# py-gis-utility
A GIS utility library which contains some regularly required math and image operations.
[](https://pepy.tech/project/py-gis-utility)
## Installation
pip install py-gis-utility
## Requirements
- *_Geopandas - [installation](https://anaconda.org/conda-forge/geopandas)_*
- *_Rasterio - [installation](https://anaconda.org/conda-forge/rasterio)_*
- *_GDAL 2.4.4 - [installation](https://anaconda.org/conda-forge/gdal)_*
- *_Fiona - [installation](https://anaconda.org/conda-forge/fiona)_*
- *_Shapely - [installation](https://anaconda.org/conda-forge/shapely)_*
## Math Operations
1. Get perpendicular point with reference to start and end point of the segment
2. Get perpendicular distance from point to line_segment
3. Given a Point find a new point at an given 'angle' with given 'distance'
4. Calculate a new point on the line segment given the distance from the start
5. Euclidean computation
## Image Operations
### Save Multi Band Imagery
```python
import numpy as np
from affine import Affine
from py_gis_utility.image_func import save_16bit_multi_band, save_8bit_multi_band
image = np.zeros((512, 512, 6))
transform = Affine(1.0, 0.0, 3422098.682455578,
0.0, -1.0, 5289611.291479621)
# Save 8bit
save_8bit_multi_band(image, transform, 26910, r"8bit.tiff")
# Save 16bit
save_16bit_multi_band(image, transform, 26910, r"16bit.tiff")
```
### Generate bitmap from shape file
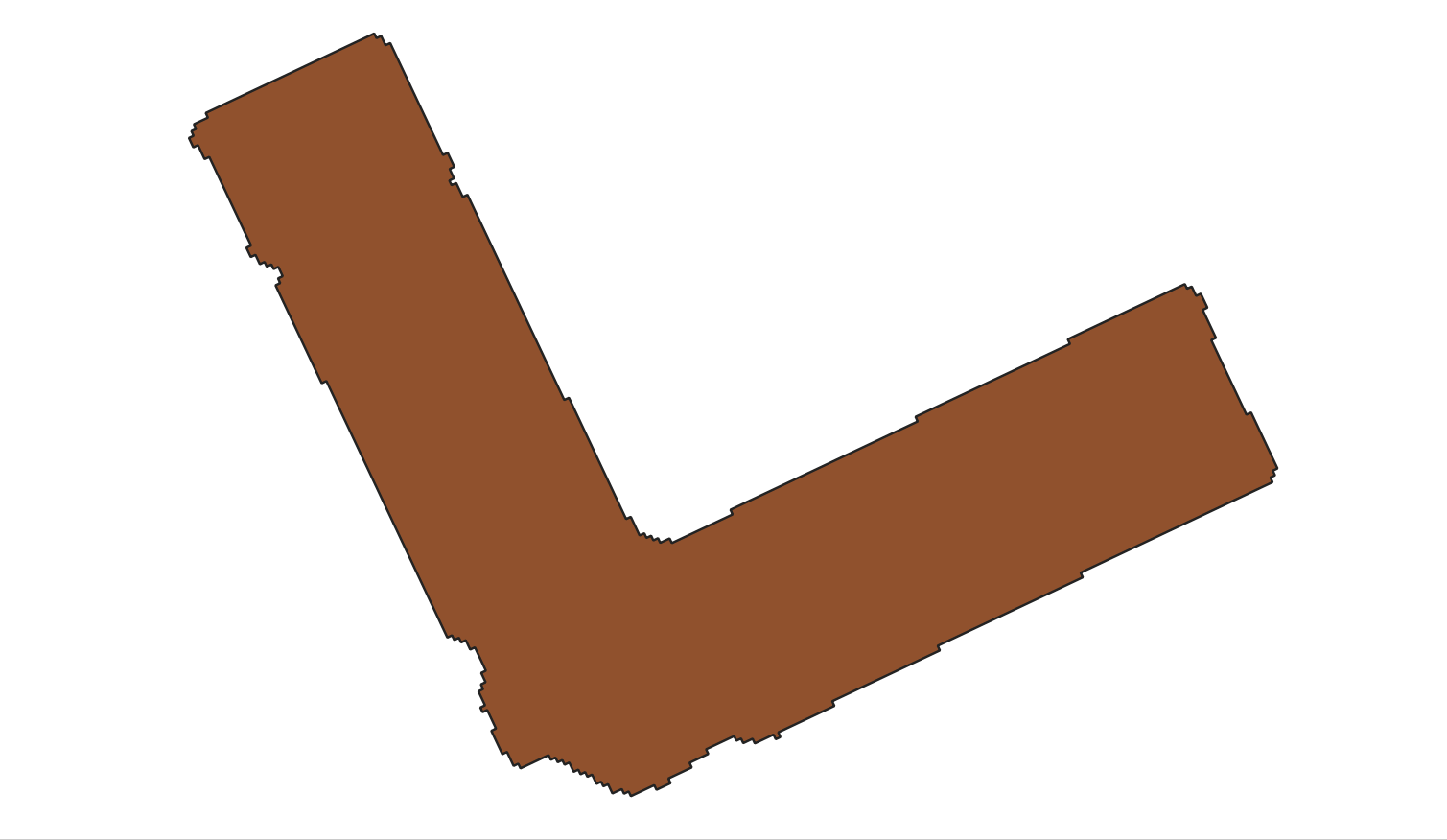
```python
from py_gis_utility.helper import (
read_data_frame,
save_image_with_geo_transform,
)
from py_gis_utility.image_func import shape_geometry_to_bitmap_from_data_frame_generator
data_frame = read_data_frame(r"path_to_geometry_file")
bitmap_gen = shape_geometry_to_bitmap_from_data_frame_generator(data_frame, (50, 50), (1, 1),
allow_output_to_overlap=True)
for i, bitmap in enumerate(bitmap_gen):
save_image_with_geo_transform(f"{i}.tiff", bitmap.array, bitmap.transform)
```
### Generate shape geometry from geo reference bitmap
```python
from py_gis_utility.helper import (read_image_with_geo_transform,
)
