# Mail Reply Parser 📧🐍
[](https://www.python.org/)
[](https://github.com/alfonsrv/mail-parser-reply)
### Multi-language email reply parsing for international environments 🌍
Mail clients handle reply formatting differently, making reliable parsing difficult. Thank god we have
[standards](https://xkcd.com/927/). This library splits *text-based* emails into separate replies based on common
headers produced by different, multilingual clients usually indicating separation.
Replies can either present the whole mail message body, or strip headers, signatures and common disclaimers if required.
Currently supported languages are:
* Dutch (`nl`) 🇳🇱
* English (`en`) 🇬🇧
* French (`fr`) 🇫🇷
* German (`de`) 🇩🇪
* Italian (`it`) 🇮🇹
* Japanese (`ja`) 🇯🇵
* Polish (`pl`) 🇵🇱
🏳️🌈 **Adding more languages is quite easy!**
This is an improved Python implementation of GitHub's Ruby-based [email_reply_parser](https://github.com/github/email_reply_parser/)
and an adaptation of Zapier's [email-reply-parser](https://github.com/zapier/email-reply-parser) which both split the
mails in fragments instead of distinct replies. They also only support English.
## ⭐ Features
⭐ Easy to implement
⭐ Multilanguage Support
⭐ Text-based mail parsing
⭐ Detect headers, signatures and disclaimers
⭐ Fully type annotated
⭐ Easy-to-read code and well-tested
## Overview 🔭
This library makes it easy to split an incoming mail into replies, making working with emails much more manageable
and easily providing the text content for each reply – with or without signatures, disclaimers and headers.
For example, it can turn the following email:
```
Awesome! I haven't had another problem with it.
Thanks,
alfonsrv
On Wed, Dec 20, 2023 at 13:37, RAUSYS <info@rausys.de> wrote:
> The good news is that I've found a much better query for lastLocation.
> It should run much faster now. Can you double-check?
```
Into just the replied text content:
```
Awesome! I haven't had another problem with it.
```
## Get started 👾
### Installation
```bash
pip install mail-parser-reply
```
### Parse Replies
```python
from mailparser_reply import EmailReplyParser
mail_body = 'foobar'; languages = ['en', 'de']
mail_message = EmailReplyParser(languages=languages).read(text=mail_body)
print(mail_message.replies)
```
*Or* get only the latest reply using:
```python
latest_reply = EmailReplyParser(languages=languages).parse_reply(text=mail_body)
```
### Parser API
```
EmailMessage.text: Mail body
EmailMessage.languages: Languages to use for parsing headers
EmailMessage.replies: List of EmailReply; single parsed replies
EmailMessage.include_english: Always include English language for parsing
EmailMessage.default_language: Default language to use if language dictionary
doesn't include any other language codes
EmailMessage.HEADER_REGEX: RegEx for identifying headers, separating mails
EmailMessage.SIGNATURE_REGEX: RegEx for identifying signatures
EmailMessage.DISCLAIMERS_REGEX: RegEx for identifying disclaimers
EmailMessage.read(): Parse EmailMessage.text to EmailReply which
are then stored in EmailMessage.replies
```
```
EmailReply.content: Unprocessed mail body with headers, signatures, disclaimers
EmailReply.body: Mail body without headers, signatures, disclaimers
EmailReply.full_body: Mail body; just without headers
EmailReply.headers: Identified Headers
EmailReply.signatures: Identified Signatures
EmailReply.disclaimers: Identified disclaimers
```
---
[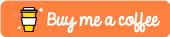](https://www.buymeacoffee.com/alfonsrv)
Raw data
{
"_id": null,
"home_page": "https://github.com/alfonsrv/mail-parser-reply",
"name": "mail-parser-reply",
"maintainer": "Alfons R.",
"docs_url": null,
"requires_python": null,
"maintainer_email": "alfonsrv@protonmail.com",
"keywords": "mail, email, parser",
"author": "Alfons R.",
"author_email": "alfonsrv@protonmail.com",
"download_url": "https://files.pythonhosted.org/packages/63/34/45e2dcf227ee8e97e2ec8ec53abbdc96528e154d84f77cf7d327a8e11c38/mail_parser_reply-1.32.tar.gz",
"platform": null,
"description": "# Mail Reply Parser \ud83d\udce7\ud83d\udc0d \n\n[](https://www.python.org/) \n[](https://github.com/alfonsrv/mail-parser-reply)\n\n\n### Multi-language email reply parsing for international environments \ud83c\udf0d\n\nMail clients handle reply formatting differently, making reliable parsing difficult. Thank god we have \n[standards](https://xkcd.com/927/). This library splits *text-based* emails into separate replies based on common \nheaders produced by different, multilingual clients usually indicating separation.\n\nReplies can either present the whole mail message body, or strip headers, signatures and common disclaimers if required. \nCurrently supported languages are: \n\n* Dutch (`nl`) \ud83c\uddf3\ud83c\uddf1\n* English (`en`) \ud83c\uddec\ud83c\udde7\n* French (`fr`) \ud83c\uddeb\ud83c\uddf7\n* German (`de`) \ud83c\udde9\ud83c\uddea\n* Italian (`it`) \ud83c\uddee\ud83c\uddf9\n* Japanese (`ja`) \ud83c\uddef\ud83c\uddf5\n* Polish (`pl`) \ud83c\uddf5\ud83c\uddf1 \n\n\n\ud83c\udff3\ufe0f\u200d\ud83c\udf08 **Adding more languages is quite easy!