# netlogopy
`netlogopy` is a Python library that bridges NetLogo and Python, enabling advanced agent-based simulations by combining NetLogo’s simulation environment with Python’s powerful libraries for computation, optimization, and AI. `netlogopy` allows direct manipulation of NetLogo agents from Python.
## License
This project is licensed under the terms of the [MIT License](./LICENSE).
## Developed by
- **Nourddine Bouaziz** - [@Bouaziz19](https://github.com/Bouaziz19/netlogopy)
### Requirements
* NL4Py has been tested Python 3.6.2
* netlogopy works with NetLogo 6.0, 6.1, and 6.2
* netlogopy requires JDK 1.8
<!-- * NL4Py requires [NL4Py](https://pypi.org/project/NL4Py) to be installed with your Python distrubtion -->
## Installation
### Step 1: Install NetLogo
Download and install NetLogo from the official site.
[](https://ccl.northwestern.edu/netlogo/download.shtml)
### Step 2: Install Conda
If you do not already have Conda installed, download and install [Anaconda](https://www.anaconda.com/products/distribution) or [Miniconda](https://docs.conda.io/en/latest/miniconda.html).
### Step 3: Create the Conda Environment
Use the provided environment.yml file to create a new Conda environment.
```bash
conda env create -f environment.yml
```
file is provided below. For convenience, you can also download it directly from our GitHub repository.
```bash
name: netlogopy-env
channels:
- defaults
- conda-forge
dependencies:
- python=3.9
- openjdk=11
- pip
- pip:
- netlogopy
```
Or install `netlogopy` and `openjdk` in conda env
```bash
conda install openjdk -y
pip install netlogopy
# pip install netlogopy --upgrade
```
<!-- ### Step 2: Or install `netlogopy`
```bash
conda install openjdk -y
pip install netlogopy
# pip install netlogopy --upgrade
``` -->
### Configure IDE (Optional)
To set up your IDE (e.g., VS Code) for Command Line Interface (CLI) compatibility, check:
- [Set Default Terminal](https://www.w3schools.io/editor/vscode-change-default-terminal/)
- [Change Default Terminal in VS Code](https://stackoverflow.com/questions/44435697/change-the-default-terminal-in-visual-studio-code)
## Colors Reference
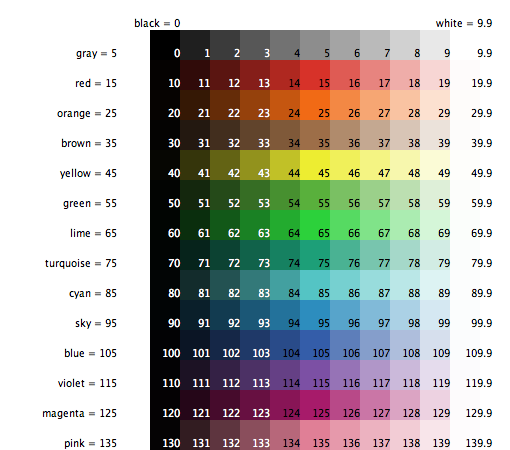
## Example Test
The following example demonstrates a basic setup of `netlogopy`, initializing a NetLogo world and creating an agent named `car01` with simple movement in the simulation environment.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home="C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
for i in range(10):
time.sleep(1)
print(f"*********** {i} ********")
n.close_model()
```
## Usage Examples
### Example 1: `pyturtle`
This example shows how to create an agent (a "turtle") in NetLogo using Python. Here, we create a `car`-shaped agent named `car01` and move it within the world.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
for i in range(10):
time.sleep(1)
print(f"*********** {i} ********")
n.close_model()
```
### Example 2: `set_background`
In this example, we set a custom background image in the NetLogo world. The background can be any image file accessible from your system.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
path_image = "path/to/image/nelogopy.png"
set_background(n, path_image)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
for i in range(10):
time.sleep(1)
print(f"*********** {i} ********")
n.close_model()
```
### Example 3: `street`
This example demonstrates creating a link between two agents in the NetLogo world. Here, a `street` link connects two car agents (`car01` and `car02`).
