[](https://kernc.github.io/backtesting.py/)
Backtesting.py
==============
[](https://github.com/kernc/backtesting.py/actions)
[](https://codecov.io/gh/kernc/backtesting.py)
[](https://pypi.org/project/backtesting)
[](https://pypi.org/project/backtesting)
[](https://github.com/sponsors/kernc)
Backtest trading strategies with Python.
[**Project website**](https://kernc.github.io/backtesting.py) + [Documentation]
[Documentation]: https://kernc.github.io/backtesting.py/doc/backtesting/
Installation
------------
$ pip install backtesting
Usage
-----
```python
from backtesting import Backtest, Strategy
from backtesting.lib import crossover
from backtesting.test import SMA, GOOG
class SmaCross(Strategy):
def init(self):
price = self.data.Close
self.ma1 = self.I(SMA, price, 10)
self.ma2 = self.I(SMA, price, 20)
def next(self):
if crossover(self.ma1, self.ma2):
self.buy()
elif crossover(self.ma2, self.ma1):
self.sell()
bt = Backtest(GOOG, SmaCross, commission=.002,
exclusive_orders=True)
stats = bt.run()
bt.plot()
```
Results in:
```text
Start 2004-08-19 00:00:00
End 2013-03-01 00:00:00
Duration 3116 days 00:00:00
Exposure Time [%] 94.27
Equity Final [$] 68935.12
Equity Peak [$] 68991.22
Return [%] 589.35
Buy & Hold Return [%] 703.46
Return (Ann.) [%] 25.42
Volatility (Ann.) [%] 38.43
Sharpe Ratio 0.66
Sortino Ratio 1.30
Calmar Ratio 0.77
Max. Drawdown [%] -33.08
Avg. Drawdown [%] -5.58
Max. Drawdown Duration 688 days 00:00:00
Avg. Drawdown Duration 41 days 00:00:00
# Trades 93
Win Rate [%] 53.76
Best Trade [%] 57.12
Worst Trade [%] -16.63
Avg. Trade [%] 1.96
Max. Trade Duration 121 days 00:00:00
Avg. Trade Duration 32 days 00:00:00
Profit Factor 2.13
Expectancy [%] 6.91
SQN 1.78
Kelly Criterion 0.6134
_strategy SmaCross(n1=10, n2=20)
_equity_curve Equ...
_trades Size EntryB...
dtype: object
```
[](https://kernc.github.io/backtesting.py/#example)
Find more usage examples in the [documentation].
Features
--------
* Simple, well-documented API
* Blazing fast execution
* Built-in optimizer
* Library of composable base strategies and utilities
* Indicator-library-agnostic
* Supports _any_ financial instrument with candlestick data
* Detailed results
* Interactive visualizations
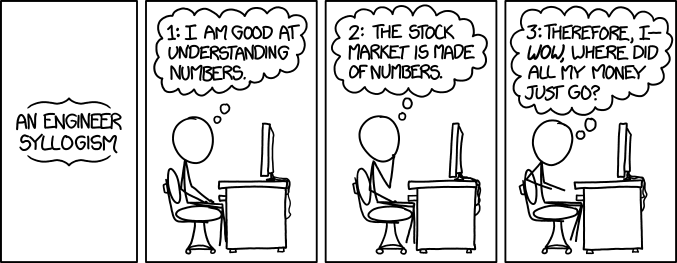
Bugs
----
Before reporting bugs or posting to the
[discussion board](https://github.com/kernc/backtesting.py/discussions),
please read [contributing guidelines](CONTRIBUTING.md), particularly the section
about crafting useful bug reports and ```` ``` ````-fencing your code. We thank you!
Alternatives
------------
See [alternatives.md] for a list of alternative Python
backtesting frameworks and related packages.
