Name | ndf-parse JSON |
Version |
0.2.0
JSON |
| download |
home_page | None |
Summary | A package for parsing and editing Eugen Systems ndf files. |
upload_time | 2024-08-04 16:32:44 |
maintainer | None |
docs_url | None |
author | Ulibos |
requires_python | >=3.8 |
license | The MIT License (MIT) Copyright (c) 2023 Ulibos Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
ndf
parser
parsing
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
<!-- this README was generated with `scripts/build_readme.py` -->

## ndf-parse
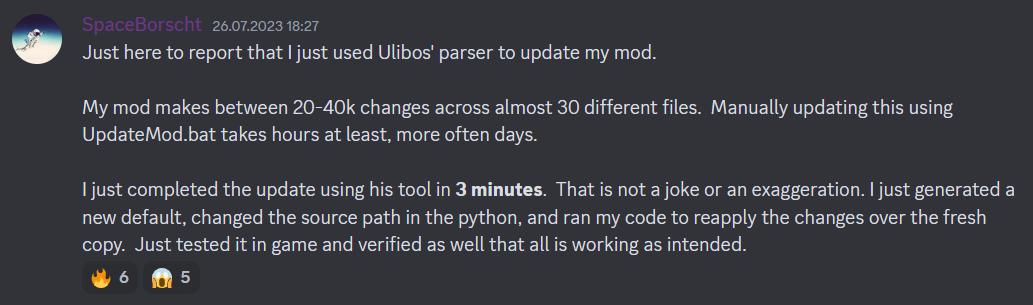
This package allows to parse Eugen Systems ndf files, modify them and write back
modified versions as a valid ndf code. It was created to allow easier editing of
warno mods than what is currently available with game's own tools.
An example of a script doubling logistics capacity for all vehicles:
```python
import ndf_parse as ndf
# setup mod donor and destination mods
mod = ndf.Mod("path/to/src/mod", "path/to/dst/mod")
# create/update destination mod
mod.check_if_src_is_newer()
with mod.edit(r"GameData\Generated\Gameplay\Gfx\UniteDescriptor.ndf") as source:
# filter out root descriptors that have supply in them
for logi_descr in source.match_pattern(
"TEntityDescriptor(ModulesDescriptors = [TSupplyModuleDescriptor()])"
):
descriptors = logi_descr.v.by_member("ModulesDescriptors").v # get modules list
supply_row = descriptors.find_by_cond( # find supply module
# safe way to check if row has type and equals the one we search for
lambda x: getattr(x.v, "type", None) == "TSupplyModuleDescriptor"
)
# get capacity row
supply_capacity_row = supply_row.v.by_member("SupplyCapacity")
old_capacity = supply_capacity_row.v
new_capacity = float(old_capacity) * 2 # process value
supply_capacity_row.v = str(new_capacity)
print(f"{logi_descr.namespace}: new capacity = {new_capacity}") # log result
```
> [!NOTE]
> This package was created for and tested with Windows only! More on that in
> [caveats](#caveats) section.
### Prerequisites
- [python](https://www.python.org/downloads/) >= 3.8 (this package
was developed on 3.8 and tested on minor versions from 3.8 to 3.12).
### Installation
```bat
pip install ndf-parse
```
> [!NOTE]
> Note that if you didn't add python to the `PATH` variable during
> installation, you'll have to run it with full path to **pip**, for example
> `"C:\Users\User\AppData\Local\Python311-64\Scripts\pip.exe"
> install ndf_parse`
As an alternative, you can download and install this package from
[github releases][releases].
[releases]: https://github.com/Ulibos/ndf-parse/releases
### Using This Package
For usage please refer to the [documentation][docs].
[docs]: https://ulibos.github.io/ndf-parse
### Caveats
This package gets shipped with an `ndf.dll` containing the tree-sitter
language parser. It's linkage is also hardcoded in module's `__init__`. If
you're planning to use this on linux or MacOS (why?..), you will have to [build
the lib yourself][ndf] and set an env variable
`NDF_LIB_PATH=path/to/your/lib`.
[ndf]: https://github.com/Ulibos/tree-sitter-ndf
### Developing
In order to develop this module you will need to fork this repo, clone the fork
and run the following command:
```powershell
pip install -e "path\to\cloned\repo[dev]"
```
It will load most dependencies automatically. Only thing you will have to
provide manually is an `ndf.dll` ([see below][custom-ndf]). You
can then build a release package using `scripts\build_package.bat`
script. It outputs the result to a `build\package` folder. By default
the build sctipt uses a local library (`ndf_parse\bin\ndf.dll`). If
there is none, it copies one from tree-sitter's default build path to local
path. If there is also none then it refuses to build.
