# 🌳 iTree - an Interval Tree library
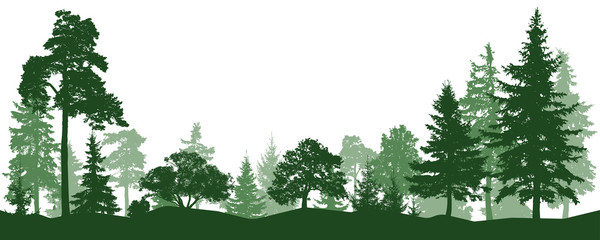
In computer science, an `interval tree` is a tree data structure to hold intervals. Every node in `itree` has a `start` and an `end` value.
## Installation
`itree` supports Linux, MacOS and Windows operating systems.
### Installation With `pip`
You can install ``itree`` by running:
```bash
pip install py-itree
```
### Build From Source With `cmake`
🍀 For MacOS with M1 Chip, some Windows OS, embedded systems, or different python versions where the method above doesn't work, please use the following workaround.
- Install [cmake](https://cmake.org/) (version>3.4)
- Run the following command to build it locally:
```bash
pip install https://github.com/juncongmoo/itree/archive/refs/tags/v0.0.18.tar.gz
```
## Quick Start
- Symbol For Node And Tree
```
🟢 - normal node;
🔵 - zero interval node;
🍁 - leaf node, which means no child node
🌳 - a tree
```
- Create Some Nodes
```python
>>> import itree
>>> itree.Node('fruit', 0, 40)
[🍁 n=fruit,s=0.00,e=40.00,x=0,c=0]
>>> itree.Node('fruit')
[🔵 n=fruit]
>>> a=itree.Node('fruit', 0, 200)
>>> b=itree.Node('apple', 10, 20)
>>> print(a)
[🍁 n=fruit,s=0.00,e=40.00,x=0,c=0]
>>> a.append(b)
>>> print(a)
[🟢 n=fruit,s=0.00,e=200.00,x=0,c=1]
>>> print(b)
[🍁 n=apple,s=10.00,e=20.00,x=0,c=0]
>>> print(a.nodes)
[[🍁 n=apple,s=10.00,e=20.00,x=0,c=0]]
>>>
```
- Create And Render A Tree
```python
>>> from itree import Tree
>>> def demo_tree():
t = Tree(tid="123", extra={"img": "1241241313.png"})
t.start("root", 1, {"name": "itree"})
t.start("math", 2, {"age": 10})
t.start("music", 3, {"location": [1, 2, 3]})
t.end("music", 4, {"price": 12.3})
t.end("math", 16284000, {"memory": (1, 2, 3)})
t.start("music", 122840057.8713503)
t.end("music", 1228400500)
t.start("music", 32840057.8713503)
t.start("egg", 3284.8713503)
t.start("icecream", 32843.8713503)
t.start("pizza", 32843.8713503)
t.end("pizza", 62845003)
t.end("icecream", 62845003)
t.end("egg", 6284500)
t.end("music", 628400500)
t.start("piggy", 3284.8713503)
t.start("unicorn", 32843.8713503)
t.start("monkey", 32843.8713503)
t.end("monkey", 62845003)
t.end("unicorn", 62845003)
t.end("piggy", 6284500)
t.end("root", 1628400570.8713503)
print(f"{t.count},{t.depth}")
t.consolidate()
img_path = t.to_img()
return img_path, t
>>> img_path, t = demo_tree()
>>> t
(🌳 id=123,c=11,x=1,d=6,m=0,o=1)
```
Run the `demo_tree()` function, a tree digraph will be generated:
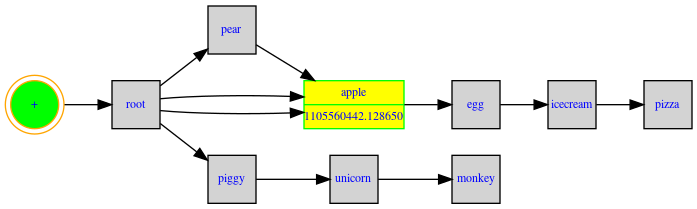
The green circle node is a virtual node. The yellow record box is the node with the longest interval.
A virtual node is a conceptual node which could have many subnodes/subtrees.
