# kornia-rs: low level computer vision library in Rust

[](https://badge.fury.io/py/kornia-rs)
[](https://docs.rs/kornia-rs)
[](LICENCE)
[](https://join.slack.com/t/kornia/shared_invite/zt-csobk21g-CnydWe5fmvkcktIeRFGCEQ)
The `kornia-rs` crate is a low level library for Computer Vision written in [Rust](https://www.rust-lang.org/) π¦
Use the library to perform image I/O, visualisation and other low level operations in your machine learning and data-science projects in a thread-safe and efficient way.
## Getting Started
`cargo run --bin hello_world -- --image-path path/to/image.jpg`
```rust
use kornia::image::Image;
use kornia::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
// read the image
let image: Image<u8, 3> = F::read_image_any("tests/data/dog.jpeg")?;
println!("Hello, world! π¦");
println!("Loaded Image size: {:?}", image.size());
println!("\nGoodbyte!");
Ok(())
}
```
```bash
Hello, world! π¦
Loaded Image size: ImageSize { width: 258, height: 195 }
Goodbyte!
```
## Features
- π¦The library is primarly written in [Rust](https://www.rust-lang.org/).
- π Multi-threaded and efficient image I/O, image processing and advanced computer vision operators.
- π’ The n-dimensional backend is based on the [`ndarray`](https://crates.io/crates/ndarray) crate.
- π Python bindings are created with [PyO3/Maturin](https://github.com/PyO3/maturin).
- π¦ We package with support for Linux [amd64/arm64], Macos and WIndows.
- Supported Python versions are 3.7/3.8/3.9/3.10/3.11
### Supported image formats
- Read images from AVIF, BMP, DDS, Farbeld, GIF, HDR, ICO, JPEG (libjpeg-turbo), OpenEXR, PNG, PNM, TGA, TIFF, WebP.
### Image processing
- Convert images to grayscale, resize, crop, rotate, flip, pad, normalize, denormalize, and other image processing operations.
### Video processing
- Capture video frames from a camera.
## π οΈ Installation
### >_ System dependencies
Dependeing on the features you want to use, you might need to install the following dependencies in your system:
#### turbojpeg
```bash
sudo apt-get install nasm
```
#### gstreamer
```bash
sudo apt-get install libgstreamer1.0-dev libgstreamer-plugins-base1.0-dev
```
** Check the gstreamr installation guide: <https://docs.rs/gstreamer/latest/gstreamer/#installation>
### π¦ Rust
Add the following to your `Cargo.toml`:
```toml
[dependencies]
kornia = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
```
Alternatively, you can use each sub-crate separately:
```toml
[dependencies]
kornia-core = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-io = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-image = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
kornia-imgproc = { git = "https://github.com/kornia/kornia-rs", tag = "v0.1.6-rc1" }
```
### π Python
```bash
pip install kornia-rs
```
## Examples: Image processing
The following example shows how to read an image, convert it to grayscale and resize it. The image is then logged to a [`rerun`](https://github.com/rerun-io/rerun) recording stream.
