






# **PriceHub**: Unified Python Package for Collecting OHLC Prices from Binance, Bybit, and Coinbase APIs into a DataFrame
It supports multiple markets, including spot and futures, and provides flexible timestamp inputs and a wide range of intervals.
Effective trading begins with thorough data analysis, visualization, and backtesting. This package simplifies access to such data, providing a unified solution for retrieving OHLC information across various broker APIs.
## Contents
- [Supported Brokers](#supported-brokers)
- [Key Features](#key-features)
- [Supported Intervals](#supported-intervals)
- [Installation](#installation)
- [Function Reference](#function-reference)
- [Example Usage](#example-usage)
- [Save data to CSV, Excel, Parquet files](#save-data-to-csv-excel-parquet-files)
- [Retrieve OHLC data from Binance Spot for a 6-hour interval](#retrieve-ohlc-data-from-binance-spot-for-a-6-hour-interval)
- [Retrieve OHLC data from Bybit Spot for a 1-day interval](#retrieve-ohlc-data-from-bybit-spot-for-a-1-day-interval)
- [Plot Close 1d data with matplotlib: BTCUSDT Futures on Binance for the last year](#plot-close-1d-data-with-matplotlib-btcusdt-futures-on-binance-for-the-last-year)
- [Plot OHLC 1w data with plotly: BTCUSDT Spot on Binance for the last five years](#plot-ohlc-1w-data-with-plotly-btcusdt-spot-on-binance-for-the-last-five-years)
- [Create custom intervals 10m for SOLUSDT Spot on Bybit for the last month](#create-custom-intervals-10m-for-solusdt-spot-on-bybit-for-the-last-month)
### Supported Brokers
- Binance Spot
- Binance Futures
- Bybit Spot
- Bybit Linear (Futures)
- Bybit Inverse
- Coinbase Spot
## Key Features
- **Unified Interface**: Supports multiple brokers and markets (spot, futures) with a single interface.
- **Unified Intervals**: Use the same interval format across all brokers.
- **Timestamp Flexibility**: Accepts timestamps (start, end) in various formats (int, float, string, Arrow, pandas, datetime).
- **No Credential Requirement**: Fetch public market data without authentication.
- **Extended Date Ranges**: This package will paginate and collect all data across large date ranges.
- **All fields from official API**: Retrieve all fields available in the official API (e.g., `Number of trades`, `Taker buy base asset volume`).
## Supported Intervals
(depends on the broker)
- **Seconds**: `1s`
- **Minutes**: `1m`, `3m`, `5m`, `15m`, `30m`
- **Hours**: `1h`, `2h`, `4h`, `6h`, `12h`
- **Days**: `1d`, `3d`
- **Weeks**: `1w`
- **Months**: `1M`
---
## Installation
```bash
pip install pricehub
```
## Function Reference
### `def get_ohlc(broker: SupportedBroker, symbol: str, interval: Interval, start: Timestamp, end: Timestamp) -> pd.DataFrame`
Retrieves OHLC data for the specified broker, symbol, interval, and date range.
- **Parameters**:
- `broker`: The broker to fetch data from (e.g., `binance_spot`, `bybit_spot`).
- `symbol`: The trading pair symbol (e.g., `BTCUSDT`).
- `interval`: The interval for OHLC data (`1m`, `1h`, `1d`, etc.).
- `start`: Start time of the data (supports various formats).
- `end`: End time of the data (supports various formats).
- **Returns**:
- `pandas.DataFrame`: A DataFrame containing OHLC data.
