# OSMLF
License: MIT
Version: 0.0.1
OpenStreetMap Location Features is a Python library that utilizes the OpenStreetMap (OSM) database and Overpass API to retrieve location features and perform various calculations based on the given location. It allows you to extract information about amenities, landuse, leisure, tourism, natural features, highways, railways, and waterways for a specified location.
The following are keys provided by OpenStreetMap (OSM). Each link is a reference to the official OSM Wiki.
* Amenities: https://wiki.openstreetmap.org/wiki/Key:amenity
* Landuse : https://wiki.openstreetmap.org/wiki/Key:landuse
* Leisure : https://wiki.openstreetmap.org/wiki/Key:leisure
* Tourism : https://wiki.openstreetmap.org/wiki/Key:tourism
* Natural : https://wiki.openstreetmap.org/wiki/Key:natural
* Highway : https://wiki.openstreetmap.org/wiki/Key:highway
* Railway : https://wiki.openstreetmap.org/wiki/Key:railway
* Waterway : https://wiki.openstreetmap.org/wiki/Key:waterway
### Important Note:
The images included in this README file are taken from the Overpass API. While these images represent the responses of queries used in this module, it should be noted that this module does not return any visual content; it only provides the corresponding response data.
## Requirements
```sh
certifi==2023.5.7
charset-normalizer==3.2.0
geographiclib==2.0
geopy==2.3.0
idna==3.4
numpy==1.25.0
overpy==0.6
pyproj==3.6.0
requests==2.31.0
shapely==2.0.1
urllib3==2.0.4
```
## Usage
Here's examples
```py
# Import the osmlf module
>>> from osmlf import osmlf
```
For example let's look New York City, USA. osmlf will find best result from given location automatically
```py
>>> lf = osmlf('New York City, USA')
>>> lf
osmlf(City of New York, New York, United States)
```
### Default Values
Here are the default values for each key. These are the values that are checked when the key parameter in the relational method's parameter is left blank by the user. You can access and modify these default values if needed:
```py
>>> lf.default_values
{
'amenity': [
'bar', 'cafe', 'fast_food', 'food_court', 'pub', 'restaurant',
'college', 'library', 'school', 'university', 'atm', 'bank',
'clinic', 'dentist', 'doctors', 'hospital', 'pharmacy', 'veterinary',
'cinema', 'conference_centre', 'theatre', 'courthouse', 'fire_station',
'police', 'post_office', 'townhall', 'marketplace', 'grave_yard'
],
'landuse': ['forest', 'residential', 'commercial', 'industrial', 'farming'],
'leisure': ['marina', 'garden', 'park', 'playground', 'stadium'],
'tourism': [
'aquarium', 'artwork', 'attraction', 'hostel', 'hotel',
'motel', 'museum', 'theme_park', 'viewpoint', 'zoo'
],
'natural': ['beach'],
'highway': [
],
'railway': ['platform', 'station', 'stop_area'],
'waterway': []
}
```
### Administrative
Retrieves and returns administrative information about the location from the Overpass API.
**Note:** The total_area variable is expressed in square kilometers.
```py
>>> admin = lf.administrative()
>>> admin
{
'core': (40.7127281, -74.0060152),
'subareas': {
'total_subareas': 5,
'subarea_relation_ids': [
8398124,
9691750,
9691819,
9691916,
9691948
]
},
'total_area': 1147.9149733707309
}
```
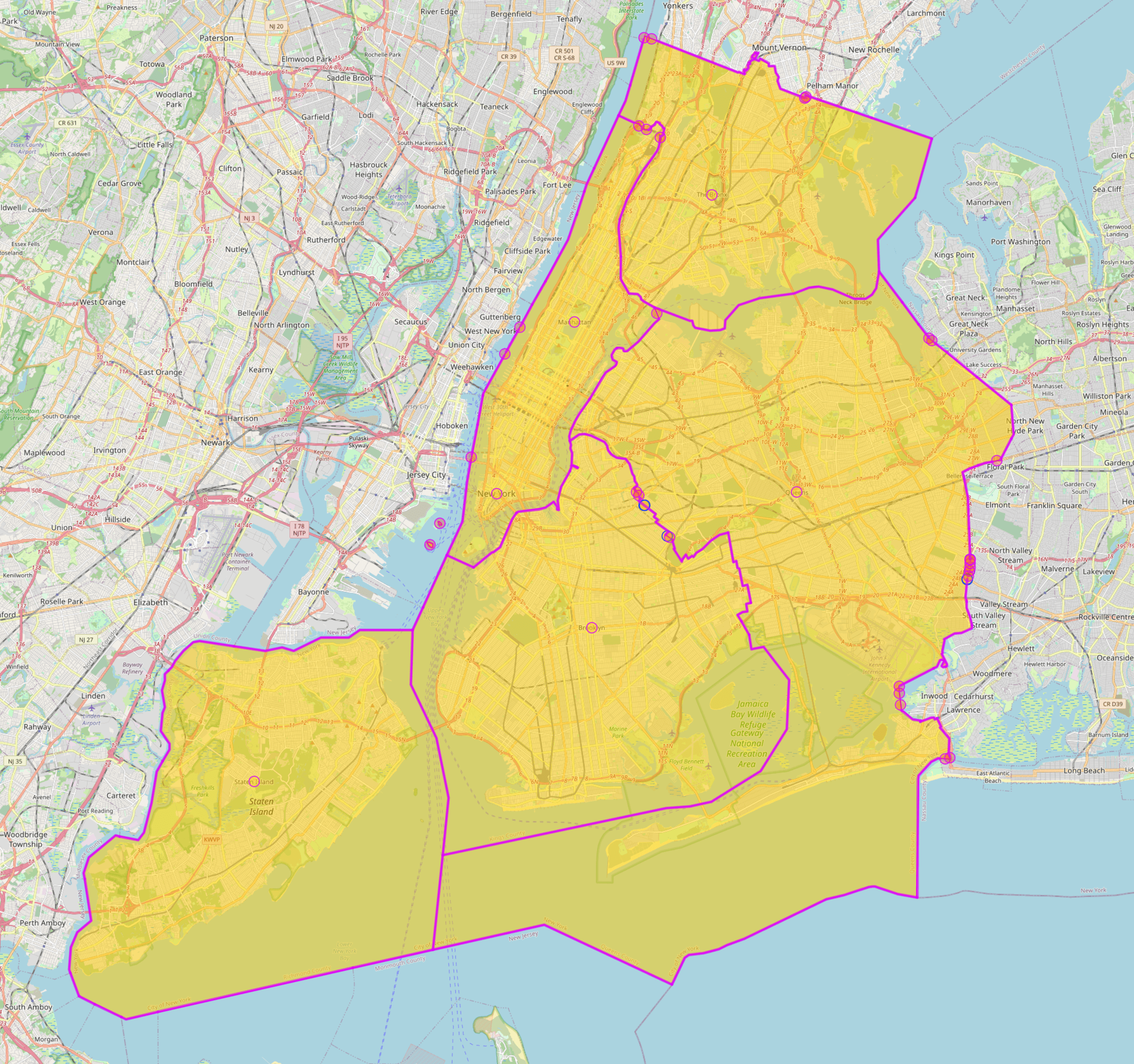
### Amenity
Let's examine the amenities in the Financial District, Manhattan:
```py
>>> lf = osmlf('Financial District, Manhattan, New York')
>>> lf
osmlf(Financial District, Manhattan, New York County, City of New York, New York, United States)
# Search with default amenity values
>>> amenity = lf.amenity()
>>> amenity.keys()
dict_keys(['nodes', 'ways'])
```
Nodes represent locations with a single latitude and longitude pair. Most often, nodes represent smaller areas, such as banks, cafes, bars, restaurants, etc.
Ways, on the other hand, represent areas with multiple latitude and longitude pairs. They usually represent relatively large areas like hospitals, schools, government buildings, etc.
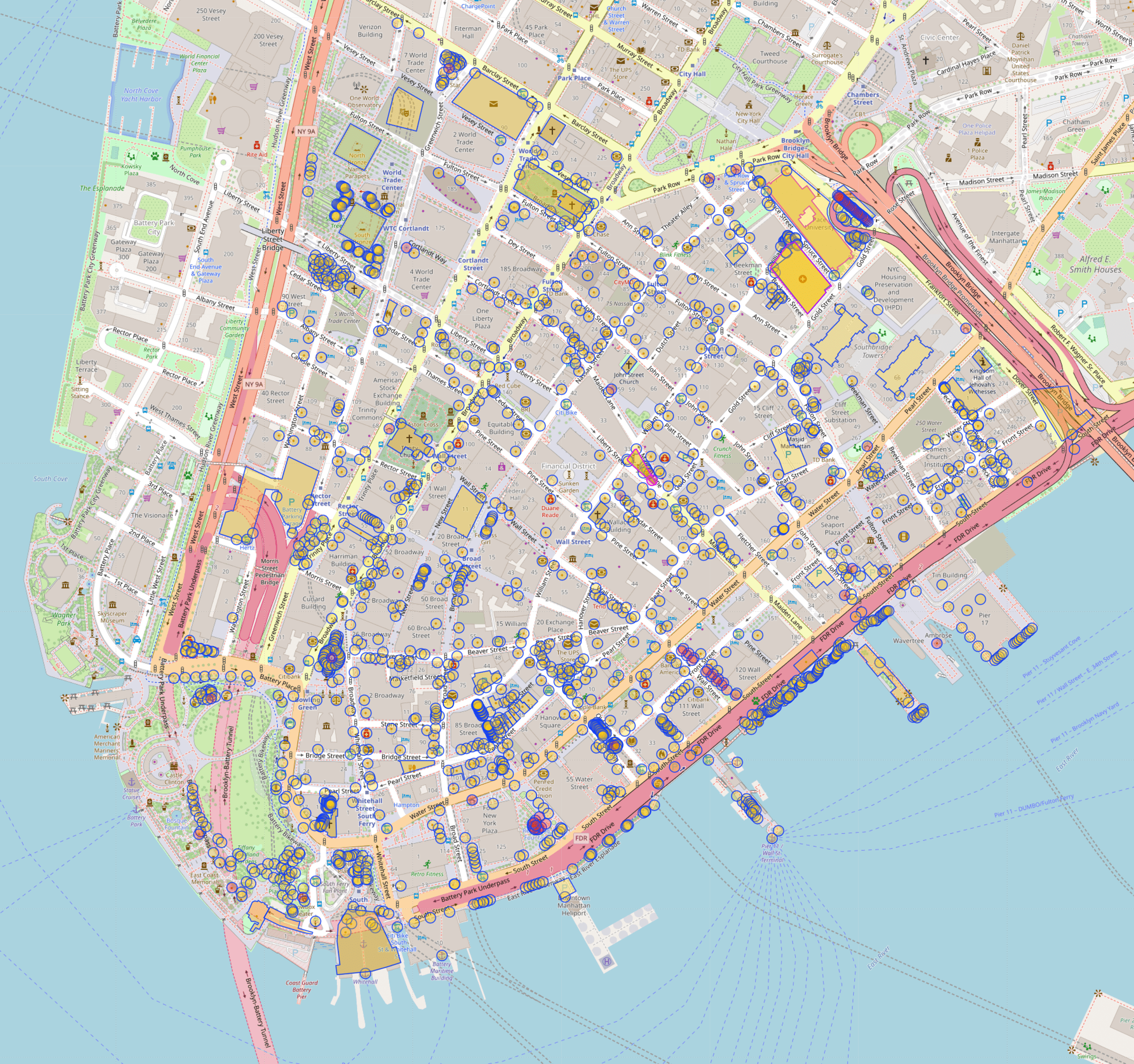
```py
>>> amenity['nodes'].keys()
dict_keys(['bar', 'cafe', 'fast_food', 'food_court', 'pub', 'restaurant', 'college', 'library', 'school', 'university', 'atm', 'bank', 'clinic', 'dentist', 'doctors', 'hospital', 'pharmacy', 'veterinary', 'cinema', 'conference_centre', 'theatre', 'courthouse', 'fire_station', 'police', 'post_office', 'townhall', 'marketplace', 'grave_yard'])
```
For example, let's examine the bars in the Financial District. To do this, we must check both nodes and ways keys:
```py
# Number of bars in Financial District
>>> len(amenity['nodes']['bar'])
11
# Lets check first bar in that list
>>> amenity['nodes']['bar'][0]
{
'id': 3450976122,
'tags': {'addr:city': 'New York',
'addr:housenumber': '36',
'addr:postcode': '10004',
'addr:state': 'NY',
'addr:street': 'Water Street',
'alt_name': 'Porterhouse Bar',
'amenity': 'bar',
'name': 'The Porterhouse Brew Co',
'opening_hours': 'Mo-Fr 11:00-22:00',
'outdoor_seating': 'yes',
'website': 'http://www.zigolinis.com',
'wheelchair': 'no'},
'coordinate': (40.703439, -74.0106749)
}
```
In this example, we retrieve all the information available from the Overpass API. There are 11 bars in the Financial District represented as nodes. Let's check if there are any bars represented as way objects:
```py
>>> amenity['ways']['bar']
{'ways': [], 'way_count': 0, 'total_area': 0} # Empty result
```
Now, let's examine the hospitals in the Financial District.
Unfortunately, we can't see the number of way objects using the len function. The number of ways is returned in the way_count key in the dictionary.