Checkout all the examples in the [`examples`](https://github.com/kornia/kornia-rs/tree/main/examples) directory to see more use cases.
```rust
use kornia::{image::{Image, ImageSize}, imgproc};
use kornia::io::functional as F;
fn main() -> Result<(), Box<dyn std::error::Error>> {
// read the image
let image: Image<u8, 3> = F::read_image_any("tests/data/dog.jpeg")?;
let image_viz = image.clone();
let image_f32: Image<f32, 3> = image.cast_and_scale::<f32>(1.0 / 255.0)?;
// convert the image to grayscale
let mut gray = Image::<f32, 1>::from_size_val(image_f32.size(), 0.0)?;
imgproc::color::gray_from_rgb(&image_f32, &mut gray)?;
// resize the image
let new_size = ImageSize {
width: 128,
height: 128,
};
let mut gray_resized = Image::<f32, 1>::from_size_val(new_size, 0.0)?;
imgproc::resize::resize_native(
&gray, &mut gray_resized,
imgproc::resize::InterpolationMode::Bilinear,
)?;
println!("gray_resize: {:?}", gray_resized.size());
// create a Rerun recording stream
let rec = rerun::RecordingStreamBuilder::new("Kornia App").connect()?;
// log the images
let _ = rec.log("image", &rerun::Image::try_from(image_viz.data)?);
let _ = rec.log("gray", &rerun::Image::try_from(gray.data)?);
let _ = rec.log("gray_resize", &rerun::Image::try_from(gray_resized.data)?);
Ok(())
}
```
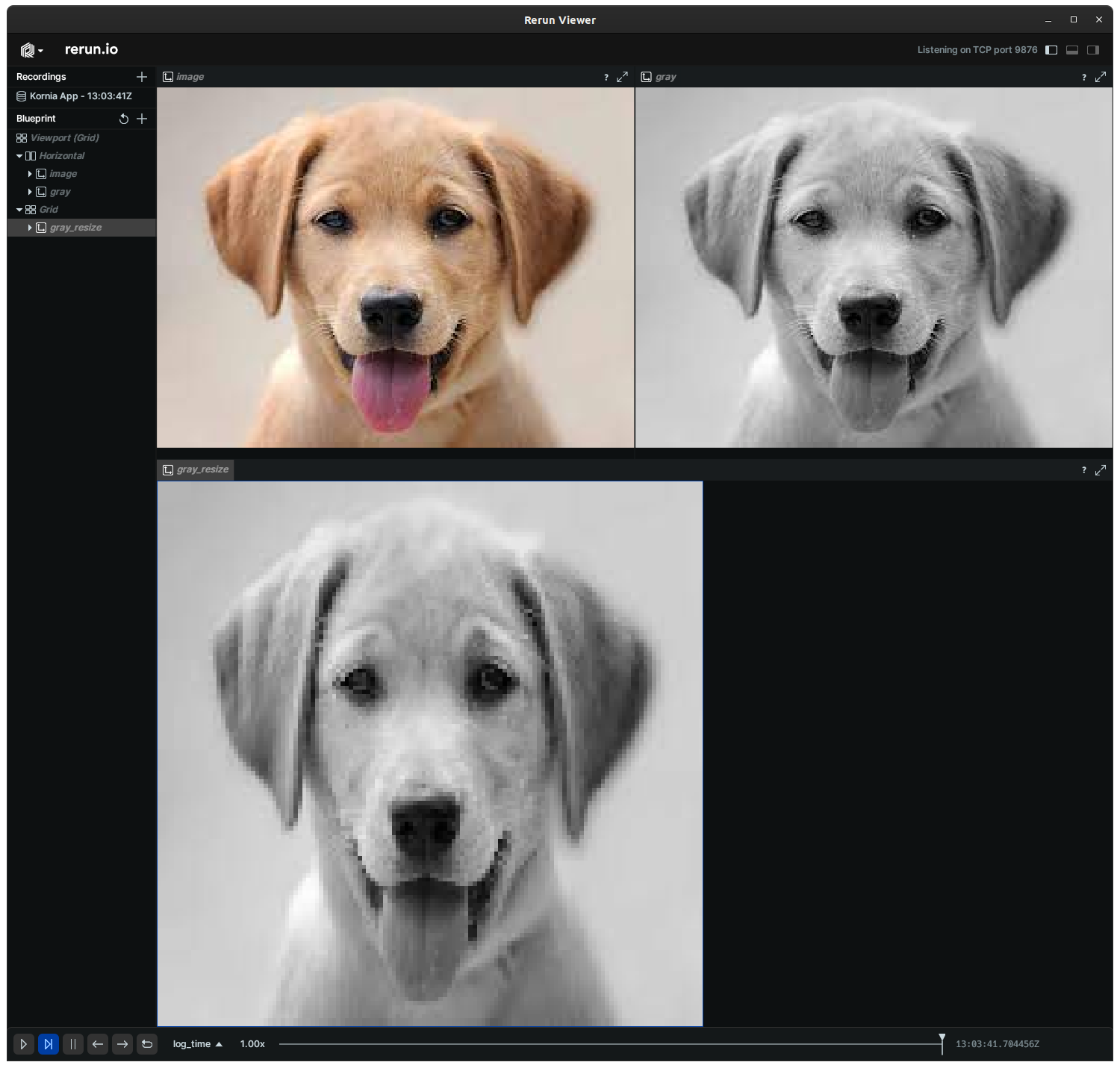
## Python usage
Load an image, that is converted directly to a numpy array to ease the integration with other libraries.
```python
import kornia_rs as K
import numpy as np
# load an image with using libjpeg-turbo
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
# alternatively, load other formats
# img: np.ndarray = K.read_image_any("dog.png")
assert img.shape == (195, 258, 3)
# convert to dlpack to import to torch
img_t = torch.from_dlpack(img)
assert img_t.shape == (195, 258, 3)
```
Write an image to disk
```python
import kornia_rs as K
import numpy as np
# load an image with using libjpeg-turbo
img: np.ndarray = K.read_image_jpeg("dog.jpeg")
# write the image to disk
K.write_image_jpeg("dog_copy.jpeg", img)
```
Encode or decode image streams using the `turbojpeg` backend
```python
import kornia_rs as K
# load image with kornia-rs
img = K.read_image_jpeg("dog.jpeg")
# encode the image with jpeg
image_encoder = K.ImageEncoder()
image_encoder.set_quality(95) # set the encoding quality
# get the encoded stream
img_encoded: list[int] = image_encoder.encode(img)
# decode back the image
image_decoder = K.ImageDecoder()
decoded_img: np.ndarray = image_decoder.decode(bytes(image_encoded))
```
Resize an image using the `kornia-rs` backend with SIMD acceleration
```python
import kornia_rs as K
# load image with kornia-rs
img = K.read_image_jpeg("dog.jpeg")
# resize the image
resized_img = K.resize(img, (128, 128), interpolation="bilinear")
assert resized_img.shape == (128, 128, 3)
```
## π§βπ» Development
Pre-requisites: install `rust` and `python3` in your system.
