# pollination-dsl
A Python Domain Specific Language (DSL) to create Pollination Plugins and Recipes.
Pollination uses [Queenbee](https://github.com/pollination/queenbee) as its workflow
language. Pollination-dsl makes it easy to create Queenbee object without the need to
learn Queenbee.
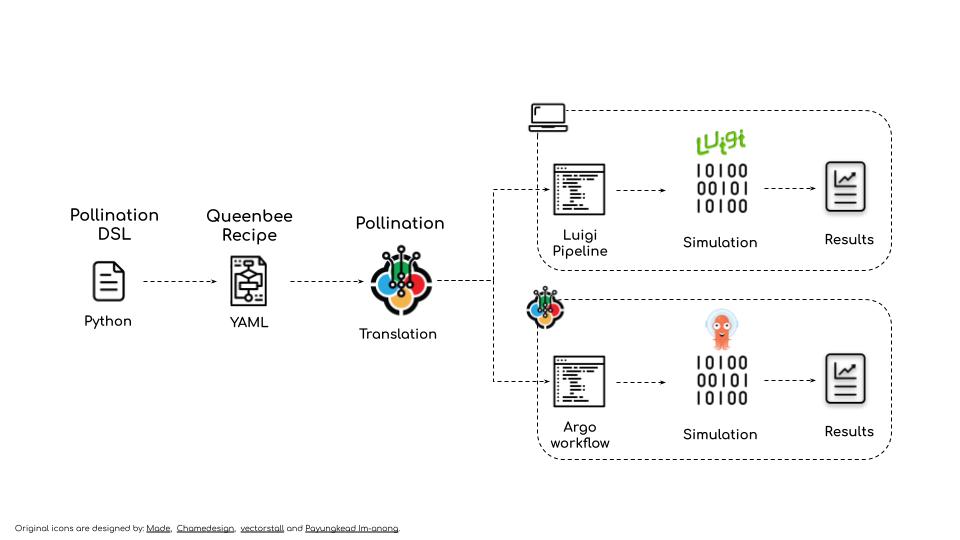
# API docs
[Pollination-DSL API docs](https://pollination.github.io/pollination-dsl/docs/pollination_dsl.html#subpackages)
# Requirements
Python >=3.7
# Installation
Using pip:
`pip install pollination-dsl`
For local development:
1. Clone this repository.
2. Change directory to root folder of the repository.
3. `pip install -e .`
## Function
```python
from dataclasses import dataclass
from pollination_dsl.function import Function, command, Inputs, Outputs
@dataclass
class CreateOctreeWithSky(Function):
"""Generate an octree from a Radiance folder and sky!"""
# inputs
include_aperture = Inputs.str(
default='include',
description='A value to indicate if the static aperture should be included in '
'octree. Valid values are include and exclude. Default is include.',
spec={'type': 'string', 'enum': ['include', 'exclude']}
)
black_out = Inputs.str(
default='default',
description='A value to indicate if the black material should be used. Valid '
'values are default and black. Default value is default.',
spec={'type': 'string', 'enum': ['black', 'default']}
)
model = Inputs.folder(description='Path to Radiance model folder.', path='model')
sky = Inputs.file(description='Path to sky file.', path='sky.sky')
@command
def create_octree(self):
return 'honeybee-radiance octree from-folder model --output scene.oct ' \
'--{{self.include_aperture}}-aperture --{{self.black_out}} ' \
'--add-before sky.sky'
# outputs
scene_file = Outputs.file(description='Output octree file.', path='scene.oct')
```
If you want to access the `Queenbee` objects you can use `queenbee` property. For example
try `print(CreateOctreeWithSky().queenbee.yaml())` and you should see the full Queenbee
definition:
```yaml
type: Function
annotations: {}
inputs:
- type: FunctionStringInput
annotations: {}
name: black-out
description: A value to indicate if the black material should be used. Valid values
are default and black. Default value is default.
default: default
alias: []
required: false
spec:
type: string
enum:
- black
- default
- type: FunctionStringInput
annotations: {}
name: include-aperture
description: A value to indicate if the static aperture should be included in octree.
