Name | linex JSON |
Version |
0.2
JSON |
| download |
home_page | |
Summary | The fast and easy-to-use LINE bot SDK. |
upload_time | 2023-09-02 12:02:05 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.9 |
license | MIT License Copyright (c) 2023 AWeirdScratcher Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
line
bot
linebot
sdk
async
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# LineX
The fast and easy-to-use LINE bot SDK. Focus on the content, we do the rest.
It's (or it has):
- Based on [FastAPI](https://fastapi.tiangolo.com)
- Feature-rich
- Async-ready
- Around 60% coverage of the LINE API [(Contribute)](https://github.com/AWeirdScratcher/linex/fork)
- Better type hints than [linelib](https://github.com/AWeirdScratcher/linelib) (my previous work)
<sub>(Not affiliated with The X Corp.)</sub>
## Feel The Simplicity
Code snippets explain more than words. Take a look:
```python
from linex import Client, TextMessageContext
client = Client("channel secret", "channel access token")
@client.event
async def on_ready():
print(f"Logged in as {client.user.name}")
@client.event
async def on_text(ctx: TextMessageContext):
await ctx.reply("Hello, World!")
client.run()
```
That's it. Say no more to additional setups — they're so annoying!
## Documentation
Currently, there is no documentation for LineX — yet I'm working on it. In the meantime, type hints and code editors are the best documentation sources.
## Extensions
<div align="center">
### Notify Support
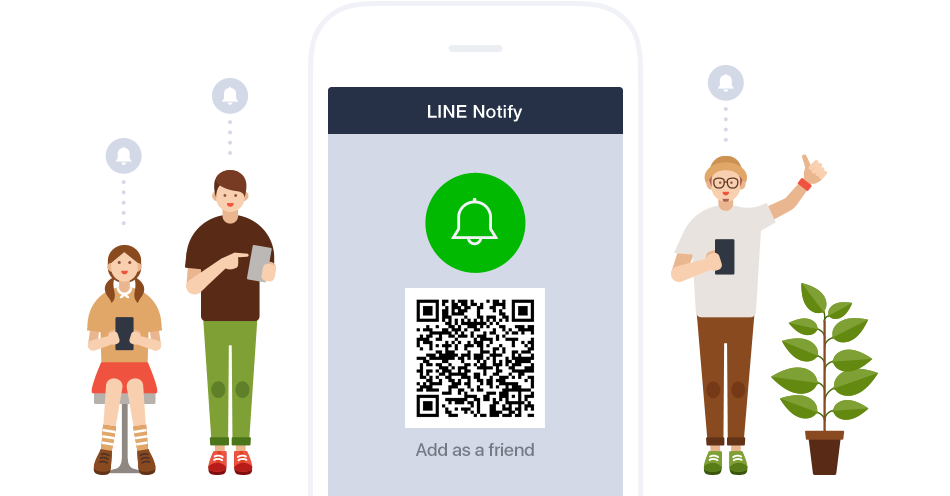
</div>
LineX also supports LINE notify, including push message sending and OAuth2.
Here's a simple notify bot:
```python
from linex.ext import Notify
notify = Notify("access token")
notify.notify_sync(
"Hello, World!"
)
```
### Locale
Have users that use different languages? Try out the `Locale` extension.
First, define a file structure like so:
```haskell
i18n/
├─ _meta.json
├─ food.json
```
Inside of `_meta.json`, define the available locales:
```json
{
"locales": ["en-US", "zh-Hant"]
}
```
The locales above are just examples.
Then, create any JSON file with a specific topic (category) under the directory that stores locale strings.
In this case, `food.json` would look like:
```json
{
"pizza": {
"en-US": "pizza",
"zh-Hant": "披薩"
},
"describe": {
"en-US": "{food} tastes good!",
"zh-Hant": "{food} 很好吃!"
}
}
```
The first key (`pizza`) and the second one (`describe`) stores locale strings with keys defined in the `locales` field in `_meta.json`.
Additionally, if you add texts surrounded by `{}`, it would be considered as an argument that's ready to be passed in. In the above example, `"{food} tastes good!"`, implies that the `{food}` field would be replaced with a food name later in the Python code.
