Name | metats JSON |
Version |
0.2.1
JSON |
| download |
home_page | |
Summary | Meta-Learning for Time Series Forecasting |
upload_time | 2023-03-30 09:33:50 |
maintainer | |
docs_url | None |
author | |
requires_python | |
license | MIT License Copyright (c) 2021 Amirabbas Asadi Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
timeseries
metalearning
forecasting
unsupervised learning
deeplearning
machine learning
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# MetaTS | Meta-Learning for Global Time Series Forecasting

[](https://pypi.python.org/pypi/metats/)
[](https://www.python.org/)
[](https://github.com/amirabbasasadi/metats/blob/master/LICENSE)
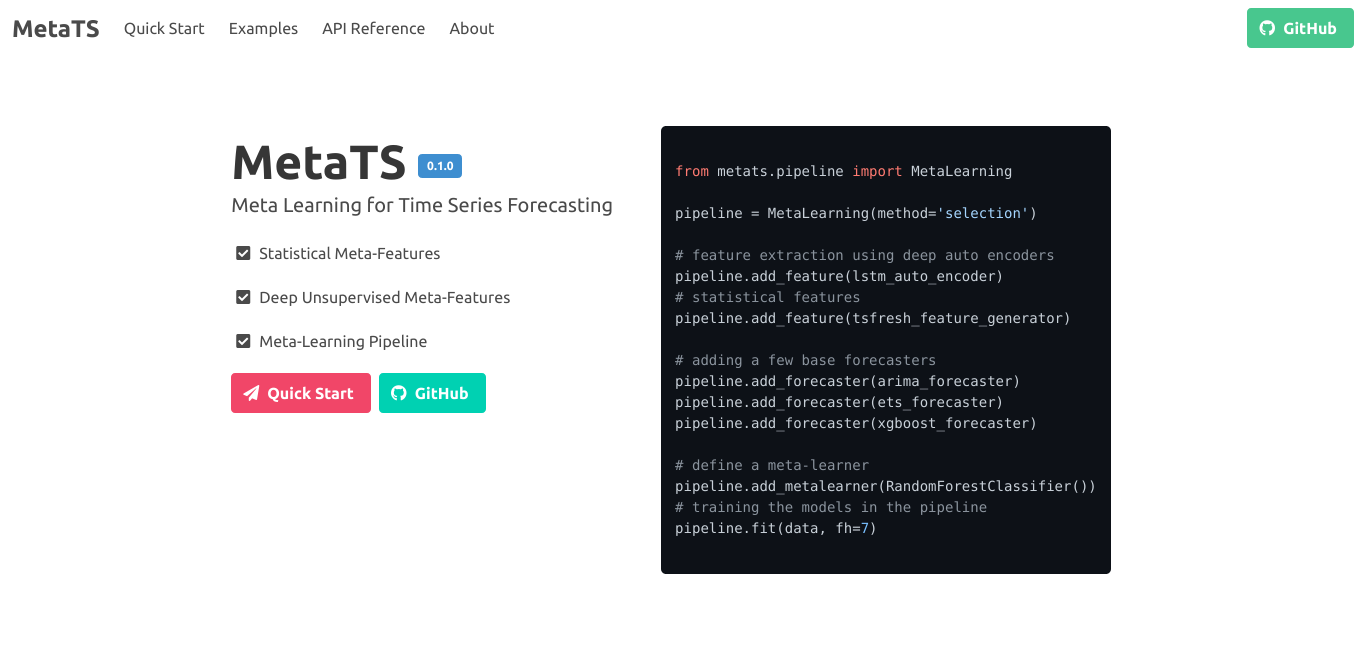
## Features:
- Generating meta features
- Statistical features : TsFresh, User defined features
- Automated feature extraction using Deep Unsupervised Learning : Deep AutoEncoder (MLP, LSTM, GRU, ot custom model)
- Supporting sktime and darts libraries for base-forecasters
- Providing a Meta-Learning pipeline
## Quick Start
### Installing the package
```
pip install metats
```
### Generating a toy dataset by sampling from two different processes
```python
from metats.datasets import ETSDataset
ets_generator = ETSDataset({'A,N,N': 512,
'M,M,M': 512}, length=30, freq=4)
data, labels = ets_generator.load(return_family=True)
colors = list(map(lambda x: (x=='A,N,N')*1, labels))
```
### Normalizing the time series
```python
from sklearn.preprocessing import StandardScaler
scaled_data = StandardScaler().fit_transform(data.T)
data = scaled_data.T[:, :, None]
```
### Checking How data looks like
```python
import matplotlib.pyplot as plt
_ = plt.plot(data[10, :, 0])
```
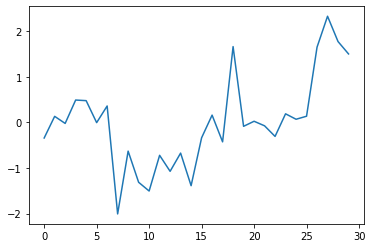
### Generating the meta-features
#### Statistical features using TsFresh
```python
from metats.features.statistical import TsFresh
stat_features = TsFresh().transform(data)
```
#### Deep Unsupervised Features
##### Training an AutoEncoder
```python
from metats.features.unsupervised import DeepAutoEncoder
from metats.features.deep import AutoEncoder, MLPEncoder, MLPDecoder
enc = MLPEncoder(input_size=1, input_length=30, latent_size=8, hidden_layers=(16,))
dec = MLPDecoder(input_size=1, input_length=30, latent_size=8, hidden_layers=(16,))
ae = AutoEncoder(encoder=enc, decoder=dec)
ae_feature = DeepAutoEncoder(auto_encoder=ae, epochs=150, verbose=True)
ae_feature.fit(data)
```
##### Generating features using the auto-encoder
```python
deep_features = ae_feature.transform(data)
```
#### Visualizing both statistical and deep meta-features
Dimensionality reduction using UMAP for visualization
