# noaa_coops
[](https://pypi.python.org/pypi/noaa-coops)
[](https://pypi.python.org/pypi/noaa-coops)
A Python wrapper for the NOAA CO-OPS Tides & Currents [Data](https://tidesandcurrents.noaa.gov/api/)
and [Metadata](https://tidesandcurrents.noaa.gov/mdapi/latest/) APIs.
## Installation
This package is distributed via [PyPi](https://pypi.org/project/noaa-coops/) and can be installed using , `pip`, `poetry`, etc.
```bash
# Install with pip
❯ pip install noaa_coops
# Install with poetry
❯ poetry add noaa_coops
```
## Getting Started
### Stations
Data is accessed via `Station` class objects. Each station is uniquely identified by an `id`. To initialize a `Station` object, run:
```python
>>> from noaa_coops import Station
>>> seattle = Station(id="9447130") # Create Station object for Seattle (ID = 9447130)
```
Stations and their IDs can be found using the Tides & Currents [mapping interface](https://tidesandcurrents.noaa.gov/). Alternatively, you can search for stations in a bounding box using the `get_stations_from_bbox` function, which will return a list of stations found in the box (if any).
```python
>>> from pprint import pprint
>>> from noaa_coops import Station, get_stations_from_bbox
>>> stations = get_stations_from_bbox(lat_coords=[40.389, 40.9397], lon_coords=[-74.4751, -73.7432])
>>> pprint(stations)
['8516945', '8518750', '8519483', '8531680']
>>> station_one = Station(id="8516945")
>>> pprint(station_one.name)
'Kings Point'
```
### Metadata
Station metadata is stored in the `.metadata` attribute of a `Station` object. Additionally, the keys of the metadata attribute dictionary are also assigned as attributes of the station object itself.
```python
>>> from pprint import pprint
>>> from noaa_coops import Station
>>> seattle = Station(id="9447130")
>>> pprint(list(seattle.metadata.items())[:5]) # Print first 3 items in metadata
[('tidal', True), ('greatlakes', False), ('shefcode', 'EBSW1')] # Metadata dictionary can be very long
>>> pprint(seattle.lat_lon['lat']) # Print latitude
47.601944
>>> pprint(seattle.lat_lon['lon']) # Print longitude
-122.339167
```
### Data Inventory
A description of a Station's data products and available dates can be accessed via the `.data_inventory` attribute of a `Station` object.
```python
>>> from noaa_coops import Station
>>> from pprint import pprint
>>> seattle = Station(id="9447130")
>>> pprint(seattle.data_inventory)
{'Air Temperature': {'end_date': '2019-01-02 18:36',
'start_date': '1991-11-09 01:00'},
'Barometric Pressure': {'end_date': '2019-01-02 18:36',
'start_date': '1991-11-09 00:00'},
'Preliminary 6-Minute Water Level': {'end_date': '2023-02-05 19:54',
'start_date': '2001-01-01 00:00'},
'Verified 6-Minute Water Level': {'end_date': '2022-12-31 23:54',
'start_date': '1995-06-01 00:00'},
'Verified High/Low Water Level': {'end_date': '2022-12-31 23:54',
'start_date': '1977-10-18 02:18'},
'Verified Hourly Height Water Level': {'end_date': '2022-12-31 23:00',
'start_date': '1899-01-01 00:00'},
'Verified Monthly Mean Water Level': {'end_date': '2022-12-31 23:54',
'start_date': '1898-12-01 00:00'},
'Water Temperature': {'end_date': '2019-01-02 18:36',
'start_date': '1991-11-09 00:00'},
'Wind': {'end_date': '2019-01-02 18:36', 'start_date': '1991-11-09 00:00'}}
```
### Data Retrieval
Available data products can be found in NOAA CO-OPS Data API docs.
Station data can be fetched using the `.get_data` method on a `Station` object. Data is returned as a Pandas [DataFrame](https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.html) for ease of use and analysis. DataFrame columns are named according to the NOAA CO-OPS API [docs](https://api.tidesandcurrents.noaa.gov/api/prod/responseHelp.html), with the `t` column (timestamp) set as the DataFrame index.