**Note:** This is a known issue that will be fixed in the future.
```py
# Number of hospitals as nodes
>>> len(amenity['nodes']['hospital'])
0
# Number of hospitals as ways
>>> amenity['ways']['hospital']['way_count']
1
# Get all the hospitals
>>> amenity['ways']['hospital']
{
'ways': [
{
'way_id': 799488173,
'tags': {'amenity': 'hospital',
'beds': '132',
'building': 'yes',
'healthcare': 'hospital',
'name': 'NewYork-Presbyterian Lower Manhattan Hospital',
'wikidata': 'Q7013458'
},
'coordinates': [
(40.7108086, -74.0050874),
(40.7102038, -74.0044209),
(40.7101336, -74.0045362),
(40.7101256, -74.0045289),
(40.7101041, -74.0045094),
(40.7098032, -74.0048554),
(40.7103763, -74.0054502),
(40.7104325, -74.0055085),
(40.7104501, -74.0054787),
(40.7106556, -74.0051304),
(40.7107336, -74.0052148),
(40.7108086, -74.0050874)
],
'area': 0.004740726486962053
}
],
'way_count': 1,
'total_area': 0.004740726486962053
}
```
In this example, amenity information is fetched using default keys, but you could also specify the keys like so:
```py
# Get bars only
>>> bars = lf.amenity('bar')
# Get hospitals only
>>> hospitals = lf.amenity('hospital')
# Get bars and hospitals both at the same time
>>> multiple_amenities = lf.amenity(['bar', 'hospital']) # TODO: Use *args
```
**Note:** The amenity function works the same way with the landuse, leisure, tourism, natural, railway, and waterway functions. The only difference is the highway function.
### Landuse
Retrieves landuse information about the location from the Overpass API
```py
# Amsterdam
>>> lf = osmlf('Amsterdam, Netherlands')
>>> lf
osmlf(Amsterdam, Noord-Holland, Nederland)
# Get general info (administrative) of Amsterdam
>>> admin = lf.administrative()
>>> admin
{
'core': (52.3730796, 4.8924534),
'subareas': {
'total_subareas': 0,
'subarea_relation_ids': []
},
'total_area': 184.6089486197638
}
```
When examining a relatively large area like Amsterdam, the landuse function can return a large amount of data, which can sometimes be challenging to process. To manage this, let's first fetch only the residential landuses instead of using the default keys, which would fetch all landuses at once.
```py
# Residential areas of Amsterdam
>>> residential = lf.landuse('residential')
```
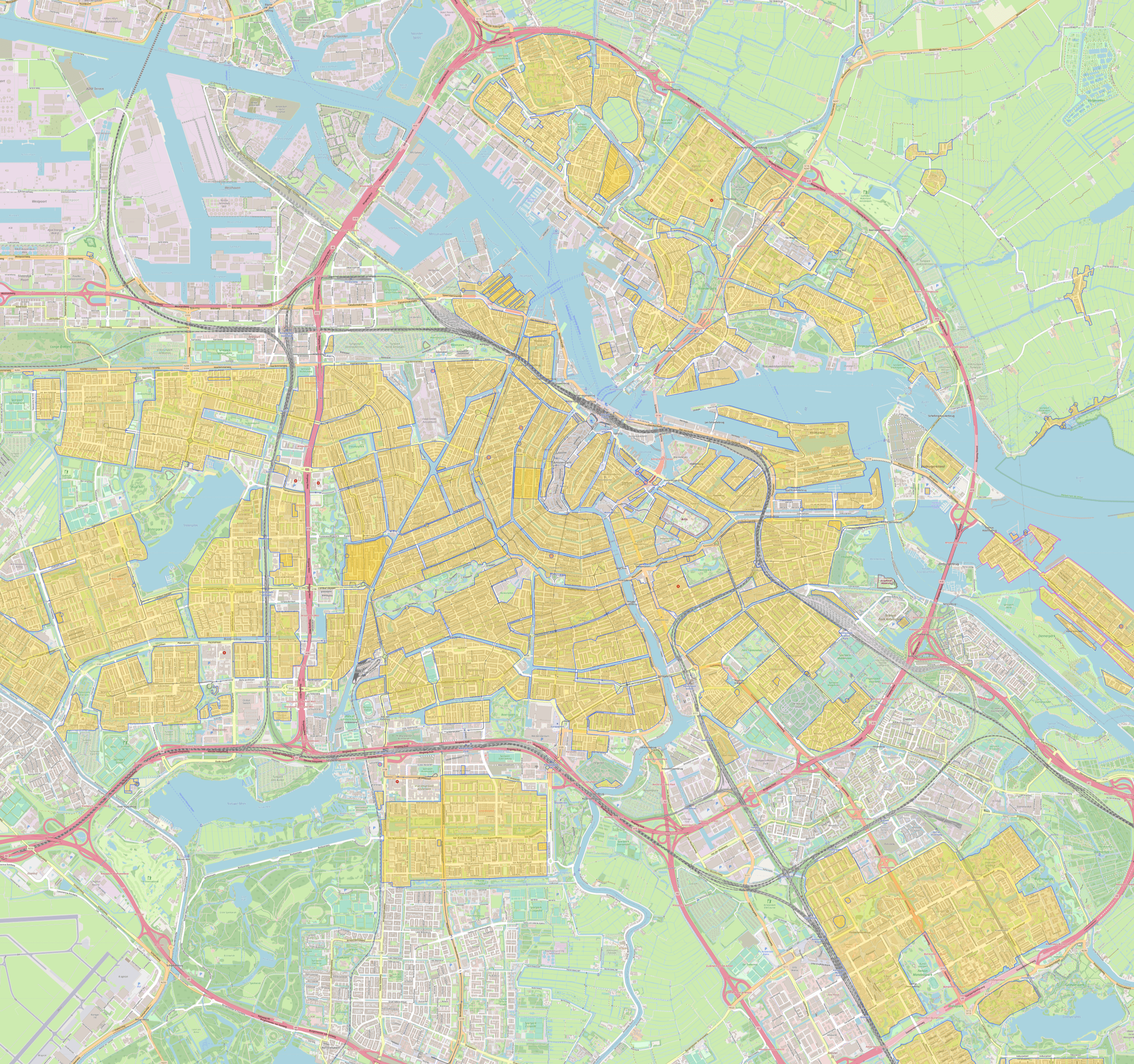
```py
>>> residential.keys()
dict_keys(['nodes', 'ways'])
# No nodes for residential
>>> residential['nodes']
{'residential': []}
# Number of residential areas
>>> residential['ways']['residential']['way_count']
192
# Total area of residential areas in square kilometers
>>> residential['ways']['residential']['total_area']
57.0395957478018
```
To retrieve the first residential area among the 192 areas:
```py
# Get first residential area
>>> residential['ways']['residential']['ways'][0]
{
'way_id': 6337974,
'tags': {
'landuse': 'residential'
},
'coordinates': [
(52.3873421, 4.7586896), (52.3873206, 4.7586027), (52.3872219, 4.7584746),
(52.3866635, 4.75788), (52.3864955, 4.7576667), (52.3863743, 4.757529),
(52.386242, 4.7574293), (52.3860862, 4.7573364), (52.3858845, 4.7572697),
(52.385709, 4.757234), (52.385652, 4.7574567), (52.3854924, 4.7581755),
(52.3851405, 4.7585499), (52.3849747, 4.7587018), (52.3848464, 4.7587884),
(52.3846829, 4.7588023), (52.3845282, 4.7588045), (52.3844951, 4.7587922),
(52.3844981, 4.7588234), (52.3845006, 4.7588549), (52.3845013, 4.7588649),
(52.3845012, 4.7588741), (52.3845008, 4.7588833), (52.384499, 4.7588903),
(52.3844969, 4.7588936), (52.3844944, 4.7588953), (52.3844892, 4.7589017),
(52.3844685, 4.7589349), (52.3844671, 4.7589385), (52.3844668, 4.7589431),
(52.3844451, 4.7589556), (52.3843481, 4.7589603), (52.3842382, 4.7589889),
(52.3842136, 4.7589874), (52.3841915, 4.7589649), (52.3841589, 4.7588225),
(52.383, 4.7588), (52.3826, 4.7586), (52.3818, 4.7589),
(52.3807655, 4.7567289), (52.3801312, 4.7558144), (52.3798, 4.755),
(52.3808, 4.7532), (52.3816, 4.7514), (52.382, 4.7506),
(52.3824794, 4.7500095), (52.3826319, 4.7498799), (52.3832, 4.7499),
(52.3842501, 4.7499516), (52.3842911, 4.7497662), (52.38449, 4.7499965),
(52.384483, 4.750057), (52.3845537, 4.7501347), (52.3845904, 4.7501106),
(52.3847177, 4.7500748), (52.3847762, 4.7500795), (52.3848649, 4.7502082),
(52.3848693, 4.750215), (52.384874, 4.7502226), (52.3849298, 4.7503128),
(52.3849465, 4.7503394), (52.384978, 4.7503975), (52.3849832, 4.7504213),
(52.3849919, 4.7504401), (52.3850028, 4.7504572), (52.3850106, 4.7504758),
(52.3850236, 4.7505078), (52.3850305, 4.7505329), (52.3850346, 4.7505578),
(52.3850667, 4.7506094), (52.3850853, 4.7506021), (52.3851002, 4.7505518),
(52.3852322, 4.7506344), (52.3852225, 4.7506759), (52.3852483, 4.7507373),
(52.3852518, 4.7507611), (52.3852675, 4.750849), (52.3852746, 4.7509073),
(52.3852804, 4.7509674), (52.3852878, 4.7510422), (52.38529, 4.7510516),
(52.3853052, 4.7510889), (52.3853194, 4.7511319), (52.3861569, 4.7519683),
(52.3871396, 4.7527243), (52.3883416, 4.7534281), (52.3881, 4.7549),
(52.3876, 4.7573), (52.3873421, 4.7586896)
],
'area': 0.39929878337602165
}
```
In this example, we're only showing residential areas, but landuse can fetch more than just residential areas—it can also fetch forests, commercial areas, industrial areas, farming areas, etc.
For example:
```py
# Get total area of forest, meadow and grass in Amsterdam
# It will take some time
>>> landuse = lf.landuse(['forest', 'meadow', 'grass'])
# Show keys
>>> landuse['ways'].keys()
dict_keys(['forest', 'meadow', 'grass'])
# Forest area in square kilometers
>>> landuse['ways']['forest']['total_area']
9.608440158531183
# Meadow area in square kilometers
>>> landuse['ways']['meadow']['total_area']
10.776521682770907
# Grass area in square kilometers
>>> landuse['ways']['grass']['total_area']
31.5818360868127
```
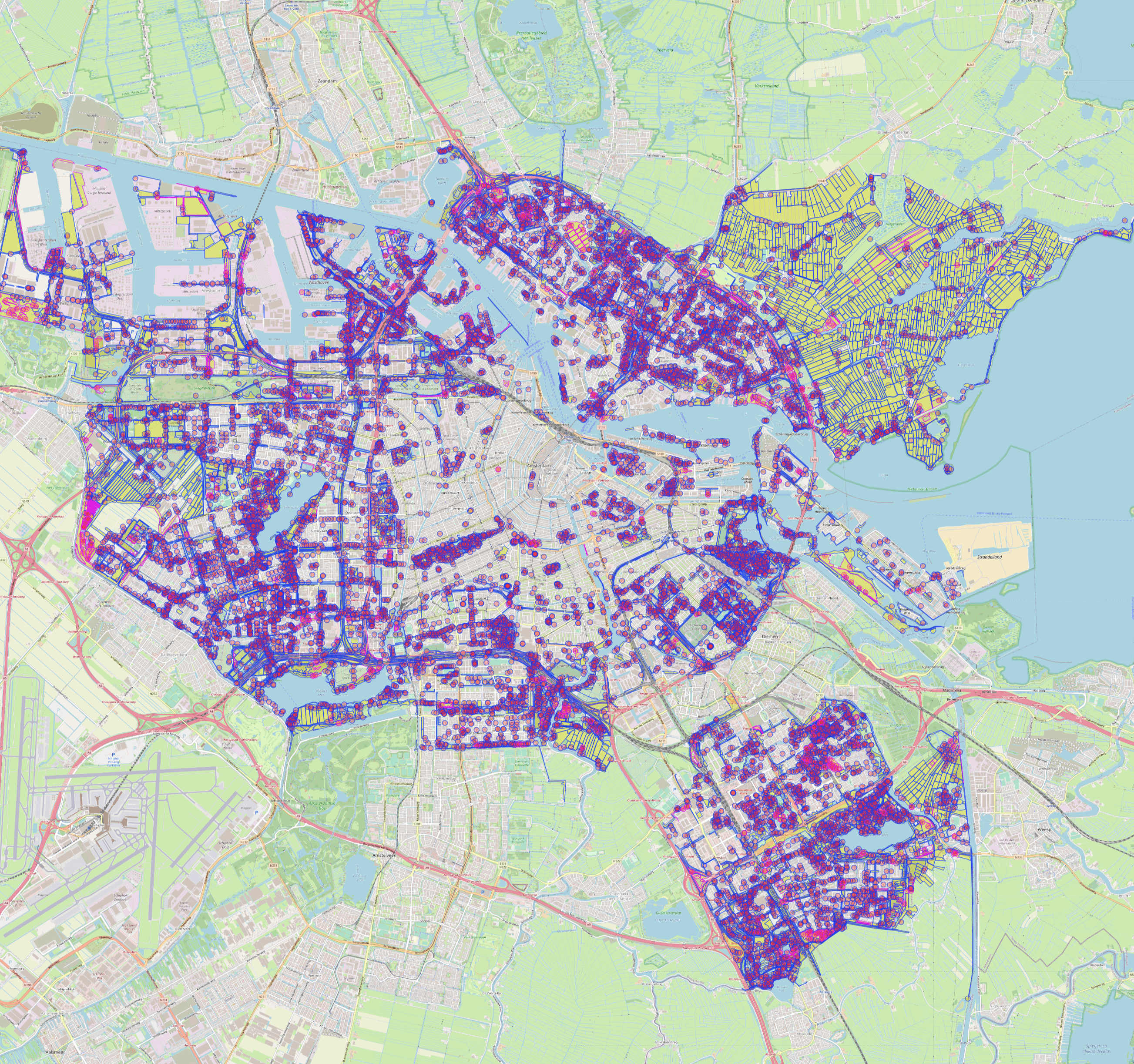
### Tourism
Retrieves tourism information about the location from the Overpass API.