from py_gis_utility.image_func import image_obj_to_coordinates_generator, image_obj_to_shape_generator
img_obj = read_image_with_geo_transform(r"path_to_geo_referenced_file")
# output in format {'geometry': <shapely.geometry.polygon.Polygon object at 0x0000022009E5EC08>, 'properties': {'id': 255.0, 'crs': CRS.from_epsg(4326)}}
shape_gen = image_obj_to_shape_generator(img_obj)
for g in shape_gen:
print(g)
# output in format {'geometry': {'type': 'Polygon', 'coordinates': [[(621000.0, 3349500.0), .... ,(621000.0, 3349489.5)]]}, 'properties': {'id': 255.0, 'crs': CRS.from_epsg(4326)}}
co_ord_gen = image_obj_to_coordinates_generator(img_obj)
for g in co_ord_gen:
print(g)
```
## Support Me
<a href='https://ko-fi.com/fuzailpalnak' target='_blank'><img height='36' style='border:0px;height:36px;' src='https://az743702.vo.msecnd.net/cdn/kofi1.png?v=0' border='0' alt='Buy Me a Coffee at ko-fi.com' /></a>
Raw data
{
"_id": null,
"home_page": "https://github.com/fuzailpalnak/py-gis-utility",
"name": "py-gis-utility",
"maintainer": "",
"docs_url": null,
"requires_python": "~=3.3",
"maintainer_email": "",
"keywords": "GIS,Image",
"author": "Fuzail Palnak",
"author_email": "fuzailpalnak@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/14/cf/15ef3369b2bc7e9253d60aca856e5bb7f69076d826c1741c9633a6c5b0cd/py-gis-utility-0.2.2.tar.gz",
"platform": null,
"description": "# py-gis-utility\n\nA GIS utility library which contains some regularly required math and image operations.\n\n[](https://pepy.tech/project/py-gis-utility)\n\n## Installation\n \n pip install py-gis-utility\n \n \n## Requirements\n\n- *_Geopandas - [installation](https://anaconda.org/conda-forge/geopandas)_*\n- *_Rasterio - [installation](https://anaconda.org/conda-forge/rasterio)_*\n- *_GDAL 2.4.4 - [installation](https://anaconda.org/conda-forge/gdal)_*\n- *_Fiona - [installation](https://anaconda.org/conda-forge/fiona)_*\n- *_Shapely - [installation](https://anaconda.org/conda-forge/shapely)_*\n\n## Math Operations\n \n1. Get perpendicular point with reference to start and end point of the segment \n2. Get perpendicular distance from point to