Name | lcsspy JSON |
Version |
1.0.1
JSON |
| download |
home_page | |
Summary | Longest Common Subsequence (LCSS) computation to measure similarity for time series. |
upload_time | 2023-12-05 14:48:35 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.9 |
license | MIT License Copyright (c) 2023 Francesco Lafratta Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
lcss
lcsspy
similarity
time series
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Introduction
lcsspy is a Python package to compute the longest common subsequence
(also known as LCSS) similarity measure for time series data.
LCSS reconstructs a common subsequence by matching similar elements
in the two series.
Two elements are matched if they are sufficiently close in time and
also have similar values.
# Getting Started
## Installation
lcsspy supports any Python version starting from 3.9, and is OS independent.
Regular installation can be performed by running the following command.
```command
pip install lcsspy
```
This will install lcsspy and its required dependencies.
If you want, you can install pytest as an optional dependency by running
a slightly different command.
```command
pip install lcsspy[tests]
```
This allows you to run existing tests, or write your own.
Refer to the [pytest documentation](https://pytest.org)
to learn more about running tests.
## Usage
### Discrete LCSS
Discrete LCSS measures similarity between time series with discrete
time indexes. It's the formulation introduced by
[Vlachos](https://ieeexplore.ieee.org/document/994784).
The following script matches elements that have a value difference
smaller than 1.1, and that are at most 1 index position apart.
```python
import matplotlib.pyplot as plt
import numpy as np
from lcsspy.lcss import discrete_lcss
ts1 = np.array([1, 2, 2, 9, 14, 11, 19, 18])
ts2 = np.array([2, 1, 4, 7, 10, 15, 12, 8, 17])
result = discrete_lcss(ts1=ts1, ts2=ts2, epsilon=1.1, delta=1, plot=True)
print(result.lcss_measure)
plt.show()
```
Running this code displays two figures.
The first one plots the input time series and signals which elements were
matched with a green line.
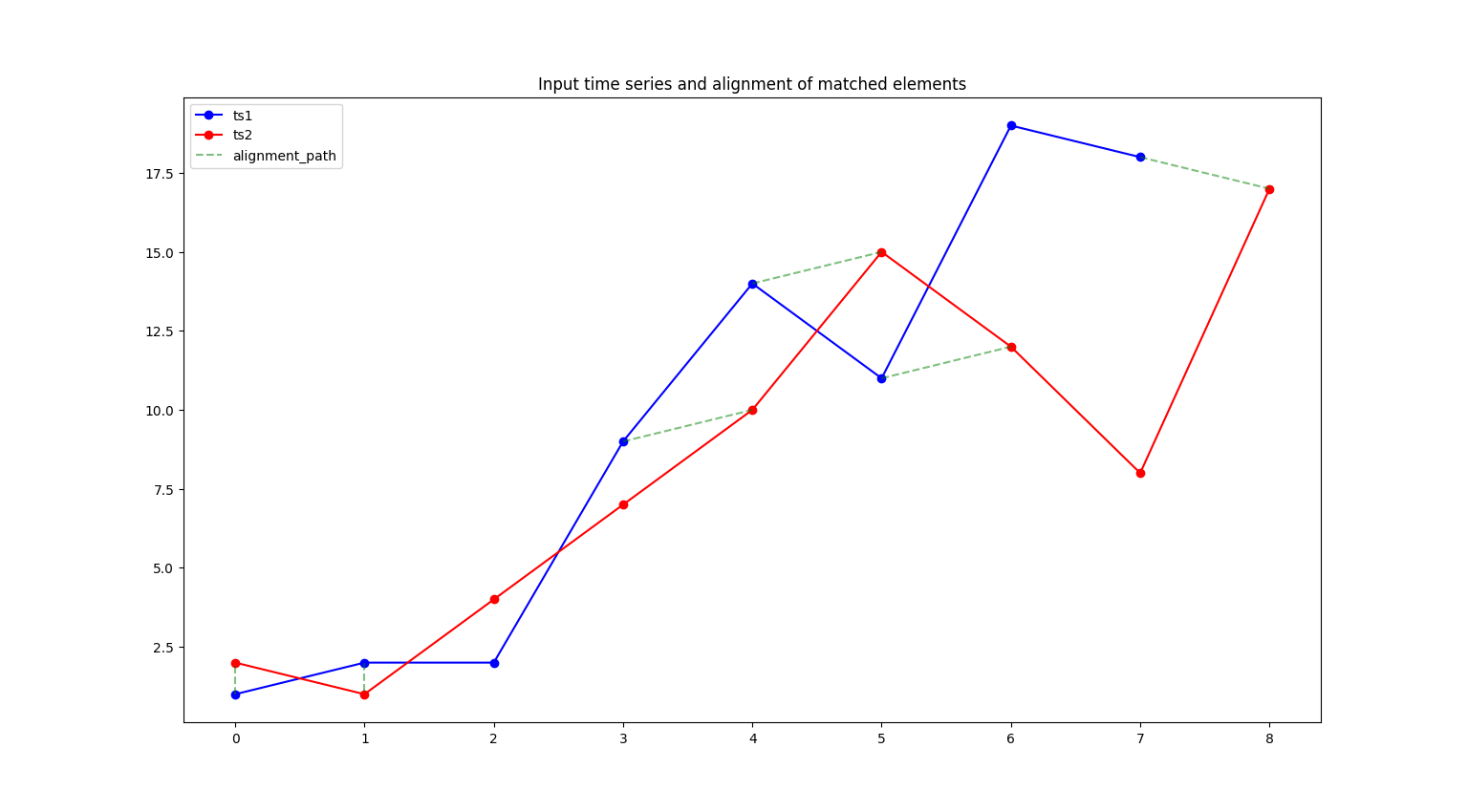
The second figure plots only the elements from the input series that are part
of the common subsequence.