The example below fetches water level data from the Seattle station (id=9447130) for a 1 month period. The corresponding [web output](https://tidesandcurrents.noaa.gov/waterlevels.html?id=9447130&units=metric&bdate=20150101&edate=20150131&timezone=GMT&datum=MLLW) is shown below the code as a reference.
```python
>>> from noaa_coops import Station
>>> seattle = Station(id="9447130")
>>> df_water_levels = seattle.get_data(
... begin_date="20150101",
... end_date="20150131",
... product="water_level",
... datum="MLLW",
... units="metric",
... time_zone="gmt")
>>> df_water_levels.head()
v s f q
t
2015-01-01 00:00:00 1.799 0.023 0,0,0,0 v
2015-01-01 00:06:00 1.718 0.018 0,0,0,0 v
2015-01-01 00:12:00 1.639 0.013 0,0,0,0 v
2015-01-01 00:18:00 1.557 0.012 0,0,0,0 v
2015-01-01 00:24:00 1.473 0.014 0,0,0,0 v
```
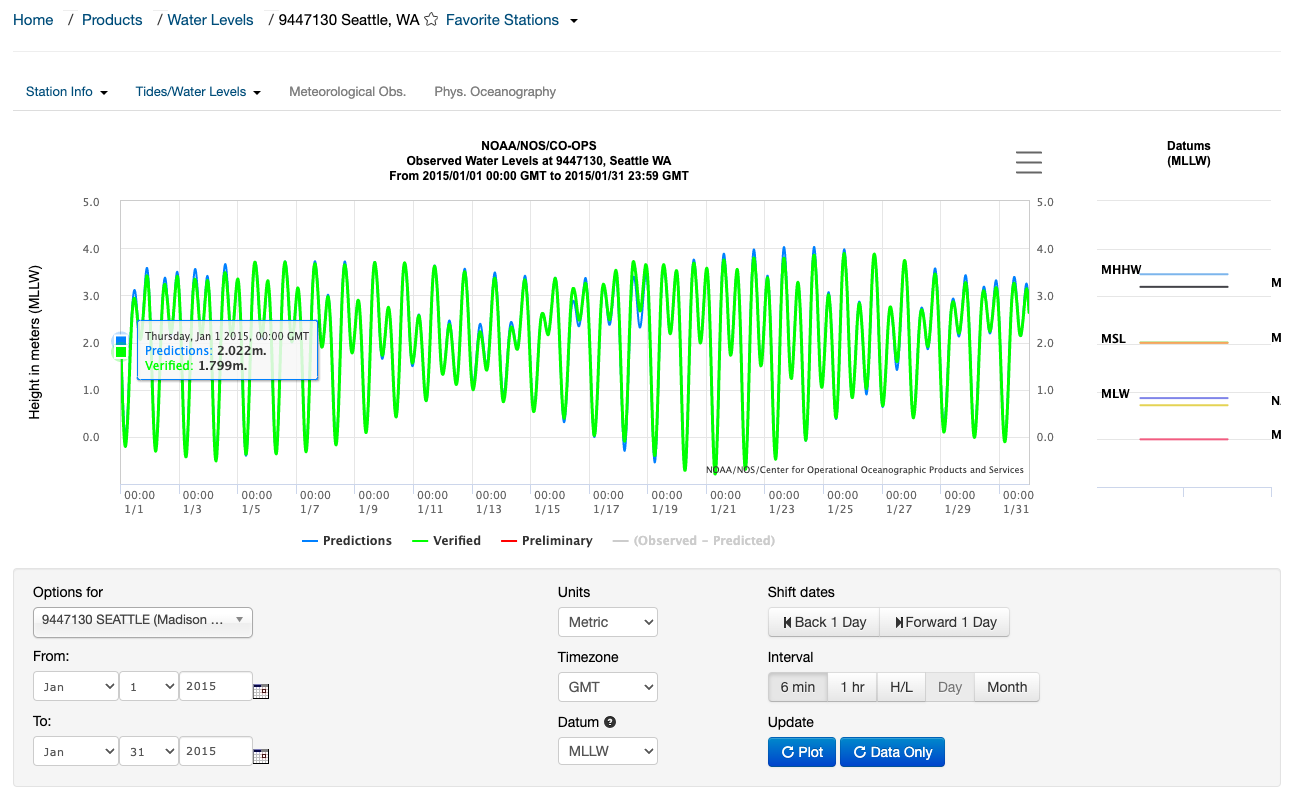
## Development
### Requirements
This package and its dependencies are managed using [poetry](https://python-poetry.org/). To install the development environment for `noaa_coops`, first install poetry, then run (inside the repo):
```bash
poetry install
```
### TODO
Click [here](https://github.com/GClunies/noaa_coops/issues) for a list of existing issues and to submit a new one.