line_segment\n3. Given a Point find a new point at an given 'angle' with given 'distance'\n4. Calculate a new point on the line segment given the distance from the start\n5. Euclidean computation\n\n## Image Operations\n### Save Multi Band Imagery\n```python\nimport numpy as np\nfrom affine import Affine\nfrom py_gis_utility.image_func import save_16bit_multi_band, save_8bit_multi_band\n\nimage = np.zeros((512, 512, 6))\ntransform = Affine(1.0, 0.0, 3422098.682455578,\n 0.0, -1.0, 5289611.291479621)\n\n# Save 8bit\nsave_8bit_multi_band(image, transform, 26910, r\"8bit.tiff\")\n\n# Save 16bit\nsave_16bit_multi_band(image, transform, 26910, r\"16bit.tiff\")\n\n```\n\n### Generate bitmap from shape file\n\n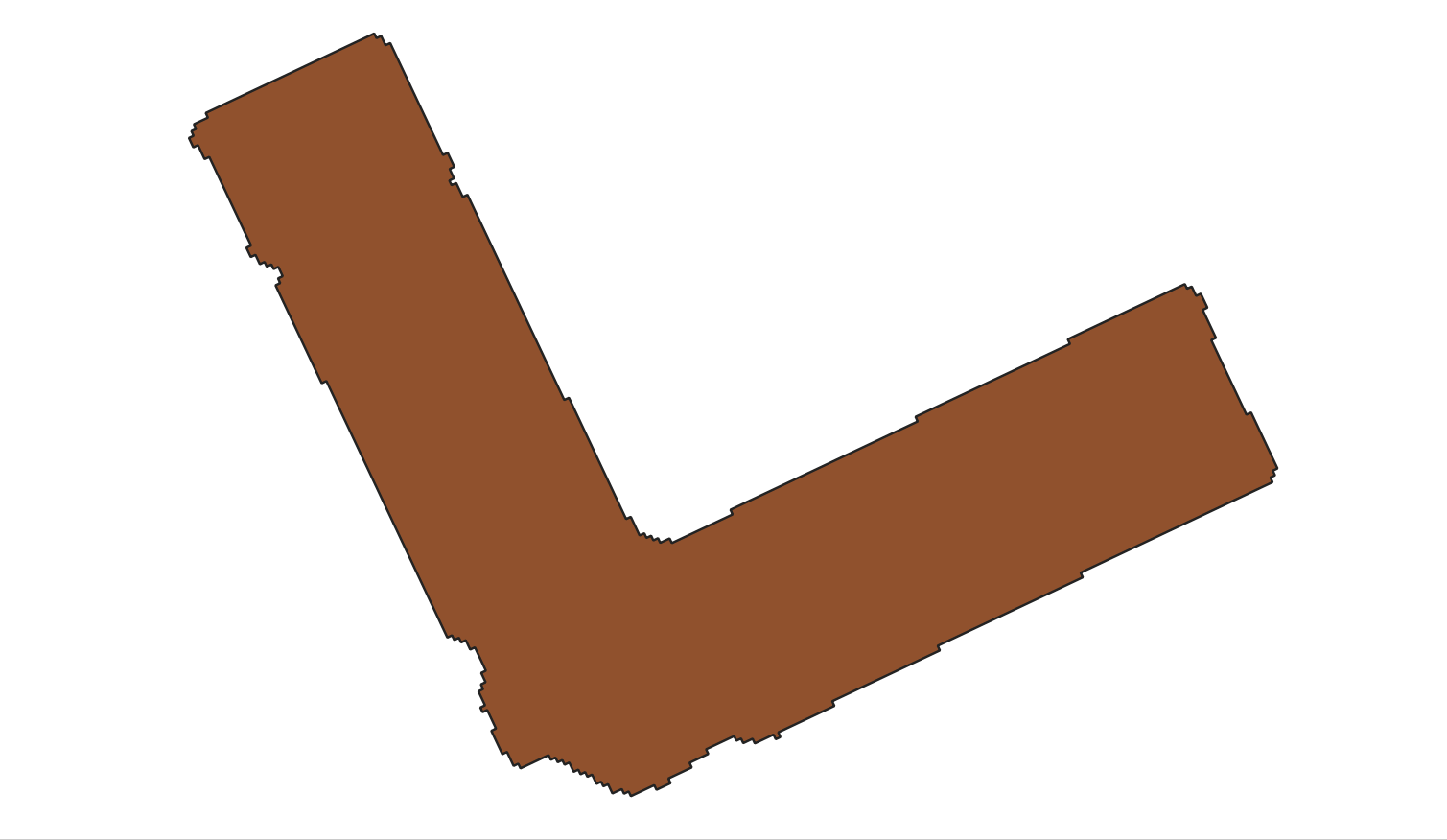\n\n```python\nfrom py_gis_utility.helper import (\n read_data_frame,\n save_image_with_geo_transform,\n)\nfrom py_gis_utility.image_func import shape_geometry_to_bitmap_from_data_frame_generator\n\ndata_frame = read_data_frame(r\"path_to_geometry_file\")\nbitmap_gen = shape_geometry_to_bitmap_from_data_frame_generator(data_frame, (50, 50), (1, 1),\n allow_output_to_overlap=True)\n\nfor i, bitmap in enumerate(bitmap_gen):\n save_image_with_geo_transform(f\"{i}.tiff\", bitmap.array, bitmap.transform)\n```\n\n### Generate shape geometry from geo reference bitmap\n\n```python\n\nfrom py_gis_utility.helper import (read_image_with_geo_transform,\n)\nfrom py_gis_utility.image_func import image_obj_to_coordinates_generator, image_obj_to_shape_generator\n\n\nimg_obj = read_image_with_geo_transform(r\"path_to_geo_referenced_file\")\n\n# output in format {'geometry': <shapely.geometry.polygon.Polygon object at 0x0000022009E5EC08>, 'properties': {'id': 255.0, 'crs': CRS.from_epsg(4326)}}\nshape_gen = image_obj_to_shape_generator(img_obj)\nfor g in shape_gen:\n print(g)\n\n# output in format {'geometry': {'type': 'Polygon', 'coordinates': [[(621000.0, 3349500.0), .... ,(621000.0, 3349489.5)]]}, 'properties': {'id': 255.0, 'crs': CRS.from_epsg(4326)}}\nco_ord_gen = image_obj_to_coordinates_generator(img_obj)\nfor g in co_ord_gen:\n print(g)\n```\n\n## Support Me\n\n<a href='https://ko-fi.com/fuzailpalnak' target='_blank'><img height='36' style='border:0px;height:36px;' src='https://az743702.vo.msecnd.net/cdn/kofi1.png?v=0' border='0' alt='Buy Me a Coffee at ko-fi.com' /></a>\n\n\n\n \n",