```bash
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
```
Clone the repository in your local directory
```bash
git clone https://github.com/kornia/kornia-rs.git
```
### π¦ Rust
Compile the project and run the tests
```bash
cargo test
```
For specific tests, you can run the following command:
```bash
cargo test image
```
### π Python
To build the Python wheels, we use the `maturin` package. Use the following command to build the wheels:
```bash
make build-python
```
To run the tests, use the following command:
```bash
make test-python
```
## π Contributing
This is a child project of [Kornia](https://github.com/kornia/kornia). Join the community to get in touch with us, or just sponsor the project: <https://opencollective.com/kornia>
Raw data
{
"_id": null,
"home_page": "http://kornia.org",
"name": "kornia-rs",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Edgar Riba <edgar@kornia.org>",
"keywords": "computer vision, rust",
"author": null,
"author_email": "Edgar Riba <edgar@kornia.org>",
"download_url": "https://files.pythonhosted.org/packages/07/f3/c526610210887108c43a0bb1560964176001a25fc72eba7d084394ef5c7a/kornia_rs-0.1.7.tar.gz",
"platform": null,
"description": "# kornia-rs: low level computer vision library in Rust\n\n\n[](https://badge.fury.io/py/kornia-rs)\n[](https://docs.rs/kornia-rs)\n[](LICENCE)\n[](https://join.slack.com/t/kornia/shared_invite/zt-csobk21g-CnydWe5fmvkcktIeRFGCEQ)\n\nThe `kornia-rs` crate is a low level library for Computer Vision written in [Rust](https://www.rust-lang.org/) \ud83e\udd80\n\nUse the library to perform image I/O, visualisation and other low level operations in your machine learning and data-science projects in a thread-safe and efficient way.\n\n## Getting Started\n\n`cargo run --bin hello_world -- --image-path path/to/image.jpg`\n\n```rust\nuse kornia::image::Image;\nuse kornia::io::functional as F;\n\nfn main() -> Result<(), Box<dyn std::error::Error>> {\n // read the image\n let image: Image<u8, 3> = F::read_image_any(\"tests/data/dog.jpeg\")?;\n\n println!(\"Hello, world! \ud83e\udd80\");\n println!(\"Loaded Image size: {:?}\", image.size());\n println!(\"\\nGoodbyte!\");\n\n Ok(())\n}\n```\n\n```bash\nHello, world! \ud83e\udd80\nLoaded Image size: ImageSize { width: 258, height: 195 }\n\nGoodbyte!\n```\n\n## Features\n\n- \ud83e\udd80The library is primarly written in [Rust](https://www.rust-lang.org/).\n- \ud83d\ude80 Multi-threaded and efficient image I/O, image processing and advanced computer vision operators.\n- \ud83d\udd22 The n-dimensional backend is based on the [`ndarray`](https://crates.io/crates/ndarray) crate.\n- \ud83d\udc0d Python bindings are created with [PyO3/Maturin](https://github.com/PyO3/maturin).\n- \ud83d\udce6 We package with support for Linux [amd64/arm64], Macos and WIndows.\n- Supported Python versions are 3.7/3.8/3.9/3.10/3.11\n\n### Supported image formats\n\n- Read images from AVIF, BMP, DDS, Farbeld, GIF, HDR, ICO, JPEG (libjpeg-turbo), OpenEXR, PNG, PNM, TGA, TIFF, WebP.\n\n### Image processing\n\n- Convert images to grayscale, resize, crop, rotate, flip, pad, normalize, denormalize, and other image processing operations.\n\n### Video processing\n\n- Capture video frames from a camera.\n\n## \ud83d\udee0\ufe0f Installation\n\n### >_ System dependencies\n\nDependeing on the features you want to use, you might need to install the following dependencies in your system:\n\n#### turbojpeg\n\n```bash\nsudo apt-get install nasm\n```\n\n#### gstreamer\n\n```bash\nsudo apt-get install libgstreamer1.0-dev libgstreamer-plugins-base1.0-dev\n```\n\n** Check the gstreamr installation guide: <https://docs.rs/gstreamer/latest/gstreamer/#installation>\n\n### \ud83e\udd80 Rust\n\nAdd the following to your `Cargo.toml`:\n\n```toml\n[dependencies]\nkornia = { git = \"https://github.com/kornia/kornia-rs\", tag = \"v0.1.6-rc1\" }\n```\n\nAlternatively, you can use each sub-crate separately:\n\n```toml\n[dependencies]\nkornia-core = { git = \"https://github.com/kornia/kornia-rs\", tag = \"v0.1.6-rc1\" }\nkornia-io = { git = \"https://github.com/kornia/kornia-rs\", tag = \"v0.1.6-rc1\" }\nkornia-image = { git = \"https://github.com/kornia/kornia-rs\", tag = \"v0.1.6-rc1\" }\nkornia-imgproc = { git = \"https://github.com/kornia/kornia-rs\", tag = \"v0.1.6-rc1\" }\n```\n\n### \ud83d\udc0d Python\n\n```bash\npip install kornia-rs\n```\n\n## Examples: Image processing\n\nThe following example shows how to read an image, convert it to grayscale and resize it. The image is then logged to a [`rerun`](https://github.com/rerun-io/rerun) recording stream.\n\nCheckout all the examples in the [`examples`](https://github.com/kornia/kornia-rs/tree/main/examples) directory to see more use cases.