### Contribution
Contributions are welcome, feel free to submit a pull request.
Raw data
{
"_id": null,
"home_page": "https://github.com/GClunies/noaa_coops",
"name": "noaa-coops",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8,<4.0",
"maintainer_email": "",
"keywords": "noaa,coops,tides,currents,weather,api",
"author": "Greg Clunies",
"author_email": "greg.clunies@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/6b/2e/aa7c7e59b31f8c5da533e9c7bf276c0601c491b50475f53fec7105c080fc/noaa_coops-0.3.2.tar.gz",
"platform": null,
"description": "# noaa_coops\n\n[](https://pypi.python.org/pypi/noaa-coops)\n[](https://pypi.python.org/pypi/noaa-coops)\n\nA Python wrapper for the NOAA CO-OPS Tides & Currents [Data](https://tidesandcurrents.noaa.gov/api/)\nand [Metadata](https://tidesandcurrents.noaa.gov/mdapi/latest/) APIs.\n\n## Installation\nThis package is distributed via [PyPi](https://pypi.org/project/noaa-coops/) and can be installed using , `pip`, `poetry`, etc.\n```bash\n# Install with pip\n\u276f pip install noaa_coops\n\n# Install with poetry\n\u276f poetry add noaa_coops\n```\n\n## Getting Started\n\n### Stations\nData is accessed via `Station` class objects. Each station is uniquely identified by an `id`. To initialize a `Station` object, run:\n\n```python\n>>> from noaa_coops import Station\n>>> seattle = Station(id=\"9447130\") # Create Station object for Seattle (ID = 9447130)\n```\n\nStations and their IDs can be found using the Tides & Currents [mapping interface](https://tidesandcurrents.noaa.gov/). Alternatively, you can search for stations in a bounding box using the `get_stations_from_bbox` function, which will return a list of stations found in the box (if any).\n```python\n>>> from pprint import pprint\n>>> from noaa_coops import Station, get_stations_from_bbox\n>>> stations = get_stations_from_bbox(lat_coords=[40.389, 40.9397], lon_coords=[-74.4751, -73.7432])\n>>> pprint(stations)\n['8516945', '8518750', '8519483', '8531680']\n>>> station_one = Station(id=\"8516945\")\n>>> pprint(station_one.name)\n'Kings Point'\n```\n\n### Metadata\nStation metadata is stored in the `.metadata` attribute of a `Station` object. Additionally, the keys of the metadata attribute dictionary are also assigned as attributes of the station object itself.