# panda3d-complexpbr
Functional node level scene shader application for Panda3D. complexpbr supports realtime environment reflections for BSDF materials. These reflections are implemented with IBL (Image-based lighting) and PBR (Physically Based Rendering) forward shading constructs. Your machine must support GLSL version 430 or higher. Sample screenshots below.
Featuring support for vertex displacement mapping, SSAO (Screen Space Ambient Occlusion), SSR (Screen Space Reflections), HSV color correction, Bloom, and Sobel based antialiasing in a screenspace kernel shader, which approximates temporal antialiasing. complexpbr.screenspace_init() automatically enables the AA, SSAO, SSR, and HSV color correction. To use the vertex displacement mapping, provide your displacement map as a shader input to your respective model node -- example below in the Usage section.
By default, the environment reflections dynamically track the camera view. You may set a custom position with the 'env_cam_pos' apply_shader() input variable to IE fix the view to a skybox somewhere on the scene graph. This env_cam_pos variable can be updated live afterwards by setting base.env_cam_pos = Vec3(some_pos). The option to disable or re-enable dynamic reflections is available.
As of version 0.5.2, complexpbr will default to a dummy BRDF LUT which it creates on the fly. complexpbr will remind you that you may create a custom BRDF LUT with the provided 'brdf_lut_calculator.py' script or copy the sample one provided. This feature is automatic, so if you provide the output_brdf_lut.png file in your program directory, it will default to that .png image ignoring the lut_fill input. The sample 'output_brdf_lut.png' and the creation script can be found in the panda3d-complexpbr git repo. For advanced users there is an option to set the LUT image RGB fill values via apply_shader(lut_fill=[r,g,b]) . See Usage section for an example of lut_fill.
As of version 0.5.3, hardware skinning support is provided via complexpbr.skin(your_actor) for models with skeletal animations. See Usage section for an example of hardware skinning.
The goal of this project is to provide extremely easy to use scene shaders to expose the full functionality of Panda3D rendering, including interoperation with CommonFilters and setting shaders on a per-node basis.


7/6/23 Lumberyard Bistro ([Amazon Lumberyard Bistro | NVIDIA Developer](https://developer.nvidia.com/orca/amazon-lumberyard-bistro))



6/1/23 Sponza ([Intel GPU Research Samples](https://www.intel.com/content/www/us/en/developer/topic-technology/graphics-research/samples.html))





## Usage:
```python
from direct.showbase.ShowBase import ShowBase
import complexpbr
class main(ShowBase):
def __init__(self):
super().__init__()
# apply a scene shader with PBR IBL
# node can be base.render or any model node, intensity is the desired AO
# (ambient occlusion reflection) intensity (float, 0.0 to 1.0)
# you may wish to define a specific position in your scene where the
# cube map is rendered from, to IE have multiple skyboxes preloaded
# somewhere on the scene graph and have their reflections map to your
# models -- to achieve this, set env_cam_pos=Vec3(your_pos)
# you may set base.env_cam_pos after this, and it will update in realtime
# env_res is the cube map resolution, can only be set once upon first call
complexpbr.apply_shader(self.render)
# complexpbr.screenspace_init() # optional, starts the screenspace effects
# apply_shader() with optional inputs
# complexpbr.apply_shader(self.render, intensity=0.9, env_cam_pos=None, env_res=256, lut_fill=[1.0,0.0,0.0])
# initialize complexpbr's screenspace effects (SSAO, SSR, AA, HSV color correction)
# this replaces CommonFilters functionality
complexpbr.screenspace_init()
# make the cubemap rendering static (performance boost)
complexpbr.set_cubebuff_inactive()
# make the cubemap rendering dynamic (this is the default state)
complexpbr.set_cubebuff_active()
# example of how to apply hardware skinning
fp_character = actor_data.player_character # this is an Actor() model
fp_character.reparent_to(self.render)
fp_character.set_scale(1)
# set hardware skinning for the Actor()
complexpbr.skin(fp_character)
# example of how to use the vertex displacement mapping
wood_sphere_3 = loader.load_model('assets/models/wood_sphere_3.gltf')
wood_sphere_3.reparent_to(base.render)
wood_sphere_3.set_pos(0,0,1)
dis_tex = Texture()
dis_tex.read('assets/textures/WoodFloor057_2K-PNG/WoodFloor057_2K_Displacement.png')
wood_sphere_3.set_shader_input('displacement_map', dis_tex)
wood_sphere_3.set_shader_input('displacement_scale', 0.1)
# example of how to set up bloom -- complexpbr.screenspace_init() must have been called first
screen_quad = base.screen_quad
bloom_intensity = 5.0 # bloom defaults to 0.0 / off
bloom_blur_width = 10
bloom_samples = 6
bloom_threshold = 0.7
screen_quad.set_shader_input("bloom_intensity", bloom_intensity)
screen_quad.set_shader_input("bloom_threshold", bloom_threshold)
screen_quad.set_shader_input("bloom_blur_width", bloom_blur_width)
screen_quad.set_shader_input("bloom_samples", bloom_samples)
# example of how to customize SSR
ssr_intensity = 0.5
ssr_step = 4.0
ssr_fresnel_pow = 3.0
ssr_samples = 128 # ssr_samples defaults to 0 / off
screen_quad.set_shader_input("ssr_intensity", ssr_intensity)
screen_quad.set_shader_input("ssr_step", ssr_step)
screen_quad.set_shader_input("ssr_fresnel_pow", ssr_fresnel_pow)
screen_quad.set_shader_input("ssr_samples", ssr_samples)
# example of how to customize SSAO
ssao_samples = 32 # ssao_samples defaults to 8
screen_quad.set_shader_input("ssao_samples", ssao_samples)
# example of how to HSV adjust the final image
screen_quad.set_shader_input("hsv_g", 1.3) # hsv_g (saturation factor) defaults to 1.0
# example of how to modify the specular contribution
self.render.set_shader_input("specular_factor", 10.0) # the specular_factor defaults to 1.0
# example of how to directly fill your BRDF LUT texture instead of providing one in your game folder
complexpbr.apply_shader(base.render, 1.0, env_res=1024, lut_fill=[1.0,0.0,0.0]) # lut_fill=[red, green, blue]
# if complexpbr.screenspace_init() has not been called, you may use CommonFilters
# scene_filters = CommonFilters(base.win, base.cam)
# scene_filters.set_bloom(size='medium')
# scene_filters.set_exposure_adjust(1.1)
# scene_filters.set_gamma_adjust(1.1)
# scene_filters.set_blur_sharpen(0.9)
```
## Building:
The module may be built using setuptools.
```bash
python3 setup.py bdist_wheel
```
```bash
pip3 install 'path/to/panda3d-complexpbr.whl'
```
## Installing with PyPI:
To-do.
