Name | locklib JSON |
Version |
0.0.16
JSON |
| download |
home_page | None |
Summary | When there are not enough locks from the standard library |
upload_time | 2024-09-06 16:14:52 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.7 |
license | None |
keywords |
locks
mutexes
threading
protocols
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
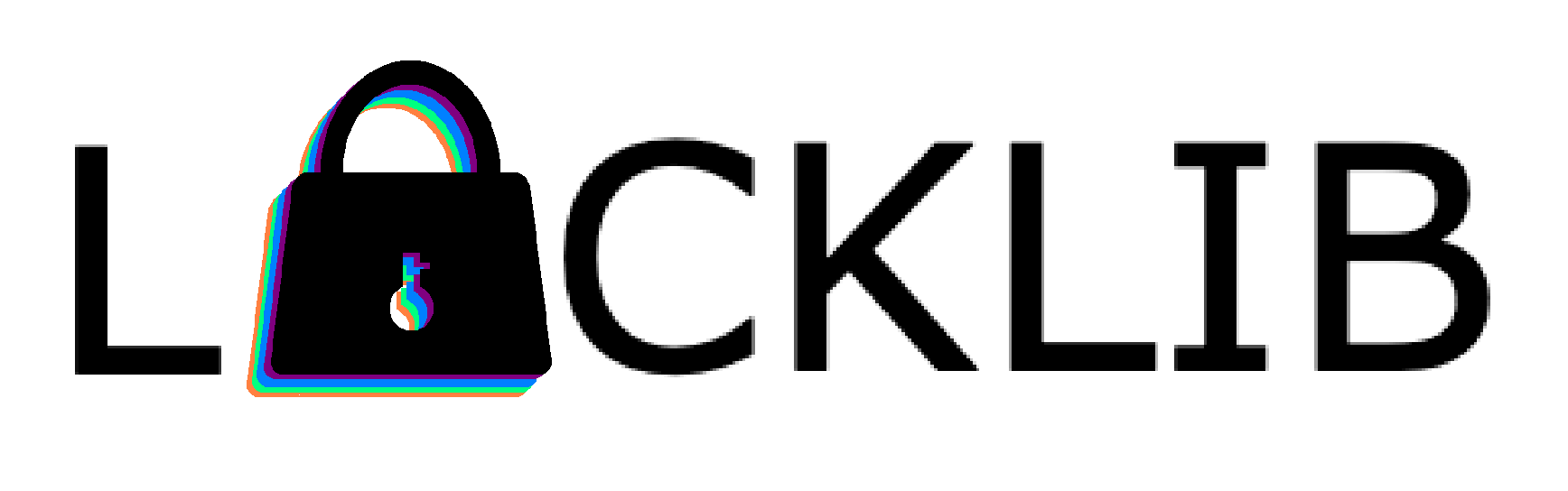
[](https://pepy.tech/project/locklib)
[](https://pepy.tech/project/locklib)
[](https://codecov.io/gh/pomponchik/locklib)
[](https://github.com/boyter/scc/)
[](https://hitsofcode.com/github/pomponchik/locklib/view?branch=main)
[](https://github.com/pomponchik/locklib/actions/workflows/tests_and_coverage.yml)
[](https://pypi.python.org/pypi/locklib)
[](https://badge.fury.io/py/locklib)
[](http://mypy-lang.org/)
[](https://github.com/astral-sh/ruff)
It contains several useful additions to the standard thread synchronization tools, such as lock protocols and locks with advanced functionality.
## Table of contents
- [**Installation**](#installation)
- [**Lock protocols**](#lock-protocols)
- [**SmartLock - deadlock is impossible with it**](#smartlock---deadlock-is-impossible-with-it)
## Installation
Get the `locklib` from the [pypi](https://pypi.org/project/locklib/):
```bash
pip install locklib
```
... or directly from git:
```bash
pip install git+https://github.com/pomponchik/locklib.git
```
You can also quickly try out this and other packages without having to install using [instld](https://github.com/pomponchik/instld).