\n\n```python\n>>> from pprint import pprint\n>>> from noaa_coops import Station\n>>> seattle = Station(id=\"9447130\")\n>>> pprint(list(seattle.metadata.items())[:5]) # Print first 3 items in metadata\n[('tidal', True), ('greatlakes', False), ('shefcode', 'EBSW1')] # Metadata dictionary can be very long\n>>> pprint(seattle.lat_lon['lat']) # Print latitude\n47.601944\n>>> pprint(seattle.lat_lon['lon']) # Print longitude\n-122.339167\n```\n\n### Data Inventory\nA description of a Station's data products and available dates can be accessed via the `.data_inventory` attribute of a `Station` object.\n\n```python\n>>> from noaa_coops import Station\n>>> from pprint import pprint\n>>> seattle = Station(id=\"9447130\")\n>>> pprint(seattle.data_inventory)\n{'Air Temperature': {'end_date': '2019-01-02 18:36',\n 'start_date': '1991-11-09 01:00'},\n 'Barometric Pressure': {'end_date': '2019-01-02 18:36',\n 'start_date': '1991-11-09 00:00'},\n 'Preliminary 6-Minute Water Level': {'end_date': '2023-02-05 19:54',\n 'start_date': '2001-01-01 00:00'},\n 'Verified 6-Minute Water Level': {'end_date': '2022-12-31 23:54',\n 'start_date': '1995-06-01 00:00'},\n 'Verified High/Low Water Level': {'end_date': '2022-12-31 23:54',\n 'start_date': '1977-10-18 02:18'},\n 'Verified Hourly Height Water Level': {'end_date': '2022-12-31 23:00',\n 'start_date': '1899-01-01 00:00'},\n 'Verified Monthly Mean Water Level': {'end_date': '2022-12-31 23:54',\n 'start_date': '1898-12-01 00:00'},\n 'Water Temperature': {'end_date': '2019-01-02 18:36',\n 'start_date': '1991-11-09 00:00'},\n 'Wind': {'end_date': '2019-01-02 18:36', 'start_date': '1991-11-09 00:00'}}\n```\n\n### Data Retrieval\nAvailable data products can be found in NOAA CO-OPS Data API docs.\n\nStation data can be fetched using the `.get_data` method on a `Station` object. Data is returned as a Pandas [DataFrame](https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.html) for ease of use and analysis. DataFrame columns are named according to the NOAA CO-OPS API [docs](https://api.tidesandcurrents.noaa.gov/api/prod/responseHelp.html), with the `t` column (timestamp) set as the DataFrame index.\n\nThe example below fetches water level data from the Seattle station (id=9447130) for a 1 month period. The corresponding [web output](https://tidesandcurrents.noaa.gov/waterlevels.html?id=9447130&units=metric&bdate=20150101&edate=20150131&timezone=GMT&datum=MLLW) is shown below the code as a reference.\n\n```python\n>>> from noaa_coops import Station\n>>> seattle = Station(id=\"9447130\")\n>>> df_water_levels = seattle.get_data(\n... begin_date=\"20150101\",\n... end_date=\"20150131\",\n... product=\"water_level\",\n... datum=\"MLLW\",\n... units=\"metric\",\n... time_zone=\"gmt\")\n>>> df_water_levels.head()\n v s f q\nt\n2015-01-01 00:00:00 1.799 0.023 0,0,0,0 v\n2015-01-01 00:06:00 1.718 