---
## Example Usage
### Save data to CSV, Excel, Parquet files
```python
from pricehub import get_ohlc
df = get_ohlc("binance_spot", "BTCUSDT", "1d", "2024-10-01", "2024-10-05")
df.to_csv("btcusdt_1d_2024-10-01_2024-10-05.csv") # Save to CSV
df.to_excel("btcusdt_1d_2024-10-01_2024-10-05.xlsx") # Save to Excel
df.to_parquet("btcusdt_1d_2024-10-01_2024-10-05.parquet") # Save to Parquet, requires 'pyarrow', 'fastparquet'
```
### Retrieve OHLC data from Binance Spot for a 6-hour interval
```python
from pricehub import get_ohlc
df = get_ohlc(
broker="binance_spot",
symbol="BTCUSDT",
interval="6h",
start="2024-10-01",
end="2024-10-02"
)
print(df)
```
```python
Open High Low Close Volume Close time Quote asset volume Number of trades Taker buy base asset volume Taker buy quote asset volume Ignore
Open time
2024-10-01 00:00:00 63309.0 63872.0 63000.0 63733.9 39397.714 2024-10-01 05:59:59.999 2.500830e+09 598784.0 19410.785 1.232417e+09 0.0
2024-10-01 06:00:00 63733.9 64092.6 63683.1 63699.9 32857.923 2024-10-01 11:59:59.999 2.100000e+09 446330.0 15865.753 1.014048e+09 0.0
2024-10-01 12:00:00 63700.0 63784.0 61100.0 62134.1 242613.990 2024-10-01 17:59:59.999 1.512287e+10 2583155.0 112641.347 7.022384e+09 0.0
2024-10-01 18:00:00 62134.1 62422.3 60128.2 60776.8 114948.208 2024-10-01 23:59:59.999 7.031801e+09 1461890.0 54123.788 3.312086e+09 0.0
2024-10-02 00:00:00 60776.7 61858.2 60703.3 61466.7 51046.012 2024-10-02 05:59:59.999 3.133969e+09 668558.0 27191.919 1.669187e+09 0.0
```
### Retrieve OHLC data from Bybit Spot for a 1-day interval
```python
from pricehub import get_ohlc
df = get_ohlc(
broker="bybit_spot",
symbol="ETHUSDT",
interval="1d",
start=1727740800.0, # Unix timestamp in seconds for "2024-10-01"
end=1728086400000, # Unix timestamp in ms for "2024-10-05"
)
print(df)
```
```python
Open High Low Close Volume Turnover
Open time
2024-10-01 2602.00 2659.31 2413.15 2447.95 376729.77293 9.623060e+08
2024-10-02 2447.95 2499.82 2351.53 2364.01 242498.88477 5.914189e+08
2024-10-03 2364.01 2403.50 2309.75 2349.91 242598.38255 5.716546e+08
2024-10-04 2349.91 2441.82 2339.15 2414.67 178050.43782 4.254225e+08
