Name | mknapsack JSON |
Version |
1.1.12
JSON |
| download |
home_page | |
Summary | Solving knapsack and bin packing problems with Python |
upload_time | 2023-04-07 15:18:06 |
maintainer | |
docs_url | None |
author | |
requires_python | <3.12,>=3.8 |
license | Copyright (c) 2022 Jesse Myrberg Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
knapsack
bin
packing
optimization
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# mknapsack
[](https://github.com/jmyrberg/mknapsack/actions/workflows/push.yml)
[](https://github.com/jmyrberg/mknapsack/actions/workflows/wheels.yml)
[](https://mknapsack.readthedocs.io/en/latest/?badge=latest)
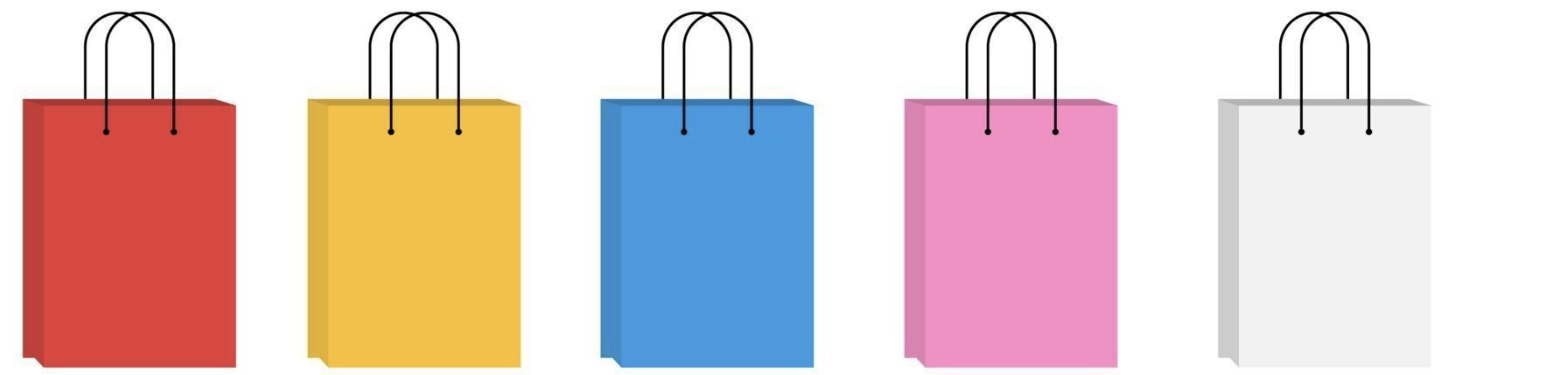
Solving knapsack problems with Python using algorithms by [Martello and Toth](https://dl.acm.org/doi/book/10.5555/98124):
* Single 0-1 knapsack problem: MT1, MT2, MT1R (real numbers)
* Bounded knapsack problem: MTB2
* Unbounded knapsack problem: MTU1, MTU2
* Multiple 0-1 knapsack problem: MTM, MTHM
* Change-making problem: MTC2
* Bounded change-making problem: MTCB
* Generalized assignment problem: MTG, MTHG
* Bin packing problem: MTP
* Subset sum problem: MTSL
Documentation is available [here](https://mknapsack.readthedocs.io).
## Installation
`pip install mknapsack`
## Example usage
### Single 0-1 Knapsack Problem
```python
from mknapsack import solve_single_knapsack
# Given ten items with the following profits and weights:
profits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]
weights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]
# ...and a knapsack with the following capacity:
capacity = 190
# Assign items into the knapsack while maximizing profits
res = solve_single_knapsack(profits, weights, capacity)
```
If your inputs are real numbers, you may set parameter `method='mt1r'`.