[custom-ndf]: #using-in-pair-with-custom-tree-sitter-ndf
[black](https://pypi.org/project/black/) is used for code styling
with line length limit == 79. Code is (mostly) type hinted.
`.gitignore` does not store editor specific excludes, I store those in
`.git\info\exclude`.
#### Repo Structure
- `build` - temp folder to store build data, untracked
- `ndf_parse` - package source code
- `sphinx` - documentation sources
- `scripts` - mostly scripts for building stuff
- `tests` - basic testing scripts
#### Docs Development
##### Current Version Build
To build docs for current commit use `scripts\build_docs.bat`. Your
docs will be in `build\docs`.
##### Multiversion Build
To build docs for all releases follow these steps:
1. Make sure to bump release version and commit all changes related to the
latest release (and stash what is left).
2. Tag the release with semver (example: `v1.0.5`, `v` is mandatory).
3. Add new tag to `publish_tags` variable in
`sphinx\conf.py`.
4. Remove `build\multiver` to ensure clean build.
5. Run `python scripts\build_multidocs.py`. Result will be in
`build\multiver`.
6. Checkout `docs` branch.
7. Remove old docs dirs (named by their releases), move new ones, including
`build\multiver\index.html` (but excluding `.doctree`
dirs in each version build, they are not needed for serving), to the root
of the repo.
8. Add new stuff to git (be careful not to include junk, there is no
`.gitignore`) and commit.
> [!NOTE]
> Things to keep in mind: sphinx-multiversion arranges releases based on commit
> date, not semver number. So be careful when rebasing/amending older releases.
#### Tests
If you're planning to test scripts from the documentation (the ones in
`sphinx\code`), you will have to setup 2 env variables in your
terminal:
```bat
set MOD_SRC="path\to\source_mod"
set MOD_DST="path\to\destination_mod"
```
Currently there are only tests for [ndf_parse.model][ref-model] and docs' code
snippets and examples. Docstrings code is tested with sphinx via
`tests\docs_tests.bat`, py script with the same name has examples
tests and a deprecated version of doctests.
[ref-model]: https://ulibos.github.io/ndf-parse/v0.2.0/ndf-parse/model.html
#### Using in Pair With Custom tree-sitter-ndf
This package looks for an `ntf.dll` in the following places
(descending priority):
1. `NDF_LIB_PATH` env variable
(`"C:\custom\path\to\ndf.dll"`),
2. default tree-sitter's build path
(`"%LocalAppData%\tree-sitter\lib\ndf.dll"`),
3. a copy bundled with the package (`"ndf_parse\bin\ndf.dll"`).
The repo itself does not hold a prebuilt copy of the library so you'll have to
either yank one from a release wheel (it's just a renamed zip) or build one
[from source][ndf].
#### Pull Requests and Issues
I have no idea on how frequently I'll be able to respond to those, so expect
delays. You might find it easier catching me on [WarYes discord][waryes] or
[Eugen discord][eugen] in case you have some blocking issue or a PR.
[waryes]: https://discord.gg/gqBgvgGj8H
[eugen]: https://discord.gg/sheyBRnqKP
### Credits
Created by Ulibos, 2023-2024.
Raw data
{
"_id": null,
"home_page": null,
"name": "ndf-parse",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "ndf, parser, parsing",
"author": "Ulibos",
"author_email": null,
"download_url": null,
"platform": null,
"description": "<!-- this README was generated with `scripts/build_readme.py` -->\r\n\r\n\r\n\r\n## ndf-parse\r\n\r\n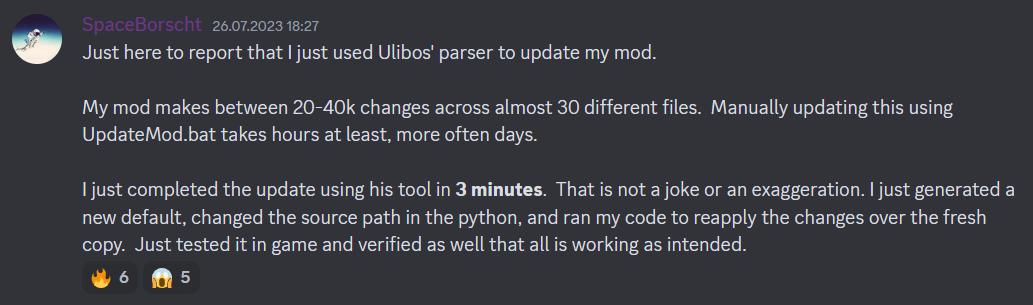\r\n\r\nThis package allows to parse Eugen Systems ndf files, modify them and write back\r\nmodified versions as a valid ndf code. It was created to allow easier editing of\r\nwarno mods than what is currently available with game's own tools.