# packaged
The easiest way to ship python applications.
### Demo apps available on the website: [packaged.live](https://packaged.live)
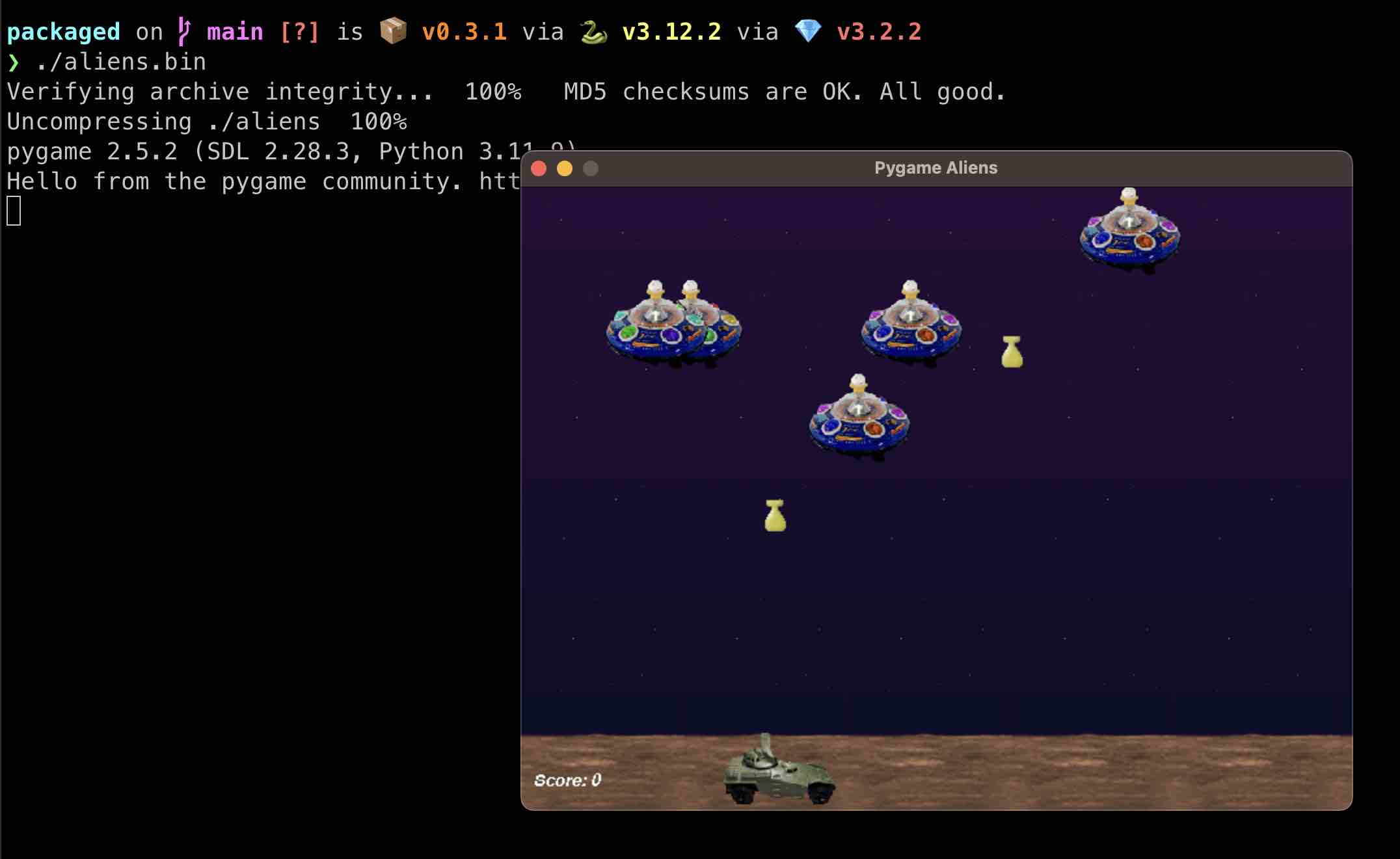
`packaged` can take any Python project, and package it into a self contained
executable, that can run on other machines without needing Python installed.
## Installation
```bash
pip install packaged
```
## Usage
```bash
packaged <output_path> <build_command> <startup_command> [<source_directory>] [--python-version=3.12]
```
Such as:
```bash
packaged my_project.sh 'pip install .' 'python -m your_package' path/to/project
```
This will package Python 3.12 with your application by default.
