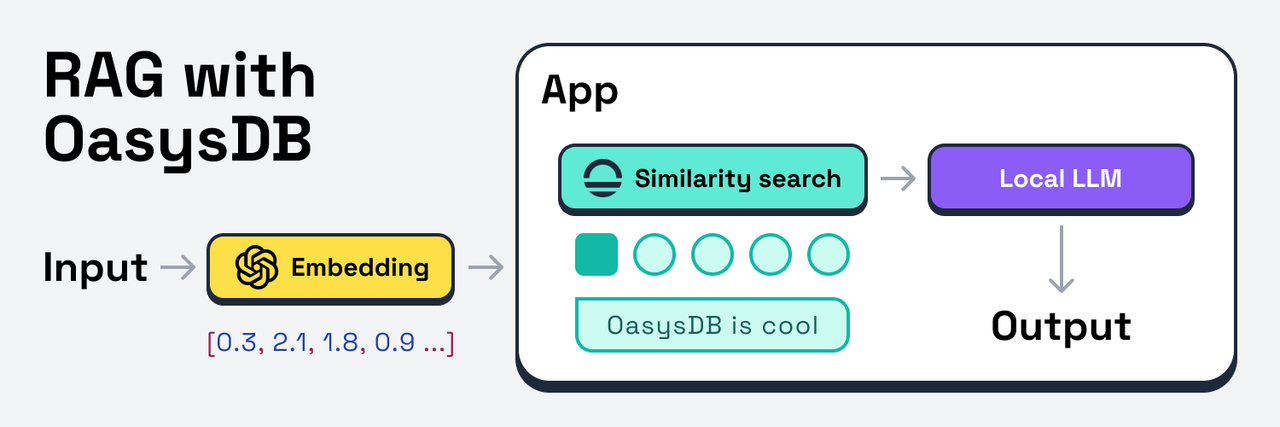
[](https://github.com/oasysai/oasysdb)
[](https://discord.gg/bDhQrkqNP4)
[](https://crates.io/crates/oasysdb)
[](https://pypi.org/project/oasysdb/)
[](https://opensource.org/licenses/Apache-2.0)
[](/docs/code_of_conduct.md)
# 👋 Meet OasysDB
OasysDB is a flexible and easy-to-use vector database written in Rust. It is designed with simplicity in mind to help you focus on building your AI application without worrying about database setup and configuration.
With 3 different runtime modes, OasysDB will accompany you throughout your journey from the early stages of development to scaling up your AI application for production workloads.
- **Embedded**: Run OasysDB directly inside your application.
- **Hosted**: Run OasysDB as a standalone server. _Coming soon_
- **Distributed**: Run sharded OasysDB instances. _Coming not so soon_ 😉
## Use Cases
OasysDB is very flexible! You can use it for almost any systems related with vector search such as:
- Local RAG (Retrieval-Augmented Generation) pipeline with an LLM and embedding model to generate a context-aware output.
- Image similarity search engine to find similar images based on their semantic content. [See Python demo](https://colab.research.google.com/drive/15_1hH7jGKzMeQ6IfnScjsc-iJRL5XyL7?usp=sharing).
- Real-time product recommendation system to suggest similar products based on the product features or user preferences.
- **Add your use case here** 😁
## Features
### Core Features
🔸 **Embedded Database**: Zero setup and no dedicated server or process required.
🔸 **Optional Persistence**: In-memory vector collections that can be persisted to disk.
🔸 **Incremental Ops**: Insert, modify, and delete vectors without rebuilding indexes.
🔸 **Flexible Schema**: Store additional and flexible metadata for each vector record.
### Technical Features
🔹 **Fast HNSW**: Efficient and accurate vector search with state-of-the-art algorithm.
🔹 **Configurable Metric**: Use Euclidean or Cosine distance depending on your use-case.
🔹 **Parallel Processing**: Multi-threaded & SIMD-optimized vector distance calculation.
🔹 **Built-in vector ID**: No headache record management with guaranteed ID uniqueness.
# 🚀 Quickstart with Rust
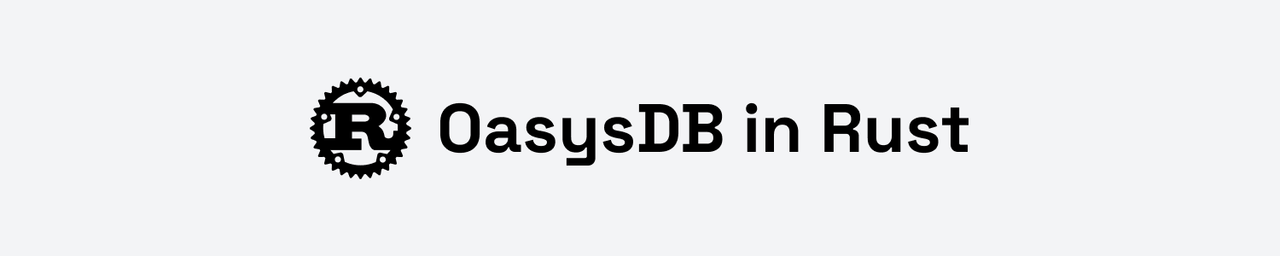
To get started with OasysDB in Rust, you need to add `oasysdb` to your `Cargo.toml`. You can do so by running the command below which will add the latest version of OasysDB to your project.
```bash
cargo add oasysdb
```
After that, you can use the code snippet below as a reference to get started with OasysDB. In short, use `Collection` to store your vector records or search similar vector and use `Database` to persist a vector collection to the disk.
```rust
use oasysdb::prelude::*;
fn main() {
// Vector dimension must be uniform.
let dimension = 128;
// Replace with your own data.
let records = Record::many_random(dimension, 100);
let mut config = Config::default();
// Optionally set the distance function. Default to Euclidean.
config.distance = Distance::Cosine;
// Create a vector collection.
let collection = Collection::build(&config, &records).unwrap();
// Optionally save the collection to persist it.
let mut db = Database::new("data/test").unwrap();
db.save_collection("vectors", &collection).unwrap();
// Search for the nearest neighbors.
let query = Vector::random(dimension);
let result = collection.search(&query, 5).unwrap();
for res in result {
let (id, distance) = (res.id, res.distance);
println!("{distance:.5} | ID: {id}");
}
}
```
## Feature Flags
OasysDB provides several feature flags to enable or disable certain features. You can do this by adding the feature flags to your project `Cargo.toml` file. Below are the available feature flags and their descriptions:
- `json`: Enables easy Serde's JSON conversion from and to the metadata type. This feature is very useful if you have a complex metadata type or if you use APIs that communicate using JSON.
- `gen`: Enables the vector generator trait and modules to extract vector embeddings from your contents using OpenAI or other embedding models. This feature allows OasysDB to handle vector embedding extraction for you without separate dependencies.
