# Multiple ADB logcat with colored output / csv export
## Tested against Windows 10 / Python 3.11 / Anaconda
## pip install logcatdevices
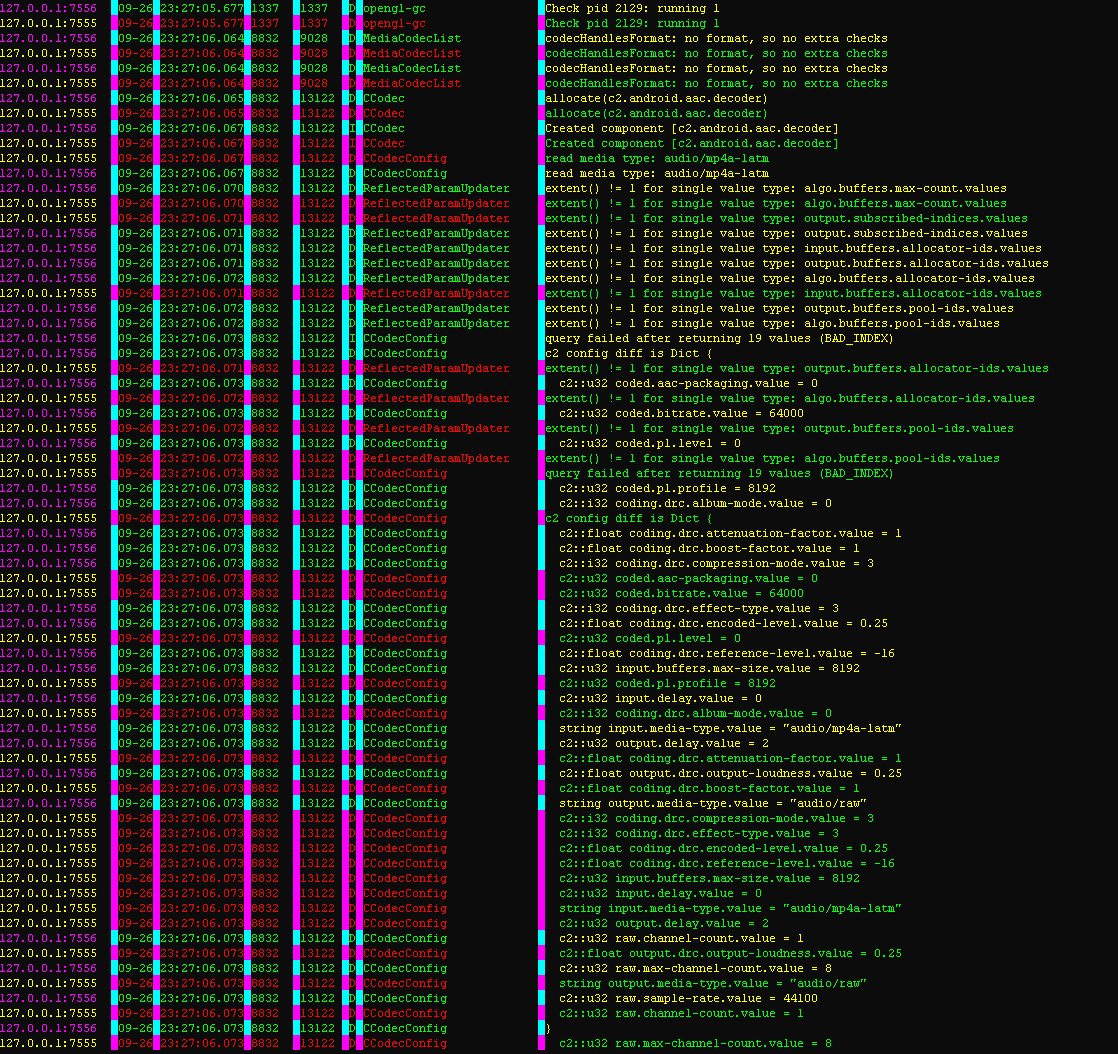
## start_logcat (ctrl+c to stop)
```python
Starts logging Android device logs using ADB.
