# Pigmento: Colorize and Trace Printing
**Due to the limitation of Github and Pypi Markdown, please visit [Pigmento](https://liu.qijiong.work/2023/10/18/Develop-Pigmento/) for a better reading experience.**
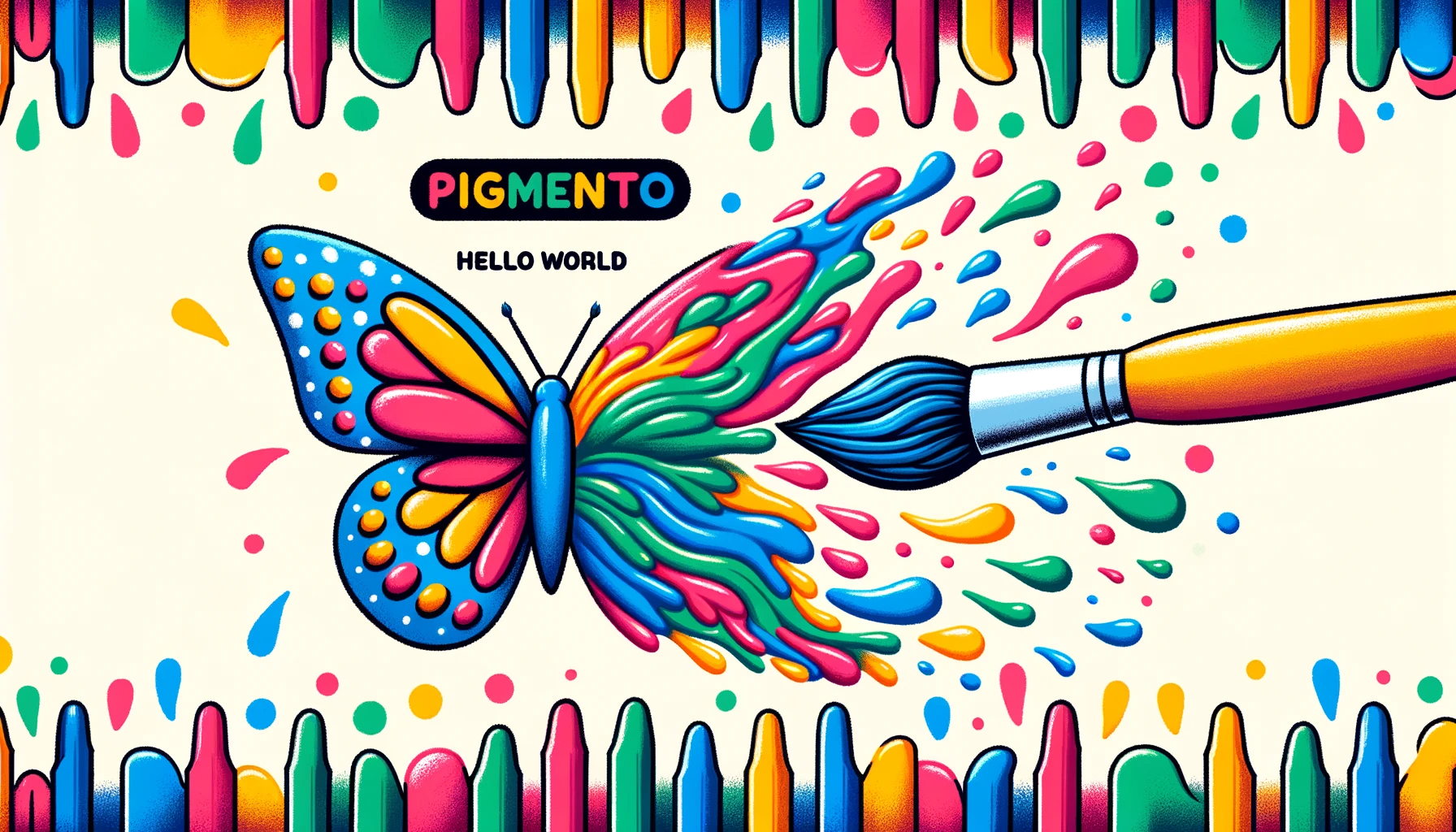
## Installation
```bash
pip install pigmento
```
## Quick Start
```python
from pigmento import pnt
class Test:
@classmethod
def class_method(cls):
pnt('Hello World')
def instance_method(self):
pnt('Hello World')
@staticmethod
def static_method():
pnt('Hello World')
def global_function():
pnt('Hello World')
Test.class_method()
Test().instance_method()
Test.static_method()
global_function()
```
<pre>
<span style="color: #E27DEA;">|Test|</span> <span style="color: #6BE7E7;">(class_method)</span> Hello World
<span style="color: #E27DEA;">|Test|</span> <span style="color: #6BE7E7;">(instance_method)</span> Hello World
<span style="color: #E27DEA;">|Test|</span> <span style="color: #6BE7E7;">(static_method)</span> Hello World
<span style="color: #6BE7E7;">(global_function)</span> Hello World
</pre>
### Style Customization
```python
from pigmento import pnt, Color
pnt.set_display_style(
method_color=Color.RED,
method_bracket=('<', '>'),
class_color=Color.BLUE,
class_bracket=('[', ']'),
)
Test.class_method()
Test().instance_method()
Test.static_method()
```
<pre>
<span style="color: #6B9BFF;">[Test]</span> <span style="color: #FF6B6B;"><class_method></span> Hello World
<span style="color: #6B9BFF;">[Test]</span> <span style="color: #FF6B6B;"><instance_method></span> Hello World
<span style="color: #6B9BFF;">[Test]</span> <span style="color: #FF6B6B;"><static_method></span> Hello World
</pre>
### Display Mode Customization
```python
from pigmento import pnt
pnt.set_display_mode(
display_method_name=False,