In this case, the common subsequence has length 6 since it contains six pairs
of elements that were matched.
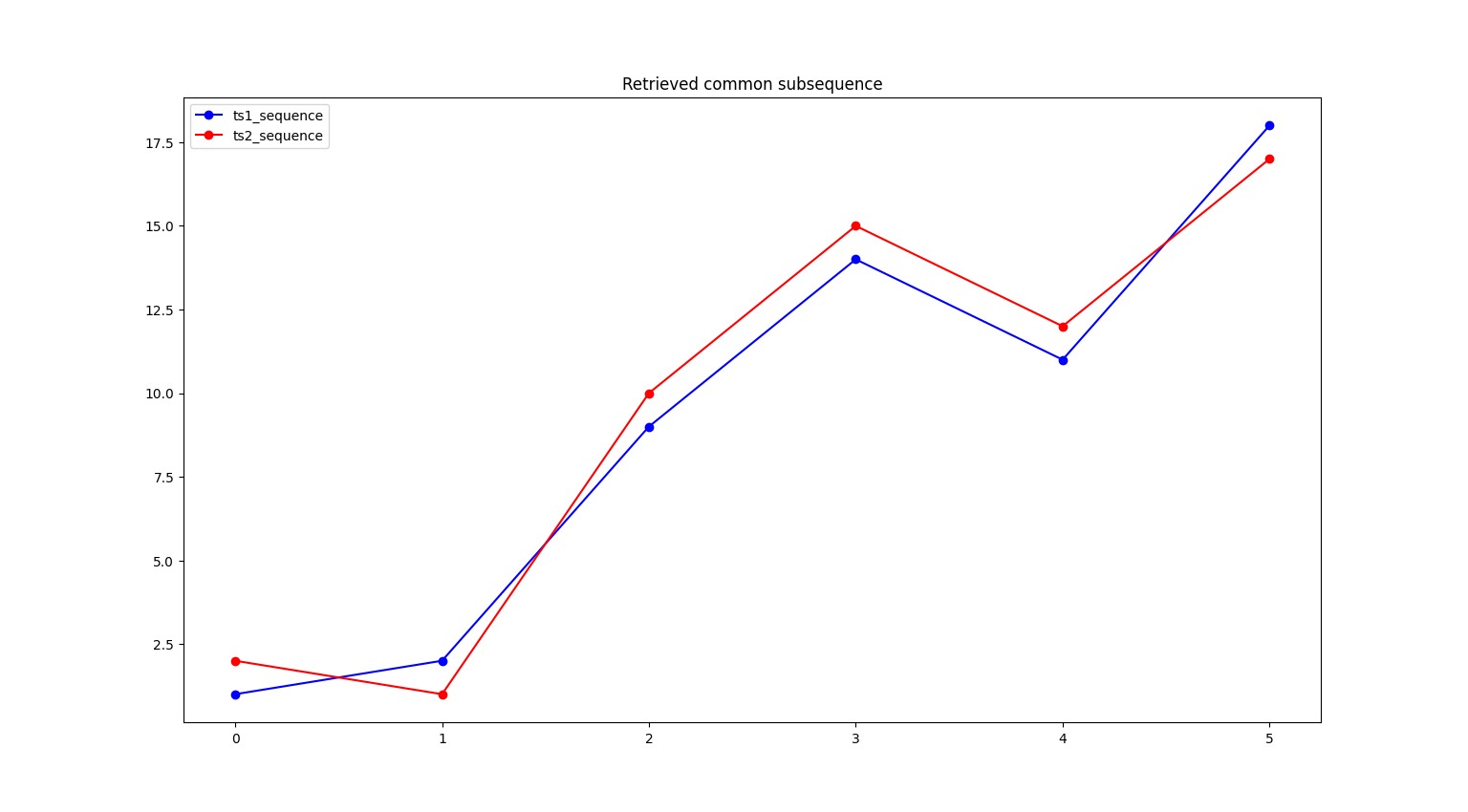
This value is divided by the length of
the shortest series to obtain the LCSS
measure which belongs to the range $[0, 1]$.
The measure equals $6/8$, which is printed to the console.
```command
0.75
```
### Continuous LCSS
Continuous LCSS deals with time series that have continuous
time indexes (timestamps).
It's particularly useful when the two series are very irregular and present
many gaps.
In the following example, elements are matched if they have a value difference
smaller than 0.9, and their timestamps are at most 1 minute apart.