## Future Project Goals:
- Function triggers for building new BRDF LUT samplers in realtime
- Installation over pip
## Requirements:
- panda3d





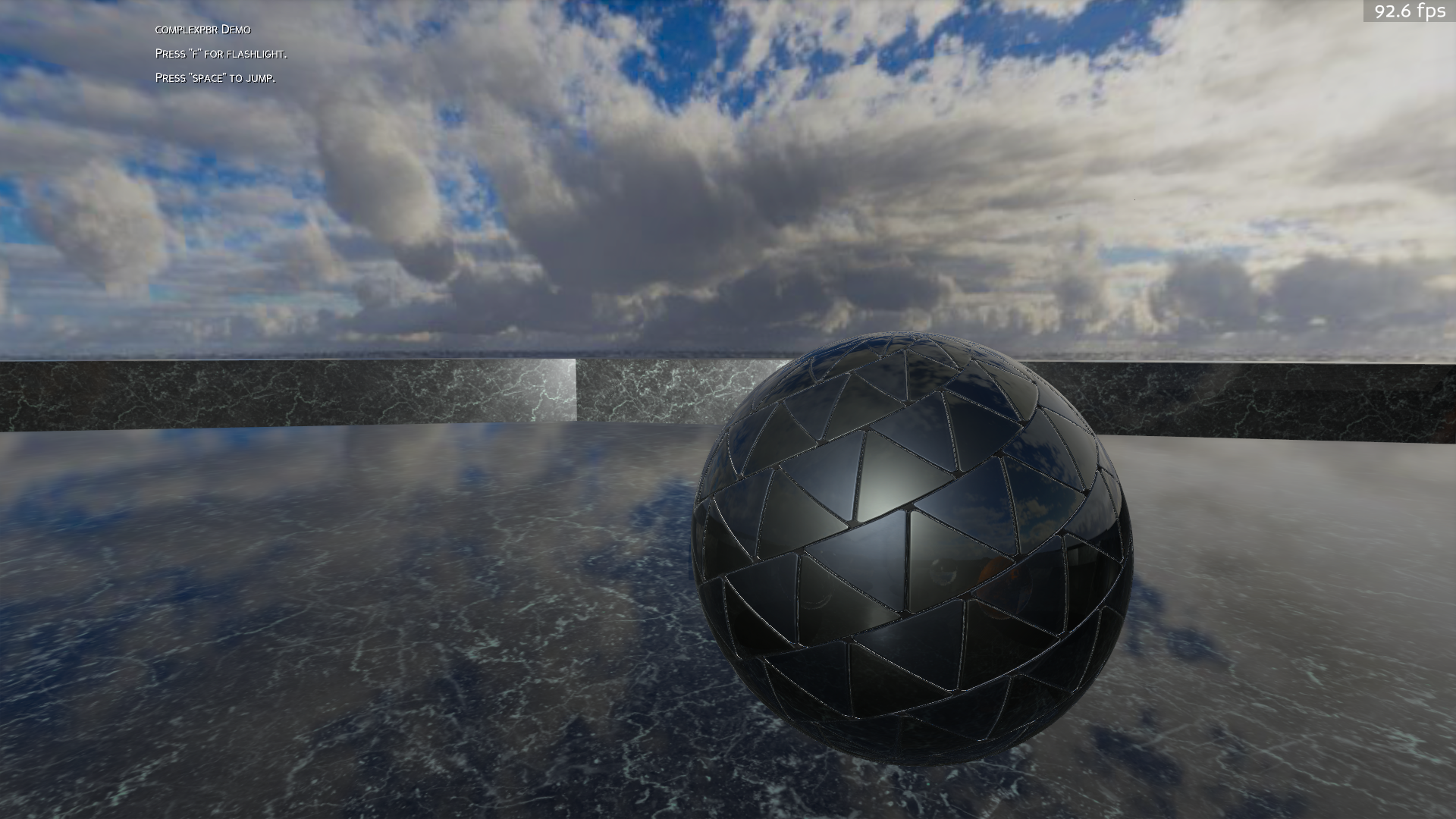
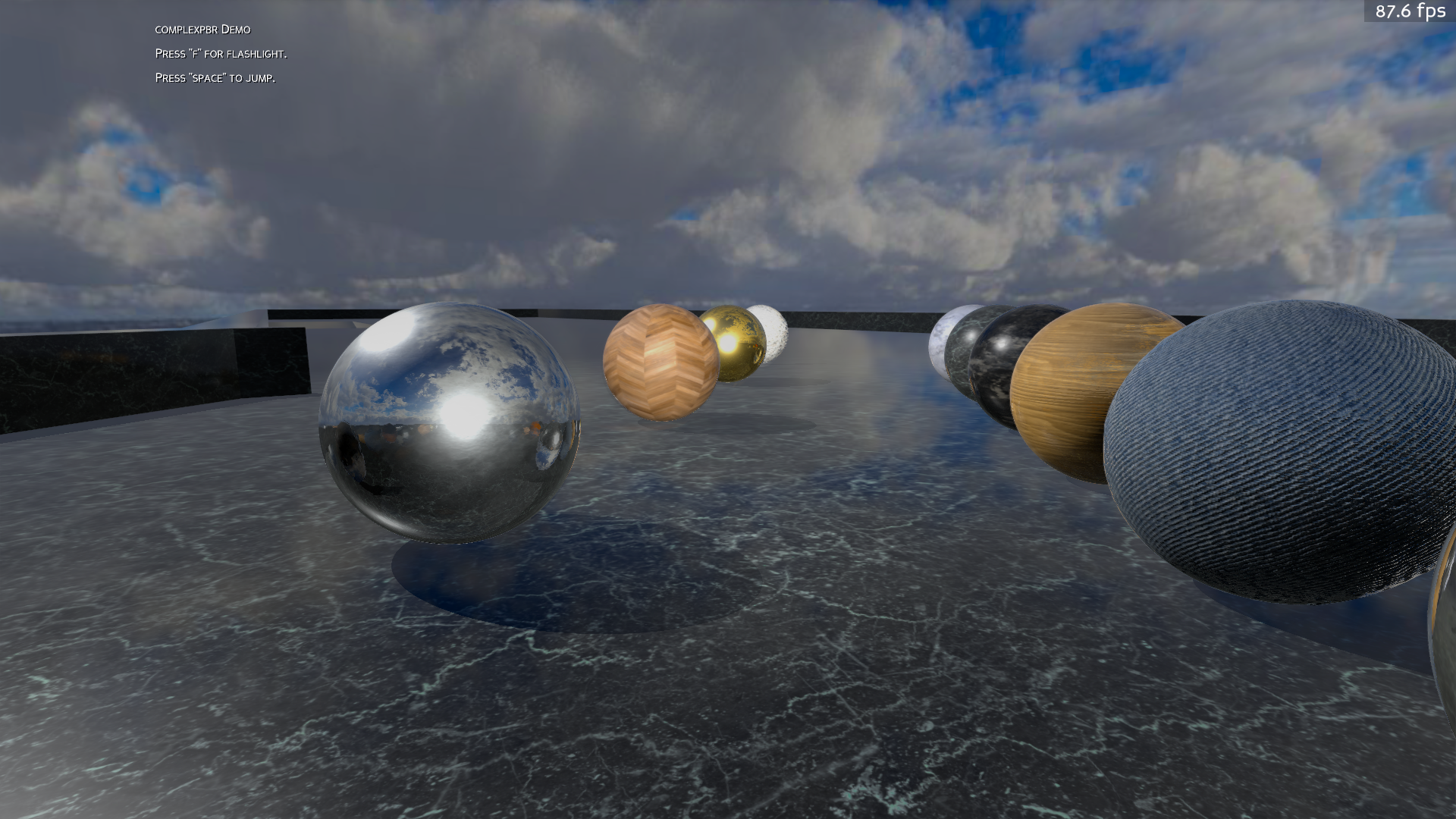
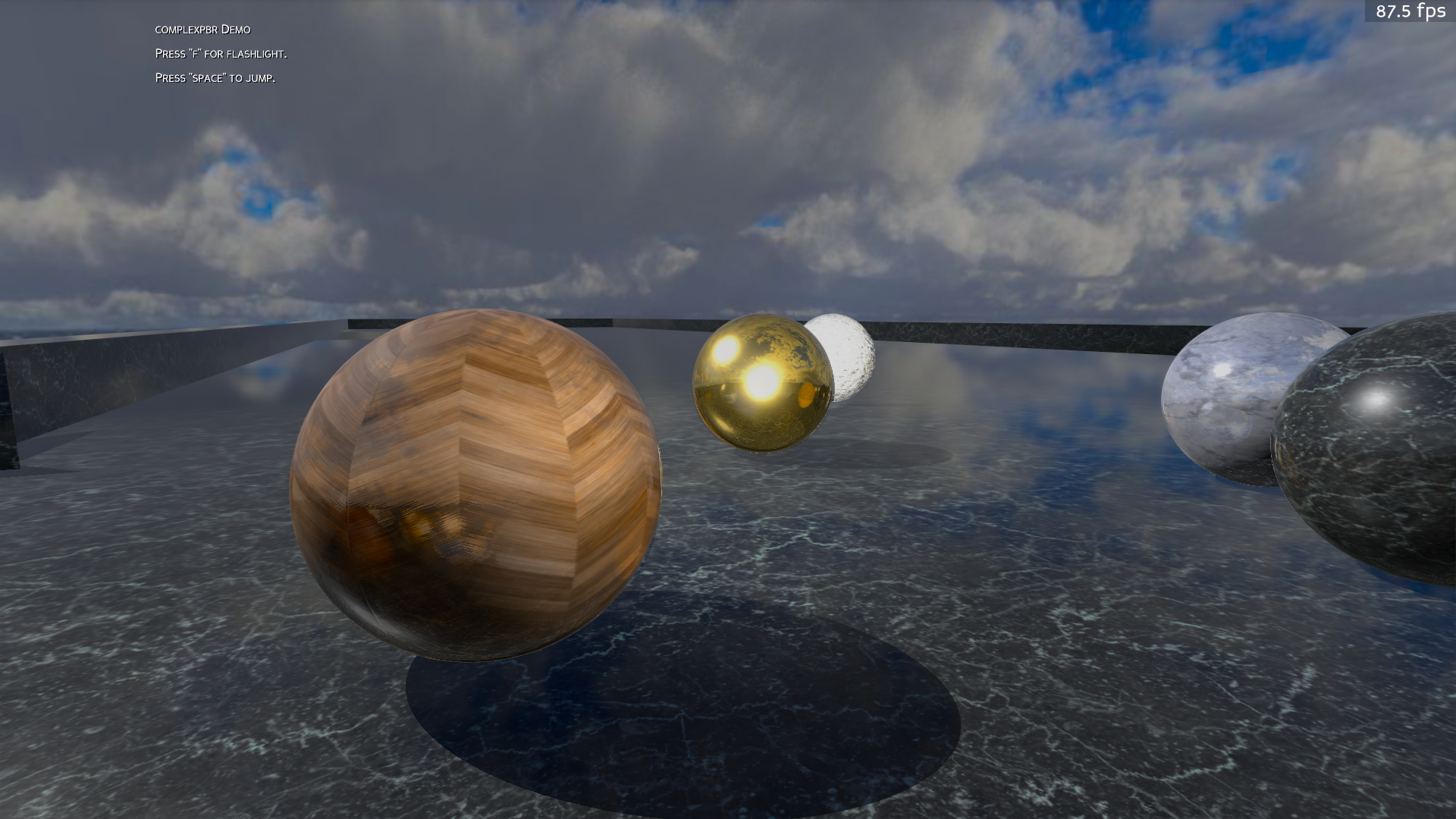
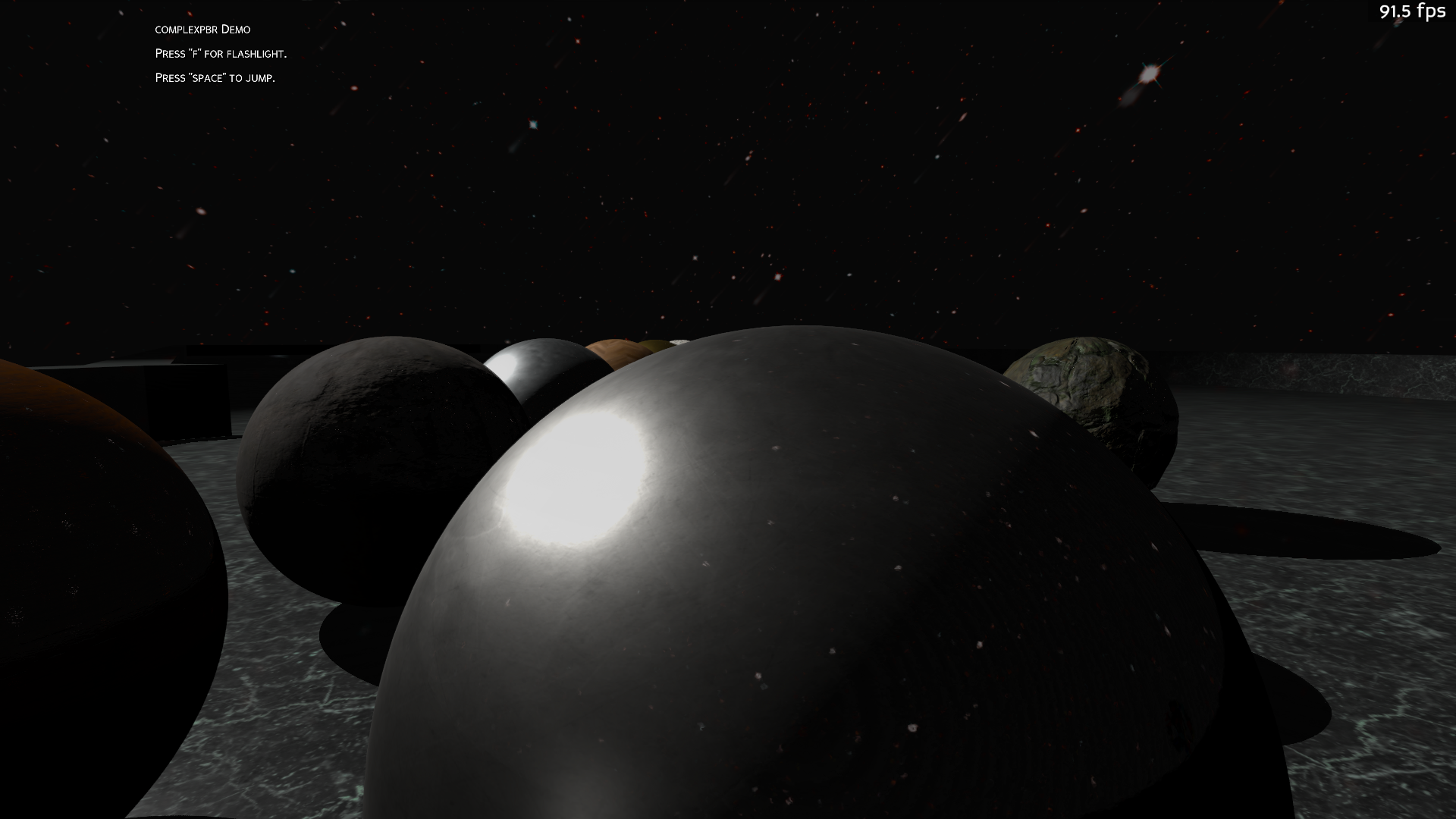
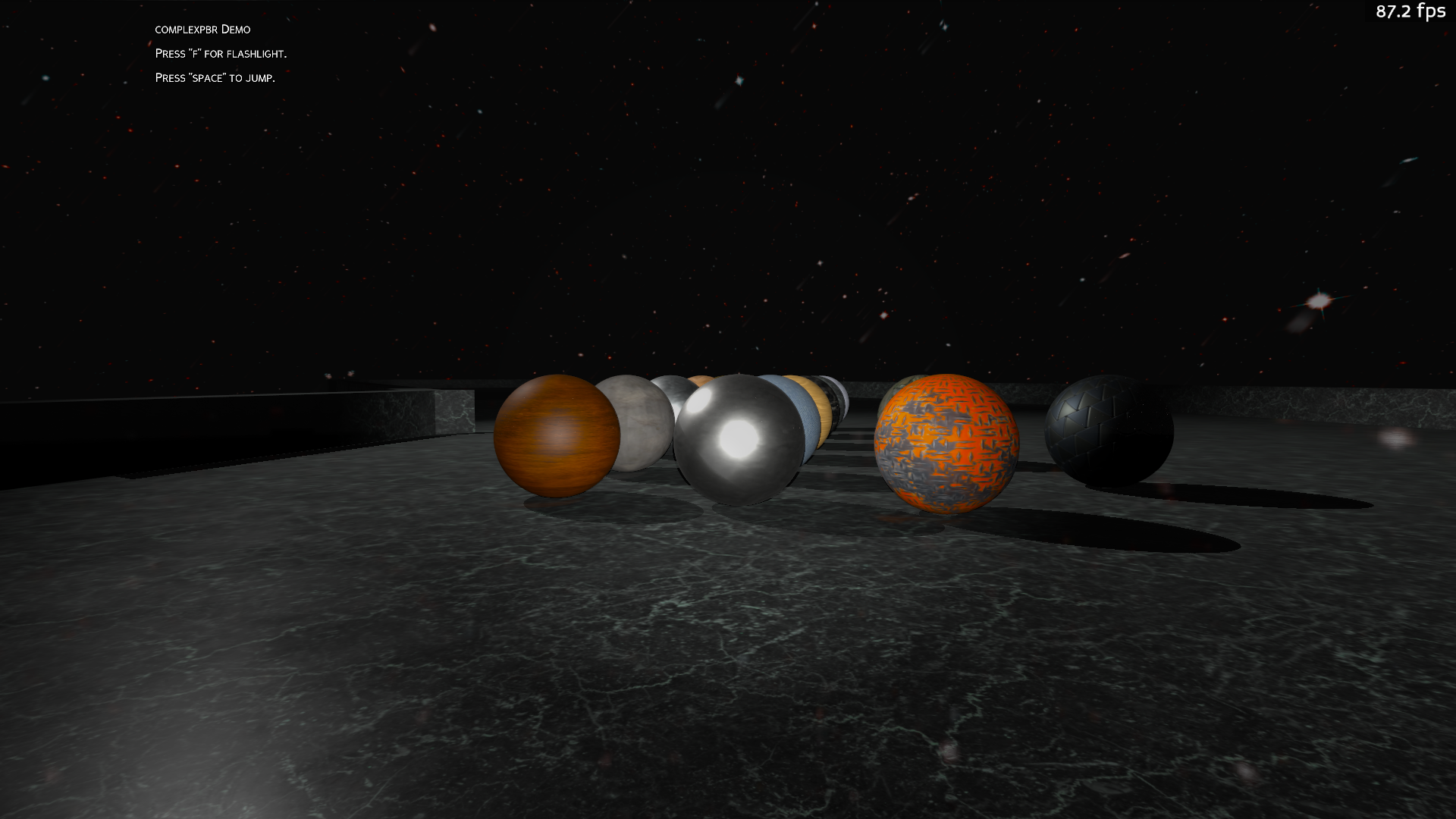
Vertex Displacement Mapping:
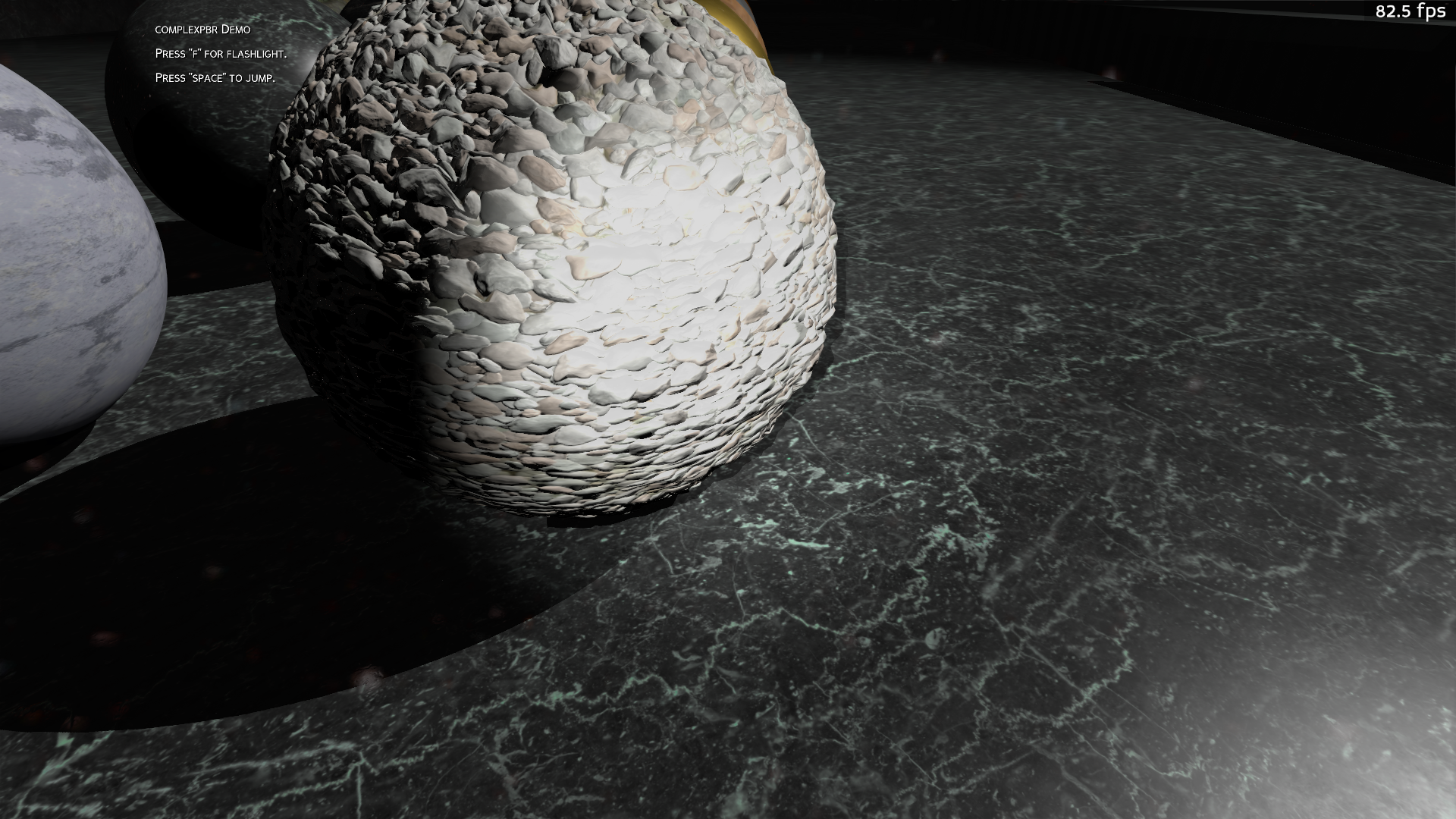
Raw data
{
"_id": null,
"home_page": "",
"name": "panda3d-complexpbr",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "panda3d,gamedev,pbr",
"author": "Logan Bier",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/4f/be/1429d10d0edb7d5689b276fb03809cebb309800364aa98351c03e58de4a7/panda3d-complexpbr-0.5.3.tar.gz",
"platform": null,
"description": "# panda3d-complexpbr\r\nFunctional node level scene shader application for Panda3D. complexpbr supports realtime environment reflections for BSDF materials. These reflections are implemented with IBL (Image-based lighting) and PBR (Physically Based Rendering) forward shading constructs. Your machine must support GLSL version 430 or higher. Sample screenshots below.\r\n\r\nFeaturing support for vertex displacement mapping, SSAO (Screen Space Ambient Occlusion), SSR (Screen Space Reflections), HSV color correction, Bloom, and Sobel based antialiasing in a screenspace kernel shader, which approximates temporal antialiasing. complexpbr.screenspace_init() automatically enables the AA, SSAO, SSR, and HSV color correction. To use the vertex displacement mapping, provide your displacement map as a shader input to your respective model node -- example below in the Usage section.