# PV_Live
A Python implementation of the PV_Live web API. See https://www.solar.sheffield.ac.uk/pvlive/
**Latest Version: 1.5.1**
## About this repository
* This Python library provides a convenient interface for the PV_Live web API to facilitate accessing PV_Live results in Python code.
* Developed and tested with Python 3.10, should work with Python 3.7+. Support for Python 2.7+ has been discontinued as of 2021-01-15.
## How do I get set up?
* Make sure you have Git installed - [Download Git](https://git-scm.com/downloads)
* Run either:
* `pip install pvlive-api`
* `pip install git+https://github.com/SheffieldSolar/PV_Live-API`
## Usage
As of 2025-07-07, the production PV_Live API is hosted on Google Cloud Platform (GCP) at https://api.pvlive.uk. There is a also non-prod test/fix-on-fail (FOF) environment hosted on TUOS IT: https://api.solar.sheffield.ac.uk. To support switching between the two, the `pvlive-api` package exposes a parameter `domain_url`, which can be set to one of `["api.pvlive.uk", "api.solar.sheffield.ac.uk"]` but defaults to `api.pvlive.uk`.
There are three methods for extracting raw data from the PV_Live API:
|Method|Description|Docs Link|
|------|-----------|---------|
|`PVLive.latest(entity_type="pes", entity_id=0, extra_fields="", period=30, dataframe=False)`|Get the latest PV_Live generation result from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.latest)|
|`PVLive.at_time(dt, entity_type="pes", entity_id=0, extra_fields="", period=30, dataframe=False)`|Get the PV_Live generation result for a given time from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.at_time)|
|`PVLive.between(start, end, entity_type="pes", entity_id=0, extra_fields="", period=30, dataframe=False)`|Get the PV_Live generation result for a given time interval from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.between)|
There are two methods for extracting derived statistics:
|Method|Description|Docs Link|
|------|-----------|---------|
|`PVLive.day_peak(d, entity_type="pes", entity_id=0, extra_fields="", period=30, dataframe=False)`|Get the peak PV_Live generation result for a given day from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.day_peak)|
|`PVLive.day_energy(d, entity_type="pes", entity_id=0)`|Get the cumulative PV generation for a given day from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.day_energy)|
These methods include the following optional parameters:
|Parameter|Usage|
|---------|-----|
|`entity_type`|Choose between `"pes"` or `"gsp"`. If querying for national data, this parameter can be set to either value (or left to it's default value) since setting `entity_id` to `0` will always return national data.|
|`entity_id`|Set `entity_id=0` (the default value) to return nationally aggregated data. If `entity_type="pes"`, specify a _pes_id_ to retrieve data for, else if `entity_id="gsp"`, specify a _gsp_id_. For a full list of GSP and PES IDs, refer to the lookup table hosted on National Grid ESO's data portal [here](https://data.nationalgrideso.com/system/gis-boundaries-for-gb-grid-supply-points).|
|`extra_fields`|Use this to extract additional fields from the API such as _installedcapacity_mwp_. For a full list of available fields, see the [PV_Live API Docs](https://www.solar.sheffield.ac.uk/pvlive/api/).|
|`period`|Set the desired temporal resolution (in minutes) for PV outturn estimates. Options are 30 (default) or 5.|
|`dataframe`|Set `dataframe=True` and the results will be returned as a Pandas DataFrame object which is generally much easier to work with. The columns of the DataFrame will be _pes_id_ or _gsp_id_, _datetime_gmt_, _generation_mw_, plus any extra fields specified.|
There is also a method for extracting PV deployment (a.k.a capacity) data:
|Method|Description|Docs Link|
|------|-----------|---------|
|`PVLive.deployment(region="gsp", include_history=False, by_system_size=False, release=0)`|Download PV deployment datasets from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.deployment)|
## Code Examples
See [pvlive_api_demo.py](https://github.com/SheffieldSolar/PV_Live-API/blob/master/pvlive_api_demo.py) for more example usage.
