Name | perlin-noise JSON |
Version |
1.13
JSON |
| download |
home_page | None |
Summary | Python implementation for Perlin Noise with unlimited coordinates space |
upload_time | 2024-07-26 08:17:47 |
maintainer | None |
docs_url | None |
author | salaxieb |
requires_python | None |
license | None |
keywords |
|
VCS |
|
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
Smooth random noise generator
read more https://en.wikipedia.org/wiki/Perlin_noise
source code: https://github.com/salaxieb/perlin_noise
noise = PerlinNoise(octaves=3.5, seed=777)
octaves : number of sub rectangles in each [0, 1] range
seed : specific seed with which you want to initialize random generator
tile_sizes : tuple of ints of you want to noise seamlessly repeat itself
```python
from perlin_noise import PerlinNoise
noise = PerlinNoise()
# accepts as argument float and/or list[float]
noise(0.5) == noise([0.5])
# --> True
# noise not limited in space dimension and seamless in any space size
noise([0.5, 0.5]) == noise([0.5, 0.5, 0, 0, 0])
# --> True
# noise can seamlessly repeat isself
noise([0.5, 0.5], tile_sizes=[2, 5]) == noise([2.5, 5.5], tile_sizes=[2, 5])
# --> True
```
Usage examples:
```python
import matplotlib.pyplot as plt
from perlin_noise import PerlinNoise
noise = PerlinNoise(octaves=10, seed=1)
xpix, ypix = 100, 100
pic = [[noise([i/xpix, j/ypix]) for j in range(xpix)] for i in range(ypix)]
plt.imshow(pic, cmap='gray')
plt.show()
```
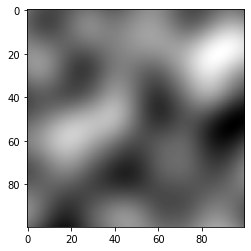
```python
import matplotlib.pyplot as plt
from perlin_noise import PerlinNoise
noise1 = PerlinNoise(octaves=3)
noise2 = PerlinNoise(octaves=6)
noise3 = PerlinNoise(octaves=12)
noise4 = PerlinNoise(octaves=24)
xpix, ypix = 100, 100
pic = []
for i in range(xpix):
row = []
for j in range(ypix):
noise_val = noise1([i/xpix, j/ypix])
noise_val += 0.5 * noise2([i/xpix, j/ypix])
noise_val += 0.25 * noise3([i/xpix, j/ypix])
noise_val += 0.125 * noise4([i/xpix, j/ypix])
row.append(noise_val)
pic.append(row)
plt.imshow(pic, cmap='gray')
plt.show()
```
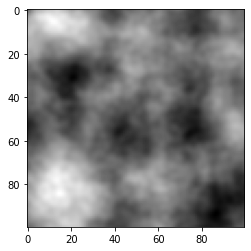
Library has a possibility to generate repetative random noise with custom tile sizes:
```python
import matplotlib.pyplot as plt
from perlin_noise import PerlinNoise
noise = PerlinNoise(octaves=2, seed=42)
xpix, ypix = 800, 1200
lim_x, lim_y = 6, 9
pic = [
[
noise([lim_x * i / xpix, lim_y * j / ypix], tile_sizes=[2, 3])
for j in range(xpix)
]
for i in range(ypix)
]
plt.imshow(pic, cmap="gray")
plt.show()
```

```python
import matplotlib.pyplot as plt
from perlin_noise import PerlinNoise
noise1 = PerlinNoise(octaves=1)
noise2 = PerlinNoise(octaves=3)
noise3 = PerlinNoise(octaves=6)
noise4 = PerlinNoise(octaves=12)
xpix, ypix = 800, 1200
lim_x, lim_y = 4, 6
tile_sizes = (2, 3)
pic = []
for i in range(ypix):
row = []
for j in range(xpix):
noise_val = noise1([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)
noise_val += 0.5 * noise2([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)
noise_val += 0.25 * noise3([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)