**\n\nThis is an improved Python implementation of GitHub's Ruby-based [email_reply_parser](https://github.com/github/email_reply_parser/) \nand an adaptation of Zapier's [email-reply-parser](https://github.com/zapier/email-reply-parser) which both split the \nmails in fragments instead of distinct replies. They also only support English.\n\n\n## \u2b50 Features\n\n\u2b50 Easy to implement \n\u2b50 Multilanguage Support \n\u2b50 Text-based mail parsing \n\u2b50 Detect headers, signatures and disclaimers \n\u2b50 Fully type annotated \n\u2b50 Easy-to-read code and well-tested \n\n\n## Overview \ud83d\udd2d\n\nThis library makes it easy to split an incoming mail into replies, making working with emails much more manageable\nand easily providing the text content for each reply \u2013 with or without signatures, disclaimers and headers.\n\nFor example, it can turn the following email:\n\n```\nAwesome! I haven't had another problem with it.\n\nThanks,\nalfonsrv\n\nOn Wed, Dec 20, 2023 at 13:37, RAUSYS <info@rausys.de> wrote:\n\n> The good news is that I've found a much better query for lastLocation.\n> It should run much faster now. Can you double-check?\n```\n\nInto just the replied text content:\n\n```\nAwesome! I haven't had another problem with it.\n```\n\n\n## Get started \ud83d\udc7e\n\n### Installation\n\n```bash\npip install mail-parser-reply\n```\n\n### Parse Replies\n\n```python\nfrom mailparser_reply import EmailReplyParser\n\nmail_body = 'foobar'; languages = ['en', 'de']\nmail_message = EmailReplyParser(languages=languages).read(text=mail_body)\nprint(mail_message.replies)\n```\n\n*Or* get only the latest reply using:\n\n```python\nlatest_reply = EmailReplyParser(languages=languages).parse_reply(text=mail_body)\n```\n\n\n### Parser API\n\n```\nEmailMessage.text: Mail body\nEmailMessage.languages: Languages to use for parsing headers\nEmailMessage.replies: List of EmailReply; single parsed replies\nEmailMessage.include_english: Always include English language for parsing\nEmailMessage.default_language: Default language to use if language dictionary \n doesn't include any other language codes\n\nEmailMessage.HEADER_REGEX: RegEx for identifying headers, separating mails\nEmailMessage.SIGNATURE_REGEX: RegEx for identifying signatures\nEmailMessage.DISCLAIMERS_REGEX: RegEx for identifying disclaimers\n\nEmailMessage.read(): Parse EmailMessage.text to EmailReply which\n are then stored in EmailMessage.replies\n```\n\n```\nEmailReply.content: Unprocessed mail body with headers, signatures, disclaimers\nEmailReply.body: Mail body without headers, signatures, disclaimers\nEmailReply.full_body: Mail body; just without headers\n\nEmailReply.headers: Identified Headers\nEmailReply.signatures: Identified Signatures\nEmailReply.disclaimers: Identified disclaimers\n```\n\n\n\n---\n\n[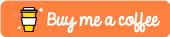](https://www.buymeacoffee.com/alfonsrv) \n",
"bugtrack_url": null,
"license": "MIT",
"summary": "\ud83d\udce7 Email reply parser library for Python with multi-language support",
"version": "1.32",
"project_urls": {
"Homepage": "https://github.com/alfonsrv/mail-parser-reply"
},
"split_keywords": [
"mail",
" email",
" parser"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4cc43d85e251dbc5ea4c64afae89be462c416f46eacd118441f6f78d5b7b217c",
"md5": "844eb6d8a75b89ae2879761a62a15c4a",
"sha256": "77eb8ac6687b57cf1ab1196fde8f697eea6165eee86de39d468567bb0e47666d"
},
"downloads": -1,
"filename": "mail_parser_reply-1.32-py3-none-any.whl",
"has_sig": false,
"md5_digest": "844eb6d8a75b89ae2879761a62a15c4a",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 10732,
"upload_time": "2024-10-09T16:50:20",
"upload_time_iso_8601": "2024-10-09T16:50:20.137417Z",
"url": "https://files.pythonhosted.org/packages/4c/c4/3d85e251dbc5ea4c64afae89be462c416f46eacd118441f6f78d5b7b217c/mail_parser_reply-1.32-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "633445e2dcf227ee8e97e2ec8ec53abbdc96528e154d84f77cf7d327a8e11c38",
"md5": "cd2211c701d584dee1a8749fe967be80",
"sha256": "17422af5a2564b7ea057f96eb69a519ce9a9a36ac53a64d29038ad1e3a555fce"
},
"downloads": -1,
"filename": "mail_parser_reply-1.32.tar.gz",
"has_sig": false,
"md5_digest": "cd2211c701d584dee1a8749fe967be80",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 12221,
"upload_time": "2024-10-09T16:50:21",
"upload_time_iso_8601": "2024-10-09T16:50:21.326288Z",
"url": "https://files.pythonhosted.org/packages/63/34/45e2dcf227ee8e97e2ec8ec53abbdc96528e154d84f77cf7d327a8e11c38/mail_parser_reply-1.32.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-09 16:50:21",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "alfonsrv",
"github_project": "mail-parser-reply",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "mail-parser-reply"
}