The examples below assume you have imported the PVLive class and created a local instance called `pvl`:
```Python
from datetime import datetime
import pytz
from pvlive_api import PVLive
pvl = PVLive()
```
|Example|Code|Example Output|
|-------|----|------|
|Get the latest nationally aggregated GB PV outturn|`pvl.latest()`|`(0, '2021-01-20T11:00:00Z', 203.0)`|
|Get the latest aggregated outturn for **PES** region **23** (Yorkshire)|`pvl.latest(entity_id=23)`|`(23, '2021-01-20T14:00:00Z', 5.8833031)`
|Get the latest aggregated outturn for **GSP** ID **120** (INDQ1 or "Indian Queens")|`pvl.latest(entity_type="gsp", entity_id=120)`|`(120, '2021-01-20T14:00:00Z', 1, 3.05604)`
|Get the nationally aggregated GB PV outturn for all of 2020 as a DataFrame|`pvl.between(start=datetime(2020, 1, 1, 0, 30, tzinfo=pytz.utc), end=datetime(2021, 1, 1, tzinfo=pytz.utc), dataframe=True)`|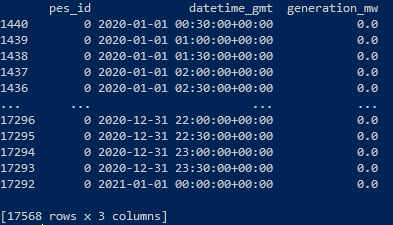|
|Get a list of GSP IDs|`pvl.gsp_ids`|`array([ 0, 1, 2, 3, ..., 315, 316, 317])`|
|Get a list of PES IDs|`pvl.pes_ids`|`array([ 0, 10, 11, 12, ..., 21, 22, 23])`|
To download data for all GSPs, use something like:
```python
def download_pvlive_by_gsp(start, end, include_national=True, extra_fields=""):
data = None
pvl = PVLive()
min_gsp_id = 0 if include_national else 1
for gsp_id in pvl.gsp_ids:
if gsp_id < min_gsp_id:
continue
data_ = pvl.between(start=start, end=end, entity_type="gsp", entity_id=gsp_id,
dataframe=True, extra_fields=extra_fields)
if data is None:
data = data_
else:
data = pd.concat((data, data_), ignore_index=True)
return data
```
## Command Line Utilities
### pv_live
This utility can be used to download data to a CSV file:
```
>> pv_live -h
usage: pv_live [-h] [-s "<yyyy-mm-dd HH:MM:SS>"] [-e "<yyyy-mm-dd HH:MM:SS>"]
[--entity_type <entity_type>] [--entity_id <entity_id>]
[--extra_fields <field1[,field2, ...]>] [--period <5|30>] [-q]
[-o </path/to/output/file>] [-http <http_proxy>] [-https <https_proxy>]
This is a command line interface (CLI) for the PV_Live API module
options:
-h, --help show this help message and exit
-s "<yyyy-mm-dd HH:MM:SS>", --start "<yyyy-mm-dd HH:MM:SS>"
Specify a UTC start date in 'yyyy-mm-dd HH:MM:SS' format
(inclusive), default behaviour is to retrieve the latest outturn.
-e "<yyyy-mm-dd HH:MM:SS>", --end "<yyyy-mm-dd HH:MM:SS>"
Specify a UTC end date in 'yyyy-mm-dd HH:MM:SS' format (inclusive),
default behaviour is to retrieve the latest outturn.
--entity_type <entity_type>
Specify an entity type, either 'gsp' or 'pes'. Default is 'gsp'.
--entity_id <entity_id>
Specify an entity ID, default is 0 (i.e. national).
--extra_fields <field1[,field2, ...]>
Specify an extra_fields (as a comma-separated list to include when
requesting data from the API, defaults to 'installedcapacity_mwp'.
--period <5|30> Desired temporal resolution (in minutes) for PV outturn estimates.
Default is 30.
-q, --quiet Specify to not print anything to stdout.
-o </path/to/output/file>, --outfile </path/to/output/file>
Specify a CSV file to write results to.