2024-10-05 2414.67 2428.69 2389.83 2414.54 106665.69595 2.573030e+08
```
### Plot Close 1d data with matplotlib: BTCUSDT Futures on Binance for the last year
```python
from datetime import datetime, timedelta
import matplotlib.pyplot as plt
from pricehub import get_ohlc
now = datetime.now()
df = get_ohlc("binance_futures", "BTCUSDT", "1d", now - timedelta(days=365), now)
df["Close"].plot()
plt.show()
```
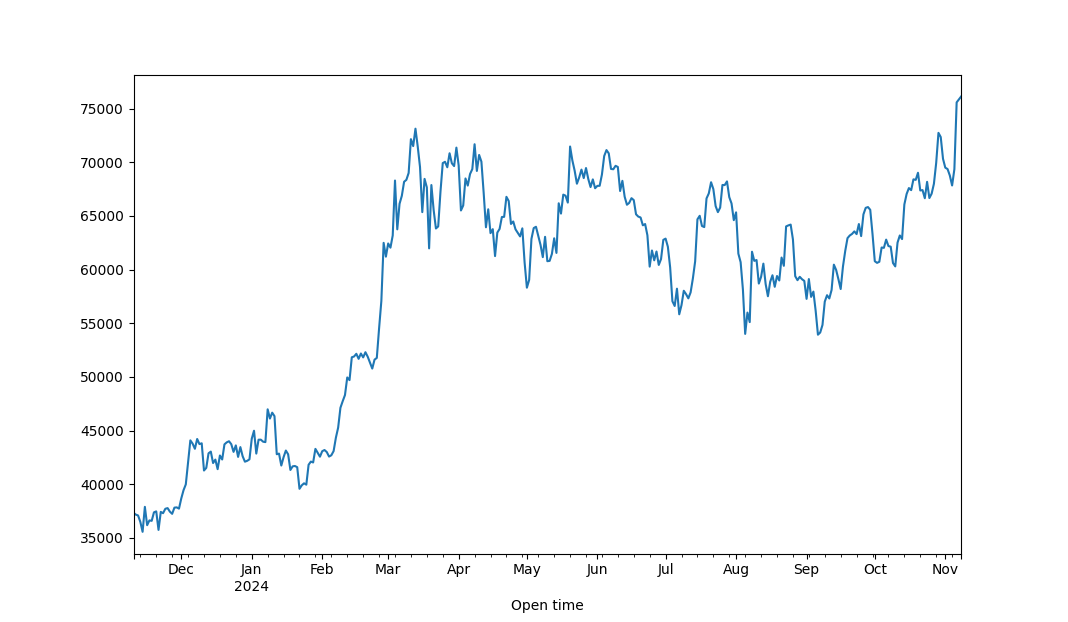
### Plot OHLC 1w data with plotly: BTCUSDT Spot on Binance for the last five years
```python
from datetime import datetime, timedelta
import plotly.graph_objects as go
from pricehub import get_ohlc
now = datetime.now()
df = get_ohlc("binance_spot", "BTCUSDT", "1w", now - timedelta(days=365 * 5), now)
fig = go.Figure(data=go.Candlestick(x=df.index, open=df['Open'], high=df['High'], low=df['Low'], close=df['Close']))
fig.update_layout()
fig.show()
```
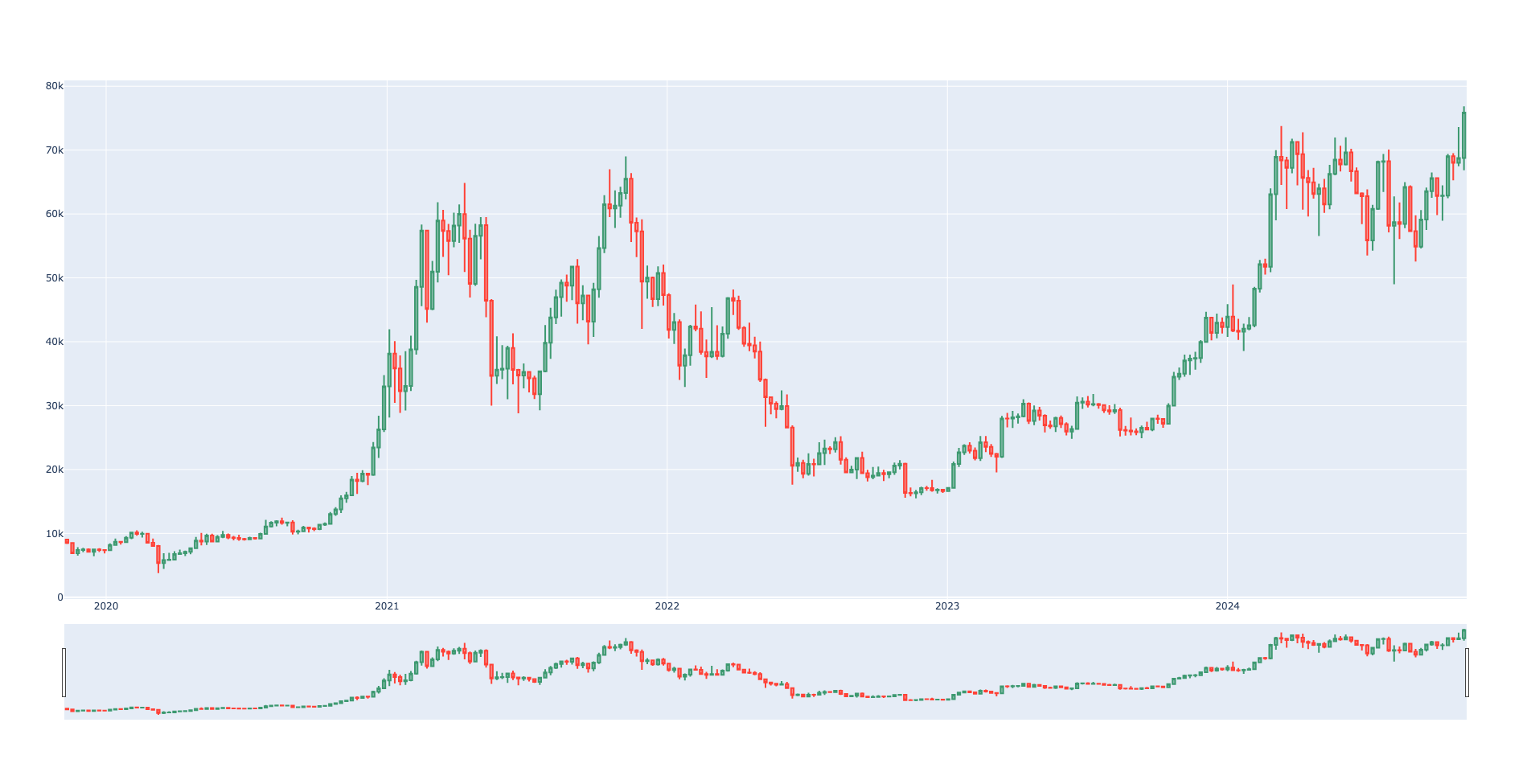
### Create custom intervals 10m for SOLUSDT Spot on Bybit for the last month
```python
from datetime import datetime, timedelta
from pricehub import get_ohlc
now = datetime.now()
df = get_ohlc("bybit_spot", "SOLUSDT", "5m", now - timedelta(days=31), now)
df_10m = (
df.resample(
"10min",
).agg(
{
"Open": "first",
"High": "max",
"Low": "min",
"Close": "last",
"Volume": "sum",
}
)
)
print(df.head())
print(df_10m.head())
```
```python
#5m
Open High Low Close Volume Turnover
Open time
2024-11-07 17:40:00 194.13 194.66 194.03 194.54 3391.378 6.592576e+05
2024-11-07 17:45:00 194.54 195.48 194.44 195.41 6075.927 1.184312e+06
2024-11-07 17:50:00 195.41 195.71 195.06 195.69 4073.276 7.961276e+05
2024-11-07 17:55:00 195.69 196.16 195.59 195.93 8774.224 1.719060e+06
2024-11-07 18:00:00 195.93 196.83 195.73 196.34 5075.807 9.973238e+05
#10m
Open High Low Close Volume
Open time
2024-11-07 17:40:00 194.13 195.48 194.03 195.41 9467.305
2024-11-07 17:50:00 195.41 196.16 195.06 195.93 12847.500
2024-11-07 18:00:00 195.93 196.83 194.66 195.29 12506.671
2024-11-07 18:10:00 195.29 196.13 194.70 195.58 20437.030
2024-11-07 18:20:00 195.58 196.00 194.84 195.81 16388.688
