# Progresspanel
The Progresspanel is a custom tkinter widget that displays a progress bar and
buttons (Start/Resume, Pause, Terminate) to control a user-defined task that
runs in a separate thread. The widget is implemented in progresspanel.py.
# Installation
You can install Progresspanel using pip for the Python environment:
```
pip install progresspanel
```
# Usage
To use the Progress Panel, you need to create an instance of the Progresspanel
class and pass it a parent widget, a total number of iterations, and a task
function to be run in a loop.
Here below is an example code that creates a window with a progress bar and
buttons to control the task as shown in the GIF:
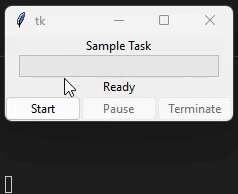
```python
import tkinter as tk
from progresspanel import Progresspanel
from time import sleep
root = tk.Tk()
panel = Progresspanel(root, title="Sample Task")
panel.pack()
def sample_task():
total = 5
panel.set_total(total)
for i in range(total):
panel.update(i)
print("Running iteration: {}".format(i))
sleep(1)
panel.set_task(sample_task)
root.mainloop()
```
The task function simply sleeps for one second five times and updates the
progress bar each time.
You can customize the task function to do whatever you want. You can also
customize the title of the progress panel and enable/disable verbose mode to
print status updates to the console. There're also advanced features that let
you pause execution in between time-consuming methods in your own task, and
features that let you repeat the last iteration right after resuming from a
pausing. Check below APIs for more details.
# API
### Progresspanel(parent, total=1, task=None, title=None, verbose=True)
Create a new Progresspanel widget.
* parent: the parent widget.
* total: the total number of iterations for the task.
* task: the task function to be run in a loop.
* title: the title of the progress panel.
* display_time_left: a bool to determine whether to display the remaining time
in the status label, default to True.
* verbose: whether to print status updates to the console.
### set_total(total)
Set the total number of iterations for the task.
### set_task(task)
Set the task function.
### update(i)
Update the progress bar with the current iteration number.
### set_verbose(verbose)
Enable/disable verbose mode to print status updates to the console.
### is_pausing_or_terminating()
Check if the task is in a pausing or terminating state. This is useful for user
to stop promptly before running other time-consuming operations in user-defined
task after user clicks pause or terminate button.
### is_pause_resumed()
Check whether the task was just resumed after a pause. It returns True if the
progress is just resumed after it was paused, until the next iteration in which
it returns False again. It also returns False in all other cases. This is useful
if user wants to repeat current iteration after the work was resumed from pause,
especially when the user configured to jump over some customized time-comsuming
operations in task() using is_pausing_or_terminating() after the pause button
was clicked.
### after_started()
Placeholder for user-defined function to be run when the task is started.
### after_resumed()
Placeholder for user-defined function to be run when the task is resumed.
### after_paused()
Placeholder for user-defined function to be run when the task is paused.
### after_terminated()
Placeholder for user-defined function to be run when the task is terminated.
### after_completed()
Placeholder for user-defined function to be run when the task is completed.
# License
Progresspanel is released under the MIT License. See LICENSE for more
information.
Raw data
{
"_id": null,
"home_page": "https://github.com/xuejianma/progresspanel",
"name": "progresspanel",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "tkinter,progress panel,progress bar,threading,multi thread",
"author": "Xuejian Ma",
"author_email": "Xuejian.Ma@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/5e/ed/64bb4a92f88d0117d0ae75eac9f7df9d4484538d698bc3662e0ccdef67d0/progresspanel-0.1.2.tar.gz",
"platform": null,
"description": "# Progresspanel\r\nThe Progresspanel is a custom tkinter widget that displays a progress bar and\r\nbuttons (Start/Resume, Pause, Terminate) to control a user-defined task that\r\nruns in a separate thread. The widget is implemented in progresspanel.py.\r\n\r\n# Installation\r\nYou can install Progresspanel using pip for the Python environment:\r\n```\r\npip install progresspanel\r\n```\r\n\r\n# Usage\r\nTo use the Progress Panel, you need to create an instance of the Progresspanel\r\nclass and pass it a parent widget, a total number of iterations, and a task\r\nfunction to be run in a loop.