# MetaMask Institutional SDK (Beta)
A Python library to create and submit Ethereum transactions to custodians connected with [MetaMask Institutional](https://metamask.io/institutions); the most trusted DeFi wallet and Web3 gateway for organizations.
> **DISCLAIMER.** THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
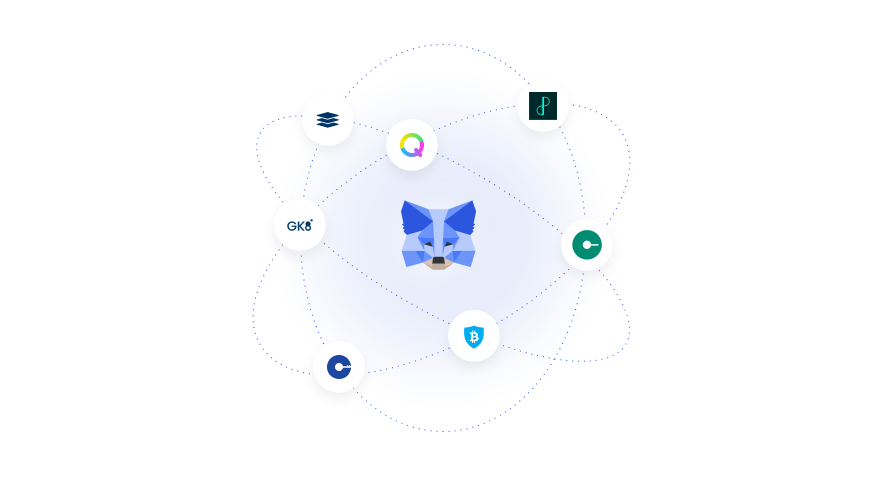
## Usage
Use this SDK to programmatically create Ethereum transactions, and submit them to custodians connected with MetaMask Institutional. Automate trading strategies on your wallets under custody, and still benefit from the institutional-grade security of your favorite qualified custodian and custody provider.
## Getting started
### Setting up
```bash
$ pip install metamask-institutional.sdk
```
```python
>>> from metamask_institutional.sdk import CustodianFactory
>>> factory = CustodianFactory()
>>> custodian = factory.create_for("qredo", "YOUR-TOKEN")
```
Use the custodian's Factory name param in the table below to instantiate a client for the right custodian.
| Custodian | Supported | As of version | Factory name param |
| ----------- | --------- | ------------- | ------------------ |
| Bitgo | ✅ | `0.3.0` | `"bitgo"` |
| Cactus | ✅ | `0.2.0` | `"cactus"` |
| FPG | ✅ | `0.4.0` | `"fpg-prod"` |
| Gnosis Safe | ✅ | `0.4.0` | `"gnosis-safe"` |
| Qredo | ✅ | `0.2.0` | `"qredo"` |
| Saturn | ✅ | `0.4.0` | `"saturn"` |
### Creating a transaction
```python
import os
from metamask_institutional.sdk import CustodianFactory
# Instantiate the factory
factory = CustodianFactory()
# Grab your token from the environment, or anywhere else
token = os.environ["MMISDK_TOKEN_QREDO"]
# Create the custodian, using the factory
custodian = factory.create_for("qredo", token)
# Build tx details
tx_params = {
"from": "0x62468FD916bF27A3b76d3de2e5280e53078e13E1",
"to": "0x62468FD916bF27A3b76d3de2e5280e53078e13E1",
"value": "100000000000000000", # in Wei
"gas": "21000",
"gasPrice": "1000",
# "data": "0xsomething",
# "type": "2"
# "maxPriorityFeePerGas": "12321321",
# "maxFeePerGas": "12321321",
}
qredo_extra_params = {
"chainID": "3",
}
# Create the tx from details and send it to the custodian
transaction = custodian.create_transaction(tx_params, qredo_extra_params)
print(type(transaction))
# <class 'metamask-institutional.sdk.common.transaction.Transaction'>
print(transaction)
# id='2EzDJkLVIjmH6LZQ2W1T4wPcTtK'
# type='1'
# from_='0x62468FD916bF27A3b76d3de2e5280e53078e13E1'
# to='0x62468FD916bF27A3b76d3de2e5280e53078e13E1'
# value='100000000000000000'
# gas='21000'
# gasPrice='1000'
# maxPriorityFeePerGas=None
# maxFeePerGas=None
# nonce='0'
# data=''
# hash=''
# status=TransactionStatus(finished=False, submitted=False, signed=False, success=False, displayText='Created', reason='Unknown')
```
### Getting a transaction
```python
import os
from metamask_institutional.sdk import CustodianFactory
# Instantiate the factory
factory = CustodianFactory()
# Grab your token from the environment, or anywhere else
token = os.environ["MMISDK_TOKEN_CACTUS"]
# Create the custodian, using the factory
custodian = factory.create_for("cactus", token)
# Get the transaction
transaction = custodian.get_transaction("5CM05NCLMRD888888000800", 5)
print(type(transaction))
# <class 'metamask-institutional.sdk.common.transaction.Transaction'>
print(transaction)
# id='5CM05NCLMRD888888000800'
# type='1'
# from_='0xFA42B2eCf59abD6d6BD4BF07021D870E2FC0eF20'
# to=None
# value=None
# gas='133997'
# gasPrice='2151'
# maxPriorityFeePerGas=None
# maxFeePerGas=None
# nonce=''
# data=None
# hash=None
# status=TransactionStatus(finished=False, submitted=False, signed=False, success=False, displayText='Created', reason='Unknown')
```
## Examples
Continue on the page [Examples](https://consensys.gitlab.io/codefi/products/mmi/mmi-sdk-py/examples/) to see all code examples.