```python
import matplotlib.pyplot as plt
import pandas as pd
from lcsspy.lcss import continuous_lcss
ts1 = pd.Series(
[12.4, 13.7, 15.8, 8.7],
index=pd.DatetimeIndex(
[
"2023-11-17 08:42:23",
"2023-11-17 08:43:35",
"2023-11-17 08:45:06",
"2023-11-17 08:50:23",
]
),
)
ts2 = pd.Series(
[13.2, 13.0, 19.0, 9.0, 9.2],
index=pd.DatetimeIndex(
[
"2023-11-17 08:42:39",
"2023-11-17 08:44:02",
"2023-11-17 08:45:32",
"2023-11-17 08:49:37",
"2023-11-17 08:51:12",
]
),
)
result = continuous_lcss(
ts1=ts1, ts2=ts2, epsilon=0.9, delta=pd.Timedelta(minutes=1), plot=True
)
print(result.lcss_measure)
plt.show()
```
The LCSS measure equals $3/4$ and similar plots to those concerning the
discrete LCSS example are displayed.
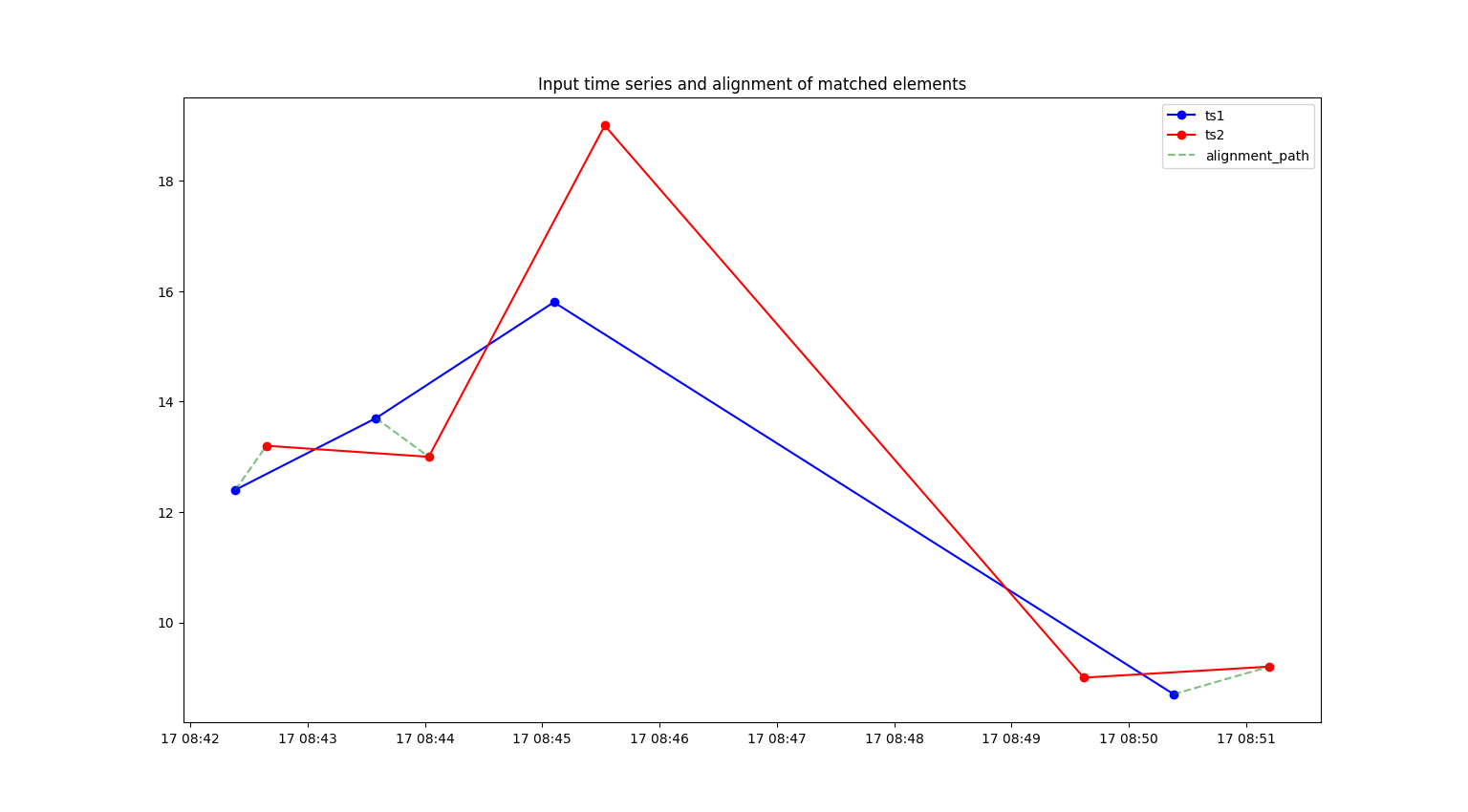
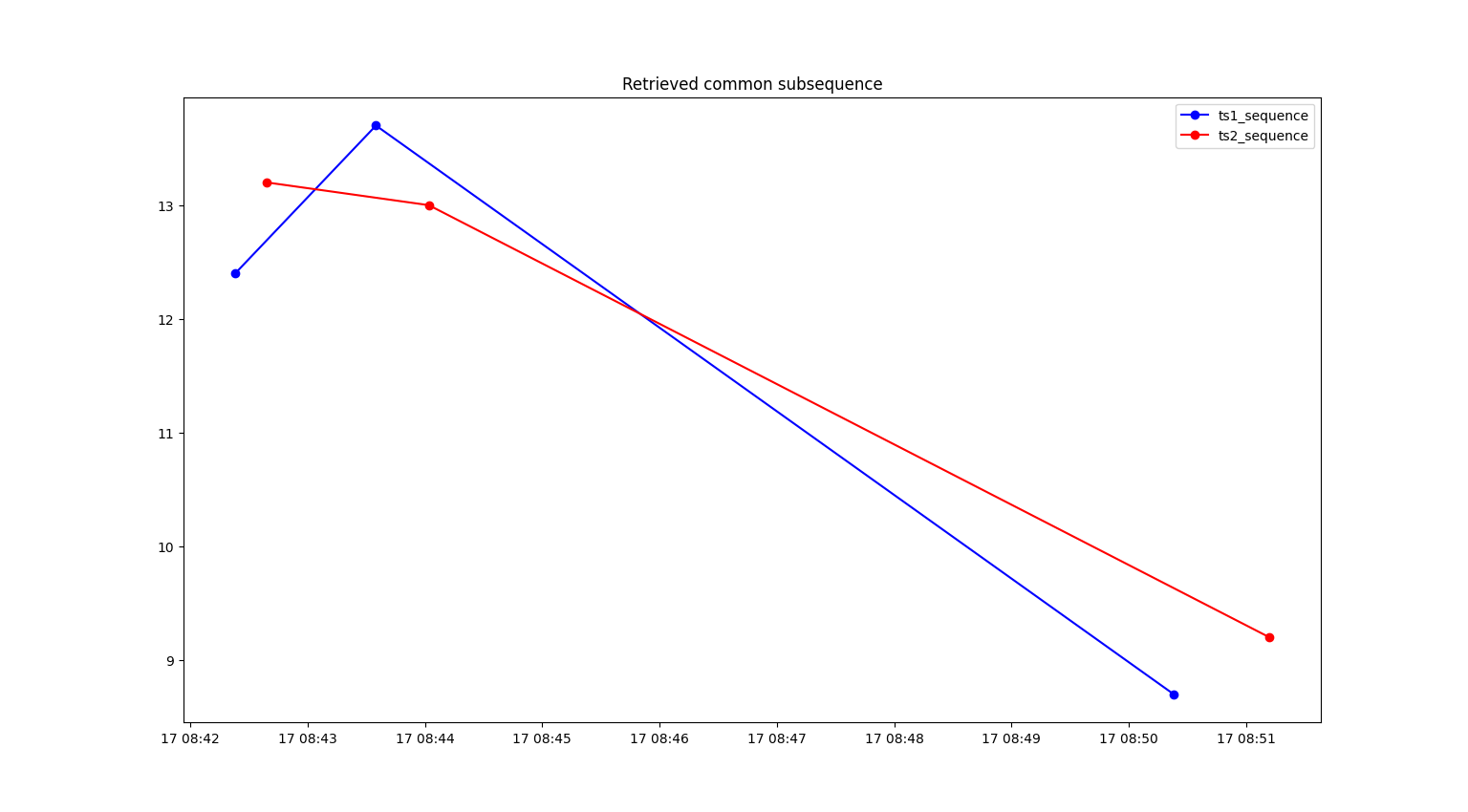
Refer to the [documentation](https://francescolafratta.github.io/lcsspy/) for more details.
# Testing
This package uses the pytest framework to run tests.
A test folder which achieves 100% code coverage is provided.
# Copyright and License
All source code is Copyright (c) 2023 Francesco Lafratta.
lcsspy is licensed under the [MIT License](https://choosealicense.com/licenses/mit).
