# Python MVC Shell Framework Package
## About the package
Python MVC Shell Framework Package (PMVCS) is a tiny framework for shell projects making in Python 3.10+.
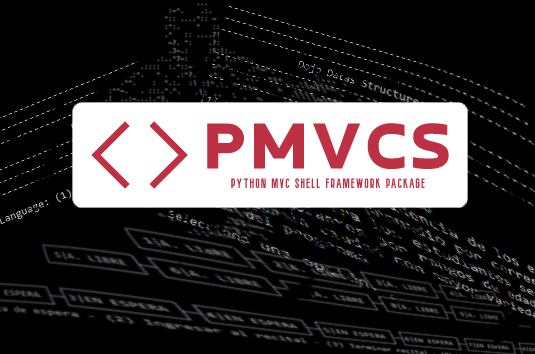
### Install with pip3
```
pip install pmvcs
or
pip3 install pmvcs
```
### Settings from console in english
```
>>> python -m pmvcs.cli setup -l en
or
>>> python -m pmvcs.cli setup -language en
or
>>> pmvcs-cli setup -l en
or
>>> pmvcs-cli setup -language en
```
### Settings from console in spanish
```
>>> python -m pmvcs.cli setup -l es
or
>>> python -m pmvcs.cli setup -language es
or
>>> pmvcs-cli setup -l es
or
>>> pmvcs-cli setup -language es
```
### Setup, step by step:
```
Insert APP name (default: "MyApp", leaves blank):
>>> My Test App
Insert APP folder name (default: "app", leaves blank):
>>> app
Enable debug? (default: "No")
(Y) Yes - (N) No:
>>> Y
View project information on exit? (default: "Yes")
(Y) Yes - (N) No:
>>> Y
Enable Multi-Language support? (default: "Yes")
(Y) Yes - (N) No:
>>> Y
Define default Language (default: "(1)")
(1) English - (2) EspaƱol:
>>> 2
Installs example data, use menu for multiple modules or unique module?
(E) Example data
(M) Multiple modules
(S) Unique module
Option:
>>> E -> Installs data examples, one module in menu with controller, model and view files.
>>> M -> Installs multiple modules in menu with controller, model and view files each one.
>>> S -> Installs a unique module in menu with controller file.
-------------------------------------------------
>>> Configuration has finished
>>> Thanks for choose PMVCS!
-------------------------------------------------
Press any key to continue . . .
```
### Add a new Multiple modules
Installs new multiple modules in menu with controller, model and view files each one.
For adding new modules in menu, run the following:
```
>>> python -m pmvcs.cli menu -l en
o
>>> python -m pmvcs.cli menu -language en
```
```
Current APP folder name:
>>> app01
Insert module name:
>>> example_two
Insert another module
(Y) Yes - (N) No:
>>> N
-------------------------------------------------
>>> Configuration has finished
>>> Thanks for choose PMVCS!
-------------------------------------------------
Press any key to continue . . .
```
### PMVCS Helpers
You can load PMVCS Helpers, for example:
```
old_value = str('5')
print(type(old_value))
>>> <class 'str'>
filters = self.pmvcs_helper.load_helper('filters', True)
new_value = filters.data_type(old_value)
print(type(new_value))
>>> <class 'int'>
```
The helper "filters" returns an integer value from a string number.
