# odsgenerator, a .ods generator.
Generate an OpenDocument Format `.ods` file from a `.json` or `.yaml` file.
When used as a script, `odsgenerator` parses a JSON or YAML description of
tables and generates an ODF document using the `odfdo` library.
When used as a library, `odsgenerator` parses a Python description of tables
and returns the ODF content as bytes (ready to be saved as a valid ODF document).
- The content description can be minimalist: a list of lists of lists,
- or description can be complex, allowing styles at row or cell level.
See also https://github.com/jdum/odsparsator which is doing the reverse
operation, `.osd` to `.json`.
`odsgenerator` is a `Python3` package, using the [odfdo](https://github.com/jdum/odfdo) library. Current version requires Python >= 3.9, see prior versions for older environments.
Project:
https://github.com/jdum/odsgenerator
Author:
jerome.dumonteil@gmail.com
License:
MIT
## Installation
Installation from Pypi (recommended):
```python
pip install odsgenerator
```
Installation from sources (requiring setuptools):
```python
pip install .
```
## CLI usage
```
odsgenerator [-h] [--version] input_file output_file
```
### arguments
`input_file`: input file containing data in JSON or YAML format
`output_file`: output file, `.ods` file generated from the input
Use ``odsgenerator --help`` for more details about input file parameters
and look at examples in the tests folder.
## Usage from python code
```python
from odsgenerator import odsgenerator
content = odsgenerator.ods_bytes([[["a", "b", "c"], [10, 20, 30]]])
with open("sample.ods", "wb") as file:
file.write(content)
```
The resulting `.ods` file loaded in a spreadsheet:
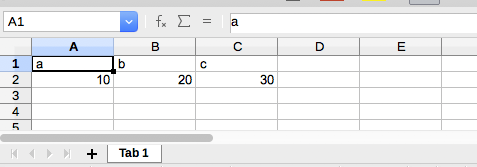
Another example with more parameters:
```python
import odsgenerator
content = odsgenerator.ods_bytes(
[
{
"name": "first tab",
"style": "cell_decimal2",
"table": [
{
"row": ["a", "b", "c"],
"style": "bold_center_bg_gray_grid_06pt",
},
[10, 20, 30],
],
}
]
)
with open("sample2.ods", "wb") as file:
file.write(content)
```
The `.ods` file loaded in a spreadsheet, with gray background on first line:
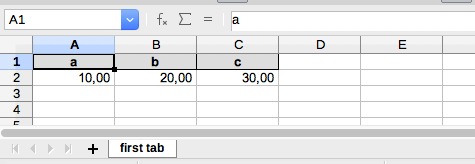
## Principle
- A document is a list or dict containing tabs,
- a tab is a list or dict containing rows,
- a row is a list or dict containing cells.
## Documentation
See in the `./doc folder:
- `html/odsgenerator.html`
- `tutorial.json` or `tutorial.yml` and `tutorial.ods`
## License
This project is licensed under the MIT License (see the
`LICENSE` file for details).
Raw data
{
"_id": null,
"home_page": "https://github.com/jdum/odsgenerator",
"name": "odsgenerator",
"maintainer": null,
"docs_url": null,
"requires_python": "<4,>=3.9",
"maintainer_email": null,
"keywords": "openDocument, ODF, ods, json, spreadsheet, generator",
"author": "J\u00e9r\u00f4me Dumonteil",
"author_email": "jerome.dumonteil@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/c1/41/a3c47fc5d41b551d1838df91403b4bffc5a9c4e5f17931445e5fc4771f99/odsgenerator-1.11.1.tar.gz",
"platform": null,
"description": "# odsgenerator, a .ods generator.\n\nGenerate an OpenDocument Format `.ods` file from a `.json` or `.yaml` file.\n\n\nWhen used as a script, `odsgenerator` parses a JSON or YAML description of\ntables and generates an ODF document using the `odfdo` library.\n\nWhen used as a library, `odsgenerator` parses a Python description of tables\nand returns the ODF content as bytes (ready to be saved as a valid ODF document).\n\n- The content description can be minimalist: a list of lists of lists,\n- or description can be complex, allowing styles at row or cell level.\n\nSee also https://github.com/jdum/odsparsator which is doing the reverse\noperation, `.osd` to `.json`.\n\n`odsgenerator` is a `Python3` package, using the [odfdo](https://github.com/jdum/odfdo) library. Current version requires Python >= 3.9, see prior versions for older environments.