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55, name="car02", labelcolor=55)
street(n, fromm=car01, too=car02, label="street", labelcolor=35, color=35, shape="aa", thickness=0.5)
for i in range(10):
time.sleep(1)
print(f"*********** {i} ********")
n.close_model()
```
### Example 4: `fd`
In this example, the `fd` function is used to move the agent forward by one unit with each loop iteration.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
for i in range(10):
time.sleep(1)
print(f"*********** {i} ********")
car01.fd(1)
n.close_model()
```
### Example 5: `netlogoshow`
Here, `netlogoshow` displays text above the agent during simulation. Each iteration, we update the displayed text.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
resize_world(n, 0, 60, 0, 60)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
for i in range(10):
time.sleep(1)
word = f"{car01.id} {i}"
netlogoshow(n, word)
print(word)
car01.fd(1)
n.close_model()
```
### Example 6: `distanceto`
This example shows how to calculate and print the distance between two agents in the world using the `distanceto` function.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55)
for i in range(10):
time.sleep(1)
distance = car01.distanceto(car02)
print(f"Distance to car02: {distance}")
car01.fd(1)
n.close_model()
```
### Example 7: `face_to`
In this example, the `face_to` function directs `car01` to face towards `car02`.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55)
for i in range(10):
if i == 5:
car01.face_to(car02)
time.sleep(1)
car01.fd(1)
n.close_model()
```
### Example 8: `move_to`
The `move_to` function moves `car01` directly to `car02`'s position when the loop reaches a specified iteration.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55)
for i in range(10):
if i == 5:
car01.move_to(car02)
time.sleep(1)
car01.fd(1)
n.close_model()
```
### Example 9: `hideturtle`
This example demonstrates how to hide an agent in the simulation using `hideturtle`.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
for i in range(10):
if i == 5:
car01.hideturtle()
time.sleep(1)
car01.fd(1)
n.close_model()
```
### Example 10: `set_shape`
In this example, `set_shape` changes the shape of `car01` to `default` at a specific iteration.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
for i in range(100):
if i == 5:
car01.set_shape('default')
time.sleep(1)
car01.fd(1)
n.close_model()
```
### Example 11: `getxyturtle`
The `getxyturtle` function retrieves the current `x` and `y` coordinates of `car01`.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
for i in range(10):
time.sleep(1)
position = getxyturtle(n, car01)
print(f"Position: {position}")
car01.fd(1)
n.close_model()
```
### Example 12: `setxy`
The `setxy` function sets the `x` and `y` coordinates of `car01` to new values during the simulation.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
for i in range(100):
if i == 5:
car01.setxy(10, 10)
time.sleep(1)
car01.fd(1)
n.close_model()
```
### Example 13: `distancebetween`
This example calculates and prints the distance between `car01` and `car02`.
```python
import time
from netlogopy.nelogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
n = run_netlogo(netlogo_home)
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55)
for i in range(10):
time.sleep(1)
distance = distancebetween(n, car01, car02)
print(f"Distance between car01 and car02: {distance}")
car01.fd(1)
n.close_model()
```
## Usage Examples
### Integrating an Existing NetLogo Model with Python
We have added a feature that allows you to use older NetLogo simulators and modify them with Python, creating a dual-layered simulator. The first layer represents the original simulator, while the second layer is created in Python using `netlogopy`. This approach enables further development on older simulators without having to rebuild them entirely in `netlogopy`.
```python
import time, os, sys
# Get the parent directory path
parent_dir = os.path.abspath(os.path.join(os.path.dirname(__file__), "../../../"))
# Add the parent directory to the system path
sys.path.insert(0, parent_dir)
from netlogopy.netlogopy import *
if __name__ == "__main__":
netlogo_home = "C:/Program Files/NetLogo 6.2.2"
path_model = os.path.join(os.path.dirname(__file__), "Wolf Sheep Predation.nlogo")
n = run_netlogo(netlogo_home, path_model)
# Resize world
resize_world(n, 0, 70, 0, 55)
# Initialize the original NetLogo model
run_command(n, "setup")
# Add Python-controlled agents
car01 = pyturtle(n, x=20, y=20, shape="car", size_shape=4, color=15, name="car01", labelcolor=15)
car02 = pyturtle(n, x=5, y=5, shape="car", size_shape=4, color=55, name="car02", labelcolor=55)
street(n, fromm=car01, too=car02, label="street", labelcolor=35, color=35, shape="default", thickness=0.5)
for i in range(100):
run_command(n, "go") # Run the NetLogo model step
time.sleep(0.1)
print(f"*********** {i} ********")
car01.fd(1)
if i % 20 == 0:
car01.setxy(10, 10)
```
# Download Examples
You can download this examples files from git [Examples](https://github.com/Bouaziz19/netlogopy/tree/main/Examples).