```py
>>> lf = osmlf('Venice, Italy')
>>> lf
osmlf(Venezia, Veneto, Italia)
>>> tourism = lf.tourism()
# The iconic Campanile di San Marco
>>> tourism['ways']['attraction']['ways'][31]
{
'way_id': 252637693,
'tags': {
'building': 'yes',
'height': '98.6',
'historic': 'yes',
'man_made': 'tower',
'name': 'Campanile di San Marco',
'name:be': 'Кампаніла сабора Святога Марка',
'name:ca': 'Campanar de Sant Marc',
'name:de': 'Markusturm',
'name:en': "St Mark's Campanile",
'name:eo': 'Kampanilo de Sankta Marko',
'name:es': 'Campanile de San Marcos',
'name:fr': 'Campanile de Saint-Marc',
'name:he': 'הקמפנילה של סן מרקו',
'name:it': 'Campanile di San Marco',
'name:ja': '鐘楼(サンマルコ広場)',
'name:ko': '산마르코의 종탑',
'name:pl': 'Dzwonnica św. Marka',
'name:pt': 'Campanário de São Marcos',
'name:ro': 'Campanila San Marco',
'name:ru': 'Кампанила собора Святого Марка',
'name:th': 'หอระฆังซันมาร์โก',
'name:tr': "Aziz Mark'ın Çan kulesi",
'name:uk': 'Кампаніла собору святого Марка',
'name:vec': 'Canpanièl de San Marco',
'name:zh': '圣马可钟楼',
'tourism': 'attraction',
'tower:construction': 'brick',
'tower:height': '95',
'tower:type': 'bell_tower',
'wheelchair': 'yes',
'wikidata': 'Q754194',
'wikipedia': 'it:Campanile di San Marco',
'wikipedia:fr': 'Campanile de Saint-Marc'
},
'coordinates': [
(45.4340712, 12.3389421),
(45.4340844, 12.3389951),
(45.4340901, 12.3389922),
(45.434095, 12.3390124),
(45.4340894, 12.3390152),
(45.4341083, 12.3390916),
(45.434001, 12.3391464),
(45.4339639, 12.338997),
(45.4340712, 12.3389421)
],
'area': 0.0001583511784691957
}
```
The data also includes information on hotels. For example, the number of hotels can be retrieved with:
```py
>>> len(tourism['nodes']['hotel']) # Hotels node object
328
>>> tourism['ways']['hotel']['way_count'] # Hotels way object
30
# First element of hotel in nodes
>>> tourism['nodes']['hotel'][0]
{
'id': 313715870,
'tags': {
'addr:housenumber': '29',
'addr:place': 'Isola Torcello',
'addr:postcode': '30142',
'amenity': 'restaurant',
'check_date:opening_hours': '2022-09-26',
'name': 'Locanda Cipriani',
'opening_hours:signed': 'no',
'tourism': 'hotel'
},
'coordinate': (45.4978252, 12.4179196)
}
# First element of hotel in ways
>>> tourism['ways']['hotel']['ways'][0]
{
'way_id': 60996087,
'tags': {
'addr:city': 'Lido di Venezia',
'addr:housenumber': '41',
'addr:postcode': '30126',
'addr:street': 'Lungomare Guglielmo Marconi',
'internet_access': 'wlan',
'internet_access:fee': 'no',
'name': 'Hotel Excelsior Venice',
'stars': '5',
'tourism': 'hotel',
'wikidata': 'Q1542590'
},
'coordinates': [(
45.4045649, 12.3668121),
(45.4038431, 12.3661057),
(45.4037498, 12.3662666),
(45.403303, 12.365826),
(45.4033521, 12.3656791),
(45.4032686, 12.3655952),
(45.4031311, 12.3659029),
(45.4043489, 12.3671408),
(45.4044618, 12.3668611),
(45.4045158, 12.366917),
(45.4045649, 12.3668121)
],
'area': 0.004546061610650756
}
```
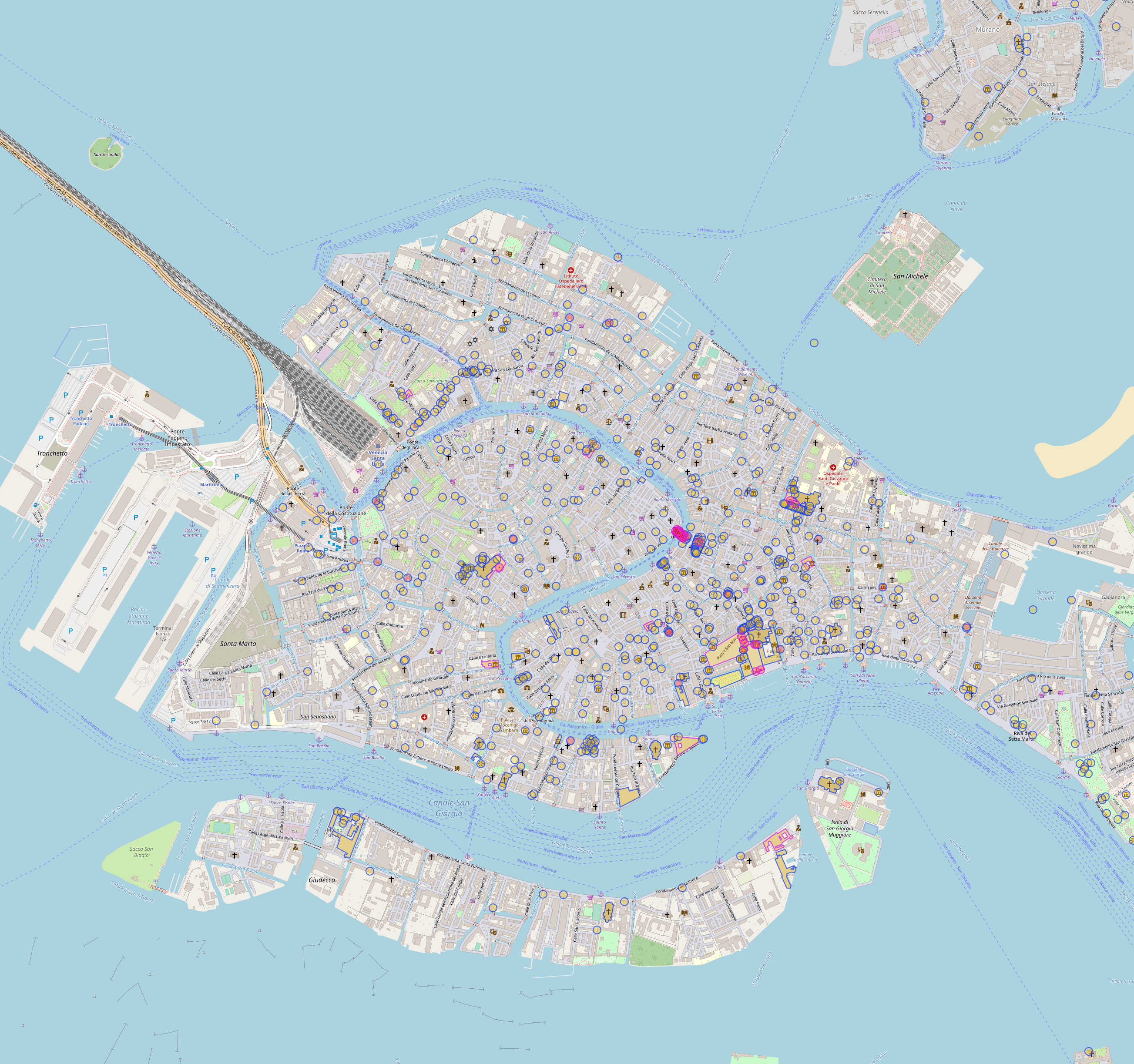
### Natural
This function retrieves natural feature information about the location from the Overpass API. The default value for the natural key is beach.
```py
>>> lf = osmlf('Fethiye, Mugla, Turkey')
>>> lf
osmlf(Fethiye, Muğla, Ege Bölgesi, Türkiye)
>>> natural = lf.natural()
# Number of beaches in Fethiye
>>> len(natural['nodes']['beach']) # Beaches as node object
5
# Get the first beach node object
>>> natural['nodes']['beach'][0]
{
'id': 769903797,
'tags': {
'fee': 'yes',
'name': 'Sun City Beach',
'natural': 'beach',
'surface': 'sand'
},
'coordinate': (36.5555012, 29.1067139)
}
# Get the first beach way object
>>> natural['ways']['beach']['ways'][0]
{
'way_id': 87294614,
'tags': {
'access': 'yes',
'fee': 'yes',
'name': 'Kıdrak Plajı',
'name:en': 'Kıdrak Beach',
'name:ru': 'Кидрак пляж',
'natural': 'beach',
'source': 'Bing'
},
'coordinates': [
(36.5282195, 29.1274236),
(36.5283159, 29.1275884),
(36.5286952, 29.1279593),
(36.5287867, 29.1279884),
(36.5290436, 29.1280701),
(36.5297094, 29.1281279),
(36.5303753, 29.1280027),
(36.5309869, 29.127757),
(36.5313553, 29.1277077),
(36.5313843, 29.1280615),
(36.5315474, 29.1280792),
(36.5320484, 29.1279282),
(36.5321612, 29.1276559),
(36.5322553, 29.127576),
(36.5321443, 29.126832),
(36.5319627, 29.1264458),
(36.5310326, 29.1270217),
(36.5299757, 29.1273724),
(36.529036, 29.1274781),
(36.5282195, 29.1274236)
],
'area': 0.030907790857999908
}
```
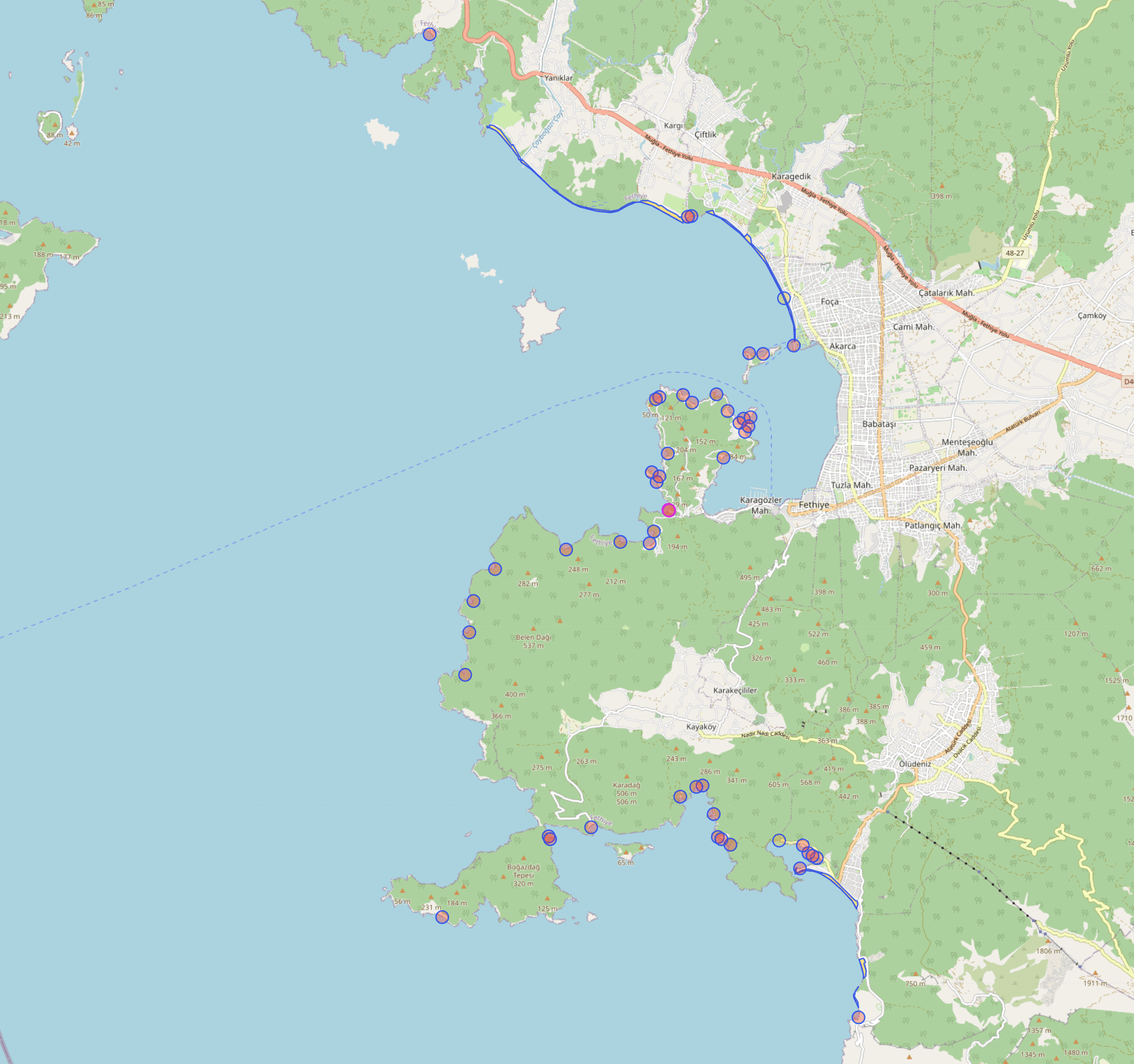
### Highway
Highways function a bit differently compared to other functions. For instance, unlike the tourism function where we can search for the hotel key, in the highway function we can't find tags by keys because it only lists the items.
```py
>>> lf = osmlf('sodermalm, stockholm, sweden')
>>> lf
osmlf(Södermalm, Södermalms stadsdelsområde, Stockholm, Stockholms kommun, Stockholms län, Sverige)
```
The total_length key provides the total length of every road listed in info in kilometers.
