Name | pcf8591-library JSON |
Version |
0.0.3
JSON |
| download |
home_page | https://github.com/xreef/PCF8591_micropython_library |
Summary | Library to use pcf8591 i2c analog IC with Arduino, Raspberry Pi Pico and rp2040 boards, esp32, SMT32 and ESP8266. Can read analog value and write analog value with only 2 wire. |
upload_time | 2023-11-07 16:27:29 |
maintainer | Renzo Mischianti |
docs_url | None |
author | Renzo Mischianti |
requires_python | >=3.7 |
license | The MIT License (MIT) Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved. You may copy, alter and reuse this code in any way you like, but please leave reference to www.mischianti.org in your comments if you redistribute this code. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
micropython
analog
digital
i2c
encoder
expander
pcf8591
pcf8591a
esp32
esp8266
stm32
samd
arduino
wire
raspberry
rp2040
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
<div>
<a href="https://www.mischianti.org/forums/forum/mischiantis-libraries/pcf8591-i2c-analog-expander/"><img
src="https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumEnglish.png" alt="Support forum pcf8591 English"
align="right"></a>
</div>
<div>
<a href="https://www.mischianti.org/it/forums/forum/le-librerie-di-mischianti/pcf8591-expander-analogico-i2c/"><img
src="https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumItaliano.png" alt="Forum supporto pcf8591 italiano"
align="right"></a>
</div>
#
#### www.mischianti.org
### MicroPython Library to use i2c analog IC with arduino and esp8266. Can read analog value and write analog value with only 2 wire (perfect for ESP-01).
#### Changelog
- 07/11/2023: v0.0.3 Fix on read when write is active and running [#Forum](https://mischianti.org/forums/topic/micropython-i2c-pcf8591-round-value-problem-raspberry-pi-pico/#post-28043)
- 16/05/2023: v0.0.2 Minor fix on read and write [#Forum](https://www.mischianti.org/forums/topic/micropython-i2c-pcf8591-round-value-problem-raspberry-pi-pico/)
- 18/04/2023: v0.0.1 Initial commit of stable version.
I try to simplify the use of this IC, with a minimal set of operation.
#### Installation
To install the library execute the following command:
```bash
pip install pcf8591-library
```
**Constructor:**
Pass the address of I2C
```python
from PCF8591 import PCF8591
from machine import I2C, Pin
# Initialize the I2C bus
i2c = I2C(0, scl=Pin(22), sda=Pin(21))
# Initialize the PCF8591
pcf8591 = PCF8591(0x48, i2c)
if pcf8591.begin():
print("PCF8591 found")
```
or
```python
from PCF8591 import PCF8591
pcf8591 = PCF8591(0x48, sda=21, scl=22)
if pcf8591.begin():
print("PCF8591 found")
```
To read all analog input in one