\n\nProject:\n https://github.com/jdum/odsgenerator\n\nAuthor:\n jerome.dumonteil@gmail.com\n\nLicense:\n MIT\n\n\n## Installation\n\nInstallation from Pypi (recommended):\n\n```python\npip install odsgenerator\n```\n\nInstallation from sources (requiring setuptools):\n\n```python\npip install .\n```\n\n\n## CLI usage\n\n```\nodsgenerator [-h] [--version] input_file output_file\n```\n\n### arguments\n\n\n`input_file`: input file containing data in JSON or YAML format\n\n`output_file`: output file, `.ods` file generated from the input\n\nUse ``odsgenerator --help`` for more details about input file parameters\nand look at examples in the tests folder.\n\n\n## Usage from python code\n\n```python\nfrom odsgenerator import odsgenerator\n\ncontent = odsgenerator.ods_bytes([[[\"a\", \"b\", \"c\"], [10, 20, 30]]])\nwith open(\"sample.ods\", \"wb\") as file:\n file.write(content)\n```\n\nThe resulting `.ods` file loaded in a spreadsheet:\n\n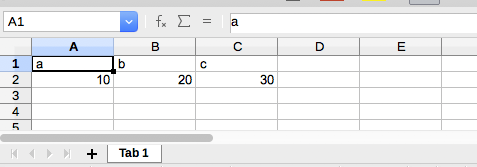\n\nAnother example with more parameters:\n\n```python\nimport odsgenerator\n\ncontent = odsgenerator.ods_bytes(\n [\n {\n \"name\": \"first tab\",\n \"style\": \"cell_decimal2\",\n \"table\": [\n {\n \"row\": [\"a\", \"b\", \"c\"],\n \"style\": \"bold_center_bg_gray_grid_06pt\",\n },\n [10, 20, 30],\n ],\n }\n ]\n)\nwith open(\"sample2.ods\", \"wb\") as file:\n file.write(content)\n```\n\nThe `.ods` file loaded in a spreadsheet, with gray background on first line:\n\n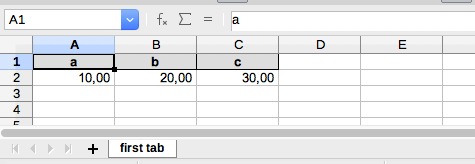\n\n\n## Principle\n\n- A document is a list or dict containing tabs,\n- a tab is a list or dict containing rows,\n- a row is a list or dict containing cells.\n\n\n## Documentation\n\nSee in the `./doc folder:\n\n- `html/odsgenerator.html`\n- `tutorial.json` or `tutorial.yml` and `tutorial.ods`\n\n\n## License\n\nThis project is licensed under the MIT License (see the\n`LICENSE` file for details).\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Generate an OpenDocument Format .ods file from json or yaml file",
"version": "1.11.1",
"project_urls": {
"Homepage": "https://github.com/jdum/odsgenerator",
"Repository": "https://github.com/jdum/odsgenerator"
},
"split_keywords": [
"opendocument",
" odf",
" ods",
" json",
" spreadsheet",
" generator"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3bedd562bb4715c694ac7c029c885a9ef4be045543e014a2244428f06546e0b6",
"md5": "fbb2bc2d6aaacce812def10bedf89176",
"sha256": "16ad1d7d6c0af0deeed5b557a5a8fb5dfe2aea9f7049d95bddd85f73cd3b01ec"
},
"downloads": -1,
"filename": "odsgenerator-1.11.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "fbb2bc2d6aaacce812def10bedf89176",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4,>=3.9",
"size": 10351,
"upload_time": "2024-10-14T18:21:42",
"upload_time_iso_8601": "2024-10-14T18:21:42.620686Z",
"url": "https://files.pythonhosted.org/packages/3b/ed/d562bb4715c694ac7c029c885a9ef4be045543e014a2244428f06546e0b6/odsgenerator-1.11.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "c141a3c47fc5d41b551d1838df91403b4bffc5a9c4e5f17931445e5fc4771f99",
"md5": "532096b60b48d66c511b7688a977038e",
"sha256": "b6d701108da5ffd6e08719161e7e3fe97d61beb1391947f4a788d64de714a165"
},
"downloads": -1,
"filename": "odsgenerator-1.11.1.tar.gz",
"has_sig": false,
"md5_digest": "532096b60b48d66c511b7688a977038e",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4,>=3.9",
"size": 10326,
"upload_time": "2024-10-14T18:21:44",
"upload_time_iso_8601": "2024-10-14T18:21:44.452249Z",
"url": "https://files.pythonhosted.org/packages/c1/41/a3c47fc5d41b551d1838df91403b4bffc5a9c4e5f17931445e5fc4771f99/odsgenerator-1.11.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-14 18:21:44",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "jdum",
"github_project": "odsgenerator",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "odsgenerator"
}