Finally, define our LINE bot:
```python
from linex import Client
from linex.ext import Locale
client = Client("channel secret", "channel access token")
locale = Locale(
"i18n", # the locale strings directory
sorted_by="categories" # files split by categories
)
@client.event
async def on_text(ctx):
loc = await locale(ctx) # get locale for this user
await ctx.reply(
loc(
"food/describe", # use <category>/<key name> to get
food=loc("food/pizza") # the argument (pizza)
)
)
client.run()
```
***
(c) 2023 AWeirdScratcher (AWeirdDev)
Raw data
{
"_id": null,
"home_page": "",
"name": "linex",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.9",
"maintainer_email": "",
"keywords": "line,bot,linebot,sdk,async",
"author": "",
"author_email": "AWeirdDev <aweirdscratcher@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/91/3b/93c36e8c4293e93e8a05857ec4c52e735dcb4b40d157dbe684c36d6f677e/linex-0.2.tar.gz",
"platform": null,
"description": "# LineX\n\nThe fast and easy-to-use LINE bot SDK. Focus on the content, we do the rest.\n\nIt's (or it has):\n\n- Based on [FastAPI](https://fastapi.tiangolo.com)\n- Feature-rich\n- Async-ready\n- Around 60% coverage of the LINE API [(Contribute)](https://github.com/AWeirdScratcher/linex/fork)\n- Better type hints than [linelib](https://github.com/AWeirdScratcher/linelib) (my previous work)\n\n<sub>(Not affiliated with The X Corp.)</sub>\n\n## Feel The Simplicity\n\nCode snippets explain more than words. Take a look:\n\n```python\nfrom linex import Client, TextMessageContext\n\nclient = Client(\"channel secret\", \"channel access token\")\n\n@client.event\nasync def on_ready():\n print(f\"Logged in as {client.user.name}\")\n\n@client.event\nasync def on_text(ctx: TextMessageContext):\n await ctx.reply(\"Hello, World!\")\n\nclient.run()\n```\n\nThat's it. Say no more to additional setups \u2014 they're so annoying!\n\n## Documentation\n\nCurrently, there is no documentation for LineX \u2014 yet I'm working on it. In the meantime, type hints and code editors are the best documentation sources.\n\n## Extensions\n\n<div align=\"center\">\n\n### Notify Support\n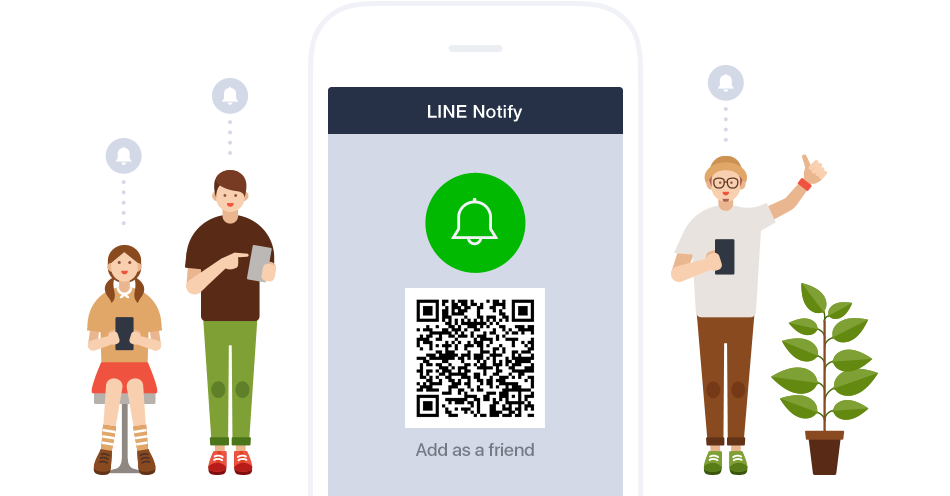\n\n\n</div>\n\nLineX also supports LINE notify, including push message sending and OAuth2.\n\nHere's a simple notify bot:\n\n```python\nfrom linex.ext import Notify\n\nnotify = Notify(\"access token\")\n\nnotify.notify_sync(\n \"Hello, World!\"\n)\n```\n\n### Locale\n\nHave users that use different languages? Try out the `Locale` extension.\n\nFirst, define a file structure like so:\n\n```haskell\ni18n/\n\u251c\u2500 _meta.json\n\u251c\u2500 food.json\n```\n\nInside of `_meta.json`, define the available locales:\n\n```json\n{\n \"locales\": [\"en-US\", \"zh-Hant\"]\n}\n```\n\nThe locales above are just examples.\n\nThen, create any JSON file with a specific topic (category) under the directory that stores locale strings.\n\nIn this case, `food.json` would look like:\n\n```json\n{\n \"pizza\": {\n \"en-US\": \"pizza\",\n \"zh-Hant\": \"\u62ab\u85a9\"\n },\n \"describe\": {\n \"en-US\": \"{food} tastes good!\",\n \"zh-Hant\": \"{food} \u5f88\u597d\u5403\uff01\"\n }\n}\n```\n\nThe first key (`pizza`) and the second one (`describe`) stores locale strings with keys defined in the `locales` field in `_meta.json`.\n\nAdditionally, if you add texts surrounded by `{}`, it would be considered as an argument that's ready to be passed in. In the above example, `\"{food} tastes good!\"`, implies that the `{food}` field would be replaced with a food name later in the Python code.\n\nFinally, define our LINE bot:\n\n```python\nfrom linex import Client\nfrom linex.ext import Locale\n\nclient = Client(\"channel secret\", \"channel access token\")\nlocale = Locale(\n \"i18n\", # the locale strings directory\n sorted_by=\"categories\" # files split by categories\n)\n\n@client.event\nasync def on_text(ctx):\n loc = await locale(ctx) # get locale for this user\n \n await ctx.reply(\n loc(\n \"food/describe\", # use <category>/<key name> to get\n food=loc(\"food/pizza\") # the argument (pizza)\n )\n )\n\nclient.run()\n```\n\n***\n\n(c) 2023 AWeirdScratcher (AWeirdDev)\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2023 AWeirdScratcher Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "The fast and easy-to-use LINE bot SDK.",
"version": "0.2",
"project_urls": {
"Bug Tracker": "https://github.com/AWeirdScratcher/linex/issues",
"Homepage": "https://github.com/AWeirdScratcher/linex"
},
"split_keywords": [
"line",
"bot",
"linebot",
"sdk",
"async"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "913b93c36e8c4293e93e8a05857ec4c52e735dcb4b40d157dbe684c36d6f677e",
"md5": "b40bbcc46a148c8f40f490094a6d4077",
"sha256": "93e8687f4a9be5f398fb125247766c647474385c0870234f93b3554056ee1377"
},
"downloads": -1,
"filename": "linex-0.2.tar.gz",
"has_sig": false,
"md5_digest": "b40bbcc46a148c8f40f490094a6d4077",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.9",
"size": 37874,
"upload_time": "2023-09-02T12:02:05",
"upload_time_iso_8601": "2023-09-02T12:02:05.300126Z",
"url": "https://files.pythonhosted.org/packages/91/3b/93c36e8c4293e93e8a05857ec4c52e735dcb4b40d157dbe684c36d6f677e/linex-0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-02 12:02:05",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "AWeirdScratcher",
"github_project": "linex",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "linex"
}