)
Test.class_method()
```
<pre>
<span style="color: #E27DEA;">|Test|</span> Hello World
</pre>
## Prefixes
Pigmento supports customized prefixes for each print.
It is important to note that all prefixes are in **first-in-first-print** order.
```python
from pigmento import pnt, Prefix, Color, Bracket
pnt.add_prefix(Prefix('DEBUG', bracket=Bracket.DEFAULT, color=Color.GREEN))
global_function()
```
<pre>
<span style="color: #9EE09E;">[DEBUG]</span> <span style="color: #6BE7E7;">(global_function)</span> Hello World
</pre>
### Dynamic Prefix
Texts inside prefix can be dynamically generated.
```python
from pigmento import pnt, Prefix, Color, Bracket
class System:
STATUS = 'TRAINING'
@classmethod
def get_status(cls):
return cls.STATUS
pnt.add_prefix(Prefix(System.get_status, bracket=Bracket.DEFAULT, color=Color.GREEN))
global_function()
System.STATUS = 'TESTING'
global_function()
```
<pre>
<span style="color: #9EE09E;">[TRAINING]</span> <span style="color: #6BE7E7;">(global_function)</span> Hello World
<span style="color: #9EE09E;">[TESTING]</span> <span style="color: #6BE7E7;">(global_function)</span> Hello World
</pre>
### Build-in Time Prefix
TimePrefix is a build-in prefix that can be used to display time.
```python
import time
import pigmento
from pigmento import pnt
pigmento.add_time_prefix()
Test.class_method()
time.sleep(1)
Test.class_method()
```
<pre>
<span style="color: #9EE09E;">[00:00:00]</span> <span style="color: #E27DEA;">|Test|</span> <span style="color: #6BE7E7;">(class_method)</span> Hello World
<span style="color: #9EE09E;">[00:00:01]</span> <span style="color: #E27DEA;">|Test|</span> <span style="color: #6BE7E7;">(class_method)</span> Hello World
</pre>
## Plugins
Pigmento supports plugins to extend its functionalities.