```python
# Main loop
while True:
# Read all analog input channels
ain0, ain1, ain2, ain3 = pcf8591.analog_read_all()
# Print the results
print("AIN0:", ain0, "AIN1:", ain1, "AIN2:", ain2, "AIN3:", ain3)
# Wait for 1 second
utime.sleep(1)
```
If you want to read a single input:
```python
print("AIN0 ", pcf8591.analog_read(PCF8591.AIN0))
print("AIN1 ", pcf8591.analog_read(PCF8591.AIN1))
print("AIN2 ", pcf8591.analog_read(PCF8591.AIN2))
print("AIN3 ", pcf8591.analog_read(PCF8591.AIN3))
```
If you want to write a value:
```python
pcf8591.analog_write(255)
utime.sleep(1)
pcf8591.analog_write(128)
utime.sleep(1)
pcf8591.analog_write(0)
```
You can also read and write voltage
```python
pcf8591.voltage_write(3.3)
utime.sleep(1)
pcf8591.voltage_write(1.65)
utime.sleep(1)
pcf8591.voltage_write(0)
```
or
```python
print("AIN0 ", pcf8591.voltage_read(PCF8591.AIN0))
print("AIN1 ", pcf8591.voltage_read(PCF8591.AIN1))
print("AIN2 ", pcf8591.voltage_read(PCF8591.AIN2))
print("AIN3 ", pcf8591.voltage_read(PCF8591.AIN3))
```
For the examples I use this wire schema on breadboard:
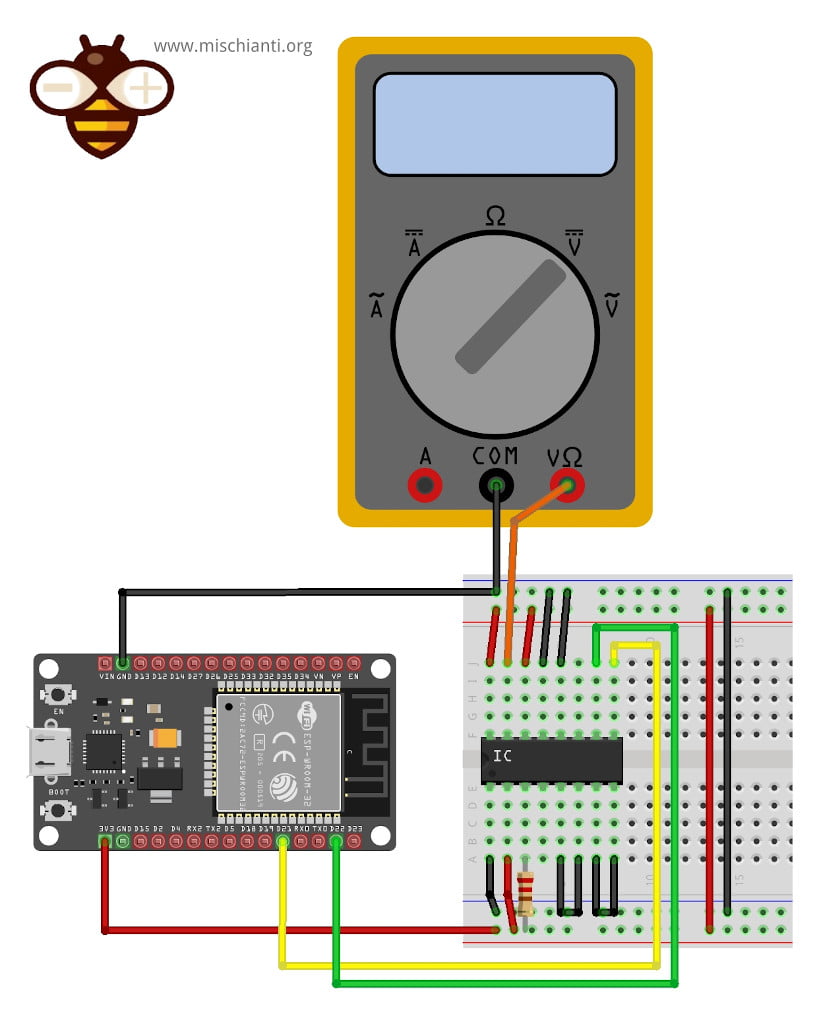
Raw data
{
"_id": null,
"home_page": "https://github.com/xreef/PCF8591_micropython_library",
"name": "pcf8591-library",
"maintainer": "Renzo Mischianti",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "Renzo Mischianti <renzo@mischianti.org>",
"keywords": "micropython,analog, digital, i2c, encoder, expander, pcf8591, pcf8591a, esp32, esp8266, stm32, SAMD, Arduino, wire, Raspberry, rp2040",
"author": "Renzo Mischianti",
"author_email": "Renzo Mischianti <renzo@mischianti.org>",
"download_url": "https://files.pythonhosted.org/packages/45/4a/bc3d4afe1edfc8225568ac0fbf27a410ff335b9a7192ab8c6665ec13ab6a/pcf8591-library-0.0.3.tar.gz",
"platform": null,
"description": "<div>\r\n<a href=\"https://www.mischianti.org/forums/forum/mischiantis-libraries/pcf8591-i2c-analog-expander/\"><img\r\n src=\"https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumEnglish.png\" alt=\"Support forum pcf8591 English\"\r\n align=\"right\"></a>\r\n</div>\r\n<div>\r\n<a href=\"https://www.mischianti.org/it/forums/forum/le-librerie-di-mischianti/pcf8591-expander-analogico-i2c/\"><img\r\n src=\"https://github.com/xreef/LoRa_E32_Series_Library/raw/master/resources/buttonSupportForumItaliano.png\" alt=\"Forum supporto pcf8591 italiano\"\r\n align=\"right\"></a>\r\n</div>\r\n\r\n#\r\n#### www.mischianti.org\r\n\r\n### MicroPython Library to use i2c analog IC with arduino and esp8266. Can read analog value and write analog value with only 2 wire (perfect for ESP-01).