\n\n```rust\nuse kornia::{image::{Image, ImageSize}, imgproc};\nuse kornia::io::functional as F;\n\nfn main() -> Result<(), Box<dyn std::error::Error>> {\n // read the image\n let image: Image<u8, 3> = F::read_image_any(\"tests/data/dog.jpeg\")?;\n let image_viz = image.clone();\n\n let image_f32: Image<f32, 3> = image.cast_and_scale::<f32>(1.0 / 255.0)?;\n\n // convert the image to grayscale\n let mut gray = Image::<f32, 1>::from_size_val(image_f32.size(), 0.0)?;\n imgproc::color::gray_from_rgb(&image_f32, &mut gray)?;\n\n // resize the image\n let new_size = ImageSize {\n width: 128,\n height: 128,\n };\n\n let mut gray_resized = Image::<f32, 1>::from_size_val(new_size, 0.0)?;\n imgproc::resize::resize_native(\n &gray, &mut gray_resized,\n imgproc::resize::InterpolationMode::Bilinear,\n )?;\n\n println!(\"gray_resize: {:?}\", gray_resized.size());\n\n // create a Rerun recording stream\n let rec = rerun::RecordingStreamBuilder::new(\"Kornia App\").connect()?;\n\n // log the images\n let _ = rec.log(\"image\", &rerun::Image::try_from(image_viz.data)?);\n let _ = rec.log(\"gray\", &rerun::Image::try_from(gray.data)?);\n let _ = rec.log(\"gray_resize\", &rerun::Image::try_from(gray_resized.data)?);\n\n Ok(())\n}\n```\n\n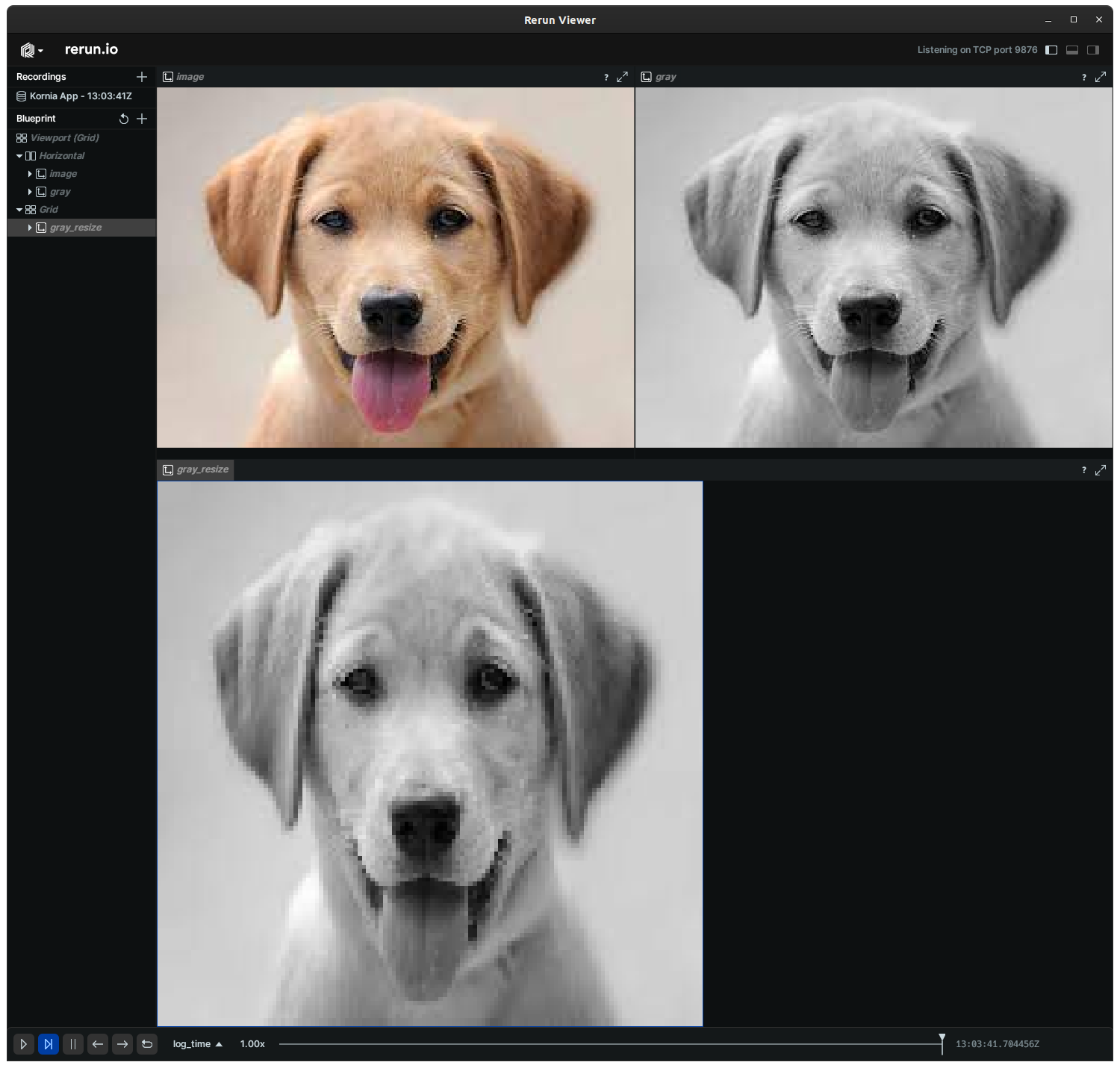\n\n## Python usage\n\nLoad an image, that is converted directly to a numpy array to ease the integration with other libraries.\n\n```python\n import kornia_rs as K\n import numpy as np\n\n # load an image with using libjpeg-turbo\n img: np.ndarray = K.read_image_jpeg(\"dog.jpeg\")\n\n # alternatively, load other formats\n # img: np.ndarray = K.read_image_any(\"dog.png\")\n\n assert img.shape == (195, 258, 3)\n\n # convert to dlpack to import to torch\n img_t = torch.from_dlpack(img)\n assert img_t.shape == (195, 258, 3)\n```\n\nWrite an image to disk\n\n```python\n import kornia_rs as K\n import numpy as np\n\n # load an image with using libjpeg-turbo\n img: np.ndarray = K.read_image_jpeg(\"dog.jpeg\")\n\n # write the image to disk\n K.write_image_jpeg(\"dog_copy.jpeg\", img)\n```\n\nEncode or decode image streams using the `turbojpeg` backend\n\n```python\nimport kornia_rs as K\n\n# load image with kornia-rs\nimg = K.read_image_jpeg(\"dog.jpeg\")\n\n# encode the image with jpeg\nimage_encoder = K.ImageEncoder()\nimage_encoder.set_quality(95) # set the encoding quality\n\n# get the encoded stream\nimg_encoded: list[int] = image_encoder.encode(img)\n\n# decode back the image\nimage_decoder = K.ImageDecoder()\n\ndecoded_img: np.ndarray = image_decoder.decode(bytes(image_encoded))\n```\n\nResize an image using the `kornia-rs` backend with SIMD acceleration\n\n```python\nimport kornia_rs as K\n\n# load image with kornia-rs\nimg = K.read_image_jpeg(\"dog.jpeg\")\n\n# resize the image\nresized_img = K.resize(img, (128, 128), interpolation=\"bilinear\")\n\nassert resized_img.shape == (128, 128, 3)\n```\n\n## \ud83e\uddd1\u200d\ud83d\udcbb Development\n\nPre-requisites: install `rust` and `python3` in your system.\n\n```bash\ncurl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh\n```\n\nClone the repository in your local directory\n\n```bash\ngit clone https://github.com/kornia/kornia-rs.git\n```\n\n### \ud83e\udd80 Rust\n\nCompile the project and run the tests\n\n```bash\ncargo test\n```\n\nFor specific tests, you can run the following command:\n\n```bash\ncargo test image\n```\n\n### \ud83d\udc0d Python\n\nTo build the Python wheels, we use the `maturin` package. Use the following command to build the wheels:\n\n```bash\nmake build-python\n```\n\nTo run the tests, use the following command:\n\n```bash\nmake test-python\n```\n\n## \ud83d\udc9c Contributing\n\nThis is a child project of [Kornia](https://github.com/kornia/kornia). Join the community to get in touch with us, or just sponsor the project: <https://opencollective.com/kornia>\n\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Low level implementations for computer vision in Rust",
"version": "0.1.7",
"project_urls": {
"Homepage": "http://kornia.org",
"documentation": "https://kornia.readthedocs.io",
"homepage": "http://www.kornia.org",
"repository": "https://github.com/kornia/kornia-rs"
},
"split_keywords": [
"computer vision",
" rust"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "90450ad5bdf0ded42ff96767d236b004deae81ee53c0a503ee4d4d65963bc864",
"md5": "6ab174583f27b3c9eac0b1182cbc37a7",
"sha256": "fe407d6bb9e830e872b7e582b7035aa56092ad8a9b7881f6826706fdb63058bc"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp310-cp310-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "6ab174583f27b3c9eac0b1182cbc37a7",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1515761,
"upload_time": "2024-10-29T07:27:10",
"upload_time_iso_8601": "2024-10-29T07:27:10.360849Z",
"url": "https://files.pythonhosted.org/packages/90/45/0ad5bdf0ded42ff96767d236b004deae81ee53c0a503ee4d4d65963bc864/kornia_rs-0.1.7-cp310-cp310-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6e244558f651560f04ce4a234911b8bbc3493706c13e76c6f844381af5c8621f",
