# video-streamer
Video streamer to be used with MXCuBE. The streamer currently supports streaming from Tango (Lima) devices, as well as Redis and MJPEG streams and can be extened to be used with other camera solutions as well. The output streams are either MJPEG or MPEG1 with an option to use a secondary output stream to a Redis Pub/Sub channel.
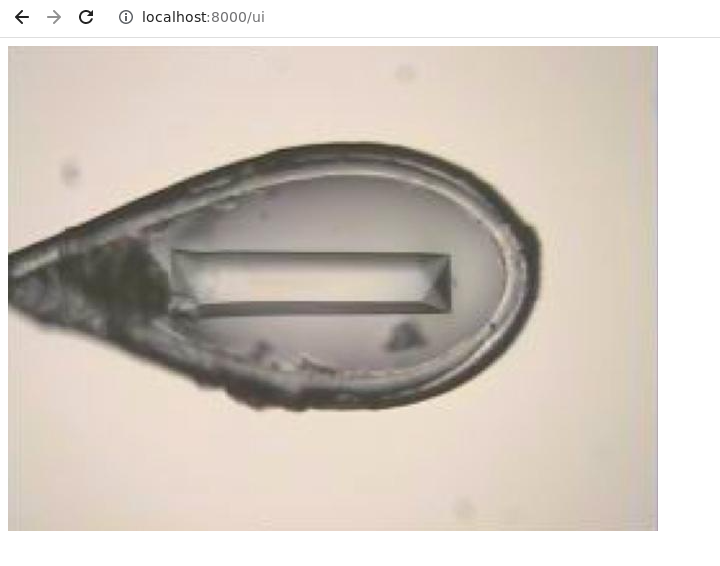
### Documentation 📚
Check out the full [documentation](https://mxcube.github.io/video-streamer/) to explore everything this project has to offer!
Including:
- Information about all supported input camera types
- A detailed guide on how to start and run the project
- A developers guide
- An FAQ
- and more!
### Installation
```
git clone https://github.com/mxcube/video-streamer.git
cd video-streamer
# optional
conda env create -f conda-environment.yml
# For development
pip install -e .
# For usage
pip install .
```
### Usage
```
usage: video-streamer [-h] [-c CONFIG_FILE_PATH] [-uri URI] [-hs HOST]
[-p PORT] [-q QUALITY] [-s SIZE] [-of OUTPUT_FORMAT]
[-id HASH] [-d] [-r] [-rhs REDIS_HOST] [-rp REDIS_PORT]
[-rk REDIS_CHANNEL] [-irc IN_REDIS_CHANNEL]
mxcube video streamer
options:
-h, --help show this help message and exit
-c CONFIG_FILE_PATH, --config CONFIG_FILE_PATH
Configuration file path
-uri URI, --uri URI Tango device URI
-hs HOST, --host HOST
Host name to listen on for incomming client
connections default (0.0.0.0)
-p PORT, --port PORT Port
-q QUALITY, --quality QUALITY
Compresion rate/quality
-s SIZE, --size SIZE size
-of OUTPUT_FORMAT, --output-format OUTPUT_FORMAT
output format, MPEG1 or MJPEG
-id HASH, --id HASH Stream id
-d, --debug Debug true or false
-r, --redis Use redis-server
-rhs REDIS_HOST, --redis-host REDIS_HOST
Host name of redis server to send to
-rp REDIS_PORT, --redis-port REDIS_PORT
Port of redis server
-rk REDIS_CHANNEL, --redis-channel REDIS_CHANNEL
Key for saving to redis database
-irc IN_REDIS_CHANNEL, --in_redis_channel IN_REDIS_CHANNEL
Channel for RedisCamera to listen to
```
There is the possibility to use a configuration file instead of command line arguments. All command line arguments except debug are ignored if a config file is used. The configuration file also makes it possible to configure several sources while the command line only allows configuration of a single source.
#### Example command line (for testing):
```
video-streamer -d -of MPEG1 -uri test
```
#### Example configuration file (config.json):
The configuration file format is JSON. A test image is used when the input_uri is set to "test". The example below creates one MPEG1 stream and one MJPEG stream from the test image. There is a defualt test/demo UI to see the video stream on http://localhost:[port]/ui. In example below case:
MPEG1: http://localhost:8000/ui
MJPEG: http://localhost:8001/ui
```
video-streamer -c config.json
config.json:
{
"sources": {
"0.0.0.0:8000": {
"input_uri": "test",
"quality": 4,
"format": "MPEG1"
},
"0.0.0.0:8001": {
"input_uri": "test",
"quality": 4,
"format": "MJPEG"
}
}
}
```
### Dual Streaming: Seamlessly Serve MJPEG and Redis Pub/Sub Video Feeds
When generating an MJPEG stream using any of the cameras (except for `MJPEGCamera`) implemented in `video-streamer`, it is possible to use a `Redis` Pub/Sub channel as additional Video feed.
