Name | neutronics-material-maker JSON |
Version |
1.2.1
JSON |
| download |
home_page | None |
Summary | A tool for making reproducible materials and standardizing use across several neutronics codes |
upload_time | 2024-10-01 13:55:27 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | MIT License Copyright (c) 2020 UKAEA and neutronics-material-maker contributors Copyright (c) 2021 neutronics-material-maker contributors Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
material
library
database
serpent
mcnp
openmc
fispact
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
[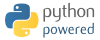](https://www.python.org)
[](https://github.com/fusion-energy/neutronics_material_maker/actions/workflows/ci_with_install.yml)
[](https://codecov.io/gh/fusion-energy/neutronics_material_maker)
[](https://badge.fury.io/py/neutronics-material-maker)
[](https://neutronics-material-maker.readthedocs.io/en/latest/?badge=latest)
[](https://frontend.code-inspector.com/public/project/13383/neutronics_material_maker/dashboard)
[](https://frontend.code-inspector.com/public/project/13383/neutronics_material_maker/dashboard)
# **Neutronics Material Maker**
The aim of this project is to facilitate the creation of materials for use in
neutronics codes such as OpenMC, Serpent, MCNP and Fispact.
The hope is that by having this collection of materials it is easier to reuse
materials across projects, use a common source with less room for user error.
One nice aspect of this material maker is the ability to change the density of
a material based on isotope enrichment (e.g. inbuild lithium ceramics),
temperature and pressure.
:point_right: [Documentation](https://neutronics-material-maker.readthedocs.io/en/latest/)
:point_right: [Video presentation]()
## Installation
### Conda
To use the code you will need to have OpenMC installed
[OpenMC](https://docs.openmc.org/en/latest/quickinstall.html).
The recommended method is to install from
[Conda Forge](https://conda-forge.org) which also installs all the dependencies
including OpenMC.
```bash
conda create --name new_env python=3.8
conda activate new_env
conda install neutronics_material_maker -c conda-forge
```
### Pip
Alternatively the code can be easily installed using pip. This method doesn't
currently include OpenMC so that will have to be installed separately using
Conda or compiled.
```bash
pip install neutronics_material_maker
```
To include the optional capability of calculating the density of coolants
additional packages are needed (CoolProp). A slightly modified pip install
is required in this case.
```bash
pip install "neutronics_material_maker[density]"
```
## Features
Materials for using in neutronics codes have two main aspects; a list of
isotopes along with their abundance and the material density.
This code contains an internal collection of materials with their isotope
abundances and densities (sometimes temperature, pressure and enrichment
dependant), but you may also import your own materials collection.
## Usage - Finding Available Materials
Each of the materials available is stored in an internal dictionary that can be
accessed using the ```AvailableMaterials()``` command.
```python
import neutronics_material_maker as nmm
all_materials = nmm.AvailableMaterials()
print(all_materials.keys())
```
### Usage - Material()
```python
import neutronics_material_maker as nmm
my_mat = nmm.Material(
name='my_custom_material',
isotopes={'Li6':0.5, 'Li7':0.5},
density=2.01,
percent_type='ao',
density_unit='g/cm3'
)
my_mat.openmc_material
```
## Usage - Material from library
Here is an example that accesses a material from the internal collection called
eurofer which has about 60 isotopes of a density of 7.78g/cm3.
```python
import neutronics_material_maker as nmm
my_mat = nmm.Material.from_library('eurofer')
my_mat.openmc_material
```
## Usage - Hot Pressurised Material
For several materials within the collection the temperature and the pressure impacts the density of the material. The neutronics_material_maker adjusts the density to take temperature and the pressure into account when appropriate. Densities are calculated either by a material specific formula (for example [FLiBe](https://github.com/fusion-energy/neutronics_material_maker/blob/main/neutronics_material_maker/data/multiplier_and_breeder_materials.json)) or using [CoolProps](https://pypi.org/project/CoolProp/) (for example [coolants](https://github.com/fusion-energy/neutronics_material_maker/blob/main/neutronics_material_maker/data/coolant_materials.json))
```python
import neutronics_material_maker as nmm
my_mat1 = nmm.Material.from_library(name='H2O', temperature=600, pressure=15e6)
my_mat1.openmc_material
```
For several materials within the collection the density is adjusted when the
material is enriched. For breeder blankets in fusion it is common to enrich the
lithium 6 content.