# 🚀 Quickstart with Python
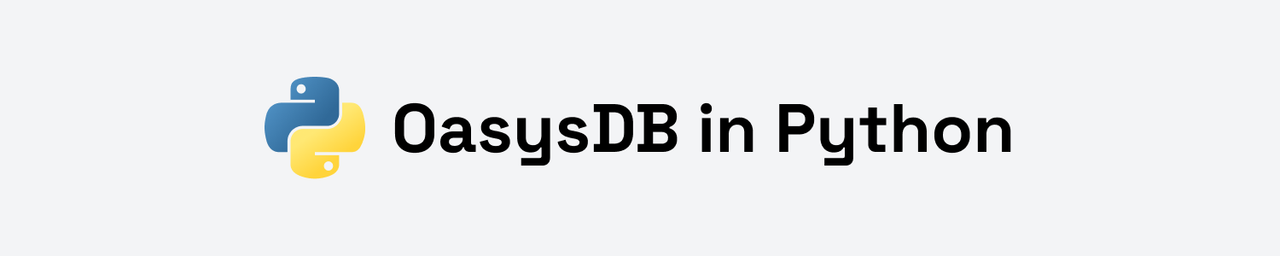
OasysDB also provides a Python binding which allows you to add it directly to your project. You can install the Python library of OasysDB by running the command below:
```bash
pip install oasysdb
```
This command will install the latest version of OasysDB to your Python environment. After you're all set with the installation, you can use the code snippet below as a reference to get started with OasysDB in Python.
```python
from oasysdb.prelude import *
if __name__ == "__main__":
# Open the database.
db = Database("data/example")
# Replace with your own records.
records = Record.many_random(dimension=128, len=100)
# Create a vector collection.
config = Config.create_default()
collection = Collection.from_records(config, records)
# Optionally, persist the collection to the database.
db.save_collection("my_collection", collection)
# Replace with your own query.
query = Vector.random(128)
# Search for the nearest neighbors.
result = collection.search(query, n=5)
# Print the result.
print("Nearest neighbors ID: {}".format(result[0].id))
```
# 🎯 Benchmarks
OasysDB uses a built-in benchmarking suite using Rust's [Criterion](https://docs.rs/criterion) crate which we use to measure the performance of the vector database.
Currently, the benchmarks are focused on the performance of the collection's vector search functionality. We are working on adding more benchmarks to measure the performance of other operations.
If you are curious and want to run the benchmarks, you can use the command below to run the benchmarks. If you do run it, please share the results with us 😉
```bash
cargo bench
```
## Memory Usage
OasysDB uses HNSW which is known to be a memory hog compared to other indexing algorithms. We decided to use it because of its performance even when storing large datasets of vectors with high dimension.
If you are curious about the memory usage of OasysDB, you can use the command below to run the memory usage measurement script. You can tweak the parameters in the `examples/measure-memory.rs` file to see how the memory usage changes.
```bash
cargo run --example measure-memory
```
## Recall Rate
In vector databases, recall is the percentage of relevant items that are successfully retrieved compared to the true set of relevant items also known as the ground truth.
To measure the recall rate, you can use the command below to run the recall rate measurement script. You can tweak the parameters in the `examples/measure-recall.rs` to see how OasysDB performs under different requirements.
```bash
cargo run --example measure-recall
```
Note: This script uses random vector records to measure the recall rate. This might not represent the real-world performance of OasysDB with proper datasets.
# 🤝 Contributing
The easiest way to contribute to this project is to star this project and share it with your friends. This will help us grow the community and make the project more visible to others.
If you want to go further and contribute your expertise, we will gladly welcome your code contributions. For more information and guidance about this, please see [contributing.md](/docs/contributing.md).
If you have deep experience in the space but don't have the free time to contribute codes, we also welcome advices, suggestions, or feature requests. We are also looking for advisors to help guide the project direction and roadmap.
If you are interested about the project in any way, please join us on [Discord](https://discord.gg/bDhQrkqNP4). Help us grow the community and make OasysDB better 😁
## Code of Conduct
We are committed to creating a welcoming community. Any participant in our project is expected to act respectfully and to follow the [Code of Conduct](/docs/code_of_conduct.md).
## Disclaimer
This project is still in the early stages of development. We are actively working on it and we expect the API and functionality to change. We do not recommend using this in production yet.
Raw data
{
"_id": null,
"home_page": "https://docs.oasysdb.com",
"name": "oasysdb",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "embedded, vector, database, hnsw, ann",
"author": "Edwin Kys, Oasys",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/c2/44/4e6782749cc6f48d6a4b56d81e43b553f32d7b24d6138cff1208c0250152/oasysdb-0.6.1.tar.gz",
"platform": null,
"description": "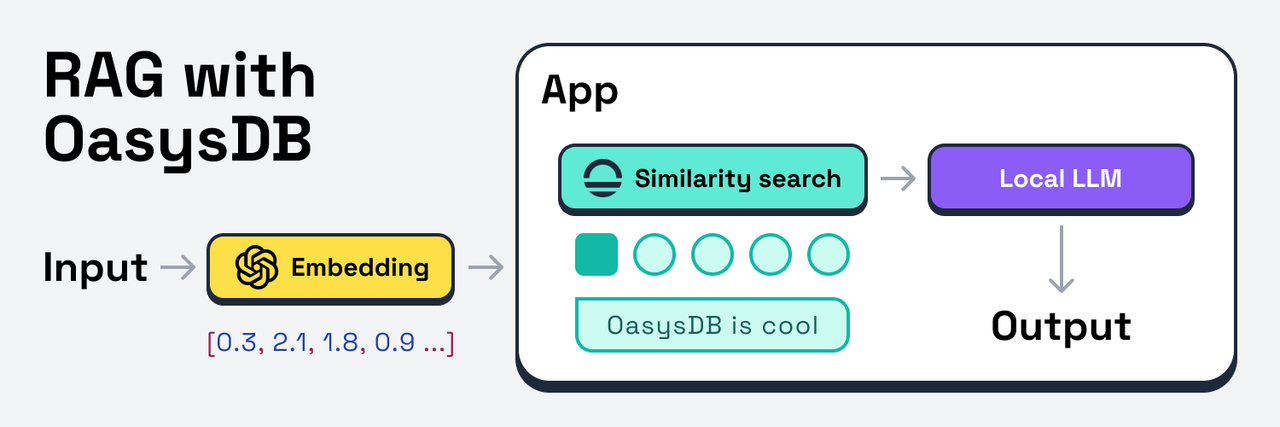\n\n[](https://github.com/oasysai/oasysdb)\n[](https://discord.gg/bDhQrkqNP4)\n\n[](https://crates.io/crates/oasysdb)\n[](https://pypi.org/project/oasysdb/)\n\n[](https://opensource.org/licenses/Apache-2.0)\n[](/docs/code_of_conduct.md)\n\n# \ud83d\udc4b Meet OasysDB\n\nOasysDB is a flexible and easy-to-use vector database written in Rust. It is designed with simplicity in mind to help you focus on building your AI application without worrying about database setup and configuration.\n\nWith 3 different runtime modes, OasysDB will accompany you throughout your journey from the early stages of development to scaling up your AI application for production workloads.\n\n- **Embedded**: Run OasysDB directly inside your application.\n- **Hosted**: Run OasysDB as a standalone server. _Coming soon_\n- **Distributed**: Run sharded OasysDB instances. _Coming not so soon_ \ud83d\ude09\n\n## Use Cases\n\nOasysDB is very flexible! You can use it for almost any systems related with vector search such as:\n\n- Local RAG (Retrieval-Augmented Generation) pipeline with an LLM and embedding model to generate a context-aware output.\n- Image similarity search engine to find similar images based on their semantic content. [See Python demo](https://colab.research.google.com/drive/15_1hH7jGKzMeQ6IfnScjsc-iJRL5XyL7?usp=sharing).\n- Real-time product recommendation system to suggest similar products based on the product features or user preferences.\n- **Add your use case here** \ud83d\ude01\n\n## Features\n\n### Core Features\n\n\ud83d\udd38 **Embedded Database**: Zero setup and no dedicated server or process required.