-http <http_proxy>, --http-proxy <http_proxy>
HTTP Proxy address
-https <https_proxy>, --https-proxy <https_proxy>
HTTPS Proxy address
Jamie Taylor & Ethan Jones, 2018-06-04
```
## Using the Docker Image
There is also a Docker Image hosted on Docker Hub which can be used to download data from the PV_Live API with minimal setup:
```
>> docker run -it --rm sheffieldsolar/pv_live-api:<release> pv_live -h
```
## Documentation
* [https://sheffieldsolar.github.io/PV_Live-API/](https://sheffieldsolar.github.io/PV_Live-API/)
## How do I upgrade?
Sheffield Solar will endeavour to update this library in sync with the [PV_Live API](https://www.solar.sheffield.ac.uk/pvlive/api/ "PV_Live API webpage") and ensure the latest version of this library always supports the latest version of the PV_Live API, but cannot guarantee this. To make sure you are forewarned of upcoming changes to the API, you should email [solar@sheffield.ac.uk](mailto:solar@sheffield.ac.uk?subject=PV_Live%20API%20email%20updates "Email Sheffield Solar") and request to be added to the PV_Live user mailing list.
To upgrade the code:
* Run `pip install --upgrade pvlive-api`
## Notes on PV_Live GB national update cycle
Users should be aware that Sheffield Solar computes continuous retrospective updates to PV_Live outturn estimates i.e. we regularly re-calculate outturn estimates retrospectively and these updated estimates are reflected immediately in the data delivered via the API.
As of 2023-05-02, the first estimate of the PV outturn for a given settlement period is computed ~5 minutes after the end of the half hour in question and is typically available via the API within 6 minutes
e.g. the initial estimate of the GB PV outturn for the period 14:30 - 15:00 UTC on 2023-05-02 (i.e. 15:30 - 16:00 BST) would become available at ~16:06 BST on 2023-05-02 and will be labelled using the timestamp at the end of the interval (in UTC): '2023-05-02 15:00:00'
This outturn estimate is not final though - Sheffield Solar will continue to make retrospective revisions as
- more PV sample data becomes available
- better PV deployment data becomes available
- the PV_Live methodology is refined
Since the PV_Live model produces outturn estimates, they will never strictly speaking be final, as there will always be things we can do to refine the model and improve accuracy. That said, there are some notable/routine retrospective revisions to be aware of:
- Outturns are re-computed in near-real-time every 5 minutes after the end of the half-hour for 3 hours, to allow for late arriving sample data
- If all data ingestion pipelines are running smoothly, this does not result in any retrospective revisions
- If some near-real-time sample data is late arriving, the outturn estimate will be revised at the next update
- Outturns are re-computed on day+1 (typically between 10:30 and 10:35 and again between 22:30 and 22:35) to make use of sample data which only becomes available on day+1
- Historical outturns may be re-computed periodically whenever our PV deployment dataset is updated retrospectively (usually every 3 - 6 months)
In order to maintain a local copy of the PV_Live GB national outturn estimates that is as in sync with our own latest/best estimates as possible, we recommend the following polling cycle:
- Every 5 minutes, pull the last 3 hours of outturns from the API
- Every day, around 11am, re-download the previous 3 days of outturns
- Every month, re-download all historical outturns
## Who do I talk to?
* Jamie Taylor - [jamie.taylor@sheffield.ac.uk](mailto:jamie.taylor@sheffield.ac.uk "Email Jamie") - [SheffieldSolar](https://github.com/SheffieldSolar)
## Authors
* **Jamie Taylor** - [SheffieldSolar](https://github.com/SheffieldSolar)
* **Ethan Jones** - [SheffieldSolar](https://github.com/SheffieldSolar)
## License
No license is defined yet - use at your own risk.
