# 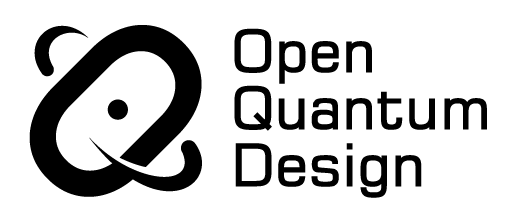
<h2 align="center">
Open Quantum Design: Core
</h2>
[](https://docs.openquantumdesign.org/open-quantum-design-core)
[](https://pypi.org/project/oqd-core)
[](https://github.com/OpenQuantumDesign/oqd-core/actions/workflows/pytest.yml)
[](https://github.com/ambv/black)
[](https://opensource.org/licenses/Apache-2.0)
## Installation <a name="installation"></a>
```bash
pip install oqd-core
```
or through `git`,
```bash
pip install git+https://github.com/OpenQuantumDesign/oqd-core.git
```
To develop, clone the repository locally:
```bash
git clone https://github.com/OpenQuantumDesign/oqd-core
pip install .
```
## Getting Started <a name="Getting Started"></a>
Please see the [documentation](https:docs.openquantumdesign.org) for tutorials, examples, and API reference.
Below is a simple example of an analog quantum program, in which a single qubit evolves under
a $\sigma_X$ Hamiltonian for 10 units of time and is then measured.
```python
from oqd_core.interface.analog.operator import *
from oqd_core.interface.analog.operation import *
X = PauliX()
Z = PauliZ()
Hx = AnalogGate(hamiltonian=X)
circuit = AnalogCircuit()
circuit.evolve(duration=10, gate=Hx)
circuit.measure()
```
## Documentation <a name="documentation"></a>
Documentation is implemented with [MkDocs](https://www.mkdocs.org/).
To install the dependencies for documentation, run:
```
pip install -e ".[docs]"
```
To deploy the documentation server locally:
```
cp -r examples/ docs/examples/
mkdocs serve
```
### Where in the stack
```mermaid
block-beta
columns 3
block:Interface
columns 1
InterfaceTitle("<i><b>Interfaces</b><i/>")
InterfaceDigital["<b>Digital Interface</b>\nQuantum circuits with discrete gates"]
space
InterfaceAnalog["<b>Analog Interface</b>\n Continuous-time evolution with Hamiltonians"]
space
InterfaceAtomic["<b>Atomic Interface</b>\nLight-matter interactions between lasers and ions"]
space
end
block:IR
columns 1
IRTitle("<i><b>IRs</b><i/>")
IRDigital["Quantum circuit IR\nopenQASM, LLVM+QIR"]
space
IRAnalog["openQSIM"]
space
IRAtomic["openAPL"]
space
end
block:Emulator
columns 1
EmulatorsTitle("<i><b>Classical Emulators</b><i/>")
EmulatorDigital["Pennylane, Qiskit"]
space
EmulatorAnalog["QuTiP, QuantumOptics.jl"]
space
EmulatorAtomic["TrICal, QuantumIon.jl"]
space
end
space
block:RealTime
columns 1
RealTimeTitle("<i><b>Real-Time</b><i/>")
space
RTSoftware["ARTIQ, DAX, OQDAX"]
space
RTGateware["Sinara Real-Time Control"]
space
RTHardware["Lasers, Modulators, Photodetection, Ion Trap"]
space
RTApparatus["Trapped-Ion QPU (<sup>171</sup>Yt<sup>+</sup>, <sup>133</sup>Ba<sup>+</sup>)"]
space
end
space
InterfaceDigital --> IRDigital
InterfaceAnalog --> IRAnalog
InterfaceAtomic --> IRAtomic
IRDigital --> IRAnalog
IRAnalog --> IRAtomic
IRDigital --> EmulatorDigital
IRAnalog --> EmulatorAnalog
IRAtomic --> EmulatorAtomic
IRAtomic --> RealTimeTitle
RTSoftware --> RTGateware
RTGateware --> RTHardware
RTHardware --> RTApparatus
classDef title fill:#d6d4d4,stroke:#333,color:#333;
classDef digital fill:#E7E08B,stroke:#333,color:#333;
classDef analog fill:#E4E9B2,stroke:#333,color:#333;
classDef atomic fill:#D2E4C4,stroke:#333,color:#333;
classDef realtime fill:#B5CBB7,stroke:#333,color:#333;
classDef highlight fill:#f2bbbb,stroke:#333,color:#333,stroke-dasharray: 5 5;
class InterfaceTitle,IRTitle,EmulatorsTitle,RealTimeTitle title
class InterfaceDigital,IRDigital,EmulatorDigital digital
class InterfaceAnalog,IRAnalog,EmulatorAnalog analog
class InterfaceAtomic,IRAtomic,EmulatorAtomic atomic
class RTSoftware,RTGateware,RTHardware,RTApparatus realtime
class Interface,IRAnalog,IRAtomic highlight
```
The stack components highlighted in red are contained in this repository.