\r\n\r\nBy default, the environment reflections dynamically track the camera view. You may set a custom position with the 'env_cam_pos' apply_shader() input variable to IE fix the view to a skybox somewhere on the scene graph. This env_cam_pos variable can be updated live afterwards by setting base.env_cam_pos = Vec3(some_pos). The option to disable or re-enable dynamic reflections is available. \r\n\r\nAs of version 0.5.2, complexpbr will default to a dummy BRDF LUT which it creates on the fly. complexpbr will remind you that you may create a custom BRDF LUT with the provided 'brdf_lut_calculator.py' script or copy the sample one provided. This feature is automatic, so if you provide the output_brdf_lut.png file in your program directory, it will default to that .png image ignoring the lut_fill input. The sample 'output_brdf_lut.png' and the creation script can be found in the panda3d-complexpbr git repo. For advanced users there is an option to set the LUT image RGB fill values via apply_shader(lut_fill=[r,g,b]) . See Usage section for an example of lut_fill.\r\n\r\nAs of version 0.5.3, hardware skinning support is provided via complexpbr.skin(your_actor) for models with skeletal animations. See Usage section for an example of hardware skinning.\r\n\r\nThe goal of this project is to provide extremely easy to use scene shaders to expose the full functionality of Panda3D rendering, including interoperation with CommonFilters and setting shaders on a per-node basis.\r\n\r\n\r\n \r\n\r\n\r\n7/6/23 Lumberyard Bistro ([Amazon Lumberyard Bistro | NVIDIA Developer](https://developer.nvidia.com/orca/amazon-lumberyard-bistro))\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n6/1/23 Sponza ([Intel GPU Research Samples](https://www.intel.com/content/www/us/en/developer/topic-technology/graphics-research/samples.html))\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n## Usage:\r\n```python\r\nfrom direct.showbase.ShowBase import ShowBase\r\nimport complexpbr\r\n\r\nclass main(ShowBase):\r\n def __init__(self):\r\n super().__init__()\r\n \r\n # apply a scene shader with PBR IBL\r\n # node can be base.render or any model node, intensity is the desired AO\r\n # (ambient occlusion reflection) intensity (float, 0.0 to 1.0)\r\n # you may wish to define a specific position in your scene where the \r\n # cube map is rendered from, to IE have multiple skyboxes preloaded\r\n # somewhere on the scene graph and have their reflections map to your\r\n # models -- to achieve this, set env_cam_pos=Vec3(your_pos)\r\n # you may set base.env_cam_pos after this, and it will update in realtime\r\n # env_res is the cube map resolution, can only be set once upon first call\r\n \r\n complexpbr.apply_shader(self.render)\r\n # complexpbr.screenspace_init() # optional, starts the