### Build-in Logger
Everytime you print something, it will be logged to a file.
```python
import pigmento
from pigmento import pnt
pigmento.add_log_plugin('log.txt')
global_function()
```
<pre>
<span style="color: #6BE7E7;">(global_function)</span> Hello World
</pre>
The log file will be created in the current working directory and the content will be removed the color codes.
```bash
cat log.txt
```
<pre>
[00:00:00] (global_function) Hello World
</pre>
### Build-in Dynamic Color
DynamicColor will map caller class names to colors.
```python
import pigmento
from pigmento import pnt
class A:
@staticmethod
def print():
pnt(f'Hello from A')
class B:
@staticmethod
def print():
pnt(f'Hello from B')
class D:
@staticmethod
def print():
pnt(f'Hello from C')
A().print()
B().print()
D().print()
pigmento.add_dynamic_color_plugin()
A().print()
B().print()
D().print()
```
<pre>
<span style="color: #E27DEA;">|A|</span> <span style="color: #6BE7E7;">(print)</span> Hello from A
<span style="color: #E27DEA;">|B|</span> <span style="color: #6BE7E7;">(print)</span> Hello from B
<span style="color: #E27DEA;">|D|</span> <span style="color: #6BE7E7;">(print)</span> Hello from C
<span style="color: #E27DEA;">|A|</span> <span style="color: #6BE7E7;">(print)</span> Hello from A
<span style="color: #FF6B6B;">|B|</span> <span style="color: #6BE7E7;">(print)</span> Hello from B
<span style="color: #FFE156;">|D|</span> <span style="color: #6BE7E7;">(print)</span> Hello from C
</pre>
### Plugin Customization
```python
from pigmento import pnt, BasePlugin
class RenamePlugin(BasePlugin):
def middleware_before_class_prefix(self, name, bracket, color):
return name.lower(), bracket, color
def middleware_before_method_prefix(self, name, bracket, color):
return name.replace('_', '-'), bracket, color
pnt.add_plugin(RenamePlugin())
Test.class_method()
Test().instance_method()
Test.static_method()
```
<pre>
<span style="color: #E27DEA;">|test|</span> <span style="color: #6BE7E7;">(class-method)</span> Hello World
<span style="color: #E27DEA;">|test|</span> <span style="color: #6BE7E7;">(instance-method)</span> Hello World
<span style="color: #E27DEA;">|test|</span> <span style="color: #6BE7E7;">(static-method)</span> Hello World
</pre>
## Basic Printer Customization
```python
import sys
import time
from pigmento import pnt
def flush_printer(prefix_s, prefix_s_with_color, text, **kwargs):
sys.stdout.write(f'\r{prefix_s_with_color} {text}')
sys.stdout.flush()
pnt.set_basic_printer(flush_printer)
def progress(total):
for i in range(total):
time.sleep(0.1) # 模拟工作
bar = f"Progress: |{'#' * (i + 1)}{'-' * (total - i - 1)}| {i + 1}/{total}"
pnt(bar)
progress(30)
```
<pre>
(progress) Progress: |#############-----------------| 13/30
</pre>
## Multiple Printers
Pigmento supports multiple printers.