### Bounded Knapsack Problem
```python
from mknapsack import solve_bounded_knapsack
# Given ten item types with the following profits and weights:
profits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]
weights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]
# ...and the number of items available for each item type:
n_items = [1, 2, 3, 2, 2, 1, 2, 2, 1, 4]
# ...and a knapsack with the following capacity:
capacity = 190
# Assign items into the knapsack while maximizing profits
res = solve_bounded_knapsack(profits, weights, capacity, n_items)
```
### Unbounded Knapsack Problem
```python
from mknapsack import solve_unbounded_knapsack
# Given ten item types with the following profits and weights:
profits = [16, 72, 35, 89, 36, 94, 75, 74, 100, 80]
weights = [30, 18, 9, 23, 20, 59, 61, 70, 75, 76]
# ...and a knapsack with the following capacity:
capacity = 190
# Assign items repeatedly into the knapsack while maximizing profits
res = solve_unbounded_knapsack(profits, weights, capacity, n_items)
```
### Multiple 0-1 Knapsack Problem
```python
from mknapsack import solve_multiple_knapsack
# Given ten items with the following profits and weights:
profits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]
weights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]
# ...and two knapsacks with the following capacities:
capacities = [90, 100]
# Assign items into the knapsacks while maximizing profits
res = solve_multiple_knapsack(profits, weights, capacities)
```
### Change-Making Problem
```python
from mknapsack import solve_change_making
# Given ten item types with the following weights:
weights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]
# ...and a knapsack with the following capacity:
capacity = 190
# Fill the knapsack while minimizing the number of items
res = solve_change_making(weights, capacity)
```
### Bounded Change-Making Problem
```python
from mknapsack import solve_bounded_change_making
# Given ten item types with the following weights:
weights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]
# ...and the number of items available for each item type:
n_items = [1, 2, 3, 2, 1, 1, 1, 2, 1, 2]
# ...and a knapsack with the following capacity:
capacity = 190
# Fill the knapsack while minimizing the number of items
res = solve_bounded_change_making(weights, n_items, capacity)
```
### Generalized Assignment Problem
```python
from mknapsack import solve_generalized_assignment
# Given seven item types with the following knapsack dependent profits:
profits = [[6, 9, 4, 2, 10, 3, 6],
[4, 8, 9, 1, 7, 5, 4]]
# ...and the following knapsack dependent weights:
weights = [[4, 1, 2, 1, 4, 3, 8],
[9, 9, 8, 1, 3, 8, 7]]
# ...and two knapsacks with the following capacities:
capacities = [11, 22]
# Assign items into the knapsacks while maximizing profits
res = solve_generalized_assignment(profits, weights, capacities)
```
### Bin Packing Problem
```python
from mknapsack import solve_bin_packing
# Given six items with the following weights:
weights = [4, 1, 8, 1, 4, 2]
# ...and bins with the following capacity:
capacity = 10
# Assign items into bins while minimizing the number of bins required
res = solve_bin_packing(weights, capacity)
```
### Subset Sum Problem
```python
from mknapsack import solve_subset_sum
# Given six items with the following weights:
weights = [4, 1, 8, 1, 4, 2]
# ...and a knapsack with the following capacity:
capacity = 10
# Choose items to fill the knapsack to the fullest
res = solve_subset_sum(weights, capacity)
```
## References
* [Knapsack problems: algorithms and computer implementations](https://dl.acm.org/doi/book/10.5555/98124) by S. Martello and P. Toth, 1990
* [Original Fortran77 source code](http://people.sc.fsu.edu/~jburkardt/f77_src/knapsack/knapsack.f) by S. Martello and P. Toth