Valid values are include and exclude. Default is include.
default: include
alias: []
required: false
spec:
type: string
enum:
- include
- exclude
- type: FunctionFolderInput
annotations: {}
name: model
description: Path to Radiance model folder.
default: null
alias: []
required: true
spec: null
path: model
- type: FunctionFileInput
annotations: {}
name: sky
description: Path to sky file.
default: null
alias: []
required: true
spec: null
path: sky.sky
extensions: null
outputs:
- type: FunctionFileOutput
annotations: {}
name: scene-file
description: Output octree file.
path: scene.oct
name: create-octree-with-sky
description: Generate an octree from a Radiance folder and sky!
command: honeybee-radiance octree from-folder model --output scene.oct --{{inputs.include-aperture}}-aperture
--{{inputs.black-out}} --add-before sky.sky
```
Since the functions are standard Python classes you can also subclass them from one
another as long as you use the same name for the `@command` method. Otherwise it will
create an invalid function with two commands.
## Plugin
To create a Pollination plugin use the functions to create a standard Python module.
The only change is that you need to provide the information for Pollination plugin in
the `__init__.py` file as dictionary assigned to `__pollination__` variable.
Follow the standard way to install a Python package. Once the package is installed you
can use `pollination-dsl` to load the package or write it to a folder.
```python
from pollination_dsl.package import load, write
# name of the pollination package
python_package = 'pollination_honeybee_radiance'
# load this package as Pollination Plugin
plugin = load(python_package)
# or write the package as a Pollination plugin to a folder directly
write(python_package, './pollination-honeybee-radiance')
```
Here are two real world examples of Pollination plugins:
- [`pollination-honeybee-radiance` plugin](https://github.com/pollination/honeybee-radiance)
- [`pollination-honeybee-energy` plugin](https://github.com/pollination/honeybee-energy)
## Recipe
`Recipe` is a collection of `DAG`s. Each `DAG` is a collection of interrelated `task`s.
You can use pollination-dsl to create complex recipes with minimum code by reusing the
`functions` as templates for each task.
Packaging a plugin is exactly the same as packaging a plugin.
```python
from pollination_dsl.package import load, translate
# name of the pollination package
python_package = 'daylight-factor'
# load this package as Pollination Recipe
recipe = load(python_package, baked=True)
# or translate and write the package as a Pollination plugin to a folder directly
translate(python_package, './daylight-factor')
```
Here are number of real world examples of Pollination recipes:
- [`Annual energy use` recipe](https://github.com/pollination/annual-energy-use)
- [`Daylight factor` recipe](https://github.com/pollination/daylight-factor)
- [`Annual daylight` recipe](https://github.com/pollination/annual-daylight)
# How to create a pollination-dsl package
Pollination-dsl uses Python's standard packaging to package pollination plugins and recipes.
It parses most of the data from inputs in `setup.py` file and some Pollination specific
information from `__init__.py` file. Below is an example of how these file should look
like.
By taking advantage of [Python's native namespace packaging](https://packaging.python.org/guides/packaging-namespace-packages/#native-namespace-packages)
we keep all the packages under the `pollination` namespace.
## setup.py
Here is an example `setup.py` file. You can see the latest version of the file [here](https://github.com/pollination/honeybee-radiance/blob/master/setup.py).