```
Raw data
{
"_id": null,
"home_page": "https://github.com/eslazarev/pricehub",
"name": "pricehub",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "OHLC OHLCV prices binance bybit coinbase trading finance quant algotrading cryptocurrency crypto futures spot broker exchange market quantitative historical candlestick back-testing dataframe",
"author": "Evgenii Lazarev",
"author_email": "elazarev@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/8c/bf/7280b24e97328eaa2df125030956d83ba768416cdde50b6e8d418d8ac4ab/pricehub-0.0.3.13.tar.gz",
"platform": null,
"description": "\n\n\n\n\n\n\n\n\n# **PriceHub**: Unified Python Package for Collecting OHLC Prices from Binance, Bybit, and Coinbase APIs into a DataFrame\n\nIt supports multiple markets, including spot and futures, and provides flexible timestamp inputs and a wide range of intervals.\n\nEffective trading begins with thorough data analysis, visualization, and backtesting. This package simplifies access to such data, providing a unified solution for retrieving OHLC information across various broker APIs.\n\n## Contents\n- [Supported Brokers](#supported-brokers)\n- [Key Features](#key-features)\n- [Supported Intervals](#supported-intervals)\n- [Installation](#installation)\n- [Function Reference](#function-reference)\n- [Example Usage](#example-usage)\n - [Save data to CSV, Excel, Parquet files](#save-data-to-csv-excel-parquet-files)\n - [Retrieve OHLC data from Binance Spot for a 6-hour interval](#retrieve-ohlc-data-from-binance-spot-for-a-6-hour-interval)\n - [Retrieve OHLC data from Bybit Spot for a 1-day interval](#retrieve-ohlc-data-from-bybit-spot-for-a-1-day-interval)\n - [Plot Close 1d data with matplotlib: BTCUSDT Futures on Binance for the last year](#plot-close-1d-data-with-matplotlib-btcusdt-futures-on-binance-for-the-last-year)\n - [Plot OHLC 1w data with plotly: BTCUSDT Spot on Binance for the last five years](#plot-ohlc-1w-data-with-plotly-btcusdt-spot-on-binance-for-the-last-five-years)\n - [Create custom intervals 10m for SOLUSDT Spot on Bybit for the last month](#create-custom-intervals-10m-for-solusdt-spot-on-bybit-for-the-last-month)\n\n\n### Supported Brokers\n- Binance Spot\n- Binance Futures\n- Bybit Spot\n- Bybit Linear (Futures)\n- Bybit Inverse\n- Coinbase Spot\n\n## Key Features\n\n- **Unified Interface**: Supports multiple brokers and markets (spot, futures) with a single interface.