[alternatives.md]: https://github.com/kernc/backtesting.py/blob/master/doc/alternatives.md
Raw data
{
"_id": null,
"home_page": "https://kernc.github.io/backtesting.py/",
"name": "multiple-backtesting",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "algo, algorithmic, ashi, backtest, backtesting, bitcoin, bokeh, bonds, candle, candlestick, cboe, chart, cme, commodities, crash, crypto, currency, doji, drawdown, equity, etf, ethereum, exchange, finance, financial, forecast, forex, fund, futures, fx, fxpro, gold, heiken, historical, indicator, invest, investing, investment, macd, market, mechanical, money, oanda, ohlc, ohlcv, order, price, profit, quant, quantitative, rsi, silver, simulation, stocks, strategy, ticker, trader, trading, tradingview, usd",
"author": "Zach L\u00fbster",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/9f/34/9f8bca95f32621bc3e69bfe249e6ef06cd0b917b51550d8fe0146c48c666/multiple_backtesting-0.0.3.tar.gz",
"platform": null,
"description": "[](https://kernc.github.io/backtesting.py/)\r\n\r\nBacktesting.py\r\n==============\r\n[](https://github.com/kernc/backtesting.py/actions)\r\n[](https://codecov.io/gh/kernc/backtesting.py)\r\n[](https://pypi.org/project/backtesting)\r\n[](https://pypi.org/project/backtesting)\r\n[](https://github.com/sponsors/kernc)\r\n\r\nBacktest trading strategies with Python.\r\n\r\n[**Project website**](https://kernc.github.io/backtesting.py) + [Documentation]\r\n\r\n[Documentation]: https://kernc.github.io/backtesting.py/doc/backtesting/\r\n\r\n\r\nInstallation\r\n------------\r\n\r\n $ pip install backtesting\r\n\r\n\r\nUsage\r\n-----\r\n```python\r\nfrom backtesting import Backtest, Strategy\r\nfrom backtesting.lib import crossover\r\n\r\nfrom backtesting.test import SMA, GOOG\r\n\r\n\r\nclass SmaCross(Strategy):\r\n def init(self):\r\n price = self.data.Close\r\n self.ma1 = self.I(SMA, price, 10)\r\n self.ma2 = self.I(SMA, price, 20)\r\n\r\n def next(self):\r\n if crossover(self.ma1, self.ma2):\r\n self.buy()\r\n elif crossover(self.ma2, self.ma1):\r\n self.sell()\r\n\r\n\r\nbt = Backtest(GOOG, SmaCross, commission=.002,\r\n exclusive_orders=True)\r\nstats = bt.run()\r\nbt.plot()\r\n```\r\n\r\nResults in:\r\n\r\n```text\r\nStart 2004-08-19 00:00:00\r\nEnd 2013-03-01 00:00:00\r\nDuration 3116 days 00:00:00\r\nExposure Time [%] 94.27\r\nEquity Final [$] 68935.12\r\nEquity Peak [$] 68991.22\r\nReturn [%] 589.35\r\nBuy & Hold Return [%] 703.46\r\nReturn (Ann.) [%] 25.42\r\nVolatility (Ann.) [%] 38.43\r\nSharpe Ratio 0.66\r\nSortino Ratio 1.30\r\nCalmar Ratio 0.77\r\nMax. Drawdown [%] -33.08\r\nAvg. Drawdown [%] -5.58\r\nMax. Drawdown Duration 688 days 00:00:00\r\nAvg. Drawdown Duration 41 days 00:00:00\r\n# Trades 93\r\nWin Rate [%] 53.76\r\nBest Trade [%] 57.12\r\nWorst Trade [%] -16.63\r\nAvg. Trade [%] 1.96\r\nMax. Trade Duration 121 days 00:00:00\r\nAvg. Trade Duration 32 days 00:00:00\r\nProfit Factor 2.13\r\nExpectancy [%] 6.91\r\nSQN 1.78\r\nKelly Criterion 0.6134\r\n_strategy SmaCross(n1=10, n2=20)\r\n_equity_curve Equ...\r\n_trades Size EntryB...\r\ndtype: object\r\n```\r\n[](https://kernc.github.io/backtesting.py/#example)\r\n\r\nFind more usage examples in the [documentation].