\r\n\r\nAn example of a script doubling logistics capacity for all vehicles:\r\n\r\n```python\r\nimport ndf_parse as ndf\r\n\r\n# setup mod donor and destination mods\r\nmod = ndf.Mod(\"path/to/src/mod\", \"path/to/dst/mod\")\r\n# create/update destination mod\r\nmod.check_if_src_is_newer()\r\n\r\nwith mod.edit(r\"GameData\\Generated\\Gameplay\\Gfx\\UniteDescriptor.ndf\") as source:\r\n # filter out root descriptors that have supply in them\r\n for logi_descr in source.match_pattern(\r\n \"TEntityDescriptor(ModulesDescriptors = [TSupplyModuleDescriptor()])\"\r\n ):\r\n descriptors = logi_descr.v.by_member(\"ModulesDescriptors\").v # get modules list\r\n supply_row = descriptors.find_by_cond( # find supply module\r\n # safe way to check if row has type and equals the one we search for\r\n lambda x: getattr(x.v, \"type\", None) == \"TSupplyModuleDescriptor\"\r\n )\r\n # get capacity row\r\n supply_capacity_row = supply_row.v.by_member(\"SupplyCapacity\")\r\n old_capacity = supply_capacity_row.v\r\n new_capacity = float(old_capacity) * 2 # process value\r\n supply_capacity_row.v = str(new_capacity)\r\n\r\n print(f\"{logi_descr.namespace}: new capacity = {new_capacity}\") # log result\r\n```\r\n\r\n> [!NOTE]\r\n> This package was created for and tested with Windows only! More on that in\r\n> [caveats](#caveats) section.\r\n\r\n### Prerequisites\r\n\r\n- [python](https://www.python.org/downloads/) >= 3.8 (this package\r\n was developed on 3.8 and tested on minor versions from 3.8 to 3.12).\r\n\r\n### Installation\r\n\r\n```bat\r\npip install ndf-parse\r\n```\r\n\r\n> [!NOTE]\r\n> Note that if you didn't add python to the `PATH` variable during\r\n> installation, you'll have to run it with full path to **pip**, for example\r\n> `\"C:\\Users\\User\\AppData\\Local\\Python311-64\\Scripts\\pip.exe\"\r\n> install ndf_parse`\r\n\r\nAs an alternative, you can download and install this package from\r\n[github releases][releases].\r\n\r\n[releases]: https://github.com/Ulibos/ndf-parse/releases\r\n\r\n### Using This Package\r\n\r\nFor usage please refer to the [documentation][docs].\r\n\r\n[docs]: https://ulibos.github.io/ndf-parse\r\n\r\n### Caveats\r\n\r\nThis package gets shipped with an `ndf.dll` containing the tree-sitter\r\nlanguage parser. It's linkage is also hardcoded in module's `__init__`. If\r\nyou're planning to use this on linux or MacOS (why?..), you will have to [build\r\nthe lib yourself][ndf] and set an env variable\r\n`NDF_LIB_PATH=path/to/your/lib`.\r\n\r\n[ndf]: https://github.com/Ulibos/tree-sitter-ndf\r\n\r\n### Developing\r\n\r\nIn order to develop this module you will need to fork this repo, clone the fork\r\nand run the following command:\r\n\r\n```powershell\r\npip install -e \"path\\to\\cloned\\repo[dev]\"\r\n```\r\n\r\nIt will load most dependencies automatically. Only thing you will have to\r\nprovide manually is an `ndf.dll` ([see below][custom-ndf]). You\r\ncan then build a release package using `scripts\\build_package.bat`\r\nscript. It outputs the result to a `build\\package` folder. By default\r\nthe build sctipt uses a local library (`ndf_parse\\bin\\ndf.dll`). If\r\nthere is none, it copies one from tree-sitter's default build path to local\r\npath. If there is also none then it refuses to build.\r\n\r\n[custom-ndf]: #using-in-pair-with-custom-tree-sitter-ndf\r\n\r\n[black](https://pypi.org/project/black/) is used for code styling\r\nwith line length limit == 79. Code is (mostly) type hinted.\r\n\r\n`.gitignore` does not store editor specific excludes, I store those in\r\n`.git\\info\\exclude`.\r\n\r\n#### Repo Structure\r\n\r\n- `build` - temp folder to store build data, untracked\r\n- `ndf_parse` - package source code\r\n- `sphinx` - documentation sources\r\n- `scripts` - mostly scripts for building stuff\r\n- `tests` - basic testing scripts\r\n\r\n#### Docs Development\r\n\r\n##### Current Version Build\r\n\r\nTo build docs for current commit use `scripts\\build_docs.bat`. Your\r\ndocs will be in `build\\docs`.