 Acoustic Piano
# Syn Piano 2 Stage Piano
# 3 BriteGrand
# 4 ClassicPiano&Vox
# Octav- Octav+ Panic Sample Chan- Chan+
# Navigate around the UI a bit:
c.up()
c.down()
c.number(125)
c.enter()
# Access object data:
name, program_data = c.programs[125]
print(f"Got {len(program_data):,} bytes of program data for Program \"{name}\".")
# prints: Got 586 bytes of program data for Program "Fast Solo Tenor".
# Dump all effect data, for example:
for i, value in c.effects.items():
if value is None:
continue
effect_name, effect_data = value
do_something_with(effect_name, effect_data)
# Take screenshots!
image = c.screenshot()
image.save("screenshot.png")
# Which gives...
```
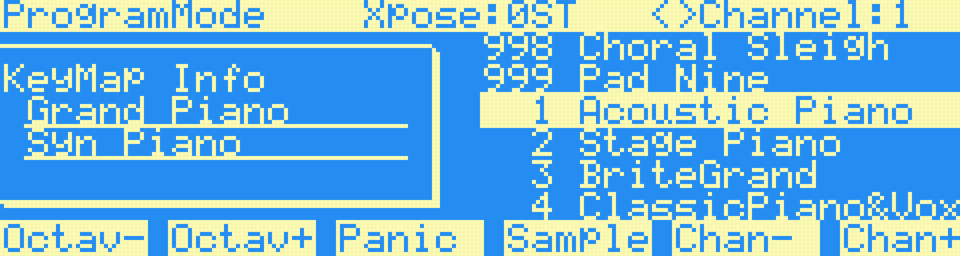
### _Why?_
I was doing some reverse engineering and this library helped make that reverse engineering easier.
More generally, though; you could use this library if you wanted to:
- Load or dump programs (or setups, or effects, etc) from your K2-series synth via Python.
- Control the interface of your K2-series synth (i.e. push buttons, read the text and graphics) over MIDI
- Take screenshots of your K2's display over MIDI
- Automate the control of your K2 for scraping, testing, etc.
Ironically, this library doesn't support sending MIDI notes; just the SysEx commands to control specific functions of the K2.
### License
```
MIT License
Copyright (c) 2019-2023 Peter Sobot
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
```
Raw data
{
"_id": null,
"home_page": "https://github.com/psobot/k2000",
"name": "k2000",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "kurzweil midi synthesizer k2000 k2vx k2500 k2600 sysex",
"author": "Peter Sobot",
"author_email": "github@petersobot.com",
"download_url": "https://files.pythonhosted.org/packages/bc/ae/400acdeca04266174c9e8bcb84fe8a3af9371dca49a6f8e1e58daceee5bd/k2000-0.1.2.tar.gz",
"platform": null,
"description": "# k2000\n\n[](https://github.com/psobot/k2000/actions/workflows/test.yaml)\n\nA Python package for communicating with the Kurzweil K2000/K2500/K2600 family of synthesizers over MIDI.\n\n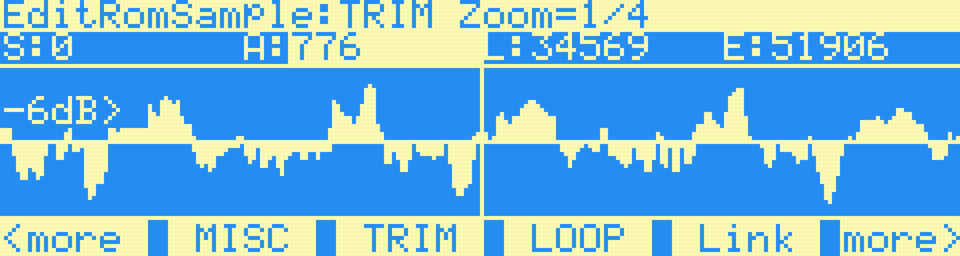\n\n\nTo use, just:\n```\npip install k2000\n```\n\nMake sure you're on Python 3.8, 3.9, or 3.10. (Other versions may work too.)\n\n### _What?_\n\nBack in the 1990s, [Kurzweil Music Systems](https://en.wikipedia.org/wiki/Kurzweil_Music_Systems) - founded by Raymond Kurzweil and Stevie Wonder - released an extremely advanced line of synthesizers known as [the _K2_ series](https://en.wikipedia.org/wiki/Kurzweil_Music_Systems#K2xxx_synthesizers). Compared to the music technology we have today in the mid-2020s, these synthesizers were... actually not bad. They still hold up today.\n\nThis library contains code for communicating with an attached K2-series synthesizer (specifically the **K2000, K2500, or K2600**) via MIDI, implementing its entire SysEx protocol, allowing for full interface control and full object read and write support.\n\n### _How?_\n\nA quick-start example:\n```python\nfrom k2000.client import K2500Client\n\nc = K2500Client(\"My MIDI Interface Name\")\nassert c.is_connected\n\nprint(c.get_screen_text())\n# ProgramMode Xpose:0ST <>Channel:1 \n# 998 Choral Sleigh \n# KeyMap Info 999 Pad Nine \n# Grand Piano 1 Acoustic Piano \n# Syn Piano 2 Stage Piano \n# 3 BriteGrand \n# 4 ClassicPiano&Vox\n# Octav- Octav+ Panic Sample Chan- Chan+\n\n# Navigate around the UI a bit:\nc.up()\nc.down()\nc.number(125)\nc.enter()\n\n# Access object data:\nname, program_data = c.programs[125]\nprint(f\"Got {len(program_data):,} bytes of program data for Program \\\"{name}\\\".