```python
from pigmento import Pigmento, Bracket, Color, Prefix
debug = Pigmento()
debug.add_prefix(Prefix('DEBUG', bracket=Bracket.DEFAULT, color=Color.GREEN))
info = Pigmento()
info.add_prefix(Prefix('INFO', bracket=Bracket.DEFAULT, color=Color.BLUE))
error = Pigmento()
error.add_prefix(Prefix('ERROR', bracket=Bracket.DEFAULT, color=Color.RED))
def divide(a, b):
if not isinstance(a, int) or not isinstance(b, int):
error('Inputs must be integers')
return
if b == 0:
debug('Cannot divide by zero')
return
info(f'{a} / {b} = {a / b}')
divide(1, 2)
divide(1, 0)
divide('x', 'y')
```
<pre>
<span style="color: #6B9BFF;">[INFO]</span> <span style="color: #6BE7E7;">(divide)</span> 1 / 2 = 0.5
<span style="color: #9EE09E;">[DEBUG]</span> <span style="color: #6BE7E7;">(divide)</span> Cannot divide by zero
<span style="color: #FF6B6B;">[ERROR]</span> <span style="color: #6BE7E7;">(divide)</span> Inputs must be integers
</pre>
## License
MIT License
## Author
[Jyonn](https://liu.qijiong.work)
Raw data
{
"_id": null,
"home_page": "https://github.com/Jyonn/printi",
"name": "pigmento",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "print, color",
"author": "Jyonn Liu",
"author_email": "i@6-79.cn",
"download_url": "https://files.pythonhosted.org/packages/d8/86/30e567753bee8a6994c515c5540168fd50de101cec6536a3581e9d07b88d/pigmento-0.2.2.tar.gz",
"platform": "any",
"description": "# Pigmento: Colorize and Trace Printing\n\n**Due to the limitation of Github and Pypi Markdown, please visit [Pigmento](https://liu.qijiong.work/2023/10/18/Develop-Pigmento/) for a better reading experience.**\n\n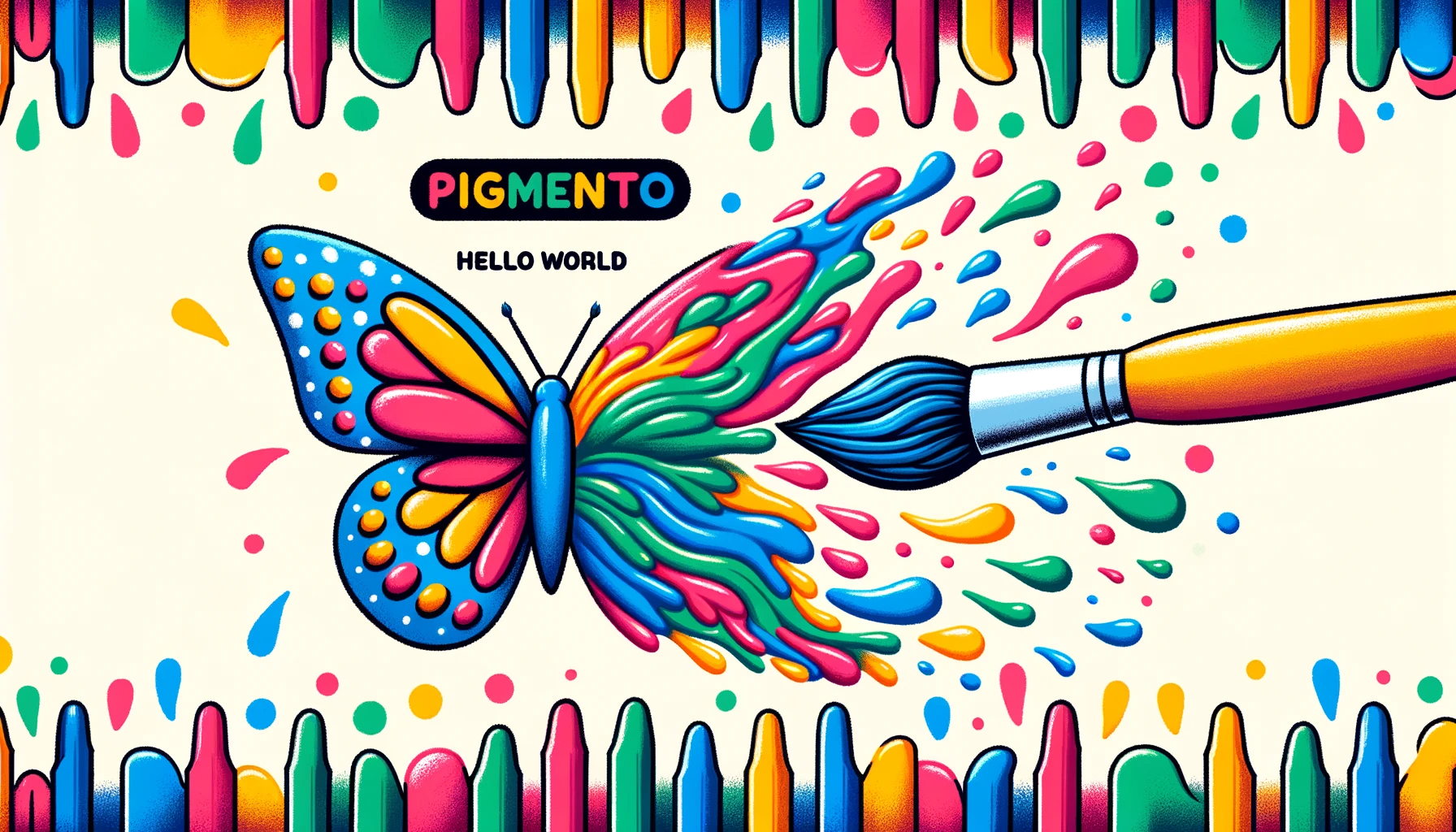\n\n## Installation\n\n```bash\npip install pigmento\n```\n\n## Quick Start\n\n```python\nfrom pigmento import pnt\n\nclass Test:\n @classmethod\n def class_method(cls):\n pnt('Hello World')\n\n def instance_method(self):\n pnt('Hello World')\n\n @staticmethod\n def static_method():\n pnt('Hello World')\n \n\ndef global_function():\n pnt('Hello World')\n\nTest.class_method()\nTest().instance_method()\nTest.static_method()\nglobal_function()\n```\n\n<pre>\n<span style=\"color: #E27DEA;\">|Test|</span> <span style=\"color: #6BE7E7;\">(class_method)</span> Hello World\n<span style=\"color: #E27DEA;\">|Test|</span> <span style=\"color: #6BE7E7;\">(instance_method)</span> Hello World\n<span style=\"color: #E27DEA;\">|Test|</span> <span style=\"color: #6BE7E7;\">(static_method)</span> Hello World\n<span style=\"color: #6BE7E7;\">(global_function)</span> Hello World\n</pre>\n\n### Style Customization\n\n```python\nfrom pigmento import