Name | polars-bloomberg JSON |
Version |
0.4.2
JSON |
| download |
home_page | None |
Summary | Python library providing a Polars DataFrame interface for easy and intuitive access to Bloomberg API. |
upload_time | 2024-12-21 18:52:03 |
maintainer | None |
docs_url | None |
author | Marek Ozana, Ph.D. |
requires_python | >=3.11 |
license | Apache-2.0 |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
blpapi
polars
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
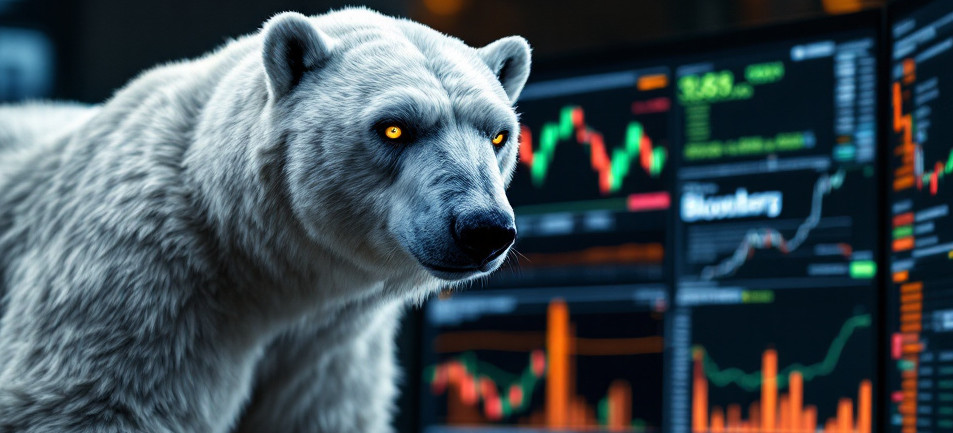
# Polars + Bloomberg Open API
[](https://github.com/MarekOzana/polars-bloomberg/actions/workflows/python-package.yml)
[](LICENSE)
**polars-bloomberg** is a Python library that extracts Bloomberg’s financial data directly into [Polars](https://www.pola.rs/) DataFrames.
If you’re a quant financial analyst, data scientist, or quant developer working in capital markets, this library makes it easy to fetch, transform, and analyze Bloomberg data right in Polars—offering speed, efficient memory usage, and a lot of fun to use!
**Why use polars-bloomberg?**
- **User-Friendly Functions:** Shortcuts like `bdp()`, `bdh()`, and `bql()` (inspired by Excel-like Bloomberg calls) let you pull data with minimal boilerplate.
- **High-Performance Analytics:** Polars is a lightning-fast DataFrame library. Combined with Bloomberg’s rich dataset, you get efficient data retrieval and minimal memory footprint
- **No Pandas Dependency:** Enjoy a clean integration that relies solely on Polars for speed and simplicity.
---
## Table of Contents
1. [Introduction](#introduction)
2. [Prerequisites](#prerequisites)
3. [Installation](#installation)
4. [Quick Start](#quick-start)
5. [Core Methods](#core-methods)
- [BDP (Bloomberg Data Point)](#bdp)
- [BDH (Bloomberg Data History)](#bdh)
- [BQL (Bloomberg Query Language)](#bql) <details><summary>BQL Examples</summary>
- [Single Item and Single Security](#1-basic-example-single-item-and-single-security)
- [Multiple Securities with Single Item](#2-multiple-securities-with-a-single-item)
- [Multiple Items](#3-multiple-items)
- [SRCH](#4-advanced-example-screening-securities)
- [Aggregation (AVG)](#average-pe-per-sector)
- [Axes](#axes)
- [Axes with All Columns](#axes-with-all-columns)
- [Segments](#segments)
- [Average Spread per Bucket](#average-issuer-oas-spread-per-maturity-bucket)
- [Technical Analysis Screening](#technical-analysis-stocks-with-20d-ema--200d-ema-and-rsi--53)
- [Bonds Universe from Equity](#bond-universe-from-equity-ticker)
- [Bonds Total Return](#bonds-total-returns)
- [Maturity Wall for US HY](#maturity-wall-for-us-hy-bonds)
</details>
6. [Additional Documentation and Resources](#additional-documentation--resources)
## Introduction
Working with Bloomberg data in Python often feels more complicated than using their well-known Excel interface.
Great projects like [blp](https://github.com/matthewgilbert/blp), [xbbg](https://github.com/alpha-xone/xbbg), and [pdblp](https://github.com/matthewgilbert/pdblp) have made this easier by pulling data directly into pandas.
With polars-bloomberg, you can enjoy the speed and simplicity of [Polars](https://www.pola.rs/) DataFrames—accessing both familiar Excel-style calls (`bdp`, `bdh`) and advanced `bql` queries—without extra pandas conversions.
For detailed documentation and function references, visit the documentation site [https://marekozana.github.io/polars-bloomberg](https://marekozana.github.io/polars-bloomberg/).
I hope you enjoy using it as much as I had fun building it!
## Prerequisites
- **Bloomberg Access:** A valid Bloomberg terminal license.
- **Bloomberg Python API:** The `blpapi` library must be installed. See the [Bloomberg API Library](https://www.bloomberg.com/professional/support/api-library/) for guidance.
- **Python Version:** Python 3.12+ recommended.
## Installation
```bash
pip install polars-bloomberg
```
# Quick Start
"Hello World" Example (under 1 minute):
```python
from polars_bloomberg import BQuery
# Fetch the latest price for Apple (AAPL US Equity)
with BQuery() as bq:
df = bq.bdp(["AAPL US Equity"], ["PX_LAST"])
print(df)
┌────────────────┬─────────┐
│ security ┆ PX_LAST │
│ --- ┆ --- │
│ str ┆ f64 │
╞════════════════╪═════════╡
│ AAPL US Equity ┆ 248.13 │
└────────────────┴─────────┘
```
What this does:
- Establishes a Bloomberg connection using the context manager.
- Retrieves the last price of Apple shares.
- Returns the result as a Polars DataFrame.
If you see a price in `df`, your setup is working 🤩!!!
## Core Methods
`BQuery` is your main interface. Using a context manager ensures the connection opens and closes cleanly. Within this session, you can use:
- `bq.bdp()` for Bloomberg Data Points (single-value fields).
- `bq.bdh()` for Historical Data (time series).
- `bq.bql()` for complex Bloomberg Query Language requests.
## BDP
Use Case: Fetch the latest single-value data points (like last price, currency, or descriptive fields).
### Example: Fetching the Last Price & Currency of Apple and SEB
```python
with BQuery() as bq:
df = bq.bdp(["AAPL US Equity", "SEBA SS Equity"], ["PX_LAST", "CRNCY"])
print(df)
┌────────────────┬─────────┬───────┐
│ security ┆ PX_LAST ┆ CRNCY │
│ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ str │
╞════════════════╪═════════╪═══════╡
│ AAPL US Equity ┆ 248.13 ┆ USD │
│ SEBA SS Equity ┆ 155.2 ┆ SEK │
└────────────────┴─────────┴───────┘
```
<details><summary>Expand for more BDP Examples</summary>
### BDP with different column types
`polars-bloomberg` correctly infers column type as shown in this example:
```python
with BQuery() as bq:
df = bq.bdp(["XS2930103580 Corp", "USX60003AC87 Corp"],
["SECURITY_DES", "YAS_ZSPREAD", "CRNCY", "NXT_CALL_DT"])
┌───────────────────┬────────────────┬─────────────┬───────┬─────────────┐
│ security ┆ SECURITY_DES ┆ YAS_ZSPREAD ┆ CRNCY ┆ NXT_CALL_DT │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ f64 ┆ str ┆ date │
╞═══════════════════╪════════════════╪═════════════╪═══════╪═════════════╡
│ XS2930103580 Corp ┆ SEB 6 3/4 PERP ┆ 304.676112 ┆ USD ┆ 2031-11-04 │
│ USX60003AC87 Corp ┆ NDAFH 6.3 PERP ┆ 292.477506 ┆ USD ┆ 2031-09-25 │
└───────────────────┴────────────────┴─────────────┴───────┴─────────────┘
```
### BDP with overrides
User can submit list of tuples with overrides
```python
with BQuery() as bq:
df = bq.bdp(
["IBM US Equity"],
["PX_LAST", "CRNCY_ADJ_PX_LAST"],
overrides=[("EQY_FUND_CRNCY", "SEK")],
)
┌───────────────┬─────────┬───────────────────┐
│ security ┆ PX_LAST ┆ CRNCY_ADJ_PX_LAST │
│ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ f64 │
╞═══════════════╪═════════╪═══════════════════╡
│ IBM US Equity ┆ 230.82 ┆ 2535.174 │
└───────────────┴─────────┴───────────────────┘
```
### BDP with date overrides
Overrides for dates has to be in format YYYYMMDD
```python
with BQuery() as bq:
df = bq.bdp(["USX60003AC87 Corp"], ["SETTLE_DT"],
overrides=[("USER_LOCAL_TRADE_DATE", "20241014")])
┌───────────────────┬────────────┐
│ security ┆ SETTLE_DT │
│ --- ┆ --- │
│ str ┆ date │
╞═══════════════════╪════════════╡
│ USX60003AC87 Corp ┆ 2024-10-15 │
└───────────────────┴────────────┘
```
```python
with BQuery() as bq:
df = bq.bdp(['USDSEK Curncy', 'SEKCZK Curncy'],
['SETTLE_DT', 'PX_LAST'],
overrides=[('REFERENCE_DATE', '20200715')]
)
┌───────────────┬────────────┬─────────┐
│ security ┆ SETTLE_DT ┆ PX_LAST │
│ --- ┆ --- ┆ --- │
│ str ┆ date ┆ f64 │
╞═══════════════╪════════════╪═════════╡
│ USDSEK Curncy ┆ 2020-07-17 ┆ 10.9778 │
│ SEKCZK Curncy ┆ 2020-07-17 ┆ 2.1698 │
└───────────────┴────────────┴─────────┘
```
</details>
## BDH
Use Case: Retrieve historical data over a date range, such as daily closing prices or volumes.