\r\n\r\nHere below is an example code that creates a window with a progress bar and\r\nbuttons to control the task as shown in the GIF:\r\n\r\n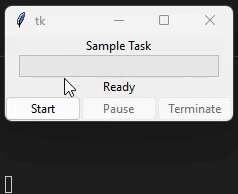\r\n\r\n```python\r\nimport tkinter as tk\r\nfrom progresspanel import Progresspanel\r\nfrom time import sleep\r\n\r\nroot = tk.Tk()\r\npanel = Progresspanel(root, title=\"Sample Task\")\r\npanel.pack()\r\n\r\ndef sample_task():\r\n total = 5\r\n panel.set_total(total)\r\n for i in range(total):\r\n panel.update(i)\r\n print(\"Running iteration: {}\".format(i))\r\n sleep(1)\r\n\r\npanel.set_task(sample_task)\r\nroot.mainloop()\r\n\r\n```\r\n\r\nThe task function simply sleeps for one second five times and updates the\r\nprogress bar each time.\r\n\r\nYou can customize the task function to do whatever you want. You can also\r\ncustomize the title of the progress panel and enable/disable verbose mode to\r\nprint status updates to the console. There're also advanced features that let\r\nyou pause execution in between time-consuming methods in your own task, and\r\nfeatures that let you repeat the last iteration right after resuming from a\r\npausing. Check below APIs for more details.\r\n\r\n# API\r\n### Progresspanel(parent, total=1, task=None, title=None, verbose=True)\r\nCreate a new Progresspanel widget.\r\n\r\n* parent: the parent widget.\r\n* total: the total number of iterations for the task.\r\n* task: the task function to be run in a loop.\r\n* title: the title of the progress panel.\r\n* display_time_left: a bool to determine whether to display the remaining time\r\nin the status label, default to True.\r\n* verbose: whether to print status updates to the console.\r\n\r\n### set_total(total)\r\nSet the total number of iterations for the task.\r\n\r\n### set_task(task)\r\nSet the task function.\r\n\r\n### update(i)\r\nUpdate the progress bar with the current iteration number.\r\n\r\n### set_verbose(verbose)\r\nEnable/disable verbose mode to print status updates to the console.\r\n\r\n### is_pausing_or_terminating()\r\nCheck if the task is in a pausing or terminating state. This is useful for user\r\nto stop promptly before running other time-consuming operations in user-defined\r\ntask after user clicks pause or terminate button.\r\n\r\n### is_pause_resumed()\r\nCheck whether the task was just resumed after a pause. It returns True if the\r\nprogress is just resumed after it was paused, until the next iteration in which\r\nit returns False again. It also returns False in all other cases. This is useful\r\nif user wants to repeat current iteration after the work was resumed from pause,\r\nespecially when the user configured to jump over some customized time-comsuming\r\noperations in task() using is_pausing_or_terminating() after the pause button\r\nwas clicked.\r\n\r\n### after_started()\r\nPlaceholder for user-defined function to be run when the task is started.\r\n\r\n### after_resumed()\r\nPlaceholder for user-defined function to be run when the task is resumed.\r\n\r\n### after_paused()\r\nPlaceholder for user-defined function to be run when the task is paused.\r\n\r\n### after_terminated()\r\nPlaceholder for user-defined function to be run when the task is terminated.\r\n\r\n### after_completed()\r\nPlaceholder for user-defined function to be run when the task is completed.\r\n\r\n# License\r\nProgresspanel is released under the MIT License. See LICENSE for more\r\ninformation.\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "The Progress Panel is a custom tkinter widget that displays a progress bar and buttons (Start/Resume, Pause, Terminate) to control a user-defined task that runs in a separate thread.",
"version": "0.1.2",
"project_urls": {
"Homepage": "https://github.com/xuejianma/progresspanel"
},
"split_keywords": [
"tkinter",
"progress panel",
"progress bar",
"threading",
"multi thread"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "19283dee0168118bd0d50c90c3c2b367886cf42cc8b6d34a271ca64e619a4d09",
"md5": "2fa5219ee40df30c2d08652074cb69df",
"sha256": "098fb0135ef1a57f71ce95f487572e77cd9ef29d1e24ef79ae8dd5d918b20224"
},
"downloads": -1,
"filename": "progresspanel-0.1.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2fa5219ee40df30c2d08652074cb69df",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 7078,
"upload_time": "2023-11-13T02:23:01",
"upload_time_iso_8601": "2023-11-13T02:23:01.176140Z",
"url": "https://files.pythonhosted.org/packages/19/28/3dee0168118bd0d50c90c3c2b367886cf42cc8b6d34a271ca64e619a4d09/progresspanel-0.1.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5eed64bb4a92f88d0117d0ae75eac9f7df9d4484538d698bc3662e0ccdef67d0",
"md5": "6e07f5792b0bd7a8930db2aaed16a263",
"sha256": "c41c23fad3126b0f336e51025d4be193d6ad154705450496db222810f9e43af5"
},
"downloads": -1,
"filename": "progresspanel-0.1.2.tar.gz",
"has_sig": false,
"md5_digest": "6e07f5792b0bd7a8930db2aaed16a263",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 6611,
"upload_time": "2023-11-13T02:23:02",
"upload_time_iso_8601": "2023-11-13T02:23:02.871883Z",
"url": "https://files.pythonhosted.org/packages/5e/ed/64bb4a92f88d0117d0ae75eac9f7df9d4484538d698bc3662e0ccdef67d0/progresspanel-0.1.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-13 02:23:02",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "xuejianma",
"github_project": "progresspanel",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "progresspanel"
}