\n\n\ud83d\udd38 **Optional Persistence**: In-memory vector collections that can be persisted to disk.\n\n\ud83d\udd38 **Incremental Ops**: Insert, modify, and delete vectors without rebuilding indexes.\n\n\ud83d\udd38 **Flexible Schema**: Store additional and flexible metadata for each vector record.\n\n### Technical Features\n\n\ud83d\udd39 **Fast HNSW**: Efficient and accurate vector search with state-of-the-art algorithm.\n\n\ud83d\udd39 **Configurable Metric**: Use Euclidean or Cosine distance depending on your use-case.\n\n\ud83d\udd39 **Parallel Processing**: Multi-threaded & SIMD-optimized vector distance calculation.\n\n\ud83d\udd39 **Built-in vector ID**: No headache record management with guaranteed ID uniqueness.\n\n# \ud83d\ude80 Quickstart with Rust\n\n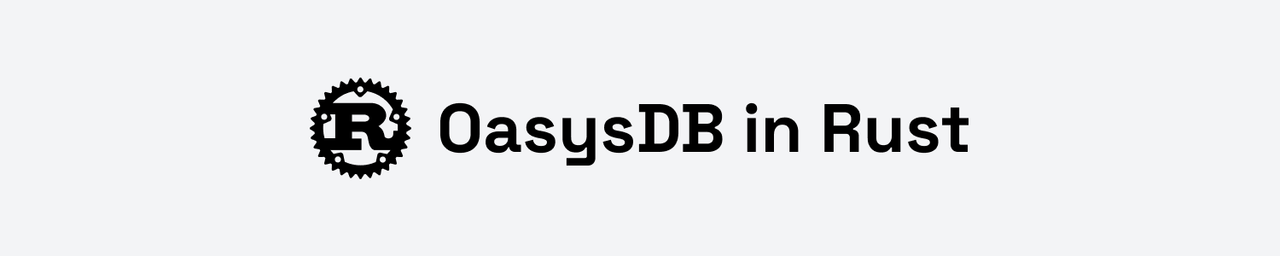\n\nTo get started with OasysDB in Rust, you need to add `oasysdb` to your `Cargo.toml`. You can do so by running the command below which will add the latest version of OasysDB to your project.\n\n```bash\ncargo add oasysdb\n```\n\nAfter that, you can use the code snippet below as a reference to get started with OasysDB. In short, use `Collection` to store your vector records or search similar vector and use `Database` to persist a vector collection to the disk.\n\n```rust\nuse oasysdb::prelude::*;\n\nfn main() {\n // Vector dimension must be uniform.\n let dimension = 128;\n\n // Replace with your own data.\n let records = Record::many_random(dimension, 100);\n\n let mut config = Config::default();\n\n // Optionally set the distance function. Default to Euclidean.\n config.distance = Distance::Cosine;\n\n // Create a vector collection.\n let collection = Collection::build(&config, &records).unwrap();\n\n // Optionally save the collection to persist it.\n let mut db = Database::new(\"data/test\").unwrap();\n db.save_collection(\"vectors\", &collection).unwrap();\n\n // Search for the nearest neighbors.\n let query = Vector::random(dimension);\n let result = collection.search(&query, 5).unwrap();\n\n for res in result {\n let (id, distance) = (res.id, res.distance);\n println!(\"{distance:.5} | ID: {id}\");\n }\n}\n```\n\n## Feature Flags\n\nOasysDB provides several feature flags to enable or disable certain features. You can do this by adding the feature flags to your project `Cargo.toml` file. Below are the available feature flags and their descriptions:\n\n- `json`: Enables easy Serde's JSON conversion from and to the metadata type. This feature is very useful if you have a complex metadata type or if you use APIs that communicate using JSON.\n\n- `gen`: Enables the vector generator trait and modules to extract vector embeddings from your contents using OpenAI or other embedding models. This feature allows OasysDB to handle vector embedding extraction for you without separate dependencies.\n\n# \ud83d\ude80 Quickstart with Python\n\n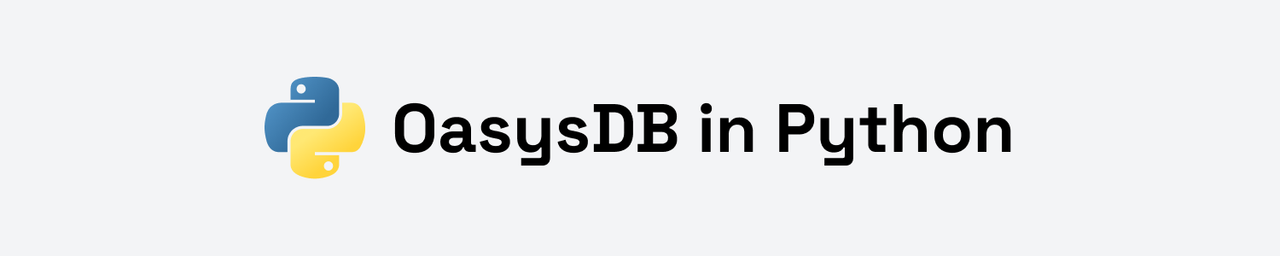\n\nOasysDB also provides a Python binding which allows you to add it directly to your project. You can install the Python library of OasysDB by running the command below:\n\n```bash\npip install oasysdb\n```\n\nThis command will install the latest version of OasysDB to your Python environment. After you're all set with the installation, you can use the code snippet below as a reference to get started with OasysDB in Python.\n\n```python\nfrom oasysdb.prelude import *\n\n\nif __name__ == \"__main__\":\n # Open the database.\n db = Database(\"data/example\")\n\n # Replace with your own records.\n records = Record.many_random(dimension=128, len=100)\n\n # Create a vector collection.\n config = Config.create_default()\n collection = Collection.from_records(config, records)\n\n # Optionally, persist the collection to the database.\n db.save_collection(\"my_collection\", collection)\n\n # Replace with your own query.\n query = Vector.random(128)\n\n # Search for the nearest neighbors.\n result = collection.search(query, n=5)\n\n # Print the result.\n print(\"Nearest neighbors ID: {}\".format(result[0].id))\n```\n\n# \ud83c\udfaf Benchmarks\n\nOasysDB uses a built-in benchmarking suite using Rust's [Criterion](https://docs.rs/criterion) crate which we use to measure the performance of the vector database.\n\nCurrently, the benchmarks are focused on the performance of the collection's vector search functionality. We are working on adding more benchmarks to measure the performance of other operations.\n\nIf you are curious and want to run the benchmarks, you can use the command below to run the benchmarks. If you do run it, please share the results with us \ud83d\ude09\n\n```bash\ncargo bench\n```\n\n## Memory Usage\n\nOasysDB uses HNSW which is known to be a memory hog compared to other indexing algorithms. We decided to use it because of its performance even when storing large datasets of vectors with high dimension.\n\nIf you are curious about the memory usage of OasysDB, you can use the command below to run the memory usage measurement script. You can tweak the parameters in the `examples/measure-memory.rs` file to see how the memory usage changes.\n\n```bash\ncargo run --example measure-memory\n```\n\n## Recall Rate\n\nIn vector databases, recall is the percentage of relevant items that are successfully retrieved compared to the true set of relevant items also known as the ground truth.\n\nTo measure the recall rate, you can use the command below to run the recall rate measurement script. You can tweak the parameters in the `examples/measure-recall.rs` to see how OasysDB performs under different requirements.\n\n```bash\ncargo run --example measure-recall\n```\n\nNote: This script uses random vector records to measure the recall rate. This might not represent the real-world performance of OasysDB with proper datasets.\n\n# \ud83e\udd1d Contributing\n\nThe easiest way to contribute to this project is to star this project and share it with your friends. This will help us grow the community and make the project more visible to others.