Raw data
{
"_id": null,
"home_page": null,
"name": "pvlive-api",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.11",
"maintainer_email": "Jamie Taylor <jamie.taylor@sheffield.ac.uk>",
"keywords": "solar, pv, pv_live, api",
"author": null,
"author_email": "Jamie Taylor <jamie.taylor@sheffield.ac.uk>",
"download_url": "https://files.pythonhosted.org/packages/56/65/f127e8aa59d1d09f5e9bc5bb987b4d3ab16038d10eb462a019ae35f4f1bc/pvlive_api-1.5.1.tar.gz",
"platform": null,
"description": "\r\n# PV_Live\r\nA Python implementation of the PV_Live web API. See https://www.solar.sheffield.ac.uk/pvlive/\r\n\r\n**Latest Version: 1.5.1**\r\n\r\n## About this repository\r\n\r\n* This Python library provides a convenient interface for the PV_Live web API to facilitate accessing PV_Live results in Python code.\r\n* Developed and tested with Python 3.10, should work with Python 3.7+. Support for Python 2.7+ has been discontinued as of 2021-01-15.\r\n\r\n## How do I get set up?\r\n\r\n* Make sure you have Git installed - [Download Git](https://git-scm.com/downloads)\r\n* Run either:\r\n * `pip install pvlive-api`\r\n * `pip install git+https://github.com/SheffieldSolar/PV_Live-API`\r\n\r\n## Usage\r\n\r\nAs of 2025-07-07, the production PV_Live API is hosted on Google Cloud Platform (GCP) at https://api.pvlive.uk. There is a also non-prod test/fix-on-fail (FOF) environment hosted on TUOS IT: https://api.solar.sheffield.ac.uk. To support switching between the two, the `pvlive-api` package exposes a parameter `domain_url`, which can be set to one of `[\"api.pvlive.uk\", \"api.solar.sheffield.ac.uk\"]` but defaults to `api.pvlive.uk`. \r\n\r\nThere are three methods for extracting raw data from the PV_Live API:\r\n\r\n|Method|Description|Docs Link|\r\n|------|-----------|---------|\r\n|`PVLive.latest(entity_type=\"pes\", entity_id=0, extra_fields=\"\", period=30, dataframe=False)`|Get the latest PV_Live generation result from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.latest)|\r\n|`PVLive.at_time(dt, entity_type=\"pes\", entity_id=0, extra_fields=\"\", period=30, dataframe=False)`|Get the PV_Live generation result for a given time from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.at_time)|\r\n|`PVLive.between(start, end, entity_type=\"pes\", entity_id=0, extra_fields=\"\", period=30, dataframe=False)`|Get the PV_Live generation result for a given time interval from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.between)|\r\n\r\nThere are two methods for extracting derived statistics:\r\n\r\n|Method|Description|Docs Link|\r\n|------|-----------|---------|\r\n|`PVLive.day_peak(d, entity_type=\"pes\", entity_id=0, extra_fields=\"\", period=30, dataframe=False)`|Get the peak PV_Live generation result for a given day from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.day_peak)|\r\n|`PVLive.day_energy(d, entity_type=\"pes\", entity_id=0)`|Get the cumulative PV generation for a given day from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.day_energy)|\r\n\r\nThese methods include the following optional parameters:\r\n\r\n|Parameter|Usage|\r\n|---------|-----|\r\n|`entity_type`|Choose between `\"pes\"` or `\"gsp\"`. If querying for national data, this parameter can be set to either value (or left to it's default value) since setting `entity_id` to `0` will always return national data.|\r\n|`entity_id`|Set `entity_id=0` (the default value) to return nationally aggregated data. If `entity_type=\"pes\"`, specify a _pes_id_ to retrieve data for, else if `entity_id=\"gsp\"`, specify a _gsp_id_. For a full list of GSP and PES IDs, refer to the lookup table hosted on National Grid ESO's data portal [here](https://data.nationalgrideso.com/system/gis-boundaries-for-gb-grid-supply-points).