### Custom Helpers
You can load custom helpers, for example:
```
example = self.pmvcs_helper.load_helper('example')
example.my_func()
```
Path to save your helper: (app_folder)/helpers/
Format in helper's file:
```
from pmvcs.core.helpers.base_helper import BaseHelper
class ExampleHelper(BaseHelper):
""" Class for Example Helper """
def __init__(self, **kwargs) -> None:
"""
Init PMVCS Example Helper requirements
"""
super().__init__(**kwargs)
def my_func(self):
"""
Returns a float or int value
"""
pass
```
### Custom Helpers: Pass variables:
In __init__ it should have:
```
def __init__(self, **kwargs) -> None:
self.pmvcs_helper = kwargs['pmvcs_helper']
self.kwargs = { 'pmvcs_cfg': kwargs['pmvcs_cfg'],
'pmvcs_lang': kwargs['pmvcs_lang'],
'pmvcs_helper': kwargs['pmvcs_helper']}
```
And in the function that calls the helper:
```
def to_string_table(self, data: dict) -> str:
kwargs2 = { 'data': data,
'file_name': 'temp_file'}
kwargs2.update(self.kwargs)
table_helper = self.pmvcs_helper.load_helper('table', **kwargs2)
table_helper.record_file()
```
Finally in the helper we retrieve the values as:
```
def __init__(self, **kwargs) -> None:
super().__init__(**kwargs)
self._data = kwargs['data']
self._file_name = f"{kwargs['file_name']}.{self._file_extension}"
```
### Get configuration constants from config.ini:
Get a configuration constant in string type from "OPTIONS":
```
Code: >>> self.cfg.get("EXAMPLE_CONSTANT", "OPTIONS")
Returns: This is an example value from config file
Value Type: <class 'str'>
```
Get a configuration constant in string type from "DEFAULT":
```
Code: >>> self.cfg.get("DEFAULT_TITLE", "DEFAULT")
Returns: Example App
Value Type: <class 'str'>
```
Get a configuration constant in integer type:
```
Code: >>> self.cfg.get("EXAMPLE_INT", "OPTIONS", "int")
Returns: 8
Value Type: <class 'int'>
```
Get a configuration constant in float type:
```
Code: >>> self.cfg.get("EXAMPLE_FLOAT", "OPTIONS", "float")
Returns: 1.57
Value Type: <class 'float'>
```
Get a configuration constant in boolean type:
```
Code: >>> self.cfg.get("EXAMPLE_BOOLEAN", "OPTIONS", "boolean")
Returns: True
Value Type: <class 'bool'>
```
Get a configuration constant in a list:
```
Code: >>> self.cfg.get("EXAMPLE_LIST", "OPTIONS", "list")
Returns: ['1', '2']
Value Type: <class 'list'>
```
Get a configuration constant in a dictionary:
```
Code: >>> self.cfg.get("EXAMPLE_DICT", "OPTIONS", "dict")
Returns: {'value_one': '1', 'value_two': '2'}
Value Type: <class 'dict'>
```
### Get language constants from languages/en.ini:
Get current language tag:
```
Code: >>> self.lang.tag
Returns: en
Value Type: <class 'str'>
```
Get a language constant:
```
Code: >>> self.lang.get("LANG_EXAMPLE_STRING")
Returns: This is an example string
Value Type: <class 'str'>
```
Get a language constant by passing a value in String-Print-Format.
In the language file you will see for example: "The value here: "{}"
```
Code: >>> self.lang.sprintf("LANG_EXAMPLE_SPRINTF", "3")
Returns: String-Print-Format value here: "3"
Value Type: <class 'str'>
```
Get a language constant by passing many values in String-Print-Format.