```python
with BQuery() as bq:
df = bq.bdh(
["TLT US Equity"],
["PX_LAST"],
start_date=date(2019, 1, 1),
end_date=date(2019, 1, 7),
)
print(df)
┌───────────────┬────────────┬─────────┐
│ security ┆ date ┆ PX_LAST │
│ --- ┆ --- ┆ --- │
│ str ┆ date ┆ f64 │
╞═══════════════╪════════════╪═════════╡
│ TLT US Equity ┆ 2019-01-02 ┆ 122.15 │
│ TLT US Equity ┆ 2019-01-03 ┆ 123.54 │
│ TLT US Equity ┆ 2019-01-04 ┆ 122.11 │
│ TLT US Equity ┆ 2019-01-07 ┆ 121.75 │
└───────────────┴────────────┴─────────┘
```
<details><summary>Expand for more BDH examples</summary>
### BDH with multiple securities / fields
```python
with BQuery() as bq:
df = bq.bdh(
securities=["SPY US Equity", "TLT US Equity"],
fields=["PX_LAST", "VOLUME"],
start_date=date(2019, 1, 1),
end_date=date(2019, 1, 10),
options={"adjustmentSplit": True},
)
print(df)
shape: (14, 4)
┌───────────────┬────────────┬─────────┬──────────────┐
│ security ┆ date ┆ PX_LAST ┆ VOLUME │
│ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ date ┆ f64 ┆ f64 │
╞═══════════════╪════════════╪═════════╪══════════════╡
│ SPY US Equity ┆ 2019-01-02 ┆ 250.18 ┆ 1.26925199e8 │
│ SPY US Equity ┆ 2019-01-03 ┆ 244.21 ┆ 1.44140692e8 │
│ SPY US Equity ┆ 2019-01-04 ┆ 252.39 ┆ 1.42628834e8 │
│ SPY US Equity ┆ 2019-01-07 ┆ 254.38 ┆ 1.031391e8 │
│ SPY US Equity ┆ 2019-01-08 ┆ 256.77 ┆ 1.02512587e8 │
│ … ┆ … ┆ … ┆ … │
│ TLT US Equity ┆ 2019-01-04 ┆ 122.11 ┆ 1.2970226e7 │
│ TLT US Equity ┆ 2019-01-07 ┆ 121.75 ┆ 8.498104e6 │
│ TLT US Equity ┆ 2019-01-08 ┆ 121.43 ┆ 7.737103e6 │
│ TLT US Equity ┆ 2019-01-09 ┆ 121.24 ┆ 9.349245e6 │
│ TLT US Equity ┆ 2019-01-10 ┆ 120.46 ┆ 8.22286e6 │
└───────────────┴────────────┴─────────┴──────────────┘
```
### BDH with options - periodicitySelection: Monthly
```python
with BQuery() as bq:
df = bq.bdh(['AAPL US Equity'],
['PX_LAST'],
start_date=date(2019, 1, 1),
end_date=date(2019, 3, 29),
options={"periodicitySelection": "MONTHLY"})
┌────────────────┬────────────┬─────────┐
│ security ┆ date ┆ PX_LAST │
│ --- ┆ --- ┆ --- │
│ str ┆ date ┆ f64 │
╞════════════════╪════════════╪═════════╡
│ AAPL US Equity ┆ 2019-01-31 ┆ 41.61 │
│ AAPL US Equity ┆ 2019-02-28 ┆ 43.288 │
│ AAPL US Equity ┆ 2019-03-29 ┆ 47.488 │
└────────────────┴────────────┴─────────┘
```
</details>
## BQL
*Use Case*: Run more advanced queries to screen securities, calculate analytics (like moving averages), or pull fundamental data with complex conditions.
*Returns*: The `bql()` method returns a `BqlResult` object, which:
- Acts like a list of Polars DataFrames (one for each item in BQL `get` statement).
- Provides a `.combine()` method to merge DataFrames on common columns.
### 1. Basic Example: Single Item and Single Security
```python
# Fetch the last price of IBM stock
with BQuery() as bq:
results = bq.bql("get(px_last) for(['IBM US Equity'])")
print(results[0]) # Access the first DataFrame
```
Output:
```python
┌───────────────┬─────────┬────────────┬──────────┐
│ ID ┆ px_last ┆ DATE ┆ CURRENCY │
│ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ date ┆ str │
╞═══════════════╪═════════╪════════════╪══════════╡
│ IBM US Equity ┆ 230.82 ┆ 2024-12-14 ┆ USD │
└───────────────┴─────────┴────────────┴──────────┘
```
### 2. Multiple Securities with a Single Item
```python
# Fetch the last price for IBM and SEB
with BQuery() as bq:
results = bq.bql("get(px_last) for(['IBM US Equity', 'SEBA SS Equity'])")
print(results[0])
```
Output:
```python
┌────────────────┬─────────┬────────────┬──────────┐
│ ID ┆ px_last ┆ DATE ┆ CURRENCY │
│ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ date ┆ str │
╞════════════════╪═════════╪════════════╪══════════╡
│ IBM US Equity ┆ 230.82 ┆ 2024-12-14 ┆ USD │
│ SEBA SS Equity ┆ 155.2 ┆ 2024-12-14 ┆ SEK │
└────────────────┴─────────┴────────────┴──────────┘
```
### 3. Multiple Items
When querying for multiple items, `bql()` returns a list of DataFrames
```python
# Fetch name and last price of IBM (two items)
with BQuery() as bq:
results = bq.bql("get(name, px_last) for(['IBM US Equity'])")
```
Output:
```python
>>> print(len(results)) # 2 DataFrames
n=2
>>> print(results[0]) # First DataFrame: 'name'
┌───────────────┬────────────────────────────────┐
│ ID ┆ name │
│ --- ┆ --- │
│ str ┆ str │
╞═══════════════╪════════════════════════════════╡
│ IBM US Equity ┆ International Business Machine │
└───────────────┴────────────────────────────────┘
>>> print(results[1]) # Second DataFrame: 'px_last'
┌───────────────┬─────────┬────────────┬──────────┐
│ ID ┆ px_last ┆ DATE ┆ CURRENCY │
│ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ date ┆ str │
╞═══════════════╪═════════╪════════════╪══════════╡
│ IBM US Equity ┆ 230.82 ┆ 2024-12-14 ┆ USD │
└───────────────┴─────────┴────────────┴──────────┘
```
#### Combining Results
```python
>>> combined_df = results.combine()
>>> print(combined_df)
```
Output:
```python
┌───────────────┬────────────────────────────────┬─────────┬────────────┬──────────┐
│ ID ┆ name ┆ px_last ┆ DATE ┆ CURRENCY │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ f64 ┆ date ┆ str │
╞═══════════════╪════════════════════════════════╪═════════╪════════════╪══════════╡
│ IBM US Equity ┆ International Business Machine ┆ 230.82 ┆ 2024-12-14 ┆ USD │
└───────────────┴────────────────────────────────┴─────────┴────────────┴──────────┘
```
### 4. Advanced Example: Screening Securities
Find list of SEB and Handelsbanken's AT1 bonds and print their names, duration and Z-Spread.
```python
query="""
let(#dur=duration(duration_type=MODIFIED);
#zsprd=spread(spread_type=Z);)
get(name(), #dur, #zsprd)
for(filter(screenresults(type=SRCH, screen_name='@COCO'),
ticker in ['SEB', 'SHBASS']))
"""
with BQuery() as bq:
results = bq.bql(query)
combined_df = results.combine()
print(combined_df)
```
Output:
```python
┌───────────────┬─────────────────┬──────┬────────────┬────────┐
│ ID ┆ name() ┆ #dur ┆ DATE ┆ #zsprd │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ f64 ┆ date ┆ f64 │
╞═══════════════╪═════════════════╪══════╪════════════╪════════╡
│ BW924993 Corp ┆ SEB 6 ⅞ PERP ┆ 2.23 ┆ 2024-12-16 ┆ 212.0 │
│ YV402592 Corp ┆ SEB Float PERP ┆ 0.21 ┆ 2024-12-16 ┆ 233.0 │
│ ZQ349286 Corp ┆ SEB 5 ⅛ PERP ┆ 0.39 ┆ 2024-12-16 ┆ 186.0 │
│ ZO703315 Corp ┆ SHBASS 4 ⅜ PERP ┆ 1.95 ┆ 2024-12-16 ┆ 213.0 │
│ ZO703956 Corp ┆ SHBASS 4 ¾ PERP ┆ 4.94 ┆ 2024-12-16 ┆ 256.0 │
│ YU819930 Corp ┆ SEB 6 ¾ PERP ┆ 5.37 ┆ 2024-12-16 ┆ 309.0 │
└───────────────┴─────────────────┴──────┴────────────┴────────┘
```
### Average PE per Sector
This example shows aggregation (average) per group (sector) for members of an index.