To specify a different Python version, use the `--python-version` flag, like so:
```bash
packaged my_project.sh 'pip install .' 'python -m your_package' path/to/project --python-version=3.10
```
## Examples
All examples below create a self contained executable. You can send the produced
binary file to another machine with the same OS and architecture, and it will
run the same.
You can also find the pre-built binaries on the [Releases page](https://github.com/tusharsadhwani/packaged/releases/latest).
### Mandelbrot (`numpy`, `matplotlib`, GUI)
```bash
packaged ./mandelbrot.sh 'pip install -r requirements.txt' 'python mandelbrot.py' ./example/mandelbrot --python-version=3.10
```
This produces a `./mandelbrot.sh` binary with:
- Python 3.10
- `matplotlib`
- `numba`
- `llvmlite`
- `pillow`
That outputs an interactive mandelbrot set GUI.
### Minesweeper (using `packaged.toml` for configuration)
You can use a `packaged.toml` file and simply do `packaged path/to/project` to
create your package. For example, try the `minesweeper` project:
```bash
packaged ./example/minesweeper
```
[This configuration](https://github.com/tusharsadhwani/packaged/blob/main/example/minesweeper/packaged.toml)
is used for building the package. The equivalent command to build the project
without `pyproject.toml` would be:
```bash
packaged minesweeper.sh 'pip install .' 'python -m minesweeper' ./example/minesweeper
```
### Textual (TUI) Demo
Since the dependencies themselves contain all the source code needed, you can
skip the last argument. With this, no other files will be packaged other than
what is produced in the build step.
```bash
packaged ./textual.sh 'pip install textual' 'python -m textual'
```
This will simply package the `textual` library's own demo into a single file.
### Aliens (pygame)
Pygame ships with various games as well, `pygame.examples.aliens` is one of them:
```bash
packaged ./aliens 'pip install pygame' 'python -m pygame.examples.aliens'
```
Another one that you can try out is `pygame.examples.chimp`.
### IPython (console scripts)
Packages that expose shell scripts (like `ipython`) should also just work when
creating a package, and these scripts can be used as the startup command:
```bash
packaged ./ipython 'pip install ipython' 'ipython'
```
Now running `./ipython` runs a portable version of IPython!
## Local Development / Testing
To test and modify the package locally:
- Create and activate a virtual environment
- Run `pip install -r requirements-dev.txt` to do an editable install
- Run `pytest` to run tests
- Make changes as needed
### Type Checking
Run `mypy .`
### Create and upload a package to PyPI
Make sure to bump the version in `setup.cfg`.
Then run the following commands:
```bash
rm -rf build dist
python setup.py sdist bdist_wheel
```
Then upload it to PyPI using [twine](https://twine.readthedocs.io/en/latest/#installation):
```bash
twine upload dist/*
```
## License
The package is Licensed under GNU General Public License v2 (GPLv2). However,
note that **the packages created with `packaged` are NOT licensed under GPL**.
This is because the archives created are just data for the package, and
`packaged` is not a part of the archives created.
That means that you can freely use `packaged` for commercial use.