```py
# Get highway information
>>> highway = lf.highway()
>>> highway.keys()
dict_keys(['total_length', 'info'])
```
`total_lenght` is total length of every road in `info` in kilometers
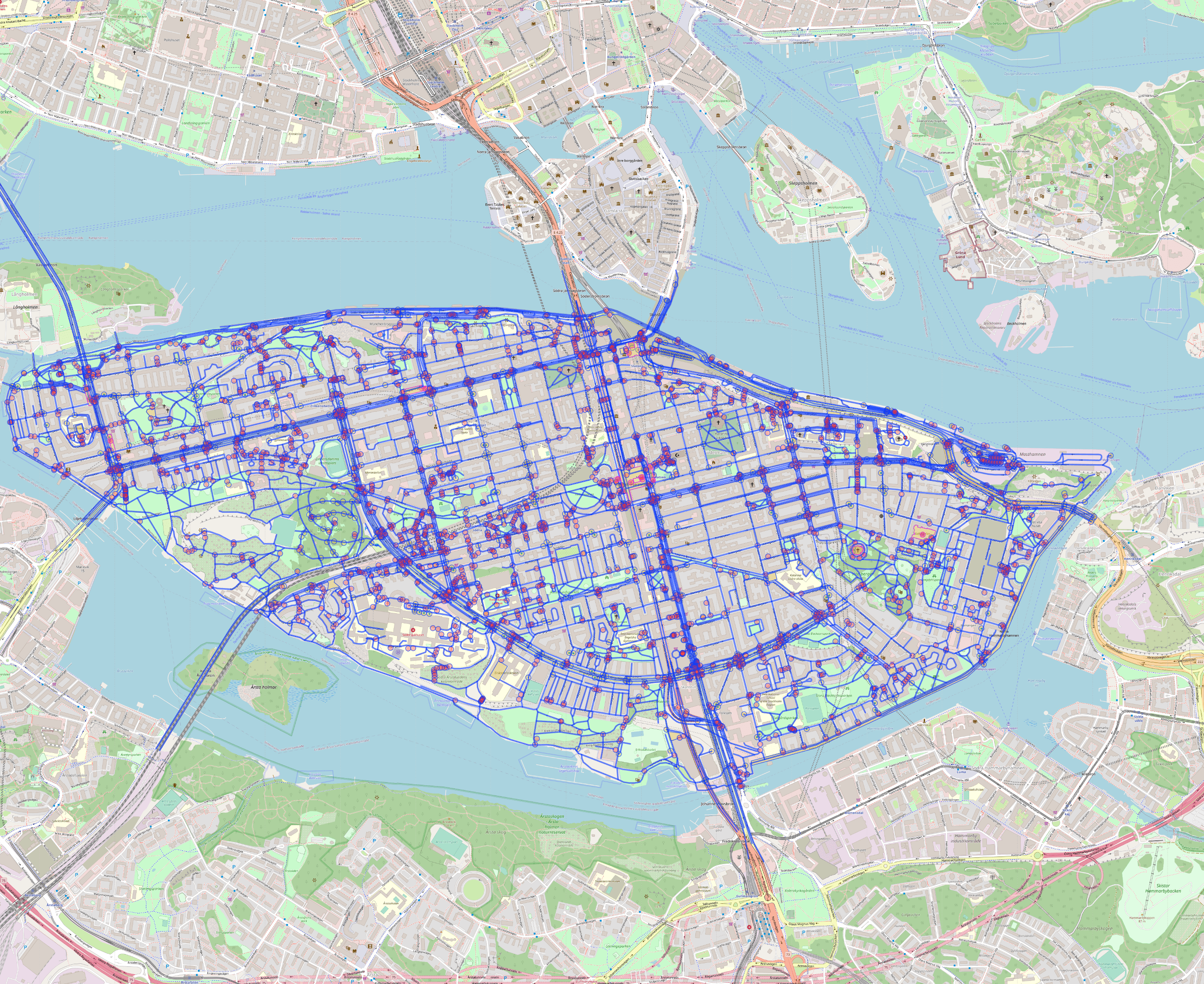
```py
# Get total length of all roads returned in kilometers
>>> highway['total_length']
279.45176280917764
```
In the info list, we have details about every road. We can filter by type, such as motorways, pedestrian, residential roads, etc.
```py
# Get residential roads
>>> residential = lf.highway('residential')
# Total length of residential
>>> residential['total_length']
58.977220710878655
# Get first residential road in list
>>> residential['info'][0]
{
'tags': {
'highway': 'residential',
'maxspeed': '30',
'name': 'Hornsgatan',
'surface': 'asphalt',
'wikidata': 'Q3140664',
'wikipedia': 'sv:Hornsgatan'
},
'coordinates': [
(59.3148503, 18.0316059),
(59.3150171, 18.0318446),
(59.315038, 18.031897),
(59.3150498, 18.0319613),
(59.3152164, 18.0333084)
],
'length': 0.10962269653879471
}
```
### Railway
Retrieves railway information about the location from the Overpass API.
```py
>>> lf = osmlf('Moscow, Russia')
>>> lf
osmlf(Москва, Центральный федеральный округ, Россия)
>>> railway = lf.railway()
>>> railway.keys()
dict_keys(['nodes', 'ways'])
# Number of stations
>>> len(railway['nodes']['station'])
312
# Get first station
>>> railway['nodes']['station'][0]
{
'id': 26999673,
'tags': {
'alt_name': 'Москва-Октябрьская',
'esr:user': '060073',
'loc_name': 'Москва-Ленинградская',
'loc_name:website': 'http://www.tutu.ru/station.php?nnst=79310',
'name': 'Москва-Пассажирская',
'nat_name': 'Москва',
'network': 'РЖД',
'official_name': 'Москва-Пассажирская',
'official_name:esr': 'Москва-Пассажирская',
'official_name:esr:website': 'http://cargo.rzd.ru/cargostation/public/ru?STRUCTURE_ID=5101&layer_id=4829&page4821_2705=1&refererLayerId=4821&id=1090',
'official_name:express-3': 'Москва-Октябрьская',
'official_name:express-3:website': 'http://bilet.ru/rus/TrainDirectory.htm?firstsymb=%u041c',
'official_name:website': 'http://pass.rzd.ru/timetable/public/ru?STRUCTURE_ID=5104&layer_id=5368&id=278&node_id=19',
'operator': 'Октябрьская железная дорога',
'public_transport': 'station',
'railway': 'station',
'train': 'yes',
'uic_name': 'Moskva Oktiabrskaia',
'uic_ref': '2006004'
},
'coordinate': (55.7788343, 37.6537207)
}
```
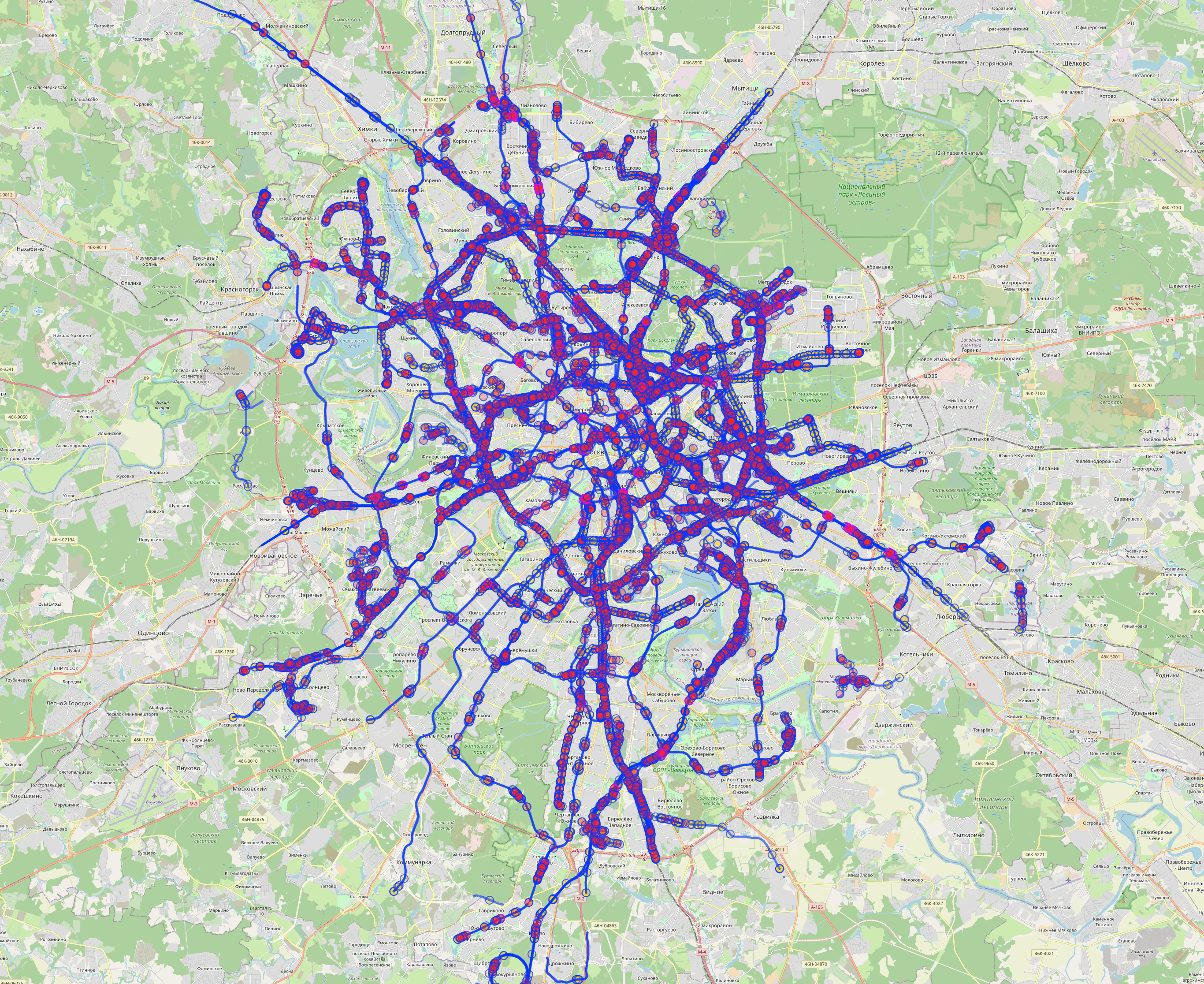
### Waterway
Doesn't work properly. TODO: Fix it
Raw data
{
"_id": null,
"home_page": "https://github.com/ccan23/osmlf",
"name": "osmlf",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "python,map,openstreetmap,amenities,location,coordinates,latitude,longitude",
"author": "ccan23",
"author_email": "dev.ccanb@protonmail.com",
"download_url": "https://files.pythonhosted.org/packages/0b/69/ea78b31d0fb97ad6aa63a2937df4732b78e9b31d6811c800a588bed947f3/osmlf-0.0.1.tar.gz",
"platform": null,
"description": "# OSMLF\nLicense: MIT\n\nVersion: 0.0.1\n\nOpenStreetMap Location Features is a Python library that utilizes the OpenStreetMap (OSM) database and Overpass API to retrieve location features and perform various calculations based on the given location. It allows you to extract information about amenities, landuse, leisure, tourism, natural features, highways, railways, and waterways for a specified location.\n\nThe following are keys provided by OpenStreetMap (OSM). Each link is a reference to the official OSM Wiki.\n* Amenities: https://wiki.openstreetmap.org/wiki/Key:amenity\n* Landuse : https://wiki.openstreetmap.org/wiki/Key:landuse\n* Leisure : https://wiki.openstreetmap.org/wiki/Key:leisure\n* Tourism : https://wiki.openstreetmap.org/wiki/Key:tourism\n* Natural : https://wiki.openstreetmap.org/wiki/Key:natural\n* Highway : https://wiki.openstreetmap.org/wiki/Key:highway\n* Railway : https://wiki.openstreetmap.org/wiki/Key:railway\n* Waterway : https://wiki.openstreetmap.org/wiki/Key:waterway\n\n### Important Note: \nThe images included in this README file are taken from the Overpass API. While these images represent the responses of queries used in this module, it should be noted that this module does not return any visual content; it only provides the corresponding response data.\n## Requirements\n```sh\ncertifi==2023.5.7\ncharset-normalizer==3.2.0\ngeographiclib==2.0\ngeopy==2.3.0\nidna==3.4\nnumpy==1.25.0\noverpy==0.6\npyproj==3.6.0\nrequests==2.31.0\nshapely==2.0.1\nurllib3==2.0.4\n```\n## Usage\nHere's examples\n```py\n# Import the osmlf module\n>>> from osmlf import osmlf\n```\n\nFor example let's look New York City, USA. osmlf will find best result from given location automatically\n```py\n>>> lf = osmlf('New York City, USA')\n>>> lf\nosmlf(City of New York, New York, United States)\n```\n### Default Values\nHere are the default values for each key. These are the values that are checked when the key parameter in the relational method's parameter is left blank by the user. You can access and modify these default values if needed:\n```py\n>>> lf.default_values\n{\n 'amenity': [\n 'bar', 'cafe', 'fast_food', 'food_court', 'pub', 'restaurant',\n 'college', 'library', 'school', 'university', 'atm', 'bank',\n 'clinic', 'dentist', 'doctors', 'hospital', 'pharmacy', 'veterinary',\n 'cinema', 'conference_centre', 'theatre', 'courthouse', 'fire_station',\n 'police', 'post_office', 'townhall', 'marketplace', 'grave_yard'\n ],\n 'landuse': ['forest', 'residential', 'commercial', 'industrial', 'farming'],\n 'leisure': ['marina', 'garden', 'park', 'playground', 'stadium'],\n 'tourism': [\n 'aquarium', 'artwork', 'attraction', 'hostel', 'hotel', \n 'motel', 'museum', 'theme_park', 'viewpoint', 'zoo'\n ],\n 'natural': ['beach'],\n 'highway': [\n \n ],\n 'railway': ['platform', 'station', 'stop_area'],\n 'waterway': []\n}\n```\n\n### Administrative\nRetrieves and returns administrative information about the location from the Overpass API.