"md5": "c69a21626ca0fb49ac4f03a4830b43ed",
"sha256": "6c67c7c13a380fb6f8a16d03e9e4311550f789196051197217f910af8e21158d"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "c69a21626ca0fb49ac4f03a4830b43ed",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1334356,
"upload_time": "2024-10-29T07:27:12",
"upload_time_iso_8601": "2024-10-29T07:27:12.417887Z",
"url": "https://files.pythonhosted.org/packages/6e/24/4558f651560f04ce4a234911b8bbc3493706c13e76c6f844381af5c8621f/kornia_rs-0.1.7-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "446288e056a7090cfbe461c9537f134c87de7f1018e7ca2ba6736f2366b2ea32",
"md5": "92c3598869a6f1af68b6e57a736157c8",
"sha256": "a57a2c8484fecae5a9dbe1e3ad8e5b507b7f643549441d6309f404f45f27aa8b"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "92c3598869a6f1af68b6e57a736157c8",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1447673,
"upload_time": "2024-10-29T07:27:14",
"upload_time_iso_8601": "2024-10-29T07:27:14.379387Z",
"url": "https://files.pythonhosted.org/packages/44/62/88e056a7090cfbe461c9537f134c87de7f1018e7ca2ba6736f2366b2ea32/kornia_rs-0.1.7-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3917960320728ada483600330bd8f50dc576cef05c06acab96e8373fe4d70ad3",
"md5": "9a5a6d0a8385e6bf0ad3ca66f39e8b30",
"sha256": "b4641703b3498e84c47ceadf1ecc9c8f05bcb9b9aa9e8f0d48e3dc4aa2e2946a"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "9a5a6d0a8385e6bf0ad3ca66f39e8b30",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1613752,
"upload_time": "2024-10-29T07:27:16",
"upload_time_iso_8601": "2024-10-29T07:27:16.091149Z",
"url": "https://files.pythonhosted.org/packages/39/17/960320728ada483600330bd8f50dc576cef05c06acab96e8373fe4d70ad3/kornia_rs-0.1.7-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "954a1bc1d0fae0a8a9e3ba82cfae375760003f6786edac12cab2654f487682f8",
"md5": "8cd210cfe7de2331370ef35916df8663",
"sha256": "c409b9c9612d7025847b4e8d93f43a235cee69a2a990481d072cb90cec94badd"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp310-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "8cd210cfe7de2331370ef35916df8663",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1277568,
"upload_time": "2024-10-29T07:27:17",
"upload_time_iso_8601": "2024-10-29T07:27:17.806242Z",
"url": "https://files.pythonhosted.org/packages/95/4a/1bc1d0fae0a8a9e3ba82cfae375760003f6786edac12cab2654f487682f8/kornia_rs-0.1.7-cp310-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3ad1104e9f575c27679ffedf994e53e6ac39067a0e77b2ea0d1567d4738686ed",
"md5": "9772ae9435c75cc52941be1d7cea1774",
"sha256": "fd3506c8e61fb7f55a289fb34998804b0cd35be1e8f2374ee5eb9081b026c472"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp311-cp311-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "9772ae9435c75cc52941be1d7cea1774",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1513199,
"upload_time": "2024-10-29T07:27:19",
"upload_time_iso_8601": "2024-10-29T07:27:19.505840Z",
"url": "https://files.pythonhosted.org/packages/3a/d1/104e9f575c27679ffedf994e53e6ac39067a0e77b2ea0d1567d4738686ed/kornia_rs-0.1.7-cp311-cp311-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a3c7ba101a4d9b534e61970b33ccdf895b91af14914c89438cd3e1d439680bcb",
"md5": "2c3a4d0112ef01f328b11b5e16c2588b",
"sha256": "b67f07d435d55a4f32051bc65a324b51f43252bf6f7f3405695d1015819c9d30"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "2c3a4d0112ef01f328b11b5e16c2588b",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1330507,
"upload_time": "2024-10-29T07:27:21",
"upload_time_iso_8601": "2024-10-29T07:27:21.015650Z",
"url": "https://files.pythonhosted.org/packages/a3/c7/ba101a4d9b534e61970b33ccdf895b91af14914c89438cd3e1d439680bcb/kornia_rs-0.1.7-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "efe231a0b8b925a561a2beb14e4472ed359b9670fda21f2eb44798f0fc435522",
"md5": "053ba7e521d96cb891cc17f003c41f83",
"sha256": "5c44e9b36f47bccbaeae99f37d312a3aa75da1c0f0fafb1a581a5a0981e94f8a"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "053ba7e521d96cb891cc17f003c41f83",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1448407,
"upload_time": "2024-10-29T07:27:23",
"upload_time_iso_8601": "2024-10-29T07:27:23.477849Z",
"url": "https://files.pythonhosted.org/packages/ef/e2/31a0b8b925a561a2beb14e4472ed359b9670fda21f2eb44798f0fc435522/kornia_rs-0.1.7-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "66047de2357432d3281d7784db220b42063f28ac2f0821072a9a18ee655f1576",
"md5": "b98f0c1f7c4cfe6b72883846c37c4ccd",
"sha256": "7d7ba0dd9c680c28444eed2cf7611faccf3f85eeb9bfa600df53d62d14deefba"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "b98f0c1f7c4cfe6b72883846c37c4ccd",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1613979,
"upload_time": "2024-10-29T07:27:25",
"upload_time_iso_8601": "2024-10-29T07:27:25.206772Z",
