[](https://github.com/clugen/pyclugen/actions/workflows/tests.yml)
[](https://codecov.io/gh/clugen/pyclugen)
[](https://clugen.github.io/pyclugen/)
[](https://pypi.org/project/pyclugen/)

[](https://tldrlegal.com/license/mit-license)
# pyclugen
**pyclugen** is a Python implementation of the *clugen* algorithm for
generating multidimensional clusters with arbitrary distributions. Each cluster
is supported by a line segment, the position, orientation and length of which
guide where the respective points are placed.
See the [documentation](https://clugen.github.io/pyclugen/) and
[examples](https://clugen.github.io/pyclugen/generated/gallery/) for more
details.
## Installation
Install from PyPI:
```sh
pip install --upgrade pip
pip install pyclugen
```
Or directly from GitHub:
```text
pip install --upgrade pip
pip install git+https://github.com/clugen/pyclugen.git#egg=pyclugen
```
## Quick start
```python
from pyclugen import clugen
import matplotlib.pyplot as plt
```
```python
out2 = clugen(2, 4, 400, [1, 0], 0.4, [50, 10], 20, 1, 2)
plt.scatter(out2.points[:, 0], out2.points[:, 1], c=out2.clusters)
plt.show()
```
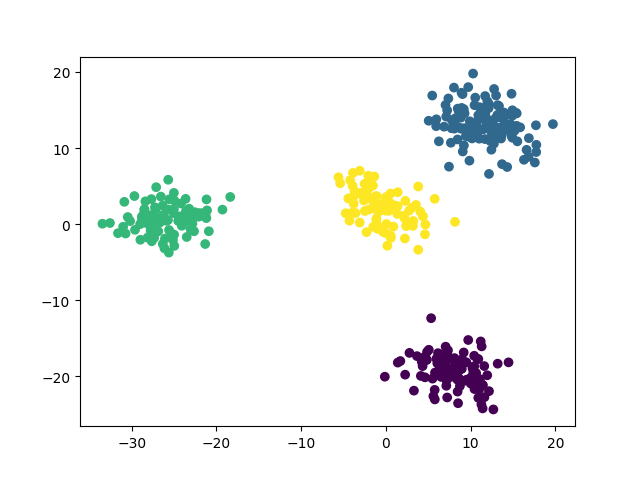
```python
out3 = clugen(3, 5, 10000, [0.5, 0.5, 0.5], 0.2, [10, 10, 10], 10, 1, 2)
fig = plt.figure()
ax = fig.add_subplot(projection="3d")
ax.scatter(out3.points[:, 0], out3.points[:, 1], out3.points[:, 2], c=out3.clusters)
plt.show()
```
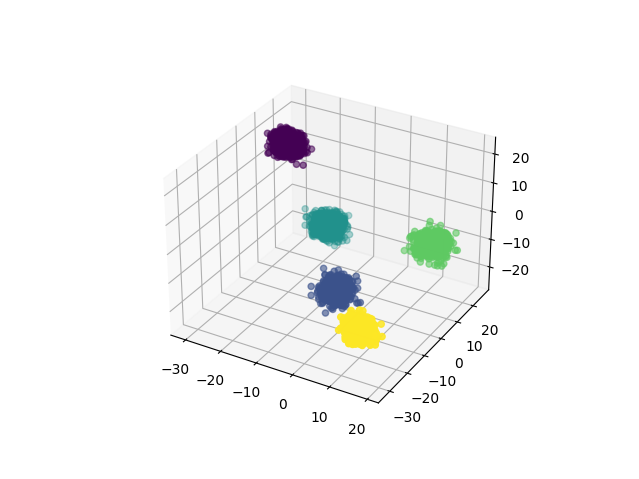
## See also
* [CluGen.jl](https://github.com/clugen/CluGen.jl/), a Julia implementation of
the *clugen* algorithm.
* [clugenr](https://github.com/clugen/clugenr/), an R implementation
of the *clugen* algorithm.