"bugtrack_url": null,
"license": "",
"summary": "Utility for image and math related operation in GIS",
"version": "0.2.2",
"project_urls": {
"Homepage": "https://github.com/fuzailpalnak/py-gis-utility"
},
"split_keywords": [
"gis",
"image"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "06778b83f62ee38e90b9f292b5c553d30c8c2cc851e63a90455a303d061d3a58",
"md5": "acd877ac2827c16b5261a449c4cb1977",
"sha256": "e20097e20af2d2faf0f58769b945f1b4e05fd83451dc21abea8deecaeec43366"
},
"downloads": -1,
"filename": "py_gis_utility-0.2.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "acd877ac2827c16b5261a449c4cb1977",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "~=3.3",
"size": 13020,
"upload_time": "2023-05-31T10:39:27",
"upload_time_iso_8601": "2023-05-31T10:39:27.099679Z",
"url": "https://files.pythonhosted.org/packages/06/77/8b83f62ee38e90b9f292b5c553d30c8c2cc851e63a90455a303d061d3a58/py_gis_utility-0.2.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "14cf15ef3369b2bc7e9253d60aca856e5bb7f69076d826c1741c9633a6c5b0cd",
"md5": "5f5cc6c8d08675dd306562feafe133ac",
"sha256": "d68d5e83cf6910c8af20a5a441299414ba529573cdef26cef17d3c2d3c3ba0c6"
},
"downloads": -1,
"filename": "py-gis-utility-0.2.2.tar.gz",
"has_sig": false,
"md5_digest": "5f5cc6c8d08675dd306562feafe133ac",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "~=3.3",
"size": 12122,
"upload_time": "2023-05-31T10:39:28",
"upload_time_iso_8601": "2023-05-31T10:39:28.627418Z",
"url": "https://files.pythonhosted.org/packages/14/cf/15ef3369b2bc7e9253d60aca856e5bb7f69076d826c1741c9633a6c5b0cd/py-gis-utility-0.2.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-31 10:39:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "fuzailpalnak",
"github_project": "py-gis-utility",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "py-gis-utility"
}