\")\n# prints: Got 586 bytes of program data for Program \"Fast Solo Tenor\".\n\n# Dump all effect data, for example:\nfor i, value in c.effects.items():\n if value is None:\n continue\n effect_name, effect_data = value\n do_something_with(effect_name, effect_data)\n\n# Take screenshots!\nimage = c.screenshot()\nimage.save(\"screenshot.png\")\n# Which gives...\n```\n\n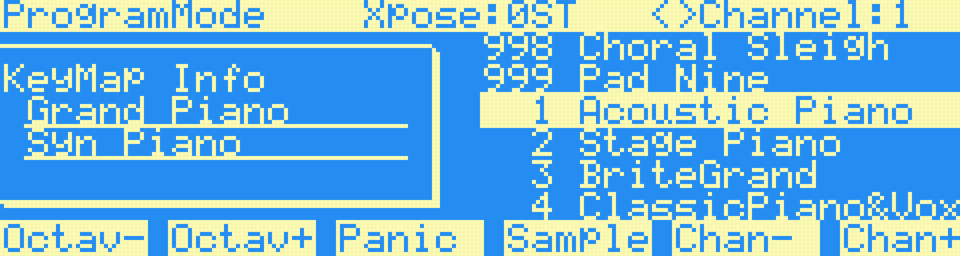\n\n### _Why?_\n\nI was doing some reverse engineering and this library helped make that reverse engineering easier.\n\nMore generally, though; you could use this library if you wanted to:\n - Load or dump programs (or setups, or effects, etc) from your K2-series synth via Python.\n - Control the interface of your K2-series synth (i.e. push buttons, read the text and graphics) over MIDI\n - Take screenshots of your K2's display over MIDI\n - Automate the control of your K2 for scraping, testing, etc.\n\nIronically, this library doesn't support sending MIDI notes; just the SysEx commands to control specific functions of the K2.\n\n### License\n\n```\nMIT License\n\nCopyright (c) 2019-2023 Peter Sobot\n\nPermission is hereby granted, free of charge, to any person obtaining a copy\nof this software and associated documentation files (the \"Software\"), to deal\nin the Software without restriction, including without limitation the rights\nto use, copy, modify, merge, publish, distribute, sublicense, and/or sell\ncopies of the Software, and to permit persons to whom the Software is\nfurnished to do so, subject to the following conditions:\n\nThe above copyright notice and this permission notice shall be included in all\ncopies or substantial portions of the Software.\n\nTHE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR\nIMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,\nFITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE\nAUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER\nLIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,\nOUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE\nSOFTWARE.\n```\n",
"bugtrack_url": null,
"license": "",
"summary": "A library for working with the Kurzweil K2000/K2500/K2600 series of synthesizers.",
"version": "0.1.2",
"split_keywords": [
"kurzweil",
"midi",
"synthesizer",
"k2000",
"k2vx",
"k2500",
"k2600",
"sysex"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "34cafa1dec8830466fbd18b8a9960f36",
"sha256": "36f973834be189361fc4a3fb2cb78f6f1e4746e20a7f38d6e304d3ab563011c2"
},
"downloads": -1,
"filename": "k2000-0.1.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "34cafa1dec8830466fbd18b8a9960f36",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 19706,
"upload_time": "2022-12-26T18:35:21",
"upload_time_iso_8601": "2022-12-26T18:35:21.381482Z",
"url": "https://files.pythonhosted.org/packages/32/0d/561a111df348480570991576223982d7f20c02849ba1704076cd36411649/k2000-0.1.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "e580051c534c189d8b9360afc7e5f7d0",
"sha256": "3c15bcb38bc2149103c1ad0d8195becaeed264510e1ec4f377e08d898beaf4a0"
},
"downloads": -1,
"filename": "k2000-0.1.2.tar.gz",
"has_sig": false,
"md5_digest": "e580051c534c189d8b9360afc7e5f7d0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 20010,
"upload_time": "2022-12-26T18:35:22",
"upload_time_iso_8601": "2022-12-26T18:35:22.696302Z",
"url": "https://files.pythonhosted.org/packages/bc/ae/400acdeca04266174c9e8bcb84fe8a3af9371dca49a6f8e1e58daceee5bd/k2000-0.1.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-12-26 18:35:22",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "psobot",
"github_project": "k2000",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "k2000"
}