\r\n\r\n#### Changelog\r\n - 07/11/2023: v0.0.3 Fix on read when write is active and running [#Forum](https://mischianti.org/forums/topic/micropython-i2c-pcf8591-round-value-problem-raspberry-pi-pico/#post-28043)\r\n - 16/05/2023: v0.0.2 Minor fix on read and write [#Forum](https://www.mischianti.org/forums/topic/micropython-i2c-pcf8591-round-value-problem-raspberry-pi-pico/)\r\n - 18/04/2023: v0.0.1 Initial commit of stable version.\r\n\r\nI try to simplify the use of this IC, with a minimal set of operation.\r\n\r\n#### Installation\r\nTo install the library execute the following command:\r\n\r\n```bash\r\npip install pcf8591-library\r\n```\r\n\r\n**Constructor:**\r\nPass the address of I2C \r\n```python\r\nfrom PCF8591 import PCF8591\r\nfrom machine import I2C, Pin\r\n \r\n# Initialize the I2C bus\r\ni2c = I2C(0, scl=Pin(22), sda=Pin(21)) \r\n\r\n# Initialize the PCF8591\r\npcf8591 = PCF8591(0x48, i2c)\r\nif pcf8591.begin():\r\n print(\"PCF8591 found\")\r\n\r\n```\r\nor\r\n```python\r\nfrom PCF8591 import PCF8591\r\n\r\npcf8591 = PCF8591(0x48, sda=21, scl=22)\r\nif pcf8591.begin():\r\n print(\"PCF8591 found\")\r\n\r\n```\r\n\r\nTo read all analog input in one \r\n```python\r\n# Main loop\r\nwhile True:\r\n # Read all analog input channels\r\n ain0, ain1, ain2, ain3 = pcf8591.analog_read_all()\r\n\r\n # Print the results\r\n print(\"AIN0:\", ain0, \"AIN1:\", ain1, \"AIN2:\", ain2, \"AIN3:\", ain3)\r\n\r\n # Wait for 1 second\r\n utime.sleep(1)\r\n```\r\n\r\nIf you want to read a single input:\r\n```python\r\nprint(\"AIN0 \", pcf8591.analog_read(PCF8591.AIN0))\r\nprint(\"AIN1 \", pcf8591.analog_read(PCF8591.AIN1))\r\nprint(\"AIN2 \", pcf8591.analog_read(PCF8591.AIN2))\r\nprint(\"AIN3 \", pcf8591.analog_read(PCF8591.AIN3))\r\n```\r\n\r\nIf you want to write a value:\r\n```python\r\npcf8591.analog_write(255)\r\nutime.sleep(1)\r\npcf8591.analog_write(128)\r\nutime.sleep(1)\r\npcf8591.analog_write(0)\r\n```\r\n\r\nYou can also read and write voltage\r\n\r\n```python\r\npcf8591.voltage_write(3.3)\r\nutime.sleep(1)\r\npcf8591.voltage_write(1.65)\r\nutime.sleep(1)\r\npcf8591.voltage_write(0)\r\n```\r\nor\r\n```python\r\nprint(\"AIN0 \", pcf8591.voltage_read(PCF8591.AIN0))\r\nprint(\"AIN1 \", pcf8591.voltage_read(PCF8591.AIN1))\r\nprint(\"AIN2 \", pcf8591.voltage_read(PCF8591.AIN2))\r\nprint(\"AIN3 \", pcf8591.voltage_read(PCF8591.AIN3))\r\n```\r\n\r\n\r\nFor the examples I use this wire schema on breadboard:\r\n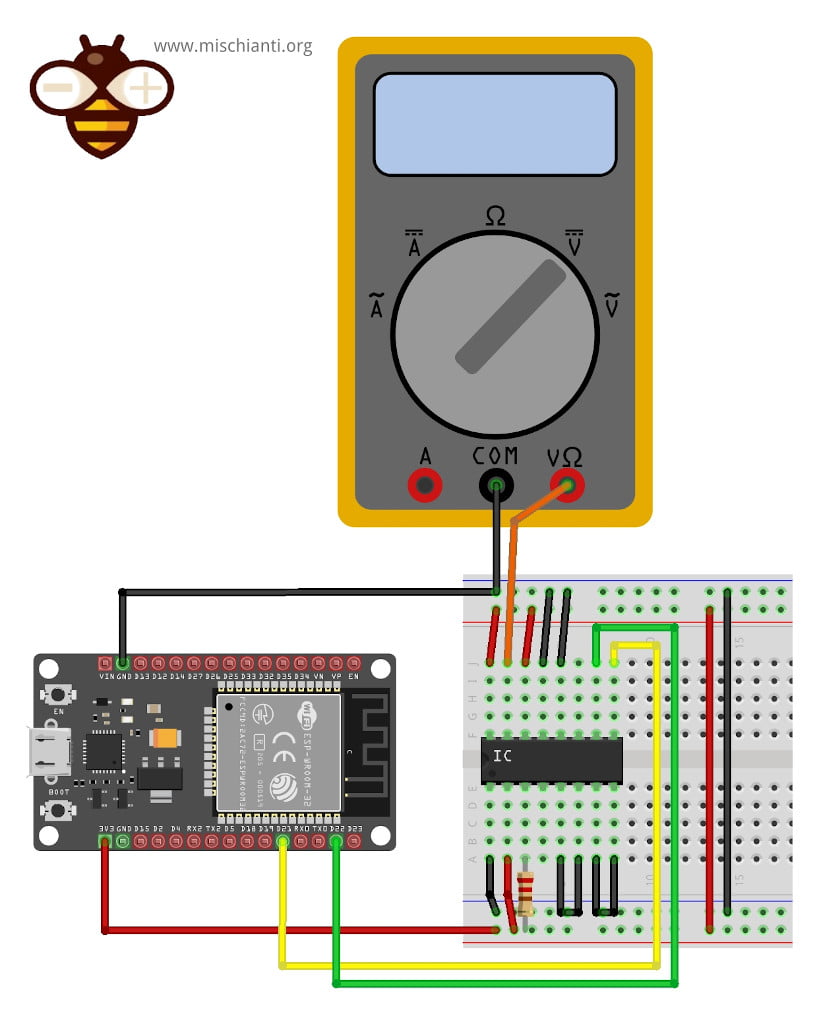\r\n",
"bugtrack_url": null,