\r\n\r\n##### Multiversion Build\r\n\r\nTo build docs for all releases follow these steps:\r\n\r\n1. Make sure to bump release version and commit all changes related to the\r\n latest release (and stash what is left).\r\n2. Tag the release with semver (example: `v1.0.5`, `v` is mandatory).\r\n3. Add new tag to `publish_tags` variable in\r\n `sphinx\\conf.py`.\r\n4. Remove `build\\multiver` to ensure clean build.\r\n5. Run `python scripts\\build_multidocs.py`. Result will be in\r\n `build\\multiver`.\r\n6. Checkout `docs` branch.\r\n7. Remove old docs dirs (named by their releases), move new ones, including\r\n `build\\multiver\\index.html` (but excluding `.doctree`\r\n dirs in each version build, they are not needed for serving), to the root\r\n of the repo.\r\n8. Add new stuff to git (be careful not to include junk, there is no\r\n `.gitignore`) and commit.\r\n\r\n> [!NOTE]\r\n> Things to keep in mind: sphinx-multiversion arranges releases based on commit\r\n> date, not semver number. So be careful when rebasing/amending older releases.\r\n\r\n#### Tests\r\n\r\nIf you're planning to test scripts from the documentation (the ones in\r\n`sphinx\\code`), you will have to setup 2 env variables in your\r\nterminal:\r\n\r\n```bat\r\nset MOD_SRC=\"path\\to\\source_mod\"\r\nset MOD_DST=\"path\\to\\destination_mod\"\r\n```\r\n\r\nCurrently there are only tests for [ndf_parse.model][ref-model] and docs' code\r\nsnippets and examples. Docstrings code is tested with sphinx via\r\n`tests\\docs_tests.bat`, py script with the same name has examples\r\ntests and a deprecated version of doctests.\r\n\r\n[ref-model]: https://ulibos.github.io/ndf-parse/v0.2.0/ndf-parse/model.html\r\n\r\n#### Using in Pair With Custom tree-sitter-ndf\r\n\r\nThis package looks for an `ntf.dll` in the following places\r\n(descending priority):\r\n\r\n1. `NDF_LIB_PATH` env variable\r\n (`\"C:\\custom\\path\\to\\ndf.dll\"`),\r\n2. default tree-sitter's build path\r\n (`\"%LocalAppData%\\tree-sitter\\lib\\ndf.dll\"`),\r\n3. a copy bundled with the package (`\"ndf_parse\\bin\\ndf.dll\"`).\r\n\r\nThe repo itself does not hold a prebuilt copy of the library so you'll have to\r\neither yank one from a release wheel (it's just a renamed zip) or build one\r\n[from source][ndf].\r\n\r\n#### Pull Requests and Issues\r\n\r\nI have no idea on how frequently I'll be able to respond to those, so expect\r\ndelays. You might find it easier catching me on [WarYes discord][waryes] or\r\n[Eugen discord][eugen] in case you have some blocking issue or a PR.\r\n\r\n[waryes]: https://discord.gg/gqBgvgGj8H\r\n[eugen]: https://discord.gg/sheyBRnqKP\r\n\r\n### Credits\r\n\r\nCreated by Ulibos, 2023-2024.\r\n",
"bugtrack_url": null,
"license": "The MIT License (MIT) Copyright (c) 2023 Ulibos Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "A package for parsing and editing Eugen Systems ndf files.",
"version": "0.2.0",
"project_urls": {
"Documentation": "https://ulibos.github.io/ndf-parse",
"Homepage": "https://github.com/Ulibos/ndf-parse"
},
"split_keywords": [
"ndf",
" parser",
" parsing"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "056f8e60f41e3d665237a005ba9f2c28288cb9790c172cd8e2606c3c1e80a82b",
"md5": "0c7977059181e2feb35b8b56a831ab94",
"sha256": "4ae33a5cf472960107835f0ca00b9a502813d8c5b612f8302ec76b258b371c2d"
},
"downloads": -1,
"filename": "ndf_parse-0.2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "0c7977059181e2feb35b8b56a831ab94",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 103948,
"upload_time": "2024-08-04T16:32:44",
"upload_time_iso_8601": "2024-08-04T16:32:44.717708Z",
"url": "https://files.pythonhosted.org/packages/05/6f/8e60f41e3d665237a005ba9f2c28288cb9790c172cd8e2606c3c1e80a82b/ndf_parse-0.2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-04 16:32:44",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Ulibos",
"github_project": "ndf-parse",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ndf-parse"
}