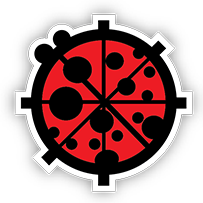
[](https://github.com/ladybug-tools/ladybug-comfort/actions)
[](https://coveralls.io/github/ladybug-tools/ladybug-comfort)
[](https://www.python.org/downloads/release/python-3100/) [](https://www.python.org/downloads/release/python-370/) [](https://www.python.org/downloads/release/python-270/) [](https://github.com/IronLanguages/ironpython2/releases/tag/ipy-2.7.8/)
# ladybug-comfort
Ladybug-comfort is a Python library that adds thermal comfort functionalities to
[ladybug-core](https://github.com/ladybug-tools/ladybug/).
## [API Documentation](https://www.ladybug.tools/ladybug-comfort/docs/)
## Installation
To install the library use:
`pip install ladybug-comfort`
If you want to also include the dependencies needed for thermal mapping use:
`pip install -U honeybee-energy[mapping]`
To check if the Ladybug-comfort command line interface is installed correctly,
use `ladybug-comfort --help`.
## Usage
```python
"""Get the percentage of time outdoor conditions are comfortable with/without sun + wind"""
from ladybug.epw import EPW
from ladybug_comfort.collection.utci import UTCI
epw_file_path = './tests/epw/chicago.epw'
epw = EPW(epw_file_path)
utci_obj_exposed = UTCI.from_epw(epw, include_wind=True, include_sun=True)
utci_obj_protected = UTCI.from_epw(epw, include_wind=False, include_sun=False)
print(utci_obj_exposed.percent_neutral) # comfortable percent of time with sun + wind
print(utci_obj_protected.percent_neutral) # comfortable percent of time without sun + wind
```
## Local Development
1. Clone this repo locally
```console
git clone git@github.com:ladybug-tools/ladybug-comfort.git
# or
git clone https://github.com/ladybug-tools/ladybug-comfort.git
```
2. Install dependencies:
```console
cd ladybug-comfort
pip install -r dev-requirements.txt
pip install -r requirements.txt
```
3. Run Tests:
```console
python -m pytest ./tests
```
4. Generate Documentation:
```console
sphinx-apidoc -f -e -d 4 -o ./docs ./ladybug_comfort
sphinx-build -b html ./docs ./docs/_build/docs
```
### Derivative Work
Ladybug-comfort is a derivative work of the following software projects:
* [CBE Comfort Tool](https://github.com/CenterForTheBuiltEnvironment/comfort_tool) for indoor thermal comfort calculations. Available under GPL.
* [UTCI Fortran Code](http://www.utci.org/utci_doku.php) for outdoor thermal comfort calculations. Available under MIT.
Applicable copyright notices for these works can be found within the relevant .py files.
Raw data
{
"_id": null,
"home_page": "https://github.com/ladybug-tools/ladybug-comfort",
"name": "ladybug-comfort",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "Ladybug Tools",
"author_email": "info@ladybug.tools",
"download_url": "https://files.pythonhosted.org/packages/b4/a2/f01f13e1f49bb8096414623b6a73687d5a007f3b84d663e49eff218b8cfa/ladybug_comfort-0.18.56.tar.gz",
"platform": null,
"description": "\n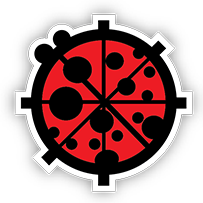\n\n[](https://github.com/ladybug-tools/ladybug-comfort/actions)\n[](https://coveralls.io/github/ladybug-tools/ladybug-comfort)\n\n[](https://www.python.org/downloads/release/python-3100/) [](https://www.python.org/downloads/release/python-370/) [](https://www.python.org/downloads/release/python-270/) [](https://github.com/IronLanguages/ironpython2/releases/tag/ipy-2.7.8/)\n\n# ladybug-comfort\n\nLadybug-comfort is a Python library that adds thermal comfort functionalities to\n[ladybug-core](https://github.com/ladybug-tools/ladybug/).\n\n## [API Documentation](https://www.ladybug.tools/ladybug-comfort/docs/)\n\n## Installation\n\nTo install the library use:\n\n`pip install ladybug-comfort`\n\nIf you want to also include the dependencies needed for thermal mapping use:\n\n`pip install -U honeybee-energy[mapping]`\n\nTo check if the Ladybug-comfort command line interface is installed correctly,\nuse `ladybug-comfort --help`.