\n- **Unified Intervals**: Use the same interval format across all brokers.\n- **Timestamp Flexibility**: Accepts timestamps (start, end) in various formats (int, float, string, Arrow, pandas, datetime).\n- **No Credential Requirement**: Fetch public market data without authentication.\n- **Extended Date Ranges**: This package will paginate and collect all data across large date ranges.\n- **All fields from official API**: Retrieve all fields available in the official API (e.g., `Number of trades`, `Taker buy base asset volume`). \n\n## Supported Intervals\n(depends on the broker)\n- **Seconds**: `1s`\n- **Minutes**: `1m`, `3m`, `5m`, `15m`, `30m`\n- **Hours**: `1h`, `2h`, `4h`, `6h`, `12h`\n- **Days**: `1d`, `3d`\n- **Weeks**: `1w`\n- **Months**: `1M`\n\n---\n\n## Installation\n\n```bash\npip install pricehub\n```\n\n## Function Reference\n\n### `def get_ohlc(broker: SupportedBroker, symbol: str, interval: Interval, start: Timestamp, end: Timestamp) -> pd.DataFrame`\n\nRetrieves OHLC data for the specified broker, symbol, interval, and date range.\n\n- **Parameters**:\n - `broker`: The broker to fetch data from (e.g., `binance_spot`, `bybit_spot`).\n - `symbol`: The trading pair symbol (e.g., `BTCUSDT`).\n - `interval`: The interval for OHLC data (`1m`, `1h`, `1d`, etc.).\n - `start`: Start time of the data (supports various formats).\n - `end`: End time of the data (supports various formats).\n\n- **Returns**:\n - `pandas.DataFrame`: A DataFrame containing OHLC data.\n\n---\n\n## Example Usage\n\n### Save data to CSV, Excel, Parquet files\n```python\n\nfrom pricehub import get_ohlc\ndf = get_ohlc(\"binance_spot\", \"BTCUSDT\", \"1d\", \"2024-10-01\", \"2024-10-05\")\ndf.to_csv(\"btcusdt_1d_2024-10-01_2024-10-05.csv\") # Save to CSV\ndf.to_excel(\"btcusdt_1d_2024-10-01_2024-10-05.xlsx\") # Save to