## Lock protocols
Protocols are needed so that you can write typed code without being bound to specific classes. Protocols from this library allow you to "equalize" locks from the standard library and third-party locks, including those provided by this library.
We consider the basic characteristic of the lock protocol to be the presence of two methods for an object:
```python
def acquire() -> None: pass
def release() -> None: pass
```
All the locks from the standard library correspond to this, as well as the locks presented in this one.
To check for compliance with this minimum standard, `locklib` contains the `LockProtocol`. You can check for yourself that all the locks match it:
```python
from multiprocessing import Lock as MLock
from threading import Lock as TLock, RLock as TRLock
from asyncio import Lock as ALock
from locklib import SmartLock, LockProtocol
print(isinstance(MLock(), LockProtocol)) # True
print(isinstance(TLock(), LockProtocol)) # True
print(isinstance(TRLock(), LockProtocol)) # True
print(isinstance(ALock(), LockProtocol)) # True
print(isinstance(SmartLock(), LockProtocol)) # True
```
However! Most idiomatic python code using locks uses them as context managers. If your code is like that too, you can use one of the two inheritors of the regular `LockProtocol`: `ContextLockProtocol` or `AsyncContextLockProtocol`. Thus, the protocol inheritance hierarchy looks like this:
```
LockProtocol
├── ContextLockProtocol
└── AsyncContextLockProtocol
```
`ContextLockProtocol` describes the objects described by `LockProtocol`, which are also [context managers](https://docs.python.org/3/library/stdtypes.html#typecontextmanager). `AsyncContextLockProtocol`, by analogy, describes objects that are instances of `LockProtocol`, as well as [asynchronous context managers](https://docs.python.org/3/reference/datamodel.html#async-context-managers).
Almost all the locks from the standard library are instances of `ContextLockProtocol`, as well as `SmartLock`.
```python
from multiprocessing import Lock as MLock
from threading import Lock as TLock, RLock as TRLock
from locklib import SmartLock, ContextLockProtocol
print(isinstance(MLock(), ContextLockProtocol)) # True
print(isinstance(TLock(), ContextLockProtocol)) # True
print(isinstance(TRLock(), ContextLockProtocol)) # True
print(isinstance(SmartLock(), ContextLockProtocol)) # True
```
However, the [`Lock` from asyncio](https://docs.python.org/3/library/asyncio-sync.html#asyncio.Lock) belongs to a separate category and `AsyncContextLockProtocol` is needed to describe it:
```python
from asyncio import Lock
from locklib import AsyncContextLockProtocol
print(isinstance(Lock(), AsyncContextLockProtocol)) # True
```
If you use type hints and static verification tools like [mypy](https://github.com/python/mypy), we highly recommend using the narrowest of the presented categories for lock protocols, which describe the requirements for your locales.
## `SmartLock` - deadlock is impossible with it
`locklib` contains a lock that cannot get into the [deadlock](https://en.wikipedia.org/wiki/Deadlock) - `SmartLock`, based on [Wait-for Graph](https://en.wikipedia.org/wiki/Wait-for_graph). You can use it as a usual [```Lock``` from the standard library](https://docs.python.org/3/library/threading.html#lock-objects). Let's check that it can protect us from the [race condition](https://en.wikipedia.org/wiki/Race_condition) in the same way:
```python
from threading import Thread
from locklib import SmartLock
lock = SmartLock()
counter = 0
def function():
global counter
for _ in range(1000):
with lock:
counter += 1
thread_1 = Thread(target=function)
thread_2 = Thread(target=function)
thread_1.start()
thread_2.start()
assert counter == 2000
```
Yeah, in this case the lock helps us not to get a race condition, as the standard ```Lock``` does. But! Let's trigger a deadlock and look what happens:
```python
from threading import Thread
from locklib import SmartLock
lock_1 = SmartLock()
lock_2 = SmartLock()
def function_1():
while True:
with lock_1:
with lock_2:
pass
def function_2():
while True:
with lock_2:
with lock_1:
pass
thread_1 = Thread(target=function_1)
thread_2 = Thread(target=function_2)
thread_1.start()
thread_2.start()
```
And... We have an exception like this:
```
...
locklib.errors.DeadLockError: A cycle between 1970256th and 1970257th threads has been detected.