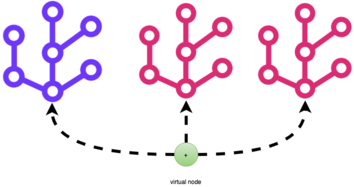
## Development
Turn on local build to `ON` in `itree/CMakeLists.txt`:
```
option(LOCAL_BUILD "build locally" OFF)
```
- Test
```bash
$python -m unittest discover
...............
----------------------------------------------------------------------
Ran 15 tests in 1.209s
OK
```
- Format
```bash
find itree -path itree/pybind11 -prune -o -iname *.h -o -iname *.cpp | xargs clang-format -i
black -S . --exclude '(\.history|\.vscode|\.git|\.VSCodeCounter|venv|workspace|pybind11)'
```
- Build
```bash
rm -fr itree/build/ && ./release.sh && yes | cp itree/build/_itree.* itree/
pip install --editable .
```
## License
Tree is licensed under the Apache 2.0 License.
Raw data
{
"_id": null,
"home_page": "https://github.com/juncongmoo/itree",
"name": "py-itree",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "tree, itree, interval tree",
"author": "Juncong Moo",
"author_email": "<juncongmoo@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/a2/81/df4ca915d83cbaccea7d68e3e137bb46d5fd71c7df682c5817ef41c10d88/py_itree-0.0.21.tar.gz",
"platform": null,
"description": "# \ud83c\udf33 iTree - an Interval Tree library\n\n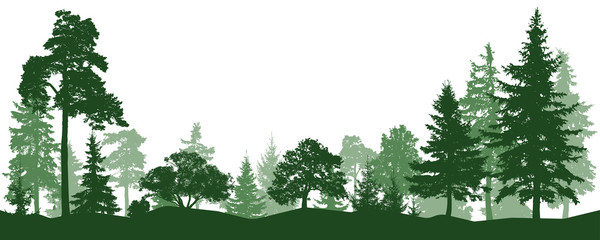\n\nIn computer science, an `interval tree` is a tree data structure to hold intervals. Every node in `itree` has a `start` and an `end` value.\n\n## Installation\n\n`itree` supports Linux, MacOS and Windows operating systems.\n\n### Installation With `pip`\n\nYou can install ``itree`` by running:\n\n```bash\n pip install py-itree\n```\n\n### Build From Source With `cmake`\n\n\ud83c\udf40 For MacOS with M1 Chip, some Windows OS, embedded systems, or different python versions where the method above doesn't work, please use the following workaround.\n\n- Install [cmake](https://cmake.org/) (version>3.4)\n- Run the following command to build it locally:\n```bash\npip install https://github.com/juncongmoo/itree/archive/refs/tags/v0.0.18.tar.gz\n``` \n\n\n## Quick Start\n\n- Symbol For Node And Tree\n\n```\n \ud83d\udfe2 - normal node; \n \ud83d\udd35 - zero interval node; \n \ud83c\udf41 - leaf node, which means no child node\n \ud83c\udf33 - a tree\n```\n\n- Create Some Nodes\n\n```python\n >>> import itree\n >>> itree.Node('fruit', 0, 40)\n [\ud83c\udf41 n=fruit,s=0.00,e=40.00,x=0,c=0]\n >>> itree.Node('fruit')\n [\ud83d\udd35 n=fruit]\n >>> a=itree.Node('fruit', 0, 200)\n >>> b=itree.Node('apple', 10, 20)\n >>> print(a)\n [\ud83c\udf41 n=fruit,s=0.00,e=40.00,x=0,c=0]\n >>> a.append(b)\n >>> print(a)\n [\ud83d\udfe2 n=fruit,s=0.00,e=200.00,x=0,c=1]\n >>> print(b)\n [\ud83c\udf41 n=apple,s=10.00,e=20.00,x=0,c=0]\n >>> print(a.nodes)\n [[\ud83c\udf41 n=apple,s=10.00,e=20.00,x=0,c=0]]\n >>> \n```\n\n- Create And Render A Tree\n\n\n```python\n >>> from itree import Tree\n >>> def demo_tree():\n t = Tree(tid=\"123\", extra={\"img\": \"1241241313.png\"})\n t.start(\"root\", 1, {\"name\": \"itree\"})\n t.start(\"math\", 2, {\"age\": 10})\n t.start(\"music\", 3, {\"location\": [1, 2, 3]})\n