Raw data
{
"_id": null,
"home_page": "",
"name": "lcsspy",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": "Francesco Lafratta <fl.novacta@outlook.com>",
"keywords": "lcss,lcsspy,similarity,time series",
"author": "",
"author_email": "Francesco Lafratta <fl.novacta@outlook.com>",
"download_url": "https://files.pythonhosted.org/packages/44/8f/347db56098e4269032f763012ed1b1d3fed946c0b096bb5e939f9eb4c98b/lcsspy-1.0.1.tar.gz",
"platform": null,
"description": "# Introduction \nlcsspy is a Python package to compute the longest common subsequence \n(also known as LCSS) similarity measure for time series data.\n\nLCSS reconstructs a common subsequence by matching similar elements\nin the two series. \nTwo elements are matched if they are sufficiently close in time and\nalso have similar values.\n\n# Getting Started\n## Installation\nlcsspy supports any Python version starting from 3.9, and is OS independent.\n\nRegular installation can be performed by running the following command.\n```command\npip install lcsspy\n```\nThis will install lcsspy and its required dependencies.\n\nIf you want, you can install pytest as an optional dependency by running\na slightly different command.\n```command\npip install lcsspy[tests]\n```\nThis allows you to run existing tests, or write your own.\nRefer to the [pytest documentation](https://pytest.org)\nto learn more about running tests.\n## Usage\n### Discrete LCSS\nDiscrete LCSS measures similarity between time series with discrete\ntime indexes. It's the formulation introduced by\n[Vlachos](https://ieeexplore.ieee.org/document/994784).