In the language file you will see: "One: "{}". Two: "{}". Three: "{}".
```
Code: >>> self.lang.sprintf("LANG_EXAMPLE_SPRINTF2", "1", "2", "3")
Returns: One: "1". Two: "2". Three: "3".
Value Type: <class 'str'>
```
Translating a string:
```
Code: >>> value = 'dictionary'
>>> self.lang.translate(value)
Returns: Dictionary
Value Type: <class 'str'>
```
### Using PMVCS View functions
This shows the banner with the App's name:
```
Code: >>> self.pmvcs_view.get_intro()
```
This shows the exit message:
```
Code: >>> self.pmvcs_view.get_exit()
```
This inserts a line break without print():
```
Code: >>> self.pmvcs_view.line_brake()
```
This inserts a line break with print():
```
Code: >>> self.pmvcs_view.line_brake(True)
```
This inserts a pause to press ENTER to start:
```
Code: >>> self.pmvcs_view.input_start()
```
This inserts a pause to press ENTER to continue:
```
Code: >>> self.pmvcs_view.input_pause()
```
This inserts a select an option input():
```
Code: >>> self.pmvcs_view.input_options()
```
This inserts a select an input():
```
Code: >>> self.pmvcs_view.input_generic(text)
```
## PMVCS captures
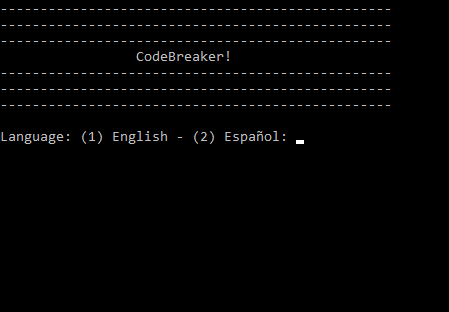
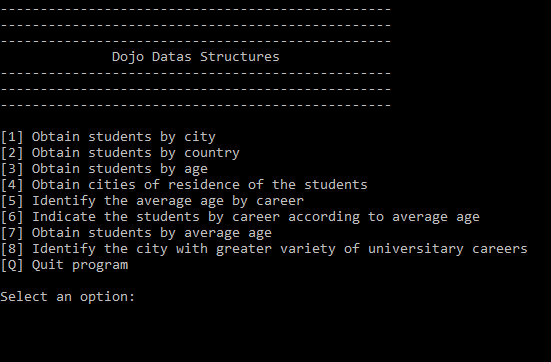
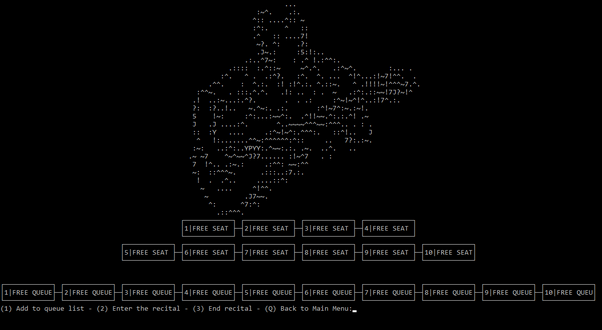
## PMVCS implementation examples
* [PMVCS Examples](https://github.com/gsmx64/pmvcs/tree/main/examples)
## Repository
* [Repository PMVCS](https://github.com/gsmx64/pmvcs)
Raw data
{
"_id": null,
"home_page": "",
"name": "pmvcs",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "pmvcs,python shell,python mvc,python shell framework,python mvc,python mvc shell framework",
"author": "",
"author_email": "Gonzalo Mahserdjian <gsmx64@outlook.com>",
"download_url": "https://files.pythonhosted.org/packages/31/45/7f9f972e958336780cdc291300969e0dc9b4c2f5a4f971cf177fd68a9798/pmvcs-1.0.4.tar.gz",
"platform": null,
"description": "# Python MVC Shell Framework Package\r\n\r\n## About the package\r\n\r\nPython MVC Shell Framework Package (PMVCS) is a tiny framework for shell projects making in Python 3.10+.\r\n\r\n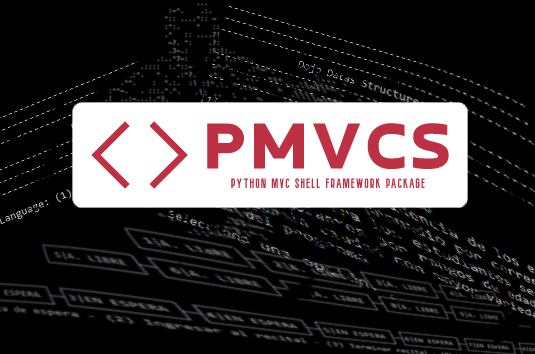\r\n\r\n\r\n### Install with pip3\r\n\r\n```\r\npip install pmvcs\r\n\r\nor\r\n\r\npip3 install pmvcs\r\n```\r\n\r\n\r\n### Settings from console in english\r\n\r\n```\r\n>>> python -m pmvcs.cli setup -l en\r\nor\r\n>>> python -m pmvcs.cli setup -language en\r\nor\r\n>>> pmvcs-cli setup -l en\r\nor\r\n>>> pmvcs-cli setup -language en\r\n```\r\n\r\n### Settings from console in spanish\r\n\r\n```\r\n>>> python -m pmvcs.cli setup -l es\r\nor\r\n>>> python -m pmvcs.cli setup -language es\r\nor\r\n>>> pmvcs-cli setup -l es\r\nor\r\n>>> pmvcs-cli setup -language es\r\n```\r\n\r\n\r\n### Setup, step by step:\r\n\r\n```\r\nInsert APP name (default: \"MyApp\", leaves blank):\r\n>>> My Test App\r\n\r\nInsert APP folder name (default: \"app\", leaves blank):\r\n>>> app\r\n\r\nEnable debug? (default: \"No\")\r\n(Y) Yes - (N) No:\r\n>>> Y\r\n\r\nView project information on exit? (default: \"Yes\")\r\n(Y) Yes - (N) No:\r\n>>> Y\r\n\r\nEnable Multi-Language support? (default: \"Yes\")\r\n(Y) Yes - (N) No:\r\n>>> Y\r\n\r\nDefine default Language (default: \"(1)\")\r\n(1) English - (2) Espa\u00f1ol:\r\n>>> 2\r\n\r\nInstalls example data, use menu for multiple modules or unique module?