Raw data
{
"_id": null,
"home_page": null,
"name": "oqd-core",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": null,
"keywords": "quantum, computing, analog, digital, compiler, transpilation, atomic",
"author": null,
"author_email": null,
"download_url": null,
"platform": null,
"description": "# 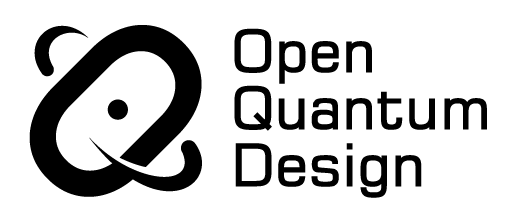\n\n<h2 align=\"center\">\n Open Quantum Design: Core\n</h2>\n\n\n[](https://docs.openquantumdesign.org/open-quantum-design-core)\n[](https://pypi.org/project/oqd-core)\n[](https://github.com/OpenQuantumDesign/oqd-core/actions/workflows/pytest.yml)\n[](https://github.com/ambv/black)\n[](https://opensource.org/licenses/Apache-2.0)\n\n\n## Installation <a name=\"installation\"></a>\n\n```bash\npip install oqd-core\n```\nor through `git`,\n\n```bash\npip install git+https://github.com/OpenQuantumDesign/oqd-core.git\n```\n\nTo develop, clone the repository locally:\n\n```bash\ngit clone https://github.com/OpenQuantumDesign/oqd-core\npip install .\n```\n\n## Getting Started <a name=\"Getting Started\"></a>\nPlease see the [documentation](https:docs.openquantumdesign.org) for tutorials, examples, and API reference.\nBelow is a simple example of an analog quantum program, in which a single qubit evolves under \na $\\sigma_X$ Hamiltonian for 10 units of time and is then measured.\n```python\nfrom oqd_core.interface.analog.operator import *\nfrom oqd_core.interface.analog.operation import *\n\nX = PauliX()\nZ = PauliZ()\n\nHx = AnalogGate(hamiltonian=X)\n\ncircuit = AnalogCircuit()\ncircuit.evolve(duration=10, gate=Hx)\ncircuit.measure()\n```\n\n## Documentation <a name=\"documentation\"></a>\n\nDocumentation is implemented with [MkDocs](https://www.mkdocs.org/).\nTo install the dependencies for documentation, run:\n\n```\npip install -e \".[docs]\"\n```\n\nTo deploy the documentation server locally:\n\n```\ncp -r examples/ docs/examples/\nmkdocs serve\n```\n\n### Where in the stack\n```mermaid\nblock-beta\n columns 3\n \n block:Interface\n columns 1\n InterfaceTitle(\"<i><b>Interfaces</b><i/>\")\n InterfaceDigital[\"<b>Digital Interface</b>\\nQuantum circuits with discrete gates\"] \n space\n InterfaceAnalog[\"<b>Analog Interface</b>\\n Continuous-time evolution with Hamiltonians\"] \n space\n InterfaceAtomic[\"<b>Atomic Interface</b>\\nLight-matter interactions between lasers and ions\"]\n space\n end\n \n block:IR\n columns 1\n IRTitle(\"<i><b>IRs</b><i/>\")\n IRDigital[\"Quantum circuit IR\\nopenQASM, LLVM+QIR\"] \n space\n IRAnalog[\"openQSIM\"]\n space\n IRAtomic[\"openAPL\"]\n space\n end\n \n block:Emulator\n columns 1\n EmulatorsTitle(\"<i><b>Classical Emulators</b><i/>\")\n \n EmulatorDigital[\"Pennylane, Qiskit\"] \n space\n EmulatorAnalog[\"QuTiP, QuantumOptics.jl\"]\n space\n EmulatorAtomic[\"TrICal, QuantumIon.jl\"]\n space\n end\n \n