## Usage - Enriched Material
Lithium ceramics used in fusion breeder blankets often contain enriched
lithium-6 content. This slight change in density is accounted for by the
neutronics_material_maker.
```python
import neutronics_material_maker as nmm
my_mat2 = nmm.Material.from_library(name='Li4SiO4', enrichment=60)
my_mat2.openmc_material
```
## Usage - Material.from_mixture
Materials can also be mixed together using the from_mixture method. This
accepts a list of ```neutronics_material_maker.Materials``` or
```openmc.Material``` objects along with the material fractions (fracs).
```python
import neutronics_material_maker as nmm
my_mat1 = nmm.Material.from_library(name='Li4SiO4', packing_fraction=0.64)
my_mat2 = nmm.Material.from_library(name='Be12Ti')
my_mat3 = nmm.Material.from_mixture(materials=[my_mat1, my_mat2],
fracs=[0.4, 0.6],
percent_type='vo')
my_mat3.openmc_material
```
## Usage - Importing Your Own Material Library
Assuming you have a JSON file saved as ```mat_lib.json``` containing materials
defined in the same format as the
[exisiting materials](https://github.com/fusion-energy/neutronics_material_maker/tree/main/neutronics_material_maker/data)
then you can import this file into the package using
```AddMaterialFromFile()```. Another option is to use ```AddMaterialFromDir()```
to import a directory of json files.
```python
import neutronics_material_maker as nmm
nmm.AddMaterialFromFile('mat_lib.json')
nmm.AvailableMaterials() # this will print the new larger list of materials
```
## Usage - Exporting To Different Neutronics Codes
You can export to OpenMC, Serpent, MCNP and Fispact with the appropiate
arguments.
```python
import neutronics_material_maker as nmm
my_mat = nmm.Materialfrom_library('tungsten')
# openmc
my_mat.openmc_material
# mcnp - required a material_id to be set
my_mat.material_id = 1
my_mat.mcnp_material
# serpent
my_mat.serpent_material
# fispact - requires volume to be specified
my_mat.volume_in_cm3 = 1 / my_mat.density
my_mat.fispact_material
```
## Further Examples
Further examples can be found in the
[neutronics workshop task 11](https://github.com/fusion-energy/openmc_workshop/tree/master/tasks/task_11)
and in the [Documentation](https://neutronics-material-maker.readthedocs.io/en/latest/)
# Acknowledgement
Inspired by software projects [Pyne](https://pyne.io/) and making use of
[OpenMC](https://docs.openmc.org/en/stable/) functionality and the
[JSON](https://www.json.org/json-en.html) file format and
[PNNL](https://www.pnnl.gov/main/publications/external/technical_reports/PNNL-15870Rev1.pdf)
for many definitions.
Raw data
{
"_id": null,
"home_page": null,
"name": "neutronics-material-maker",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "material, library, database, serpent, mcnp, openmc, fispact",
"author": null,
"author_email": "Jonathan Shimwell <mail@jshimwell.com>",
"download_url": "https://files.pythonhosted.org/packages/80/48/6b455c30b000dda94be0432fb5144de9341fa6ab9a7d80bc11e6e633841a/neutronics_material_maker-1.2.1.tar.gz",
"platform": null,
"description": "\n[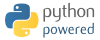](https://www.python.org)\n[](https://github.com/fusion-energy/neutronics_material_maker/actions/workflows/ci_with_install.yml)\n[](https://codecov.io/gh/fusion-energy/neutronics_material_maker)\n[](https://badge.fury.io/py/neutronics-material-maker)\n[](https://neutronics-material-maker.readthedocs.io/en/latest/?badge=latest)\n[](https://frontend.code-inspector.com/public/project/13383/neutronics_material_maker/dashboard)\n[](https://frontend.code-inspector.com/public/project/13383/neutronics_material_maker/dashboard)\n\n\n# **Neutronics Material Maker**\n\nThe aim of this project is to facilitate the creation of materials for use in\nneutronics codes such as OpenMC, Serpent, MCNP and Fispact.\n\nThe hope is that by having this collection of materials it is easier to reuse\nmaterials across projects, use a common source with less room for user error.\n\nOne nice aspect of this material maker is the ability to change the density of\na material based on isotope enrichment (e.g. inbuild lithium ceramics),\ntemperature and pressure.