\n\n**Note:** The total_area variable is expressed in square kilometers.\n```py\n>>> admin = lf.administrative()\n>>> admin\n{\n 'core': (40.7127281, -74.0060152),\n 'subareas': {\n 'total_subareas': 5,\n 'subarea_relation_ids': [\n 8398124,\n 9691750,\n 9691819,\n 9691916,\n 9691948\n ]\n },\n 'total_area': 1147.9149733707309\n}\n```\n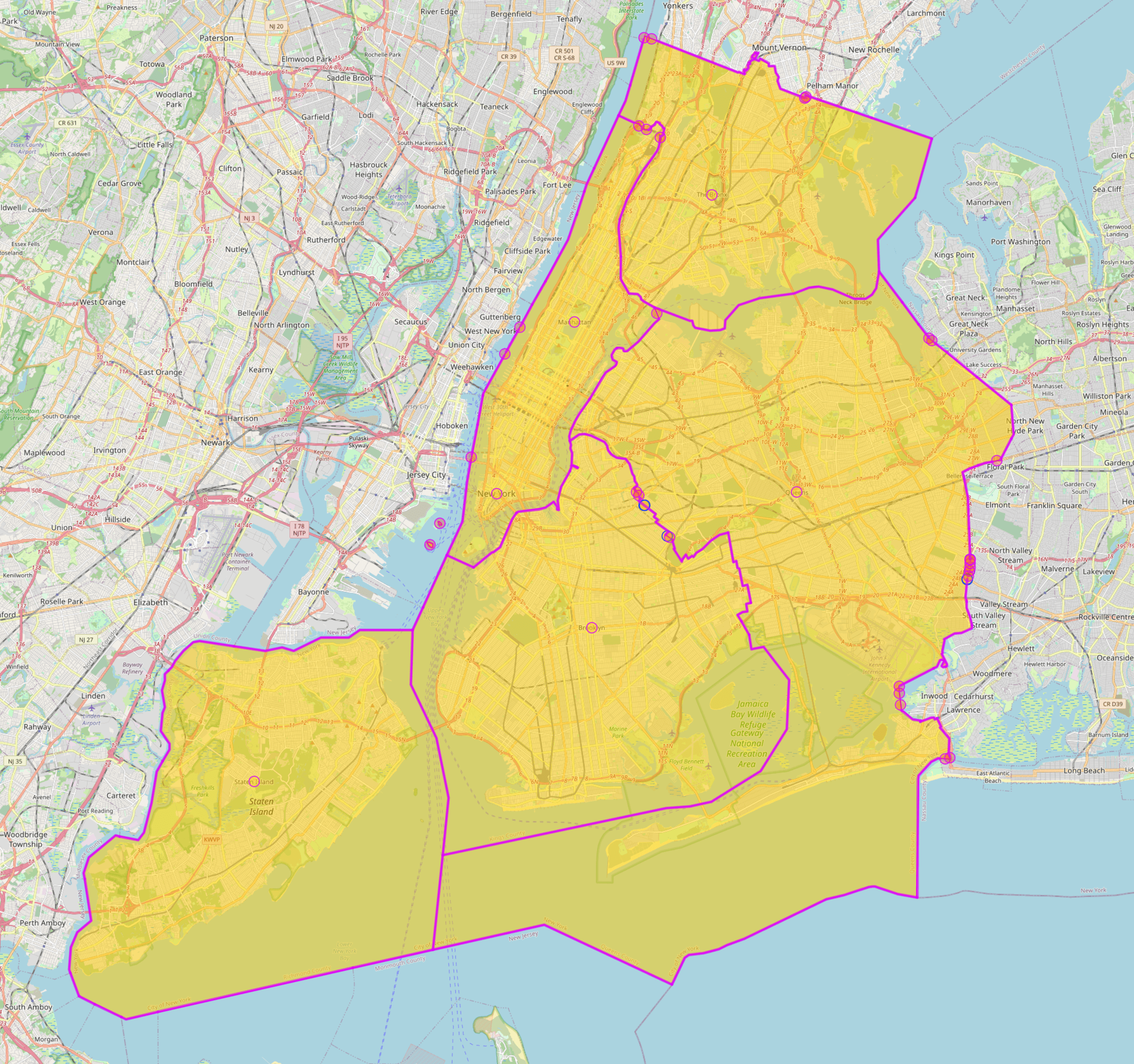\n\n### Amenity\nLet's examine the amenities in the Financial District, Manhattan:\n```py\n>>> lf = osmlf('Financial District, Manhattan, New York')\n>>> lf\nosmlf(Financial District, Manhattan, New York County, City of New York, New York, United States)\n\n# Search with default amenity values\n>>> amenity = lf.amenity()\n>>> amenity.keys()\ndict_keys(['nodes', 'ways'])\n```\nNodes represent locations with a single latitude and longitude pair. Most often, nodes represent smaller areas, such as banks, cafes, bars, restaurants, etc.\n\nWays, on the other hand, represent areas with multiple latitude and longitude pairs. They usually represent relatively large areas like hospitals, schools, government buildings, etc.\n\n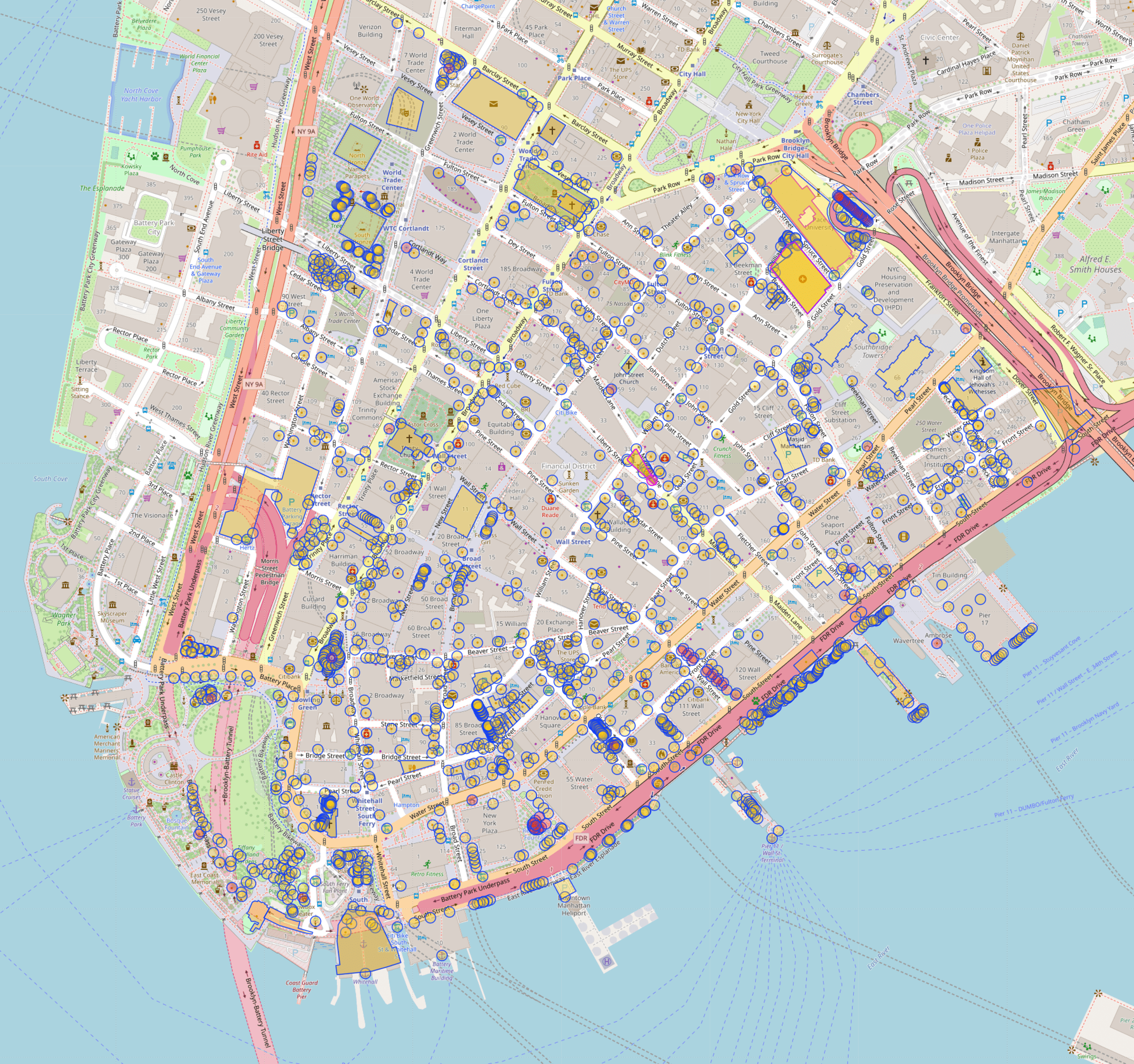\n\n```py\n>>> amenity['nodes'].keys()\ndict_keys(['bar', 'cafe', 'fast_food', 'food_court', 'pub', 'restaurant', 'college', 'library', 'school', 'university', 'atm', 'bank', 'clinic', 'dentist', 'doctors', 'hospital', 'pharmacy', 'veterinary', 'cinema', 'conference_centre', 'theatre', 'courthouse', 'fire_station', 'police', 'post_office', 'townhall', 'marketplace', 'grave_yard'])\n```\nFor example, let's examine the bars in the Financial District. To do this, we must check both nodes and ways keys:\n\n```py\n# Number of bars in Financial District\n>>> len(amenity['nodes']['bar'])\n11\n\n# Lets check first bar in that list\n>>> amenity['nodes']['bar'][0]\n{\n 'id': 3450976122,\n 'tags': {'addr:city': 'New York',\n 'addr:housenumber': '36',\n 'addr:postcode': '10004',\n 'addr:state': 'NY',\n 'addr:street': 'Water Street',\n 'alt_name': 'Porterhouse Bar',\n 'amenity': 'bar',\n 'name': 'The Porterhouse Brew Co',\n 'opening_hours': 'Mo-Fr 11:00-22:00',\n 'outdoor_seating': 'yes',\n 'website': 'http://www.zigolinis.com',\n 'wheelchair': 'no'},\n 'coordinate': (40.703439, -74.0106749)\n}\n```\nIn this example, we retrieve all the information available from the Overpass API. There are 11 bars in the Financial District represented as nodes. Let's check if there are any bars represented as way objects:\n\n```py\n>>> amenity['ways']['bar']\n{'ways': [], 'way_count': 0, 'total_area': 0} # Empty result\n```\n\nNow, let's examine the hospitals in the Financial District.\n\nUnfortunately, we can't see the number of way objects using the len function. The number of ways is returned in the way_count key in the dictionary.\n\n**Note:** This is a known issue that will be fixed in the future.\n```py\n# Number of hospitals as nodes\n>>> len(amenity['nodes']['hospital'])\n0\n\n# Number of hospitals as ways\n>>> amenity['ways']['hospital']['way_count']\n1\n\n# Get all the hospitals\n>>> amenity['ways']['hospital']\n{\n 'ways': [\n {\n 'way_id': 799488173,\n 'tags': {'amenity': 'hospital',\n 'beds': '132',\n 'building': 'yes',\n 'healthcare': 'hospital',\n 'name': 'NewYork-Presbyterian Lower Manhattan Hospital',\n 'wikidata': 'Q7013458'\n },\n 'coordinates': [\n (40.7108086, -74.0050874),\n (40.7102038, -74.0044209),\n (40.7101336, -74.0045362),\n (40.7101256, -74.0045289),\n (40.7101041, -74.0045094),\n (40.7098032, -74.0048554),\n (40.7103763, -74.0054502),\n (40.7104325, -74.0055085),\n (40.7104501, -74.0054787),\n (40.7106556, -74.0051304),\n (40.7107336, -74.0052148),\n (40.7108086, -74.0050874)\n ],\n 'area': 0.004740726486962053\n }\n ],\n 'way_count': 1,\n 'total_area': 0.004740726486962053\n}\n```\n\nIn this example, amenity information is fetched using default keys, but you could also specify the keys like so:\n\n```py\n# Get bars only\n>>> bars = lf.amenity('bar')\n\n# Get hospitals only\n>>> hospitals = lf.amenity('hospital')\n\n# Get bars and hospitals both at the same time\n>>> multiple_amenities = lf.amenity(['bar', 'hospital']) # TODO: Use *args\n```\n\n**Note:** The amenity function works the same way with the landuse, leisure, tourism, natural, railway, and waterway functions. The only difference is the highway function.