```python
#!/usr/bin/env python
import setuptools
with open("README.md", "r") as fh:
long_description = fh.read()
with open('requirements.txt') as f:
requirements = f.read().splitlines()
setuptools.setup(
name='pollination-honeybee-radiance', # required - will be used for package name
author='ladybug-tools', # required - author must match the owner account name on Pollination
author_email='info@ladybug.tools',
packages=setuptools.find_namespace_packages(include=['pollination.*']), # required - that's how pollination find the package
version='0.1.0', # required - will be used as package tag. you can also use semantic versioning
install_requires=requirements,
url='https://github.com/pollination/pollination-honeybee-radiance', # optional - will be translated to home
project_urls={
'icon': 'https://raw.githubusercontent.com/ladybug-tools/artwork/master/icons_bugs/grasshopper_tabs/HB-Radiance.png', # optional but strongly encouraged - link to package icon
},
description='Honeybee Radiance plugin for Pollination.', # optional - will be used as package description
long_description=long_description, # optional - will be translated to ReadMe content on Pollination
long_description_content_type="text/markdown",
maintainer='maintainer_1, maintainer_2', # optional - will be translated to maintainers. For multiple maintainers
maintainer_email='maintainer_1@example.come, maintainer_2@example.com', # use comma inside the string.
keywords='honeybee, radiance, ladybug-tools, daylight', # optional - will be used as keywords
license='PolyForm Shield License 1.0.0, https://polyformproject.org/wp-content/uploads/2020/06/PolyForm-Shield-1.0.0.txt', # optional - the license link should be separated by a comma
zip_safe=False # required - set to False to ensure the packaging will always work
)
```
## __init__.py
Here is an example `__init__.py` for a plugin. The latest version of the file is
accessible [here](https://github.com/pollination/honeybee-radiance/blob/master/pollination/honeybee_radiance/__init__.py).
```python
"""Honeybee Radiance plugin for Pollination."""
from pollination_dsl.common import get_docker_image_from_dependency
# set the version for docker image dynamically based on honeybee-radiance version
# in dependencies
image_id = get_docker_image_from_dependency(
__package__, 'honeybee-radiance', 'ladybugtools'
)
__pollination__ = {
'app_version': '5.4', # optional - tag for version of Radiance
'config': {
'docker': {
'image': image_id,
'workdir': '/home/ladybugbot/run'
}
}
}
```
Here is an example `__init__.py` for a recipe. The latest version of the file is
accessible [here](https://github.com/pollination/annual-daylight/blob/master/pollination/annual_daylight/__init__.py).
```python
from .entry import AnnualDaylightEntryPoint
__pollination__ = {
'entry_point': AnnualDaylightEntryPoint
}
```
Raw data
{
"_id": null,
"home_page": "https://github.com/pollination/pollination-dsl",
"name": "pollination-dsl",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": null,
"keywords": null,
"author": "Pollination",
"author_email": "info@pollination.solutions",
"download_url": "https://files.pythonhosted.org/packages/23/2f/e4b2ac8e7156b8288b8588eb2804c2191a5f0ec511963576ea621304f8c0/pollination_dsl-0.17.1.tar.gz",
"platform": null,