noise_val += 0.125 * noise4([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)
row.append(noise_val)
pic.append(row)
plt.imshow(pic, cmap="gray")
plt.savefig("pics/multy_noise_tiled.png", transparent=True)
plt.show()
```

for tiles to work correctly, number of octaves **MUST** be integer
```python
import matplotlib.pyplot as plt
from perlin_noise import PerlinNoise
noise = PerlinNoise(octaves=2.5, seed=42)
xpix, ypix = 800, 1200
lim_x, lim_y = 6, 9
pic = [
[
noise([lim_x * i / xpix, lim_y * j / ypix], tile_sizes=(2, 3))
for j in range(xpix)
]
for i in range(ypix)
]
plt.imshow(pic, cmap="gray")
plt.savefig('pics/tiled_with_step.png', transparent=True)
plt.show()
```

Raw data
{
"_id": null,
"home_page": null,
"name": "perlin-noise",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": null,
"author": "salaxieb",
"author_email": "salaxieb.ildar@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/bd/1e/571287a516c1ef00d2c65316ffa39ce1078510a015fa72c27338e3ecbfff/perlin_noise-1.13.tar.gz",
"platform": null,
"description": "Smooth random noise generator \nread more https://en.wikipedia.org/wiki/Perlin_noise \n\nsource code: https://github.com/salaxieb/perlin_noise\n\n\nnoise = PerlinNoise(octaves=3.5, seed=777) \n octaves : number of sub rectangles in each [0, 1] range \n seed : specific seed with which you want to initialize random generator\n tile_sizes : tuple of ints of you want to noise seamlessly repeat itself \n\n\n```python\nfrom perlin_noise import PerlinNoise\n\n\nnoise = PerlinNoise()\n# accepts as argument float and/or list[float]\nnoise(0.5) == noise([0.5])\n# --> True\n# noise not limited in space dimension and seamless in any space size\nnoise([0.5, 0.5]) == noise([0.5, 0.5, 0, 0, 0])\n# --> True\n# noise can seamlessly repeat isself\nnoise([0.5, 0.5], tile_sizes=[2, 5]) == noise([2.5, 5.5], tile_sizes=[2, 5])\n# --> True\n```\n\nUsage examples:\n```python\nimport matplotlib.pyplot as plt\nfrom perlin_noise import PerlinNoise\n\nnoise = PerlinNoise(octaves=10, seed=1)\nxpix, ypix = 100, 100\npic = [[noise([i/xpix, j/ypix]) for j in range(xpix)] for i in range(ypix)]\n\nplt.imshow(pic, cmap='gray')\nplt.show()\n```\n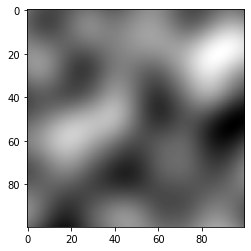\n\n```python\nimport matplotlib.pyplot as plt\nfrom perlin_noise import PerlinNoise\n\nnoise1 = PerlinNoise(octaves=3)\nnoise2 = PerlinNoise(octaves=6)\nnoise3 = PerlinNoise(octaves=12)\nnoise4 = PerlinNoise(octaves=24)\n\nxpix, ypix = 100, 100\npic = []\nfor i in range(xpix):\n row = []\n for j in range(ypix):\n noise_val = noise1([i/xpix, j/ypix])\n noise_val += 0.5 * noise2([i/xpix, j/ypix])\n noise_val += 0.25 * noise3([i/xpix, j/ypix])\n noise_val += 0.125 * noise4([i/xpix, j/ypix])\n\n row.append(noise_val)\n pic.append(row)\n\nplt.imshow(pic, cmap='gray')\nplt.show()\n```\n\n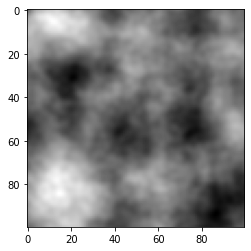\n\n\nLibrary has a possibility to generate repetative random noise with custom tile sizes:\n\n```python\nimport matplotlib.pyplot as plt\nfrom perlin_noise import PerlinNoise\n\nnoise = PerlinNoise(octaves=2, seed=42)\nxpix, ypix = 800, 1200\nlim_x, lim_y = 6, 9\npic = [\n [\n noise([lim_x * i / xpix, lim_y * j / ypix], tile_sizes=[2, 3])\n for j in range(xpix)\n ]\n for i in range(ypix)\n]\n\nplt.imshow(pic, cmap=\"gray\")\nplt.show()\n```\n\n\n\n```python\nimport matplotlib.pyplot as plt\nfrom perlin_noise import PerlinNoise\n\nnoise1 = PerlinNoise(octaves=1)\nnoise2 = PerlinNoise(octaves=3)\nnoise3 = PerlinNoise(octaves=6)\nnoise4 = PerlinNoise(octaves=12)\n\nxpix, ypix = 800, 1200\nlim_x, lim_y = 4, 6\ntile_sizes = (2, 3)\npic = []\nfor i in range(ypix):\n row = []\n for j in range(xpix):\n noise_val = noise1([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)\n noise_val += 0.5 * noise2([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)\n noise_val += 0.25 * noise3([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)\n noise_val += 0.125 * noise4([lim_x * i / xpix, lim_y * j / ypix], tile_sizes)\n\n row.append(noise_val)\n pic.append(row)\n\nplt.imshow(pic, cmap=\"gray\")\nplt.savefig(\"pics/multy_noise_tiled.png\", transparent=True)\nplt.show()\n```\n\n\nfor tiles to work correctly, number of octaves **MUST** be integer\n```python\nimport matplotlib.pyplot as plt\nfrom perlin_noise import PerlinNoise\n\nnoise = PerlinNoise(octaves=2.5, seed=42)\nxpix, ypix = 800, 1200\nlim_x, lim_y = 6, 9\npic = [\n [\n noise([lim_x * i / xpix, lim_y * j / ypix], tile_sizes=(2, 3))\n for j in range(xpix)\n ]\n for i in range(ypix)\n]\n\nplt.imshow(pic, cmap=\"gray\")\nplt.savefig('pics/tiled_with_step.png', transparent=True)\nplt.show()\n```\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Python implementation for Perlin Noise with unlimited coordinates space",
"version": "1.13",
"project_urls": null,
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "4db2c7bd5f926f0e2cf3a4cf21c527f6d1b8f43c84bbfcd5aa32f47c7c14cc89",
"md5": "da609b1495bff001c7b794fd4955dd63",
"sha256": "09f274c8da38f5a2a48b1626032a1b7738990df758a3bc7549ff3464869c6ca4"
},
"downloads": -1,
"filename": "perlin_noise-1.13-py3-none-any.whl",
"has_sig": false,
"md5_digest": "da609b1495bff001c7b794fd4955dd63",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 5847,
"upload_time": "2024-07-26T08:17:45",
"upload_time_iso_8601": "2024-07-26T08:17:45.785316Z",
"url": "https://files.pythonhosted.org/packages/4d/b2/c7bd5f926f0e2cf3a4cf21c527f6d1b8f43c84bbfcd5aa32f47c7c14cc89/perlin_noise-1.13-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "bd1e571287a516c1ef00d2c65316ffa39ce1078510a015fa72c27338e3ecbfff",
"md5": "493542861756622e5e91ed80aed4bfbf",
"sha256": "0e0f074743336fbe9cf2cdbdeb74c75d43780a8b3cbc3415859cd396ab4da177"
},
"downloads": -1,
"filename": "perlin_noise-1.13.tar.gz",
"has_sig": false,
"md5_digest": "493542861756622e5e91ed80aed4bfbf",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 6247,
"upload_time": "2024-07-26T08:17:47",
"upload_time_iso_8601": "2024-07-26T08:17:47.219405Z",
"url": "https://files.pythonhosted.org/packages/bd/1e/571287a516c1ef00d2c65316ffa39ce1078510a015fa72c27338e3ecbfff/perlin_noise-1.13.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-26 08:17:47",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "perlin-noise"
}