Raw data
{
"_id": null,
"home_page": "https://gitlab.com/ConsenSys/codefi/products/mmi/mmi-sdk-py/-/issues",
"name": "metamask-institutional.sdk",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "python sdk custodian interact get create transaction",
"author": "Xavier Brochard",
"author_email": "xavier.brochard@consensys.net",
"download_url": "https://files.pythonhosted.org/packages/91/61/d5e0ee48ca49674167c9f8f27f1ace249da24298ea33aa0480894bcaef84/metamask-institutional.sdk-0.6.2.tar.gz",
"platform": null,
"description": "# MetaMask Institutional SDK (Beta)\n\nA Python library to create and submit Ethereum transactions to custodians connected with [MetaMask Institutional](https://metamask.io/institutions); the most trusted DeFi wallet and Web3 gateway for organizations.\n\n> **DISCLAIMER.** THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.\n\n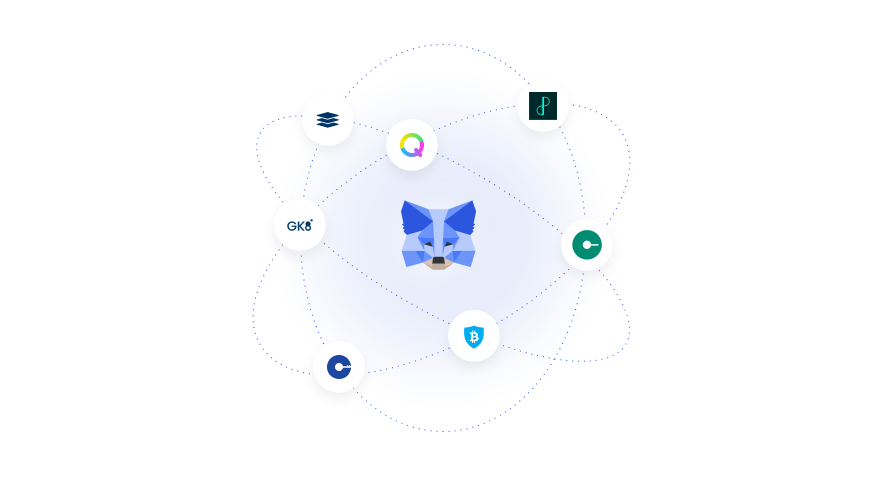\n\n## Usage\n\nUse this SDK to programmatically create Ethereum transactions, and submit them to custodians connected with MetaMask Institutional. Automate trading strategies on your wallets under custody, and still benefit from the institutional-grade security of your favorite qualified custodian and custody provider.\n\n## Getting started\n\n### Setting up\n\n```bash\n$ pip install metamask-institutional.sdk\n```\n\n```python\n>>> from metamask_institutional.sdk import CustodianFactory\n\n>>> factory = CustodianFactory()\n\n>>> custodian = factory.create_for(\"qredo\", \"YOUR-TOKEN\")\n```\n\nUse the custodian's Factory name param in the table below to instantiate a client for the right custodian.\n\n| Custodian | Supported | As of version | Factory name param |\n| ----------- | --------- | ------------- | ------------------ |\n| Bitgo | \u2705 | `0.3.0` | `\"bitgo\"` |\n| Cactus | \u2705 | `0.2.0` | `\"cactus\"` |\n| FPG | \u2705 | `0.4.0` | `\"fpg-prod\"` |\n| Gnosis Safe | \u2705 | `0.4.0` | `\"gnosis-safe\"` |\n| Qredo | \u2705 | `0.2.0` | `\"qredo\"` |\n| Saturn | \u2705 | `0.4.0` | `\"saturn\"` |\n\n### Creating a transaction\n\n```python\nimport os\n\nfrom metamask_institutional.sdk import CustodianFactory\n\n# Instantiate the factory\nfactory = CustodianFactory()\n\n# Grab your token from the environment, or anywhere else\ntoken = os.environ[\"MMISDK_TOKEN_QREDO\"]\n\n# Create the custodian, using the factory\ncustodian = factory.create_for(\"qredo\", token)\n\n# Build tx details\ntx_params = {\n \"from\": \"0x62468FD916bF27A3b76d3de2e5280e53078e13E1\",\n \"to\": \"0x62468FD916bF27A3b76d3de2e5280e53078e13E1\",\n \"value\": \"100000000000000000\", # in Wei\n \"gas\": \"21000\",\n \"gasPrice\": \"1000\",\n # \"data\": \"0xsomething\",\n # \"type\": \"2\"\n # \"maxPriorityFeePerGas\": \"12321321\",\n # \"maxFeePerGas\": \"12321321\",\n}\nqredo_extra_params = {\n \"chainID\": \"3\",\n}\n\n# Create the tx from details and send it to the custodian\ntransaction = custodian.create_transaction(tx_params, qredo_extra_params)\nprint(type(transaction))\n# <class 'metamask-institutional.sdk.common.transaction.Transaction'>\n\nprint(transaction)\n# id='2EzDJkLVIjmH6LZQ2W1T4wPcTtK'\n# type='1'\n# from_='0x62468FD916bF27A3b76d3de2e5280e53078e13E1'\n# to='0x62468FD916bF27A3b76d3de2e5280e53078e13E1'\n# value='100000000000000000'\n# gas='21000'\n# gasPrice='1000'\n# maxPriorityFeePerGas=None\n# maxFeePerGas=None\n# nonce='0'\n# data=''\n# hash=''\n# status=TransactionStatus(finished=False, submitted=False, signed=False, success=False, displayText='Created', reason='Unknown')\n```\n\n### Getting