The resulting list has only one element since there is only one data-item in `get`
```python
query = """
let(#avg_pe=avg(group(pe_ratio(), gics_sector_name()));)
get(#avg_pe)
for(members('OMX Index'))
"""
with BQuery() as bq:
results = bq.bql(query)
print(results[0].head(5))
```
Output:
```python
┌──────────────┬───────────┬──────────────┬────────────┬──────────────┬──────────────┬─────────────┐
│ ID ┆ #avg_pe ┆ REVISION_DAT ┆ AS_OF_DATE ┆ PERIOD_END_D ┆ ORIG_IDS ┆ GICS_SECTOR │
│ --- ┆ --- ┆ E ┆ --- ┆ ATE ┆ --- ┆ _NAME() │
│ str ┆ f64 ┆ --- ┆ date ┆ --- ┆ str ┆ --- │
│ ┆ ┆ date ┆ ┆ date ┆ ┆ str │
╞══════════════╪═══════════╪══════════════╪════════════╪══════════════╪══════════════╪═════════════╡
│ Communicatio ┆ 19.561754 ┆ 2024-10-24 ┆ 2024-12-14 ┆ 2024-09-30 ┆ null ┆ Communicati │
│ n Services ┆ ┆ ┆ ┆ ┆ ┆ on Services │
│ Consumer Dis ┆ 19.117295 ┆ 2024-10-24 ┆ 2024-12-14 ┆ 2024-09-30 ┆ null ┆ Consumer │
│ cretionary ┆ ┆ ┆ ┆ ┆ ┆ Discretiona │
│ ┆ ┆ ┆ ┆ ┆ ┆ ry │
│ Consumer ┆ 15.984743 ┆ 2024-10-24 ┆ 2024-12-14 ┆ 2024-09-30 ┆ ESSITYB SS ┆ Consumer │
│ Staples ┆ ┆ ┆ ┆ ┆ Equity ┆ Staples │
│ Financials ┆ 6.815895 ┆ 2024-10-24 ┆ 2024-12-14 ┆ 2024-09-30 ┆ null ┆ Financials │
│ Health Care ┆ 22.00628 ┆ 2024-11-12 ┆ 2024-12-14 ┆ 2024-09-30 ┆ null ┆ Health Care │
└──────────────┴───────────┴──────────────┴────────────┴──────────────┴──────────────┴─────────────┘
```
### Axes
Get current axes of all Swedish USD AT1 bonds
```python
# Get current axes for Swedish AT1 bonds in USD
query="""
let(#ax=axes();)
get(security_des, #ax)
for(filter(bondsuniv(ACTIVE),
crncy()=='USD' and
basel_iii_designation() == 'Additional Tier 1' and
country_iso() == 'SE'))
"""
with BQuery() as bq:
results = bq.bql(query)
print(results.combine())
┌───────────────┬─────────────────┬─────┬───────────┬───────────┬────────────────┬────────────────┐
│ ID ┆ security_des ┆ #ax ┆ ASK_DEPTH ┆ BID_DEPTH ┆ ASK_TOTAL_SIZE ┆ BID_TOTAL_SIZE │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ str ┆ i64 ┆ i64 ┆ f64 ┆ f64 │
╞═══════════════╪═════════════════╪═════╪═══════════╪═══════════╪════════════════╪════════════════╡
│ YU819930 Corp ┆ SEB 6 ¾ PERP ┆ Y ┆ 2 ┆ null ┆ 5.6e6 ┆ null │
│ ZO703315 Corp ┆ SHBASS 4 ⅜ PERP ┆ Y ┆ 1 ┆ 2 ┆ 5e6 ┆ 6e6 │
│ BR069680 Corp ┆ SWEDA 4 PERP ┆ Y ┆ null ┆ 1 ┆ null ┆ 3e6 │
│ ZL122341 Corp ┆ SWEDA 7 ⅝ PERP ┆ Y ┆ null ┆ 6 ┆ null ┆ 2.04e7 │
│ ZQ349286 Corp ┆ SEB 5 ⅛ PERP ┆ Y ┆ 2 ┆ 4 ┆ 5.5e6 ┆ 3e7 │
│ ZF859199 Corp ┆ SWEDA 7 ¾ PERP ┆ Y ┆ 1 ┆ 1 ┆ 2e6 ┆ 2e6 │
│ ZO703956 Corp ┆ SHBASS 4 ¾ PERP ┆ Y ┆ 1 ┆ 3 ┆ 1.2e6 ┆ 1.1e7 │
│ BW924993 Corp ┆ SEB 6 ⅞ PERP ┆ Y ┆ 1 ┆ 3 ┆ 5e6 ┆ 1.1e7 │
└───────────────┴─────────────────┴─────┴───────────┴───────────┴────────────────┴────────────────┘
```
### Axes with all columns
```python
# RT1 Axes with all columns
query = """
let(#ax=axes();)
get(name, #ax, amt_outstanding)
for(filter(bondsuniv(ACTIVE),
crncy() in ['USD', 'EUR'] and
solvency_ii_designation() == 'Restricted Tier 1' and
amt_outstanding() > 7.5e8 and
is_axed('Bid') == True))
preferences(addcols=all)
"""
with BQuery() as bq:
results = bq.bql(query)
print(results.combine())
```
Output:
<div>
<small>shape: (3, 33)</small><table border="1" class="dataframe"><thead><tr><th>ID</th><th>name</th><th>#ax</th><th>ASK_PRICE</th><th>BID_PRICE</th><th>ASK_DEPTH</th><th>BID_DEPTH</th><th>ASK_DEALER</th><th>BID_DEALER</th><th>ASK_SIZE</th><th>BID_SIZE</th><th>ASK_TOTAL_SIZE</th><th>BID_TOTAL_SIZE</th><th>ASK_PRICE_IS_DERIVED</th><th>BID_PRICE_IS_DERIVED</th><th>ASK_SPREAD</th><th>BID_SPREAD</th><th>ASK_SPREAD_IS_DERIVED</th><th>BID_SPREAD_IS_DERIVED</th><th>ASK_YIELD</th><th>BID_YIELD</th><th>ASK_YIELD_IS_DERIVED</th><th>BID_YIELD_IS_DERIVED</th><th>ASK_AXE_SOURCE</th><th>BID_AXE_SOURCE</th><th>ASK_BROKER</th><th>BID_BROKER</th><th>ASK_HIST_AGG_SIZE</th><th>BID_HIST_AGG_SIZE</th><th>amt_outstanding</th><th>CURRENCY_OF_ISSUE</th><th>MULTIPLIER</th><th>CURRENCY</th></tr><tr><td>str</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>i64</td><td>i64</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>str</td><td>str</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>f64</td><td>str</td><td>f64</td><td>str</td></tr></thead><tbody><tr><td>"BM368057 Corp"</td><td>"ALVGR 2 ⅝ PERP"</td><td>"Y"</td><td>88.034</td><td>87.427</td><td>5</td><td>1</td><td>"BARC"</td><td>"IMI"</td><td>1.2e6</td><td>1e6</td><td>7.2e6</td><td>1e6</td><td>null</td><td>null</td><td>287.031</td><td>300.046</td><td>true</td><td>true</td><td>4.854</td><td>4.976</td><td>true</td><td>true</td><td>"ERUN"</td><td>"ERUN"</td><td>"BXOL"</td><td>"IMIC"</td><td>6.68e6</td><td>8.92e6</td><td>1.2500e9</td><td>"EUR"</td><td>1.0</td><td>"EUR"</td></tr><tr><td>"EK588238 Corp"</td><td>"ASSGEN 4.596 PERP"</td><td>"Y"</td><td>101.0</td><td>100.13</td><td>4</td><td>6</td><td>"MSAX"</td><td>"A2A"</td><td>500000.0</td><td>100000.0</td><td>1.556e7</td><td>3.83e7</td><td>null</td><td>null</td><td>108.9</td><td>207.889</td><td>true</td><td>true</td><td>3.466</td><td>4.434</td><td>null</td><td>true</td><td>"ERUN"</td><td>"BBX"</td><td>"MSAX"</td><td>"A2A"</td><td>1.70424e7</td><td>3.17e7</td><td>1.0004e9</td><td>"EUR"</td><td>1.0</td><td>"EUR"</td></tr><tr><td>"BR244025 Corp"</td><td>"ALVGR 3.2 PERP"</td><td>"Y"</td><td>88.0</td><td>86.875</td><td>3</td><td>4</td><td>"UBS"</td><td>"DB"</td><td>5e6</td><td>1e6</td><td>1.1e7</td><td>1.4e7</td><td>null</td><td>null</td><td>49.33</td><td>414.602</td><td>true</td><td>true</td><td>7.34258</td><td>8.553</td><td>null</td><td>true</td><td>"ERUN"</td><td>"ERUN"</td><td>"UBSW"</td><td>"DABC"</td><td>1.6876e6</td><td>3.6e7</td><td>1.2500e9</td><td>"USD"</td><td>1.0</td><td>"USD"</td></tr></tbody></table></div>
### Segments
The following example shows handling of two data-items with different length. The first dataframe
describes the segments (and has length 5 in this case), while the second dataframe contains time series.
One can join the dataframes on common columns and pivot the segments into columns as shown below:
```python
# revenue per segment
query = """
let(#segment=segment_name();
#revenue=sales_Rev_turn(fpt=q, fpr=range(2023Q3, 2024Q3));
)
get(#segment, #revenue)
for(segments('GTN US Equity',type=reported,hierarchy=PRODUCT, level=1))
"""
with BQuery() as bq:
results = bq.bql(query)
df = results.combine().pivot(
index="PERIOD_END_DATE", on="#segment", values="#revenue"
)
print(df)
```
Output:
```python
┌─────────────────┬──────────────┬──────────────────────┬────────┬────────────┐
│ PERIOD_END_DATE ┆ Broadcasting ┆ Production Companies ┆ Other ┆ Adjustment │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ date ┆ f64 ┆ f64 ┆ f64 ┆ f64 │
╞═════════════════╪══════════════╪══════════════════════╪════════╪════════════╡
│ 2023-09-30 ┆ 7.83e8 ┆ 2e7 ┆ 1.6e7 ┆ null │
│ 2023-12-31 ┆ 8.13e8 ┆ 3.2e7 ┆ 1.9e7 ┆ null │
│ 2024-03-31 ┆ 7.8e8 ┆ 2.4e7 ┆ 1.9e7 ┆ null │
│ 2024-06-30 ┆ 8.08e8 ┆ 1.8e7 ┆ 0.0 ┆ null │
│ 2024-09-30 ┆ 9.24e8 ┆ 2.6e7 ┆ 1.7e7 ┆ null │
└─────────────────┴──────────────┴──────────────────────┴────────┴────────────┘
```
### Actual and Forward EPS Estimates
```python
with BQuery() as bq:
results = bq.bql("""
let(#eps=is_eps(fa_period_type='A',
fa_period_offset=range(-4,2));)
get(#eps)
for(['IBM US Equity'])
""")
print(results[0])
┌───────────────┬───────┬───────────────┬────────────┬─────────────────┬──────────┐
│ ID ┆ #eps ┆ REVISION_DATE ┆ AS_OF_DATE ┆ PERIOD_END_DATE ┆ CURRENCY │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ date ┆ date ┆ date ┆ str │
╞═══════════════╪═══════╪═══════════════╪════════════╪═════════════════╪══════════╡
│ IBM US Equity ┆ 10.63 ┆ 2022-02-22 ┆ 2024-12-14 ┆ 2019-12-31 ┆ USD │