\n\nThe following script matches elements that have a value difference\nsmaller than 1.1, and that are at most 1 index position apart.\n\n```python\nimport matplotlib.pyplot as plt\nimport numpy as np\nfrom lcsspy.lcss import discrete_lcss\n\nts1 = np.array([1, 2, 2, 9, 14, 11, 19, 18])\nts2 = np.array([2, 1, 4, 7, 10, 15, 12, 8, 17])\nresult = discrete_lcss(ts1=ts1, ts2=ts2, epsilon=1.1, delta=1, plot=True)\nprint(result.lcss_measure)\nplt.show()\n```\nRunning this code displays two figures.\nThe first one plots the input time series and signals which elements were\nmatched with a green line.\n\n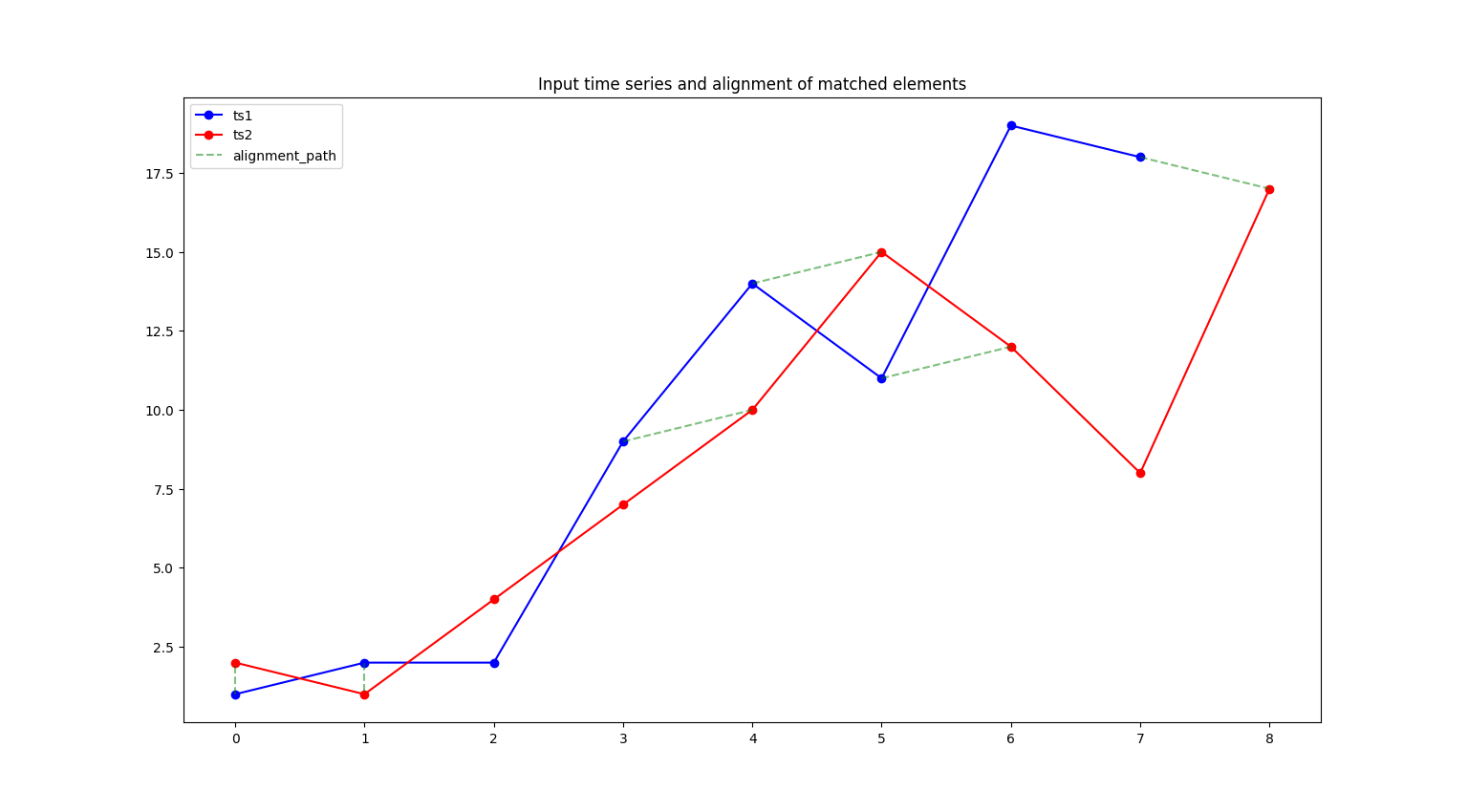\n\nThe second figure plots only the elements from the input series that are part\nof the common subsequence.\nIn this case, the common subsequence has length 6 since it contains six pairs\nof elements that were matched.\n\n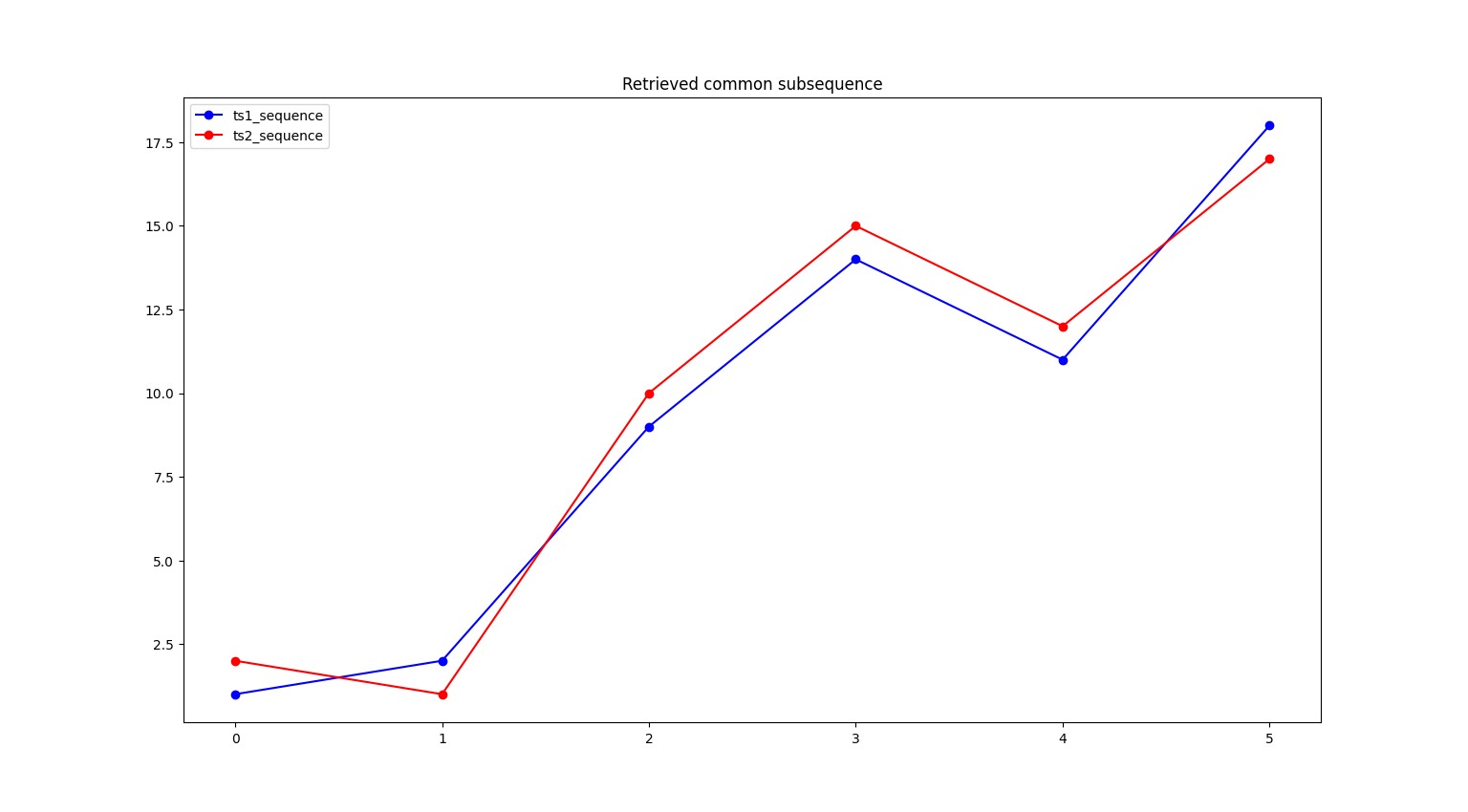\n\nThis value is divided by the length of\nthe shortest series to obtain the LCSS\nmeasure which belongs to the range $[0, 1]$.\n\nThe measure equals $6/8$, which is printed to the console.\n```command\n0.75\n```\n### Continuous LCSS\nContinuous LCSS deals with time series that have continuous\ntime indexes (timestamps).\nIt's particularly useful when the two series are very irregular and present\nmany gaps.\n\nIn the following example, elements are matched if they have a value difference\nsmaller than 0.9, and their timestamps are at most 1 minute apart.