```python
from umap import UMAP
deep_reduced = UMAP().fit_transform(deep_features)
stat_reduced = UMAP().fit_transform(stat_features)
```
Visualizing the statistical features:
```python
plt.scatter(stat_reduced[:512, 0], stat_reduced[:512, 1], c='#e74c3c', label='ANN')
plt.scatter(stat_reduced[512:, 0], stat_reduced[512:, 1], c='#9b59b6', label='MMM')
plt.legend()
plt.title('TsFresh Meta-Features')
_ = plt.show()
```
And similarly the auto encoder's features
```python
plt.scatter(deep_reduced[:512, 0], deep_reduced[:512, 1], c='#e74c3c', label='ANN')
plt.scatter(deep_reduced[512:, 0], deep_reduced[512:, 1], c='#9b59b6', label='MMM')
plt.legend()
plt.title('Deep Unsupervised Meta-Features')
_ = plt.show()
```
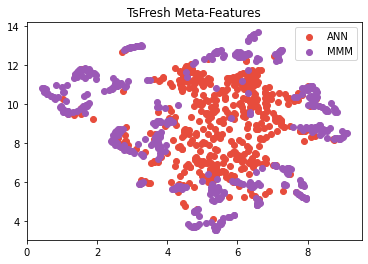
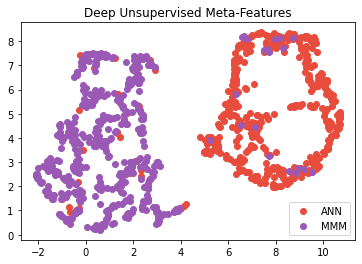
## Meta-Learning Pipeline
Creating a meta-learning pipeline with selection strategy:
```python
from metats.pipeline import MetaLearning
pipeline = MetaLearning(method='selection', loss='mse')
```
Adding AutoEncoder features:
```python
from metats.features.unsupervised import DeepAutoEncoder
from metats.features.deep import AutoEncoder, MLPEncoder, MLPDecoder
enc = MLPEncoder(input_size=1, input_length=23, latent_size=8, hidden_layers=(16,))
dec = MLPDecoder(input_size=1, input_length=23, latent_size=8, hidden_layers=(16,))
ae = AutoEncoder(encoder=enc, decoder=dec)
ae_features = DeepAutoEncoder(auto_encoder=ae, epochs=200, verbose=True)
pipeline.add_feature(ae_features)
```
You can add as many features as you like:
```python
from metats.features.statistical import TsFresh
stat_features = TsFresh()
pipeline.add_feature(stat_features)
```
Adding two sktime forecaster as base-forecasters
```python
from sktime.forecasting.naive import NaiveForecaster
from sktime.forecasting.compose import make_reduction
from sklearn.neighbors import KNeighborsRegressor
regressor = KNeighborsRegressor(n_neighbors=1)
forecaster1 = make_reduction(regressor, window_length=15, strategy="recursive")
forecaster2 = NaiveForecaster()
pipeline.add_forecaster(forecaster1)
pipeline.add_forecaster(forecaster2)
```
Specify some meta-learner
```python
from sklearn.ensemble import RandomForestClassifier
pipeline.add_metalearner(RandomForestClassifier())
```
Training the pipeline
```python
pipeline.fit(data, fh=7)
```
Prediction for another set of data
```python
pipeline.predict(data, fh=7)
```
## About the package
### Contributors
- Sasan Barak
- Amirabbas Asadi
We wish to see your name in the list of contributors, So we are waiting for pull requests!