\r\n\r\n\r\nFeatures\r\n--------\r\n* Simple, well-documented API\r\n* Blazing fast execution\r\n* Built-in optimizer\r\n* Library of composable base strategies and utilities\r\n* Indicator-library-agnostic\r\n* Supports _any_ financial instrument with candlestick data\r\n* Detailed results\r\n* Interactive visualizations\r\n\r\n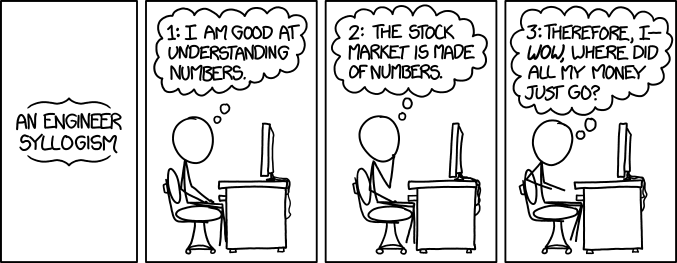\r\n\r\n\r\nBugs\r\n----\r\nBefore reporting bugs or posting to the\r\n[discussion board](https://github.com/kernc/backtesting.py/discussions),\r\nplease read [contributing guidelines](CONTRIBUTING.md), particularly the section\r\nabout crafting useful bug reports and ```` ``` ````-fencing your code. We thank you!\r\n\r\n\r\nAlternatives\r\n------------\r\nSee [alternatives.md] for a list of alternative Python\r\nbacktesting frameworks and related packages.\r\n\r\n[alternatives.md]: https://github.com/kernc/backtesting.py/blob/master/doc/alternatives.md\r\n",
"bugtrack_url": null,
"license": "AGPL-3.0",
"summary": "Backtest trading strategies in Python",
"version": "0.0.3",
"project_urls": {
"Documentation": "https://kernc.github.io/backtesting.py/doc/backtesting/",
"Homepage": "https://kernc.github.io/backtesting.py/",
"Source": "https://github.com/Scottman625/backtesting.py/",
"Tracker": "https://github.com/Scottman625/backtesting.py/issues"
},
"split_keywords": [
"algo",
" algorithmic",
" ashi",
" backtest",
" backtesting",
" bitcoin",
" bokeh",
" bonds",
" candle",
" candlestick",
" cboe",
" chart",
" cme",
" commodities",
" crash",
" crypto",
" currency",
" doji",
" drawdown",
" equity",
" etf",
" ethereum",
" exchange",
" finance",
" financial",
" forecast",
" forex",
" fund",
" futures",
" fx",
" fxpro",
" gold",
" heiken",
" historical",
" indicator",
" invest",
" investing",
" investment",
" macd",
" market",
" mechanical",
" money",
" oanda",
" ohlc",
" ohlcv",
" order",
" price",
" profit",
" quant",
" quantitative",
" rsi",
" silver",
" simulation",
" stocks",
" strategy",
" ticker",
" trader",
" trading",
" tradingview",
" usd"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "747d27553a55c6f65d6f743df88bbec415680d047050ac036d91afc4e4c876e3",
"md5": "6778ed8ec1d89f5830395b86b9416724",
"sha256": "5dbaf78afa128821820cfd36046f04f0efe49cb2badf7b8cc9fa04fd4d2808cf"
},
"downloads": -1,
"filename": "multiple_backtesting-0.0.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6778ed8ec1d89f5830395b86b9416724",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 180072,
"upload_time": "2024-07-06T13:43:32",
"upload_time_iso_8601": "2024-07-06T13:43:32.816907Z",
"url": "https://files.pythonhosted.org/packages/74/7d/27553a55c6f65d6f743df88bbec415680d047050ac036d91afc4e4c876e3/multiple_backtesting-0.0.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "9f349f8bca95f32621bc3e69bfe249e6ef06cd0b917b51550d8fe0146c48c666",
"md5": "a968ee7593f34d9ad17da104d5a0a9fc",
"sha256": "2ea16a7fa65c0633f82889fce67e739e89b1f78075b6e17a4d6a310e35b81552"
},
"downloads": -1,
"filename": "multiple_backtesting-0.0.3.tar.gz",
"has_sig": false,
"md5_digest": "a968ee7593f34d9ad17da104d5a0a9fc",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 183148,
"upload_time": "2024-07-06T13:43:35",
"upload_time_iso_8601": "2024-07-06T13:43:35.517414Z",
"url": "https://files.pythonhosted.org/packages/9f/34/9f8bca95f32621bc3e69bfe249e6ef06cd0b917b51550d8fe0146c48c666/multiple_backtesting-0.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-06 13:43:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Scottman625",
"github_project": "backtesting.py",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "multiple-backtesting"
}