This function initiates log capturing for specified Android devices using ADB (Android Debug Bridge).
Logs are saved to a CSV file for further analysis.
Args:
adb_path (str): Path to the ADB executable.
csv_output (str): Path to the CSV file where the logs will be saved.
device_serials (str or list): A single device serial number as a string, or a list of serial numbers
for multiple devices. Serial numbers are used to identify connected devices.
print_stdout (bool, optional): If True, prints log messages to the console. Default is True.
clear_log (bool, optional): If True, clears the device log before capturing. Default is True.
ljustserial (int, optional): Left-justifies the device serial number in the printed output. Default is 16.
Returns:
None
Example:
from logcatdevices import start_logcat
androidcsv = "c:\\csvandroisad.csv"
_ = start_logcat(
adb_path=r"C:\Android\android-sdk\platform-tools\adb.exe",
csv_output=androidcsv,
device_serials=[
"127.0.0.1:5565",
"127.0.0.1:7555",
"127.0.0.1:7556",
"emulator-5554",
"emulator-5564",
],
print_stdout=True,
clear_log=True,
ljustserial=16,
)
```
## csv2df
```python
Converts a CSV log file to a pandas DataFrame.
This function reads a CSV file containing Android device log data and converts it into a structured
pandas DataFrame, enabling easy data manipulation and analysis.
Args:
csvfile (str): Path to the CSV log file.
Returns:
pandas.DataFrame: A DataFrame containing log data with the following columns:
- aa_source (str): Source of the log message.
- aa_date (datetime): Date and time of the log message.
- aa_processID (int): Process ID associated with the log message.
- aa_threadID (int): Thread ID associated with the log message.
- aa_logType (str): Type of log message (e.g., 'V', 'D', 'I', 'W', 'E').
- aa_tag (str): Tag associated with the log message.
- aa_message (str): Log message content.
Example:
from logcatdevices import csv2df
androidcsv = "c:\\csvandroisad.csv"
df = csv2df(androidcsv)
print(df.to_string())
```
Raw data
{
"_id": null,
"home_page": "https://github.com/hansalemaos/logcatdevices",
"name": "logcatdevices",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "adb,connect,logcat",
"author": "Johannes Fischer",
"author_email": "aulasparticularesdealemaosp@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/9d/39/374ed57441308c3878e6fb0a0385803d9bad70df2be0f1898731b845ae86/logcatdevices-0.11.tar.gz",