screenspace effects\r\n \r\n # apply_shader() with optional inputs\r\n # complexpbr.apply_shader(self.render, intensity=0.9, env_cam_pos=None, env_res=256, lut_fill=[1.0,0.0,0.0])\r\n\r\n # initialize complexpbr's screenspace effects (SSAO, SSR, AA, HSV color correction)\r\n # this replaces CommonFilters functionality\r\n complexpbr.screenspace_init()\r\n \r\n # make the cubemap rendering static (performance boost)\r\n complexpbr.set_cubebuff_inactive()\r\n \r\n # make the cubemap rendering dynamic (this is the default state)\r\n complexpbr.set_cubebuff_active()\r\n\r\n # example of how to apply hardware skinning\r\n fp_character = actor_data.player_character # this is an Actor() model\r\n fp_character.reparent_to(self.render)\r\n fp_character.set_scale(1)\r\n # set hardware skinning for the Actor()\r\n complexpbr.skin(fp_character)\r\n\r\n # example of how to use the vertex displacement mapping\r\n wood_sphere_3 = loader.load_model('assets/models/wood_sphere_3.gltf')\r\n wood_sphere_3.reparent_to(base.render)\r\n wood_sphere_3.set_pos(0,0,1)\r\n dis_tex = Texture()\r\n dis_tex.read('assets/textures/WoodFloor057_2K-PNG/WoodFloor057_2K_Displacement.png')\r\n wood_sphere_3.set_shader_input('displacement_map', dis_tex)\r\n wood_sphere_3.set_shader_input('displacement_scale', 0.1)\r\n \r\n # example of how to set up bloom -- complexpbr.screenspace_init() must have been called first\r\n screen_quad = base.screen_quad\r\n \r\n bloom_intensity = 5.0 # bloom defaults to 