pnt, Color\n\npnt.set_display_style(\n method_color=Color.RED,\n method_bracket=('<', '>'),\n class_color=Color.BLUE,\n class_bracket=('[', ']'),\n)\n\nTest.class_method()\nTest().instance_method()\nTest.static_method()\n```\n\n<pre>\n<span style=\"color: #6B9BFF;\">[Test]</span> <span style=\"color: #FF6B6B;\"><class_method></span> Hello World\n<span style=\"color: #6B9BFF;\">[Test]</span> <span style=\"color: #FF6B6B;\"><instance_method></span> Hello World\n<span style=\"color: #6B9BFF;\">[Test]</span> <span style=\"color: #FF6B6B;\"><static_method></span> Hello World\n</pre>\n\n### Display Mode Customization\n\n```python\nfrom pigmento import pnt\n\npnt.set_display_mode(\n display_method_name=False,\n)\n\nTest.class_method()\n```\n\n<pre>\n<span style=\"color: #E27DEA;\">|Test|</span> Hello World\n</pre>\n\n## Prefixes\n\nPigmento supports customized prefixes for each print.\nIt is important to note that all prefixes are in **first-in-first-print** order.\n\n```python\nfrom pigmento import pnt, Prefix, Color, Bracket\n\npnt.add_prefix(Prefix('DEBUG', bracket=Bracket.DEFAULT, color=Color.GREEN))\n\nglobal_function()\n```\n\n<pre>\n<span style=\"color: #9EE09E;\">[DEBUG]</span> <span style=\"color: #6BE7E7;\">(global_function)</span> Hello World\n</pre>\n\n### Dynamic Prefix\n\nTexts inside prefix can be dynamically generated.\n\n```python\nfrom pigmento import pnt, Prefix, Color, Bracket\n\nclass System:\n STATUS = 'TRAINING'\n \n @classmethod\n def get_status(cls):\n return cls.STATUS\n \n \npnt.add_prefix(Prefix(System.get_status, bracket=Bracket.DEFAULT, color=Color.GREEN))\n\nglobal_function()\nSystem.STATUS = 'TESTING'\nglobal_function()\n```\n\n<pre>\n<span style=\"color: #9EE09E;\">[TRAINING]</span> <span style=\"color: #6BE7E7;\">(global_function)</span> Hello World\n<span style=\"color: #9EE09E;\">[TESTING]</span> <span style=\"color: #6BE7E7;\">(global_function)</span> Hello World\n</pre>\n\n### Build-in Time Prefix\n\nTimePrefix is a build-in prefix that can be used to display time.\n\n```python\nimport time\nimport pigmento\nfrom pigmento import pnt\n\npigmento.add_time_prefix()\n\nTest.class_method()\ntime.sleep(1)\nTest.class_method()\n```\n\n<pre>\n<span style=\"color: #9EE09E;\">[00:00:00]</span> <span style=\"color: #E27DEA;\">|Test|</span> <span style=\"color: #6BE7E7;\">(class_method)</span> Hello World\n<span style=\"color: #9EE09E;\">[00:00:01]</span> <span style=\"color: #E27DEA;\">|Test|</span> <span style=\"color: #6BE7E7;\">(class_method)</span> Hello World\n</pre>\n\n## Plugins\n\nPigmento supports plugins to extend its functionalities.