Below you can see an example on how to do that from the command line:
```
video-streamer -d -of MPEG1 -uri test -r -rhs localhost -rp 6379 -rk video-streamer
```
where `-r` flag is needed to allow the stream to redis , `-rhs`,`-rp`, `-rk` define the host, port and channel of the targeted `Redis` Pub/Sub respectively.
The format of the frames send to `Redis` looks as follows:
```
frame_dict = {
"data": [encoded image data],
"size": [image size],
"time": [timestamp of image_polling],
"frame_number": [number of frame send to Redis starting at 0],
}
```
Raw data
{
"_id": null,
"home_page": "http://github.com/mxcube/video-streamer",
"name": "mxcube_video_streamer",
"maintainer": "Marcus Oskarsson",
"docs_url": null,
"requires_python": "<3.12,>=3.10",
"maintainer_email": "oscarsso@esrf.fr",
"keywords": "mxcube, fast-api-streamer, video_streamer, mxcubeweb",
"author": "Marcus Oskarsson",
"author_email": "oscarsso@esrf.fr",
"download_url": "https://files.pythonhosted.org/packages/d7/bc/102c90b237d0163408e485375d0fb67ebd8675a8b7557eb39c4caeeb0532/mxcube_video_streamer-1.8.3.tar.gz",
"platform": null,
"description": "# video-streamer\nVideo streamer to be used with MXCuBE. The streamer currently supports streaming from Tango (Lima) devices, as well as Redis and MJPEG streams and can be extened to be used with other camera solutions as well. The output streams are either MJPEG or MPEG1 with an option to use a secondary output stream to a Redis Pub/Sub channel.\n\n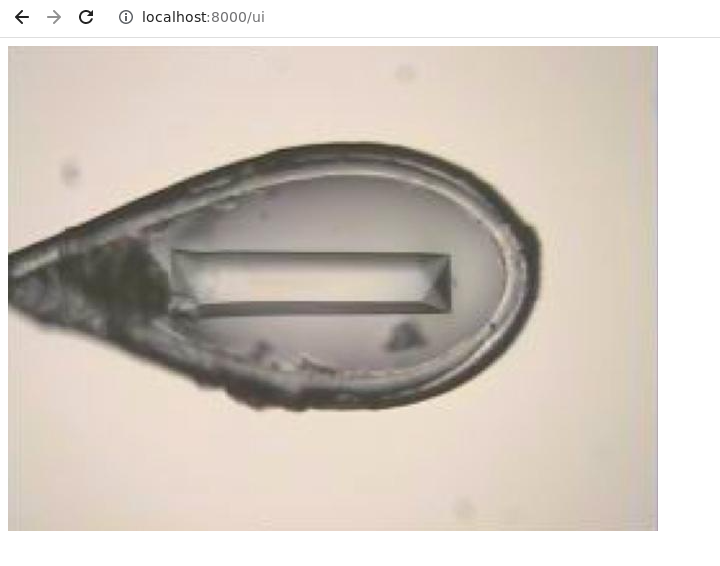\n\n### Documentation \ud83d\udcda\n\nCheck out the full [documentation](https://mxcube.github.io/video-streamer/) to explore everything this project has to offer!\nIncluding:\n\n- Information about all supported input camera types\n- A detailed guide on how to start and run the project\n- A developers guide\n- An FAQ\n- and more!\n\n### Installation\n\n```\ngit clone https://github.com/mxcube/video-streamer.git\ncd video-streamer\n\n# optional \nconda env create -f conda-environment.yml\n\n# For development\npip install -e .\n\n# For usage \npip install .\n```\n\n### Usage\n```\nusage: video-streamer [-h] [-c CONFIG_FILE_PATH] [-uri URI] [-hs HOST]\n [-p PORT] [-q QUALITY] [-s SIZE] [-of OUTPUT_FORMAT]\n [-id HASH] [-d] [-r] [-rhs REDIS_HOST] [-rp REDIS_PORT]\n [-rk REDIS_CHANNEL] [-irc IN_REDIS_CHANNEL]\n\nmxcube video streamer\n\noptions:\n -h, --help show this help message and exit\n -c CONFIG_FILE_PATH, --config CONFIG_FILE_PATH\n Configuration file path\n -uri URI, --uri URI Tango device URI\n -hs HOST, --host HOST\n Host name to listen on for incomming client\n connections default (0.0.0.0)\n -p PORT, --port PORT Port\n -q QUALITY, --quality QUALITY\n Compresion rate/quality\n -s SIZE, --size SIZE size\n -of OUTPUT_FORMAT, --output-format OUTPUT_FORMAT\n output format, MPEG1 or MJPEG\n -id HASH, --id HASH Stream id\n -d, --debug Debug true or false\n -r, --redis Use redis-server\n -rhs REDIS_HOST, --redis-host REDIS_HOST\n Host name of redis server to send to\n -rp REDIS_PORT, --redis-port REDIS_PORT\n Port of redis server\n -rk REDIS_CHANNEL, --redis-channel REDIS_CHANNEL\n Key for saving to redis database\n -irc IN_REDIS_CHANNEL, --in_redis_channel IN_REDIS_CHANNEL\n Channel for RedisCamera to listen to\n\n```\n\nThere is the possibility to use a configuration file instead of command line arguments. All command line arguments except debug are ignored if a config file is used. The configuration file also makes it possible to configure several sources while the command line only allows configuration of a single source.