Excel\ndf.to_parquet(\"btcusdt_1d_2024-10-01_2024-10-05.parquet\") # Save to Parquet, requires 'pyarrow', 'fastparquet'\n```\n\n\n### Retrieve OHLC data from Binance Spot for a 6-hour interval\n```python\nfrom pricehub import get_ohlc\n\ndf = get_ohlc(\n broker=\"binance_spot\",\n symbol=\"BTCUSDT\",\n interval=\"6h\",\n start=\"2024-10-01\",\n end=\"2024-10-02\"\n)\nprint(df)\n```\n\n```python\n Open High Low Close Volume Close time Quote asset volume Number of trades Taker buy base asset volume Taker buy quote asset volume Ignore\nOpen time \n2024-10-01 00:00:00 63309.0 63872.0 63000.0 63733.9 39397.714 2024-10-01 05:59:59.999 2.500830e+09 598784.0 19410.785 1.232417e+09 0.0\n2024-10-01 06:00:00 63733.9 64092.6 63683.1 63699.9 32857.923 2024-10-01 11:59:59.999 2.100000e+09 446330.0 15865.753 1.014048e+09 0.0\n2024-10-01 12:00:00 63700.0 63784.0 61100.0 62134.1 242613.990 2024-10-01 17:59:59.999 1.512287e+10 2583155.0 112641.347 7.022384e+09 0.0\n2024-10-01 18:00:00 62134.1 62422.3 60128.2 60776.8 114948.208 2024-10-01 23:59:59.999 7.031801e+09 1461890.0 54123.788 3.312086e+09 0.0\n2024-10-02 00:00:00 60776.7 61858.2 60703.3 61466.7 51046.012 2024-10-02 05:59:59.999 3.133969e+09 668558.0 27191.919 1.669187e+09 0.0\n```\n\n### Retrieve OHLC data from Bybit Spot for a 1-day interval\n```python\nfrom pricehub import get_ohlc\n\ndf = get_ohlc(\n broker=\"bybit_spot\",\n symbol=\"ETHUSDT\",\n interval=\"1d\",\n start=1727740800.0, # Unix timestamp in seconds for \"2024-10-01\"\n end=1728086400000, # Unix timestamp in ms for \"2024-10-05\"\n)\nprint(df)\n```\n\n```python\n Open High Low Close Volume Turnover\nOpen time \n2024-10-01 2602.00 2659.31 2413.15 2447.95 376729.77293 9.623060e+08\n2024-10-02 2447.95 2499.82 2351.53 2364.01 242498.88477 5.914189e+08\n2024-10-03 2364.01 2403.50 2309.75 2349.91 242598.38255 5.716546e+08\n2024-10-04 2349.91 2441.82 2339.15 2414.67 178050.43782 4.254225e+08\n2024-10-05 2414.67 2428.69 2389.83 2414.54 106665.69595 2.573030e+08\n```\n\n### Plot Close 1d data with matplotlib: BTCUSDT Futures on Binance for the last year\n```python\nfrom datetime import