0.018 0,0,0,0 v\n2015-01-01 00:12:00 1.639 0.013 0,0,0,0 v\n2015-01-01 00:18:00 1.557 0.012 0,0,0,0 v\n2015-01-01 00:24:00 1.473 0.014 0,0,0,0 v\n\n```\n\n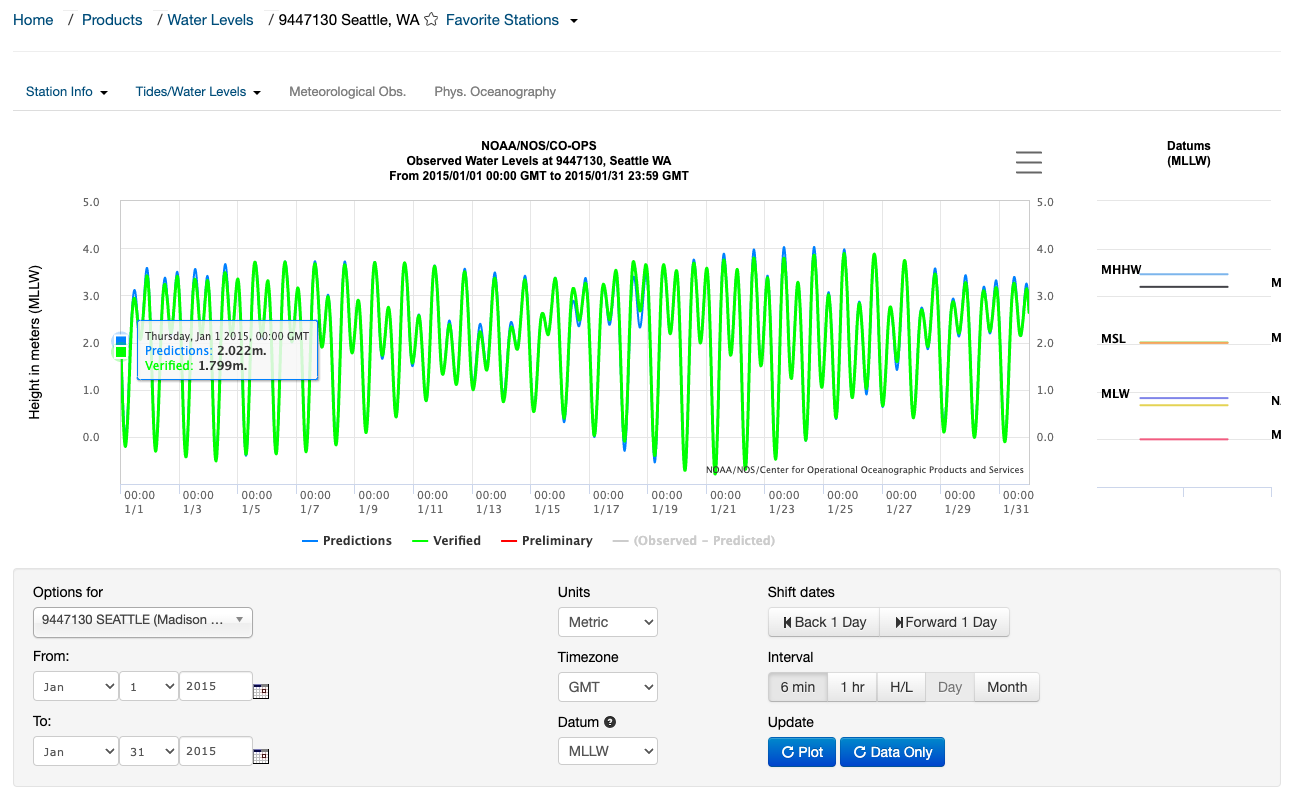\n\n\n## Development\n\n### Requirements\nThis package and its dependencies are managed using [poetry](https://python-poetry.org/). To install the development environment for `noaa_coops`, first install poetry, then run (inside the repo):\n\n```bash\npoetry install\n```\n\n### TODO\nClick [here](https://github.com/GClunies/noaa_coops/issues) for a list of existing issues and to submit a new one.\n\n### Contribution\nContributions are welcome, feel free to submit a pull request.\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Python wrapper for NOAA Tides & Currents Data and Metadata.",
"version": "0.3.2",
"project_urls": {
"Homepage": "https://github.com/GClunies/noaa_coops",
"Repository": "https://github.com/GClunies/noaa_coops"
},
"split_keywords": [
"noaa",
"coops",
"tides",
"currents",
"weather",
"api"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5e6c07e3221aa1ad763602b09eb1e85850a458cac5478161927e35c175017a54",
"md5": "6876b37569e1fbec074aee52093dc515",
"sha256": "c66a5faf36dda49c107b191a6fcf8d444d271a7d29caa6ddcef4d0f587a91bea"
},
"downloads": -1,
"filename": "noaa_coops-0.3.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6876b37569e1fbec074aee52093dc515",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8,<4.0",
"size": 13618,
"upload_time": "2023-05-15T05:34:50",
"upload_time_iso_8601": "2023-05-15T05:34:50.301164Z",
"url": "https://files.pythonhosted.org/packages/5e/6c/07e3221aa1ad763602b09eb1e85850a458cac5478161927e35c175017a54/noaa_coops-0.3.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6b2eaa7c7e59b31f8c5da533e9c7bf276c0601c491b50475f53fec7105c080fc",
"md5": "f637d502562a49d4d4445043ad48570e",
"sha256": "094770431e161e45ffcd9bf99a6aa1874cfa5191a3afbad76bd8b51ce6bb7c7b"
},
"downloads": -1,
"filename": "noaa_coops-0.3.2.tar.gz",
"has_sig": false,
"md5_digest": "f637d502562a49d4d4445043ad48570e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8,<4.0",
"size": 15905,
"upload_time": "2023-05-15T05:34:52",
"upload_time_iso_8601": "2023-05-15T05:34:52.005798Z",
"url": "https://files.pythonhosted.org/packages/6b/2e/aa7c7e59b31f8c5da533e9c7bf276c0601c491b50475f53fec7105c080fc/noaa_coops-0.3.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-15 05:34:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "GClunies",
"github_project": "noaa_coops",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "noaa-coops"
}