* [MOCluGen](https://github.com/clugen/MOCluGen/), a MATLAB/Octave
implementation of the *clugen* algorithm.
## Reference
If you use this software, please cite the following reference:
* Fachada, N. & de Andrade, D. (2023). Generating multidimensional clusters
with support lines. *Knowledge-Based Systems*, 277, 110836.
<https://doi.org/10.1016/j.knosys.2023.110836>
([arXiv preprint](https://doi.org/10.48550/arXiv.2301.10327))
## License
[MIT License](LICENSE.txt)
Raw data
{
"_id": null,
"home_page": null,
"name": "pyclugen",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "multidimensional data, synthetic clusters, synthetic data generation, synthetic data generator, multidimensional clusters, clustering",
"author": null,
"author_email": "Nuno Fachada <faken@fakenmc.com>",
"download_url": "https://files.pythonhosted.org/packages/1d/40/8bc85e38da40ca937eb2823886dbe82fe0abb23f69418456acb95818c59c/pyclugen-1.1.3.tar.gz",
"platform": null,
"description": "[](https://github.com/clugen/pyclugen/actions/workflows/tests.yml)\n[](https://codecov.io/gh/clugen/pyclugen)\n[](https://clugen.github.io/pyclugen/)\n[](https://pypi.org/project/pyclugen/)\n\n[](https://tldrlegal.com/license/mit-license)\n\n# pyclugen\n\n**pyclugen** is a Python implementation of the *clugen* algorithm for\ngenerating multidimensional clusters with arbitrary distributions. Each cluster\nis supported by a line segment, the position, orientation and length of which\nguide where the respective points are placed.\n\nSee the [documentation](https://clugen.github.io/pyclugen/) and\n[examples](https://clugen.github.io/pyclugen/generated/gallery/) for more\ndetails.\n\n## Installation\n\nInstall from PyPI:\n\n```sh\npip install --upgrade pip\npip install pyclugen\n```\n\nOr directly from GitHub:\n\n```text\npip install --upgrade pip\npip install git+https://github.com/clugen/pyclugen.git#egg=pyclugen\n```\n\n## Quick start\n\n```python\nfrom pyclugen import clugen\nimport matplotlib.pyplot as plt\n```\n\n```python\nout2 = clugen(2, 4, 400, [1, 0], 0.4, [50, 10], 20, 1, 2)\nplt.scatter(out2.points[:, 0], out2.points[:, 1], c=out2.clusters)\nplt.show()\n```\n\n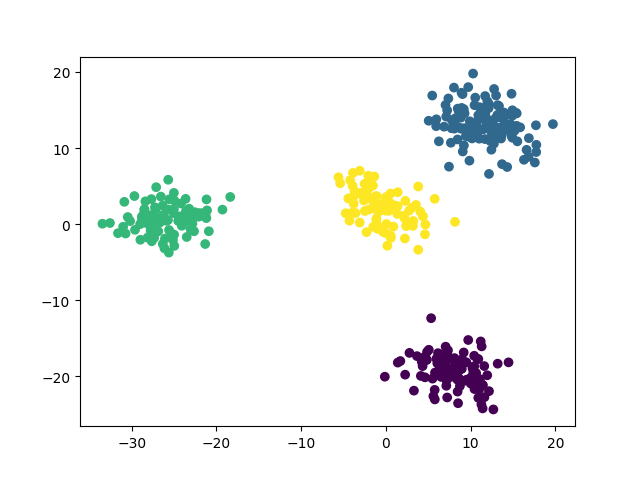\n\n```python\nout3 = clugen(3, 5, 10000, [0.5, 0.5, 0.5], 0.2, [10, 10, 10], 10, 1, 2)\nfig = plt.figure()\nax = fig.add_subplot(projection=\"3d\")\nax.scatter(out3.points[:, 0], out3.points[:, 1], out3.points[:, 2], c=out3.clusters)\nplt.show()\n```\n\n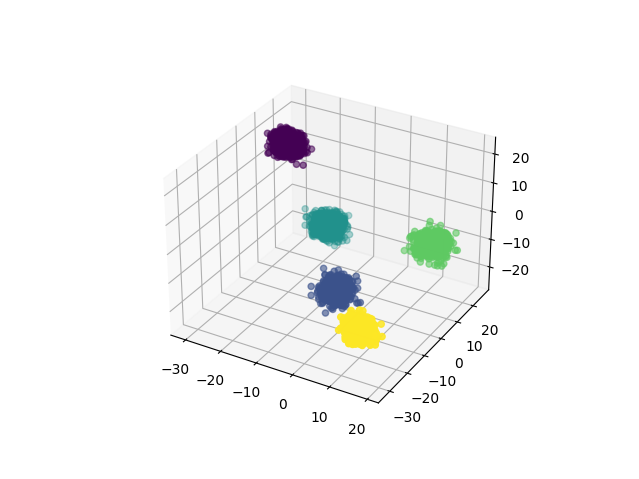\n\n## See also\n\n* [CluGen.jl](https://github.com/clugen/CluGen.jl/), a Julia implementation of\n the *clugen* algorithm.