---
Jesse Myrberg (jesse.myrberg@gmail.com)
Raw data
{
"_id": null,
"home_page": "",
"name": "mknapsack",
"maintainer": "",
"docs_url": null,
"requires_python": "<3.12,>=3.8",
"maintainer_email": "",
"keywords": "knapsack,bin,packing,optimization",
"author": "",
"author_email": "Jesse Myrberg <jesse.myrberg@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/d4/bb/f8a43ec75014fec16b6ba843eb2b7c21c31fad3b93cb835c7278d2201148/mknapsack-1.1.12.tar.gz",
"platform": null,
"description": "# mknapsack\n\n[](https://github.com/jmyrberg/mknapsack/actions/workflows/push.yml)\n[](https://github.com/jmyrberg/mknapsack/actions/workflows/wheels.yml)\n[](https://mknapsack.readthedocs.io/en/latest/?badge=latest)\n\n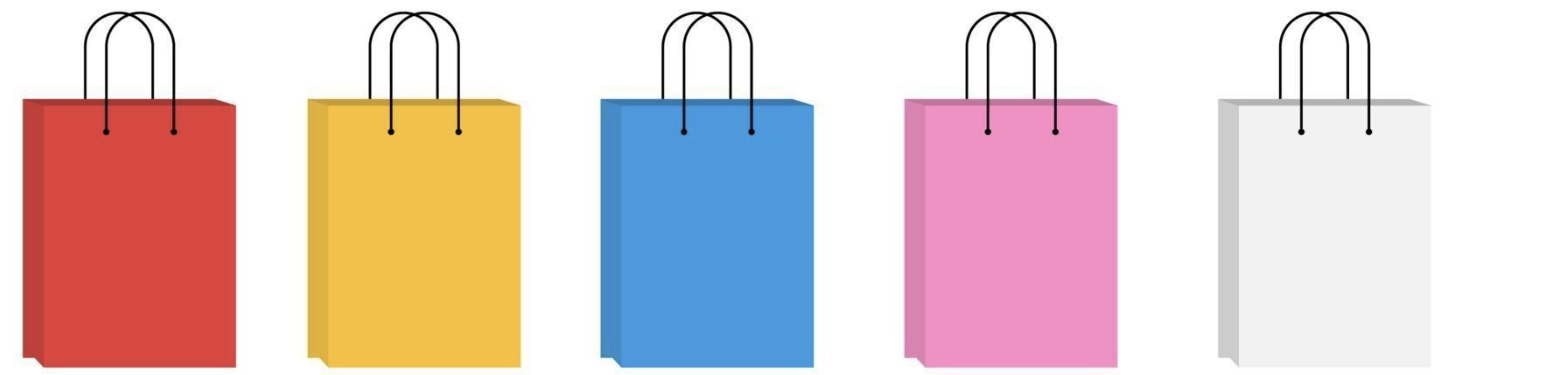\n\nSolving knapsack problems with Python using algorithms by [Martello and Toth](https://dl.acm.org/doi/book/10.5555/98124):\n\n* Single 0-1 knapsack problem: MT1, MT2, MT1R (real numbers)\n* Bounded knapsack problem: MTB2\n* Unbounded knapsack problem: MTU1, MTU2\n* Multiple 0-1 knapsack problem: MTM, MTHM\n* Change-making problem: MTC2\n* Bounded change-making problem: MTCB\n* Generalized assignment problem: MTG, MTHG\n* Bin packing problem: MTP\n* Subset sum problem: MTSL\n\nDocumentation is available [here](https://mknapsack.readthedocs.io).\n\n\n## Installation\n\n`pip install mknapsack`\n\n\n## Example usage\n\n### Single 0-1 Knapsack Problem\n\n```python\nfrom mknapsack import solve_single_knapsack\n\n# Given ten items with the following profits and weights:\nprofits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]\nweights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]\n\n# ...and a knapsack with the following capacity:\ncapacity = 190\n\n# Assign items into the knapsack while maximizing profits\nres = solve_single_knapsack(profits, weights, capacity)\n```\n\nIf your inputs are real numbers, you may set parameter `method='mt1r'`.\n\n### Bounded Knapsack Problem\n\n```python\nfrom mknapsack import solve_bounded_knapsack\n\n# Given ten item types with the following profits and weights:\nprofits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]\nweights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]\n\n# ...and the number of items available for each item type:\nn_items = [1, 2, 3, 2, 2, 1, 2, 2, 1, 4]\n\n# ...and a knapsack with the following capacity:\ncapacity = 190\n\n# Assign items into the knapsack while maximizing profits\nres = solve_bounded_knapsack(profits, weights, capacity, n_items)\n```\n\n### Unbounded Knapsack Problem\n\n```python\nfrom mknapsack import solve_unbounded_knapsack\n\n# Given ten item types with the following profits and weights:\nprofits = [16, 72, 35, 89, 36, 94, 75, 74, 100, 80]\nweights = [30, 18, 9, 23, 20, 59, 61, 70, 75, 76]\n\n# ...and a knapsack with the following capacity:\ncapacity = 190\n\n# Assign items repeatedly into the knapsack while maximizing profits\nres = solve_unbounded_knapsack(profits, weights, capacity, n_items)\n```\n\n### Multiple 0-1 Knapsack Problem\n\n```python\nfrom mknapsack import solve_multiple_knapsack\n\n# Given ten items with the following profits and weights:\nprofits = [78, 35, 89, 36, 94, 75, 74, 79, 80, 16]\nweights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]\n\n# ...and two knapsacks with the following capacities:\ncapacities = [90, 100]\n\n# Assign items into the knapsacks while maximizing profits\nres = solve_multiple_knapsack(profits, weights, capacities)\n```\n\n### Change-Making Problem\n\n```python\nfrom mknapsack import solve_change_making\n\n# Given ten item types with the following weights:\nweights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]\n\n# ...and a knapsack with the following