|\r\n|`extra_fields`|Use this to extract additional fields from the API such as _installedcapacity_mwp_. For a full list of available fields, see the [PV_Live API Docs](https://www.solar.sheffield.ac.uk/pvlive/api/).|\r\n|`period`|Set the desired temporal resolution (in minutes) for PV outturn estimates. Options are 30 (default) or 5.|\r\n|`dataframe`|Set `dataframe=True` and the results will be returned as a Pandas DataFrame object which is generally much easier to work with. The columns of the DataFrame will be _pes_id_ or _gsp_id_, _datetime_gmt_, _generation_mw_, plus any extra fields specified.|\r\n\r\nThere is also a method for extracting PV deployment (a.k.a capacity) data:\r\n|Method|Description|Docs Link|\r\n|------|-----------|---------|\r\n|`PVLive.deployment(region=\"gsp\", include_history=False, by_system_size=False, release=0)`|Download PV deployment datasets from the API.|[🔗](https://sheffieldsolar.github.io/PV_Live-API/build/html/modules.html#pvlive_api.pvlive.PVLive.deployment)|\r\n\r\n\r\n## Code Examples\r\n\r\nSee [pvlive_api_demo.py](https://github.com/SheffieldSolar/PV_Live-API/blob/master/pvlive_api_demo.py) for more example usage.\r\n\r\nThe examples below assume you have imported the PVLive class and created a local instance called `pvl`:\r\n\r\n```Python\r\nfrom datetime import datetime\r\nimport pytz\r\n\r\nfrom pvlive_api import PVLive\r\n\r\npvl = PVLive()\r\n```\r\n\r\n|Example|Code|Example Output|\r\n|-------|----|------|\r\n|Get the latest nationally aggregated GB PV outturn|`pvl.latest()`|`(0, '2021-01-20T11:00:00Z', 203.0)`|\r\n|Get the latest aggregated outturn for **PES** region **23** (Yorkshire)|`pvl.latest(entity_id=23)`|`(23, '2021-01-20T14:00:00Z', 5.8833031)`\r\n|Get the latest aggregated outturn for **GSP** ID **120** (INDQ1 or \"Indian Queens\")|`pvl.latest(entity_type=\"gsp\", entity_id=120)`|`(120, '2021-01-20T14:00:00Z', 1, 3.05604)`\r\n|Get the nationally aggregated GB PV outturn for all of 2020 as a DataFrame|`pvl.between(start=datetime(2020, 1, 1, 0, 30, tzinfo=pytz.utc), end=datetime(2021, 1, 1, tzinfo=pytz.utc), dataframe=True)`|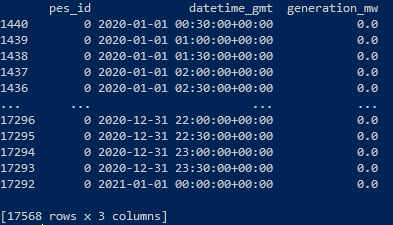|\r\n|Get a list of GSP IDs|`pvl.gsp_ids`|`array([ 0, 1, 2, 3, ..., 315, 316, 317])`|\r\n|Get a list of PES IDs|`pvl.pes_ids`|`array([ 0, 10, 11, 12, ..., 21, 22, 23])`|\r\n\r\nTo download data for all GSPs, use something like:\r\n\r\n```python\r\ndef download_pvlive_by_gsp(start, end, include_national=True, extra_fields=\"\"):\r\n data = None\r\n pvl = PVLive()\r\n min_gsp_id = 0 if include_national else 1\r\n for gsp_id in pvl.gsp_ids:\r\n if gsp_id < min_gsp_id:\r\n continue\r\n data_ = pvl.between(start=start, end=end, entity_type=\"gsp\", entity_id=gsp_id,\r\n dataframe=True, extra_fields=extra_fields)\r\n if data is None:\r\n data = data_\r\n else:\r\n data = pd.concat((data, data_), ignore_index=True)\r\n return data\r\n```\r\n\r\n## Command Line Utilities\r\n\r\n### pv_live\r\n\r\nThis utility can be used to download data to a CSV file:\r\n\r\n```\r\n>> pv_live -h\r\nusage: pv_live [-h] [-s \"<yyyy-mm-dd HH:MM:SS>\"] [-e \"<yyyy-mm-dd HH:MM:SS>\"]\r\n [--entity_type <entity_type>] [--entity_id <entity_id>]\r\n [--extra_fields <field1[,field2, ...]>] [--period <5|30>] [-q]\r\n [-o </path/to/output/file>] [-http <http_proxy>] [-https <https_proxy>]\r\n\r\nThis is a command line interface (CLI) for the PV_Live API module\r\n\r\noptions:\r\n -h, --help show this help message and exit\r\n -s \"<yyyy-mm-dd HH:MM:SS>\", --start \"<yyyy-mm-dd HH:MM:SS>\"\r\n Specify a UTC start date in 'yyyy-mm-dd HH:MM:SS' format\r\n (inclusive), default behaviour is to retrieve the latest outturn.