│ IBM US Equity ┆ 6.28 ┆ 2023-02-28 ┆ 2024-12-14 ┆ 2020-12-31 ┆ USD │
│ IBM US Equity ┆ 6.41 ┆ 2023-02-28 ┆ 2024-12-14 ┆ 2021-12-31 ┆ USD │
│ IBM US Equity ┆ 1.82 ┆ 2024-03-18 ┆ 2024-12-14 ┆ 2022-12-31 ┆ USD │
│ IBM US Equity ┆ 8.23 ┆ 2024-03-18 ┆ 2024-12-14 ┆ 2023-12-31 ┆ USD │
│ IBM US Equity ┆ 7.891 ┆ 2024-12-13 ┆ 2024-12-14 ┆ 2024-12-31 ┆ USD │
│ IBM US Equity ┆ 9.236 ┆ 2024-12-13 ┆ 2024-12-14 ┆ 2025-12-31 ┆ USD │
└───────────────┴───────┴───────────────┴────────────┴─────────────────┴──────────┘
```
### Average issuer OAS spread per maturity bucket
```python
# Example: Average OAS-spread per maturity bucket
query = """
let(
#bins = bins(maturity_years,
[3,9,18,30],
['(1) 0-3','(2) 3-9','(3) 9-18','(4) 18-30','(5) 30+']);
#average_spread = avg(group(spread(st=oas),#bins));
)
get(#average_spread)
for(filter(bonds('NVDA US Equity', issuedby = 'ENTITY'),
maturity_years != NA))
"""
with BQuery() as bq:
results = bq.bql(query)
print(results[0])
```
Output:
```python
┌───────────┬─────────────────┬────────────┬───────────────┬───────────┐
│ ID ┆ #average_spread ┆ DATE ┆ ORIG_IDS ┆ #BINS │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ f64 ┆ date ┆ str ┆ str │
╞═══════════╪═════════════════╪════════════╪═══════════════╪═══════════╡
│ (1) 0-3 ┆ 31.195689 ┆ 2024-12-14 ┆ QZ552396 Corp ┆ (1) 0-3 │
│ (2) 3-9 ┆ 59.580383 ┆ 2024-12-14 ┆ null ┆ (2) 3-9 │
│ (3) 9-18 ┆ 110.614416 ┆ 2024-12-14 ┆ BH393780 Corp ┆ (3) 9-18 │
│ (4) 18-30 ┆ 135.160279 ┆ 2024-12-14 ┆ BH393781 Corp ┆ (4) 18-30 │
│ (5) 30+ ┆ 150.713405 ┆ 2024-12-14 ┆ BH393782 Corp ┆ (5) 30+ │
└───────────┴─────────────────┴────────────┴───────────────┴───────────┘
```
### Technical Analysis: stocks with 20d EMA > 200d EMA and RSI > 53
```python
with BQuery() as bq:
results = bq.bql(
"""
let(#ema20=emavg(period=20);
#ema200=emavg(period=200);
#rsi=rsi(close=px_last());)
get(name(), #ema20, #ema200, #rsi)
for(filter(members('OMX Index'),
and(#ema20 > #ema200, #rsi > 53)))
with(fill=PREV)
"""
)
print(results.combine())
```
Output:
```python
┌─────────────────┬──────────────────┬────────────┬────────────┬──────────┬────────────┬───────────┐
│ ID ┆ name() ┆ #ema20 ┆ DATE ┆ CURRENCY ┆ #ema200 ┆ #rsi │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ f64 ┆ date ┆ str ┆ f64 ┆ f64 │
╞═════════════════╪══════════════════╪════════════╪════════════╪══════════╪════════════╪═══════════╡
│ ERICB SS Equity ┆ Telefonaktiebola ┆ 90.152604 ┆ 2024-12-16 ┆ SEK ┆ 75.072151 ┆ 56.010028 │
│ ┆ get LM Ericsso ┆ ┆ ┆ ┆ ┆ │
│ ABB SS Equity ┆ ABB Ltd ┆ 630.622469 ┆ 2024-12-16 ┆ SEK ┆ 566.571183 ┆ 53.763102 │
│ SEBA SS Equity ┆ Skandinaviska ┆ 153.80595 ┆ 2024-12-16 ┆ SEK ┆ 150.742394 ┆ 56.460733 │
│ ┆ Enskilda Banken ┆ ┆ ┆ ┆ ┆ │
│ ASSAB SS Equity ┆ Assa Abloy AB ┆ 339.017591 ┆ 2024-12-16 ┆ SEK ┆ 317.057573 ┆ 53.351619 │
└─────────────────┴──────────────────┴────────────┴────────────┴──────────┴────────────┴───────────┘
```
### Bond Universe from Equity Ticker
```python
# Get Bond Universe from Equity Ticker
query = """
let(#rank=normalized_payment_rank();
#oas=spread(st=oas);
#nxt_call=nxt_call_dt();
)
get(name(), #rank, #nxt_call, #oas)
for(filter(bonds('GTN US Equity'), series() == '144A'))
"""
with BQuery() as bq:
results = bq.bql(query)
df = results.combine()
print(df)
```
Output:
```
┌───────────────┬───────────────────┬──────────────────┬────────────┬────────────┬────────────┐
│ ID ┆ name() ┆ #rank ┆ #nxt_call ┆ #oas ┆ DATE │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ str ┆ str ┆ str ┆ date ┆ f64 ┆ date │
╞═══════════════╪═══════════════════╪══════════════════╪════════════╪════════════╪════════════╡
│ YX231113 Corp ┆ GTN 10 ½ 07/15/29 ┆ 1st Lien Secured ┆ 2026-07-15 ┆ 598.66491 ┆ 2024-12-17 │
│ BS116983 Corp ┆ GTN 5 ⅜ 11/15/31 ┆ Sr Unsecured ┆ 2026-11-15 ┆ 1193.17529 ┆ 2024-12-17 │
│ AV438089 Corp ┆ GTN 7 05/15/27 ┆ Sr Unsecured ┆ 2024-12-24 ┆ 400.340456 ┆ 2024-12-17 │
│ ZO860846 Corp ┆ GTN 4 ¾ 10/15/30 ┆ Sr Unsecured ┆ 2025-10-15 ┆ 1249.34346 ┆ 2024-12-17 │
│ LW375188 Corp ┆ GTN 5 ⅞ 07/15/26 ┆ Sr Unsecured ┆ 2025-01-13 ┆ 173.761744 ┆ 2024-12-17 │
└───────────────┴───────────────────┴──────────────────┴────────────┴────────────┴────────────┘
```
### Bonds Total Returns
This is example of a single-item query returning total return for all GTN bonds in a long dataframe.
We can easily pivot it into wide format, as in the example below
```python
# Total Return of GTN Bonds
query = """
let(#rng = range(-1M, 0D);
#rets = return_series(calc_interval=#rng,per=W);)
get(#rets)
for(filter(bonds('GTN US Equity'), series() == '144A'))
"""
with BQuery() as bq:
results = bq.bql(query)
df = results[0].pivot(on="ID", index="DATE", values="#rets")
print(df)
```
Output:
```python
shape: (6, 6)
┌────────────┬───────────────┬───────────────┬───────────────┬───────────────┬───────────────┐
│ DATE ┆ YX231113 Corp ┆ BS116983 Corp ┆ AV438089 Corp ┆ ZO860846 Corp ┆ LW375188 Corp │
│ --- ┆ --- ┆ --- ┆ --- ┆ --- ┆ --- │
│ date ┆ f64 ┆ f64 ┆ f64 ┆ f64 ┆ f64 │
╞════════════╪═══════════════╪═══════════════╪═══════════════╪═══════════════╪═══════════════╡
│ 2024-11-17 ┆ null ┆ null ┆ null ┆ null ┆ null │
│ 2024-11-24 ┆ 0.001653 ┆ 0.051179 ┆ 0.020363 ┆ 0.001371 ┆ -0.002939 │
│ 2024-12-01 ┆ 0.002837 ┆ 0.010405 ┆ -0.001466 ┆ 0.007275 ┆ 0.000581 │
│ 2024-12-08 ┆ -0.000041 ┆ 0.016145 ┆ 0.000766 ┆ 0.024984 ┆ 0.000936 │
│ 2024-12-15 ┆ 0.001495 ┆ -0.047 ┆ -0.000233 ┆ -0.043509 ┆ 0.002241 │
│ 2024-12-17 ┆ 0.00008 ┆ -0.000004 ┆ -0.0035 ┆ -0.007937 ┆ 0.000064 │
└────────────┴───────────────┴───────────────┴───────────────┴───────────────┴───────────────┘
```
### Maturity Wall for US HY Bonds
```python
query = """
let(#mv=sum(group(amt_outstanding(currency=USD),
by=[year(maturity()), industry_sector()]));)
get(#mv)
for(members('LF98TRUU Index'))
"""
with BQuery() as bq:
results = bq.bql(query)
df = results.combine().rename(
{"YEAR(MATURITY())": "maturity", "INDUSTRY_SECTOR()": "sector", "#mv": "mv"}
)
print(df.pivot(index="maturity", on="sector", values="mv").head())
```
Output:
```python
shape: (5, 11)
┌──────────┬───────────┬───────────┬───────────┬───┬───────────┬───────────┬───────────┬───────────┐
│ maturity ┆ Basic ┆ Consumer, ┆ Energy ┆ … ┆ Financial ┆ Technolog ┆ Utilities ┆ Diversifi │
│ --- ┆ Materials ┆ Non-cycli ┆ --- ┆ ┆ --- ┆ y ┆ --- ┆ ed │
│ i64 ┆ --- ┆ cal ┆ f64 ┆ ┆ f64 ┆ --- ┆ f64 ┆ --- │
│ ┆ f64 ┆ --- ┆ ┆ ┆ ┆ f64 ┆ ┆ f64 │
│ ┆ ┆ f64 ┆ ┆ ┆ ┆ ┆ ┆ │
╞══════════╪═══════════╪═══════════╪═══════════╪═══╪═══════════╪═══════════╪═══════════╪═══════════╡
│ 2025 ┆ 1.5e8 ┆ 5.34916e8 ┆ 5e8 ┆ … ┆ null ┆ null ┆ null ┆ null │
│ 2026 ┆ 4.4013e9 ┆ 9.3293e9 ┆ 8.2931e9 ┆ … ┆ 1.3524e10 ┆ 4.0608e9 ┆ 2.5202e9 ┆ null │
│ 2027 ┆ 8.3921e9 ┆ 2.3409e10 ┆ 1.2427e10 ┆ … ┆ 1.9430e10 ┆ 4.3367e9 ┆ 3.6620e9 ┆ null │
│ 2028 ┆ 1.4701e10 ┆ 3.7457e10 ┆ 2.2442e10 ┆ … ┆ 2.3341e10 ┆ 9.9143e9 ┆ 7.6388e9 ┆ 5e8 │
│ 2029 ┆ 1.6512e10 ┆ 5.7381e10 ┆ 3.9286e10 ┆ … ┆ 4.2337e10 ┆ 2.2660e10 ┆ 5.8558e9 ┆ null │
└──────────┴───────────┴───────────┴───────────┴───┴───────────┴───────────┴───────────┴───────────┘
```
## Additional Documentation & Resources
- *API Documentation*: Detailed documentation and function references are available in https://marekozana.github.io/polars-bloomberg
- *Additional Examples*: Check out [examples/](examples/) for hands-on notebooks demonstrating a variety of use cases.
- BQL examples and use cases: [examples/Examples-BQL.ipynb](https://github.com/MarekOzana/polars-bloomberg/blob/main/examples/Examples-BQL.ipynb)
- *Bloomberg Developer Resources*: For more details on the Bloomberg API itself, visit the [Bloomberg Developer's page](https://developer.bloomberg.com/).