\n\nIf you want to go further and contribute your expertise, we will gladly welcome your code contributions. For more information and guidance about this, please see [contributing.md](/docs/contributing.md).\n\nIf you have deep experience in the space but don't have the free time to contribute codes, we also welcome advices, suggestions, or feature requests. We are also looking for advisors to help guide the project direction and roadmap.\n\nIf you are interested about the project in any way, please join us on [Discord](https://discord.gg/bDhQrkqNP4). Help us grow the community and make OasysDB better \ud83d\ude01\n\n## Code of Conduct\n\nWe are committed to creating a welcoming community. Any participant in our project is expected to act respectfully and to follow the [Code of Conduct](/docs/code_of_conduct.md).\n\n## Disclaimer\n\nThis project is still in the early stages of development. We are actively working on it and we expect the API and functionality to change. We do not recommend using this in production yet.\n\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Fast & flexible embedded vector database with incremental HNSW indexing.",
"version": "0.6.1",
"project_urls": {
"Homepage": "https://docs.oasysdb.com",
"changelog": "https://github.com/oasysai/oasysdb/blob/main/docs/changelog.md",
"issues": "https://github.com/oasysai/oasysdb/issues",
"repository": "https://github.com/oasysai/oasysdb"
},
"split_keywords": [
"embedded",
" vector",
" database",
" hnsw",
" ann"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "38480ada4b5ba10e79267b7d8b81cea139b21275c2cb32523d2fe8302ebbe595",
"md5": "6041d5a0e6460475619e617ee0f25ac2",
"sha256": "aebc45214493193b604e9a89b4600fcd1e93c17d310c0fd7381974f41a8ee28d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-cp310-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "6041d5a0e6460475619e617ee0f25ac2",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 664385,
"upload_time": "2024-06-12T19:45:37",
"upload_time_iso_8601": "2024-06-12T19:45:37.754228Z",
"url": "https://files.pythonhosted.org/packages/38/48/0ada4b5ba10e79267b7d8b81cea139b21275c2cb32523d2fe8302ebbe595/oasysdb-0.6.1-cp310-cp310-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4aabbb4cb8b505928507eb157e5117054fe14c7594629629164d97e358b095a9",
"md5": "25693b97e818841feb4e0f50f466454a",
"sha256": "fab64cdf238a4e618c1fca9345a60917125093537d44aac3884ec86f2742a11a"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "25693b97e818841feb4e0f50f466454a",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 684092,
"upload_time": "2024-06-12T19:45:40",
"upload_time_iso_8601": "2024-06-12T19:45:40.314211Z",
"url": "https://files.pythonhosted.org/packages/4a/ab/bb4cb8b505928507eb157e5117054fe14c7594629629164d97e358b095a9/oasysdb-0.6.1-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d3d175526dfde616e19aa8864c8c49c996425f7684cb1ae40aeb15154b251cf2",
"md5": "0d58efc747f04d9f70f1b3ca4751e05d",
"sha256": "bd20d4deed0f7bc1cf46ab2c09755848e2f95985c30d2d8503dab67db3904aea"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-cp310-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "0d58efc747f04d9f70f1b3ca4751e05d",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1171439,
"upload_time": "2024-06-12T19:45:42",
"upload_time_iso_8601": "2024-06-12T19:45:42.310362Z",
"url": "https://files.pythonhosted.org/packages/d3/d1/75526dfde616e19aa8864c8c49c996425f7684cb1ae40aeb15154b251cf2/oasysdb-0.6.1-cp310-cp310-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "9f476db4bb09475e3ac60749791b9a847be6446244bc467bac105ee86d0a6884",
"md5": "01293226a91242540fa2e2e5ba575f7a",
"sha256": "0ae394cbf5a5f9a5da23001e576b2d8109715c76c969dc2aec2a58022590c078"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "01293226a91242540fa2e2e5ba575f7a",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1173158,
"upload_time": "2024-06-12T19:45:44",
"upload_time_iso_8601": "2024-06-12T19:45:44.279496Z",
"url": "https://files.pythonhosted.org/packages/9f/47/6db4bb09475e3ac60749791b9a847be6446244bc467bac105ee86d0a6884/oasysdb-0.6.1-cp310-cp310-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a8a362395789a6e6d0e6e60c42de4aaaf40b74591fa6addeb5c2b289f6b2d179",
"md5": "e389a6021cda51221e4adacd33af5466",
"sha256": "7e6b31eb09cfd4e3115a6d0008153f546894b10d8bdf760ca1acd4552be7d64a"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "e389a6021cda51221e4adacd33af5466",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 1122604,
"upload_time": "2024-06-12T19:45:46",
"upload_time_iso_8601": "2024-06-12T19:45:46.264983Z",
"url": "https://files.pythonhosted.org/packages/a8/a3/62395789a6e6d0e6e60c42de4aaaf40b74591fa6addeb5c2b289f6b2d179/oasysdb-0.6.1-cp310-cp310-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6f2d9d5d615932142793557aacb6e210f0f833f9fe2e950ebc5dd1abfc427af2",
"md5": "f409b7c195d5567a383c1aadb28ab5fc",
"sha256": "c13c41f117b21273fc1af4e0704550dd81c40b3cb7013e2392e3529acacb6932"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-none-win32.whl",
"has_sig": false,
"md5_digest": "f409b7c195d5567a383c1aadb28ab5fc",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 493905,
"upload_time": "2024-06-12T19:45:48",
"upload_time_iso_8601": "2024-06-12T19:45:48.395319Z",
"url": "https://files.pythonhosted.org/packages/6f/2d/9d5d615932142793557aacb6e210f0f833f9fe2e950ebc5dd1abfc427af2/oasysdb-0.6.1-cp310-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "b9575262df953a6e3a1e3d992308d389fad65878c1957c695c21a0691b1b3808",
"md5": "da170fe317d41cc6c3574b799ae95c27",
"sha256": "d466cc16910d8b1efb602d6ef09da709587802e30ac2463faa6570bf04a847a5"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp310-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "da170fe317d41cc6c3574b799ae95c27",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8",
"size": 518447,
"upload_time": "2024-06-12T19:45:50",
"upload_time_iso_8601": "2024-06-12T19:45:50.404233Z",
"url": "https://files.pythonhosted.org/packages/b9/57/5262df953a6e3a1e3d992308d389fad65878c1957c695c21a0691b1b3808/oasysdb-0.6.1-cp310-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5f68af2bbb2940a16bb3af5d626ac7185c5177f990c76d0fe43335730294be20",