\n\n### Landuse\nRetrieves landuse information about the location from the Overpass API\n\n```py\n# Amsterdam\n>>> lf = osmlf('Amsterdam, Netherlands')\n>>> lf\nosmlf(Amsterdam, Noord-Holland, Nederland)\n\n# Get general info (administrative) of Amsterdam\n>>> admin = lf.administrative()\n>>> admin\n{\n 'core': (52.3730796, 4.8924534), \n 'subareas': {\n 'total_subareas': 0, \n 'subarea_relation_ids': []\n }, \n 'total_area': 184.6089486197638\n}\n```\nWhen examining a relatively large area like Amsterdam, the landuse function can return a large amount of data, which can sometimes be challenging to process. To manage this, let's first fetch only the residential landuses instead of using the default keys, which would fetch all landuses at once.\n```py\n# Residential areas of Amsterdam\n>>> residential = lf.landuse('residential')\n```\n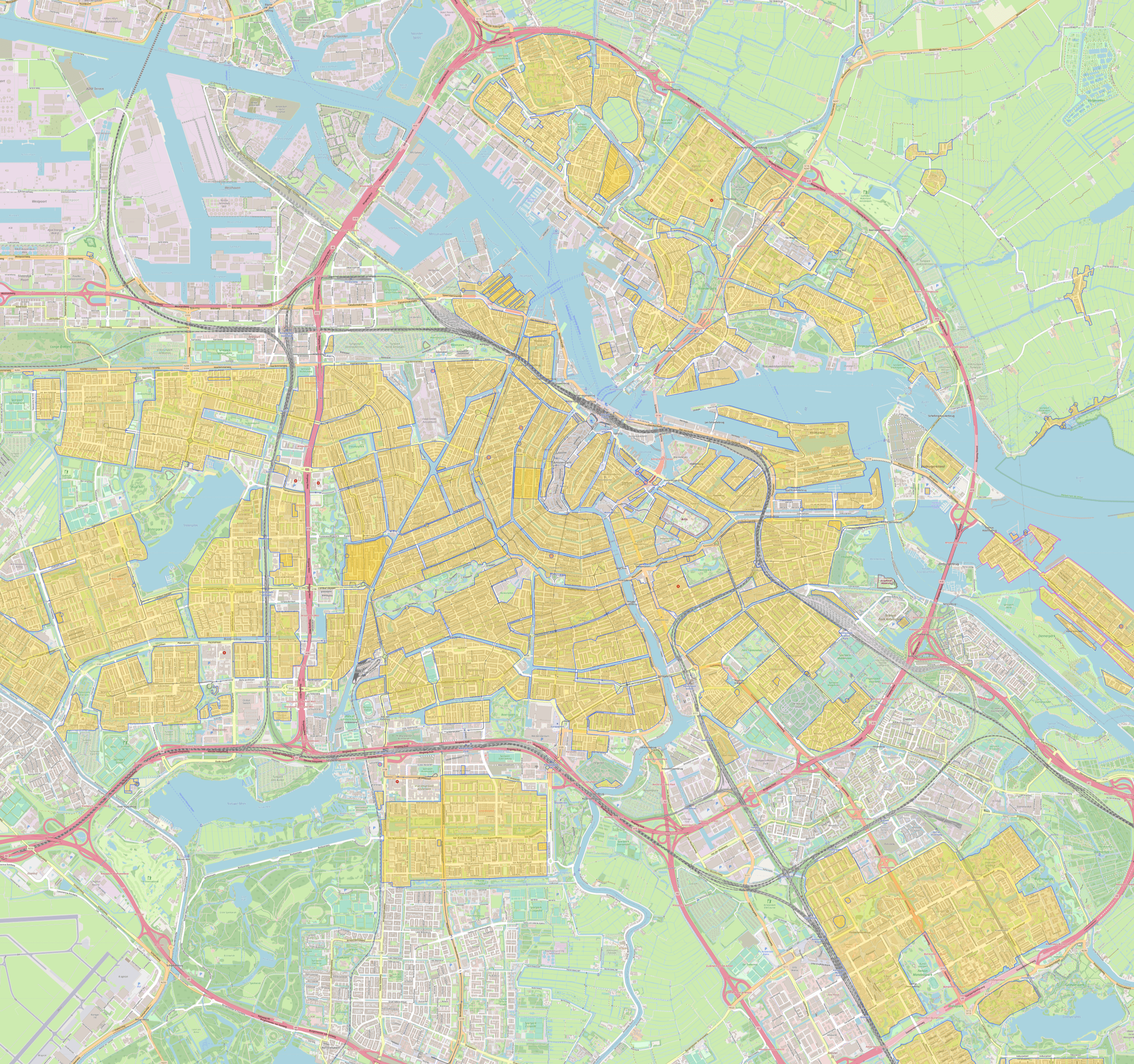\n```py\n>>> residential.keys()\ndict_keys(['nodes', 'ways'])\n\n# No nodes for residential\n>>> residential['nodes']\n{'residential': []}\n\n# Number of residential areas\n>>> residential['ways']['residential']['way_count']\n192\n\n# Total area of residential areas in square kilometers\n>>> residential['ways']['residential']['total_area']\n57.0395957478018\n```\nTo retrieve the first residential area among the 192 areas:\n\n```py\n# Get first residential area\n>>> residential['ways']['residential']['ways'][0]\n{\n 'way_id': 6337974, \n 'tags': {\n 'landuse': 'residential'\n }, \n 'coordinates': [\n (52.3873421, 4.7586896), (52.3873206, 4.7586027), (52.3872219, 4.7584746), \n (52.3866635, 4.75788), (52.3864955, 4.7576667), (52.3863743, 4.757529), \n (52.386242, 4.7574293), (52.3860862, 4.7573364), (52.3858845, 4.7572697), \n (52.385709, 4.757234), (52.385652, 4.7574567), (52.3854924, 4.7581755), \n (52.3851405, 4.7585499), (52.3849747, 4.7587018), (52.3848464, 4.7587884), \n (52.3846829, 4.7588023), (52.3845282, 4.7588045), (52.3844951, 4.7587922), \n (52.3844981, 4.7588234), (52.3845006, 4.7588549), (52.3845013, 4.7588649), \n (52.3845012, 4.7588741), (52.3845008, 4.7588833), (52.384499, 4.7588903), \n (52.3844969, 4.7588936), (52.3844944, 4.7588953), (52.3844892, 4.7589017),\n (52.3844685, 4.7589349), (52.3844671, 4.7589385), (52.3844668, 4.7589431), \n (52.3844451, 4.7589556), (52.3843481, 4.7589603), (52.3842382, 4.7589889),\n (52.3842136, 4.7589874), (52.3841915, 4.7589649), (52.3841589, 4.7588225), \n (52.383, 4.7588), (52.3826, 4.7586), (52.3818, 4.7589), \n (52.3807655, 4.7567289), (52.3801312, 4.7558144), (52.3798, 4.755), \n (52.3808, 4.7532), (52.3816, 4.7514), (52.382, 4.7506), \n (52.3824794, 4.7500095), (52.3826319, 4.7498799), (52.3832, 4.7499), \n (52.3842501, 4.7499516), (52.3842911, 4.7497662), (52.38449, 4.7499965), \n (52.384483, 4.750057), (52.3845537, 4.7501347), (52.3845904, 4.7501106), \n (52.3847177, 4.7500748), (52.3847762, 4.7500795), (52.3848649, 4.7502082),\n (52.3848693, 4.750215), (52.384874, 4.7502226), (52.3849298, 4.7503128), \n (52.3849465, 4.7503394), (52.384978, 4.7503975), (52.3849832, 4.7504213), \n (52.3849919, 4.7504401), (52.3850028, 4.7504572), (52.3850106, 4.7504758), \n (52.3850236, 4.7505078), (52.3850305, 4.7505329), (52.3850346, 4.7505578),\n (52.3850667, 4.7506094), (52.3850853, 4.7506021), (52.3851002, 4.7505518),\n (52.3852322, 4.7506344), (52.3852225, 4.7506759), (52.3852483, 4.7507373),\n (52.3852518, 4.7507611), (52.3852675, 4.750849), (52.3852746, 4.7509073),\n (52.3852804, 4.7509674), (52.3852878, 4.7510422), (52.38529, 4.7510516), \n (52.3853052, 4.7510889), (52.3853194, 4.7511319), (52.3861569, 4.7519683), \n (52.3871396, 4.7527243), (52.3883416, 4.7534281), (52.3881, 4.7549), \n (52.3876, 4.7573), (52.3873421, 4.7586896)\n ], \n 'area': 0.39929878337602165\n}\n```\n\nIn this example, we're only showing residential areas, but landuse can fetch more than just residential areas\u2014it can also fetch forests, commercial areas, industrial areas, farming areas, etc.\n\nFor example:\n\n```py\n# Get total area of forest, meadow and grass in Amsterdam\n# It will take some time\n>>> landuse = lf.landuse(['forest', 'meadow', 'grass'])\n\n# Show keys\n>>> landuse['ways'].keys()\ndict_keys(['forest', 'meadow', 'grass'])\n\n# Forest area in square kilometers\n>>> landuse['ways']['forest']['total_area']\n9.608440158531183\n\n# Meadow area in square kilometers\n>>> landuse['ways']['meadow']['total_area']\n10.776521682770907\n\n# Grass area in square kilometers\n>>> landuse['ways']['grass']['total_area']\n31.5818360868127\n```\n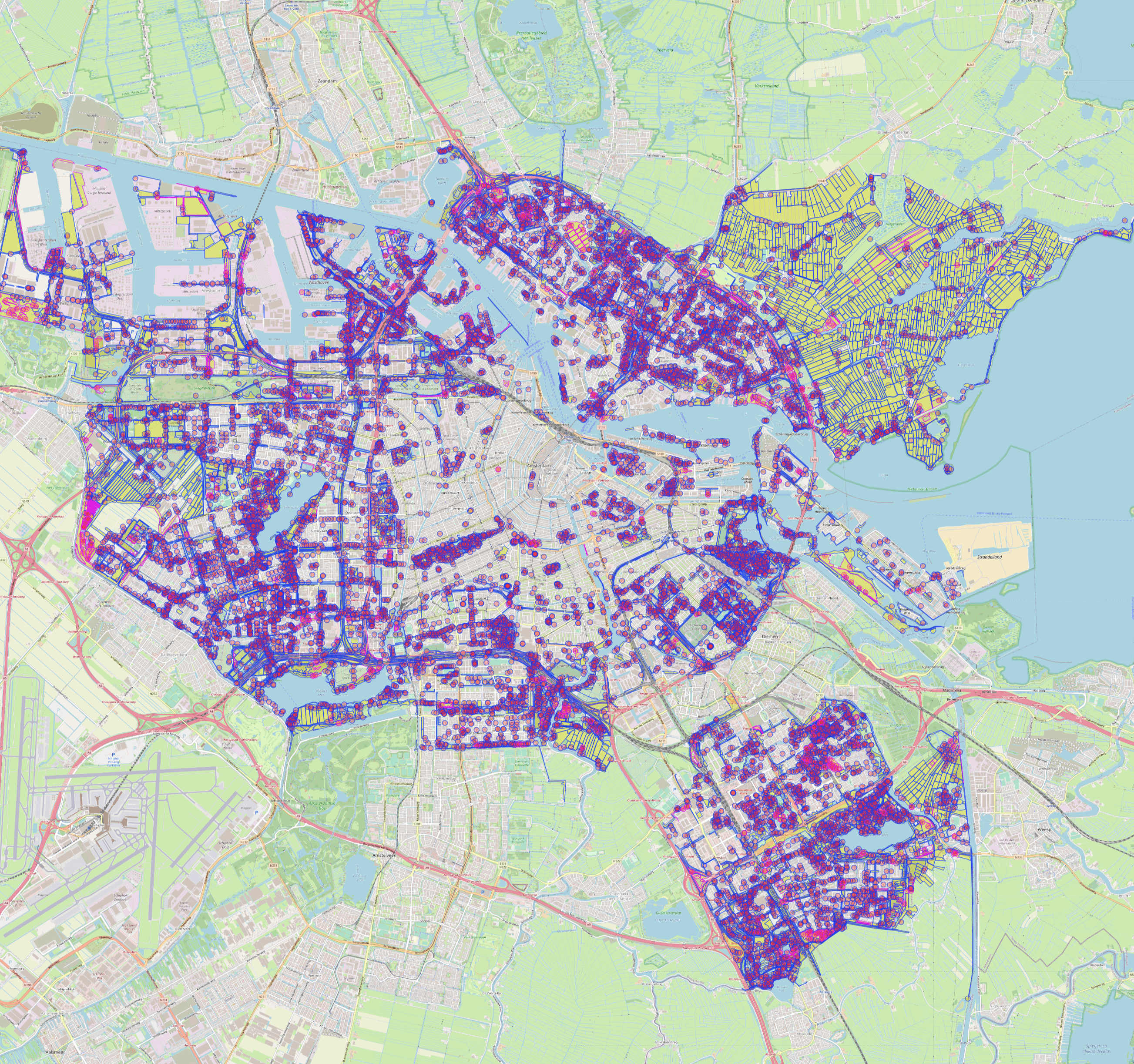\n\n### Tourism\nRetrieves tourism information about the location from the Overpass API.\n```py\n>>> lf = osmlf('Venice, Italy')\n>>> lf\nosmlf(Venezia, Veneto, Italia)\n\n>>> tourism = lf.tourism()\n\n# The iconic Campanile di San Marco\n>>> tourism['ways']['attraction']['ways'][31]\n{\n 'way_id': 252637693,\n 'tags': {\n 'building': 'yes',\n 'height': '98.6',\n 'historic': 'yes',\n 'man_made': 'tower',\n 'name': 'Campanile di San Marco',\n 'name:be': '\u041a\u0430\u043c\u043f\u0430\u043d\u0456\u043b\u0430 \u0441\u0430\u0431\u043e\u0440\u0430 \u0421\u0432\u044f\u0442\u043e\u0433\u0430 \u041c\u0430\u0440\u043a\u0430',\n 'name:ca': 'Campanar de Sant Marc',\n 'name:de': 'Markusturm',\n 'name:en': \"St Mark's Campanile\",\n 'name:eo': 'Kampanilo de Sankta Marko',\n 'name:es': 'Campanile de San Marcos',\n 'name:fr': 'Campanile de Saint-Marc',\n 'name:he': '\u05d4\u05e7\u05de\u05e4\u05e0\u05d9\u05dc\u05d4 \u05e9\u05dc \u05e1\u05df \u05de\u05e8\u05e7\u05d5',\n 'name:it': 