datetime, timedelta\n\nimport matplotlib.pyplot as plt\nfrom pricehub import get_ohlc\n\nnow = datetime.now()\ndf = get_ohlc(\"binance_futures\", \"BTCUSDT\", \"1d\", now - timedelta(days=365), now)\ndf[\"Close\"].plot()\nplt.show()\n```\n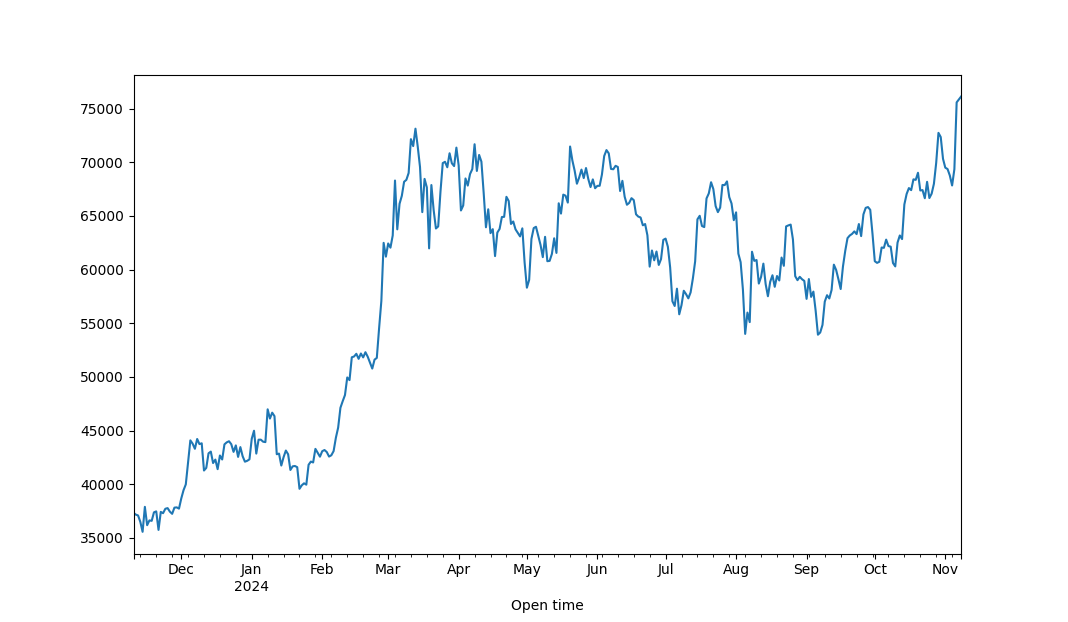\n\n\n### Plot OHLC 1w data with plotly: BTCUSDT Spot on Binance for the last five years\n```python\nfrom datetime import datetime, timedelta\nimport plotly.graph_objects as go\nfrom pricehub import get_ohlc\n\nnow = datetime.now()\ndf = get_ohlc(\"binance_spot\", \"BTCUSDT\", \"1w\", now - timedelta(days=365 * 5), now)\n\nfig = go.Figure(data=go.Candlestick(x=df.index, open=df['Open'], high=df['High'], low=df['Low'], close=df['Close']))\n\nfig.update_layout()\nfig.show()\n```\n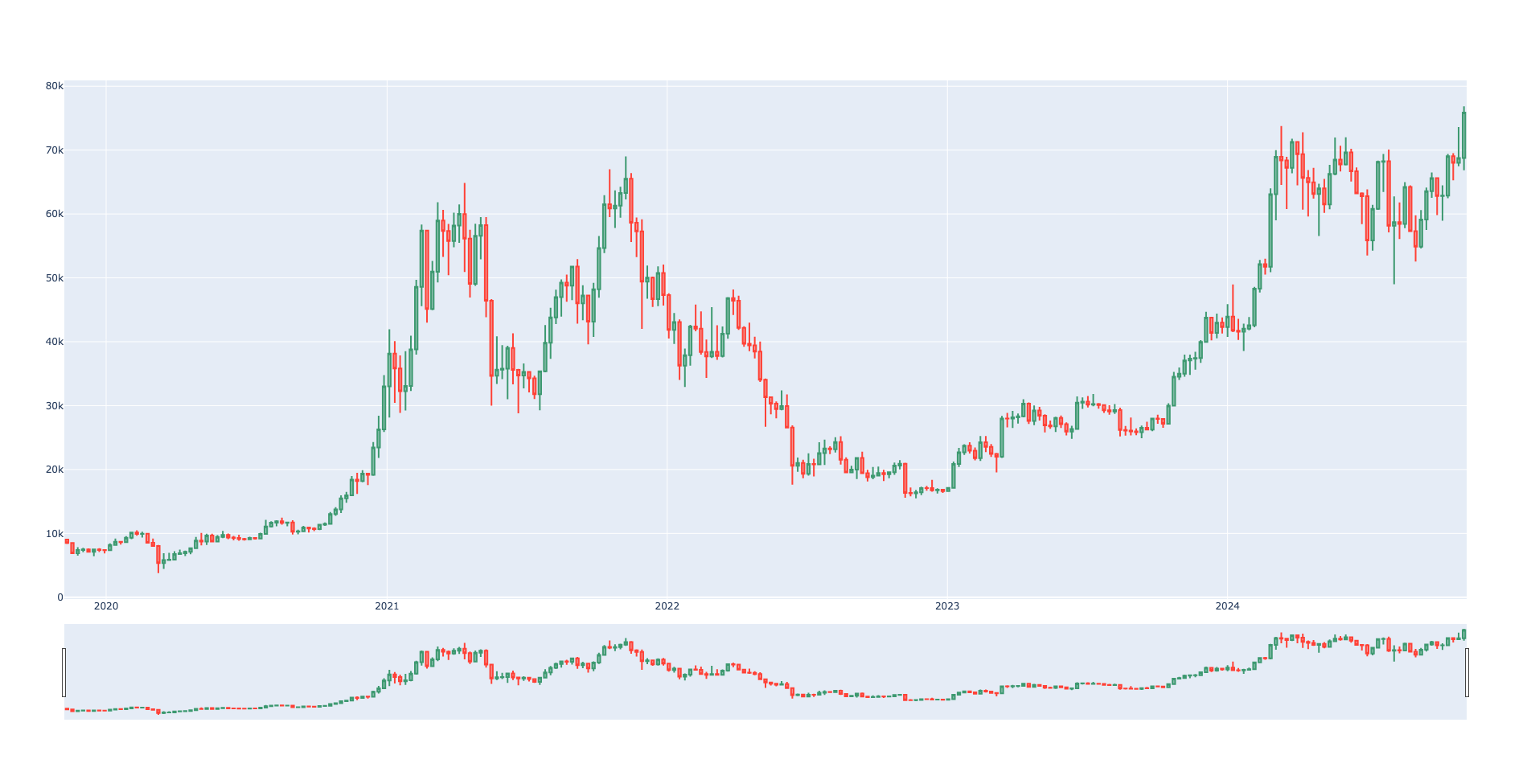\n\n\n\n### Create custom intervals 10m for SOLUSDT Spot on Bybit for the last month\n```python\nfrom datetime import datetime, timedelta\nfrom pricehub import get_ohlc\nnow = datetime.now()\ndf = get_ohlc(\"bybit_spot\", \"SOLUSDT\", \"5m\", now - timedelta(days=31), now)\ndf_10m = (\n df.resample(\n \"10min\",\n ).agg(\n {\n \"Open\": \"first\",\n \"High\": \"max\",\n \"Low\": \"min\",\n \"Close\": \"last\",\n \"Volume\": \"sum\",\n }\n )\n)\nprint(df.head())\nprint(df_10m.head())\n```\n\n\n```python\n#5m\n Open High Low Close Volume Turnover\nOpen time \n2024-11-07 17:40:00 194.13 194.66 194.03 194.54 3391.378 6.592576e+05\n2024-11-07 17:45:00 194.54 195.48 194.44 195.41 6075.927 1.184312e+06\n2024-11-07 17:50:00 195.41 195.71 195.06 195.69 4073.276 7.961276e+05\n2024-11-07 17:55:00 195.69 196.16 195.59 195.93 8774.224 1.719060e+06\n2024-11-07 18:00:00 195.93 196.83 195.73 196.34 5075.807 9.973238e+05\n\n#10m\n Open High Low Close Volume\nOpen time \n2024-11-07 17:40:00 194.13 195.48 194.03 195.41 9467.305\n2024-11-07 17:50:00 195.41 196.16 195.06 195.93 12847.500\n2024-11-07 18:00:00 195.93 196.83 194.66 195.29 12506.671\n2024-11-07 18:10:00 195.29 196.13 194.70 195.58 20437.030\n2024-11-07 18:20:00 195.58 196.00 194.84 195.81 16388.688\n```\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Pricehub: Unified Python Package for Collecting OHLC Prices from Binance, Bybit, and Coinbase APIs into a DataFrame",