---
**Happy simulating!** If you have any questions, issues, or ideas for new features, please open an [issue](https://github.com/Bouaziz19) or submit a [pull request](https://github.com/Bouaziz19).
## Final Steps
1. **Save** this text as your new `README.md` in the root of the repository.
2. Ensure the [LICENSE](./LICENSE) file is in the same directory.
3. Commit and push both files to GitHub.
You will then have an updated README with a clear reference to the MIT License, while providing a quick overview of the project’s purpose.
Raw data
{
"_id": null,
"home_page": null,
"name": "netlogopy",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "python, netlogo, simulation, multi agent",
"author": "BOUAZIZ (BOUAZIZ NOURDDINE)",
"author_email": "<nourddine.bouaziz.dz@gmail.com>",
"download_url": null,
"platform": null,
"description": "\r\n\r\n\r\n# netlogopy\r\n\r\n`netlogopy` is a Python library that bridges NetLogo and Python, enabling advanced agent-based simulations by combining NetLogo\u2019s simulation environment with Python\u2019s powerful libraries for computation, optimization, and AI. `netlogopy` allows direct manipulation of NetLogo agents from Python.\r\n\r\n## License\r\n\r\nThis project is licensed under the terms of the [MIT License](./LICENSE).\r\n\r\n## Developed by\r\n- **Nourddine Bouaziz** - [@Bouaziz19](https://github.com/Bouaziz19/netlogopy)\r\n\r\n### Requirements\r\n* NL4Py has been tested Python 3.6.2\r\n* netlogopy works with NetLogo 6.0, 6.1, and 6.2\r\n* netlogopy requires JDK 1.8 \r\n<!-- * NL4Py requires [NL4Py](https://pypi.org/project/NL4Py) to be installed with your Python distrubtion -->\r\n## Installation\r\n\r\n### Step 1: Install NetLogo\r\nDownload and install NetLogo from the official site.\r\n[](https://ccl.northwestern.edu/netlogo/download.shtml)\r\n\r\n\r\n### Step 2: Install Conda\r\nIf you do not already have Conda installed, download and install [Anaconda](https://www.anaconda.com/products/distribution) or [Miniconda](https://docs.conda.io/en/latest/miniconda.html).\r\n\r\n### Step 3: Create the Conda Environment\r\nUse the provided environment.yml file to create a new Conda environment.\r\n\r\n```bash\r\nconda env create -f environment.yml\r\n```\r\n file is provided below. For convenience, you can also download it directly from our GitHub repository.\r\n```bash\r\nname: netlogopy-env\r\nchannels:\r\n - defaults\r\n - conda-forge\r\ndependencies:\r\n - python=3.9\r\n - openjdk=11\r\n - pip\r\n - pip:\r\n - netlogopy\r\n\r\n```\r\nOr install `netlogopy` and `openjdk` in conda env \r\n```bash\r\nconda install openjdk -y\r\npip install netlogopy\r\n# pip install netlogopy --upgrade\r\n```\r\n<!-- ### Step 2: Or install `netlogopy`\r\n```bash\r\nconda install openjdk -y\r\npip install netlogopy\r\n# pip install netlogopy --upgrade\r\n``` -->\r\n\r\n### Configure IDE (Optional)\r\nTo set up your IDE (e.g., VS Code) for Command Line Interface (CLI) compatibility, check:\r\n- [Set Default Terminal](https://www.w3schools.io/editor/vscode-change-default-terminal/)\r\n- [Change Default Terminal in VS Code](https://stackoverflow.com/questions/44435697/change-the-default-terminal-in-visual-studio-code)\r\n\r\n## Colors Reference\r\n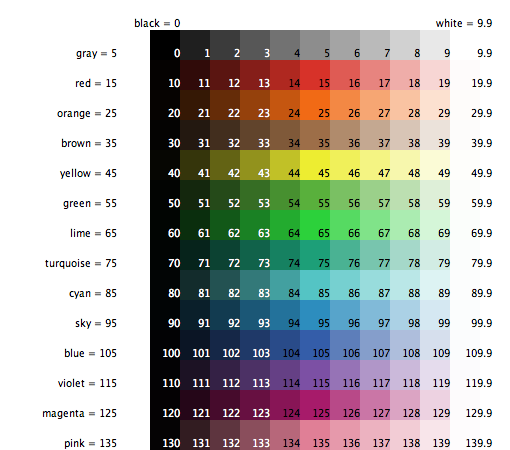\r\n\r\n## Example Test\r\nThe following example demonstrates a basic setup of `netlogopy`, initializing a NetLogo world and creating an agent named `car01` with simple movement in the simulation environment.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home=\"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n print(f\"*********** {i} ********\") \r\n n.close_model()\r\n\r\n```\r\n\r\n## Usage Examples\r\n\r\n### Example 1: `pyturtle`\r\nThis example shows how to create an agent (a \"turtle\") in NetLogo using Python. Here, we create a `car`-shaped agent named `car01` and move it within the world.