'Campanile di San Marco',\n 'name:ja': '\u9418\u697c\uff08\u30b5\u30f3\u30de\u30eb\u30b3\u5e83\u5834\uff09',\n 'name:ko': '\uc0b0\ub9c8\ub974\ucf54\uc758 \uc885\ud0d1',\n 'name:pl': 'Dzwonnica \u015bw. Marka',\n 'name:pt': 'Campan\u00e1rio de S\u00e3o Marcos',\n 'name:ro': 'Campanila San Marco',\n 'name:ru': '\u041a\u0430\u043c\u043f\u0430\u043d\u0438\u043b\u0430 \u0441\u043e\u0431\u043e\u0440\u0430 \u0421\u0432\u044f\u0442\u043e\u0433\u043e \u041c\u0430\u0440\u043a\u0430',\n 'name:th': '\u0e2b\u0e2d\u0e23\u0e30\u0e06\u0e31\u0e07\u0e0b\u0e31\u0e19\u0e21\u0e32\u0e23\u0e4c\u0e42\u0e01',\n 'name:tr': \"Aziz Mark'\u0131n \u00c7an kulesi\",\n 'name:uk': '\u041a\u0430\u043c\u043f\u0430\u043d\u0456\u043b\u0430 \u0441\u043e\u0431\u043e\u0440\u0443 \u0441\u0432\u044f\u0442\u043e\u0433\u043e \u041c\u0430\u0440\u043a\u0430',\n 'name:vec': 'Canpani\u00e8l de San Marco',\n 'name:zh': '\u5723\u9a6c\u53ef\u949f\u697c',\n 'tourism': 'attraction',\n 'tower:construction': 'brick',\n 'tower:height': '95',\n 'tower:type': 'bell_tower',\n 'wheelchair': 'yes',\n 'wikidata': 'Q754194',\n 'wikipedia': 'it:Campanile di San Marco',\n 'wikipedia:fr': 'Campanile de Saint-Marc'\n },\n 'coordinates': [\n (45.4340712, 12.3389421),\n (45.4340844, 12.3389951),\n (45.4340901, 12.3389922),\n (45.434095, 12.3390124),\n (45.4340894, 12.3390152),\n (45.4341083, 12.3390916),\n (45.434001, 12.3391464),\n (45.4339639, 12.338997),\n (45.4340712, 12.3389421)\n ],\n 'area': 0.0001583511784691957\n}\n```\n\nThe data also includes information on hotels. For example, the number of hotels can be retrieved with:\n\n```py\n>>> len(tourism['nodes']['hotel']) # Hotels node object\n328\n\n>>> tourism['ways']['hotel']['way_count'] # Hotels way object\n30\n\n# First element of hotel in nodes\n>>> tourism['nodes']['hotel'][0]\n{\n 'id': 313715870,\n 'tags': {\n 'addr:housenumber': '29',\n 'addr:place': 'Isola Torcello',\n 'addr:postcode': '30142',\n 'amenity': 'restaurant',\n 'check_date:opening_hours': '2022-09-26',\n 'name': 'Locanda Cipriani',\n 'opening_hours:signed': 'no',\n 'tourism': 'hotel'\n },\n 'coordinate': (45.4978252, 12.4179196)\n}\n\n# First element of hotel in ways\n>>> tourism['ways']['hotel']['ways'][0]\n{\n 'way_id': 60996087,\n 'tags': {\n 'addr:city': 'Lido di Venezia',\n 'addr:housenumber': '41',\n 'addr:postcode': '30126',\n 'addr:street': 'Lungomare Guglielmo Marconi',\n 'internet_access': 'wlan',\n 'internet_access:fee': 'no',\n 'name': 'Hotel Excelsior Venice',\n 'stars': '5',\n 'tourism': 'hotel',\n 'wikidata': 'Q1542590'\n },\n 'coordinates': [(\n 45.4045649, 12.3668121),\n (45.4038431, 12.3661057),\n (45.4037498, 12.3662666),\n (45.403303, 12.365826),\n (45.4033521, 12.3656791),\n (45.4032686, 12.3655952),\n (45.4031311, 12.3659029),\n (45.4043489, 12.3671408),\n (45.4044618, 12.3668611),\n (45.4045158, 12.366917),\n (45.4045649, 12.3668121)\n ],\n 'area': 0.004546061610650756\n}\n```\n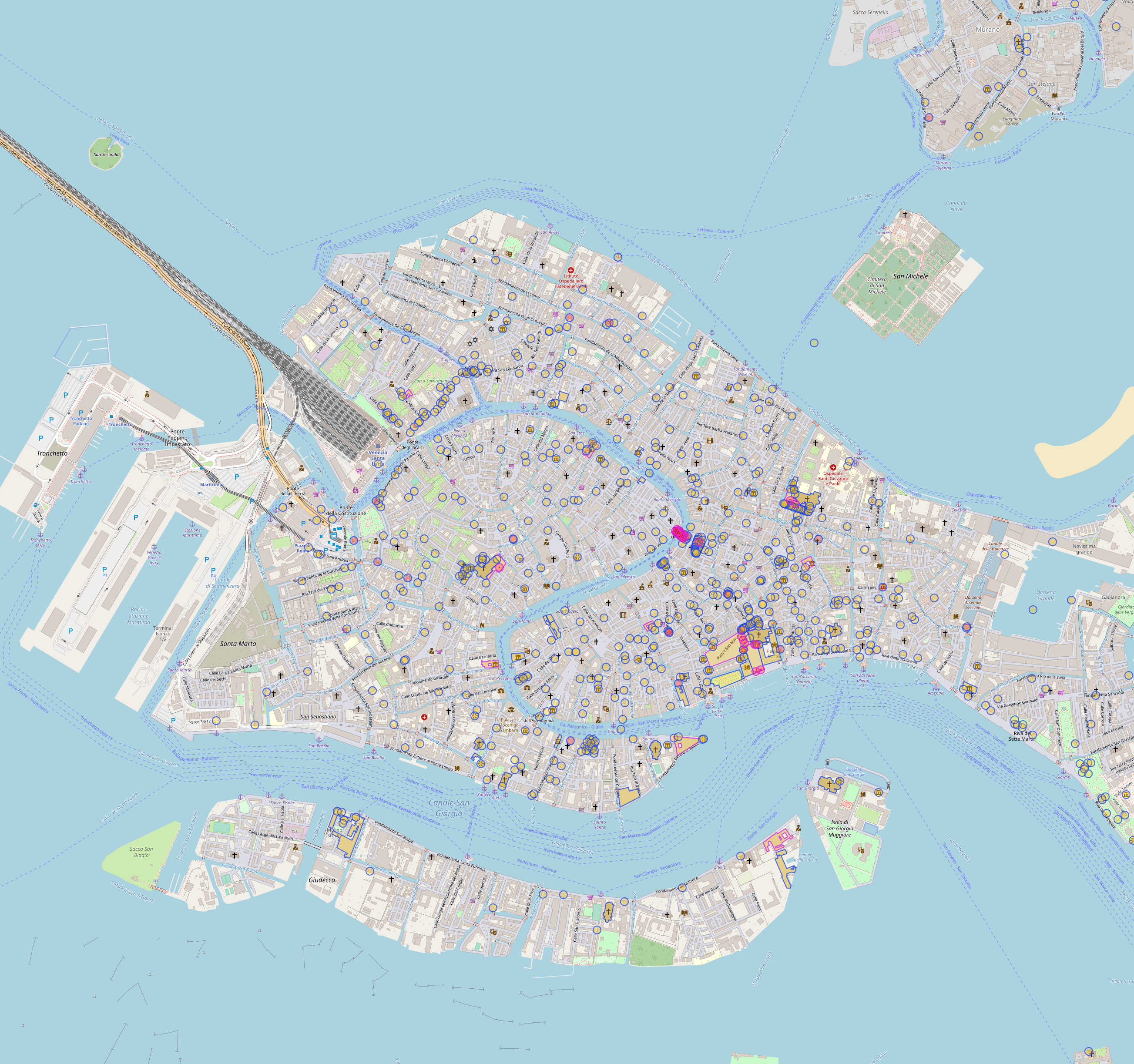\n\n### Natural\nThis function retrieves natural feature information about the location from the Overpass API. The default value for the natural key is beach.\n\n```py\n>>> lf = osmlf('Fethiye, Mugla, Turkey')\n>>> lf\nosmlf(Fethiye, Mu\u011fla, Ege B\u00f6lgesi, T\u00fcrkiye)\n\n>>> natural = lf.natural()\n\n# Number of beaches in Fethiye\n>>> len(natural['nodes']['beach']) # Beaches as node object\n5\n\n# Get the first beach node object\n>>> natural['nodes']['beach'][0]\n{\n 'id': 769903797,\n 'tags': {\n 'fee': 'yes',\n 'name': 'Sun City Beach',\n 'natural': 'beach',\n 'surface': 'sand'\n },\n 'coordinate': (36.5555012, 29.1067139)\n}\n\n# Get the first beach way object\n>>> natural['ways']['beach']['ways'][0]\n{\n 'way_id': 87294614,\n 'tags': {\n 'access': 'yes',\n 'fee': 'yes',\n 'name': 'K\u0131drak Plaj\u0131',\n 'name:en': 'K\u0131drak Beach',\n 'name:ru': '\u041a\u0438\u0434\u0440\u0430\u043a \u043f\u043b\u044f\u0436',\n 'natural': 'beach',\n 'source': 'Bing'\n },\n 'coordinates': [\n (36.5282195, 29.1274236),\n (36.5283159, 29.1275884),\n (36.5286952, 29.1279593),\n (36.5287867, 29.1279884),\n (36.5290436, 29.1280701),\n (36.5297094, 29.1281279),\n (36.5303753, 29.1280027),\n (36.5309869, 29.127757),\n (36.5313553, 29.1277077),\n (36.5313843, 29.1280615),\n (36.5315474, 29.1280792),\n (36.5320484, 29.1279282),\n (36.5321612, 29.1276559),\n (36.5322553, 29.127576),\n (36.5321443, 29.126832),\n (36.5319627, 29.1264458),\n (36.5310326, 29.1270217),\n (36.5299757, 29.1273724),\n (36.529036, 29.1274781),\n (36.5282195, 29.1274236)\n ],\n 'area': 0.030907790857999908\n}\n```\n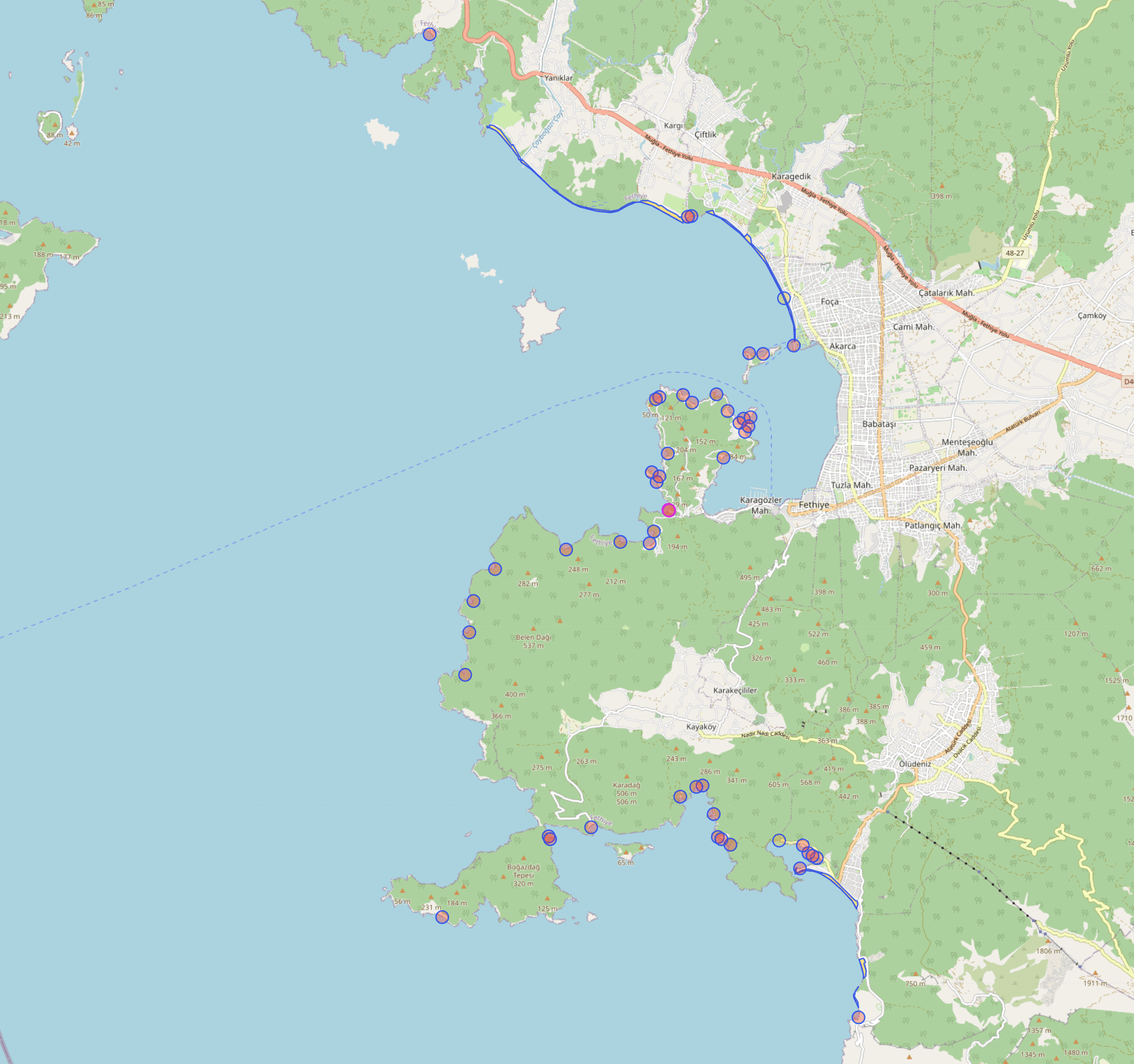\n\n### Highway\nHighways function a bit differently compared to other functions. For instance, unlike the tourism function where we can search for the hotel key, in the highway function we can't find tags by keys because it only lists the items.\n```py\n>>> lf = osmlf('sodermalm, stockholm, sweden')\n>>> lf\nosmlf(S\u00f6dermalm, S\u00f6dermalms stadsdelsomr\u00e5de, Stockholm, Stockholms kommun, Stockholms l\u00e4n, Sverige)\n```\n\nThe total_length key provides the total length of every road listed in info in kilometers.