\n\n:point_right: [Documentation](https://neutronics-material-maker.readthedocs.io/en/latest/)\n\n:point_right: [Video presentation]()\n\n## Installation\n\n### Conda\n\nTo use the code you will need to have OpenMC installed\n[OpenMC](https://docs.openmc.org/en/latest/quickinstall.html).\n\nThe recommended method is to install from\n[Conda Forge](https://conda-forge.org) which also installs all the dependencies\nincluding OpenMC.\n\n```bash\nconda create --name new_env python=3.8\nconda activate new_env\nconda install neutronics_material_maker -c conda-forge\n```\n### Pip\nAlternatively the code can be easily installed using pip. This method doesn't\ncurrently include OpenMC so that will have to be installed separately using\nConda or compiled.\n\n```bash\npip install neutronics_material_maker\n```\n\nTo include the optional capability of calculating the density of coolants\nadditional packages are needed (CoolProp). A slightly modified pip install\nis required in this case.\n```bash\npip install \"neutronics_material_maker[density]\"\n```\n\n## Features\n\nMaterials for using in neutronics codes have two main aspects; a list of\nisotopes along with their abundance and the material density.\n\nThis code contains an internal collection of materials with their isotope\nabundances and densities (sometimes temperature, pressure and enrichment\ndependant), but you may also import your own materials collection.\n\n\n## Usage - Finding Available Materials\n\nEach of the materials available is stored in an internal dictionary that can be\naccessed using the ```AvailableMaterials()``` command.\n\n```python\nimport neutronics_material_maker as nmm\nall_materials = nmm.AvailableMaterials()\nprint(all_materials.keys())\n```\n\n### Usage - Material()\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat = nmm.Material(\n name='my_custom_material',\n isotopes={'Li6':0.5, 'Li7':0.5},\n density=2.01,\n percent_type='ao',\n density_unit='g/cm3'\n)\nmy_mat.openmc_material\n```\n\n## Usage - Material from library\n\nHere is an example that accesses a material from the internal collection called\neurofer which has about 60 isotopes of a density of 7.78g/cm3.\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat = nmm.Material.from_library('eurofer')\nmy_mat.openmc_material\n```\n\n\n## Usage - Hot Pressurised Material\n\nFor several materials within the collection the temperature and the pressure impacts the density of the material. The neutronics_material_maker adjusts the density to take temperature and the pressure into account when appropriate. Densities are calculated either by a material specific formula (for example [FLiBe](https://github.com/fusion-energy/neutronics_material_maker/blob/main/neutronics_material_maker/data/multiplier_and_breeder_materials.json)) or using [CoolProps](https://pypi.org/project/CoolProp/) (for example [coolants](https://github.com/fusion-energy/neutronics_material_maker/blob/main/neutronics_material_maker/data/coolant_materials.json))\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat1 = nmm.Material.from_library(name='H2O', temperature=600, pressure=15e6)\nmy_mat1.openmc_material\n```\n\nFor several materials within the collection the density is adjusted when the\nmaterial is enriched. For breeder blankets in fusion it is common to enrich the\nlithium 6 content.\n\n\n## Usage - Enriched Material\n\nLithium ceramics used in fusion breeder blankets often contain enriched\nlithium-6 content. This slight change in density is accounted for by the\nneutronics_material_maker.\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat2 = nmm.Material.from_library(name='Li4SiO4', enrichment=60)\nmy_mat2.openmc_material\n```\n\n\n## Usage - Material.from_mixture\n\nMaterials can also be mixed together using the from_mixture method. This\naccepts a list of ```neutronics_material_maker.Materials``` or \n```openmc.Material``` objects along with the material fractions (fracs).