Read the [License section for Makeself](https://github.com/megastep/makeself?tab=readme-ov-file#license) for more information.
Raw data
{
"_id": null,
"home_page": "https://github.com/tusharsadhwani/packaged",
"name": "packaged",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": null,
"author": "Tushar Sadhwani",
"author_email": "tushar.sadhwani000@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/d0/6a/2439d8c1c96e5532aec76cfce6de879a5714884e3f20abd02943e5f338b9/packaged-0.6.0.tar.gz",
"platform": null,
"description": "# packaged\n\nThe easiest way to ship python applications.\n\n### Demo apps available on the website: [packaged.live](https://packaged.live)\n\n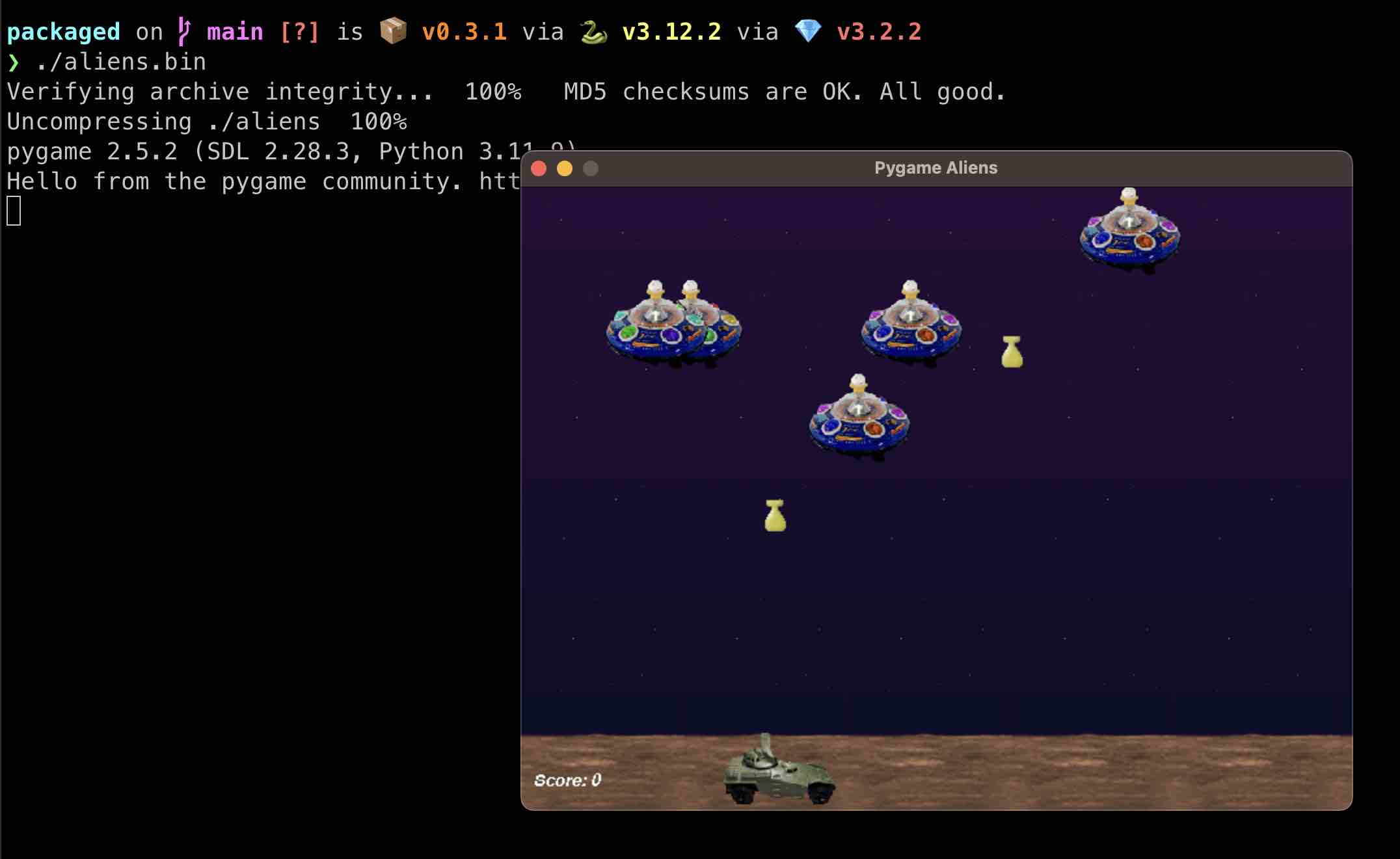\n\n`packaged` can take any Python project, and package it into a self contained\nexecutable, that can run on other machines without needing Python installed.\n\n## Installation\n\n```bash\npip install packaged\n```\n\n## Usage\n\n```bash\npackaged <output_path> <build_command> <startup_command> [<source_directory>] [--python-version=3.12]\n```\n\nSuch as:\n\n```bash\npackaged my_project.sh 'pip install .' 'python -m your_package' path/to/project\n```\n\nThis will package Python 3.12 with your application by default.\n\nTo specify a different Python version, use the `--python-version` flag, like so:\n\n```bash\npackaged my_project.sh 'pip install .' 'python -m your_package' path/to/project --python-version=3.10\n```\n\n## Examples\n\nAll examples below create a self contained executable. You can send the produced\nbinary file to another machine with the same OS and architecture, and it will\nrun the same.\n\nYou can also find the pre-built binaries on the [Releases page](https://github.com/tusharsadhwani/packaged/releases/latest).\n\n### Mandelbrot (`numpy`, `matplotlib`, GUI)\n\n```bash\npackaged ./mandelbrot.sh 'pip install -r requirements.txt' 'python mandelbrot.py' ./example/mandelbrot --python-version=3.10\n```\n\nThis produces a `./mandelbrot.sh` binary with:\n\n- Python 3.10\n- `matplotlib`\n- `numba`\n- `llvmlite`\n- `pillow`\n\nThat outputs an interactive mandelbrot set GUI.\n\n### Minesweeper (using `packaged.toml` for configuration)\n\nYou can use a `packaged.toml` file and simply do `packaged path/to/project` to\ncreate your package. For example, try the `minesweeper` project:\n\n```bash\npackaged ./example/minesweeper\n```\n\n[This configuration](https://github.com/tusharsadhwani/packaged/blob/main/example/minesweeper/packaged.toml)\nis used for building the package. The equivalent command to build the project\nwithout `pyproject.toml` would be:\n\n```bash\npackaged minesweeper.sh 'pip install .' 'python -m minesweeper' ./example/minesweeper\n```\n\n### Textual (TUI) Demo\n\nSince the dependencies themselves contain all the source code needed, you can\nskip the last argument. With this, no other files will be packaged other than\nwhat is produced in the build step.\n\n```bash\npackaged ./textual.sh 'pip install textual' 'python -m textual'\n```\n\nThis will simply package the `textual` library's own demo into a single file.\n\n### Aliens (pygame)\n\nPygame ships with various games as well, `pygame.examples.aliens` is one of them:\n\n```bash\npackaged ./aliens 'pip install pygame' 'python -m pygame.examples.aliens'\n```\n\nAnother one that you can try out is `pygame.examples.chimp`.