"description": "# pollination-dsl\nA Python Domain Specific Language (DSL) to create Pollination Plugins and Recipes.\n\nPollination uses [Queenbee](https://github.com/pollination/queenbee) as its workflow\nlanguage. Pollination-dsl makes it easy to create Queenbee object without the need to\nlearn Queenbee.\n\n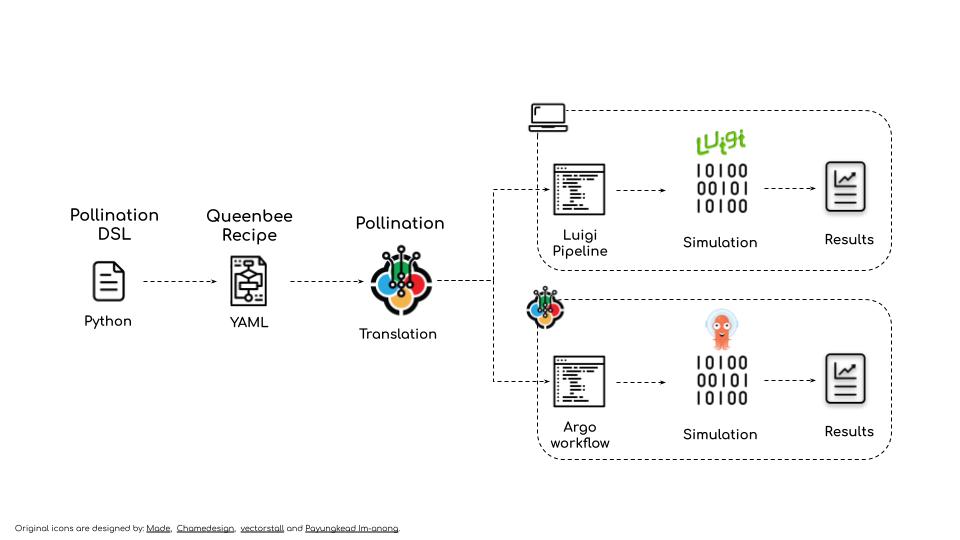\n\n# API docs\n[Pollination-DSL API docs](https://pollination.github.io/pollination-dsl/docs/pollination_dsl.html#subpackages)\n\n# Requirements\nPython >=3.7\n\n# Installation\n\nUsing pip:\n\n`pip install pollination-dsl`\n\nFor local development:\n\n1. Clone this repository.\n2. Change directory to root folder of the repository.\n3. `pip install -e .`\n\n## Function\n\n```python\nfrom dataclasses import dataclass\nfrom pollination_dsl.function import Function, command, Inputs, Outputs\n\n\n@dataclass\nclass CreateOctreeWithSky(Function):\n \"\"\"Generate an octree from a Radiance folder and sky!\"\"\"\n\n # inputs\n include_aperture = Inputs.str(\n default='include',\n description='A value to indicate if the static aperture should be included in '\n 'octree. Valid values are include and exclude. Default is include.',\n spec={'type': 'string', 'enum': ['include', 'exclude']}\n )\n\n black_out = Inputs.str(\n default='default',\n description='A value to indicate if the black material should be used. Valid '\n 'values are default and black. Default value is default.',\n spec={'type': 'string', 'enum': ['black', 'default']}\n )\n\n model = Inputs.folder(description='Path to Radiance model folder.', path='model')\n\n sky = Inputs.file(description='Path to sky file.', path='sky.sky')\n\n @command\n def create_octree(self):\n return 'honeybee-radiance octree from-folder model --output scene.oct ' \\\n '--{{self.include_aperture}}-aperture --{{self.black_out}} ' \\\n '--add-before sky.sky'\n\n # outputs\n scene_file = Outputs.file(description='Output octree file.', path='scene.oct')\n\n```\n\nIf you want to access the `Queenbee` objects you can use `queenbee` property. For example\ntry `print(CreateOctreeWithSky().queenbee.yaml())` and you should see the full Queenbee\ndefinition:\n\n```yaml\ntype: Function\nannotations: {}\ninputs:\n- type: FunctionStringInput\n annotations: {}\n name: black-out\n description: A value to indicate if the black material should be used. Valid values\n are default and black. Default value is default.\n default: default\n alias: []\n required: false\n spec:\n type: string\n enum:\n - black\n - default\n- type: FunctionStringInput\n annotations: {}\n name: include-aperture\n description: A value to indicate if the static aperture should be included in octree.\n Valid values are include and exclude. Default is include.\n default: include\n alias: []\n required: false\n spec:\n type: string\n enum:\n - include\n - exclude\n- type: FunctionFolderInput\n annotations: {}\n name: model\n description: Path to Radiance model folder.\n default: null\n alias: []\n required: true\n spec: null\n path: model\n- type: FunctionFileInput\n annotations: {}\n name: sky\n description: Path to sky file.\n default: null\n alias: []\n required: true\n spec: null\n path: sky.sky\n extensions: null\noutputs:\n- type: FunctionFileOutput\n annotations: {}\n name: scene-file\n description: Output octree file.\n path: scene.oct\nname: create-octree-with-sky\ndescription: Generate an octree from a Radiance folder and sky!\ncommand: honeybee-radiance octree from-folder model --output scene.oct --{{inputs.include-aperture}}-aperture\n --{{inputs.black-out}} --add-before sky.sky\n```\n\nSince the functions are standard Python classes you can also subclass them from one\nanother as long as you use the same name for the `@command` method. Otherwise it will\ncreate an invalid function with two commands.\n\n## Plugin\n\nTo create a Pollination plugin use the functions to create a standard Python module.\nThe only change is that you need to provide the information for Pollination plugin in\nthe `__init__.py` file as dictionary assigned to `__pollination__` variable.