capacity:\ncapacity = 190\n\n# Fill the knapsack while minimizing the number of items\nres = solve_change_making(weights, capacity)\n```\n\n### Bounded Change-Making Problem\n\n```python\nfrom mknapsack import solve_bounded_change_making\n\n# Given ten item types with the following weights:\nweights = [18, 9, 23, 20, 59, 61, 70, 75, 76, 30]\n\n# ...and the number of items available for each item type:\nn_items = [1, 2, 3, 2, 1, 1, 1, 2, 1, 2]\n\n# ...and a knapsack with the following capacity:\ncapacity = 190\n\n# Fill the knapsack while minimizing the number of items\nres = solve_bounded_change_making(weights, n_items, capacity)\n```\n\n### Generalized Assignment Problem\n\n```python\nfrom mknapsack import solve_generalized_assignment\n\n# Given seven item types with the following knapsack dependent profits:\nprofits = [[6, 9, 4, 2, 10, 3, 6],\n [4, 8, 9, 1, 7, 5, 4]]\n\n# ...and the following knapsack dependent weights:\nweights = [[4, 1, 2, 1, 4, 3, 8],\n [9, 9, 8, 1, 3, 8, 7]]\n\n# ...and two knapsacks with the following capacities:\ncapacities = [11, 22]\n\n# Assign items into the knapsacks while maximizing profits\nres = solve_generalized_assignment(profits, weights, capacities)\n```\n\n### Bin Packing Problem\n\n```python\nfrom mknapsack import solve_bin_packing\n\n# Given six items with the following weights:\nweights = [4, 1, 8, 1, 4, 2]\n\n# ...and bins with the following capacity:\ncapacity = 10\n\n# Assign items into bins while minimizing the number of bins required\nres = solve_bin_packing(weights, capacity)\n```\n\n### Subset Sum Problem\n\n```python\nfrom mknapsack import solve_subset_sum\n\n# Given six items with the following weights:\nweights = [4, 1, 8, 1, 4, 2]\n\n# ...and a knapsack with the following capacity:\ncapacity = 10\n\n# Choose items to fill the knapsack to the fullest\nres = solve_subset_sum(weights, capacity)\n```\n\n\n## References\n\n* [Knapsack problems: algorithms and computer implementations](https://dl.acm.org/doi/book/10.5555/98124) by S. Martello and P. Toth, 1990\n* [Original Fortran77 source code](http://people.sc.fsu.edu/~jburkardt/f77_src/knapsack/knapsack.f) by S. Martello and P. Toth\n\n---\nJesse Myrberg (jesse.myrberg@gmail.com)\n",
"bugtrack_url": null,
"license": "Copyright (c) 2022 Jesse Myrberg Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Solving knapsack and bin packing problems with Python",
"version": "1.1.12",
"split_keywords": [
"knapsack",
"bin",
"packing",
"optimization"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3eaecce565230b57f6eb2688a503427e870bac44d7de3807eaa3d09cc009f5db",
"md5": "bc7f63fc496a8ff032ccf6f3da58a249",
"sha256": "2e4cef34b7aceb83384f0a3742b08a215dd36c290e65b67ca11e0957e3769567"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "bc7f63fc496a8ff032ccf6f3da58a249",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.12,>=3.8",
"size": 1668541,
"upload_time": "2023-04-07T15:17:47",
"upload_time_iso_8601": "2023-04-07T15:17:47.564441Z",
"url": "https://files.pythonhosted.org/packages/3e/ae/cce565230b57f6eb2688a503427e870bac44d7de3807eaa3d09cc009f5db/mknapsack-1.1.12-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8d175848a0ec5c6457627383b11be2fd2dd52526d9c276795f959495da94b25d",
"md5": "fc41e6a622acd1b753eba5327a657c5b",
"sha256": "6c3cca52d794da94ab3829aedb356951072cdaeec4f99d9aa4338d828711281c"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "fc41e6a622acd1b753eba5327a657c5b",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.12,>=3.8",
"size": 1794990,
"upload_time": "2023-04-07T15:17:49",
"upload_time_iso_8601": "2023-04-07T15:17:49.388981Z",
"url": "https://files.pythonhosted.org/packages/8d/17/5848a0ec5c6457627383b11be2fd2dd52526d9c276795f959495da94b25d/mknapsack-1.1.12-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2a76dc20c258aa83ed6bdea569d3a1ed05b1826e197180ed87832f0c4f0b9cf5",