Raw data
{
"_id": null,
"home_page": null,
"name": "polars-bloomberg",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.11",
"maintainer_email": null,
"keywords": null,
"author": "Marek Ozana, Ph.D.",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/03/ab/10b62c398a358f545bcc8284e2f963c99931f45a41f00e71f4c5f105a1c0/polars_bloomberg-0.4.2.tar.gz",
"platform": null,
"description": "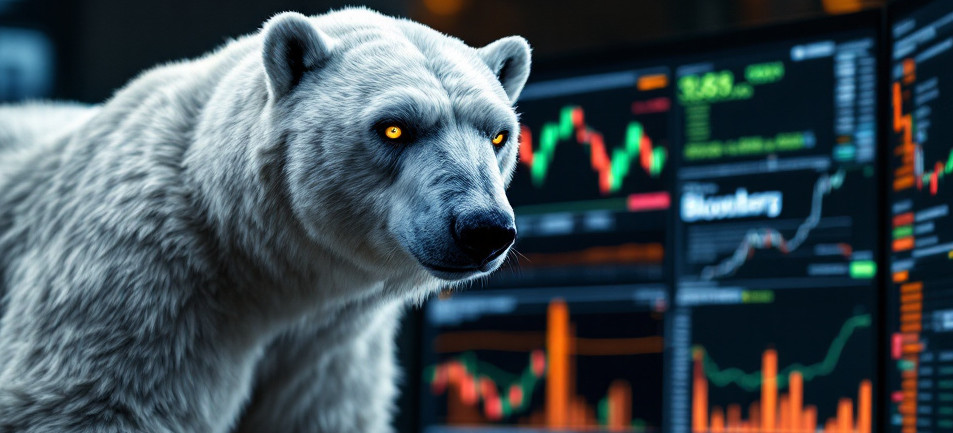\r\n\r\n# Polars + Bloomberg Open API\r\n[](https://github.com/MarekOzana/polars-bloomberg/actions/workflows/python-package.yml)\r\n[](LICENSE)\r\n\r\n**polars-bloomberg** is a Python library that extracts Bloomberg\u2019s financial data directly into [Polars](https://www.pola.rs/) DataFrames. \r\nIf you\u2019re a quant financial analyst, data scientist, or quant developer working in capital markets, this library makes it easy to fetch, transform, and analyze Bloomberg data right in Polars\u2014offering speed, efficient memory usage, and a lot of fun to use!\r\n\r\n**Why use polars-bloomberg?**\r\n\r\n- **User-Friendly Functions:** Shortcuts like `bdp()`, `bdh()`, and `bql()` (inspired by Excel-like Bloomberg calls) let you pull data with minimal boilerplate.\r\n- **High-Performance Analytics:** Polars is a lightning-fast DataFrame library. Combined with Bloomberg\u2019s rich dataset, you get efficient data retrieval and minimal memory footprint\r\n- **No Pandas Dependency:** Enjoy a clean integration that relies solely on Polars for speed and simplicity.\r\n\r\n---\r\n\r\n## Table of Contents\r\n\r\n1. [Introduction](#introduction)\r\n2. [Prerequisites](#prerequisites)\r\n3. [Installation](#installation)\r\n4. [Quick Start](#quick-start)\r\n5. [Core Methods](#core-methods)\r\n - [BDP (Bloomberg Data Point)](#bdp)\r\n - [BDH (Bloomberg Data History)](#bdh)\r\n - [BQL (Bloomberg Query Language)](#bql) <details><summary>BQL Examples</summary>\r\n - [Single Item and Single Security](#1-basic-example-single-item-and-single-security)\r\n - [Multiple Securities with Single Item](#2-multiple-securities-with-a-single-item)\r\n - [Multiple Items](#3-multiple-items)\r\n - [SRCH](#4-advanced-example-screening-securities)\r\n - [Aggregation (AVG)](#average-pe-per-sector)\r\n - [Axes](#axes)\r\n - [Axes with All Columns](#axes-with-all-columns)\r\n - [Segments](#segments)\r\n - [Average Spread per Bucket](#average-issuer-oas-spread-per-maturity-bucket)\r\n - [Technical Analysis Screening](#technical-analysis-stocks-with-20d-ema--200d-ema-and-rsi--53)\r\n - [Bonds Universe from Equity](#bond-universe-from-equity-ticker)\r\n - [Bonds Total Return](#bonds-total-returns)\r\n - [Maturity Wall for US HY](#maturity-wall-for-us-hy-bonds)\r\n </details>\r\n6. [Additional Documentation and Resources](#additional-documentation--resources)\r\n\r\n## Introduction\r\nWorking with Bloomberg data in Python often feels more complicated than using their well-known Excel interface.\r\nGreat projects like [blp](https://github.com/matthewgilbert/blp), [xbbg](https://github.com/alpha-xone/xbbg), and [pdblp](https://github.com/matthewgilbert/pdblp) have made this easier by pulling data directly into pandas. \r\n\r\nWith polars-bloomberg, you can enjoy the speed and simplicity of [Polars](https://www.pola.rs/) DataFrames\u2014accessing both familiar Excel-style calls (`bdp`, `bdh`) and advanced `bql` queries\u2014without extra pandas conversions. \r\n\r\nFor detailed documentation and function references, visit the documentation site [https://marekozana.github.io/polars-bloomberg](https://marekozana.github.io/polars-bloomberg/).\r\n\r\nI hope you enjoy using it as much as I had fun building it!\r\n\r\n\r\n## Prerequisites\r\n\r\n- **Bloomberg Access:** A valid Bloomberg terminal license.\r\n- **Bloomberg Python API:** The `blpapi` library must be installed. See the [Bloomberg API Library](https://www.bloomberg.com/professional/support/api-library/) for guidance.\r\n- **Python Version:** Python 3.12+ recommended.\r\n\r\n## Installation\r\n\r\n```bash\r\npip install polars-bloomberg\r\n```\r\n\r\n# Quick Start\r\n\"Hello World\" Example (under 1 minute):\r\n```python\r\nfrom polars_bloomberg import BQuery\r\n\r\n# Fetch the latest price for Apple (AAPL US Equity)\r\nwith BQuery() as bq:\r\n df = bq.bdp([\"AAPL US Equity\"], [\"PX_LAST\"])\r\n print(df)\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 PX_LAST \u2502\r\n\u2502 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 AAPL US Equity \u2506 248.13 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\nWhat this does:\r\n- Establishes a Bloomberg connection using the context manager.\r\n- Retrieves the last price of Apple shares.\r\n- Returns the result as a Polars DataFrame.\r\n\r\nIf you see a price in `df`, your setup is working \ud83e\udd29!!!\r\n\r\n## Core Methods\r\n`BQuery` is your main interface. Using a context manager ensures the connection opens and closes cleanly. Within this session, you can use:\r\n- `bq.bdp()` for Bloomberg Data Points (single-value fields).\r\n- `bq.bdh()` for Historical Data (time series).\r\n- `bq.bql()` for complex Bloomberg Query Language requests.\r\n\r\n## BDP\r\nUse Case: Fetch the latest single-value data points (like last price, currency, or descriptive fields).\r\n\r\n### Example: Fetching the Last Price & Currency of Apple and SEB\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdp([\"AAPL US Equity\", \"SEBA SS Equity\"], [\"PX_LAST\", \"CRNCY\"])\r\n print(df)\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 PX_LAST \u2506 CRNCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 AAPL US Equity \u2506 248.13 \u2506 USD \u2502\r\n\u2502 SEBA SS Equity \u2506 155.2 \u2506 SEK \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n<details><summary>Expand for more BDP Examples</summary>\r\n\r\n### BDP with different column types\r\n\r\n`polars-bloomberg` correctly infers column type as shown in this example:\r\n\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdp([\"XS2930103580 Corp\", \"USX60003AC87 Corp\"],\r\n [\"SECURITY_DES\", \"YAS_ZSPREAD\", \"CRNCY\", \"NXT_CALL_DT\"])\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 SECURITY_DES \u2506 YAS_ZSPREAD \u2506 CRNCY \u2506 NXT_CALL_DT \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 f64 \u2506 str \u2506 date \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 XS2930103580 Corp \u2506 SEB 6 3/4 PERP \u2506 304.676112 \u2506 USD \u2506 2031-11-04 \u2502\r\n\u2502 USX60003AC87 Corp \u2506 NDAFH 6.3 PERP \u2506 292.477506 \u2506 USD \u2506 2031-09-25 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### BDP with overrides\r\nUser can submit list of tuples with overrides\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdp(\r\n [\"IBM US Equity\"],\r\n [\"PX_LAST\", \"CRNCY_ADJ_PX_LAST\"],\r\n overrides=[(\"EQY_FUND_CRNCY\", \"SEK\")],\r\n )\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 PX_LAST \u2506 CRNCY_ADJ_PX_LAST \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 230.82 \u2506 2535.174 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### BDP with date overrides\r\nOverrides for dates has to be in format YYYYMMDD\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdp([\"USX60003AC87 Corp\"], [\"SETTLE_DT\"],\r\n overrides=[(\"USER_LOCAL_TRADE_DATE\", \"20241014\")])\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 SETTLE_DT \u2502\r\n\u2502 --- \u2506 --- \u2502\r\n\u2502 str \u2506 date \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 USX60003AC87 Corp \u2506 2024-10-15 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdp(['USDSEK Curncy', 'SEKCZK Curncy'], \r\n ['SETTLE_DT', 'PX_LAST'], \r\n overrides=[('REFERENCE_DATE', '20200715')]\r\n )\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 SETTLE_DT \u2506 PX_LAST \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 date \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 USDSEK Curncy \u2506 2020-07-17 \u2506 10.9778 \u2502\r\n\u2502 SEKCZK Curncy \u2506 2020-07-17 \u2506 2.1698 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n</details>\r\n\r\n## BDH\r\nUse Case: Retrieve historical data over a date range, such as daily closing prices or volumes.