# py-ulid - A ULID Implementation in Python
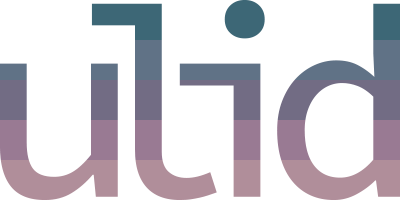
[](https://py-ulid.readthedocs.io/en/latest/?badge=latest)
The py-ulid library is a minimal and self-contained implementation of the ULID (Universally Unique Lexicographically Sortable Identifier) specification in Python.
For more information, please refer to the [official specification](https://github.com/ulid/spec).
UUID can be suboptimal for many uses-cases because:
- It isn't the most character efficient way of encoding 128 bits of randomness
- UUID v1/v2 is impractical in many environments, as it requires access to a unique, stable MAC address
- UUID v3/v5 requires a unique seed and produces randomly distributed IDs, which can cause fragmentation in many data structures
- UUID v4 provides no other information than randomness which can cause fragmentation in many data structures
Instead, herein is proposed ULID:
- 128-bit compatibility with UUID
- 1.21e+24 unique ULIDs per millisecond
- Lexicographically sortable!
- Canonically encoded as a 26 character string, as opposed to the 36 character UUID
- Uses Crockford's base32 for better efficiency and readability (5 bits per character)
- Case insensitive
- No special characters (URL safe)
- Monotonic sort order (correctly detects and handles the same millisecond)
## Installation
You can install the py-ulid library from [PyPi](https://pypi.org/project/py-ulid)
```shell
pip install py-ulid
```
The py-ulid library can be used in any version of python >= 3.5 and does not require any additional packages or modules.
## How to use
To generate a ULID, simple run the generate() function
```python
from ulid import ULID
#Instantiate the ULID class
ulid = ULID()
ulid.generate() #01BX5ZZKBKACTAV9WEVGEMMVRZ
```
### Seeding Time
You can instantiate the instance of the ULID class with a seed time which will output the same string for the time component. This could be useful when migrating to ulid
```python
from ulid import ULID
#Instantiate the ULID class
ulid = ULID(1469918176385)
ulid.generate() #01ARYZ6S41TSV4RRFFQ69G5FAV
```
### Monotonic ULIDs
```python
from ulid import Monotonic
#Instantiate the Monotonic Class
ulid = Monotonic()
# Same timestamp when calls are made within the same
# millisecond and least-significant random bit is incremented by 1
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR11
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR12
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR13
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR14
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR15
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR16
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR17
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR18
ulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR19
```
## Prior Art
Partly inspired by:
- [Instagram](http://instagram-engineering.tumblr.com/post/10853187575/sharding-ids-at-instagram)
- [Firebase](https://firebase.googleblog.com/2015/02/the-2120-ways-to-ensure-unique_68.html)
Raw data
{
"_id": null,
"home_page": "https://github.com/tsmanikandan/py-ulid",
"name": "py-ulid",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "",
"author": "Manikandan Sundararajan",
"author_email": "me@tsmanikandan.com",
"download_url": "https://files.pythonhosted.org/packages/0d/11/995c21b3fad440b6ca07c0ebca8aba7abe25e66c5027c02dae6cf1883068/py-ulid-1.0.3.tar.gz",
"platform": "",
"description": "# py-ulid - A ULID Implementation in Python\n\n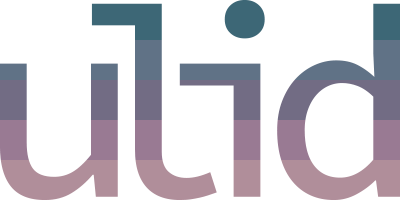\n\n[](https://py-ulid.readthedocs.io/en/latest/?badge=latest)\n\nThe py-ulid library is a minimal and self-contained implementation of the ULID (Universally Unique Lexicographically Sortable Identifier) specification in Python.\nFor more information, please refer to the [official specification](https://github.com/ulid/spec).\n\nUUID can be suboptimal for many uses-cases because:\n\n- It isn't the most character efficient way of encoding 128 bits of randomness\n- UUID v1/v2 is impractical in many environments, as it requires access to a unique, stable MAC address\n- UUID v3/v5 requires a unique seed and produces randomly distributed IDs, which can cause fragmentation in many data structures\n- UUID v4 provides no other information than randomness which can cause fragmentation in many data structures\n\nInstead, herein is proposed ULID:\n\n- 128-bit compatibility with UUID\n- 1.21e+24 unique ULIDs per millisecond\n- Lexicographically sortable!\n- Canonically encoded as a 26 character string, as opposed to the 36 character UUID\n- Uses Crockford's base32 for better efficiency and readability (5 bits per character)\n- Case insensitive\n- No special characters (URL safe)\n- Monotonic sort order (correctly detects and handles the same millisecond)\n\n## Installation\n\nYou can install the py-ulid library from [PyPi](https://pypi.org/project/py-ulid)\n\n```shell\npip install py-ulid\n```\n\nThe py-ulid library can be used in any version of python >= 3.5 and does not require any additional packages or modules.\n\n## How to use\n\nTo generate a ULID, simple run the generate() function\n\n```python\nfrom ulid import ULID\n\n#Instantiate the ULID class\nulid = ULID()\nulid.generate() #01BX5ZZKBKACTAV9WEVGEMMVRZ\n```\n\n### Seeding Time\n\nYou can instantiate the instance of the ULID class with a seed time which will output the same string for the time component. This could be useful when migrating to ulid\n\n```python\nfrom ulid import ULID\n\n#Instantiate the ULID class\nulid = ULID(1469918176385)\nulid.generate() #01ARYZ6S41TSV4RRFFQ69G5FAV\n```\n\n### Monotonic ULIDs\n\n```python\nfrom ulid import Monotonic\n\n#Instantiate the Monotonic Class\nulid = Monotonic()\n\n# Same timestamp when calls are made within the same\n# millisecond and least-significant random bit is incremented by 1\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR11\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR12\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR13\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR14\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR15\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR16\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR17\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR18\nulid.generate() #01DC8Y7RBV4RSXX0437Z1RQR19\n```\n\n## Prior Art\n\nPartly inspired by:\n\n- [Instagram](http://instagram-engineering.tumblr.com/post/10853187575/sharding-ids-at-instagram)\n- [Firebase](https://firebase.googleblog.com/2015/02/the-2120-ways-to-ensure-unique_68.html)",
"bugtrack_url": null,
"license": "MIT",
"summary": "Python library that provides an implementation of the ULID Specification",
"version": "1.0.3",
"project_urls": {
"Homepage": "https://github.com/tsmanikandan/py-ulid"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "0d11995c21b3fad440b6ca07c0ebca8aba7abe25e66c5027c02dae6cf1883068",
"md5": "95b885e5a7b9dd9a713496f34f802d93",
"sha256": "e30fcd14386fb6568efe6345b4656cb79bf5b9f54b83822d2e36ca728fe0a661"
},
"downloads": -1,
"filename": "py-ulid-1.0.3.tar.gz",
"has_sig": false,
"md5_digest": "95b885e5a7b9dd9a713496f34f802d93",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 5410,
"upload_time": "2019-07-14T22:05:43",
"upload_time_iso_8601": "2019-07-14T22:05:43.641888Z",
"url": "https://files.pythonhosted.org/packages/0d/11/995c21b3fad440b6ca07c0ebca8aba7abe25e66c5027c02dae6cf1883068/py-ulid-1.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2019-07-14 22:05:43",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tsmanikandan",
"github_project": "py-ulid",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "py-ulid"
}