\n\nFollow the standard way to install a Python package. Once the package is installed you\ncan use `pollination-dsl` to load the package or write it to a folder.\n\n```python\nfrom pollination_dsl.package import load, write\n\n# name of the pollination package\npython_package = 'pollination_honeybee_radiance'\n\n# load this package as Pollination Plugin\nplugin = load(python_package)\n\n# or write the package as a Pollination plugin to a folder directly\nwrite(python_package, './pollination-honeybee-radiance')\n\n```\n\nHere are two real world examples of Pollination plugins:\n\n- [`pollination-honeybee-radiance` plugin](https://github.com/pollination/honeybee-radiance)\n- [`pollination-honeybee-energy` plugin](https://github.com/pollination/honeybee-energy)\n\n## Recipe\n\n`Recipe` is a collection of `DAG`s. Each `DAG` is a collection of interrelated `task`s.\nYou can use pollination-dsl to create complex recipes with minimum code by reusing the\n`functions` as templates for each task.\n\nPackaging a plugin is exactly the same as packaging a plugin.\n\n```python\nfrom pollination_dsl.package import load, translate\n\n# name of the pollination package\npython_package = 'daylight-factor'\n\n# load this package as Pollination Recipe\nrecipe = load(python_package, baked=True)\n\n# or translate and write the package as a Pollination plugin to a folder directly\ntranslate(python_package, './daylight-factor')\n\n```\n\nHere are number of real world examples of Pollination recipes:\n\n- [`Annual energy use` recipe](https://github.com/pollination/annual-energy-use)\n- [`Daylight factor` recipe](https://github.com/pollination/daylight-factor)\n- [`Annual daylight` recipe](https://github.com/pollination/annual-daylight)\n\n\n# How to create a pollination-dsl package\n\nPollination-dsl uses Python's standard packaging to package pollination plugins and recipes.\nIt parses most of the data from inputs in `setup.py` file and some Pollination specific\ninformation from `__init__.py` file. Below is an example of how these file should look\nlike.\n\nBy taking advantage of [Python's native namespace packaging](https://packaging.python.org/guides/packaging-namespace-packages/#native-namespace-packages)\nwe keep all the packages under the `pollination` namespace.\n\n## setup.py\n\nHere is an example `setup.py` file. You can see the latest version of the file [here](https://github.com/pollination/honeybee-radiance/blob/master/setup.py).\n\n```python\n\n#!/usr/bin/env python\nimport setuptools\n\nwith open(\"README.md\", \"r\") as fh:\n long_description = fh.read()\n\nwith open('requirements.txt') as f:\n requirements = f.read().splitlines()\n\nsetuptools.setup(\n name='pollination-honeybee-radiance', # required - will be used for package name\n author='ladybug-tools', # required - author must match the owner account name on Pollination\n author_email='info@ladybug.tools',\n packages=setuptools.find_namespace_packages(include=['pollination.*']), # required - that's how pollination find the package\n version='0.1.0', # required - will be used as package tag. you can also use semantic versioning\n install_requires=requirements,\n url='https://github.com/pollination/pollination-honeybee-radiance', # optional - will be translated to home\n project_urls={\n 'icon': 'https://raw.githubusercontent.com/ladybug-tools/artwork/master/icons_bugs/grasshopper_tabs/HB-Radiance.png', # optional but strongly encouraged - link to package icon\n },\n description='Honeybee Radiance plugin for Pollination.', # optional - will be used as package description\n long_description=long_description, # optional - will be translated to ReadMe content on Pollination\n long_description_content_type=\"text/markdown\",\n maintainer='maintainer_1, maintainer_2', # optional - will be translated to maintainers. For multiple maintainers\n maintainer_email='maintainer_1@example.come, maintainer_2@example.com', # use comma inside the string.