\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdh(\r\n [\"TLT US Equity\"],\r\n [\"PX_LAST\"],\r\n start_date=date(2019, 1, 1),\r\n end_date=date(2019, 1, 7),\r\n )\r\n print(df)\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 date \u2506 PX_LAST \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 date \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 TLT US Equity \u2506 2019-01-02 \u2506 122.15 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-03 \u2506 123.54 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-04 \u2506 122.11 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-07 \u2506 121.75 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n<details><summary>Expand for more BDH examples</summary>\r\n\r\n### BDH with multiple securities / fields\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdh(\r\n securities=[\"SPY US Equity\", \"TLT US Equity\"],\r\n fields=[\"PX_LAST\", \"VOLUME\"],\r\n start_date=date(2019, 1, 1),\r\n end_date=date(2019, 1, 10),\r\n options={\"adjustmentSplit\": True},\r\n )\r\n print(df)\r\n\r\nshape: (14, 4)\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 date \u2506 PX_LAST \u2506 VOLUME \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 date \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 SPY US Equity \u2506 2019-01-02 \u2506 250.18 \u2506 1.26925199e8 \u2502\r\n\u2502 SPY US Equity \u2506 2019-01-03 \u2506 244.21 \u2506 1.44140692e8 \u2502\r\n\u2502 SPY US Equity \u2506 2019-01-04 \u2506 252.39 \u2506 1.42628834e8 \u2502\r\n\u2502 SPY US Equity \u2506 2019-01-07 \u2506 254.38 \u2506 1.031391e8 \u2502\r\n\u2502 SPY US Equity \u2506 2019-01-08 \u2506 256.77 \u2506 1.02512587e8 \u2502\r\n\u2502 \u2026 \u2506 \u2026 \u2506 \u2026 \u2506 \u2026 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-04 \u2506 122.11 \u2506 1.2970226e7 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-07 \u2506 121.75 \u2506 8.498104e6 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-08 \u2506 121.43 \u2506 7.737103e6 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-09 \u2506 121.24 \u2506 9.349245e6 \u2502\r\n\u2502 TLT US Equity \u2506 2019-01-10 \u2506 120.46 \u2506 8.22286e6 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### BDH with options - periodicitySelection: Monthly\r\n```python\r\nwith BQuery() as bq:\r\n df = bq.bdh(['AAPL US Equity'], \r\n ['PX_LAST'], \r\n start_date=date(2019, 1, 1), \r\n end_date=date(2019, 3, 29),\r\n options={\"periodicitySelection\": \"MONTHLY\"})\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 security \u2506 date \u2506 PX_LAST \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 date \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 AAPL US Equity \u2506 2019-01-31 \u2506 41.61 \u2502\r\n\u2502 AAPL US Equity \u2506 2019-02-28 \u2506 43.288 \u2502\r\n\u2502 AAPL US Equity \u2506 2019-03-29 \u2506 47.488 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n</details>\r\n\r\n\r\n## BQL\r\n*Use Case*: Run more advanced queries to screen securities, calculate analytics (like moving averages), or pull fundamental data with complex conditions.\r\n\r\n*Returns*: The `bql()` method returns a `BqlResult` object, which:\r\n- Acts like a list of Polars DataFrames (one for each item in BQL `get` statement).\r\n- Provides a `.combine()` method to merge DataFrames on common columns.\r\n\r\n### 1. Basic Example: Single Item and Single Security\r\n```python\r\n# Fetch the last price of IBM stock\r\nwith BQuery() as bq:\r\n results = bq.bql(\"get(px_last) for(['IBM US Equity'])\")\r\n print(results[0]) # Access the first DataFrame\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 px_last \u2506 DATE \u2506 CURRENCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 date \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 230.82 \u2506 2024-12-14 \u2506 USD \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n \r\n### 2. Multiple Securities with a Single Item\r\n```python\r\n# Fetch the last price for IBM and SEB\r\nwith BQuery() as bq:\r\n results = bq.bql(\"get(px_last) for(['IBM US Equity', 'SEBA SS Equity'])\")\r\n print(results[0])\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 px_last \u2506 DATE \u2506 CURRENCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 date \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 230.82 \u2506 2024-12-14 \u2506 USD \u2502\r\n\u2502 SEBA SS Equity \u2506 155.2 \u2506 2024-12-14 \u2506 SEK \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### 3. Multiple Items\r\nWhen querying for multiple items, `bql()` returns a list of DataFrames\r\n```python\r\n# Fetch name and last price of IBM (two items)\r\nwith BQuery() as bq:\r\n results = bq.bql(\"get(name, px_last) for(['IBM US Equity'])\")\r\n```\r\nOutput:\r\n```python\r\n>>> print(len(results)) # 2 DataFrames\r\nn=2\r\n\r\n>>> print(results[0]) # First DataFrame: 'name'\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 name \u2502\r\n\u2502 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 International Business Machine \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n\r\n>>> print(results[1]) # Second DataFrame: 'px_last'\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 px_last \u2506 DATE \u2506 CURRENCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 date \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 230.82 \u2506 2024-12-14 \u2506 USD \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n#### Combining Results\r\n```python\r\n>>> combined_df = results.combine()\r\n>>> print(combined_df)\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 name \u2506 px_last \u2506 DATE \u2506 CURRENCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 f64 \u2506 date \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 International Business Machine \u2506 230.82 \u2506 2024-12-14 \u2506 USD \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### 4. Advanced Example: Screening Securities\r\nFind list of SEB and Handelsbanken's AT1 bonds and print their names, duration and Z-Spread.\r\n```python\r\nquery=\"\"\"\r\n let(#dur=duration(duration_type=MODIFIED); \r\n #zsprd=spread(spread_type=Z);) \r\n get(name(), #dur, #zsprd) \r\n for(filter(screenresults(type=SRCH, screen_name='@COCO'), \r\n ticker in ['SEB', 'SHBASS']))\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n combined_df = results.combine()\r\n print(combined_df)\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 name() \u2506 #dur \u2506 DATE \u2506 #zsprd \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 f64 \u2506 date \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 BW924993 Corp \u2506 SEB 6 \u215e PERP \u2506 2.23 \u2506 2024-12-16 \u2506 212.0 \u2502\r\n\u2502 YV402592 Corp \u2506 SEB Float PERP \u2506 0.21 \u2506 2024-12-16 \u2506 233.0 \u2502\r\n\u2502 ZQ349286 Corp \u2506 SEB 5 \u215b PERP \u2506 0.39 \u2506 2024-12-16 \u2506 186.0 \u2502\r\n\u2502 ZO703315 Corp \u2506 SHBASS 4 \u215c PERP \u2506 1.95 \u2506 2024-12-16 \u2506 213.0 \u2502\r\n\u2502 ZO703956 Corp \u2506 SHBASS 4 \u00be PERP \u2506 4.94 \u2506 2024-12-16 \u2506 256.0 \u2502\r\n\u2502 YU819930 Corp \u2506 SEB 6 \u00be PERP \u2506 5.37 \u2506 2024-12-16 \u2506 309.0 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Average PE per Sector\r\nThis example shows aggregation (average) per group (sector) for members of an index.\r\nThe resulting list has only one element since there is only one data-item in `get`\r\n```python\r\nquery = \"\"\"\r\n let(#avg_pe=avg(group(pe_ratio(), gics_sector_name()));)\r\n get(#avg_pe)\r\n for(members('OMX Index'))\r\n\"\"\"\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n print(results[0].head(5))\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 #avg_pe \u2506 REVISION_DAT \u2506 AS_OF_DATE \u2506 PERIOD_END_D \u2506 ORIG_IDS \u2506 GICS_SECTOR \u2502\r\n\u2502 --- \u2506 --- \u2506 E \u2506 --- \u2506 ATE \u2506 --- \u2506 _NAME() \u2502\r\n\u2502 str \u2506 f64 \u2506 --- \u2506 date \u2506 --- \u2506 str \u2506 --- \u2502\r\n\u2502 \u2506 \u2506 date \u2506 \u2506 date \u2506 \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 Communicatio \u2506 19.561754 \u2506 2024-10-24 \u2506 2024-12-14 \u2506 2024-09-30 \u2506 null \u2506 Communicati \u2502\r\n\u2502 n Services \u2506 \u2506 \u2506 \u2506 \u2506 \u2506 on Services \u2502\r\n\u2502 Consumer Dis \u2506 19.117295 \u2506 2024-10-24 \u2506 2024-12-14 \u2506 2024-09-30 \u2506 null \u2506 Consumer \u2502\r\n\u2502 cretionary \u2506 \u2506 \u2506 \u2506 \u2506 \u2506 Discretiona \u2502\r\n\u2502 \u2506 \u2506 \u2506 \u2506 \u2506 \u2506 ry \u2502\r\n\u2502 Consumer \u2506 15.984743 \u2506 2024-10-24 \u2506 2024-12-14 \u2506 2024-09-30 \u2506 ESSITYB SS \u2506 Consumer \u2502\r\n\u2502 Staples \u2506 \u2506 \u2506 \u2506 \u2506 Equity \u2506 Staples \u2502\r\n\u2502 Financials \u2506 6.815895 \u2506 2024-10-24 \u2506 2024-12-14 \u2506 2024-09-30 \u2506 null \u2506 Financials \u2502\r\n\u2502 Health Care \u2506 22.00628 \u2506 2024-11-12 \u2506 2024-12-14 \u2506 2024-09-30 \u2506 null \u2506 Health Care \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Axes\r\nGet current axes of all Swedish USD AT1 bonds\r\n```python\r\n# Get current axes for Swedish AT1 bonds in USD\r\nquery=\"\"\"\r\n let(#ax=axes();)\r\n get(security_des, #ax)\r\n for(filter(bondsuniv(ACTIVE),\r\n crncy()=='USD' and\r\n basel_iii_designation() == 'Additional Tier 1' and\r\n country_iso() == 'SE'))\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n