Name | prettier-prints JSON |
Version |
3.0.0
JSON |
| download |
home_page | None |
Summary | Lightweight library for prettier terminal outputs and debugging similar to chalk for Javascript |
upload_time | 2024-08-18 17:25:05 |
maintainer | None |
docs_url | None |
author | Dylan Stocking |
requires_python | None |
license | MIT |
keywords |
prints
chalk
output
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Prettier_prints
Lightweight library for prettier terminal outputs similar to [chalk](https://github.com/chalk/chalk) for JavaScript.
Helping make Python outputs easier to read and styled to your desires.
### Install
```python
pip install prettier-prints
```
Examples:
out()
```python
from prettier_prints.prettier_prints import PrettierPrints
pp = PrettierPrints()
pp.style = 'red;underline' # <-- Can optionally predefine styling and can be overwritten
# Old style (v1)
print(pp.out(msg="Lets test the output"))
print(pp.out(msg="Lets override the styling here", style="blue;bold"))
print(pp.out(json_out={'msg': 'For those who prefer a JSON object param, this works too'}))
print(pp.out(json_out={'msg': 'Can also overwrite this way', 'style': 'yellow;highlight'}))
print(f'Works great for output messages as well -> {pp.out(msg="See :)", style="magenta")}')
# New v2 style
from prettier_prints.prettier_printsv2 import Output
pp = Output()
print(f"\n{pp.msg('Hello').red().bold().underline()}")
print(f"{pp.msg('Hello').green().bold().out()}")
```

json()
```python
from prettier_prints.prettier_prints import PrettierPrints
pp = PrettierPrints()
json_obj = {'test': 'cool', 'test_two': 'cool_two', 'dict_check': {'test': 'hello'},
'list_check': [
'test',
'test two',
{
'test': 'hello',
'test_two': 'bloop'
},
{
'test': 'hello',
'test_two': 'bloop'
}
]}
print(prettier_prints.json(json_obj=json_obj, style='list=blue;underline&dict=red;bold&string=green;'))
```
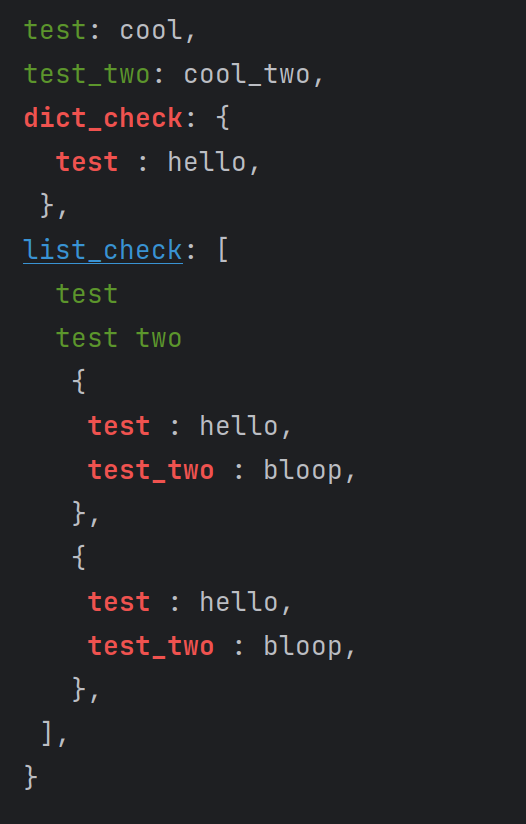
### Current functions:
- out()
- json()
### Function parameters:
- out()
- msg: str Output / display message[required]
- style: str Styling to apply to the message[optional]
- json_out: dict JSON object containing the message and styling[optional]
- json()
- style: str Styling to apply to the message[optional]
- json_obj: dict JSON object containing the message and styling[required]
### Styling Examples By Function (v1 style):
- out()
- style: 'red;underline'
- json_out: {'msg': 'Lets test the output', 'style': 'blue;bold'}
- json()
- style: 'list=red;underline&dict=blue;bold&str=yellow;highlight' # <- Break up the styling by type (list, dict, str)
### Current available styling:
| Modifiers | Colors / Background Colors |
|:----------|:--------------------------:|
| Bold | Red |
| Underline | Green |
| Highlight | Yellow |
| | Blue |
| | Magenta |
| | Cyan |
| | White |
| | Bright Red |
| | Bright Green |
| | Bright Yellow |
| | Bright Blue |
| | Bright Magenta |
| | Bright Cyan |
| | Bright White |
### Contributors / Thanks:
- [SheepDogg586](https://github.com/SheepDogg586)
Raw data
{
"_id": null,
"home_page": null,
"name": "prettier-prints",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "prints, chalk, output",
"author": "Dylan Stocking",
"author_email": "phantomleak@protonmail.com",
"download_url": "https://files.pythonhosted.org/packages/b4/68/181b5c02552bc6f0d43f5db2dc2846acdfbd42e7dce82d95f0a5958f8b33/prettier_prints-3.0.0.tar.gz",
"platform": null,