"md5": "cf3203b2ec7a611f0fda4629dff1db2f",
"sha256": "103ddc9c06e8f313d9e7b75ff88e2835ab0fc1945f6c1641403be24c4998d3d6"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-cp311-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "cf3203b2ec7a611f0fda4629dff1db2f",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 664281,
"upload_time": "2024-06-12T19:45:52",
"upload_time_iso_8601": "2024-06-12T19:45:52.370809Z",
"url": "https://files.pythonhosted.org/packages/5f/68/af2bbb2940a16bb3af5d626ac7185c5177f990c76d0fe43335730294be20/oasysdb-0.6.1-cp311-cp311-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "99c65b647a2b5e3529ef8fd5dc983b6413fa7ca3af366f6ae833492e8a418e1b",
"md5": "cca7b20ace4486ed94cd38447ed342ff",
"sha256": "ac1f8c487665841389427e86cf809a441b441a8d2705501c51c3a06996d47e32"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "cca7b20ace4486ed94cd38447ed342ff",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 684065,
"upload_time": "2024-06-12T19:45:54",
"upload_time_iso_8601": "2024-06-12T19:45:54.366095Z",
"url": "https://files.pythonhosted.org/packages/99/c6/5b647a2b5e3529ef8fd5dc983b6413fa7ca3af366f6ae833492e8a418e1b/oasysdb-0.6.1-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "29edd1a2ec3e277519845e7fd863171fb93f4d6ee8286df1f56e709b3736b64c",
"md5": "878fe2a179295cc881b999496c6f8a3c",
"sha256": "4b0e1d2510fa010608ee4d3f2dc84697f711379efadd70ccdd364b330e663fcf"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-cp311-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "878fe2a179295cc881b999496c6f8a3c",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1171429,
"upload_time": "2024-06-12T19:45:56",
"upload_time_iso_8601": "2024-06-12T19:45:56.279246Z",
"url": "https://files.pythonhosted.org/packages/29/ed/d1a2ec3e277519845e7fd863171fb93f4d6ee8286df1f56e709b3736b64c/oasysdb-0.6.1-cp311-cp311-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3a98a37304bc9d3fd5437396fb45fe9689804d8efdfeae3e34136e8256477e17",
"md5": "39fbc21205b2e149f030114e94ce8958",
"sha256": "865b76b9bc4c1d46c32ab9cb258c2acea304357350881039544c7aa0519c8d79"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "39fbc21205b2e149f030114e94ce8958",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1173288,
"upload_time": "2024-06-12T19:45:58",
"upload_time_iso_8601": "2024-06-12T19:45:58.903372Z",
"url": "https://files.pythonhosted.org/packages/3a/98/a37304bc9d3fd5437396fb45fe9689804d8efdfeae3e34136e8256477e17/oasysdb-0.6.1-cp311-cp311-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "4b285a1f6085189a2a639d64faec3b654edaeae99fddf9a17132d89cbfaa1408",
"md5": "0f2ecdca9d614a0d47cb6248c44248c9",
"sha256": "2172d1a9902db298b57f1676a9b090120674af718d103a6ed503e79d195c24ef"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "0f2ecdca9d614a0d47cb6248c44248c9",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 1122557,
"upload_time": "2024-06-12T19:46:00",
"upload_time_iso_8601": "2024-06-12T19:46:00.945235Z",
"url": "https://files.pythonhosted.org/packages/4b/28/5a1f6085189a2a639d64faec3b654edaeae99fddf9a17132d89cbfaa1408/oasysdb-0.6.1-cp311-cp311-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7e9956a847ff863b823f0e0c9815fd1803987b111c2e5b5e2e79d6767b41e147",
"md5": "c246ce67817a9342b9b7f52b5a157a61",
"sha256": "8921cc2ee7095503ab94714eee121d75f874d67051591996b182d7dbbaa1aeaf"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-none-win32.whl",
"has_sig": false,
"md5_digest": "c246ce67817a9342b9b7f52b5a157a61",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 493892,
"upload_time": "2024-06-12T19:46:02",
"upload_time_iso_8601": "2024-06-12T19:46:02.844454Z",
"url": "https://files.pythonhosted.org/packages/7e/99/56a847ff863b823f0e0c9815fd1803987b111c2e5b5e2e79d6767b41e147/oasysdb-0.6.1-cp311-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5cf38c976795e434f742686a2ffa6976d1a453dc4f9272816c050e34129dcca9",
"md5": "0e1bccfbe3682799b7919cfe9f1698a3",
"sha256": "ad6d4f6b9582e384cd4dbbbf55716ae1d63b7c3807406709956bcfa1180a31a1"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp311-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "0e1bccfbe3682799b7919cfe9f1698a3",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8",
"size": 518333,
"upload_time": "2024-06-12T19:46:04",
"upload_time_iso_8601": "2024-06-12T19:46:04.504001Z",
"url": "https://files.pythonhosted.org/packages/5c/f3/8c976795e434f742686a2ffa6976d1a453dc4f9272816c050e34129dcca9/oasysdb-0.6.1-cp311-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3371ee12f9084505d7b8a22a3f2a603575ebaf85eb00b220a495b6b484c8ead8",
"md5": "a703a7f3151521a680960f8c01dcc322",
"sha256": "ee1453f6e9e1ecfc0f5c61044bfbbe673e8beceb02a2d84fa383217e52903f04"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-cp312-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "a703a7f3151521a680960f8c01dcc322",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 661660,
"upload_time": "2024-06-12T19:46:06",
"upload_time_iso_8601": "2024-06-12T19:46:06.285428Z",
"url": "https://files.pythonhosted.org/packages/33/71/ee12f9084505d7b8a22a3f2a603575ebaf85eb00b220a495b6b484c8ead8/oasysdb-0.6.1-cp312-cp312-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "153866a3346b27130760e8f9eb399c9aac128a511dbef244cd823447bad95f96",
"md5": "a3293085404937027684a6f9ad51dde4",
"sha256": "3c2f54f996f1b2d346c32696acc2dbb8d14af1a5f14eea046a4b90db6132ed75"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-cp312-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "a3293085404937027684a6f9ad51dde4",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 682122,
"upload_time": "2024-06-12T19:46:08",
"upload_time_iso_8601": "2024-06-12T19:46:08.198644Z",
"url": "https://files.pythonhosted.org/packages/15/38/66a3346b27130760e8f9eb399c9aac128a511dbef244cd823447bad95f96/oasysdb-0.6.1-cp312-cp312-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d64eedf43f9a2527c9bc9d6496f2edafe068994e382e4b94ae229aafc76576a5",
"md5": "45b9c8b1507faa00116fcbcf5559f4b0",
