# mmcq_numba
Faster MMCQ algorithm ( analyze dominant colors in image) with numba in python
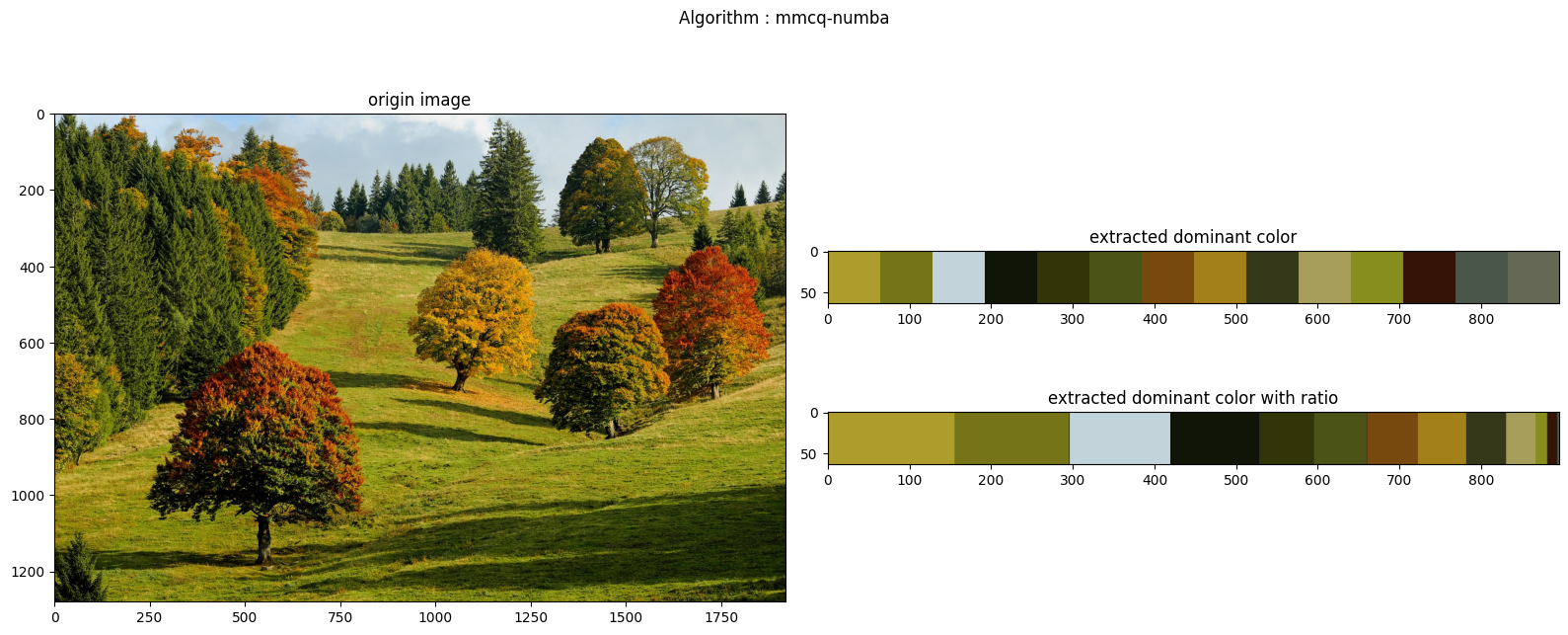
## Installation
``` pip install mmcq-numba ```
## Usage
```python
from mmcq_numba.quantize import mmcq
color_count = 8 # the number of dominant colors
quantize = 5
path = <path to image>
rgb = cv2.cvtColor(cv2.imread(path),cv2.COLOR_BGR2RGB)
width,height,c = rgb.shape
rgb_resize = cv2.resize(rgb, (width//quantize, height//quantize))
width,height,c = rgb_resize.shape
colors = rgb_resize.reshape(width*height, c).astype(np.int64)
# input type must be 2d arrays((size, channels)), and dtype=np.int64
c_map = mmcq(colors, color_count)
```
## Reference
This project is based on [mmcq.py](https://github.com/kanghyojun/mmcq.py)
Raw data
{
"_id": null,
"home_page": "https://github.com/hirokic5/mmcq_numba.git",
"name": "mmcq-numba",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "mmcq dominant-color",
"author": "hirokic5",
"author_email": "kanbac5@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/26/dc/0137ead51e4efbd69c7ee553e81b260a76036e0fca9076b1c0bde7c2a4f7/mmcq_numba-0.1.1.tar.gz",
"platform": null,
"description": "# mmcq_numba\nFaster MMCQ algorithm ( analyze dominant colors in image) with numba in python\n\n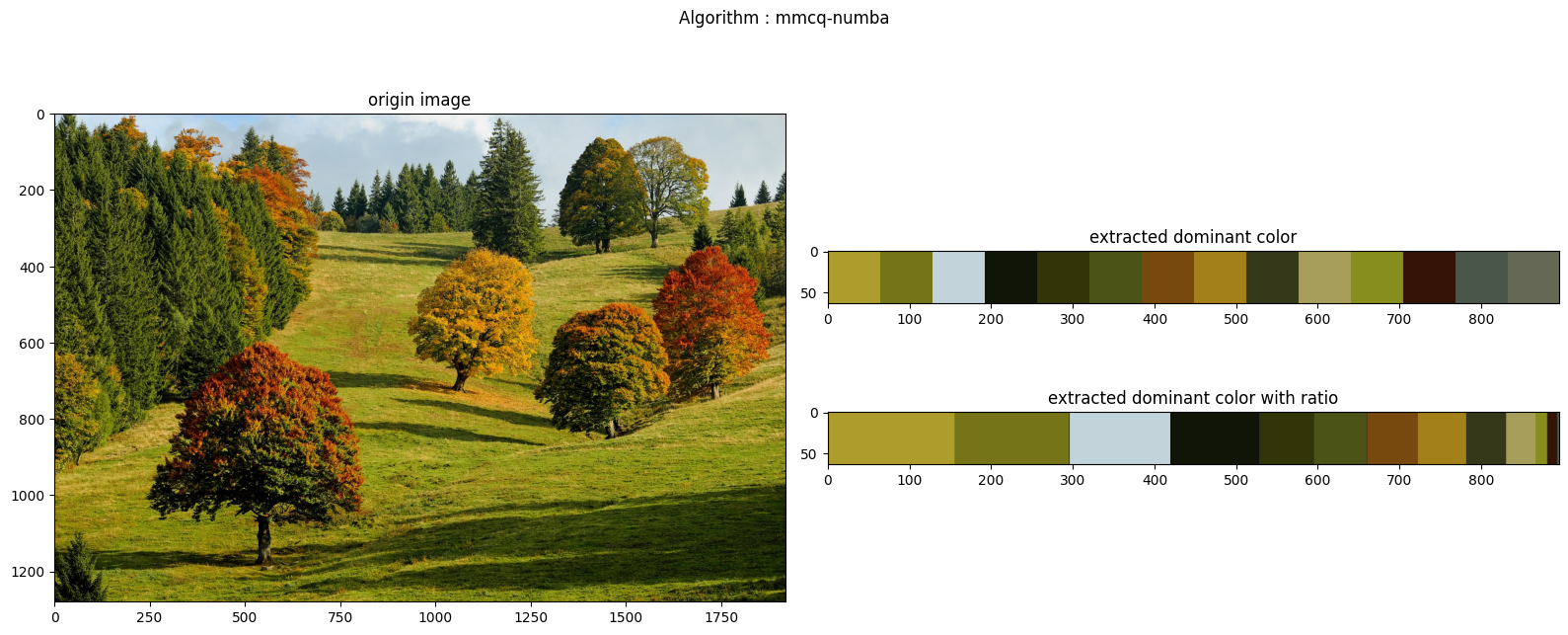\n\n## Installation\n``` pip install mmcq-numba ```\n\n## Usage\n```python\nfrom mmcq_numba.quantize import mmcq\n\ncolor_count = 8 # the number of dominant colors\nquantize = 5 \n\n\npath = <path to image>\nrgb = cv2.cvtColor(cv2.imread(path),cv2.COLOR_BGR2RGB)\nwidth,height,c = rgb.shape\nrgb_resize = cv2.resize(rgb, (width//quantize, height//quantize))\nwidth,height,c = rgb_resize.shape\ncolors = rgb_resize.reshape(width*height, c).astype(np.int64)\n \n# input type must be 2d arrays((size, channels)), and dtype=np.int64\nc_map = mmcq(colors, color_count)\n\n```\n\n## Reference\nThis project is based on [mmcq.py](https://github.com/kanghyojun/mmcq.py)\n",