\n\n### Build-in Logger\n\nEverytime you print something, it will be logged to a file.\n\n```python\nimport pigmento\nfrom pigmento import pnt\n\npigmento.add_log_plugin('log.txt')\n\nglobal_function()\n```\n\n<pre>\n<span style=\"color: #6BE7E7;\">(global_function)</span> Hello World\n</pre>\n\nThe log file will be created in the current working directory and the content will be removed the color codes.\n\n```bash\ncat log.txt\n```\n\n<pre>\n[00:00:00] (global_function) Hello World\n</pre>\n\n### Build-in Dynamic Color\n\nDynamicColor will map caller class names to colors.\n\n```python\nimport pigmento\nfrom pigmento import pnt\n\n\nclass A:\n @staticmethod\n def print():\n pnt(f'Hello from A')\n\n\nclass B:\n @staticmethod\n def print():\n pnt(f'Hello from B')\n\n\nclass D:\n @staticmethod\n def print():\n pnt(f'Hello from C')\n\n\nA().print()\nB().print()\nD().print()\n\npigmento.add_dynamic_color_plugin()\n\nA().print()\nB().print()\nD().print()\n```\n\n<pre>\n<span style=\"color: #E27DEA;\">|A|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from A\n<span style=\"color: #E27DEA;\">|B|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from B\n<span style=\"color: #E27DEA;\">|D|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from C\n<span style=\"color: #E27DEA;\">|A|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from A\n<span style=\"color: #FF6B6B;\">|B|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from B\n<span style=\"color: #FFE156;\">|D|</span> <span style=\"color: #6BE7E7;\">(print)</span> Hello from C\n</pre>\n\n### Plugin Customization\n\n```python\nfrom pigmento import pnt, BasePlugin\n\n\nclass RenamePlugin(BasePlugin):\n def middleware_before_class_prefix(self, name, bracket, color):\n return name.lower(), bracket, color\n\n def middleware_before_method_prefix(self, name, bracket, color):\n return name.replace('_', '-'), bracket, color\n\n\npnt.add_plugin(RenamePlugin())\n\nTest.class_method()\nTest().instance_method()\nTest.static_method()\n```\n\n<pre>\n<span style=\"color: #E27DEA;\">|test|</span> <span style=\"color: #6BE7E7;\">(class-method)</span> Hello World\n<span style=\"color: #E27DEA;\">|test|</span> <span style=\"color: #6BE7E7;\">(instance-method)</span> Hello World\n<span style=\"color: #E27DEA;\">|test|</span> <span style=\"color: #6BE7E7;\">(static-method)</span> Hello World\n</pre>\n\n## Basic Printer Customization\n\n```python\nimport sys\nimport time\n\nfrom pigmento import pnt\n\n\ndef flush_printer(prefix_s, prefix_s_with_color, text, **kwargs):\n sys.stdout.write(f'\\r{prefix_s_with_color} {text}')\n