"md5": "aad730b358940f1499b7549073a85073",
"sha256": "9163a736d552b2b5bc8f8a46e3755573ef937338f9aebfd92590346160ffcdb8"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp310-cp310-win_amd64.whl",
"has_sig": false,
"md5_digest": "aad730b358940f1499b7549073a85073",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": "<3.12,>=3.8",
"size": 791947,
"upload_time": "2023-04-07T15:17:51",
"upload_time_iso_8601": "2023-04-07T15:17:51.110161Z",
"url": "https://files.pythonhosted.org/packages/2a/76/dc20c258aa83ed6bdea569d3a1ed05b1826e197180ed87832f0c4f0b9cf5/mknapsack-1.1.12-cp310-cp310-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e1ac5b78df00775420f5d168a03bae38cdd07e0a165b990f1ea8f889b5a5b4e2",
"md5": "e8e6d010e6e5f9cb10c73c030daef350",
"sha256": "4dea5a2b5bf41deb3cbbcfca7bd8b185f21b65b4bb20a158f008e7119d4f9a99"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "e8e6d010e6e5f9cb10c73c030daef350",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.12,>=3.8",
"size": 1668542,
"upload_time": "2023-04-07T15:17:53",
"upload_time_iso_8601": "2023-04-07T15:17:53.451078Z",
"url": "https://files.pythonhosted.org/packages/e1/ac/5b78df00775420f5d168a03bae38cdd07e0a165b990f1ea8f889b5a5b4e2/mknapsack-1.1.12-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2b30e9db087389a695483c84e875e4de2e31012cc615425bcd686d852112072f",
"md5": "80969a515618e6828577c49dc3efd3cd",
"sha256": "03e1733b029efd38114f05afa9286a932bf236001f48ae2c24c3d4571db4a5ac"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "80969a515618e6828577c49dc3efd3cd",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.12,>=3.8",
"size": 1795769,
"upload_time": "2023-04-07T15:17:55",
"upload_time_iso_8601": "2023-04-07T15:17:55.103096Z",
"url": "https://files.pythonhosted.org/packages/2b/30/e9db087389a695483c84e875e4de2e31012cc615425bcd686d852112072f/mknapsack-1.1.12-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2730fefe5f5cdb4e8887673d025261de509b8a7dbbd4e9dd80b1b77b87756161",
"md5": "32ac8308811652eebc99704c31732c90",
"sha256": "3680d40d6e30a3f887448725b9f3f2ce714812f18ddb869efc3a2fe29f4f0871"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp311-cp311-win_amd64.whl",
"has_sig": false,
"md5_digest": "32ac8308811652eebc99704c31732c90",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": "<3.12,>=3.8",
"size": 791943,
"upload_time": "2023-04-07T15:17:56",
"upload_time_iso_8601": "2023-04-07T15:17:56.740957Z",
"url": "https://files.pythonhosted.org/packages/27/30/fefe5f5cdb4e8887673d025261de509b8a7dbbd4e9dd80b1b77b87756161/mknapsack-1.1.12-cp311-cp311-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9c5bac42d7865c8bcda3abc708b975c3154b94c595d894cf7567923b0ff18183",
"md5": "bf1a6eb7a3f291d4313f4aad81bdc219",
"sha256": "944db401d2dbada8ea3a44b8f57e49ee05e5ac3f36488b518b49ba47c9b773df"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp38-cp38-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "bf1a6eb7a3f291d4313f4aad81bdc219",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.12,>=3.8",
"size": 1668313,
"upload_time": "2023-04-07T15:17:57",
"upload_time_iso_8601": "2023-04-07T15:17:57.914557Z",
"url": "https://files.pythonhosted.org/packages/9c/5b/ac42d7865c8bcda3abc708b975c3154b94c595d894cf7567923b0ff18183/mknapsack-1.1.12-cp38-cp38-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "aa371739e4f3231f32cdb4ffca9a27cc0e873902d5d6f1da49707b4825ba44d0",
"md5": "3110a47874ea2ad6c5dfbc49959912f0",
"sha256": "e462d94e8e9e28b6d4be21e581ff64ac48741a633e358a89030acd755121a076"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "3110a47874ea2ad6c5dfbc49959912f0",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.12,>=3.8",