"url": "https://files.pythonhosted.org/packages/66/04/7de2357432d3281d7784db220b42063f28ac2f0821072a9a18ee655f1576/kornia_rs-0.1.7-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f37b1f5d35a77a9bfd3566954ceb2e1b38c7673628a2d6a96a8568558454fb27",
"md5": "61d15046e3ac56530eb6c11f1f15bab3",
"sha256": "4f90e5c1f7dfa422889c2af8a570e774604352fc1596f77a00992c1195528c59"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp311-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "61d15046e3ac56530eb6c11f1f15bab3",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1277436,
"upload_time": "2024-10-29T07:27:27",
"upload_time_iso_8601": "2024-10-29T07:27:27.030413Z",
"url": "https://files.pythonhosted.org/packages/f3/7b/1f5d35a77a9bfd3566954ceb2e1b38c7673628a2d6a96a8568558454fb27/kornia_rs-0.1.7-cp311-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2048b8e507a03610ce52c07ebda95f0937d65468ea1db608ad7d1a6ec1190cd4",
"md5": "9bd55678e9540920cc69cc4d34774d3c",
"sha256": "fffb88d9a37251e0e2ca4fbe09589e66da4b4929e9dee428b73302a9d2d6c820"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp312-cp312-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "9bd55678e9540920cc69cc4d34774d3c",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1509003,
"upload_time": "2024-10-29T07:27:28",
"upload_time_iso_8601": "2024-10-29T07:27:28.777498Z",
"url": "https://files.pythonhosted.org/packages/20/48/b8e507a03610ce52c07ebda95f0937d65468ea1db608ad7d1a6ec1190cd4/kornia_rs-0.1.7-cp312-cp312-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2cdcbd4a7ca35b6202c24d0393a100e951d4be106774a60c1f7b61d274ff3904",
"md5": "eac1398df386ff129193d9f0025edd84",
"sha256": "29f4b18989d08bc96af393997890a8578a619696f842811879fa1172b267efd1"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "eac1398df386ff129193d9f0025edd84",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1328581,
"upload_time": "2024-10-29T07:27:30",
"upload_time_iso_8601": "2024-10-29T07:27:30.320362Z",
"url": "https://files.pythonhosted.org/packages/2c/dc/bd4a7ca35b6202c24d0393a100e951d4be106774a60c1f7b61d274ff3904/kornia_rs-0.1.7-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "9ee7a8db14d77adc3b8e8011675e69f7460a0299561d377e3dde542d3c418b87",
"md5": "b4db2e2788b96cbc8fa0f05c9e76b9d5",
"sha256": "3fccf8d4bb1b28ff7f9c252006379d1426317afc215f03dfec99b3fe5ce7773d"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "b4db2e2788b96cbc8fa0f05c9e76b9d5",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1447383,
"upload_time": "2024-10-29T07:27:32",
"upload_time_iso_8601": "2024-10-29T07:27:32.153589Z",
"url": "https://files.pythonhosted.org/packages/9e/e7/a8db14d77adc3b8e8011675e69f7460a0299561d377e3dde542d3c418b87/kornia_rs-0.1.7-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "df366079761c72771c5b720b12bf86832dc0cff4cb04748f81c24f71124e2507",
"md5": "9d61a950fa3910eb340f3eaadb20d8b7",
"sha256": "80d09d41c0bc31f308d27ae49f0c647f036c9cc205caee3bbc8e5bd0bbb30912"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "9d61a950fa3910eb340f3eaadb20d8b7",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1611490,
"upload_time": "2024-10-29T07:27:33",
"upload_time_iso_8601": "2024-10-29T07:27:33.641399Z",
"url": "https://files.pythonhosted.org/packages/df/36/6079761c72771c5b720b12bf86832dc0cff4cb04748f81c24f71124e2507/kornia_rs-0.1.7-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0e496882d3014271d7905263249b36e27c566a6ed0f3234deca75f04407363f2",
"md5": "ff75455be82b7eead071763148b2f2bc",
"sha256": "3fb9aeebf1676b2f2be442905b2112510aea8333e8949ceaaf348a4094887727"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp312-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "ff75455be82b7eead071763148b2f2bc",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1277821,
"upload_time": "2024-10-29T07:27:35",
"upload_time_iso_8601": "2024-10-29T07:27:35.373029Z",
"url": "https://files.pythonhosted.org/packages/0e/49/6882d3014271d7905263249b36e27c566a6ed0f3234deca75f04407363f2/kornia_rs-0.1.7-cp312-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4671a0e7ab0f211027986314cfee01891ddc85c42da8859c4e4d6e14d88aa563",
"md5": "8addc7591202ef3e31495795ffc39cb3",
"sha256": "e9bf3a264683b730aa90aeb138fa29c0905c220be36d32869eaf1e3227e25075"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp37-cp37m-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "8addc7591202ef3e31495795ffc39cb3",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1516655,
"upload_time": "2024-10-29T07:27:36",
"upload_time_iso_8601": "2024-10-29T07:27:36.603840Z",
"url": "https://files.pythonhosted.org/packages/46/71/a0e7ab0f211027986314cfee01891ddc85c42da8859c4e4d6e14d88aa563/kornia_rs-0.1.7-cp37-cp37m-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f4194d889949731d3b604fa6cf125888c346ac696d423fabf5433c1cdce0bd0c",
"md5": "f3a13afb99e63cdc2c89951c6c245f69",