"platform": null,
"description": "\r\n# Multiple ADB logcat with colored output / csv export \r\n\r\n## Tested against Windows 10 / Python 3.11 / Anaconda\r\n\r\n## pip install logcatdevices\r\n\r\n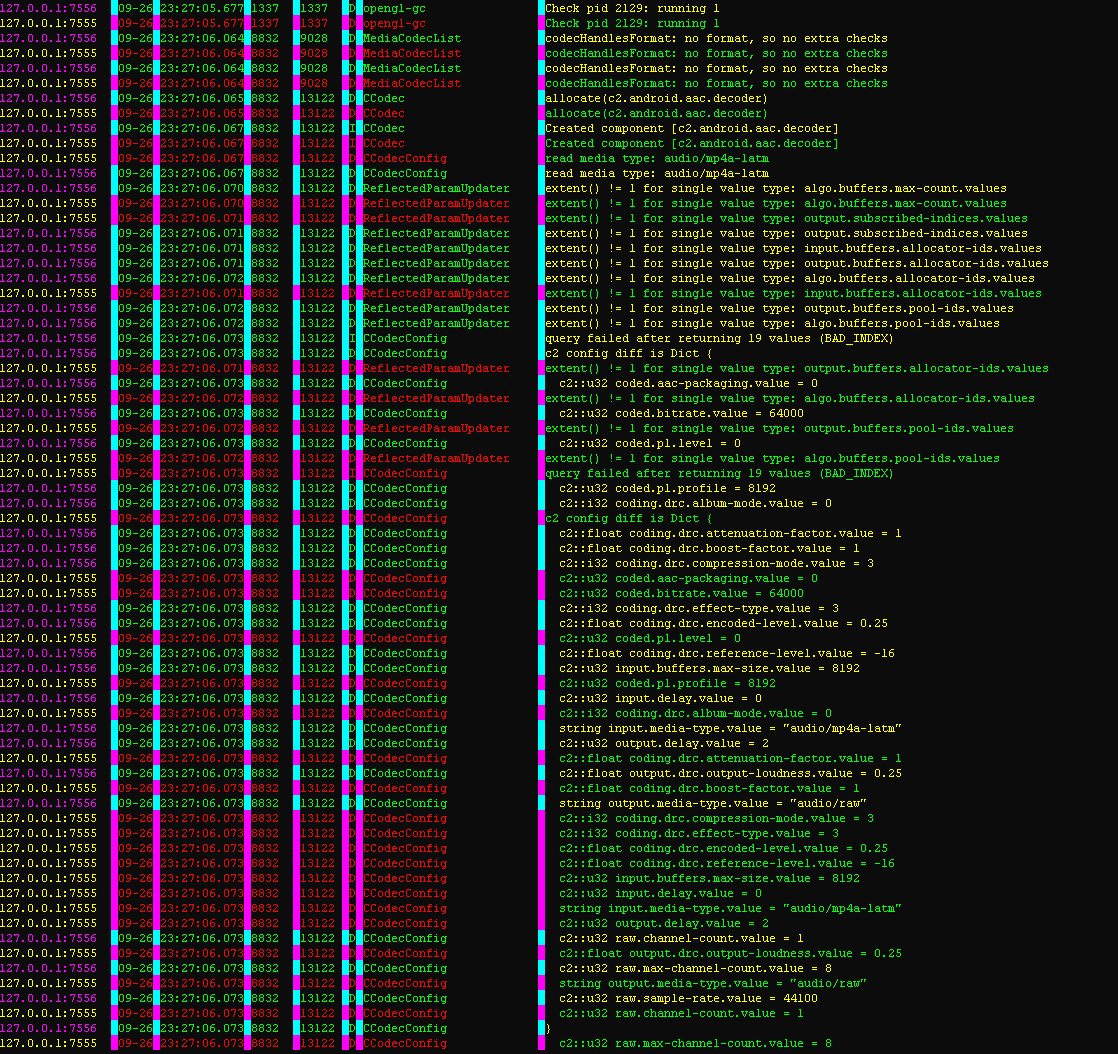\r\n\r\n\r\n\r\n## start_logcat (ctrl+c to stop)\r\n\r\n```python\r\nStarts logging Android device logs using ADB.\r\n\r\nThis function initiates log capturing for specified Android devices using ADB (Android Debug Bridge).\r\nLogs are saved to a CSV file for further analysis.\r\n\r\nArgs:\r\n\tadb_path (str): Path to the ADB executable.\r\n\tcsv_output (str): Path to the CSV file where the logs will be saved.\r\n\tdevice_serials (str or list): A single device serial number as a string, or a list of serial numbers\r\n\t\t\t\t\t\t\t\t for multiple devices. Serial numbers are used to identify connected devices.\r\n\tprint_stdout (bool, optional): If True, prints log messages to the console. Default is True.\r\n\tclear_log (bool, optional): If True, clears the device log before capturing. Default is True.\r\n\tljustserial (int, optional): Left-justifies the device serial number in the printed output. Default is 16.\r\n\r\nReturns:\r\n\tNone\r\n\r\nExample:\r\n\tfrom logcatdevices import start_logcat\r\n\r\n\tandroidcsv = \"c:\\\\csvandroisad.csv\"\r\n\t_ = start_logcat(\r\n\t\tadb_path=r\"C:\\Android\\android-sdk\\platform-tools\\adb.exe\",\r\n\t\tcsv_output=androidcsv,\r\n\t\tdevice_serials=[\r\n\t\t\t\"127.0.0.1:5565\",\r\n\t\t\t\"127.0.0.1:7555\",\r\n\t\t\t\"127.0.0.1:7556\",\r\n\t\t\t\"emulator-5554\",\r\n\t\t\t\"emulator-5564\",\r\n\t\t],\r\n\t\tprint_stdout=True,\r\n\t\tclear_log=True,\r\n\t\tljustserial=16,\r\n\t)\r\n```\r\n\r\n\r\n## csv2df\r\n\r\n\r\n```python\r\nConverts a CSV log file to a pandas DataFrame.\r\n\r\nThis function reads a CSV file containing Android device log data and converts it into a structured\r\npandas DataFrame, enabling easy data manipulation and analysis.\r\n\r\nArgs:\r\n\tcsvfile (str): Path to the CSV log file.\r\n\r\nReturns:\r\n\tpandas.DataFrame: A DataFrame containing log data with the following columns:\r\n\t\t- aa_source (str): Source of the log message.\r\n\t\t- aa_date (datetime): Date and time of the log message.