"size": 1810467,
"upload_time": "2023-04-07T15:17:59",
"upload_time_iso_8601": "2023-04-07T15:17:59.026980Z",
"url": "https://files.pythonhosted.org/packages/aa/37/1739e4f3231f32cdb4ffca9a27cc0e873902d5d6f1da49707b4825ba44d0/mknapsack-1.1.12-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8252fc8f44bcf487ca96382160aeb6d1356295390a65c35f8f9ee54933f6f5f9",
"md5": "76aeb06ed06f444345bf9f6e92bd6787",
"sha256": "90792822fcda970b561b7fbbc9abf29309a090408ef6c45a594d76dda5bfbac9"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp38-cp38-win_amd64.whl",
"has_sig": false,
"md5_digest": "76aeb06ed06f444345bf9f6e92bd6787",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": "<3.12,>=3.8",
"size": 791913,
"upload_time": "2023-04-07T15:18:00",
"upload_time_iso_8601": "2023-04-07T15:18:00.813124Z",
"url": "https://files.pythonhosted.org/packages/82/52/fc8f44bcf487ca96382160aeb6d1356295390a65c35f8f9ee54933f6f5f9/mknapsack-1.1.12-cp38-cp38-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1b9c25a9caa7806a644c195c2defc370fb7fa946a20b32f05b2d470bb2bc29cc",
"md5": "bd4b4bde876f00b8f88dac9f32cbe7fc",
"sha256": "e28a787d79e17d13280decded3ae7d7d354216b28355adc49dcfe57c74a75e61"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "bd4b4bde876f00b8f88dac9f32cbe7fc",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.12,>=3.8",
"size": 1668397,
"upload_time": "2023-04-07T15:18:02",
"upload_time_iso_8601": "2023-04-07T15:18:02.572188Z",
"url": "https://files.pythonhosted.org/packages/1b/9c/25a9caa7806a644c195c2defc370fb7fa946a20b32f05b2d470bb2bc29cc/mknapsack-1.1.12-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ec6b53f9bee7707f17f5761bb2638a1879c4410c3b26a78bf4bf776d8e94a389",
"md5": "e90f25d1d22253a7291bde634de18ac7",
"sha256": "d67767a49e69baad5a87169d5d71d37b0bbc561e8cb99da56b93abef153ab285"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "e90f25d1d22253a7291bde634de18ac7",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.12,>=3.8",
"size": 1794578,
"upload_time": "2023-04-07T15:18:03",
"upload_time_iso_8601": "2023-04-07T15:18:03.835267Z",
"url": "https://files.pythonhosted.org/packages/ec/6b/53f9bee7707f17f5761bb2638a1879c4410c3b26a78bf4bf776d8e94a389/mknapsack-1.1.12-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6dcad7177c0420aec1564b79857b4a27d725d4aedccb7dbb8cf50af49ef14feb",
"md5": "ef0450a8a329a17f724ab10eb353a850",
"sha256": "76485a4bbb0d63775be99d0fcb49273613abde77df044a1c69144a2ab11a1e6f"
},
"downloads": -1,
"filename": "mknapsack-1.1.12-cp39-cp39-win_amd64.whl",
"has_sig": false,
"md5_digest": "ef0450a8a329a17f724ab10eb353a850",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": "<3.12,>=3.8",
"size": 791916,
"upload_time": "2023-04-07T15:18:05",
"upload_time_iso_8601": "2023-04-07T15:18:05.559338Z",
"url": "https://files.pythonhosted.org/packages/6d/ca/d7177c0420aec1564b79857b4a27d725d4aedccb7dbb8cf50af49ef14feb/mknapsack-1.1.12-cp39-cp39-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d4bbf8a43ec75014fec16b6ba843eb2b7c21c31fad3b93cb835c7278d2201148",
"md5": "4c1846fcd43e055de8dca2e9ed46971b",
"sha256": "176d161bf30db094ede1f829981b4c7578a3677e908702c3cad65146575db83b"
},
"downloads": -1,
"filename": "mknapsack-1.1.12.tar.gz",
"has_sig": false,
"md5_digest": "4c1846fcd43e055de8dca2e9ed46971b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<3.12,>=3.8",
"size": 164270,
"upload_time": "2023-04-07T15:18:06",
"upload_time_iso_8601": "2023-04-07T15:18:06.745037Z",
"url": "https://files.pythonhosted.org/packages/d4/bb/f8a43ec75014fec16b6ba843eb2b7c21c31fad3b93cb835c7278d2201148/mknapsack-1.1.12.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-04-07 15:18:06",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "mknapsack"
}