```
Deadlocks are impossible for this lock!
If you want to catch the exception, import this from the `locklib` too:
```python
from locklib import DeadLockError
```
Raw data
{
"_id": null,
"home_page": null,
"name": "locklib",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": null,
"keywords": "locks, mutexes, threading, protocols",
"author": null,
"author_email": "Evgeniy Blinov <zheni-b@yandex.ru>",
"download_url": "https://files.pythonhosted.org/packages/3d/c5/f3d5b8635cd4ac93ea27843dd80c5d5d5c85a3d50974f6c9fc668408865b/locklib-0.0.16.tar.gz",
"platform": null,
"description": "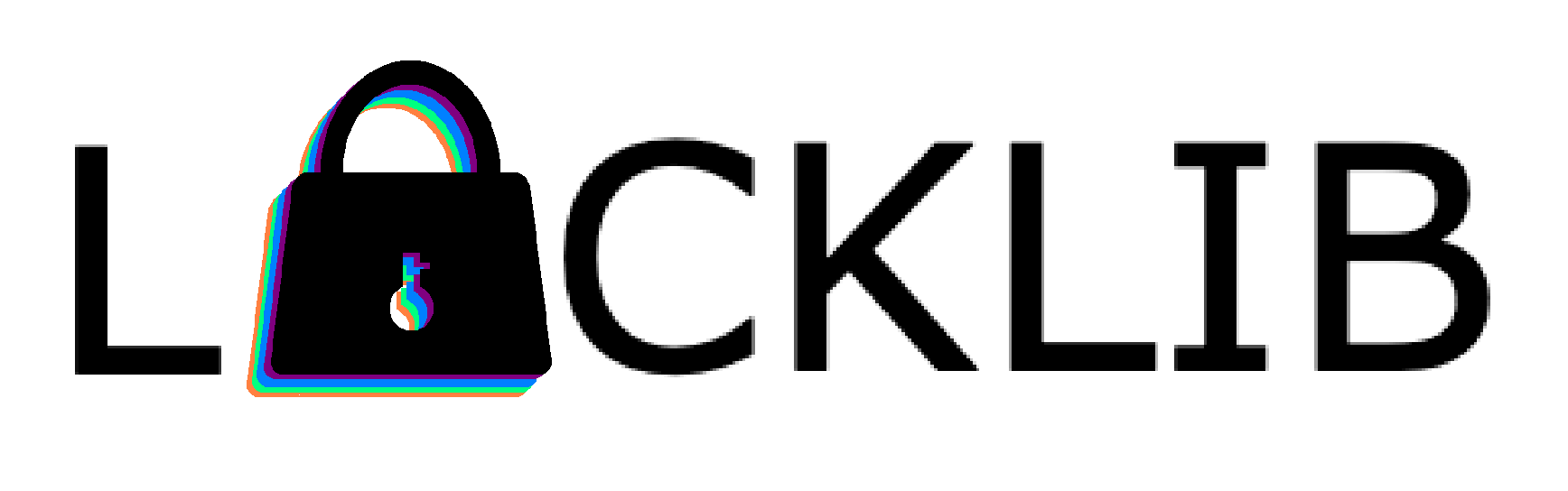\n\n[](https://pepy.tech/project/locklib)\n[](https://pepy.tech/project/locklib)\n[](https://codecov.io/gh/pomponchik/locklib)\n[](https://github.com/boyter/scc/)\n[](https://hitsofcode.com/github/pomponchik/locklib/view?branch=main)\n[](https://github.com/pomponchik/locklib/actions/workflows/tests_and_coverage.yml)\n[](https://pypi.python.org/pypi/locklib)\n[](https://badge.fury.io/py/locklib)\n[](http://mypy-lang.org/)\n[](https://github.com/astral-sh/ruff)\n\nIt contains several useful additions to the standard thread synchronization tools, such as lock protocols and locks with advanced functionality.\n\n\n## Table of contents\n\n- [**Installation**](#installation)\n- [**Lock protocols**](#lock-protocols)\n- [**SmartLock - deadlock is impossible with it**](#smartlock---deadlock-is-impossible-with-it)\n\n\n## Installation\n\nGet the `locklib` from the [pypi](https://pypi.org/project/locklib/):\n\n```bash\npip install locklib\n```\n\n... or directly from git:\n\n```bash\npip install git+https://github.com/pomponchik/locklib.git\n```\n\nYou can also quickly try out this and other packages without having to install using [instld](https://github.com/pomponchik/instld).\n\n\n## Lock protocols\n\nProtocols are needed so that you can write typed code without being bound to specific classes. Protocols from this library allow you to \"equalize\" locks from the standard library and third-party locks, including those provided by this library.\n\nWe consider the basic characteristic of the lock protocol to be the presence of two methods for an object:\n\n```python\ndef acquire() -> None: pass\ndef release() -> None: pass\n```\n\nAll the locks from the standard library correspond to this, as well as the locks presented in this one.\n\nTo check for compliance with this minimum standard, `locklib` contains the `LockProtocol`. You can check for yourself that all the locks match it:\n\n```python\nfrom multiprocessing import Lock as MLock\nfrom threading import Lock as TLock, RLock as TRLock\nfrom asyncio import Lock as ALock\n\nfrom locklib import