MarkdownMail
============
Purpose
-------
Send e-mails with generated html content.
The content has to be written in Markdown syntax. The text part of the e-mail
will be filled verbatim; the html part will be a converted HTML from the
Markdown content.
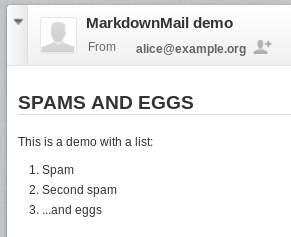
Install
-------
`$ pip install markdownmail`
Basic Usage
-----------
```python
import markdownmail
CONTENT = u"""
SPAMS AND EGGS
==============
This is a demo with a list:
1. Spam
2. Second spam
3. ...and eggs
"""
email = markdownmail.MarkdownMail(
from_addr=u'alice@example.org',
to_addr=u'bob@example.org',
subject=u'MarkdownMail demo',
content=CONTENT
)
email.send('localhost')
```
Content must be unicode.
More infos
----------
Additional informations are addable:
```python
email = markdownmail.MarkdownMail(
from_addr=(u'alice@example.org', u'Alice'),
to_addr=(u'bob@example.org', u'Bob'),
subject=u'MarkdownMail demo',
content=CONTENT
)
```
`cc_addr` and `bcc_addr` are optional.
The `from_addr`, `to_addr`, `cc_addr` and `bcc_addr` parameters are the same as
[Enveloppe](http://pypi.org/pypi/Envelopes/) library.
Change SMTP port:
```python
email.send("example.org", port=3325)
```
Change SMTP login and password:
```python
email.send("example.org", login="user", password="password")
```
Use TLS:
```python
email.send("example.org", tls=True)
```
Style
-----
A default CSS is automatically added to the e-mail. It includes a font sans serif and minor improvements.
To override the default CSS, pass a string including the style to the `css` optional parameter of `MardownMail`:
```python
import markdownmail
email = markdownmail.MarkdownMail(
from_addr=u'alice@example.org',
to_addr=u'bob@example.org',
subject=u'MarkdownMail demo',
content="CONTENT",
css="font-family:monospace; color:green;"
)
```
Run tests
---------
Tox is automatically installed in virtualenvs before executing the tests.
Execute them with:
`$ python setup.py test`
Disable sending e-mails in your tests
-------------------------------------
The e-mail is not send if the parameter passed to `send()` method is an instance of `NullServer`.
```python
email = markdownmail.MarkdownMail(
#params
)
email.send(markdownmail.NullServer())
```
Assert about e-mails in your tests
----------------------------------
Subclassing `NullServer` allows to provide a custom behaviour in the `check()`
method:
```python
class MyServer(markdownmail.NullServer):
def check(self, email):
assert u'bob@example.org' == email.to_addr[0]
email.send(MyServer())
```
Useful links
------------
[Envelopes library](https://pypi.org/pypi/Envelopes/0.4)
(MardownMail is a wrapper around Envelopes library.)
[Markdown syntax](https://daringfireball.net/projects/markdown/syntax)
Raw data
{
"_id": null,
"home_page": "https://gitlab.com/yaal/markdownmail",
"name": "markdownmail",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "mail markdown yaal",
"author": "Yaal Team",
"author_email": "contact@yaal.coop",
"download_url": "https://files.pythonhosted.org/packages/a8/5f/efbc0edc1bd7cf1ae3a04be2862085bdeb4b7561f1c0d407a2535d8b9f94/markdownmail-0.11.1.tar.gz",
"platform": null,
"description": "MarkdownMail\n============\n\nPurpose\n-------\n\nSend e-mails with generated html content.\n\nThe content has to be written in Markdown syntax. The text part of the e-mail\nwill be filled verbatim; the html part will be a converted HTML from the\nMarkdown content.\n\n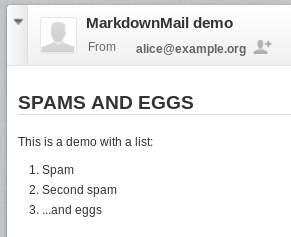\n\nInstall\n-------\n\n`$ pip install markdownmail`\n\n\nBasic Usage\n-----------\n\n```python\nimport markdownmail\n\nCONTENT = u\"\"\"\nSPAMS AND EGGS\n==============\n\nThis is a demo with a list:\n\n1. Spam\n2. Second spam\n3. ...and eggs\n\"\"\"\n\nemail = markdownmail.MarkdownMail(\n from_addr=u'alice@example.org',\n to_addr=u'bob@example.org',\n subject=u'MarkdownMail demo',\n content=CONTENT\n)\n\nemail.send('localhost')\n```\n\nContent must be unicode.