sys.stdout.flush()\n\n\npnt.set_basic_printer(flush_printer)\n\ndef progress(total):\n for i in range(total):\n time.sleep(0.1) # \u6a21\u62df\u5de5\u4f5c\n bar = f\"Progress: |{'#' * (i + 1)}{'-' * (total - i - 1)}| {i + 1}/{total}\"\n pnt(bar)\n\nprogress(30)\n```\n\n<pre>\n(progress) Progress: |#############-----------------| 13/30\n</pre>\n\n## Multiple Printers\n\nPigmento supports multiple printers.\n\n```python\nfrom pigmento import Pigmento, Bracket, Color, Prefix\n\ndebug = Pigmento()\ndebug.add_prefix(Prefix('DEBUG', bracket=Bracket.DEFAULT, color=Color.GREEN))\n\ninfo = Pigmento()\ninfo.add_prefix(Prefix('INFO', bracket=Bracket.DEFAULT, color=Color.BLUE))\n\nerror = Pigmento()\nerror.add_prefix(Prefix('ERROR', bracket=Bracket.DEFAULT, color=Color.RED))\n\n\ndef divide(a, b):\n if not isinstance(a, int) or not isinstance(b, int):\n error('Inputs must be integers')\n return\n\n if b == 0:\n debug('Cannot divide by zero')\n return\n\n info(f'{a} / {b} = {a / b}')\n\n\ndivide(1, 2)\ndivide(1, 0)\ndivide('x', 'y')\n```\n\n<pre>\n<span style=\"color: #6B9BFF;\">[INFO]</span> <span style=\"color: #6BE7E7;\">(divide)</span> 1 / 2 = 0.5\n<span style=\"color: #9EE09E;\">[DEBUG]</span> <span style=\"color: #6BE7E7;\">(divide)</span> Cannot divide by zero\n<span style=\"color: #FF6B6B;\">[ERROR]</span> <span style=\"color: #6BE7E7;\">(divide)</span> Inputs must be integers\n</pre>\n\n## License\n\nMIT License\n\n## Author\n\n[Jyonn](https://liu.qijiong.work)\n\n\n",
"bugtrack_url": null,
"license": "MIT Licence",
"summary": "Colorful and Prefix-supported Print Toolkit",
"version": "0.2.2",
"project_urls": {
"Homepage": "https://github.com/Jyonn/printi"
},
"split_keywords": [
"print",
" color"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "d88630e567753bee8a6994c515c5540168fd50de101cec6536a3581e9d07b88d",
"md5": "af30f81b931060cccefc52c8e2aeb00c",
"sha256": "0f1f55ecdb93ada70b75a8987a5a085923cef10099342ff6792019d7eb530886"
},
"downloads": -1,
"filename": "pigmento-0.2.2.tar.gz",
"has_sig": false,
"md5_digest": "af30f81b931060cccefc52c8e2aeb00c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 8241,
"upload_time": "2024-06-17T02:45:45",
"upload_time_iso_8601": "2024-06-17T02:45:45.088107Z",
"url": "https://files.pythonhosted.org/packages/d8/86/30e567753bee8a6994c515c5540168fd50de101cec6536a3581e9d07b88d/pigmento-0.2.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-17 02:45:45",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Jyonn",
"github_project": "printi",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "pigmento"
}