print(results.combine())\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 security_des \u2506 #ax \u2506 ASK_DEPTH \u2506 BID_DEPTH \u2506 ASK_TOTAL_SIZE \u2506 BID_TOTAL_SIZE \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 str \u2506 i64 \u2506 i64 \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 YU819930 Corp \u2506 SEB 6 \u00be PERP \u2506 Y \u2506 2 \u2506 null \u2506 5.6e6 \u2506 null \u2502\r\n\u2502 ZO703315 Corp \u2506 SHBASS 4 \u215c PERP \u2506 Y \u2506 1 \u2506 2 \u2506 5e6 \u2506 6e6 \u2502\r\n\u2502 BR069680 Corp \u2506 SWEDA 4 PERP \u2506 Y \u2506 null \u2506 1 \u2506 null \u2506 3e6 \u2502\r\n\u2502 ZL122341 Corp \u2506 SWEDA 7 \u215d PERP \u2506 Y \u2506 null \u2506 6 \u2506 null \u2506 2.04e7 \u2502\r\n\u2502 ZQ349286 Corp \u2506 SEB 5 \u215b PERP \u2506 Y \u2506 2 \u2506 4 \u2506 5.5e6 \u2506 3e7 \u2502\r\n\u2502 ZF859199 Corp \u2506 SWEDA 7 \u00be PERP \u2506 Y \u2506 1 \u2506 1 \u2506 2e6 \u2506 2e6 \u2502\r\n\u2502 ZO703956 Corp \u2506 SHBASS 4 \u00be PERP \u2506 Y \u2506 1 \u2506 3 \u2506 1.2e6 \u2506 1.1e7 \u2502\r\n\u2502 BW924993 Corp \u2506 SEB 6 \u215e PERP \u2506 Y \u2506 1 \u2506 3 \u2506 5e6 \u2506 1.1e7 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Axes with all columns\r\n```python\r\n# RT1 Axes with all columns\r\nquery = \"\"\"\r\nlet(#ax=axes();)\r\nget(name, #ax, amt_outstanding)\r\nfor(filter(bondsuniv(ACTIVE),\r\n crncy() in ['USD', 'EUR'] and\r\n solvency_ii_designation() == 'Restricted Tier 1' and\r\n amt_outstanding() > 7.5e8 and\r\n is_axed('Bid') == True))\r\npreferences(addcols=all)\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n print(results.combine())\r\n```\r\nOutput:\r\n<div>\r\n<small>shape: (3, 33)</small><table border=\"1\" class=\"dataframe\"><thead><tr><th>ID</th><th>name</th><th>#ax</th><th>ASK_PRICE</th><th>BID_PRICE</th><th>ASK_DEPTH</th><th>BID_DEPTH</th><th>ASK_DEALER</th><th>BID_DEALER</th><th>ASK_SIZE</th><th>BID_SIZE</th><th>ASK_TOTAL_SIZE</th><th>BID_TOTAL_SIZE</th><th>ASK_PRICE_IS_DERIVED</th><th>BID_PRICE_IS_DERIVED</th><th>ASK_SPREAD</th><th>BID_SPREAD</th><th>ASK_SPREAD_IS_DERIVED</th><th>BID_SPREAD_IS_DERIVED</th><th>ASK_YIELD</th><th>BID_YIELD</th><th>ASK_YIELD_IS_DERIVED</th><th>BID_YIELD_IS_DERIVED</th><th>ASK_AXE_SOURCE</th><th>BID_AXE_SOURCE</th><th>ASK_BROKER</th><th>BID_BROKER</th><th>ASK_HIST_AGG_SIZE</th><th>BID_HIST_AGG_SIZE</th><th>amt_outstanding</th><th>CURRENCY_OF_ISSUE</th><th>MULTIPLIER</th><th>CURRENCY</th></tr><tr><td>str</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>i64</td><td>i64</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>f64</td><td>f64</td><td>bool</td><td>bool</td><td>str</td><td>str</td><td>str</td><td>str</td><td>f64</td><td>f64</td><td>f64</td><td>str</td><td>f64</td><td>str</td></tr></thead><tbody><tr><td>"BM368057 Corp"</td><td>"ALVGR 2 \u215d PERP"</td><td>"Y"</td><td>88.034</td><td>87.427</td><td>5</td><td>1</td><td>"BARC"</td><td>"IMI"</td><td>1.2e6</td><td>1e6</td><td>7.2e6</td><td>1e6</td><td>null</td><td>null</td><td>287.031</td><td>300.046</td><td>true</td><td>true</td><td>4.854</td><td>4.976</td><td>true</td><td>true</td><td>"ERUN"</td><td>"ERUN"</td><td>"BXOL"</td><td>"IMIC"</td><td>6.68e6</td><td>8.92e6</td><td>1.2500e9</td><td>"EUR"</td><td>1.0</td><td>"EUR"</td></tr><tr><td>"EK588238 Corp"</td><td>"ASSGEN 4.596 PERP"</td><td>"Y"</td><td>101.0</td><td>100.13</td><td>4</td><td>6</td><td>"MSAX"</td><td>"A2A"</td><td>500000.0</td><td>100000.0</td><td>1.556e7</td><td>3.83e7</td><td>null</td><td>null</td><td>108.9</td><td>207.889</td><td>true</td><td>true</td><td>3.466</td><td>4.434</td><td>null</td><td>true</td><td>"ERUN"</td><td>"BBX"</td><td>"MSAX"</td><td>"A2A"</td><td>1.70424e7</td><td>3.17e7</td><td>1.0004e9</td><td>"EUR"</td><td>1.0</td><td>"EUR"</td></tr><tr><td>"BR244025 Corp"</td><td>"ALVGR 3.2 PERP"</td><td>"Y"</td><td>88.0</td><td>86.875</td><td>3</td><td>4</td><td>"UBS"</td><td>"DB"</td><td>5e6</td><td>1e6</td><td>1.1e7</td><td>1.4e7</td><td>null</td><td>null</td><td>49.33</td><td>414.602</td><td>true</td><td>true</td><td>7.34258</td><td>8.553</td><td>null</td><td>true</td><td>"ERUN"</td><td>"ERUN"</td><td>"UBSW"</td><td>"DABC"</td><td>1.6876e6</td><td>3.6e7</td><td>1.2500e9</td><td>"USD"</td><td>1.0</td><td>"USD"</td></tr></tbody></table></div>\r\n\r\n\r\n### Segments\r\nThe following example shows handling of two data-items with different length. The first dataframe \r\ndescribes the segments (and has length 5 in this case), while the second dataframe contains time series.\r\nOne can join the dataframes on common columns and pivot the segments into columns as shown below:\r\n```python\r\n# revenue per segment\r\nquery = \"\"\"\r\n let(#segment=segment_name();\r\n #revenue=sales_Rev_turn(fpt=q, fpr=range(2023Q3, 2024Q3));\r\n )\r\n get(#segment, #revenue)\r\n for(segments('GTN US Equity',type=reported,hierarchy=PRODUCT, level=1))\r\n\"\"\"\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n df = results.combine().pivot(\r\n index=\"PERIOD_END_DATE\", on=\"#segment\", values=\"#revenue\"\r\n )\r\n print(df)\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 PERIOD_END_DATE \u2506 Broadcasting \u2506 Production Companies \u2506 Other \u2506 Adjustment \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 date \u2506 f64 \u2506 f64 \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 2023-09-30 \u2506 7.83e8 \u2506 2e7 \u2506 1.6e7 \u2506 null \u2502\r\n\u2502 2023-12-31 \u2506 8.13e8 \u2506 3.2e7 \u2506 1.9e7 \u2506 null \u2502\r\n\u2502 2024-03-31 \u2506 7.8e8 \u2506 2.4e7 \u2506 1.9e7 \u2506 null \u2502\r\n\u2502 2024-06-30 \u2506 8.08e8 \u2506 1.8e7 \u2506 0.0 \u2506 null \u2502\r\n\u2502 2024-09-30 \u2506 9.24e8 \u2506 2.6e7 \u2506 1.7e7 \u2506 null \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Actual and Forward EPS Estimates\r\n```python\r\nwith BQuery() as bq:\r\n results = bq.bql(\"\"\"\r\n let(#eps=is_eps(fa_period_type='A',\r\n fa_period_offset=range(-4,2));)\r\n get(#eps)\r\n for(['IBM US Equity'])\r\n \"\"\")\r\n print(results[0])\r\n\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 #eps \u2506 REVISION_DATE \u2506 AS_OF_DATE \u2506 PERIOD_END_DATE \u2506 CURRENCY \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 date \u2506 date \u2506 date \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 IBM US Equity \u2506 10.63 \u2506 2022-02-22 \u2506 2024-12-14 \u2506 2019-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 6.28 \u2506 2023-02-28 \u2506 2024-12-14 \u2506 2020-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 6.41 \u2506 2023-02-28 \u2506 2024-12-14 \u2506 2021-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 1.82 \u2506 2024-03-18 \u2506 2024-12-14 \u2506 2022-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 8.23 \u2506 2024-03-18 \u2506 2024-12-14 \u2506 2023-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 7.891 \u2506 2024-12-13 \u2506 2024-12-14 \u2506 2024-12-31 \u2506 USD \u2502\r\n\u2502 IBM US Equity \u2506 9.236 \u2506 2024-12-13 \u2506 2024-12-14 \u2506 2025-12-31 \u2506 USD \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Average issuer OAS spread per maturity bucket\r\n```python\r\n# Example: Average OAS-spread per maturity bucket\r\nquery = \"\"\"\r\nlet(\r\n #bins = bins(maturity_years,\r\n [3,9,18,30],\r\n ['(1) 0-3','(2) 3-9','(3) 9-18','(4) 18-30','(5) 30+']);\r\n #average_spread = avg(group(spread(st=oas),#bins));\r\n)\r\nget(#average_spread)\r\nfor(filter(bonds('NVDA US Equity', issuedby = 'ENTITY'),\r\n maturity_years != NA))\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n print(results[0])\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 #average_spread \u2506 DATE \u2506 ORIG_IDS \u2506 #BINS \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 f64 \u2506 date \u2506 str \u2506 str \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 (1) 0-3 \u2506 31.195689 \u2506 2024-12-14 \u2506 QZ552396 Corp \u2506 (1) 0-3 \u2502\r\n\u2502 (2) 3-9 \u2506 59.580383 \u2506 2024-12-14 \u2506 null \u2506 (2) 3-9 \u2502\r\n\u2502 (3) 9-18 \u2506 110.614416 \u2506 2024-12-14 \u2506 BH393780 Corp \u2506 (3) 9-18 \u2502\r\n\u2502 (4) 18-30 \u2506 135.160279 \u2506 2024-12-14 \u2506 BH393781 Corp \u2506 (4) 18-30 \u2502\r\n\u2502 (5) 30+ \u2506 150.713405 \u2506 2024-12-14 \u2506 BH393782 Corp \u2506 (5) 30+ \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Technical Analysis: stocks with 20d EMA > 200d EMA and RSI > 53\r\n```python\r\nwith BQuery() as bq:\r\n results = bq.bql(\r\n \"\"\"\r\n let(#ema20=emavg(period=20);\r\n #ema200=emavg(period=200);\r\n #rsi=rsi(close=px_last());)\r\n get(name(), #ema20, #ema200, #rsi)\r\n for(filter(members('OMX Index'),\r\n and(#ema20 > #ema200, #rsi > 53)))\r\n with(fill=PREV)\r\n \"\"\"\r\n )\r\n print(results.combine())\r\n```\r\nOutput:\r\n```python\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 