# postgrest-py
[PostgREST](https://postgrest.org) client for Python. This library provides an "ORM-like" interface to PostgREST.
## INSTALLATION
### Requirements
- Python >= 3.9
- PostgreSQL >= 13
- PostgREST >= 11
### Local PostgREST server
If you want to use a local PostgREST server for development, you can use our preconfigured instance via Docker Compose.
```sh
docker-compose up
```
Once Docker Compose started, PostgREST is accessible at <http://localhost:3000>.
### Instructions
#### With Poetry (recommended)
```sh
poetry add postgrest
```
#### With Pip
```sh
pip install postgrest
```
## USAGE
### Getting started
```py
import asyncio
from postgrest import AsyncPostgrestClient
async def main():
async with AsyncPostgrestClient("http://localhost:3000") as client:
r = await client.from_("countries").select("*").execute()
countries = r.data
asyncio.run(main())
```
### Create
```py
await client.from_("countries").insert({ "name": "Việt Nam", "capital": "Hà Nội" }).execute()
```
### Read
```py
r = await client.from_("countries").select("id", "name").execute()
countries = r.data
```
### Update
```py
await client.from_("countries").update({"capital": "Hà Nội"}).eq("name", "Việt Nam").execute()
```
### Delete
```py
await client.from_("countries").delete().eq("name", "Việt Nam").execute()
```
### General filters
### Stored procedures (RPC)
```py
await client.rpc("foobar", {"arg1": "value1", "arg2": "value2"}).execute()
```
## DEVELOPMENT
```sh
git clone https://github.com/supabase/postgrest-py.git
cd postgrest-py
poetry install
poetry run pre-commit install
```
### Testing
```sh
poetry run pytest
```
## CHANGELOG
Read more [here](https://github.com/supabase/postgrest-py/blob/main/CHANGELOG.md).
## SPONSORS
We are building the features of Firebase using enterprise-grade, open source products. We support existing communities wherever possible, and if the products don’t exist we build them and open source them ourselves. Thanks to these sponsors who are making the OSS ecosystem better for everyone.
[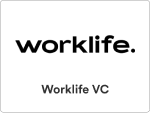](https://www.worklife.vc)
[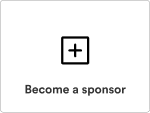](https://github.com/sponsors/supabase)
Raw data
{
"_id": null,
"home_page": "https://github.com/supabase/postgrest-py",
"name": "postgrest",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.9",
"maintainer_email": null,
"keywords": null,
"author": "L\u01b0\u01a1ng Quang M\u1ea1nh",
"author_email": "luongquangmanh85@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/63/80/b0306469da7ad89db165ce4c76de2f12eccc7fadb900cab9cbaff760a587/postgrest-0.19.3.tar.gz",
"platform": null,
"description": "# postgrest-py\n\n[PostgREST](https://postgrest.org) client for Python. This library provides an \"ORM-like\" interface to PostgREST.\n\n## INSTALLATION\n\n### Requirements\n\n- Python >= 3.9\n- PostgreSQL >= 13\n- PostgREST >= 11\n\n### Local PostgREST server\n\nIf you want to use a local PostgREST server for development, you can use our preconfigured instance via Docker Compose.\n\n```sh\ndocker-compose up\n```\n\nOnce Docker Compose started, PostgREST is accessible at <http://localhost:3000>.