\n\n```py\n# Get highway information\n>>> highway = lf.highway()\n\n>>> highway.keys()\ndict_keys(['total_length', 'info'])\n```\n`total_lenght` is total length of every road in `info` in kilometers\n\n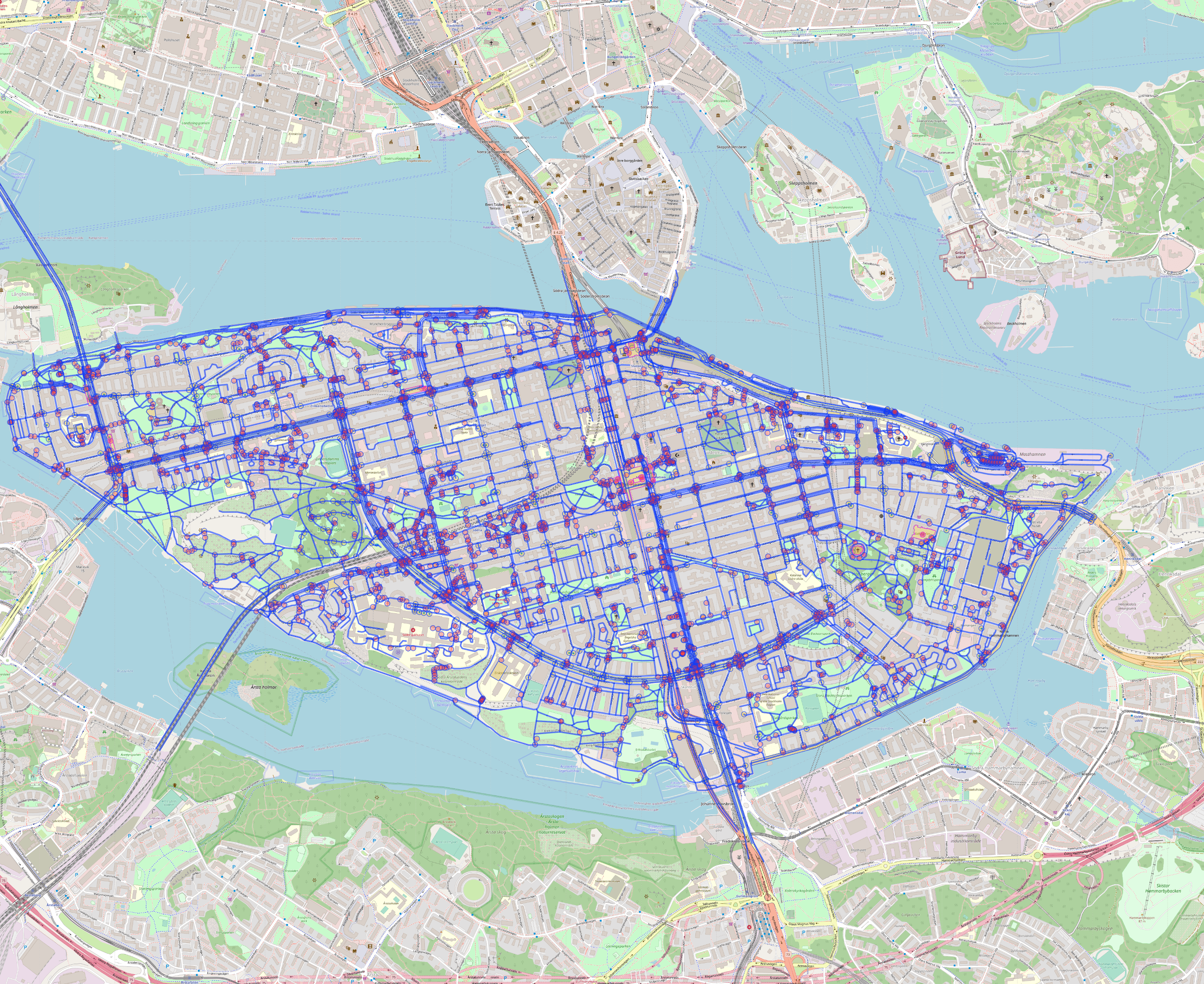\n\n```py\n# Get total length of all roads returned in kilometers\n>>> highway['total_length']\n279.45176280917764\n```\n\nIn the info list, we have details about every road. We can filter by type, such as motorways, pedestrian, residential roads, etc.\n\n```py\n# Get residential roads\n>>> residential = lf.highway('residential')\n\n# Total length of residential\n>>> residential['total_length']\n58.977220710878655\n\n# Get first residential road in list\n>>> residential['info'][0]\n{\n 'tags': {\n 'highway': 'residential',\n 'maxspeed': '30',\n 'name': 'Hornsgatan',\n 'surface': 'asphalt',\n 'wikidata': 'Q3140664',\n 'wikipedia': 'sv:Hornsgatan'\n },\n 'coordinates': [\n (59.3148503, 18.0316059),\n (59.3150171, 18.0318446),\n (59.315038, 18.031897),\n (59.3150498, 18.0319613),\n (59.3152164, 18.0333084)\n ],\n 'length': 0.10962269653879471\n}\n```\n### Railway\nRetrieves railway information about the location from the Overpass API.\n```py\n>>> lf = osmlf('Moscow, Russia')\n>>> lf\nosmlf(\u041c\u043e\u0441\u043a\u0432\u0430, \u0426\u0435\u043d\u0442\u0440\u0430\u043b\u044c\u043d\u044b\u0439 \u0444\u0435\u0434\u0435\u0440\u0430\u043b\u044c\u043d\u044b\u0439 \u043e\u043a\u0440\u0443\u0433, \u0420\u043e\u0441\u0441\u0438\u044f)\n\n>>> railway = lf.railway()\n\n\n>>> railway.keys()\ndict_keys(['nodes', 'ways'])\n\n# Number of stations\n>>> len(railway['nodes']['station'])\n312\n\n# Get first station\n>>> railway['nodes']['station'][0]\n{\n 'id': 26999673,\n 'tags': {\n 'alt_name': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041e\u043a\u0442\u044f\u0431\u0440\u044c\u0441\u043a\u0430\u044f',\n 'esr:user': '060073',\n 'loc_name': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041b\u0435\u043d\u0438\u043d\u0433\u0440\u0430\u0434\u0441\u043a\u0430\u044f',\n 'loc_name:website': 'http://www.tutu.ru/station.php?nnst=79310',\n 'name': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041f\u0430\u0441\u0441\u0430\u0436\u0438\u0440\u0441\u043a\u0430\u044f',\n 'nat_name': '\u041c\u043e\u0441\u043a\u0432\u0430',\n 'network': '\u0420\u0416\u0414',\n 'official_name': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041f\u0430\u0441\u0441\u0430\u0436\u0438\u0440\u0441\u043a\u0430\u044f',\n 'official_name:esr': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041f\u0430\u0441\u0441\u0430\u0436\u0438\u0440\u0441\u043a\u0430\u044f',\n 'official_name:esr:website': 'http://cargo.rzd.ru/cargostation/public/ru?STRUCTURE_ID=5101&layer_id=4829&page4821_2705=1&refererLayerId=4821&id=1090',\n 'official_name:express-3': '\u041c\u043e\u0441\u043a\u0432\u0430-\u041e\u043a\u0442\u044f\u0431\u0440\u044c\u0441\u043a\u0430\u044f',\n 'official_name:express-3:website': 'http://bilet.ru/rus/TrainDirectory.htm?firstsymb=%u041c',\n 'official_name:website': 'http://pass.rzd.ru/timetable/public/ru?STRUCTURE_ID=5104&layer_id=5368&id=278&node_id=19',\n 'operator': '\u041e\u043a\u0442\u044f\u0431\u0440\u044c\u0441\u043a\u0430\u044f \u0436\u0435\u043b\u0435\u0437\u043d\u0430\u044f \u0434\u043e\u0440\u043e\u0433\u0430',\n 'public_transport': 'station',\n 'railway': 'station',\n 'train': 'yes',\n 'uic_name': 'Moskva Oktiabrskaia',\n 'uic_ref': '2006004'\n },\n 'coordinate': (55.7788343, 37.6537207)\n}\n```\n\n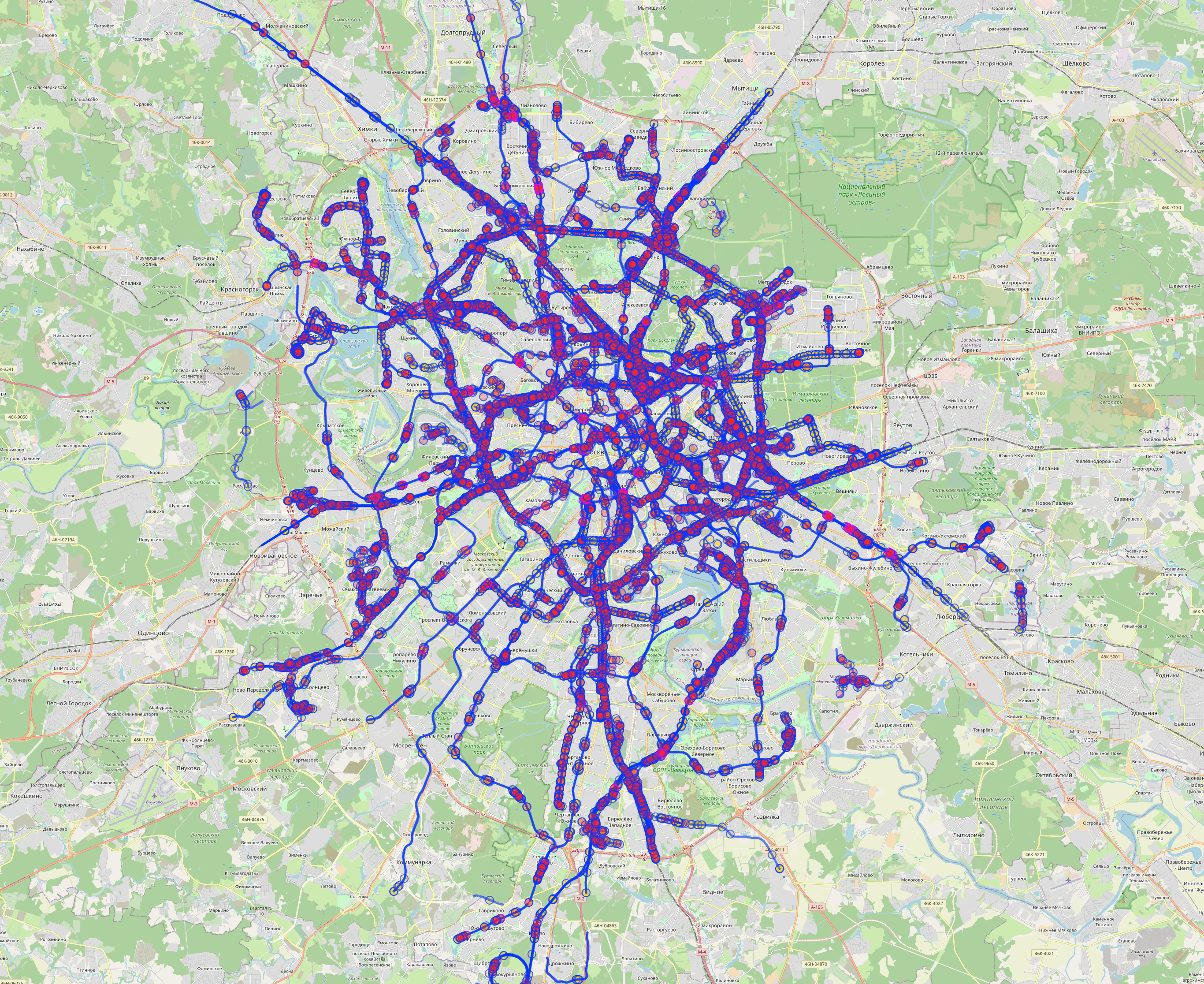\n\n### Waterway\nDoesn't work properly. TODO: Fix it\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Retrieve given location features using OpenStreetMap",
"version": "0.0.1",
"project_urls": {
"Homepage": "https://github.com/ccan23/osmlf"
},
"split_keywords": [
"python",
"map",
"openstreetmap",
"amenities",
"location",
"coordinates",
"latitude",
"longitude"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8669dd0497a7774e6b7fa9cd94c2b35d045d6e4c95211ea507842107c9997f62",
"md5": "920f77f90a06765f837fabd53c02f542",
"sha256": "8c3e83b7ebbb231ce5a5182842089e64b6041ee287b4b1c26cc6ae6421e320e3"
},
"downloads": -1,
"filename": "osmlf-0.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "920f77f90a06765f837fabd53c02f542",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 18054,
"upload_time": "2023-10-25T17:46:57",
"upload_time_iso_8601": "2023-10-25T17:46:57.674560Z",
"url": "https://files.pythonhosted.org/packages/86/69/dd0497a7774e6b7fa9cd94c2b35d045d6e4c95211ea507842107c9997f62/osmlf-0.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0b69ea78b31d0fb97ad6aa63a2937df4732b78e9b31d6811c800a588bed947f3",
"md5": "b45d74fa51256f279b01247b61c2e61b",
"sha256": "a06b93f5098a4f62bb643f64edf729ceca617478261573e5776b89e9258f8539"
},
"downloads": -1,
"filename": "osmlf-0.0.1.tar.gz",
"has_sig": false,
"md5_digest": "b45d74fa51256f279b01247b61c2e61b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 23903,
"upload_time": "2023-10-25T17:47:00",
"upload_time_iso_8601": "2023-10-25T17:47:00.520534Z",
"url": "https://files.pythonhosted.org/packages/0b/69/ea78b31d0fb97ad6aa63a2937df4732b78e9b31d6811c800a588bed947f3/osmlf-0.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-25 17:47:00",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "ccan23",
"github_project": "osmlf",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "osmlf"
}