Raw data
{
"_id": null,
"home_page": "",
"name": "metats",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "timeseries,metalearning,forecasting,unsupervised learning,deeplearning,machine learning",
"author": "",
"author_email": "Sasan Barak <s.barak@soton.ac.uk>, Amirabbas Asadi <amir137825@gmail.com>, Mohammad Joshaghani <mjoshaghani10@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/df/83/4c9d15851cc5d10d90136a9aa691212cc1fd5dd8dc7840b56ccce58762f0/metats-0.2.1.tar.gz",
"platform": null,
"description": "# MetaTS | Meta-Learning for Global Time Series Forecasting\n\n[](https://pypi.python.org/pypi/metats/)\n[](https://www.python.org/)\n[](https://github.com/amirabbasasadi/metats/blob/master/LICENSE)\n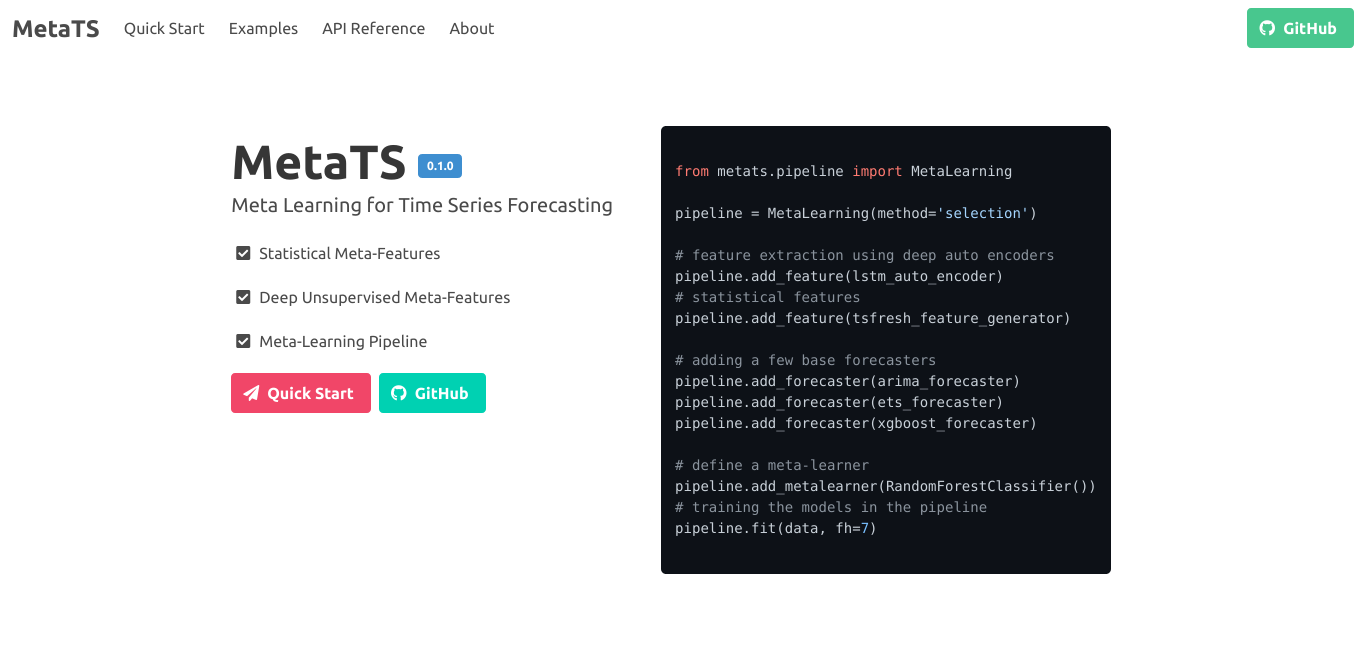\n\n## Features:\n- Generating meta features\n - Statistical features : TsFresh, User defined features\n - Automated feature extraction using Deep Unsupervised Learning : Deep AutoEncoder (MLP, LSTM, GRU, ot custom model)\n- Supporting sktime and darts libraries for base-forecasters\n- Providing a Meta-Learning pipeline\n\n## Quick Start\n\n### Installing the package\n```\npip install metats\n```\n\n### Generating a toy dataset by sampling from two different processes\n```python\nfrom metats.datasets import ETSDataset\n\nets_generator = ETSDataset({'A,N,N': 512,\n 'M,M,M': 512}, length=30, freq=4)\n\ndata, labels = ets_generator.load(return_family=True)\ncolors = list(map(lambda x: (x=='A,N,N')*1, labels))\n```\n\n### Normalizing the time series\n```python\nfrom sklearn.preprocessing import StandardScaler\n\nscaled_data = StandardScaler().fit_transform(data.T)\ndata = scaled_data.T[:, :, None]\n```\n### Checking How data looks like\n```python\nimport matplotlib.pyplot as plt\n_ = plt.plot(data[10, :, 0])\n```\n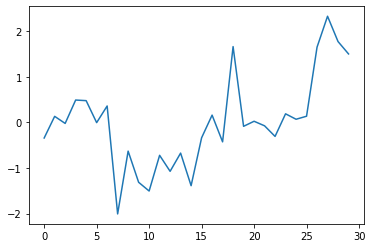\n\n### Generating the meta-features\n#### Statistical features using TsFresh\n```python\nfrom metats.features.statistical import TsFresh\n\nstat_features = TsFresh().transform(data)\n```\n#### Deep Unsupervised Features\n##### Training an AutoEncoder\n```python\nfrom metats.features.unsupervised import DeepAutoEncoder\nfrom metats.features.deep import AutoEncoder, MLPEncoder, MLPDecoder\n\nenc = MLPEncoder(input_size=1, input_length=30, latent_size=8, hidden_layers=(16,))\ndec = MLPDecoder(input_size=1, input_length=30, latent_size=8, hidden_layers=(16,))\n\nae = AutoEncoder(encoder=enc, decoder=dec)\nae_feature = DeepAutoEncoder(auto_encoder=ae, epochs=150, verbose=True)\n\nae_feature.fit(data)\n```\n##### Generating features using the auto-encoder\n```python\ndeep_features = ae_feature.transform(data)\n```\n\n#### Visualizing both statistical and deep meta-features\nDimensionality reduction using UMAP for visualization\n```python\nfrom umap import UMAP\ndeep_reduced = UMAP().fit_transform(deep_features)\nstat_reduced = UMAP().fit_transform(stat_features)\n```\nVisualizing the statistical features:\n```python\nplt.scatter(stat_reduced[:512, 0], stat_reduced[:512, 1], c='#e74c3c', label='ANN')\nplt.scatter(stat_reduced[512:, 0], stat_reduced[512:, 1], c='#9b59b6', label='MMM')\nplt.legend()\nplt.title('TsFresh Meta-Features')\n_ = plt.show()\n```\nAnd similarly the auto encoder's features\n```python\nplt.scatter(deep_reduced[:512, 0], deep_reduced[:512, 1], c='#e74c3c', label='ANN')\nplt.scatter(deep_reduced[512:, 0], deep_reduced[512:, 1], c='#9b59b6', label='MMM')\nplt.legend()\nplt.title('Deep Unsupervised Meta-Features')\n_ = plt.show()\n```\n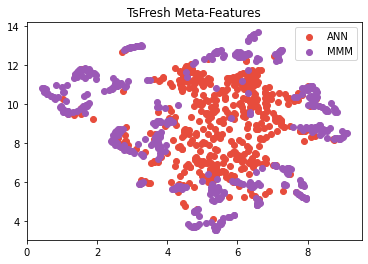\n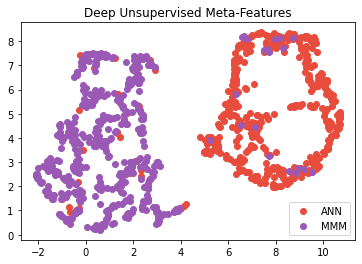\n\n\n\n## Meta-Learning Pipeline\nCreating a meta-learning pipeline with selection strategy:\n```python\nfrom metats.pipeline import MetaLearning\n\npipeline = MetaLearning(method='selection', loss='mse')\n```\nAdding AutoEncoder features:\n```python\nfrom metats.features.unsupervised import DeepAutoEncoder\nfrom metats.features.deep import AutoEncoder, MLPEncoder, MLPDecoder\n\nenc = MLPEncoder(input_size=1, input_length=23, latent_size=8, hidden_layers=(16,))\ndec = MLPDecoder(input_size=1, input_length=23, latent_size=8, hidden_layers=(16,))\n\nae = AutoEncoder(encoder=enc, decoder=dec)\nae_features = DeepAutoEncoder(auto_encoder=ae, epochs=200, verbose=True)\n\npipeline.add_feature(ae_features)\n```\nYou can add as many features as you like:\n```python\nfrom metats.features.statistical import TsFresh\n\nstat_features = TsFresh()\npipeline.add_feature(stat_features)\n```\nAdding two sktime forecaster as base-forecasters\n```python\nfrom sktime.forecasting.naive import NaiveForecaster\nfrom sktime.forecasting.compose import make_reduction\nfrom sklearn.neighbors import KNeighborsRegressor\n\nregressor = KNeighborsRegressor(n_neighbors=1)\nforecaster1 = make_reduction(regressor, window_length=15, strategy=\"recursive\")\n\nforecaster2 = NaiveForecaster() \n\npipeline.add_forecaster(forecaster1)\npipeline.add_forecaster(forecaster2)\n```\nSpecify some meta-learner\n```python\nfrom sklearn.ensemble import RandomForestClassifier\n\npipeline.add_metalearner(RandomForestClassifier())\n```\n\nTraining the pipeline\n```python\npipeline.fit(data, fh=7)\n```\nPrediction for another set of data\n```python\npipeline.predict(data, fh=7)\n```\n\n## About the package\n### Contributors\n- Sasan Barak\n- Amirabbas Asadi\n\n\nWe wish to see your name in the list of contributors, So we are waiting for pull requests!\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2021 Amirabbas Asadi Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Meta-Learning for Time Series Forecasting",
"version": "0.2.1",
"split_keywords": [
"timeseries",
"metalearning",
"forecasting",
"unsupervised learning",
"deeplearning",
"machine learning"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ca4167430b8b854556a07199f06b7bc444a9f782c9f015c660d6c7dc20071cd4",
"md5": "cd87eb75b3858794e834e98dcaa29543",
"sha256": "db2124dcc6034a3d682469b4106750de926ae1066025dbef3576394cfd0915ce"
},
"downloads": -1,
"filename": "metats-0.2.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "cd87eb75b3858794e834e98dcaa29543",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 16653,
"upload_time": "2023-03-30T09:33:45",
"upload_time_iso_8601": "2023-03-30T09:33:45.581828Z",
"url": "https://files.pythonhosted.org/packages/ca/41/67430b8b854556a07199f06b7bc444a9f782c9f015c660d6c7dc20071cd4/metats-0.2.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "df834c9d15851cc5d10d90136a9aa691212cc1fd5dd8dc7840b56ccce58762f0",
"md5": "120ddafd18251e6dbc172b7b74d97bc9",
"sha256": "941cc8245be1b5599cd91a2ae5bf88db19a075a0b223387ee8c46c9325b6d27c"
},
"downloads": -1,
"filename": "metats-0.2.1.tar.gz",
"has_sig": false,
"md5_digest": "120ddafd18251e6dbc172b7b74d97bc9",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 16896,
"upload_time": "2023-03-30T09:33:50",
"upload_time_iso_8601": "2023-03-30T09:33:50.286195Z",
"url": "https://files.pythonhosted.org/packages/df/83/4c9d15851cc5d10d90136a9aa691212cc1fd5dd8dc7840b56ccce58762f0/metats-0.2.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-03-30 09:33:50",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "metats"
}