0.0 / off\r\n bloom_blur_width = 10\r\n bloom_samples = 6\r\n bloom_threshold = 0.7\r\n\r\n screen_quad.set_shader_input(\"bloom_intensity\", bloom_intensity)\r\n screen_quad.set_shader_input(\"bloom_threshold\", bloom_threshold)\r\n screen_quad.set_shader_input(\"bloom_blur_width\", bloom_blur_width)\r\n screen_quad.set_shader_input(\"bloom_samples\", bloom_samples)\r\n \r\n # example of how to customize SSR\r\n ssr_intensity = 0.5 \r\n ssr_step = 4.0\r\n ssr_fresnel_pow = 3.0\r\n ssr_samples = 128 # ssr_samples defaults to 0 / off\r\n \r\n screen_quad.set_shader_input(\"ssr_intensity\", ssr_intensity)\r\n screen_quad.set_shader_input(\"ssr_step\", ssr_step)\r\n screen_quad.set_shader_input(\"ssr_fresnel_pow\", ssr_fresnel_pow)\r\n screen_quad.set_shader_input(\"ssr_samples\", ssr_samples)\r\n \r\n # example of how to customize SSAO\r\n ssao_samples = 32 # ssao_samples defaults to 8\r\n \r\n screen_quad.set_shader_input(\"ssao_samples\", ssao_samples)\r\n \r\n # example of how to HSV adjust the final image\r\n screen_quad.set_shader_input(\"hsv_g\", 1.3) # hsv_g (saturation factor) defaults to 1.0\r\n \r\n # example of how to modify the specular contribution\r\n self.render.set_shader_input(\"specular_factor\", 10.0) # the specular_factor defaults to 1.0\r\n \r\n # example of how to directly fill your BRDF LUT texture instead of providing one in your game folder\r\n complexpbr.apply_shader(base.render, 1.0, env_res=1024, lut_fill=[1.0,0.0,0.0]) # lut_fill=[red, green, blue]\r\n \r\n # if complexpbr.screenspace_init() has not been called, you may use CommonFilters\r\n # scene_filters = CommonFilters(base.win, base.cam)\r\n # scene_filters.set_bloom(size='medium')\r\n # scene_filters.set_exposure_adjust(1.1)\r\n # scene_filters.set_gamma_adjust(1.1)\r\n # scene_filters.set_blur_sharpen(0.9)\r\n```\r\n## Building:\r\n\r\nThe module may be built using setuptools. \r\n```bash\r\npython3 setup.py bdist_wheel\r\n```\r\n```bash\r\npip3 install 'path/to/panda3d-complexpbr.whl'\r\n```\r\n## Installing with PyPI:\r\n\r\nTo-do.\r\n\r\n## Future Project Goals:\r\n- Function triggers for building new BRDF LUT samplers in realtime\r\n- Installation over pip\r\n\r\n## Requirements:\r\n\r\n- panda3d\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n\r\n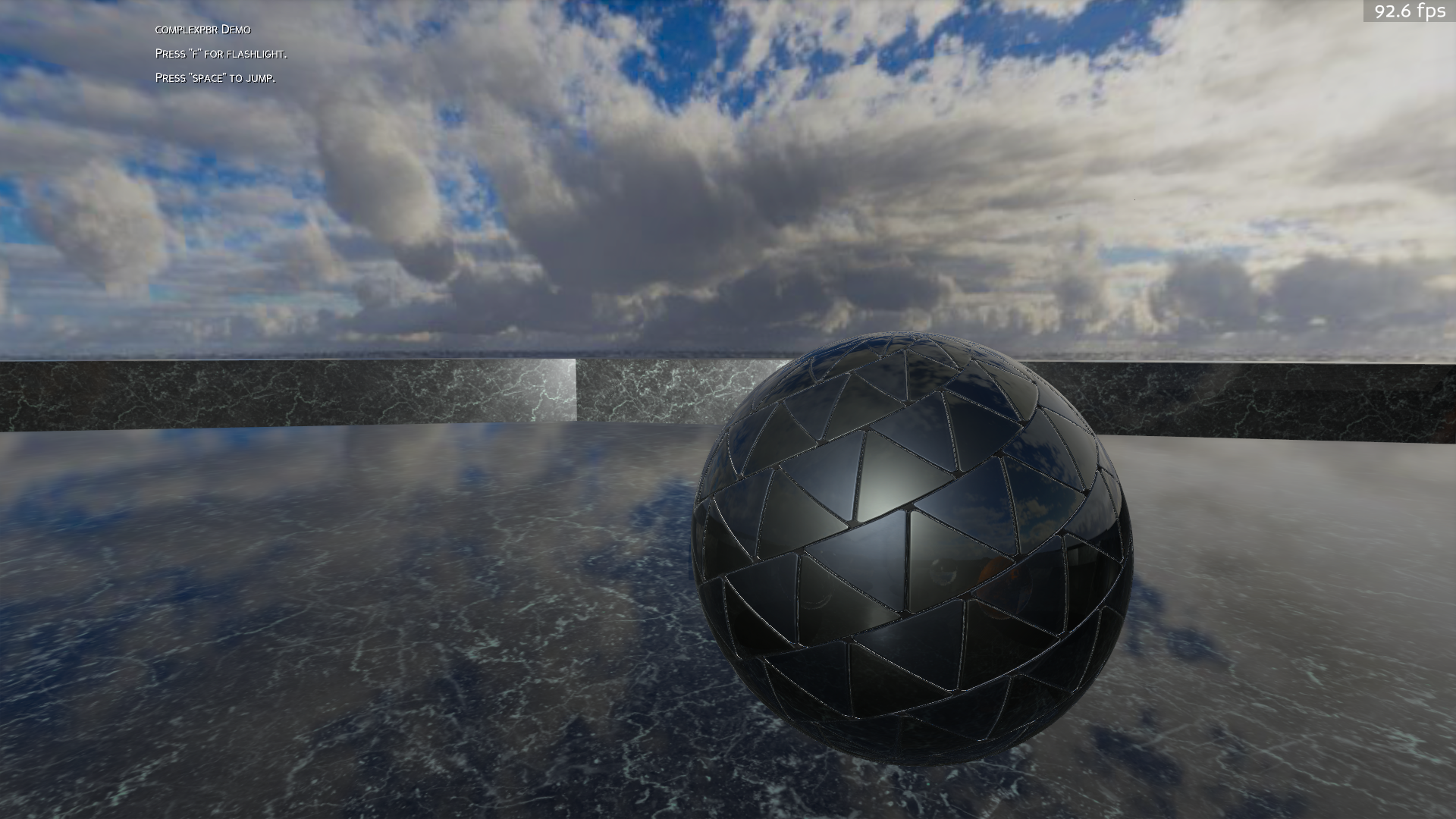\r\n\r\n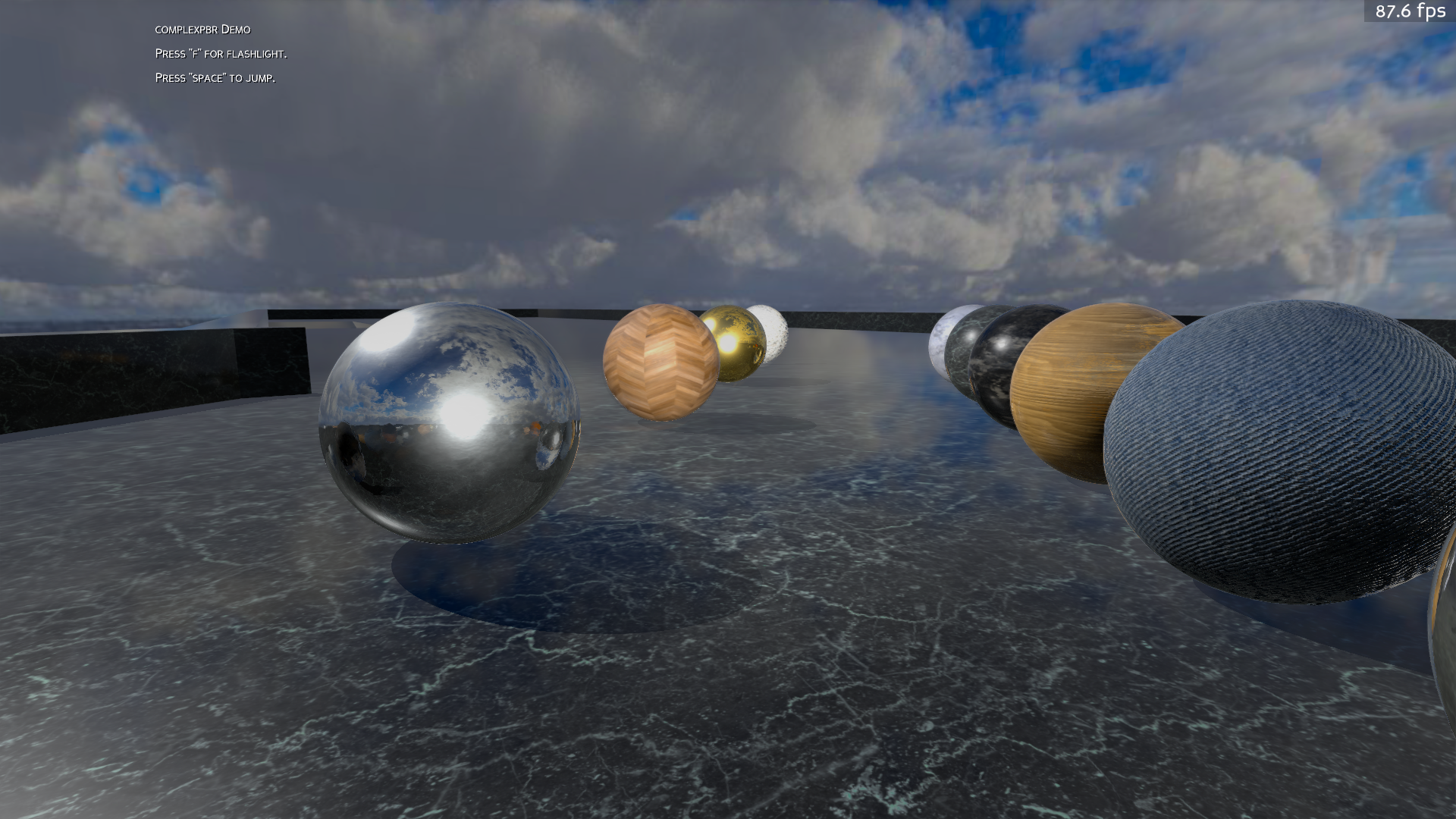\r\n\r\n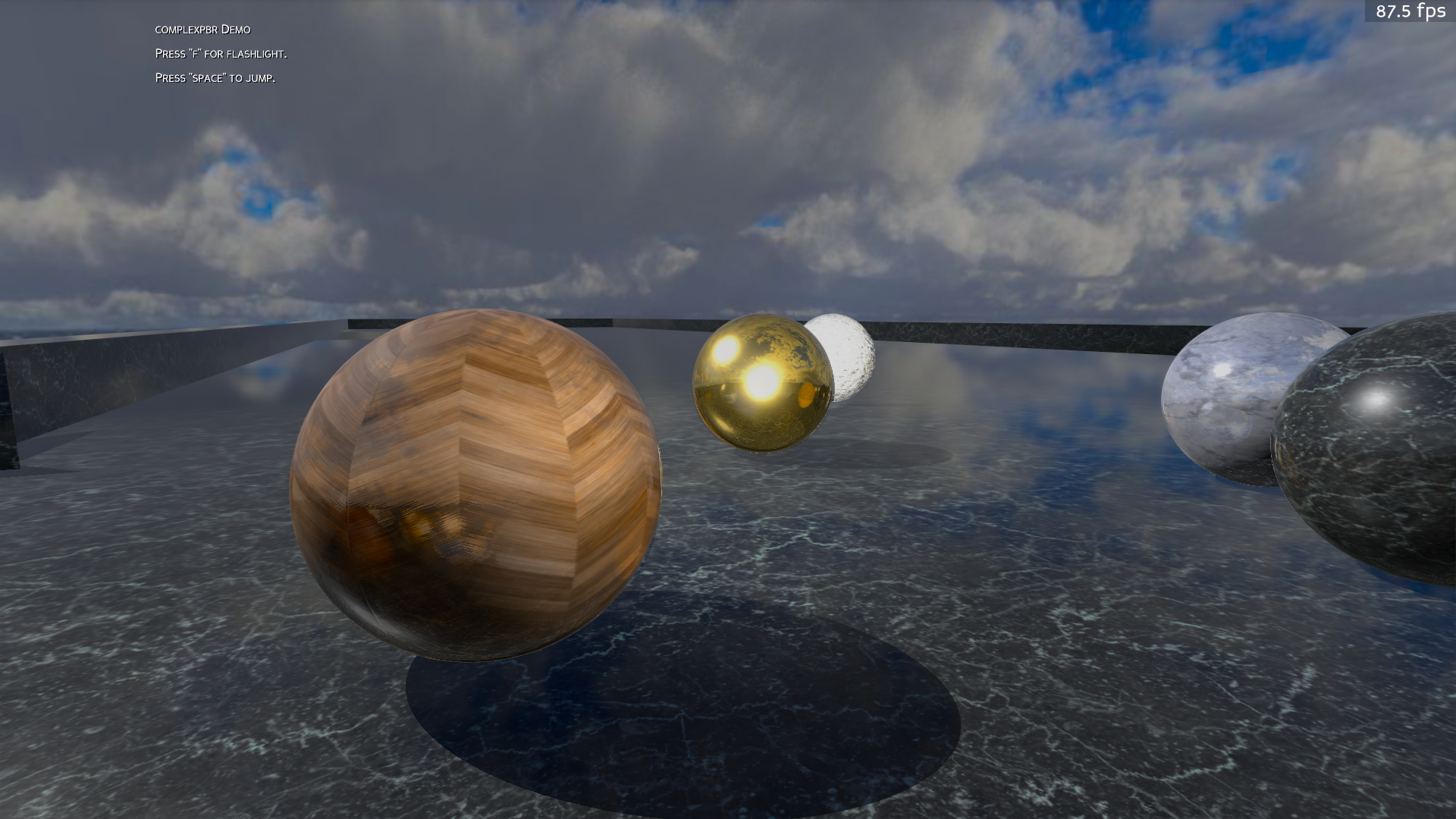\r\n\r\n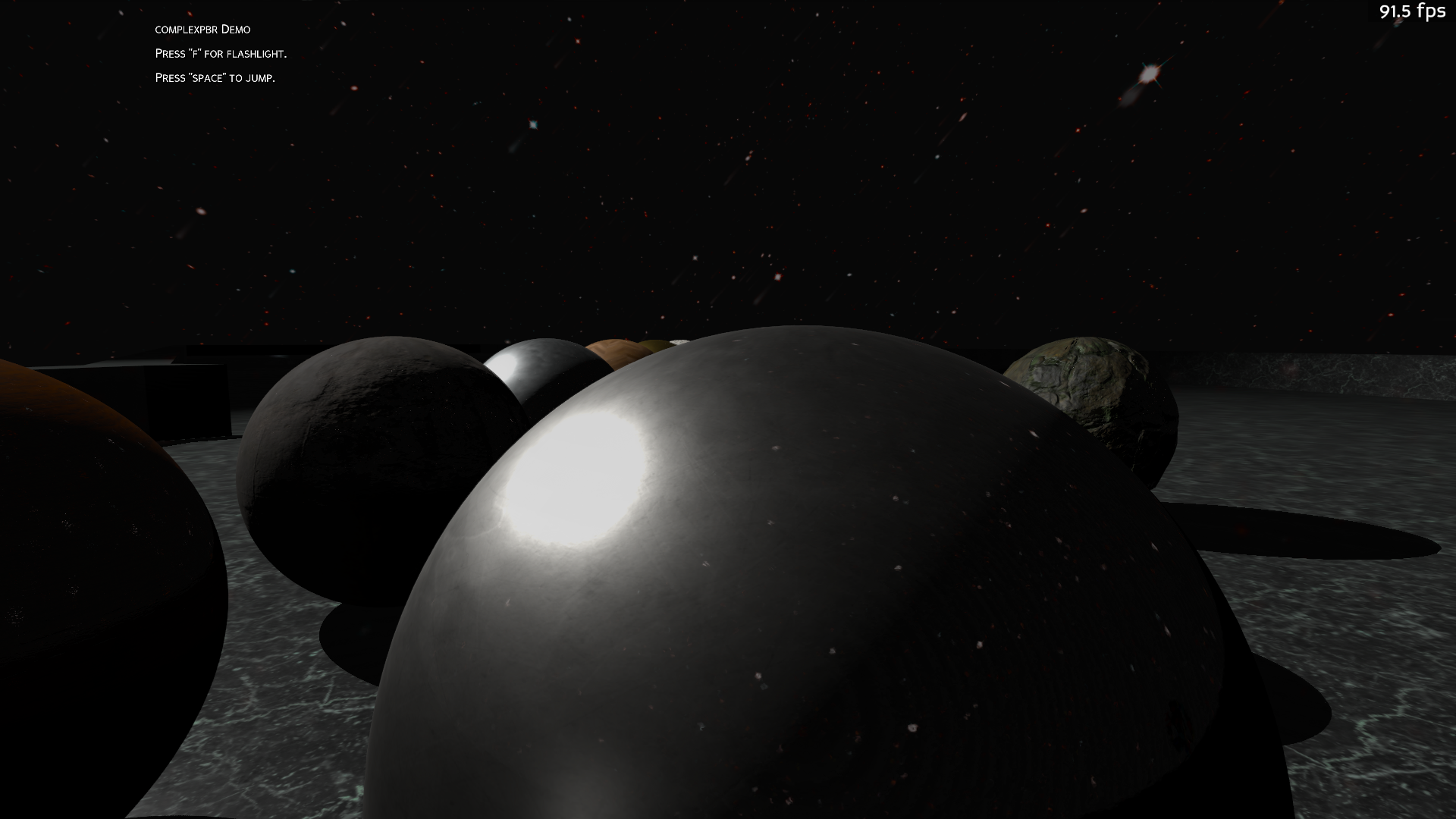\r\n\r\n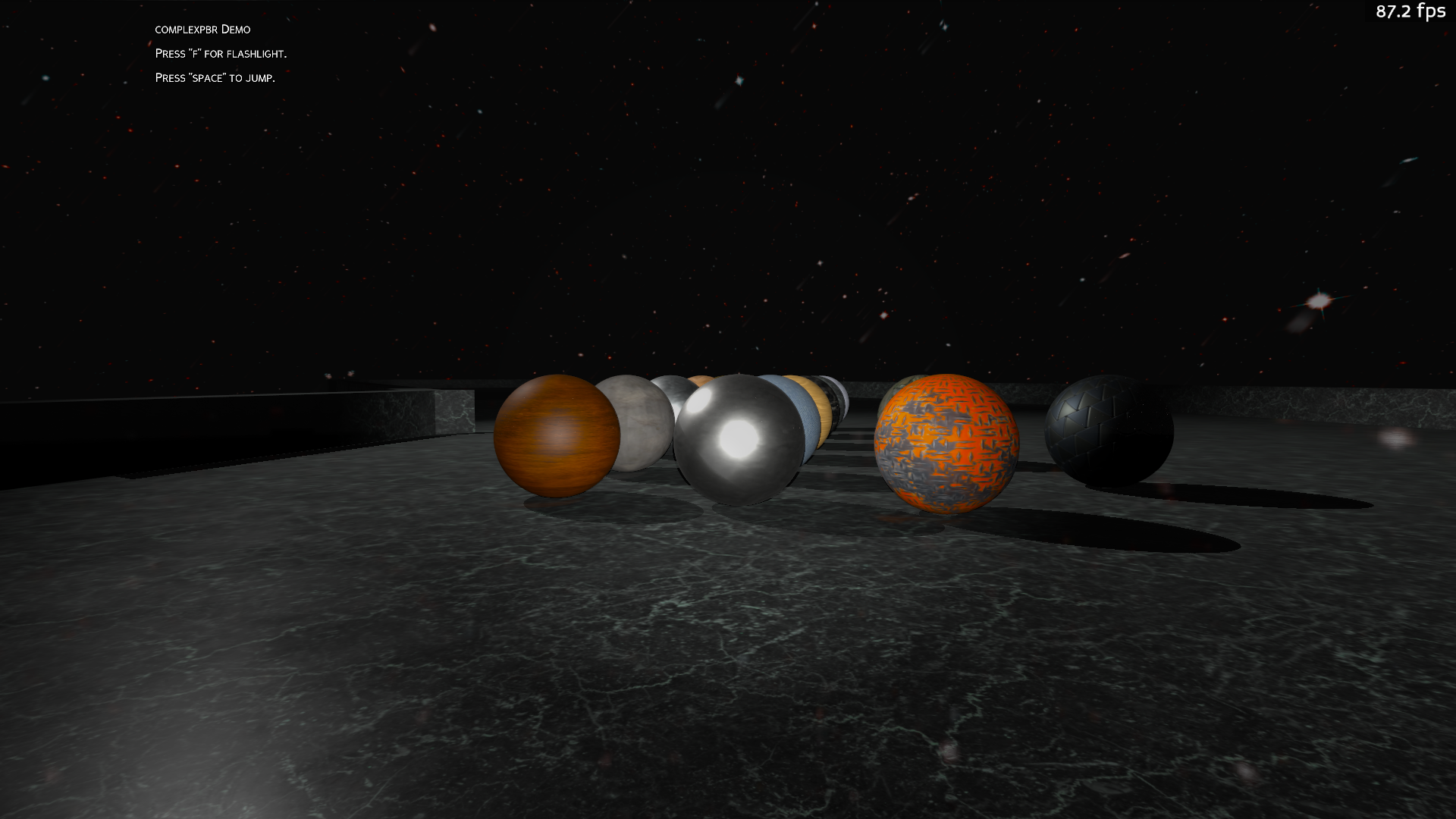\r\n\r\nVertex Displacement Mapping:\r\n\r\n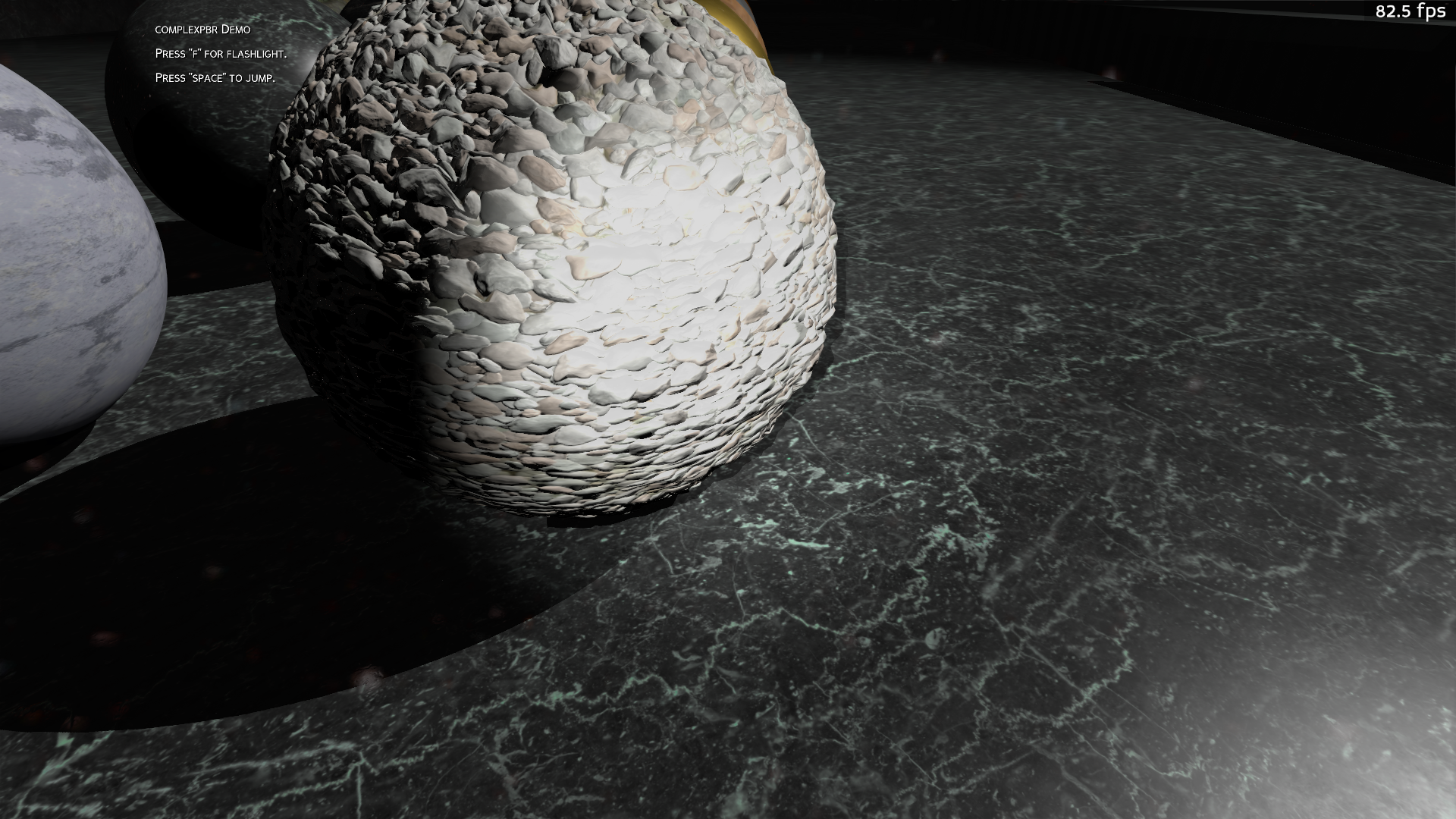\r\n\r\n",
"bugtrack_url": null,
"license": "BSD-3-Clause",
"summary": "Functional node level shader application for Panda3D",
"version": "0.5.3",
"project_urls": {
"homepage": "https://github.com/rayanalysis/panda3d-complexpbr"
},
"split_keywords": [
"panda3d",
"gamedev",
"pbr"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "7373574c6209a902854884647e1c8fed1462cdd675477ecaa96bf3580bf75d9f",