\r\n(E) Example data\r\n(M) Multiple modules\r\n(S) Unique module\r\n\r\nOption:\r\n>>> E -> Installs data examples, one module in menu with controller, model and view files.\r\n>>> M -> Installs multiple modules in menu with controller, model and view files each one.\r\n>>> S -> Installs a unique module in menu with controller file.\r\n\r\n-------------------------------------------------\r\n >>> Configuration has finished\r\n >>> Thanks for choose PMVCS!\r\n-------------------------------------------------\r\n\r\nPress any key to continue . . .\r\n```\r\n\r\n\r\n### Add a new Multiple modules\r\n\r\nInstalls new multiple modules in menu with controller, model and view files each one.\r\nFor adding new modules in menu, run the following:\r\n\r\n```\r\n>>> python -m pmvcs.cli menu -l en\r\no\r\n>>> python -m pmvcs.cli menu -language en\r\n```\r\n\r\n```\r\nCurrent APP folder name:\r\n>>> app01\r\n\r\nInsert module name:\r\n>>> example_two\r\n\r\nInsert another module\r\n(Y) Yes - (N) No:\r\n>>> N\r\n\r\n-------------------------------------------------\r\n >>> Configuration has finished\r\n >>> Thanks for choose PMVCS!\r\n-------------------------------------------------\r\n\r\nPress any key to continue . . .\r\n```\r\n\r\n\r\n### PMVCS Helpers\r\n\r\nYou can load PMVCS Helpers, for example:\r\n\r\n```\r\nold_value = str('5')\r\nprint(type(old_value))\r\n>>> <class 'str'>\r\n\r\nfilters = self.pmvcs_helper.load_helper('filters', True)\r\nnew_value = filters.data_type(old_value)\r\n\r\nprint(type(new_value))\r\n>>> <class 'int'>\r\n```\r\n\r\nThe helper \"filters\" returns an integer value from a string number.\r\n\r\n\r\n### Custom Helpers\r\n\r\nYou can load custom helpers, for example:\r\n\r\n```\r\nexample = self.pmvcs_helper.load_helper('example')\r\nexample.my_func()\r\n```\r\n\r\nPath to save your helper: (app_folder)/helpers/\r\n\r\nFormat in helper's file:\r\n```\r\nfrom pmvcs.core.helpers.base_helper import BaseHelper\r\n\r\n\r\nclass ExampleHelper(BaseHelper):\r\n \"\"\" Class for Example Helper \"\"\"\r\n\r\n def __init__(self, **kwargs) -> None:\r\n \"\"\"\r\n Init PMVCS Example Helper requirements\r\n \"\"\"\r\n super().__init__(**kwargs)\r\n\r\n def my_func(self):\r\n \"\"\"\r\n Returns a float or int value\r\n \"\"\"\r\n pass\r\n```\r\n\r\n\r\n### Custom Helpers: Pass variables:\r\n\r\nIn __init__ it should have:\r\n\r\n```\r\ndef __init__(self, **kwargs) -> None:\r\n\tself.pmvcs_helper = kwargs['pmvcs_helper']\r\n\tself.kwargs = { 'pmvcs_cfg': kwargs['pmvcs_cfg'],\r\n\t\t\t\t\t'pmvcs_lang': kwargs['pmvcs_lang'],\r\n\t\t\t\t\t'pmvcs_helper': kwargs['pmvcs_helper']}\r\n\t\t\t\t\t\t\r\n```\r\n\r\nAnd in the function that calls the helper:\r\n\r\n```\r\ndef to_string_table(self, data: dict) -> str:\r\n\tkwargs2 = { 'data': data,\r\n\t\t\t\t'file_name': 'temp_file'}\r\n\tkwargs2.update(self.kwargs)\r\n\ttable_helper = self.pmvcs_helper.load_helper('table', **kwargs2)\r\n\ttable_helper.record_file()\r\n```\r\n\r\nFinally in the helper we retrieve the values as:\r\n\r\n```\r\ndef __init__(self, **kwargs) -> None:\r\n\tsuper().