"sha256": "9831328360d70f412f13d22d29cfbcb95976f7deaf9860c84058510f4b84fd7d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-cp312-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "45b9c8b1507faa00116fcbcf5559f4b0",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1168599,
"upload_time": "2024-06-12T19:46:10",
"upload_time_iso_8601": "2024-06-12T19:46:10.230445Z",
"url": "https://files.pythonhosted.org/packages/d6/4e/edf43f9a2527c9bc9d6496f2edafe068994e382e4b94ae229aafc76576a5/oasysdb-0.6.1-cp312-cp312-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0863f4c6ed2a89437420a1122c011dd43781c7a2655c0519ea1b6b078317d87e",
"md5": "956d751b1b06efffb9b85644031e5dec",
"sha256": "81aae6738e3ff0e6d3df31c65b543a3ebf645aff83ec9533ce3d0595df278fab"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "956d751b1b06efffb9b85644031e5dec",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1171994,
"upload_time": "2024-06-12T19:46:12",
"upload_time_iso_8601": "2024-06-12T19:46:12.381954Z",
"url": "https://files.pythonhosted.org/packages/08/63/f4c6ed2a89437420a1122c011dd43781c7a2655c0519ea1b6b078317d87e/oasysdb-0.6.1-cp312-cp312-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fdb4fa681f6288c94e46ff2252624e9d6db2d9a75e4eb29e1f30de0c50703c95",
"md5": "f5c7c478b388c4e7935868b3e6c366c4",
"sha256": "8acf0eaea796ec8e2a554db340cd67a51f0b52671fc8d5faf9f8174c4bfe6e4d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "f5c7c478b388c4e7935868b3e6c366c4",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 1120369,
"upload_time": "2024-06-12T19:46:14",
"upload_time_iso_8601": "2024-06-12T19:46:14.488815Z",
"url": "https://files.pythonhosted.org/packages/fd/b4/fa681f6288c94e46ff2252624e9d6db2d9a75e4eb29e1f30de0c50703c95/oasysdb-0.6.1-cp312-cp312-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "58a17977e8bfaf078e0d80273865578ed61af65700b2e74f4fad11539da1b756",
"md5": "a3e1f3d5b958a7a21e49ce7557ed0241",
"sha256": "07a554dbd3174d44cc9b35425e6459dfc2c5e1e54e5a4a0057748e90e477c7f8"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-none-win32.whl",
"has_sig": false,
"md5_digest": "a3e1f3d5b958a7a21e49ce7557ed0241",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 491805,
"upload_time": "2024-06-12T19:46:16",
"upload_time_iso_8601": "2024-06-12T19:46:16.111065Z",
"url": "https://files.pythonhosted.org/packages/58/a1/7977e8bfaf078e0d80273865578ed61af65700b2e74f4fad11539da1b756/oasysdb-0.6.1-cp312-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "8e4a1b1a7f68478663c4df2d8ecbf2a6c4894250bffa6c861aa27127de5cd1ef",
"md5": "6e5ef8583937c64e65bb855513912288",
"sha256": "f98aef7cfa6f991b434b57cb49ae65d3a2e4ae1f2ffc3e79e9adb8a16241a329"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp312-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "6e5ef8583937c64e65bb855513912288",
"packagetype": "bdist_wheel",
"python_version": "cp312",
"requires_python": ">=3.8",
"size": 516204,
"upload_time": "2024-06-12T19:46:17",
"upload_time_iso_8601": "2024-06-12T19:46:17.788592Z",
"url": "https://files.pythonhosted.org/packages/8e/4a/1b1a7f68478663c4df2d8ecbf2a6c4894250bffa6c861aa27127de5cd1ef/oasysdb-0.6.1-cp312-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "57a688dd07a9d7740b7ad4162781c2bda56dfa3849bce1467fc4cbbf61ca0fc5",
"md5": "f7705cb8a3cc3d853187f0d2ee54717f",
"sha256": "30249af5365b879f29663b724b61c0688a6098336b38831e24e465995c5f7e76"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp38-cp38-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "f7705cb8a3cc3d853187f0d2ee54717f",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1171755,
"upload_time": "2024-06-12T19:46:19",
"upload_time_iso_8601": "2024-06-12T19:46:19.599755Z",
"url": "https://files.pythonhosted.org/packages/57/a6/88dd07a9d7740b7ad4162781c2bda56dfa3849bce1467fc4cbbf61ca0fc5/oasysdb-0.6.1-cp38-cp38-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "800750690e56e1460ad625bdad169ca4af12b07f01013e5f5139240b0f0f879c",
"md5": "41bf5edd8389a507548852409adeafdc",
"sha256": "b04a9087bc04e88e7ababb83db181530b99d5c86e6c30e224da334adeee0fa40"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "41bf5edd8389a507548852409adeafdc",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1173786,
"upload_time": "2024-06-12T19:46:21",
"upload_time_iso_8601": "2024-06-12T19:46:21.853903Z",
"url": "https://files.pythonhosted.org/packages/80/07/50690e56e1460ad625bdad169ca4af12b07f01013e5f5139240b0f0f879c/oasysdb-0.6.1-cp38-cp38-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c7545bbf6c8954840be582313ae70162b6d9763f538e7a0568d385b34af2b34b",
"md5": "a630cb10a22de2c72ff4f82e377ef3e5",
"sha256": "9ee49d346fdf48759aa8d995047d9e1226fced4e46d520ed6de54446b1c93e64"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "a630cb10a22de2c72ff4f82e377ef3e5",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 1123509,
"upload_time": "2024-06-12T19:46:24",
"upload_time_iso_8601": "2024-06-12T19:46:24.311064Z",
"url": "https://files.pythonhosted.org/packages/c7/54/5bbf6c8954840be582313ae70162b6d9763f538e7a0568d385b34af2b34b/oasysdb-0.6.1-cp38-cp38-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f14fd70025a53e86b6649c38a121a2c9b90f35ddc62ca99a77df1a8104beca7b",
"md5": "b6f121371be437300ff6ea1c419a190a",
"sha256": "66e59287ad9815c0a397e8430c16a711f83f0ede3b3717498fa2a1494e63598f"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp38-none-win32.whl",
"has_sig": false,
"md5_digest": "b6f121371be437300ff6ea1c419a190a",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 494155,
"upload_time": "2024-06-12T19:46:26",
"upload_time_iso_8601": "2024-06-12T19:46:26.073376Z",
"url": "https://files.pythonhosted.org/packages/f1/4f/d70025a53e86b6649c38a121a2c9b90f35ddc62ca99a77df1a8104beca7b/oasysdb-0.6.1-cp38-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f3b2474515cd3459a89cba46aa524e6e106f3e108f86d456252b9fd202454f2e",
"md5": "b7e2dff37ed1a6c0b09004f824373630",
"sha256": "0ff3507fd7abcad1168769488f4815ef99558bf4bd8cb2c1923570d32d5c5190"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp38-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "b7e2dff37ed1a6c0b09004f824373630",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8",