\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat1 = nmm.Material.from_library(name='Li4SiO4', packing_fraction=0.64)\nmy_mat2 = nmm.Material.from_library(name='Be12Ti')\nmy_mat3 = nmm.Material.from_mixture(materials=[my_mat1, my_mat2],\n fracs=[0.4, 0.6],\n percent_type='vo')\nmy_mat3.openmc_material\n```\n\n\n## Usage - Importing Your Own Material Library\n\nAssuming you have a JSON file saved as ```mat_lib.json``` containing materials\ndefined in the same format as the\n[exisiting materials](https://github.com/fusion-energy/neutronics_material_maker/tree/main/neutronics_material_maker/data)\nthen you can import this file into the package using\n```AddMaterialFromFile()```. Another option is to use ```AddMaterialFromDir()```\nto import a directory of json files.\n\n```python\nimport neutronics_material_maker as nmm\nnmm.AddMaterialFromFile('mat_lib.json')\nnmm.AvailableMaterials() # this will print the new larger list of materials\n```\n\n## Usage - Exporting To Different Neutronics Codes\n\nYou can export to OpenMC, Serpent, MCNP and Fispact with the appropiate\narguments.\n\n```python\nimport neutronics_material_maker as nmm\nmy_mat = nmm.Materialfrom_library('tungsten')\n\n# openmc\nmy_mat.openmc_material\n\n# mcnp - required a material_id to be set\nmy_mat.material_id = 1\nmy_mat.mcnp_material\n\n# serpent\nmy_mat.serpent_material\n\n# fispact - requires volume to be specified\nmy_mat.volume_in_cm3 = 1 / my_mat.density\nmy_mat.fispact_material\n```\n\n## Further Examples\n\nFurther examples can be found in the\n[neutronics workshop task 11](https://github.com/fusion-energy/openmc_workshop/tree/master/tasks/task_11)\nand in the [Documentation](https://neutronics-material-maker.readthedocs.io/en/latest/)\n\n\n# Acknowledgement\n\nInspired by software projects [Pyne](https://pyne.io/) and making use of\n[OpenMC](https://docs.openmc.org/en/stable/) functionality and the\n[JSON](https://www.json.org/json-en.html) file format and\n[PNNL](https://www.pnnl.gov/main/publications/external/technical_reports/PNNL-15870Rev1.pdf)\nfor many definitions.\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2020 UKAEA and neutronics-material-maker contributors Copyright (c) 2021 neutronics-material-maker contributors Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "A tool for making reproducible materials and standardizing use across several neutronics codes",
"version": "1.2.1",
"project_urls": {
"Bug Tracker": "https://github.com/fusion-energy/neutronics_material_maker/issues",
"Homepage": "https://github.com/fusion-energy/neutronics_material_maker"
},
"split_keywords": [
"material",
" library",
" database",
" serpent",
" mcnp",
" openmc",
" fispact"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "45db87f33f4588414886be66f10199aeda6c84bd904aabc7314700fe7676fafb",
"md5": "b9edf8fcb051c265a519ef551645d837",
"sha256": "b4b426f12b2fe9ceac7582da4549f1f9b7afd88b8a29e1dea2a7af624adff9e6"
},
"downloads": -1,
"filename": "neutronics_material_maker-1.2.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b9edf8fcb051c265a519ef551645d837",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 44632,
"upload_time": "2024-10-01T13:55:26",
"upload_time_iso_8601": "2024-10-01T13:55:26.074379Z",
"url": "https://files.pythonhosted.org/packages/45/db/87f33f4588414886be66f10199aeda6c84bd904aabc7314700fe7676fafb/neutronics_material_maker-1.2.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "80486b455c30b000dda94be0432fb5144de9341fa6ab9a7d80bc11e6e633841a",
"md5": "020e564bca33edfa48b7a0c624c3dea8",
"sha256": "fb498efc83b6ed0687aba42c93f7c87bdcc3351fc968a92d4ae6eb7c728a46e0"
},
"downloads": -1,
"filename": "neutronics_material_maker-1.2.1.tar.gz",
"has_sig": false,
"md5_digest": "020e564bca33edfa48b7a0c624c3dea8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 61000,
"upload_time": "2024-10-01T13:55:27",
"upload_time_iso_8601": "2024-10-01T13:55:27.699631Z",
"url": "https://files.pythonhosted.org/packages/80/48/6b455c30b000dda94be0432fb5144de9341fa6ab9a7d80bc11e6e633841a/neutronics_material_maker-1.2.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-01 13:55:27",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "fusion-energy",
"github_project": "neutronics_material_maker",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "neutronics-material-maker"
}