__init__(**kwargs)\r\n\t\r\n\tself._data = kwargs['data']\r\n\tself._file_name = f\"{kwargs['file_name']}.{self._file_extension}\"\r\n```\r\n\r\n\r\n### Get configuration constants from config.ini:\r\n\r\nGet a configuration constant in string type from \"OPTIONS\":\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_CONSTANT\", \"OPTIONS\")\r\nReturns: This is an example value from config file\r\nValue Type: <class 'str'>\r\n```\r\n\r\nGet a configuration constant in string type from \"DEFAULT\":\r\n```\r\nCode: >>> self.cfg.get(\"DEFAULT_TITLE\", \"DEFAULT\")\r\nReturns: Example App\r\nValue Type: <class 'str'>\r\n```\r\n\r\nGet a configuration constant in integer type:\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_INT\", \"OPTIONS\", \"int\")\r\nReturns: 8\r\nValue Type: <class 'int'>\r\n```\r\n\r\nGet a configuration constant in float type:\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_FLOAT\", \"OPTIONS\", \"float\")\r\nReturns: 1.57\r\nValue Type: <class 'float'>\r\n```\r\n\r\nGet a configuration constant in boolean type:\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_BOOLEAN\", \"OPTIONS\", \"boolean\")\r\nReturns: True\r\nValue Type: <class 'bool'>\r\n```\r\n\r\nGet a configuration constant in a list:\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_LIST\", \"OPTIONS\", \"list\")\r\nReturns: ['1', '2']\r\nValue Type: <class 'list'>\r\n```\r\n\r\nGet a configuration constant in a dictionary:\r\n```\r\nCode: >>> self.cfg.get(\"EXAMPLE_DICT\", \"OPTIONS\", \"dict\")\r\nReturns: {'value_one': '1', 'value_two': '2'}\r\nValue Type: <class 'dict'>\r\n```\r\n\r\n\r\n### Get language constants from languages/en.ini:\r\n\r\nGet current language tag:\r\n```\r\nCode: >>> self.lang.tag\r\nReturns: en\r\nValue Type: <class 'str'>\r\n```\r\n\r\nGet a language constant:\r\n```\r\nCode: >>> self.lang.get(\"LANG_EXAMPLE_STRING\")\r\nReturns: This is an example string\r\nValue Type: <class 'str'>\r\n```\r\n\r\nGet a language constant by passing a value in String-Print-Format.\r\nIn the language file you will see for example: \"The value here: \"{}\"\r\n```\r\nCode: >>> self.lang.sprintf(\"LANG_EXAMPLE_SPRINTF\", \"3\")\r\nReturns: String-Print-Format value here: \"3\"\r\nValue Type: <class 'str'>\r\n```\r\n\r\nGet a language constant by passing many values in String-Print-Format.\r\nIn the language file you will see: \"One: \"{}\". Two: \"{}\". Three: \"{}\".\r\n```\r\nCode: >>> self.lang.sprintf(\"LANG_EXAMPLE_SPRINTF2\", \"1\", \"2\", \"3\")\r\nReturns: One: \"1\". Two: \"2\". Three: \"3\".