\n\n#### Example command line (for testing):\n```\nvideo-streamer -d -of MPEG1 -uri test\n```\n\n#### Example configuration file (config.json):\nThe configuration file format is JSON. A test image is used when the input_uri is set to \"test\". The example below creates one MPEG1 stream and one MJPEG stream from the test image. There is a defualt test/demo UI to see the video stream on http://localhost:[port]/ui. In example below case:\n \n MPEG1: http://localhost:8000/ui\n \n MJPEG: http://localhost:8001/ui\n\n\n```\nvideo-streamer -c config.json\n\nconfig.json:\n{\n \"sources\": {\n \"0.0.0.0:8000\": {\n \"input_uri\": \"test\",\n \"quality\": 4,\n \"format\": \"MPEG1\"\n },\n \"0.0.0.0:8001\": {\n \"input_uri\": \"test\",\n \"quality\": 4,\n \"format\": \"MJPEG\"\n }\n }\n}\n```\n\n### Dual Streaming: Seamlessly Serve MJPEG and Redis Pub/Sub Video Feeds\n\nWhen generating an MJPEG stream using any of the cameras (except for `MJPEGCamera`) implemented in `video-streamer`, it is possible to use a `Redis` Pub/Sub channel as additional Video feed.\nBelow you can see an example on how to do that from the command line:\n```\nvideo-streamer -d -of MPEG1 -uri test -r -rhs localhost -rp 6379 -rk video-streamer\n```\n\nwhere `-r` flag is needed to allow the stream to redis , `-rhs`,`-rp`, `-rk` define the host, port and channel of the targeted `Redis` Pub/Sub respectively.\n\nThe format of the frames send to `Redis` looks as follows:\n\n```\nframe_dict = {\n \"data\": [encoded image data],\n \"size\": [image size],\n \"time\": [timestamp of image_polling],\n \"frame_number\": [number of frame send to Redis starting at 0],\n}\n```",
"bugtrack_url": null,
"license": "MIT",
"summary": "FastAPI Based video streamer",
"version": "1.8.3",
"project_urls": {
"Documentation": "https://mxcube.github.io/video-streamer/",
"Homepage": "http://github.com/mxcube/video-streamer",
"Repository": "http://github.com/mxcube/video-streamer"
},
"split_keywords": [
"mxcube",
" fast-api-streamer",
" video_streamer",
" mxcubeweb"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "04a1d703f99c01979f60bc58f7c66394ee213d49ec33af87c764f30f97432a42",
"md5": "6bd32a016e4ddc63e0a8991c92750062",
"sha256": "a296e8eb48d111eeda909633c3c940cc4ce07b1bedbb907894d9f296560e4448"
},
"downloads": -1,
"filename": "mxcube_video_streamer-1.8.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6bd32a016e4ddc63e0a8991c92750062",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<3.12,>=3.10",
"size": 6802173,
"upload_time": "2025-07-25T08:52:35",
"upload_time_iso_8601": "2025-07-25T08:52:35.943391Z",
"url": "https://files.pythonhosted.org/packages/04/a1/d703f99c01979f60bc58f7c66394ee213d49ec33af87c764f30f97432a42/mxcube_video_streamer-1.8.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d7bc102c90b237d0163408e485375d0fb67ebd8675a8b7557eb39c4caeeb0532",
"md5": "a208b0916610f89867665e24f06f4ed0",
"sha256": "a2111493931507dc192d6c0e636250e3e554405191b73325742e0570cdd01bd3"
},
"downloads": -1,
"filename": "mxcube_video_streamer-1.8.3.tar.gz",
"has_sig": false,
"md5_digest": "a208b0916610f89867665e24f06f4ed0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<3.12,>=3.10",
"size": 6758124,
"upload_time": "2025-07-25T08:52:39",
"upload_time_iso_8601": "2025-07-25T08:52:39.053780Z",
"url": "https://files.pythonhosted.org/packages/d7/bc/102c90b237d0163408e485375d0fb67ebd8675a8b7557eb39c4caeeb0532/mxcube_video_streamer-1.8.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-07-25 08:52:39",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "mxcube",
"github_project": "video-streamer",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "mxcube_video_streamer"
}