\r\n\t\t- aa_processID (int): Process ID associated with the log message.\r\n\t\t- aa_threadID (int): Thread ID associated with the log message.\r\n\t\t- aa_logType (str): Type of log message (e.g., 'V', 'D', 'I', 'W', 'E').\r\n\t\t- aa_tag (str): Tag associated with the log message.\r\n\t\t- aa_message (str): Log message content.\r\n\r\nExample:\r\n\tfrom logcatdevices import csv2df\r\n\tandroidcsv = \"c:\\\\csvandroisad.csv\"\r\n\tdf = csv2df(androidcsv)\r\n\tprint(df.to_string())\r\n```\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Multiple ADB logcat with colored output / csv export",
"version": "0.11",
"project_urls": {
"Homepage": "https://github.com/hansalemaos/logcatdevices"
},
"split_keywords": [
"adb",
"connect",
"logcat"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a11414a83bf9016a844a1f49f877c48acbf357c94975a2870c7a1c69a953f986",
"md5": "5c7fa4a01416f6071ddb861c83aaca8f",
"sha256": "904661772319848c9d68607eba31d81673945f1eeafc1d38d3261512748d4221"
},
"downloads": -1,
"filename": "logcatdevices-0.11-py3-none-any.whl",
"has_sig": false,
"md5_digest": "5c7fa4a01416f6071ddb861c83aaca8f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 9540,
"upload_time": "2023-09-27T03:48:59",
"upload_time_iso_8601": "2023-09-27T03:48:59.971922Z",
"url": "https://files.pythonhosted.org/packages/a1/14/14a83bf9016a844a1f49f877c48acbf357c94975a2870c7a1c69a953f986/logcatdevices-0.11-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9d39374ed57441308c3878e6fb0a0385803d9bad70df2be0f1898731b845ae86",
"md5": "0520c21e5c538569d8609e47446da1dd",
"sha256": "0762b0edab2dc6f71b8abb3a604ef5c097353cba9b61836ca1d79a1f5e9ef5ee"
},
"downloads": -1,
"filename": "logcatdevices-0.11.tar.gz",
"has_sig": false,
"md5_digest": "0520c21e5c538569d8609e47446da1dd",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 8132,
"upload_time": "2023-09-27T03:49:02",
"upload_time_iso_8601": "2023-09-27T03:49:02.034114Z",
"url": "https://files.pythonhosted.org/packages/9d/39/374ed57441308c3878e6fb0a0385803d9bad70df2be0f1898731b845ae86/logcatdevices-0.11.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-27 03:49:02",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "hansalemaos",
"github_project": "logcatdevices",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "logcatdevices"
}