"sha256": "df495f9aa4ea01e3126f5d07d73685f410f320e2c9aff52e215f597d8714c0c1"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp37-cp37m-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "f3a13afb99e63cdc2c89951c6c245f69",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1333953,
"upload_time": "2024-10-29T07:27:37",
"upload_time_iso_8601": "2024-10-29T07:27:37.932189Z",
"url": "https://files.pythonhosted.org/packages/f4/19/4d889949731d3b604fa6cf125888c346ac696d423fabf5433c1cdce0bd0c/kornia_rs-0.1.7-cp37-cp37m-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "43888ab3b7ad1836385c3fc2c5504a91c492c95f61ff294ca729dee71d01a10d",
"md5": "b8a2ece015dca780eda65cb35cf413ef",
"sha256": "b73f48e21f2a3ab533065d6d4600e53ec20096de4ad739dfa0edbad8b9adf499"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "b8a2ece015dca780eda65cb35cf413ef",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1447652,
"upload_time": "2024-10-29T07:27:39",
"upload_time_iso_8601": "2024-10-29T07:27:39.596344Z",
"url": "https://files.pythonhosted.org/packages/43/88/8ab3b7ad1836385c3fc2c5504a91c492c95f61ff294ca729dee71d01a10d/kornia_rs-0.1.7-cp37-cp37m-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f00440e959dfb76999ff37bc18d38294383260de21093bd88f1f09c068e7a147",
"md5": "bcd798b7a51a23032c6bd5d9e4028661",
"sha256": "0e72aea6b688b5a9ad42d0ca703c1f0fb6423a66771d2e8670df765ac38cfe85"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "bcd798b7a51a23032c6bd5d9e4028661",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1613741,
"upload_time": "2024-10-29T07:27:41",
"upload_time_iso_8601": "2024-10-29T07:27:41.464092Z",
"url": "https://files.pythonhosted.org/packages/f0/04/40e959dfb76999ff37bc18d38294383260de21093bd88f1f09c068e7a147/kornia_rs-0.1.7-cp37-cp37m-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f5ae6fb9b386925b2579a5dc0f5846493b33e9a6917f8c4664d02e33aeb327b2",
"md5": "90c6567f159e569f02ee89c2ccb57935",
"sha256": "aeab05a54d691f7978b6cd99e3d0cad3619c8316196ddc9e2aff72947ce51577"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp37-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "90c6567f159e569f02ee89c2ccb57935",
"packagetype": "bdist_wheel",
"python_version": "cp37",
"requires_python": ">=3.8",
"size": 1277635,
"upload_time": "2024-10-29T07:27:42",
"upload_time_iso_8601": "2024-10-29T07:27:42.782592Z",
"url": "https://files.pythonhosted.org/packages/f5/ae/6fb9b386925b2579a5dc0f5846493b33e9a6917f8c4664d02e33aeb327b2/kornia_rs-0.1.7-cp37-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0f3253680430bd09e3e143f6a452845323b11cdb8d164f5c51532486c27974d3",
"md5": "e3ab83376a808599b9b16dcc29c1a4f2",
"sha256": "d9c10ffcc151e1a99075b95fdf09adeab8d7f2212e93ee9aef44fe4489505a9a"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp38-cp38-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "e3ab83376a808599b9b16dcc29c1a4f2",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1515441,
"upload_time": "2024-10-29T07:27:44",
"upload_time_iso_8601": "2024-10-29T07:27:44.043050Z",
"url": "https://files.pythonhosted.org/packages/0f/32/53680430bd09e3e143f6a452845323b11cdb8d164f5c51532486c27974d3/kornia_rs-0.1.7-cp38-cp38-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "b4d57603ebe844e1642bf325b3cf25ab0663cb2b2adea53e1a534c092909c84a",
"md5": "cda84cd0c3da58f38025805d56e66935",
"sha256": "7de372e194fc43efd12d928e75d6d52e1f18590a944c073d19444658a6060aae"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "cda84cd0c3da58f38025805d56e66935",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1334244,
"upload_time": "2024-10-29T07:27:45",
"upload_time_iso_8601": "2024-10-29T07:27:45.535889Z",
"url": "https://files.pythonhosted.org/packages/b4/d5/7603ebe844e1642bf325b3cf25ab0663cb2b2adea53e1a534c092909c84a/kornia_rs-0.1.7-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "10a77791714390301c289449740758f400f610e61af94be7f6dadc20cd24062a",
"md5": "c38296d0d0929c43a6e335239d67f424",
"sha256": "0fb7b6ab2e48365bc09814d2d86e8205fab9484abf607894b96237bdd6408990"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "c38296d0d0929c43a6e335239d67f424",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1447725,
"upload_time": "2024-10-29T07:27:47",
"upload_time_iso_8601": "2024-10-29T07:27:47.678914Z",
"url": "https://files.pythonhosted.org/packages/10/a7/7791714390301c289449740758f400f610e61af94be7f6dadc20cd24062a/kornia_rs-0.1.7-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "395e2a104b205789b7ecaec8a4681d63e079326ce2f7b12e3792e37a392171aa",
"md5": "942589655b55d85de748e41adba28c82",
"sha256": "df9b0348f31e7bb5960615cb645a11036f8e0dcddc169b87ff28bad3149bdbbd"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "942589655b55d85de748e41adba28c82",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1613080,
"upload_time": "2024-10-29T07:27:49",
"upload_time_iso_8601": "2024-10-29T07:27:49.235711Z",