\r\nValue Type: <class 'str'>\r\n```\r\n\r\nTranslating a string:\r\n```\r\nCode: >>> value = 'dictionary'\r\n\t >>> self.lang.translate(value)\r\nReturns: Dictionary\r\nValue Type: <class 'str'>\r\n```\r\n \r\n \r\n### Using PMVCS View functions\r\n\r\nThis shows the banner with the App's name:\r\n```\r\nCode: >>> self.pmvcs_view.get_intro()\r\n```\r\n\r\nThis shows the exit message:\r\n```\r\nCode: >>> self.pmvcs_view.get_exit()\r\n```\r\n\r\nThis inserts a line break without print():\r\n```\r\nCode: >>> self.pmvcs_view.line_brake()\r\n```\r\n\r\nThis inserts a line break with print():\r\n```\r\nCode: >>> self.pmvcs_view.line_brake(True)\r\n```\r\n\r\nThis inserts a pause to press ENTER to start:\r\n```\r\nCode: >>> self.pmvcs_view.input_start()\r\n```\r\n\r\nThis inserts a pause to press ENTER to continue:\r\n```\r\nCode: >>> self.pmvcs_view.input_pause()\r\n```\r\n\r\nThis inserts a select an option input():\r\n```\r\nCode: >>> self.pmvcs_view.input_options()\r\n```\r\n\r\nThis inserts a select an input():\r\n```\r\nCode: >>> self.pmvcs_view.input_generic(text)\r\n```\r\n \r\n \r\n ## PMVCS captures\r\n \r\n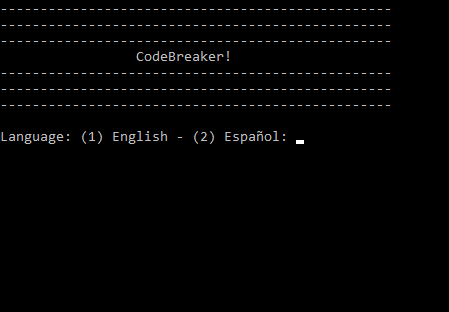\r\n\r\n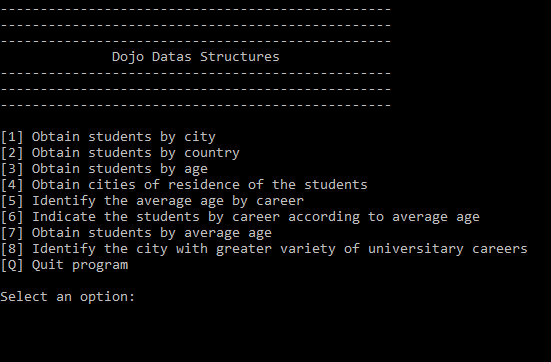\r\n\r\n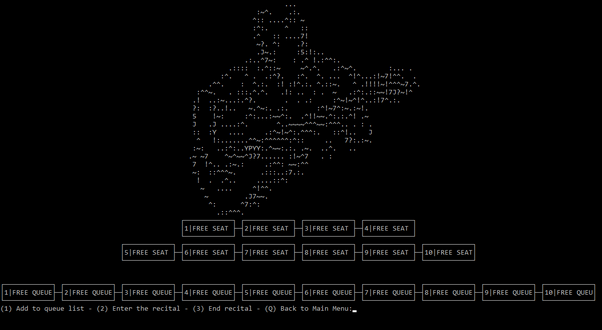\r\n\r\n\r\n## PMVCS implementation examples\r\n\r\n* [PMVCS Examples](https://github.com/gsmx64/pmvcs/tree/main/examples)\r\n\r\n\r\n## Repository\r\n\r\n* [Repository PMVCS](https://github.com/gsmx64/pmvcs)\r\n",
"bugtrack_url": null,
"license": "GNU/GPL version 3",
"summary": "Python MVC Shell Framework Package is a tiny framework for shell projects in Python.",
"version": "1.0.4",
"project_urls": {
"Bug Tracker": "https://github.com/gsmx64/pmvcs/issues",
"Homepage": "https://github.com/gsmx64/pmvcs"
},
"split_keywords": [
"pmvcs",
"python shell",
"python mvc",
"python shell framework",
"python mvc",
"python mvc shell framework"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "2070db30b1dccbe827f1ef6a7eb1531d142d1ffa0234004f141a24adfe3e4e81",
"md5": "c2e72170da9a2ab616060d572fa1a80f",
"sha256": "5bba313e0ebce5baf9cfbe3255a0154b4ef06cfa2f5b61a5e8694f6f5bbf74d8"
},
"downloads": -1,
"filename": "pmvcs-1.0.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c2e72170da9a2ab616060d572fa1a80f",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 132540,
"upload_time": "2023-10-14T22:01:05",
"upload_time_iso_8601": "2023-10-14T22:01:05.568202Z",
"url": "https://files.pythonhosted.org/packages/20/70/db30b1dccbe827f1ef6a7eb1531d142d1ffa0234004f141a24adfe3e4e81/pmvcs-1.0.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "31457f9f972e958336780cdc291300969e0dc9b4c2f5a4f971cf177fd68a9798",
"md5": "e8a06298918ca93ffea3b96ba866c1a0",
"sha256": "76798f44f51c45366b1e34c0dca49223030d679d458d1a587b048d50070ac974"
},
"downloads": -1,
"filename": "pmvcs-1.0.4.tar.gz",
"has_sig": false,
"md5_digest": "e8a06298918ca93ffea3b96ba866c1a0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 70313,
"upload_time": "2023-10-14T22:01:07",
"upload_time_iso_8601": "2023-10-14T22:01:07.240579Z",
"url": "https://files.pythonhosted.org/packages/31/45/7f9f972e958336780cdc291300969e0dc9b4c2f5a4f971cf177fd68a9798/pmvcs-1.0.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-14 22:01:07",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "gsmx64",
"github_project": "pmvcs",
"travis_ci": false,
"coveralls": true,
"github_actions": false,
"requirements": [],
"tox": true,
"lcname": "pmvcs"
}