\n\n\nMore infos\n----------\n\nAdditional informations are addable:\n\n```python\nemail = markdownmail.MarkdownMail(\n from_addr=(u'alice@example.org', u'Alice'),\n to_addr=(u'bob@example.org', u'Bob'),\n subject=u'MarkdownMail demo',\n content=CONTENT\n)\n```\n\n`cc_addr` and `bcc_addr` are optional.\nThe `from_addr`, `to_addr`, `cc_addr` and `bcc_addr` parameters are the same as\n[Enveloppe](http://pypi.org/pypi/Envelopes/) library.\n\n\nChange SMTP port:\n\n```python\nemail.send(\"example.org\", port=3325)\n```\n\nChange SMTP login and password:\n\n```python\nemail.send(\"example.org\", login=\"user\", password=\"password\")\n```\n\nUse TLS:\n\n```python\nemail.send(\"example.org\", tls=True)\n```\n\nStyle\n-----\n\nA default CSS is automatically added to the e-mail. It includes a font sans serif and minor improvements.\n\nTo override the default CSS, pass a string including the style to the `css` optional parameter of `MardownMail`:\n\n```python\nimport markdownmail\n\nemail = markdownmail.MarkdownMail(\n from_addr=u'alice@example.org',\n to_addr=u'bob@example.org',\n subject=u'MarkdownMail demo',\n content=\"CONTENT\",\n css=\"font-family:monospace; color:green;\"\n)\n```\n\nRun tests\n---------\n\nTox is automatically installed in virtualenvs before executing the tests.\nExecute them with:\n\n`$ python setup.py test`\n\n\nDisable sending e-mails in your tests\n-------------------------------------\n\nThe e-mail is not send if the parameter passed to `send()` method is an instance of `NullServer`.\n\n```python\nemail = markdownmail.MarkdownMail(\n #params\n)\n\nemail.send(markdownmail.NullServer())\n```\n\nAssert about e-mails in your tests\n----------------------------------\n\nSubclassing `NullServer` allows to provide a custom behaviour in the `check()`\nmethod:\n\n```python\nclass MyServer(markdownmail.NullServer):\n def check(self, email):\n assert u'bob@example.org' == email.to_addr[0]\n\nemail.send(MyServer())\n```\n\n\nUseful links\n------------\n\n[Envelopes library](https://pypi.org/pypi/Envelopes/0.4)\n(MardownMail is a wrapper around Envelopes library.)\n\n[Markdown syntax](https://daringfireball.net/projects/markdown/syntax)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "E-mail with text and html content provided with markdown",
"version": "0.11.1",
"project_urls": {
"Homepage": "https://gitlab.com/yaal/markdownmail"
},
"split_keywords": [
"mail",
"markdown",
"yaal"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4f03c1c2dd388ea0bde6a187479ef71ab838543c9058748875e703877dde5e09",
"md5": "2ecdb7d35a16183b82edf5eedc3f8b5e",
"sha256": "20a27ab4c1db22712bc10055ae2474030b949903cdceeeea83bf2f7fd7723259"
},
"downloads": -1,
"filename": "markdownmail-0.11.1-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "2ecdb7d35a16183b82edf5eedc3f8b5e",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": null,
"size": 8675,
"upload_time": "2024-12-28T21:41:14",
"upload_time_iso_8601": "2024-12-28T21:41:14.443391Z",
"url": "https://files.pythonhosted.org/packages/4f/03/c1c2dd388ea0bde6a187479ef71ab838543c9058748875e703877dde5e09/markdownmail-0.11.1-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a85fefbc0edc1bd7cf1ae3a04be2862085bdeb4b7561f1c0d407a2535d8b9f94",
"md5": "afefad29d5de23f40b5c5ca349e08e98",
"sha256": "fb33fdadf9961254f6ab16c09adf08474615f392c8429d42da825f9c42a8db68"
},
"downloads": -1,
"filename": "markdownmail-0.11.1.tar.gz",
"has_sig": false,
"md5_digest": "afefad29d5de23f40b5c5ca349e08e98",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 7863,
"upload_time": "2024-12-28T21:41:17",
"upload_time_iso_8601": "2024-12-28T21:41:17.007219Z",
"url": "https://files.pythonhosted.org/packages/a8/5f/efbc0edc1bd7cf1ae3a04be2862085bdeb4b7561f1c0d407a2535d8b9f94/markdownmail-0.11.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-28 21:41:17",
"github": false,
"gitlab": true,
"bitbucket": false,
"codeberg": false,
"gitlab_user": "yaal",
"gitlab_project": "markdownmail",
"lcname": "markdownmail"
}