"md5": "d99f5302f0044cd4f7a4362a25bf96d3",
"sha256": "e69073f19c3b05a722456a916651b14a09dbd1ee8232b88c4a4be9633e924b0e"
},
"downloads": -1,
"filename": "panda3d_complexpbr-0.5.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d99f5302f0044cd4f7a4362a25bf96d3",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 301631,
"upload_time": "2023-10-19T21:41:33",
"upload_time_iso_8601": "2023-10-19T21:41:33.306868Z",
"url": "https://files.pythonhosted.org/packages/73/73/574c6209a902854884647e1c8fed1462cdd675477ecaa96bf3580bf75d9f/panda3d_complexpbr-0.5.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "4fbe1429d10d0edb7d5689b276fb03809cebb309800364aa98351c03e58de4a7",
"md5": "0f267e3a4bb2df82fdb5fbc4fecdf4b7",
"sha256": "4707ebcf4abe794b3533de02340bcfc4a4949dce07c24a6062f0e4c69f557147"
},
"downloads": -1,
"filename": "panda3d-complexpbr-0.5.3.tar.gz",
"has_sig": false,
"md5_digest": "0f267e3a4bb2df82fdb5fbc4fecdf4b7",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 304962,
"upload_time": "2023-10-19T21:41:35",
"upload_time_iso_8601": "2023-10-19T21:41:35.032253Z",
"url": "https://files.pythonhosted.org/packages/4f/be/1429d10d0edb7d5689b276fb03809cebb309800364aa98351c03e58de4a7/panda3d-complexpbr-0.5.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-19 21:41:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "rayanalysis",
"github_project": "panda3d-complexpbr",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "panda3d-complexpbr"
}