name() \u2506 #ema20 \u2506 DATE \u2506 CURRENCY \u2506 #ema200 \u2506 #rsi \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 f64 \u2506 date \u2506 str \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 ERICB SS Equity \u2506 Telefonaktiebola \u2506 90.152604 \u2506 2024-12-16 \u2506 SEK \u2506 75.072151 \u2506 56.010028 \u2502\r\n\u2502 \u2506 get LM Ericsso \u2506 \u2506 \u2506 \u2506 \u2506 \u2502\r\n\u2502 ABB SS Equity \u2506 ABB Ltd \u2506 630.622469 \u2506 2024-12-16 \u2506 SEK \u2506 566.571183 \u2506 53.763102 \u2502\r\n\u2502 SEBA SS Equity \u2506 Skandinaviska \u2506 153.80595 \u2506 2024-12-16 \u2506 SEK \u2506 150.742394 \u2506 56.460733 \u2502\r\n\u2502 \u2506 Enskilda Banken \u2506 \u2506 \u2506 \u2506 \u2506 \u2502\r\n\u2502 ASSAB SS Equity \u2506 Assa Abloy AB \u2506 339.017591 \u2506 2024-12-16 \u2506 SEK \u2506 317.057573 \u2506 53.351619 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Bond Universe from Equity Ticker\r\n```python\r\n# Get Bond Universe from Equity Ticker\r\nquery = \"\"\"\r\nlet(#rank=normalized_payment_rank();\r\n #oas=spread(st=oas);\r\n #nxt_call=nxt_call_dt();\r\n )\r\nget(name(), #rank, #nxt_call, #oas)\r\nfor(filter(bonds('GTN US Equity'), series() == '144A'))\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n df = results.combine()\r\n print(df)\r\n```\r\nOutput:\r\n```\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 ID \u2506 name() \u2506 #rank \u2506 #nxt_call \u2506 #oas \u2506 DATE \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 str \u2506 str \u2506 str \u2506 date \u2506 f64 \u2506 date \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 YX231113 Corp \u2506 GTN 10 \u00bd 07/15/29 \u2506 1st Lien Secured \u2506 2026-07-15 \u2506 598.66491 \u2506 2024-12-17 \u2502\r\n\u2502 BS116983 Corp \u2506 GTN 5 \u215c 11/15/31 \u2506 Sr Unsecured \u2506 2026-11-15 \u2506 1193.17529 \u2506 2024-12-17 \u2502\r\n\u2502 AV438089 Corp \u2506 GTN 7 05/15/27 \u2506 Sr Unsecured \u2506 2024-12-24 \u2506 400.340456 \u2506 2024-12-17 \u2502\r\n\u2502 ZO860846 Corp \u2506 GTN 4 \u00be 10/15/30 \u2506 Sr Unsecured \u2506 2025-10-15 \u2506 1249.34346 \u2506 2024-12-17 \u2502\r\n\u2502 LW375188 Corp \u2506 GTN 5 \u215e 07/15/26 \u2506 Sr Unsecured \u2506 2025-01-13 \u2506 173.761744 \u2506 2024-12-17 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Bonds Total Returns\r\nThis is example of a single-item query returning total return for all GTN bonds in a long dataframe.\r\nWe can easily pivot it into wide format, as in the example below\r\n```python\r\n# Total Return of GTN Bonds\r\nquery = \"\"\"\r\nlet(#rng = range(-1M, 0D);\r\n #rets = return_series(calc_interval=#rng,per=W);)\r\nget(#rets)\r\nfor(filter(bonds('GTN US Equity'), series() == '144A'))\r\n\"\"\"\r\n\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\n df = results[0].pivot(on=\"ID\", index=\"DATE\", values=\"#rets\")\r\n print(df)\r\n```\r\nOutput:\r\n```python\r\nshape: (6, 6)\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 DATE \u2506 YX231113 Corp \u2506 BS116983 Corp \u2506 AV438089 Corp \u2506 ZO860846 Corp \u2506 LW375188 Corp \u2502\r\n\u2502 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2506 --- \u2502\r\n\u2502 date \u2506 f64 \u2506 f64 \u2506 f64 \u2506 f64 \u2506 f64 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 2024-11-17 \u2506 null \u2506 null \u2506 null \u2506 null \u2506 null \u2502\r\n\u2502 2024-11-24 \u2506 0.001653 \u2506 0.051179 \u2506 0.020363 \u2506 0.001371 \u2506 -0.002939 \u2502\r\n\u2502 2024-12-01 \u2506 0.002837 \u2506 0.010405 \u2506 -0.001466 \u2506 0.007275 \u2506 0.000581 \u2502\r\n\u2502 2024-12-08 \u2506 -0.000041 \u2506 0.016145 \u2506 0.000766 \u2506 0.024984 \u2506 0.000936 \u2502\r\n\u2502 2024-12-15 \u2506 0.001495 \u2506 -0.047 \u2506 -0.000233 \u2506 -0.043509 \u2506 0.002241 \u2502\r\n\u2502 2024-12-17 \u2506 0.00008 \u2506 -0.000004 \u2506 -0.0035 \u2506 -0.007937 \u2506 0.000064 \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n### Maturity Wall for US HY Bonds\r\n```python\r\nquery = \"\"\"\r\nlet(#mv=sum(group(amt_outstanding(currency=USD),\r\n by=[year(maturity()), industry_sector()]));)\r\nget(#mv)\r\nfor(members('LF98TRUU Index'))\r\n\"\"\"\r\nwith BQuery() as bq:\r\n results = bq.bql(query)\r\ndf = results.combine().rename(\r\n {\"YEAR(MATURITY())\": \"maturity\", \"INDUSTRY_SECTOR()\": \"sector\", \"#mv\": \"mv\"}\r\n)\r\n\r\nprint(df.pivot(index=\"maturity\", on=\"sector\", values=\"mv\").head())\r\n```\r\nOutput:\r\n```python\r\nshape: (5, 11)\r\n\u250c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u252c\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2510\r\n\u2502 maturity \u2506 Basic \u2506 Consumer, \u2506 Energy \u2506 \u2026 \u2506 Financial \u2506 Technolog \u2506 Utilities \u2506 Diversifi \u2502\r\n\u2502 --- \u2506 Materials \u2506 Non-cycli \u2506 --- \u2506 \u2506 --- \u2506 y \u2506 --- \u2506 ed \u2502\r\n\u2502 i64 \u2506 --- \u2506 cal \u2506 f64 \u2506 \u2506 f64 \u2506 --- \u2506 f64 \u2506 --- \u2502\r\n\u2502 \u2506 f64 \u2506 --- \u2506 \u2506 \u2506 \u2506 f64 \u2506 \u2506 f64 \u2502\r\n\u2502 \u2506 \u2506 f64 \u2506 \u2506 \u2506 \u2506 \u2506 \u2506 \u2502\r\n\u255e\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u256a\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2550\u2561\r\n\u2502 2025 \u2506 1.5e8 \u2506 5.34916e8 \u2506 5e8 \u2506 \u2026 \u2506 null \u2506 null \u2506 null \u2506 null \u2502\r\n\u2502 2026 \u2506 4.4013e9 \u2506 9.3293e9 \u2506 8.2931e9 \u2506 \u2026 \u2506 1.3524e10 \u2506 4.0608e9 \u2506 2.5202e9 \u2506 null \u2502\r\n\u2502 2027 \u2506 8.3921e9 \u2506 2.3409e10 \u2506 1.2427e10 \u2506 \u2026 \u2506 1.9430e10 \u2506 4.3367e9 \u2506 3.6620e9 \u2506 null \u2502\r\n\u2502 2028 \u2506 1.4701e10 \u2506 3.7457e10 \u2506 2.2442e10 \u2506 \u2026 \u2506 2.3341e10 \u2506 9.9143e9 \u2506 7.6388e9 \u2506 5e8 \u2502\r\n\u2502 2029 \u2506 1.6512e10 \u2506 5.7381e10 \u2506 3.9286e10 \u2506 \u2026 \u2506 4.2337e10 \u2506 2.2660e10 \u2506 5.8558e9 \u2506 null \u2502\r\n\u2514\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2534\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2500\u2518\r\n```\r\n\r\n\r\n## Additional Documentation & Resources\r\n\r\n- *API Documentation*: Detailed documentation and function references are available in https://marekozana.github.io/polars-bloomberg\r\n\r\n- *Additional Examples*: Check out [examples/](examples/) for hands-on notebooks demonstrating a variety of use cases.\r\n - BQL examples and use cases: [examples/Examples-BQL.ipynb](https://github.com/MarekOzana/polars-bloomberg/blob/main/examples/Examples-BQL.ipynb)\r\n\r\n- *Bloomberg Developer Resources*: For more details on the Bloomberg API itself, visit the [Bloomberg Developer's page](https://developer.bloomberg.com/).\r\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Python library providing a Polars DataFrame interface for easy and intuitive access to Bloomberg API.",
"version": "0.4.2",
"project_urls": {
"Documentation": "https://marekozana.github.io/polars-bloomberg",
"Homepage": "https://github.com/MarekOzana/polars-bloomberg"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3943dcce6d553fb2edaec4b4aaea78d42193e1cf374a2b306cda6434b6aa2585",
"md5": "1452c052320dcf0f12f8803279355276",
"sha256": "0805cabfba5bc5cc6ccc30b935e32f3df68cf2bd41ca5d74f86c9f57863a1faf"
},
"downloads": -1,
"filename": "polars_bloomberg-0.4.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "1452c052320dcf0f12f8803279355276",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11",
"size": 22152,
"upload_time": "2024-12-21T18:52:00",
"upload_time_iso_8601": "2024-12-21T18:52:00.466936Z",
"url": "https://files.pythonhosted.org/packages/39/43/dcce6d553fb2edaec4b4aaea78d42193e1cf374a2b306cda6434b6aa2585/polars_bloomberg-0.4.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "03ab10b62c398a358f545bcc8284e2f963c99931f45a41f00e71f4c5f105a1c0",
"md5": "a6a1485aa5d817a6362b4fed71420638",
"sha256": "13489dbc18c7e35dadb2a9b9fbf694c8b34d42df1ea7f11ed752ee8dc2747d2d"
},
"downloads": -1,
"filename": "polars_bloomberg-0.4.2.tar.gz",
"has_sig": false,
"md5_digest": "a6a1485aa5d817a6362b4fed71420638",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11",
"size": 48578,
"upload_time": "2024-12-21T18:52:03",
"upload_time_iso_8601": "2024-12-21T18:52:03.300356Z",
"url": "https://files.pythonhosted.org/packages/03/ab/10b62c398a358f545bcc8284e2f963c99931f45a41f00e71f4c5f105a1c0/polars_bloomberg-0.4.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-21 18:52:03",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "MarekOzana",
"github_project": "polars-bloomberg",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "blpapi",
"specs": [
[
">=",
"3.24.0"
]
]
},
{
"name": "polars",
"specs": [
[
">=",
"1.7.1"
]
]
}
],
"lcname": "polars-bloomberg"
}