\n\n### IPython (console scripts)\n\nPackages that expose shell scripts (like `ipython`) should also just work when\ncreating a package, and these scripts can be used as the startup command:\n\n```bash\npackaged ./ipython 'pip install ipython' 'ipython'\n```\n\nNow running `./ipython` runs a portable version of IPython!\n\n## Local Development / Testing\n\nTo test and modify the package locally:\n\n- Create and activate a virtual environment\n- Run `pip install -r requirements-dev.txt` to do an editable install\n- Run `pytest` to run tests\n- Make changes as needed\n\n### Type Checking\n\nRun `mypy .`\n\n### Create and upload a package to PyPI\n\nMake sure to bump the version in `setup.cfg`.\n\nThen run the following commands:\n\n```bash\nrm -rf build dist\npython setup.py sdist bdist_wheel\n```\n\nThen upload it to PyPI using [twine](https://twine.readthedocs.io/en/latest/#installation):\n\n```bash\ntwine upload dist/*\n```\n\n## License\n\nThe package is Licensed under GNU General Public License v2 (GPLv2). However,\nnote that **the packages created with `packaged` are NOT licensed under GPL**.\nThis is because the archives created are just data for the package, and\n`packaged` is not a part of the archives created.\n\nThat means that you can freely use `packaged` for commercial use.\n\nRead the [License section for Makeself](https://github.com/megastep/makeself?tab=readme-ov-file#license) for more information.\n",
"bugtrack_url": null,
"license": "GPL-2.0-or-later",
"summary": "The easiest way to ship python applications.",
"version": "0.6.0",
"project_urls": {
"Homepage": "https://github.com/tusharsadhwani/packaged"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8f3d2fdd16e4886d93f465e14f6f618fba1fc6a0c9edbd94035bd9081a68363c",
"md5": "95fb9e8cbc06737be7dd79507baa001f",
"sha256": "f9d3bd8473d78cdcdb8cd0e5583f76b7fc5c5faacb4cf305a41b01c7392dac77"
},
"downloads": -1,
"filename": "packaged-0.6.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "95fb9e8cbc06737be7dd79507baa001f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 28407,
"upload_time": "2024-06-17T18:34:18",
"upload_time_iso_8601": "2024-06-17T18:34:18.084503Z",
"url": "https://files.pythonhosted.org/packages/8f/3d/2fdd16e4886d93f465e14f6f618fba1fc6a0c9edbd94035bd9081a68363c/packaged-0.6.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d06a2439d8c1c96e5532aec76cfce6de879a5714884e3f20abd02943e5f338b9",
"md5": "fb707d0cc3d178dfe8877f92ae874286",
"sha256": "ab1579a40e4a9d5461357296b1c99f73c9a7585fbe655c0d5f26fae8cdf7b046"
},
"downloads": -1,
"filename": "packaged-0.6.0.tar.gz",
"has_sig": false,
"md5_digest": "fb707d0cc3d178dfe8877f92ae874286",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 27769,
"upload_time": "2024-06-17T18:34:27",
"upload_time_iso_8601": "2024-06-17T18:34:27.210841Z",
"url": "https://files.pythonhosted.org/packages/d0/6a/2439d8c1c96e5532aec76cfce6de879a5714884e3f20abd02943e5f338b9/packaged-0.6.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-17 18:34:27",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tusharsadhwani",
"github_project": "packaged",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"tox": true,
"lcname": "packaged"
}