\n```python\nimport matplotlib.pyplot as plt\nimport pandas as pd\nfrom lcsspy.lcss import continuous_lcss\n\nts1 = pd.Series(\n [12.4, 13.7, 15.8, 8.7],\n index=pd.DatetimeIndex(\n [\n \"2023-11-17 08:42:23\",\n \"2023-11-17 08:43:35\",\n \"2023-11-17 08:45:06\",\n \"2023-11-17 08:50:23\",\n ]\n ),\n)\n\nts2 = pd.Series(\n [13.2, 13.0, 19.0, 9.0, 9.2],\n index=pd.DatetimeIndex(\n [\n \"2023-11-17 08:42:39\",\n \"2023-11-17 08:44:02\",\n \"2023-11-17 08:45:32\",\n \"2023-11-17 08:49:37\",\n \"2023-11-17 08:51:12\",\n ]\n ),\n)\n\nresult = continuous_lcss(\n ts1=ts1, ts2=ts2, epsilon=0.9, delta=pd.Timedelta(minutes=1), plot=True\n)\n\nprint(result.lcss_measure)\nplt.show()\n```\nThe LCSS measure equals $3/4$ and similar plots to those concerning the\ndiscrete LCSS example are displayed.\n\n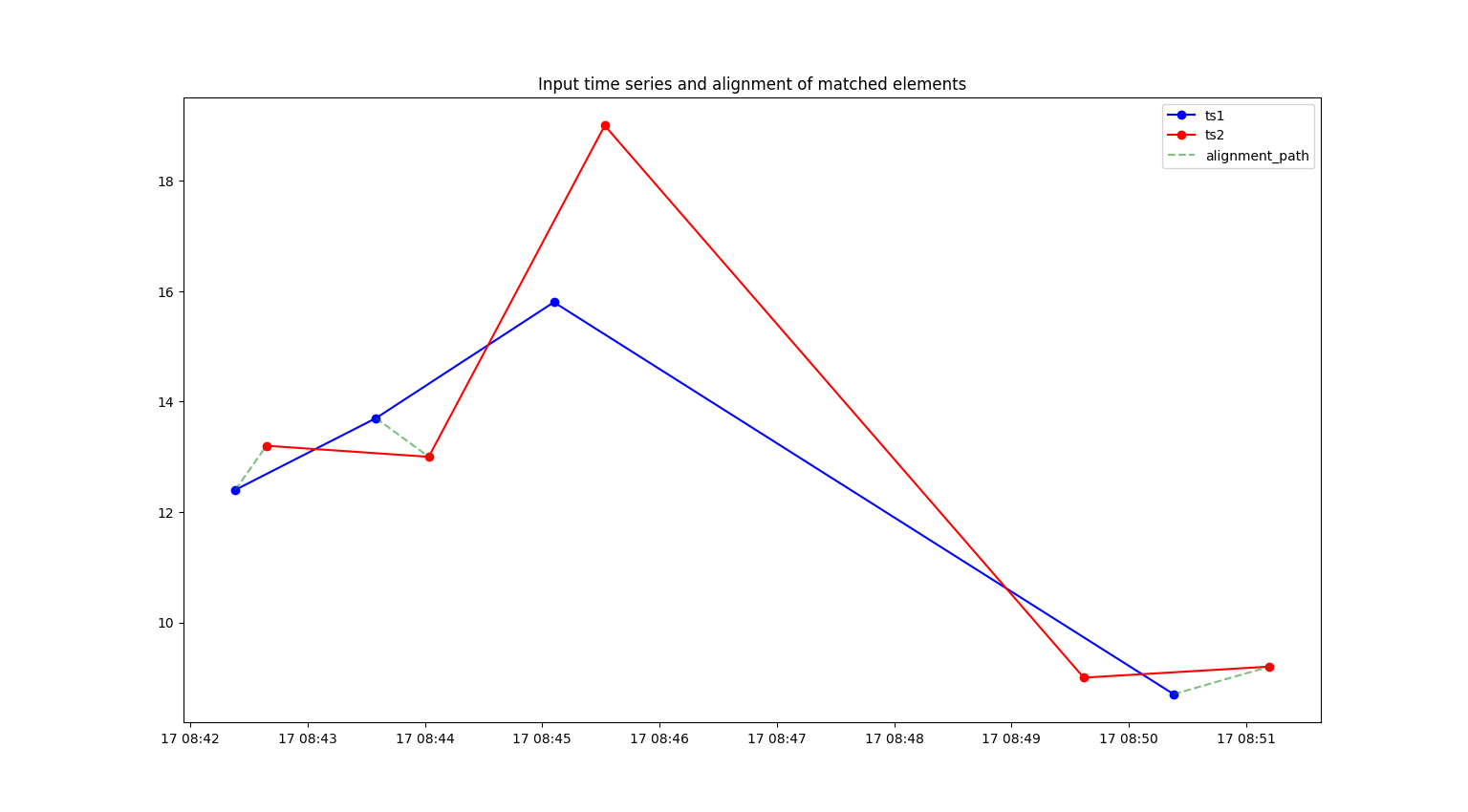\n\n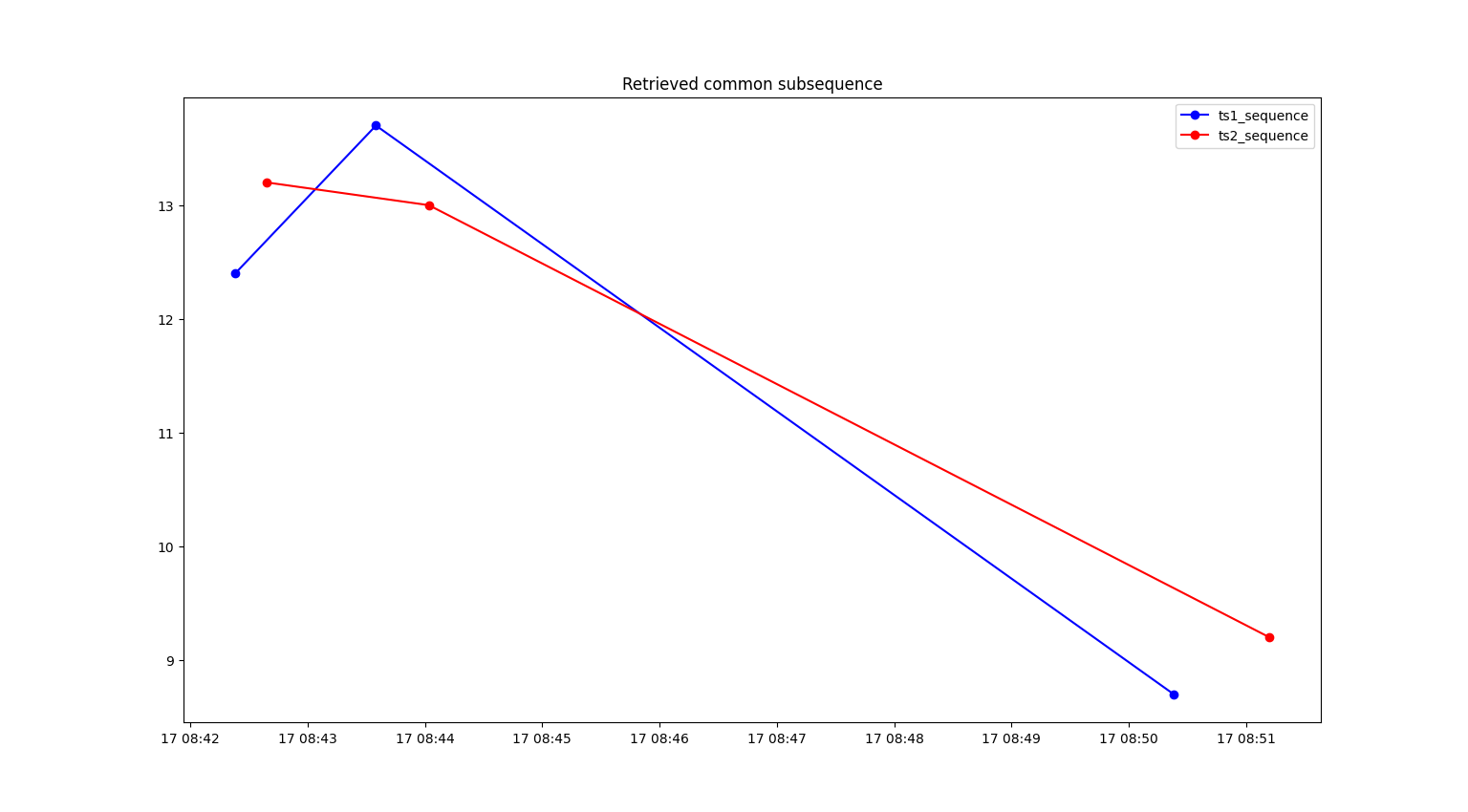\n\nRefer to the [documentation](https://francescolafratta.github.io/lcsspy/) for more details.\n\n# Testing\nThis package uses the pytest framework to run tests.\nA test folder which achieves 100% code coverage is provided.\n\n# Copyright and License\nAll source code is Copyright (c) 2023 Francesco Lafratta.\n\nlcsspy is licensed under the [MIT License](https://choosealicense.com/licenses/mit).",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2023 Francesco Lafratta Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Longest Common Subsequence (LCSS) computation to measure similarity for time series.",
"version": "1.0.1",
"project_urls": {
"Documentation": "https://francescolafratta.github.io/lcsspy/",
"Homepage": "https://github.com/francescolafratta/lcsspy",
"Issues": "https://github.com/francescolafratta/lcsspy/issues"