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n print(f\"*********** {i} ********\") \r\n n.close_model()\r\n```\r\n### Example 2: `set_background`\r\nIn this example, we set a custom background image in the NetLogo world. The background can be any image file accessible from your system.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n path_image = \"path/to/image/nelogopy.png\"\r\n set_background(n, path_image)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n print(f\"*********** {i} ********\") \r\n n.close_model()\r\n```\r\n### Example 3: `street`\r\nThis example demonstrates creating a link between two agents in the NetLogo world. Here, a `street` link connects two car agents (`car01` and `car02`).\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55, name=\"car02\", labelcolor=55)\r\n street(n, fromm=car01, too=car02, label=\"street\", labelcolor=35, color=35, shape=\"aa\", thickness=0.5)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n print(f\"*********** {i} ********\") \r\n n.close_model()\r\n```\r\n### Example 4: `fd`\r\nIn this example, the `fd` function is used to move the agent forward by one unit with each loop iteration.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n print(f\"*********** {i} ********\") \r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 5: `netlogoshow`\r\nHere, `netlogoshow` displays text above the agent during simulation. Each iteration, we update the displayed text.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n resize_world(n, 0, 60, 0, 60)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n word = f\"{car01.id} {i}\"\r\n netlogoshow(n, word)\r\n print(word)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 6: `distanceto`\r\nThis example shows how to calculate and print the distance between two agents in the world using the `distanceto` function.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n distance = car01.distanceto(car02)\r\n print(f\"Distance to car02: {distance}\")\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 7: `face_to`\r\nIn this example, the `face_to` function directs `car01` to face towards `car02`.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55)\r\n \r\n for i in range(10):\r\n if i == 5:\r\n car01.face_to(car02)\r\n time.sleep(1)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 8: `move_to`\r\nThe `move_to` function moves `car01` directly to `car02`'s position when the loop reaches a specified iteration.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55)\r\n \r\n for i in range(10):\r\n if i == 5:\r\n car01.move_to(car02)\r\n time.sleep(1)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 9: `hideturtle`\r\nThis example demonstrates how to hide an agent in the simulation using `hideturtle`.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n \r\n for i in range(10):\r\n if i == 5:\r\n car01.hideturtle()\r\n time.sleep(1)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 10: `set_shape`\r\nIn this example, `set_shape` changes the shape of `car01` to `default` at a specific iteration.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n \r\n for i in range(100):\r\n if i == 5:\r\n car01.set_shape('default')\r\n time.sleep(1)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 11: `getxyturtle`\r\nThe `getxyturtle` function retrieves the current `x` and `y` coordinates of `car01`.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n position = getxyturtle(n, car01)\r\n print(f\"Position: {position}\")\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 12: `setxy`\r\nThe `setxy` function sets the `x` and `y` coordinates of `car01` to new values during the simulation.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n \r\n for i in range(100):\r\n if i == 5:\r\n car01.setxy(10, 10)\r\n time.sleep(1)\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n### Example 13: `distancebetween`\r\nThis example calculates and prints the distance between `car01` and `car02`.