\n\n## Usage\n\n```python\n\"\"\"Get the percentage of time outdoor conditions are comfortable with/without sun + wind\"\"\"\nfrom ladybug.epw import EPW\nfrom ladybug_comfort.collection.utci import UTCI\n\nepw_file_path = './tests/epw/chicago.epw'\nepw = EPW(epw_file_path)\nutci_obj_exposed = UTCI.from_epw(epw, include_wind=True, include_sun=True)\nutci_obj_protected = UTCI.from_epw(epw, include_wind=False, include_sun=False)\n\nprint(utci_obj_exposed.percent_neutral) # comfortable percent of time with sun + wind\nprint(utci_obj_protected.percent_neutral) # comfortable percent of time without sun + wind\n```\n\n## Local Development\n1. Clone this repo locally\n```console\ngit clone git@github.com:ladybug-tools/ladybug-comfort.git\n\n# or\n\ngit clone https://github.com/ladybug-tools/ladybug-comfort.git\n```\n2. Install dependencies:\n```console\ncd ladybug-comfort\npip install -r dev-requirements.txt\npip install -r requirements.txt\n```\n\n3. Run Tests:\n```console\npython -m pytest ./tests\n```\n\n4. Generate Documentation:\n```console\nsphinx-apidoc -f -e -d 4 -o ./docs ./ladybug_comfort\nsphinx-build -b html ./docs ./docs/_build/docs\n```\n\n### Derivative Work\n\nLadybug-comfort is a derivative work of the following software projects:\n\n* [CBE Comfort Tool](https://github.com/CenterForTheBuiltEnvironment/comfort_tool) for indoor thermal comfort calculations. Available under GPL.\n* [UTCI Fortran Code](http://www.utci.org/utci_doku.php) for outdoor thermal comfort calculations. Available under MIT.\n\nApplicable copyright notices for these works can be found within the relevant .py files.\n",
"bugtrack_url": null,
"license": "AGPL-3.0",
"summary": "Ladybug comfort is a Python library that adds thermal comfort functionalities to Ladybug.",
"version": "0.18.56",
"project_urls": {
"Homepage": "https://github.com/ladybug-tools/ladybug-comfort"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9d6eee206a902680a5cbf7e813eee30a5d932e6f52f017d757ffe7f587727fbe",
"md5": "3d3caf3ab9e60f6d2bdabce78a2e55f6",
"sha256": "f48bcf2a2f0082b6465208bbbd38134a34017260df29d5794f6114c4386e76dc"
},
"downloads": -1,
"filename": "ladybug_comfort-0.18.56-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "3d3caf3ab9e60f6d2bdabce78a2e55f6",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": null,
"size": 177584,
"upload_time": "2024-10-29T21:05:39",
"upload_time_iso_8601": "2024-10-29T21:05:39.972795Z",
"url": "https://files.pythonhosted.org/packages/9d/6e/ee206a902680a5cbf7e813eee30a5d932e6f52f017d757ffe7f587727fbe/ladybug_comfort-0.18.56-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b4a2f01f13e1f49bb8096414623b6a73687d5a007f3b84d663e49eff218b8cfa",
"md5": "b7805ba1b8665d157e5149e23547927a",
"sha256": "602b64b494d7ccd3781f9b369f32fd14745fb098ce3ff62984c3a1e5064bbf34"
},
"downloads": -1,
"filename": "ladybug_comfort-0.18.56.tar.gz",
"has_sig": false,
"md5_digest": "b7805ba1b8665d157e5149e23547927a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 151772,
"upload_time": "2024-10-29T21:05:41",
"upload_time_iso_8601": "2024-10-29T21:05:41.586666Z",
"url": "https://files.pythonhosted.org/packages/b4/a2/f01f13e1f49bb8096414623b6a73687d5a007f3b84d663e49eff218b8cfa/ladybug_comfort-0.18.56.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-29 21:05:41",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "ladybug-tools",
"github_project": "ladybug-comfort",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "ladybug-core",
"specs": [
[
"==",
"0.43.13"
]
]
}
],
"tox": true,
"lcname": "ladybug-comfort"
}