\r\n -e \"<yyyy-mm-dd HH:MM:SS>\", --end \"<yyyy-mm-dd HH:MM:SS>\"\r\n Specify a UTC end date in 'yyyy-mm-dd HH:MM:SS' format (inclusive),\r\n default behaviour is to retrieve the latest outturn.\r\n --entity_type <entity_type>\r\n Specify an entity type, either 'gsp' or 'pes'. Default is 'gsp'.\r\n --entity_id <entity_id>\r\n Specify an entity ID, default is 0 (i.e. national).\r\n --extra_fields <field1[,field2, ...]>\r\n Specify an extra_fields (as a comma-separated list to include when\r\n requesting data from the API, defaults to 'installedcapacity_mwp'.\r\n --period <5|30> Desired temporal resolution (in minutes) for PV outturn estimates.\r\n Default is 30.\r\n -q, --quiet Specify to not print anything to stdout.\r\n -o </path/to/output/file>, --outfile </path/to/output/file>\r\n Specify a CSV file to write results to.\r\n -http <http_proxy>, --http-proxy <http_proxy>\r\n HTTP Proxy address\r\n -https <https_proxy>, --https-proxy <https_proxy>\r\n HTTPS Proxy address\r\n\r\nJamie Taylor & Ethan Jones, 2018-06-04\r\n```\r\n\r\n## Using the Docker Image\r\n\r\nThere is also a Docker Image hosted on Docker Hub which can be used to download data from the PV_Live API with minimal setup:\r\n\r\n```\r\n>> docker run -it --rm sheffieldsolar/pv_live-api:<release> pv_live -h\r\n```\r\n\r\n## Documentation\r\n\r\n* [https://sheffieldsolar.github.io/PV_Live-API/](https://sheffieldsolar.github.io/PV_Live-API/)\r\n\r\n## How do I upgrade?\r\n\r\nSheffield Solar will endeavour to update this library in sync with the [PV_Live API](https://www.solar.sheffield.ac.uk/pvlive/api/ \"PV_Live API webpage\") and ensure the latest version of this library always supports the latest version of the PV_Live API, but cannot guarantee this. To make sure you are forewarned of upcoming changes to the API, you should email [solar@sheffield.ac.uk](mailto:solar@sheffield.ac.uk?subject=PV_Live%20API%20email%20updates \"Email Sheffield Solar\") and request to be added to the PV_Live user mailing list.\r\n\r\nTo upgrade the code:\r\n* Run `pip install --upgrade pvlive-api`\r\n\r\n## Notes on PV_Live GB national update cycle\r\n\r\nUsers should be aware that Sheffield Solar computes continuous retrospective updates to PV_Live outturn estimates i.e. we regularly re-calculate outturn estimates retrospectively and these updated estimates are reflected immediately in the data delivered via the API.\r\n\r\nAs of 2023-05-02, the first estimate of the PV outturn for a given settlement period is computed ~5 minutes after the end of the half hour in question and is typically available via the API within 6 minutes\r\n\r\ne.g. the initial estimate of the GB PV outturn for the period 14:30 - 15:00 UTC on 2023-05-02 (i.e. 15:30 - 16:00 BST) would become available at ~16:06 BST on 2023-05-02 and will be labelled using the timestamp at the end of the interval (in UTC): '2023-05-02 15:00:00'\r\n\r\nThis outturn estimate is not final though - Sheffield Solar will continue to make retrospective revisions as\r\n\r\n- more PV sample data becomes available\r\n- better PV deployment data becomes available\r\n- the PV_Live methodology is refined\r\n\r\nSince the PV_Live model produces outturn estimates, they will never strictly speaking be final, as there will always be things we can do to refine the model and improve accuracy. That said, there are some notable/routine retrospective revisions to be aware of:\r\n\r\n- Outturns are re-computed in near-real-time every 5 minutes after the end of the half-hour for 3 hours, to allow for late