"version": "0.0.3.13",
"project_urls": {
"GitHub": "https://github.com/eslazarev",
"Homepage": "https://github.com/eslazarev/pricehub",
"LinkedIn": "https://www.linkedin.com/in/elazarev"
},
"split_keywords": [
"ohlc",
"ohlcv",
"prices",
"binance",
"bybit",
"coinbase",
"trading",
"",
"finance",
"quant",
"algotrading",
"cryptocurrency",
"crypto",
"futures",
"spot",
"broker",
"exchange",
"market",
"quantitative",
"historical",
"candlestick",
"back-testing",
"dataframe"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "92918998c23928be3d2cf9ed136bff8f2bcbfd44354cf723523a4bb4caa335c9",
"md5": "54ca1047d6f5c5a46d2f8e18dfae7ec2",
"sha256": "b991cb03558c80bd5da957f079425f2f8a7afc3656516f869e3410b6b7b25b82"
},
"downloads": -1,
"filename": "pricehub-0.0.3.13-py3-none-any.whl",
"has_sig": false,
"md5_digest": "54ca1047d6f5c5a46d2f8e18dfae7ec2",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 18045,
"upload_time": "2024-11-23T14:29:50",
"upload_time_iso_8601": "2024-11-23T14:29:50.199086Z",
"url": "https://files.pythonhosted.org/packages/92/91/8998c23928be3d2cf9ed136bff8f2bcbfd44354cf723523a4bb4caa335c9/pricehub-0.0.3.13-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8cbf7280b24e97328eaa2df125030956d83ba768416cdde50b6e8d418d8ac4ab",
"md5": "0a6cd7b6b35d3389f41a4827c80d4741",
"sha256": "02d9c466d370aae071741014ccee2b43f6217483187f4d9db122c507d61e81f2"
},
"downloads": -1,
"filename": "pricehub-0.0.3.13.tar.gz",
"has_sig": false,
"md5_digest": "0a6cd7b6b35d3389f41a4827c80d4741",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 17386,
"upload_time": "2024-11-23T14:29:51",
"upload_time_iso_8601": "2024-11-23T14:29:51.931460Z",
"url": "https://files.pythonhosted.org/packages/8c/bf/7280b24e97328eaa2df125030956d83ba768416cdde50b6e8d418d8ac4ab/pricehub-0.0.3.13.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-23 14:29:51",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "eslazarev",
"github_project": "pricehub",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "pricehub"
}