"url": "https://files.pythonhosted.org/packages/39/5e/2a104b205789b7ecaec8a4681d63e079326ce2f7b12e3792e37a392171aa/kornia_rs-0.1.7-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d58a1c633a07cc7be448e601e12501c7004890f2af6abc8a549f84604865a3ba",
"md5": "71798d9d14b137a0fc9a0c8ac2083c1e",
"sha256": "872fdb89d55f80cd3a39034669b162b801fba83be93b25683d4fc48249dd2277"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp38-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "71798d9d14b137a0fc9a0c8ac2083c1e",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1277607,
"upload_time": "2024-10-29T07:27:50",
"upload_time_iso_8601": "2024-10-29T07:27:50.573862Z",
"url": "https://files.pythonhosted.org/packages/d5/8a/1c633a07cc7be448e601e12501c7004890f2af6abc8a549f84604865a3ba/kornia_rs-0.1.7-cp38-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "9991b6c1d731ba6387042a7452b65cae89a0ff176fb1c4014d37726c786c6209",
"md5": "87a6fc0444c0db0679d84cbc811844a4",
"sha256": "4f45a6a0323655e781d513d197def2a0001b526e6191a34f6e2d99ef193a6654"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp39-cp39-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "87a6fc0444c0db0679d84cbc811844a4",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1516309,
"upload_time": "2024-10-29T07:27:52",
"upload_time_iso_8601": "2024-10-29T07:27:52.401329Z",
"url": "https://files.pythonhosted.org/packages/99/91/b6c1d731ba6387042a7452b65cae89a0ff176fb1c4014d37726c786c6209/kornia_rs-0.1.7-cp39-cp39-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3b7f62927fb553513fc07bf6ced8ba8bd61400cb6d4a64bc114628656003920e",
"md5": "9d70a6ee82e4286821fa2ab3e7f13714",
"sha256": "473d5197fa356a3230c4126867cfce8c433f9af20fec11734aace30fd8c3bc92"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "9d70a6ee82e4286821fa2ab3e7f13714",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1334309,
"upload_time": "2024-10-29T07:27:53",
"upload_time_iso_8601": "2024-10-29T07:27:53.911441Z",
"url": "https://files.pythonhosted.org/packages/3b/7f/62927fb553513fc07bf6ced8ba8bd61400cb6d4a64bc114628656003920e/kornia_rs-0.1.7-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ddf3a785452ec05cc4de4ef38335f6776630b8565ac4e307571df18d5685c07a",
"md5": "e87ba480928c1549ba03b89d5f7a8fd2",
"sha256": "c3b68c2bd10f2a3ad3eb75b993ef56f492e0b347b73f21a022dbf027fa88d0a5"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "e87ba480928c1549ba03b89d5f7a8fd2",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1448301,
"upload_time": "2024-10-29T07:27:55",
"upload_time_iso_8601": "2024-10-29T07:27:55.279305Z",
"url": "https://files.pythonhosted.org/packages/dd/f3/a785452ec05cc4de4ef38335f6776630b8565ac4e307571df18d5685c07a/kornia_rs-0.1.7-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3467c2a6ab65d6ed1870896527bd64a7dccbcf1ab465899cf37aece73d9574db",
"md5": "b2b27dbeccb0d003845e478fba8d3759",
"sha256": "33170fa6c60d9d0e544211b1a8b802559971561449e010e50cf1e4564ec0182c"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "b2b27dbeccb0d003845e478fba8d3759",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1614242,
"upload_time": "2024-10-29T07:27:56",
"upload_time_iso_8601": "2024-10-29T07:27:56.741276Z",
"url": "https://files.pythonhosted.org/packages/34/67/c2a6ab65d6ed1870896527bd64a7dccbcf1ab465899cf37aece73d9574db/kornia_rs-0.1.7-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ffbc997a4eefd5101d0d86e66a7a7d968a5115b503c3977fa2f40bbac36474d9",
"md5": "34be7b96e20c35125032f06a6bf422f2",
"sha256": "74d9018f1674fdf975941a924c4cad402a1e035578adb09ad56bbfb219a37a33"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7-cp39-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "34be7b96e20c35125032f06a6bf422f2",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1277758,
"upload_time": "2024-10-29T07:27:58",
"upload_time_iso_8601": "2024-10-29T07:27:58.075569Z",
"url": "https://files.pythonhosted.org/packages/ff/bc/997a4eefd5101d0d86e66a7a7d968a5115b503c3977fa2f40bbac36474d9/kornia_rs-0.1.7-cp39-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "07f3c526610210887108c43a0bb1560964176001a25fc72eba7d084394ef5c7a",
"md5": "ccbc11a7a4de10a9f0173a61eacd1e6c",
"sha256": "601aeacd17826d3a796e847d558d9e32c08aa686653eb21b2f93031438af93a1"
},
"downloads": -1,
"filename": "kornia_rs-0.1.7.tar.gz",
"has_sig": false,
"md5_digest": "ccbc11a7a4de10a9f0173a61eacd1e6c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 71171,
"upload_time": "2024-10-29T07:27:59",
"upload_time_iso_8601": "2024-10-29T07:27:59.368472Z",
"url": "https://files.pythonhosted.org/packages/07/f3/c526610210887108c43a0bb1560964176001a25fc72eba7d084394ef5c7a/kornia_rs-0.1.7.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-29 07:27:59",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "kornia",
"github_project": "kornia-rs",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "kornia-rs"
}