"bugtrack_url": null,
"license": "Apache-2.0 license",
"summary": "Analyze dominant colors in image with MMCQ algorithm",
"version": "0.1.1",
"split_keywords": [
"mmcq",
"dominant-color"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5d106ee6cfcaa840a06f229af587d9a972b6a1e65ad86519ff81954d4be788e4",
"md5": "c7da1a8f9803cc50fd9b1f027229d414",
"sha256": "87a1b35cf9a0b5349f39d804f775bc21675c52e634195763779743b54cf28719"
},
"downloads": -1,
"filename": "mmcq_numba-0.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c7da1a8f9803cc50fd9b1f027229d414",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 10722,
"upload_time": "2023-02-06T03:14:33",
"upload_time_iso_8601": "2023-02-06T03:14:33.863478Z",
"url": "https://files.pythonhosted.org/packages/5d/10/6ee6cfcaa840a06f229af587d9a972b6a1e65ad86519ff81954d4be788e4/mmcq_numba-0.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "26dc0137ead51e4efbd69c7ee553e81b260a76036e0fca9076b1c0bde7c2a4f7",
"md5": "67354e5b9db34bb6c8cb4c60862633e8",
"sha256": "c9ec85374bb82e1b1caf394bf2ba17b7a80b4741fdd8d448f31c5077f60e5745"
},
"downloads": -1,
"filename": "mmcq_numba-0.1.1.tar.gz",
"has_sig": false,
"md5_digest": "67354e5b9db34bb6c8cb4c60862633e8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 9832,
"upload_time": "2023-02-06T03:14:35",
"upload_time_iso_8601": "2023-02-06T03:14:35.571132Z",
"url": "https://files.pythonhosted.org/packages/26/dc/0137ead51e4efbd69c7ee553e81b260a76036e0fca9076b1c0bde7c2a4f7/mmcq_numba-0.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-02-06 03:14:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "hirokic5",
"github_project": "mmcq_numba.git",
"lcname": "mmcq-numba"
}