t.end(\"music\", 4, {\"price\": 12.3})\n t.end(\"math\", 16284000, {\"memory\": (1, 2, 3)})\n t.start(\"music\", 122840057.8713503)\n t.end(\"music\", 1228400500)\n t.start(\"music\", 32840057.8713503)\n t.start(\"egg\", 3284.8713503)\n t.start(\"icecream\", 32843.8713503)\n t.start(\"pizza\", 32843.8713503)\n t.end(\"pizza\", 62845003)\n t.end(\"icecream\", 62845003)\n t.end(\"egg\", 6284500)\n t.end(\"music\", 628400500)\n t.start(\"piggy\", 3284.8713503)\n t.start(\"unicorn\", 32843.8713503)\n t.start(\"monkey\", 32843.8713503)\n t.end(\"monkey\", 62845003)\n t.end(\"unicorn\", 62845003)\n t.end(\"piggy\", 6284500)\n t.end(\"root\", 1628400570.8713503)\n print(f\"{t.count},{t.depth}\")\n t.consolidate()\n img_path = t.to_img()\n return img_path, t\n >>> img_path, t = demo_tree()\n >>> t\n (\ud83c\udf33 id=123,c=11,x=1,d=6,m=0,o=1)\n```\n\nRun the `demo_tree()` function, a tree digraph will be generated:\n\n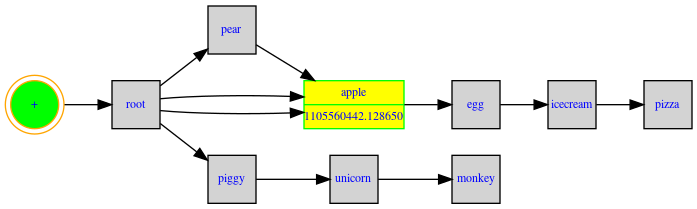\n\nThe green circle node is a virtual node. The yellow record box is the node with the longest interval.\n\nA virtual node is a conceptual node which could have many subnodes/subtrees.\n\n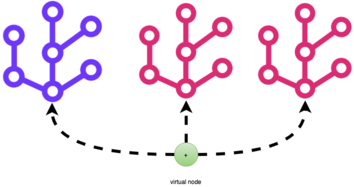\n\n## Development\n\nTurn on local build to `ON` in `itree/CMakeLists.txt`:\n\n```\noption(LOCAL_BUILD \"build locally\" OFF)\n```\n\n- Test\n\n```bash\n$python -m unittest discover\n...............\n----------------------------------------------------------------------\nRan 15 tests in 1.209s\n\nOK\n```\n\n- Format\n\n```bash\nfind itree -path itree/pybind11 -prune -o -iname *.h -o -iname *.cpp | xargs clang-format -i\nblack -S . --exclude '(\\.history|\\.vscode|\\.git|\\.VSCodeCounter|venv|workspace|pybind11)'\n```\n\n- Build\n\n```bash\nrm -fr itree/build/ && ./release.sh && yes | cp itree/build/_itree.* itree/\npip install --editable .\n```\n\n## License\n\nTree is licensed under the Apache 2.0 License.\n",
"bugtrack_url": null,
"license": "Apache 2.0",
"summary": "A Interval Tree Library",
"version": "0.0.21",
"project_urls": {
"Homepage": "https://github.com/juncongmoo/itree"
},
"split_keywords": [
"tree",
" itree",
" interval tree"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a281df4ca915d83cbaccea7d68e3e137bb46d5fd71c7df682c5817ef41c10d88",
"md5": "8a8f2fc69c0e22feb1f1fdc470fb4806",
"sha256": "7a00e1ba221b72f9f239eb336dbc7422e957fb8a8d026fc07e2d99b52327cf01"
},
"downloads": -1,
"filename": "py_itree-0.0.21.tar.gz",
"has_sig": false,
"md5_digest": "8a8f2fc69c0e22feb1f1fdc470fb4806",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 485969,
"upload_time": "2024-08-24T18:10:28",
"upload_time_iso_8601": "2024-08-24T18:10:28.385045Z",
"url": "https://files.pythonhosted.org/packages/a2/81/df4ca915d83cbaccea7d68e3e137bb46d5fd71c7df682c5817ef41c10d88/py_itree-0.0.21.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-24 18:10:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "juncongmoo",
"github_project": "itree",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "py-itree"
}