arriving sample data\r\n - If all data ingestion pipelines are running smoothly, this does not result in any retrospective revisions\r\n - If some near-real-time sample data is late arriving, the outturn estimate will be revised at the next update\r\n- Outturns are re-computed on day+1 (typically between 10:30 and 10:35 and again between 22:30 and 22:35) to make use of sample data which only becomes available on day+1\r\n- Historical outturns may be re-computed periodically whenever our PV deployment dataset is updated retrospectively (usually every 3 - 6 months)\r\n\r\nIn order to maintain a local copy of the PV_Live GB national outturn estimates that is as in sync with our own latest/best estimates as possible, we recommend the following polling cycle:\r\n\r\n- Every 5 minutes, pull the last 3 hours of outturns from the API\r\n- Every day, around 11am, re-download the previous 3 days of outturns\r\n- Every month, re-download all historical outturns\r\n\r\n## Who do I talk to?\r\n\r\n* Jamie Taylor - [jamie.taylor@sheffield.ac.uk](mailto:jamie.taylor@sheffield.ac.uk \"Email Jamie\") - [SheffieldSolar](https://github.com/SheffieldSolar)\r\n\r\n## Authors\r\n\r\n* **Jamie Taylor** - [SheffieldSolar](https://github.com/SheffieldSolar)\r\n* **Ethan Jones** - [SheffieldSolar](https://github.com/SheffieldSolar)\r\n\r\n## License\r\n\r\nNo license is defined yet - use at your own risk.\r\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python interface for the PV_Live web API from Sheffield Solar.",
"version": "1.5.1",
"project_urls": {
"Bug Tracker": "https://github.com/SheffieldSolar/PV_Live-API/issues",
"Documentation": "https://sheffieldsolar.github.io/PV_Live-API/build/html/index.html",
"Homepage": "https://www.solar.sheffield.ac.uk/pvlive/",
"Repository": "https://github.com/SheffieldSolar/PV_Live-API"
},
"split_keywords": [
"solar",
" pv",
" pv_live",
" api"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "ea56d4fc55b8a46a0b49e70efc5a4aacbe7bbbd59f294dff8028d534da0317ce",
"md5": "8f62779d2b8085fb4d7cd39e47bbf3bc",
"sha256": "c03bca97366b794c4f3e286d8e0a411425395e7b15a3510a0b4441d21665afb3"
},
"downloads": -1,
"filename": "pvlive_api-1.5.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8f62779d2b8085fb4d7cd39e47bbf3bc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11",
"size": 15188,
"upload_time": "2025-07-18T09:46:16",
"upload_time_iso_8601": "2025-07-18T09:46:16.468382Z",
"url": "https://files.pythonhosted.org/packages/ea/56/d4fc55b8a46a0b49e70efc5a4aacbe7bbbd59f294dff8028d534da0317ce/pvlive_api-1.5.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "5665f127e8aa59d1d09f5e9bc5bb987b4d3ab16038d10eb462a019ae35f4f1bc",
"md5": "fb48bb2371ff4ddb5c519e6107ff0583",
"sha256": "84e60af715c35dca965e96252ded879f52087fdc4a82a5a2f83464f82fb1bbba"
},
"downloads": -1,
"filename": "pvlive_api-1.5.1.tar.gz",
"has_sig": false,
"md5_digest": "fb48bb2371ff4ddb5c519e6107ff0583",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11",
"size": 20298,
"upload_time": "2025-07-18T09:46:17",
"upload_time_iso_8601": "2025-07-18T09:46:17.670053Z",
"url": "https://files.pythonhosted.org/packages/56/65/f127e8aa59d1d09f5e9bc5bb987b4d3ab16038d10eb462a019ae35f4f1bc/pvlive_api-1.5.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-07-18 09:46:17",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "SheffieldSolar",
"github_project": "PV_Live-API",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [
{
"name": "pytz",
"specs": []
},
{
"name": "requests",
"specs": []
},
{
"name": "numpy",
"specs": []
},
{
"name": "pandas",
"specs": []
},
{
"name": "bs4",
"specs": []
}
],
"lcname": "pvlive-api"
}