\n* [clugenr](https://github.com/clugen/clugenr/), an R implementation\n of the *clugen* algorithm.\n* [MOCluGen](https://github.com/clugen/MOCluGen/), a MATLAB/Octave\n implementation of the *clugen* algorithm.\n\n## Reference\n\nIf you use this software, please cite the following reference:\n\n* Fachada, N. & de Andrade, D. (2023). Generating multidimensional clusters\n with support lines. *Knowledge-Based Systems*, 277, 110836.\n <https://doi.org/10.1016/j.knosys.2023.110836>\n ([arXiv preprint](https://doi.org/10.48550/arXiv.2301.10327))\n\n## License\n\n[MIT License](LICENSE.txt)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Multidimensional cluster generation in Python",
"version": "1.1.3",
"project_urls": {
"Bug Reports": "https://github.com/clugen/pyclugen/issues",
"Documentation": "https://clugen.github.io/pyclugen/",
"Source": "https://github.com/clugen/pyclugen/"
},
"split_keywords": [
"multidimensional data",
" synthetic clusters",
" synthetic data generation",
" synthetic data generator",
" multidimensional clusters",
" clustering"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b90e7f6414b5118bc660f88f7c4fe0b9aead48e213468ae3886d47652529c7f6",
"md5": "b9fd82df39166a5aec6bef5e401335d2",
"sha256": "33be253795f304e818f7b5ef2f2c92a79a136e548f9df63ab22b7e8fe14ddedd"
},
"downloads": -1,
"filename": "pyclugen-1.1.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b9fd82df39166a5aec6bef5e401335d2",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 21407,
"upload_time": "2024-07-21T19:47:53",
"upload_time_iso_8601": "2024-07-21T19:47:53.751938Z",
"url": "https://files.pythonhosted.org/packages/b9/0e/7f6414b5118bc660f88f7c4fe0b9aead48e213468ae3886d47652529c7f6/pyclugen-1.1.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1d408bc85e38da40ca937eb2823886dbe82fe0abb23f69418456acb95818c59c",
"md5": "680061c78266a057bf9717274f8b35f4",
"sha256": "deb638c82db45ea010427e98a09f95381e8f38ea16f7c97fb090efbadb907f1d"
},
"downloads": -1,
"filename": "pyclugen-1.1.3.tar.gz",
"has_sig": false,
"md5_digest": "680061c78266a057bf9717274f8b35f4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 27358,
"upload_time": "2024-07-21T19:47:55",
"upload_time_iso_8601": "2024-07-21T19:47:55.189245Z",
"url": "https://files.pythonhosted.org/packages/1d/40/8bc85e38da40ca937eb2823886dbe82fe0abb23f69418456acb95818c59c/pyclugen-1.1.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-21 19:47:55",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "clugen",
"github_project": "pyclugen",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pyclugen"
}