\n\n### Instructions\n\n#### With Poetry (recommended)\n\n```sh\npoetry add postgrest\n```\n\n#### With Pip\n\n```sh\npip install postgrest\n```\n\n## USAGE\n\n### Getting started\n\n```py\nimport asyncio\nfrom postgrest import AsyncPostgrestClient\n\nasync def main():\n async with AsyncPostgrestClient(\"http://localhost:3000\") as client:\n r = await client.from_(\"countries\").select(\"*\").execute()\n countries = r.data\n\nasyncio.run(main())\n```\n\n### Create\n\n```py\nawait client.from_(\"countries\").insert({ \"name\": \"Vi\u1ec7t Nam\", \"capital\": \"H\u00e0 N\u1ed9i\" }).execute()\n```\n\n### Read\n\n```py\nr = await client.from_(\"countries\").select(\"id\", \"name\").execute()\ncountries = r.data\n```\n\n### Update\n\n```py\nawait client.from_(\"countries\").update({\"capital\": \"H\u00e0 N\u1ed9i\"}).eq(\"name\", \"Vi\u1ec7t Nam\").execute()\n```\n\n### Delete\n\n```py\nawait client.from_(\"countries\").delete().eq(\"name\", \"Vi\u1ec7t Nam\").execute()\n```\n\n### General filters\n\n### Stored procedures (RPC)\n```py\nawait client.rpc(\"foobar\", {\"arg1\": \"value1\", \"arg2\": \"value2\"}).execute()\n```\n\n## DEVELOPMENT\n\n```sh\ngit clone https://github.com/supabase/postgrest-py.git\ncd postgrest-py\npoetry install\npoetry run pre-commit install\n```\n\n### Testing\n\n```sh\npoetry run pytest\n```\n\n## CHANGELOG\n\nRead more [here](https://github.com/supabase/postgrest-py/blob/main/CHANGELOG.md).\n\n## SPONSORS\n\nWe are building the features of Firebase using enterprise-grade, open source products. We support existing communities wherever possible, and if the products don\u2019t exist we build them and open source them ourselves. Thanks to these sponsors who are making the OSS ecosystem better for everyone.\n\n[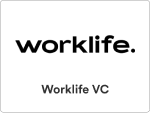](https://www.worklife.vc)\n[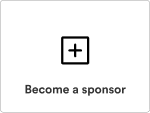](https://github.com/sponsors/supabase)\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "PostgREST client for Python. This library provides an ORM interface to PostgREST.",
"version": "0.19.3",
"project_urls": {
"Documentation": "https://postgrest-py.rtfd.io",
"Homepage": "https://github.com/supabase/postgrest-py",
"Repository": "https://github.com/supabase/postgrest-py"
},
"split_keywords": [],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "b082f1825a85745912cdd8956aad8ebc4b797d2f891c380c2b8825b35914dbd1",
"md5": "fad37793f7b245b8d754fa42f5d19fac",
"sha256": "03a7e638962454d10bb712c35e63a8a4bc452917917a4e9eb7427bd5b3c6c485"
},
"downloads": -1,
"filename": "postgrest-0.19.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "fad37793f7b245b8d754fa42f5d19fac",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.9",
"size": 22198,
"upload_time": "2025-01-24T22:24:54",
"upload_time_iso_8601": "2025-01-24T22:24:54.588494Z",
"url": "https://files.pythonhosted.org/packages/b0/82/f1825a85745912cdd8956aad8ebc4b797d2f891c380c2b8825b35914dbd1/postgrest-0.19.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "6380b0306469da7ad89db165ce4c76de2f12eccc7fadb900cab9cbaff760a587",
"md5": "2914d7de647340e02b7e406968fd4443",
"sha256": "28a70f03bf3a975aa865a10487b1ce09b7195f56453f7c318a70d3117a3d323c"
},
"downloads": -1,
"filename": "postgrest-0.19.3.tar.gz",
"has_sig": false,
"md5_digest": "2914d7de647340e02b7e406968fd4443",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.9",
"size": 15095,
"upload_time": "2025-01-24T22:24:56",
"upload_time_iso_8601": "2025-01-24T22:24:56.325181Z",
"url": "https://files.pythonhosted.org/packages/63/80/b0306469da7ad89db165ce4c76de2f12eccc7fadb900cab9cbaff760a587/postgrest-0.19.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-24 22:24:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "supabase",
"github_project": "postgrest-py",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "postgrest"
}