\r\n\r\n\r\n\r\n```python\r\nimport time\r\nfrom netlogopy.nelogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n n = run_netlogo(netlogo_home)\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55)\r\n \r\n for i in range(10):\r\n time.sleep(1)\r\n distance = distancebetween(n, car01, car02)\r\n print(f\"Distance between car01 and car02: {distance}\")\r\n car01.fd(1)\r\n n.close_model()\r\n```\r\n\r\n## Usage Examples\r\n\r\n### Integrating an Existing NetLogo Model with Python\r\nWe have added a feature that allows you to use older NetLogo simulators and modify them with Python, creating a dual-layered simulator. The first layer represents the original simulator, while the second layer is created in Python using `netlogopy`. This approach enables further development on older simulators without having to rebuild them entirely in `netlogopy`.\r\n\r\n\r\n\r\n```python\r\nimport time, os, sys\r\n\r\n# Get the parent directory path\r\nparent_dir = os.path.abspath(os.path.join(os.path.dirname(__file__), \"../../../\"))\r\n\r\n# Add the parent directory to the system path\r\nsys.path.insert(0, parent_dir)\r\nfrom netlogopy.netlogopy import *\r\n\r\nif __name__ == \"__main__\":\r\n netlogo_home = \"C:/Program Files/NetLogo 6.2.2\"\r\n path_model = os.path.join(os.path.dirname(__file__), \"Wolf Sheep Predation.nlogo\")\r\n n = run_netlogo(netlogo_home, path_model)\r\n\r\n # Resize world\r\n resize_world(n, 0, 70, 0, 55)\r\n\r\n # Initialize the original NetLogo model\r\n run_command(n, \"setup\")\r\n \r\n # Add Python-controlled agents\r\n car01 = pyturtle(n, x=20, y=20, shape=\"car\", size_shape=4, color=15, name=\"car01\", labelcolor=15)\r\n car02 = pyturtle(n, x=5, y=5, shape=\"car\", size_shape=4, color=55, name=\"car02\", labelcolor=55)\r\n street(n, fromm=car01, too=car02, label=\"street\", labelcolor=35, color=35, shape=\"default\", thickness=0.5)\r\n\r\n for i in range(100):\r\n run_command(n, \"go\") # Run the NetLogo model step\r\n time.sleep(0.1)\r\n print(f\"*********** {i} ********\")\r\n car01.fd(1)\r\n if i % 20 == 0:\r\n car01.setxy(10, 10)\r\n```\r\n\r\n# Download Examples\r\n\r\nYou can download this examples files from git [Examples](https://github.com/Bouaziz19/netlogopy/tree/main/Examples).\r\n\r\n\r\n---\r\n\r\n**Happy simulating!** If you have any questions, issues, or ideas for new features, please open an [issue](https://github.com/Bouaziz19) or submit a [pull request](https://github.com/Bouaziz19).\r\n\r\n## Final Steps\r\n\r\n1. **Save** this text as your new `README.md` in the root of the repository. \r\n2. Ensure the [LICENSE](./LICENSE) file is in the same directory. \r\n3. Commit and push both files to GitHub. \r\n\r\nYou will then have an updated README with a clear reference to the MIT License, while providing a quick overview of the project\u2019s purpose.\r\n",
"bugtrack_url": null,
"license": null,
"summary": "netlogopy : Usage netlogo by python",
"version": "0.0.19",
"project_urls": null,
"split_keywords": [
"python",
" netlogo",
" simulation",
" multi agent"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "01780bbbc3763db4bb8289956040f26684b2fca4e1c18800c9ae9235b5fcb3ed",
"md5": "27132b9bcd36913f3c311c933dc85ecd",
"sha256": "5813e360740a2261780f7e78b96dc033bb4f26f3aa987aa446321cd52986e585"
},
"downloads": -1,
"filename": "netlogopy-0.0.19-py3-none-any.whl",
"has_sig": false,
"md5_digest": "27132b9bcd36913f3c311c933dc85ecd",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 65526,
"upload_time": "2025-01-27T14:12:58",
"upload_time_iso_8601": "2025-01-27T14:12:58.752100Z",
"url": "https://files.pythonhosted.org/packages/01/78/0bbbc3763db4bb8289956040f26684b2fca4e1c18800c9ae9235b5fcb3ed/netlogopy-0.0.19-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-27 14:12:58",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "netlogopy"
}