space\n block:RealTime\n columns 1\n RealTimeTitle(\"<i><b>Real-Time</b><i/>\")\n space\n RTSoftware[\"ARTIQ, DAX, OQDAX\"] \n space\n RTGateware[\"Sinara Real-Time Control\"]\n space\n RTHardware[\"Lasers, Modulators, Photodetection, Ion Trap\"]\n space\n RTApparatus[\"Trapped-Ion QPU (<sup>171</sup>Yt<sup>+</sup>, <sup>133</sup>Ba<sup>+</sup>)\"]\n space\n end\n space\n \n InterfaceDigital --> IRDigital\n InterfaceAnalog --> IRAnalog\n InterfaceAtomic --> IRAtomic\n \n IRDigital --> IRAnalog\n IRAnalog --> IRAtomic\n \n IRDigital --> EmulatorDigital\n IRAnalog --> EmulatorAnalog\n IRAtomic --> EmulatorAtomic\n \n IRAtomic --> RealTimeTitle\n \n RTSoftware --> RTGateware\n RTGateware --> RTHardware\n RTHardware --> RTApparatus\n \n classDef title fill:#d6d4d4,stroke:#333,color:#333;\n classDef digital fill:#E7E08B,stroke:#333,color:#333;\n classDef analog fill:#E4E9B2,stroke:#333,color:#333;\n classDef atomic fill:#D2E4C4,stroke:#333,color:#333;\n classDef realtime fill:#B5CBB7,stroke:#333,color:#333;\n\n classDef highlight fill:#f2bbbb,stroke:#333,color:#333,stroke-dasharray: 5 5;\n \n class InterfaceTitle,IRTitle,EmulatorsTitle,RealTimeTitle title\n class InterfaceDigital,IRDigital,EmulatorDigital digital\n class InterfaceAnalog,IRAnalog,EmulatorAnalog analog\n class InterfaceAtomic,IRAtomic,EmulatorAtomic atomic\n class RTSoftware,RTGateware,RTHardware,RTApparatus realtime\n \n class Interface,IRAnalog,IRAtomic highlight\n```\nThe stack components highlighted in red are contained in this repository.\n",
"bugtrack_url": null,
"license": "Apache 2.0",
"summary": null,
"version": "0.1.0",
"project_urls": {
"Homepage": "https://github.com/OpenQuantumDesign/oqd-core",
"Issues": "https://github.com/OpenQuantumDesign/oqd-core/issues",
"Repository": "https://github.com/OpenQuantumDesign/oqd-core.git"
},
"split_keywords": [
"quantum",
" computing",
" analog",
" digital",
" compiler",
" transpilation",
" atomic"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "96e6b8e1ddb9ffd6bfc79e0de958bf275281077435c9c2d5684c1879292a1bfb",
"md5": "cf14c910d49a113e3c439247ee1d0cd8",
"sha256": "7beaf474fe7a9cb335f1329472d188f9b9a75b5c1124a9ec8c6cc97d7318b85b"
},
"downloads": -1,
"filename": "oqd_core-0.1.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "cf14c910d49a113e3c439247ee1d0cd8",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 52549,
"upload_time": "2024-11-12T18:36:42",
"upload_time_iso_8601": "2024-11-12T18:36:42.660781Z",
"url": "https://files.pythonhosted.org/packages/96/e6/b8e1ddb9ffd6bfc79e0de958bf275281077435c9c2d5684c1879292a1bfb/oqd_core-0.1.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-12 18:36:42",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "OpenQuantumDesign",
"github_project": "oqd-core",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "oqd-core"
}