\n keywords='honeybee, radiance, ladybug-tools, daylight', # optional - will be used as keywords\n license='PolyForm Shield License 1.0.0, https://polyformproject.org/wp-content/uploads/2020/06/PolyForm-Shield-1.0.0.txt', # optional - the license link should be separated by a comma\n zip_safe=False # required - set to False to ensure the packaging will always work\n)\n\n```\n\n## __init__.py\n\nHere is an example `__init__.py` for a plugin. The latest version of the file is\naccessible [here](https://github.com/pollination/honeybee-radiance/blob/master/pollination/honeybee_radiance/__init__.py).\n\n\n```python\n\n\"\"\"Honeybee Radiance plugin for Pollination.\"\"\"\nfrom pollination_dsl.common import get_docker_image_from_dependency\n\n# set the version for docker image dynamically based on honeybee-radiance version\n# in dependencies\nimage_id = get_docker_image_from_dependency(\n __package__, 'honeybee-radiance', 'ladybugtools'\n)\n\n__pollination__ = {\n 'app_version': '5.4', # optional - tag for version of Radiance\n 'config': {\n 'docker': {\n 'image': image_id,\n 'workdir': '/home/ladybugbot/run'\n }\n }\n}\n```\n\nHere is an example `__init__.py` for a recipe. The latest version of the file is\naccessible [here](https://github.com/pollination/annual-daylight/blob/master/pollination/annual_daylight/__init__.py).\n\n```python\nfrom .entry import AnnualDaylightEntryPoint\n\n__pollination__ = {\n 'entry_point': AnnualDaylightEntryPoint\n}\n\n```\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python DSL to create Pollination recipes and plugins.",
"version": "0.17.1",
"project_urls": {
"Homepage": "https://github.com/pollination/pollination-dsl"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "0fde1b96ef04dd8c10d71ef49b20b79bcc1292ecf6c68332c007c1c6afc561f8",
"md5": "3ad9bb4970a877f177aa4c399f32c856",
"sha256": "d9fcc6e9c6c921c3f022b6dc221f21912886a0f47bede54d944974b375edb2f6"
},
"downloads": -1,
"filename": "pollination_dsl-0.17.1-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "3ad9bb4970a877f177aa4c399f32c856",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": ">=3.7",
"size": 38618,
"upload_time": "2024-11-22T18:48:50",
"upload_time_iso_8601": "2024-11-22T18:48:50.798759Z",
"url": "https://files.pythonhosted.org/packages/0f/de/1b96ef04dd8c10d71ef49b20b79bcc1292ecf6c68332c007c1c6afc561f8/pollination_dsl-0.17.1-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "232fe4b2ac8e7156b8288b8588eb2804c2191a5f0ec511963576ea621304f8c0",
"md5": "89677239ce805af58d06523c61c035b9",
"sha256": "efd0455c074a922ef0e50478bd3a426963093806f04c033208264018a20e085e"
},
"downloads": -1,
"filename": "pollination_dsl-0.17.1.tar.gz",
"has_sig": false,
"md5_digest": "89677239ce805af58d06523c61c035b9",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 39392,
"upload_time": "2024-11-22T18:48:52",
"upload_time_iso_8601": "2024-11-22T18:48:52.470654Z",
"url": "https://files.pythonhosted.org/packages/23/2f/e4b2ac8e7156b8288b8588eb2804c2191a5f0ec511963576ea621304f8c0/pollination_dsl-0.17.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-22 18:48:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "pollination",
"github_project": "pollination-dsl",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "queenbee-pollination",
"specs": [
[
"==",
"0.7.10"
]
]
},
{
"name": "queenbee-local",
"specs": [
[
">=",
"0.6.7"
]
]
},
{
"name": "importlib-metadata",
"specs": [
[
">=",
"6.6.0"
]
]
}
],
"lcname": "pollination-dsl"
}