"description": "# Prettier_prints\n\nLightweight library for prettier terminal outputs similar to [chalk](https://github.com/chalk/chalk) for JavaScript. \nHelping make Python outputs easier to read and styled to your desires.\n\n### Install\n```python\npip install prettier-prints\n```\n\nExamples:\nout() \n```python\n from prettier_prints.prettier_prints import PrettierPrints\n pp = PrettierPrints()\n pp.style = 'red;underline' # <-- Can optionally predefine styling and can be overwritten\n \n # Old style (v1)\n print(pp.out(msg=\"Lets test the output\"))\n print(pp.out(msg=\"Lets override the styling here\", style=\"blue;bold\"))\n print(pp.out(json_out={'msg': 'For those who prefer a JSON object param, this works too'}))\n print(pp.out(json_out={'msg': 'Can also overwrite this way', 'style': 'yellow;highlight'}))\n print(f'Works great for output messages as well -> {pp.out(msg=\"See :)\", style=\"magenta\")}')\n\n # New v2 style\n from prettier_prints.prettier_printsv2 import Output\n pp = Output()\n print(f\"\\n{pp.msg('Hello').red().bold().underline()}\")\n print(f\"{pp.msg('Hello').green().bold().out()}\")\n```\n\n\njson()\n```python\n from prettier_prints.prettier_prints import PrettierPrints\n pp = PrettierPrints()\n json_obj = {'test': 'cool', 'test_two': 'cool_two', 'dict_check': {'test': 'hello'},\n 'list_check': [\n 'test',\n 'test two',\n {\n 'test': 'hello',\n 'test_two': 'bloop'\n },\n {\n 'test': 'hello',\n 'test_two': 'bloop'\n }\n ]}\n print(prettier_prints.json(json_obj=json_obj, style='list=blue;underline&dict=red;bold&string=green;'))\n```\n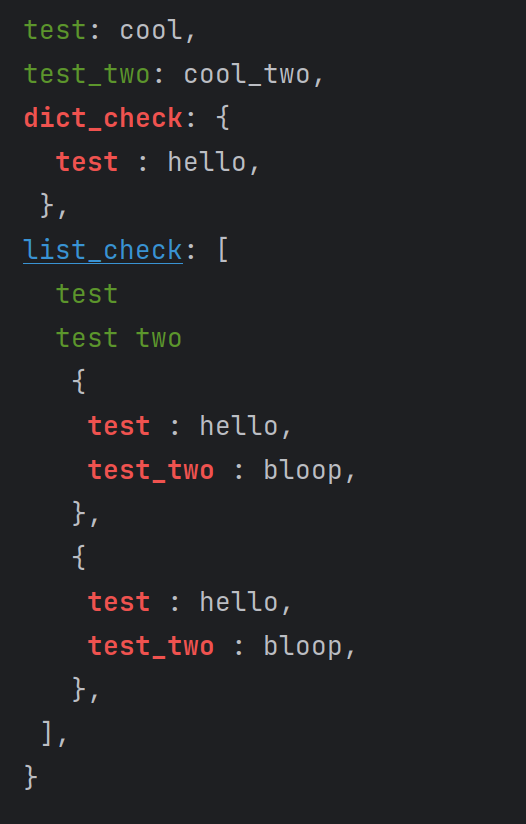\n\n### Current functions:\n - out()\n - json()\n\n### Function parameters:\n - out()\n - msg: str Output / display message[required]\n - style: str Styling to apply to the message[optional]\n - json_out: dict JSON object containing the message and styling[optional]\n\n - json()\n - style: str Styling to apply to the message[optional]\n - json_obj: dict JSON object containing the message and styling[required]\n \n### Styling Examples By Function (v1 style):\n - out()\n - style: 'red;underline'\n - json_out: {'msg': 'Lets test the output', 'style': 'blue;bold'}\n - json()\n - style: 'list=red;underline&dict=blue;bold&str=yellow;highlight' # <- Break up the styling by type (list, dict, str)\n\n### Current available styling:\n| Modifiers | Colors / Background Colors | \n|:----------|:--------------------------:|\n| Bold | Red |\n| Underline | Green | \n| Highlight | Yellow |\n| | Blue |\n| | Magenta |\n| | Cyan |\n| | White |\n| | Bright Red |\n| | Bright Green |\n| | Bright Yellow |\n| | Bright Blue |\n| | Bright Magenta |\n| | Bright Cyan |\n| | Bright White |\n\n### Contributors / Thanks:\n - [SheepDogg586](https://github.com/SheepDogg586)\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Lightweight library for prettier terminal outputs and debugging similar to chalk for Javascript",
"version": "3.0.0",
"project_urls": {
"Bug Tracker": "https://github.com/PhantomLeak/prettier_prints/issues",
"Code Repo": "https://github.com/PhantomLeak/prettier_prints"
},
"split_keywords": [
"prints",
" chalk",
" output"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b468181b5c02552bc6f0d43f5db2dc2846acdfbd42e7dce82d95f0a5958f8b33",
"md5": "2c74bec0ed6b45ffc01b0ca243226b54",
"sha256": "5715fb52d2e876ba768cb4116729f3e65e79a3d0ec7cbac7a3b4a355cf4a3f93"
},
"downloads": -1,
"filename": "prettier_prints-3.0.0.tar.gz",
"has_sig": false,
"md5_digest": "2c74bec0ed6b45ffc01b0ca243226b54",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 5224,
"upload_time": "2024-08-18T17:25:05",
"upload_time_iso_8601": "2024-08-18T17:25:05.744274Z",
"url": "https://files.pythonhosted.org/packages/b4/68/181b5c02552bc6f0d43f5db2dc2846acdfbd42e7dce82d95f0a5958f8b33/prettier_prints-3.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-18 17:25:05",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "PhantomLeak",
"github_project": "prettier_prints",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "prettier-prints"
}