"size": 518654,
"upload_time": "2024-06-12T19:46:27",
"upload_time_iso_8601": "2024-06-12T19:46:27.670882Z",
"url": "https://files.pythonhosted.org/packages/f3/b2/474515cd3459a89cba46aa524e6e106f3e108f86d456252b9fd202454f2e/oasysdb-0.6.1-cp38-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "a7c814a2dea36a8e72b33d8f92c5df0633dcbbeb8b18f1a132b0ebf756ab4c8c",
"md5": "3e08ab05325cdff7f8ac37e92a45da68",
"sha256": "61f83a8446d88c9e2e00bc63326b83715ccd017ddd6722ebeee6b61340ceeb6d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-cp39-macosx_10_12_x86_64.whl",
"has_sig": false,
"md5_digest": "3e08ab05325cdff7f8ac37e92a45da68",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 664832,
"upload_time": "2024-06-12T19:46:29",
"upload_time_iso_8601": "2024-06-12T19:46:29.353905Z",
"url": "https://files.pythonhosted.org/packages/a7/c8/14a2dea36a8e72b33d8f92c5df0633dcbbeb8b18f1a132b0ebf756ab4c8c/oasysdb-0.6.1-cp39-cp39-macosx_10_12_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "3570f8fbd631037b7bf13cdf393770dd11e5eff6fa0174895ace8776a923a6ac",
"md5": "7976d407627430dff98383d2b6798f5b",
"sha256": "172efe1f6421452d2698e5dddf70e52514c447bf30aa464f86ac8c8096a21f99"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "7976d407627430dff98383d2b6798f5b",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 684450,
"upload_time": "2024-06-12T19:46:31",
"upload_time_iso_8601": "2024-06-12T19:46:31.106206Z",
"url": "https://files.pythonhosted.org/packages/35/70/f8fbd631037b7bf13cdf393770dd11e5eff6fa0174895ace8776a923a6ac/oasysdb-0.6.1-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c82720619089e8e06b667500ae5a561b8d7568c90c99c1df2b29cbfc218f95e9",
"md5": "25bed3f711d3ad6a58cb85cd8fc7d352",
"sha256": "51e9d52a92305fad435dd31f9b79e512b2a9d1ccbeafed956f324b879aee27f7"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-cp39-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "25bed3f711d3ad6a58cb85cd8fc7d352",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1171745,
"upload_time": "2024-06-12T19:46:33",
"upload_time_iso_8601": "2024-06-12T19:46:33.088997Z",
"url": "https://files.pythonhosted.org/packages/c8/27/20619089e8e06b667500ae5a561b8d7568c90c99c1df2b29cbfc218f95e9/oasysdb-0.6.1-cp39-cp39-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5d93d7cce1f7207ee228ee1e701b01cd7e939139a1c2e4d3333b0f920cee1877",
"md5": "a2958df5f1b03039279a4fee7445a37a",
"sha256": "ec640e9ca5a35d2bdabd9c2e66ca66f98ceeba4f3adba0ab9ef5d331fd60a380"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "a2958df5f1b03039279a4fee7445a37a",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1173565,
"upload_time": "2024-06-12T19:46:35",
"upload_time_iso_8601": "2024-06-12T19:46:35.090781Z",
"url": "https://files.pythonhosted.org/packages/5d/93/d7cce1f7207ee228ee1e701b01cd7e939139a1c2e4d3333b0f920cee1877/oasysdb-0.6.1-cp39-cp39-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "7296d05951c23e710d19ab369fa3b114a8b112702aaa025d0c242abc399caa39",
"md5": "cdc8df16983a8b788b1ae3cf7033a647",
"sha256": "650a5807b7ca2210349d0adf71d74f9cfc77a0330c036808db5226b77a825eba"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "cdc8df16983a8b788b1ae3cf7033a647",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 1123339,
"upload_time": "2024-06-12T19:46:36",
"upload_time_iso_8601": "2024-06-12T19:46:36.795061Z",
"url": "https://files.pythonhosted.org/packages/72/96/d05951c23e710d19ab369fa3b114a8b112702aaa025d0c242abc399caa39/oasysdb-0.6.1-cp39-cp39-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "1899a4943a5426b2edd6ad8105f7d3ef7eb87017a8ed7c8cce0ea323c8574103",
"md5": "0bcb455dfa8032843d1bf86f5b31489e",
"sha256": "3468bbb613c511cb4bfb293fbeedfb087d18c5383649be58b3d4a600ae090038"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-none-win32.whl",
"has_sig": false,
"md5_digest": "0bcb455dfa8032843d1bf86f5b31489e",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 494069,
"upload_time": "2024-06-12T19:46:38",
"upload_time_iso_8601": "2024-06-12T19:46:38.707147Z",
"url": "https://files.pythonhosted.org/packages/18/99/a4943a5426b2edd6ad8105f7d3ef7eb87017a8ed7c8cce0ea323c8574103/oasysdb-0.6.1-cp39-none-win32.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ad7ec1bed64ed45660b9a6ec5bc38884ad87c32911b88e1fd430eb2dc7260b57",
"md5": "5762190bc0fcf7a09c03a905cce84944",
"sha256": "73bddc32e72df5e1d05b2746be668f3360603ec6e5406bc30873d30231a542a4"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-cp39-none-win_amd64.whl",
"has_sig": false,
"md5_digest": "5762190bc0fcf7a09c03a905cce84944",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8",
"size": 518659,
"upload_time": "2024-06-12T19:46:40",
"upload_time_iso_8601": "2024-06-12T19:46:40.834747Z",
"url": "https://files.pythonhosted.org/packages/ad/7e/c1bed64ed45660b9a6ec5bc38884ad87c32911b88e1fd430eb2dc7260b57/oasysdb-0.6.1-cp39-none-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "76d3c18f6095340417bf2b6501d490927b67b6d7ef5fb72ee97987e14684cb82",
"md5": "7050d7da95affa22f44771d4dbc2a91a",
"sha256": "cea3ab5e38a036356e6d2c98e8c9625e13fcac3fabdd45df48ef805d23540dab"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "7050d7da95affa22f44771d4dbc2a91a",
"packagetype": "bdist_wheel",
"python_version": "pp310",
"requires_python": ">=3.8",
"size": 1171690,
"upload_time": "2024-06-12T19:46:42",
"upload_time_iso_8601": "2024-06-12T19:46:42.673138Z",
"url": "https://files.pythonhosted.org/packages/76/d3/c18f6095340417bf2b6501d490927b67b6d7ef5fb72ee97987e14684cb82/oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "cb6bdde338c44e4cf1d6e6a91294067510ba8897913e5d85edd0460e3b71357f",
"md5": "a7b5444c45e840e7ada0be6a89790f9b",
"sha256": "cc1f08cb2ee046e8c98815efb6b5e20bd1812ae869cda3aabb4ba2eb1cc62ff3"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "a7b5444c45e840e7ada0be6a89790f9b",
"packagetype": "bdist_wheel",
"python_version": "pp310",
"requires_python": ">=3.8",
"size": 1172923,
"upload_time": "2024-06-12T19:46:44",
"upload_time_iso_8601": "2024-06-12T19:46:44.434323Z",