SmartLock, LockProtocol\n\nprint(isinstance(MLock(), LockProtocol)) # True\nprint(isinstance(TLock(), LockProtocol)) # True\nprint(isinstance(TRLock(), LockProtocol)) # True\nprint(isinstance(ALock(), LockProtocol)) # True\nprint(isinstance(SmartLock(), LockProtocol)) # True\n```\n\nHowever! Most idiomatic python code using locks uses them as context managers. If your code is like that too, you can use one of the two inheritors of the regular `LockProtocol`: `ContextLockProtocol` or `AsyncContextLockProtocol`. Thus, the protocol inheritance hierarchy looks like this:\n\n```\nLockProtocol\n \u251c\u2500\u2500 ContextLockProtocol\n \u2514\u2500\u2500 AsyncContextLockProtocol\n```\n\n`ContextLockProtocol` describes the objects described by `LockProtocol`, which are also [context managers](https://docs.python.org/3/library/stdtypes.html#typecontextmanager). `AsyncContextLockProtocol`, by analogy, describes objects that are instances of `LockProtocol`, as well as [asynchronous context managers](https://docs.python.org/3/reference/datamodel.html#async-context-managers).\n\nAlmost all the locks from the standard library are instances of `ContextLockProtocol`, as well as `SmartLock`.\n\n```python\nfrom multiprocessing import Lock as MLock\nfrom threading import Lock as TLock, RLock as TRLock\n\nfrom locklib import SmartLock, ContextLockProtocol\n\nprint(isinstance(MLock(), ContextLockProtocol)) # True\nprint(isinstance(TLock(), ContextLockProtocol)) # True\nprint(isinstance(TRLock(), ContextLockProtocol)) # True\nprint(isinstance(SmartLock(), ContextLockProtocol)) # True\n```\n\nHowever, the [`Lock` from asyncio](https://docs.python.org/3/library/asyncio-sync.html#asyncio.Lock) belongs to a separate category and `AsyncContextLockProtocol` is needed to describe it:\n\n```python\nfrom asyncio import Lock\nfrom locklib import AsyncContextLockProtocol\n\nprint(isinstance(Lock(), AsyncContextLockProtocol)) # True\n```\n\nIf you use type hints and static verification tools like [mypy](https://github.com/python/mypy), we highly recommend using the narrowest of the presented categories for lock protocols, which describe the requirements for your locales.\n\n\n## `SmartLock` - deadlock is impossible with it\n\n`locklib` contains a lock that cannot get into the [deadlock](https://en.wikipedia.org/wiki/Deadlock) - `SmartLock`, based on [Wait-for Graph](https://en.wikipedia.org/wiki/Wait-for_graph). You can use it as a usual [```Lock``` from the standard library](https://docs.python.org/3/library/threading.html#lock-objects). Let's check that it can protect us from the [race condition](https://en.wikipedia.org/wiki/Race_condition) in the same way:\n\n```python\nfrom threading import Thread\nfrom locklib import SmartLock\n\nlock = SmartLock()\ncounter = 0\n\ndef function():\n global counter\n\n for _ in range(1000):\n with lock:\n counter += 1\n\nthread_1 = Thread(target=function)\nthread_2 = Thread(target=function)\nthread_1.start()\nthread_2.start()\n\nassert counter == 2000\n```\n\nYeah, in this case the lock helps us not to get a race condition, as the standard ```Lock``` does. But! Let's trigger a deadlock and look what happens:\n\n```python\nfrom threading import Thread\nfrom locklib import SmartLock\n\nlock_1 = SmartLock()\nlock_2 = SmartLock()\n\ndef function_1():\n while True:\n with lock_1:\n with lock_2:\n pass\n\ndef function_2():\n while True:\n with lock_2:\n with lock_1:\n pass\n\nthread_1 = Thread(target=function_1)\nthread_2 = Thread(target=function_2)\nthread_1.start()\nthread_2.start()\n```\n\nAnd... We have an exception like this:\n\n```\n...\nlocklib.errors.DeadLockError: A cycle between 1970256th and 1970257th threads has been detected.\n```\n\nDeadlocks are impossible for this lock!\n\nIf you want to catch the exception, import this from the `locklib` too:\n\n```python\nfrom locklib import DeadLockError\n```\n",
"bugtrack_url": null,
"license": null,
"summary": "When there are not enough locks from the standard library",
"version": "0.0.16",
"project_urls": {
"Source": "https://github.com/pomponchik/locklib",
"Tracker": "https://github.com/pomponchik/locklib/issues"
},
"split_keywords": [
"locks",
" mutexes",
" threading",
" protocols"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fac0feb8e164490c9a11a5a43cba2fa57ab6ef27abecc78cbe08e108db337f5a",
"md5": "bf0df3b6cb1a2e0bd07bfd9080e3b593",
"sha256": "92a1c500d21bcc509cc4fcd64f29dd35d98265c3605ffe581522a0e92bc8ea04"
},
"downloads": -1,
"filename": "locklib-0.0.16-py3-none-any.whl",
"has_sig": false,
"md5_digest": "bf0df3b6cb1a2e0bd07bfd9080e3b593",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 8567,
"upload_time": "2024-09-06T16:14:50",
"upload_time_iso_8601": "2024-09-06T16:14:50.749518Z",
"url": "https://files.pythonhosted.org/packages/fa/c0/feb8e164490c9a11a5a43cba2fa57ab6ef27abecc78cbe08e108db337f5a/locklib-0.0.16-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3dc5f3d5b8635cd4ac93ea27843dd80c5d5d5c85a3d50974f6c9fc668408865b",
"md5": "1bfe1ecdb1434b69981d3d8890922db9",
"sha256": "5ff8ea178bd77a78780a2cec7b6c498b47667635a6cfa1a1a501c3a5738ca048"
},
"downloads": -1,
"filename": "locklib-0.0.16.tar.gz",
"has_sig": false,
"md5_digest": "1bfe1ecdb1434b69981d3d8890922db9",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 8881,
"upload_time": "2024-09-06T16:14:52",
"upload_time_iso_8601": "2024-09-06T16:14:52.173039Z",
"url": "https://files.pythonhosted.org/packages/3d/c5/f3d5b8635cd4ac93ea27843dd80c5d5d5c85a3d50974f6c9fc668408865b/locklib-0.0.16.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-09-06 16:14:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "pomponchik",
"github_project": "locklib",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "locklib"
}