"license": "The MIT License (MIT) Copyright (c) 2017 Renzo Mischianti www.mischianti.org All right reserved. You may copy, alter and reuse this code in any way you like, but please leave reference to www.mischianti.org in your comments if you redistribute this code. Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Library to use pcf8591 i2c analog IC with Arduino, Raspberry Pi Pico and rp2040 boards, esp32, SMT32 and ESP8266. Can read analog value and write analog value with only 2 wire.",
"version": "0.0.3",
"project_urls": {
"Bug Tracker": "https://github.com/xreef/PCF8591_micropython_library/issues",
"Documentation": "https://www.mischianti.org/2019/01/03/pcf8591-i2c-analog-i-o-expander/",
"Documentazione": "https://www.mischianti.org/it/2019/01/03/pcf8591-un-expander-i2c-i-o-analogico/",
"Examples": "https://github.com/xreef/PCF8591_micropython_library/tree/master/examples",
"Homepage": "https://github.com/xreef/PCF8591_micropython_library",
"Repository": "https://github.com/xreef/PCF8591_micropython_library",
"homepage": "https://www.mischianti.org"
},
"split_keywords": [
"micropython",
"analog",
" digital",
" i2c",
" encoder",
" expander",
" pcf8591",
" pcf8591a",
" esp32",
" esp8266",
" stm32",
" samd",
" arduino",
" wire",
" raspberry",
" rp2040"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a9ab96be293e54317f8bf7369b5b96bfab8e97d60bd21100f2ca83c399b236c8",
"md5": "3df3bb3bc80c58735cc1c3fbe4730c23",
"sha256": "701a4660f90996b3272bcf029502f65fc463eb23ca246352d60cd3dda6a52873"
},
"downloads": -1,
"filename": "pcf8591_library-0.0.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "3df3bb3bc80c58735cc1c3fbe4730c23",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 6859,
"upload_time": "2023-11-07T16:27:28",
"upload_time_iso_8601": "2023-11-07T16:27:28.196689Z",
"url": "https://files.pythonhosted.org/packages/a9/ab/96be293e54317f8bf7369b5b96bfab8e97d60bd21100f2ca83c399b236c8/pcf8591_library-0.0.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "454abc3d4afe1edfc8225568ac0fbf27a410ff335b9a7192ab8c6665ec13ab6a",
"md5": "d936d63ab01fe6c3bf3e1f856e7ff3ee",
"sha256": "169b44e5a5150ab82bfbe33c4919ecd6e06aa3ee0432406b2a2fa27b108b84b0"
},
"downloads": -1,
"filename": "pcf8591-library-0.0.3.tar.gz",
"has_sig": false,
"md5_digest": "d936d63ab01fe6c3bf3e1f856e7ff3ee",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 6168,
"upload_time": "2023-11-07T16:27:29",
"upload_time_iso_8601": "2023-11-07T16:27:29.669714Z",
"url": "https://files.pythonhosted.org/packages/45/4a/bc3d4afe1edfc8225568ac0fbf27a410ff335b9a7192ab8c6665ec13ab6a/pcf8591-library-0.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-07 16:27:29",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "xreef",
"github_project": "PCF8591_micropython_library",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pcf8591-library"
}