"url": "https://files.pythonhosted.org/packages/cb/6b/dde338c44e4cf1d6e6a91294067510ba8897913e5d85edd0460e3b71357f/oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "9929037b5a3aba6fe3aab15dd0c14a35f7faa60bba5ded5cee79e304cde56ca7",
"md5": "e645e5a97d84023eacbcd7a3be640c53",
"sha256": "5a1c174030fa5dff926dfc6e3dc7769d9997f30c86c3efe62c00895066b182ec"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "e645e5a97d84023eacbcd7a3be640c53",
"packagetype": "bdist_wheel",
"python_version": "pp310",
"requires_python": ">=3.8",
"size": 1122745,
"upload_time": "2024-06-12T19:46:46",
"upload_time_iso_8601": "2024-06-12T19:46:46.228823Z",
"url": "https://files.pythonhosted.org/packages/99/29/037b5a3aba6fe3aab15dd0c14a35f7faa60bba5ded5cee79e304cde56ca7/oasysdb-0.6.1-pp310-pypy310_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "918d5d7216a3dc1241e969ddf103ea543934ded684fa2564bdff31a8b82b2477",
"md5": "f510699b7c2391f16e3d582e88787c81",
"sha256": "597385b15a21bfd0660cc7bf3ef81719876ee1e5beb35b02d8b871fc79cf20b0"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "f510699b7c2391f16e3d582e88787c81",
"packagetype": "bdist_wheel",
"python_version": "pp38",
"requires_python": ">=3.8",
"size": 1172436,
"upload_time": "2024-06-12T19:46:47",
"upload_time_iso_8601": "2024-06-12T19:46:47.955589Z",
"url": "https://files.pythonhosted.org/packages/91/8d/5d7216a3dc1241e969ddf103ea543934ded684fa2564bdff31a8b82b2477/oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f0a5c08f64456d8bf10493e060b206ef19707b9de1d1c60397511d382b43fbae",
"md5": "dd5e7174d0d6d1d477b981b9ab66cd62",
"sha256": "9cb0d04756f17356711b4d48b98eaaba73bd37a0994b4d8741398a06379049b7"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "dd5e7174d0d6d1d477b981b9ab66cd62",
"packagetype": "bdist_wheel",
"python_version": "pp38",
"requires_python": ">=3.8",
"size": 1173909,
"upload_time": "2024-06-12T19:46:49",
"upload_time_iso_8601": "2024-06-12T19:46:49.953076Z",
"url": "https://files.pythonhosted.org/packages/f0/a5/c08f64456d8bf10493e060b206ef19707b9de1d1c60397511d382b43fbae/oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6dcf5516cfbab0abaafe7f0c11e274b51a0234a6f76be060dadf3d82c6f20c6b",
"md5": "6d75443547b00fd3882ae5bba7d67cc7",
"sha256": "a5231c9c320e0cd54f8d13c5b71249207107e7309ad34a3a977cc190377a861a"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "6d75443547b00fd3882ae5bba7d67cc7",
"packagetype": "bdist_wheel",
"python_version": "pp38",
"requires_python": ">=3.8",
"size": 1124004,
"upload_time": "2024-06-12T19:46:52",
"upload_time_iso_8601": "2024-06-12T19:46:52.399470Z",
"url": "https://files.pythonhosted.org/packages/6d/cf/5516cfbab0abaafe7f0c11e274b51a0234a6f76be060dadf3d82c6f20c6b/oasysdb-0.6.1-pp38-pypy38_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0a034bbd559f6a0419aa1d7b3f4002a8ca2e146dba5450d2778860031785db13",
"md5": "488d5a360127b4b598b8c4cd24761f15",
"sha256": "5fb9de5ba5d5f3c55f38d474ba760168f7c8f94bbef5ad679b25a359860d091d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"has_sig": false,
"md5_digest": "488d5a360127b4b598b8c4cd24761f15",
"packagetype": "bdist_wheel",
"python_version": "pp39",
"requires_python": ">=3.8",
"size": 1172270,
"upload_time": "2024-06-12T19:46:54",
"upload_time_iso_8601": "2024-06-12T19:46:54.256212Z",
"url": "https://files.pythonhosted.org/packages/0a/03/4bbd559f6a0419aa1d7b3f4002a8ca2e146dba5450d2778860031785db13/oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_12_i686.manylinux2010_i686.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c9a44b36c76988809174602aa7a86590941d00821cce0bd475d2e7ce50e93356",
"md5": "3901fd2b697a54d2b4056fb489718e6b",
"sha256": "ab1af3ac290e1edf984046685fe4d9a8f06f655aafc977587c933fffd5ae147d"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"has_sig": false,
"md5_digest": "3901fd2b697a54d2b4056fb489718e6b",
"packagetype": "bdist_wheel",
"python_version": "pp39",
"requires_python": ">=3.8",
"size": 1173366,
"upload_time": "2024-06-12T19:46:56",
"upload_time_iso_8601": "2024-06-12T19:46:56.912855Z",
"url": "https://files.pythonhosted.org/packages/c9/a4/4b36c76988809174602aa7a86590941d00821cce0bd475d2e7ce50e93356/oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_17_aarch64.manylinux2014_aarch64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "702f2389a321d61c1caa7836765a739387237d7bd278f674cbddfb1a6fc0e2e8",
"md5": "a9b54f582b351216315d6efca79eab51",
"sha256": "b38ca40c4e8f97c9598554ae817d53d7a49d7b21862d7df5ae82db79b9092d59"
},
"downloads": -1,
"filename": "oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "a9b54f582b351216315d6efca79eab51",
"packagetype": "bdist_wheel",
"python_version": "pp39",
"requires_python": ">=3.8",
"size": 1123595,
"upload_time": "2024-06-12T19:46:59",
"upload_time_iso_8601": "2024-06-12T19:46:59.224751Z",
"url": "https://files.pythonhosted.org/packages/70/2f/2389a321d61c1caa7836765a739387237d7bd278f674cbddfb1a6fc0e2e8/oasysdb-0.6.1-pp39-pypy39_pp73-manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "c2444e6782749cc6f48d6a4b56d81e43b553f32d7b24d6138cff1208c0250152",
"md5": "38d7e0eb979c1a0114e2b8b24e7c91ac",
"sha256": "0ed9f7a4711d74b2c94ad43802e7de21210c7eacf86408015365b739ea6ff761"
},
"downloads": -1,
"filename": "oasysdb-0.6.1.tar.gz",
"has_sig": false,
"md5_digest": "38d7e0eb979c1a0114e2b8b24e7c91ac",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 88301,
"upload_time": "2024-06-12T19:47:01",
"upload_time_iso_8601": "2024-06-12T19:47:01.013591Z",
"url": "https://files.pythonhosted.org/packages/c2/44/4e6782749cc6f48d6a4b56d81e43b553f32d7b24d6138cff1208c0250152/oasysdb-0.6.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-12 19:47:01",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "oasysai",
"github_project": "oasysdb",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "pytest",
"specs": [
[
"==",
"8.0.2"
]
]
},
{
"name": "black",
"specs": [
[
"==",
"24.3.0"
]
]
},
{
"name": "flake8",
"specs": [
[
"==",
"7.0.0"
]
]
},
{
"name": "asyncio",
"specs": [
[
"==",
"3.4.3"
]
]
},
{
"name": "mkdocs-material",
"specs": [
[
"==",
"9.5.26"
]
]
}
],
"lcname": "oasysdb"
}