},
"split_keywords": [
"lcss",
"lcsspy",
"similarity",
"time series"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "2354ed86f7c003314d6a2485c1beca8265451d5dca76fc8bfb37e264cd0abebb",
"md5": "2ed3e17264897db42f40e7fdcb005538",
"sha256": "32b3b1c5ff348a75b8a3de730e9943f0ad0bbc0dbb257215fc2a2bb574b1540a"
},
"downloads": -1,
"filename": "lcsspy-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2ed3e17264897db42f40e7fdcb005538",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.9",
"size": 6932,
"upload_time": "2023-12-05T14:48:33",
"upload_time_iso_8601": "2023-12-05T14:48:33.405689Z",
"url": "https://files.pythonhosted.org/packages/23/54/ed86f7c003314d6a2485c1beca8265451d5dca76fc8bfb37e264cd0abebb/lcsspy-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "448f347db56098e4269032f763012ed1b1d3fed946c0b096bb5e939f9eb4c98b",
"md5": "741fa3dcfbe2d34012be40a493d326b4",
"sha256": "98c317bc798e685738e364252291696f8962c20ea986ca4ffd81047997f1d6be"
},
"downloads": -1,
"filename": "lcsspy-1.0.1.tar.gz",
"has_sig": false,
"md5_digest": "741fa3dcfbe2d34012be40a493d326b4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 219888,
"upload_time": "2023-12-05T14:48:35",
"upload_time_iso_8601": "2023-12-05T14:48:35.145915Z",
"url": "https://files.pythonhosted.org/packages/44/8f/347db56098e4269032f763012ed1b1d3fed946c0b096bb5e939f9eb4c98b/lcsspy-1.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-12-05 14:48:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "francescolafratta",
"github_project": "lcsspy",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "lcsspy"
}