a transaction\n\n```python\nimport os\n\nfrom metamask_institutional.sdk import CustodianFactory\n\n# Instantiate the factory\nfactory = CustodianFactory()\n\n# Grab your token from the environment, or anywhere else\ntoken = os.environ[\"MMISDK_TOKEN_CACTUS\"]\n\n# Create the custodian, using the factory\ncustodian = factory.create_for(\"cactus\", token)\n\n# Get the transaction\ntransaction = custodian.get_transaction(\"5CM05NCLMRD888888000800\", 5)\n\nprint(type(transaction))\n# <class 'metamask-institutional.sdk.common.transaction.Transaction'>\n\nprint(transaction)\n# id='5CM05NCLMRD888888000800'\n# type='1'\n# from_='0xFA42B2eCf59abD6d6BD4BF07021D870E2FC0eF20'\n# to=None\n# value=None\n# gas='133997'\n# gasPrice='2151'\n# maxPriorityFeePerGas=None\n# maxFeePerGas=None\n# nonce=''\n# data=None\n# hash=None\n# status=TransactionStatus(finished=False, submitted=False, signed=False, success=False, displayText='Created', reason='Unknown')\n\n```\n\n## Examples\n\nContinue on the page [Examples](https://consensys.gitlab.io/codefi/products/mmi/mmi-sdk-py/examples/) to see all code examples.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Python library to create and submit Ethereum transactions to custodians connected with MetaMask Institutional; the most trusted DeFi wallet and Web3 gateway for organizations.",
"version": "0.6.2",
"project_urls": {
"Documentation": "https://consensys.gitlab.io/codefi/products/mmi/mmi-sdk-py/sdk-python/",
"Homepage": "https://gitlab.com/ConsenSys/codefi/products/mmi/mmi-sdk-py/-/issues"
},
"split_keywords": [
"python",
"sdk",
"custodian",
"interact",
"get",
"create",
"transaction"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9c2b15d7139c969a79d51c3866cf270a15c472e0eda40c47cda04deb48faddb1",
"md5": "ec017fb3cc789556ccf3ba92a42da387",
"sha256": "db1e97aa47297306d6fd1acefc7842714c38694a4819ae9b1fcb3542568ec41b"
},
"downloads": -1,
"filename": "metamask_institutional.sdk-0.6.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "ec017fb3cc789556ccf3ba92a42da387",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 62949,
"upload_time": "2023-09-01T12:31:40",
"upload_time_iso_8601": "2023-09-01T12:31:40.295902Z",
"url": "https://files.pythonhosted.org/packages/9c/2b/15d7139c969a79d51c3866cf270a15c472e0eda40c47cda04deb48faddb1/metamask_institutional.sdk-0.6.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9161d5e0ee48ca49674167c9f8f27f1ace249da24298ea33aa0480894bcaef84",
"md5": "031fac0e3fd889e74cd312f676c4395f",
"sha256": "0df1bb072739396d738de2e6d0d075940b5edae4ea1dcaf95c04da9ac8290082"
},
"downloads": -1,
"filename": "metamask-institutional.sdk-0.6.2.tar.gz",
"has_sig": false,
"md5_digest": "031fac0e3fd889e74cd312f676c4395f",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 232506,
"upload_time": "2023-09-01T12:31:42",
"upload_time_iso_8601": "2023-09-01T12:31:42.105217Z",
"url": "https://files.pythonhosted.org/packages/91/61/d5e0ee48ca49674167c9f8f27f1ace249da24298ea33aa0480894bcaef84/metamask-institutional.sdk-0.6.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-01 12:31:42",
"github": false,
"gitlab": true,
"bitbucket": false,
"codeberg": false,
"gitlab_user": "ConsenSys",
"gitlab_project": "codefi",
"lcname": "metamask-institutional.sdk"
}