[](https://mybinder.org/v2/gh/uber/deck.gl/binder)
[](https://pydeck.gl)
[](https://anaconda.org/conda-forge/pydeck)
[](https://pepy.tech/project/pydeck/week)
# pydeck: Large-scale interactive data visualization in Python
[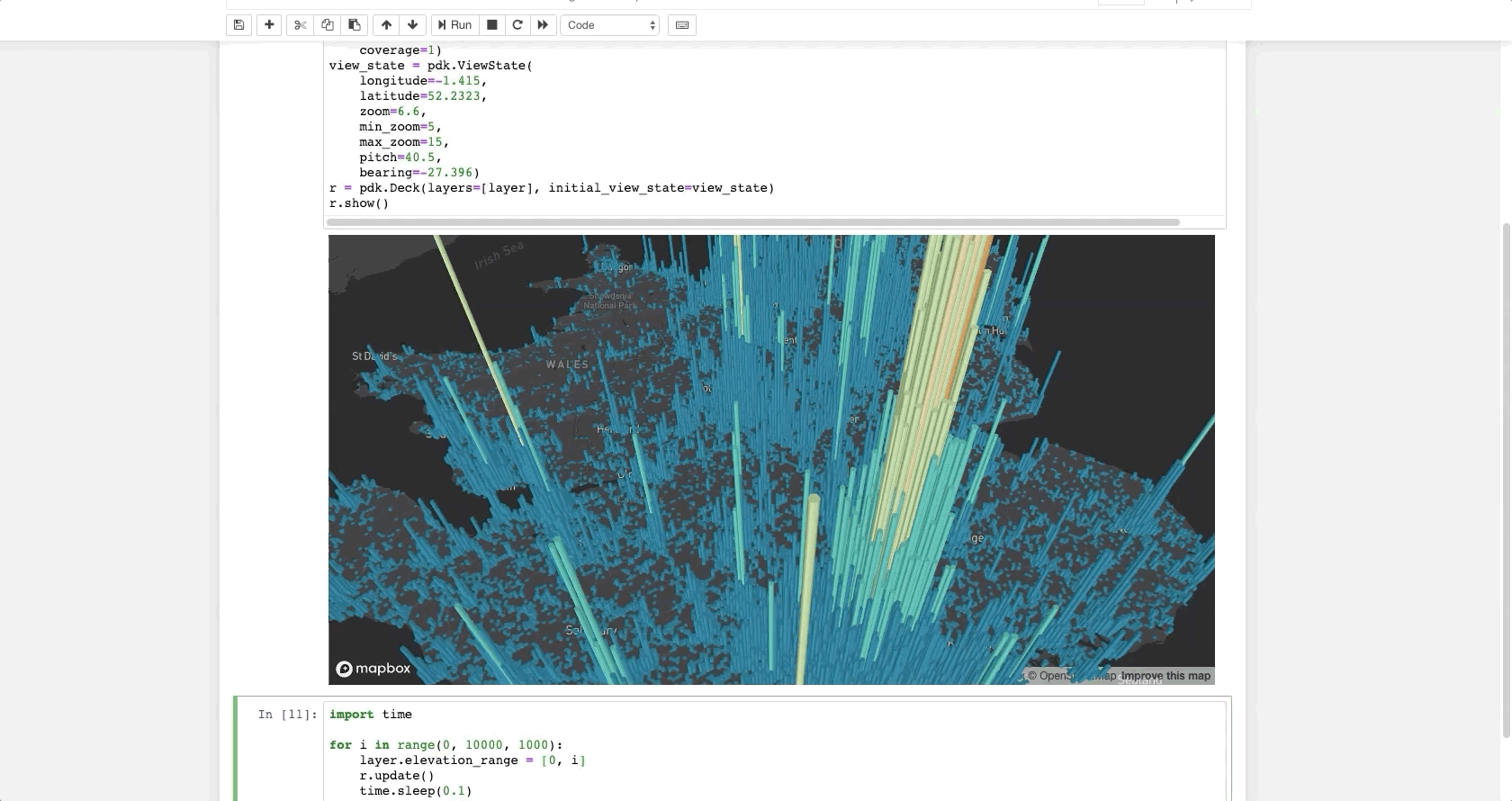](https://pydeck.gl/)
The pydeck library is a set of Python bindings for making spatial visualizations with [deck.gl](https://deck.gl),
optimized for a Jupyter environment. To get started, __[see the documentation](https://pydeck.gl/)__.
__[To install pydeck, see the instructions here](https://pydeck.gl/installation.html)__.
For __interactive demos__, click the binder logo below:
[](https://mybinder.org/v2/gh/uber/deck.gl/binder)
## Sample code
The following code renders a visualization similar to the one above in a Jupyter notebook:
```python
import pydeck as pdk
# 2014 locations of car accidents in the UK
UK_ACCIDENTS_DATA = ('https://raw.githubusercontent.com/uber-common/'
'deck.gl-data/master/examples/3d-heatmap/heatmap-data.csv')
# Define a layer to display on a map
layer = pdk.Layer(
'HexagonLayer',
UK_ACCIDENTS_DATA,
get_position=['lng', 'lat'],
auto_highlight=True,
elevation_scale=50,
pickable=True,
elevation_range=[0, 3000],
extruded=True,
coverage=1)
# Set the viewport location
view_state = pdk.ViewState(
longitude=-1.415,
latitude=52.2323,
zoom=6,
min_zoom=5,
max_zoom=15,
pitch=40.5,
bearing=-27.36)
# Render
r = pdk.Deck(layers=[layer], initial_view_state=view_state)
r.to_html('demo.html')
```
If you're developing outside a Jupyter environment, you can run:
```python
r.to_html('demo.html', notebook_display=False)
```
__[See the gallery for more examples.](https://pydeck.gl/#gallery)__
### Issues and contributing
If you encounter an issue, file it in the [deck.gl issues page](https://github.com/visgl/deck.gl/issues/new?assignees=&labels=question&template=question.md&title=)
and include your browser's console output, if any.
If you'd like to contribute to pydeck, please follow the [deck.gl contribution guidelines](https://github.com/visgl/deck.gl/blob/master/CONTRIBUTING.md)
and the [pydeck development installation instructions](https://pydeck.gl/installation.html#development-notes).
Raw data
{
"_id": null,
"home_page": "https://github.com/visgl/deck.gl/tree/master/bindings/pydeck",
"name": "pydeck",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "data, visualization, graphics, GIS, maps",
"author": "Andrew Duberstein",
"author_email": "ajduberstein@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/a1/ca/40e14e196864a0f61a92abb14d09b3d3da98f94ccb03b49cf51688140dab/pydeck-0.9.1.tar.gz",
"platform": null,
"description": "[](https://mybinder.org/v2/gh/uber/deck.gl/binder)\n[](https://pydeck.gl)\n[](https://anaconda.org/conda-forge/pydeck)\n[](https://pepy.tech/project/pydeck/week)\n\n# pydeck: Large-scale interactive data visualization in Python\n\n[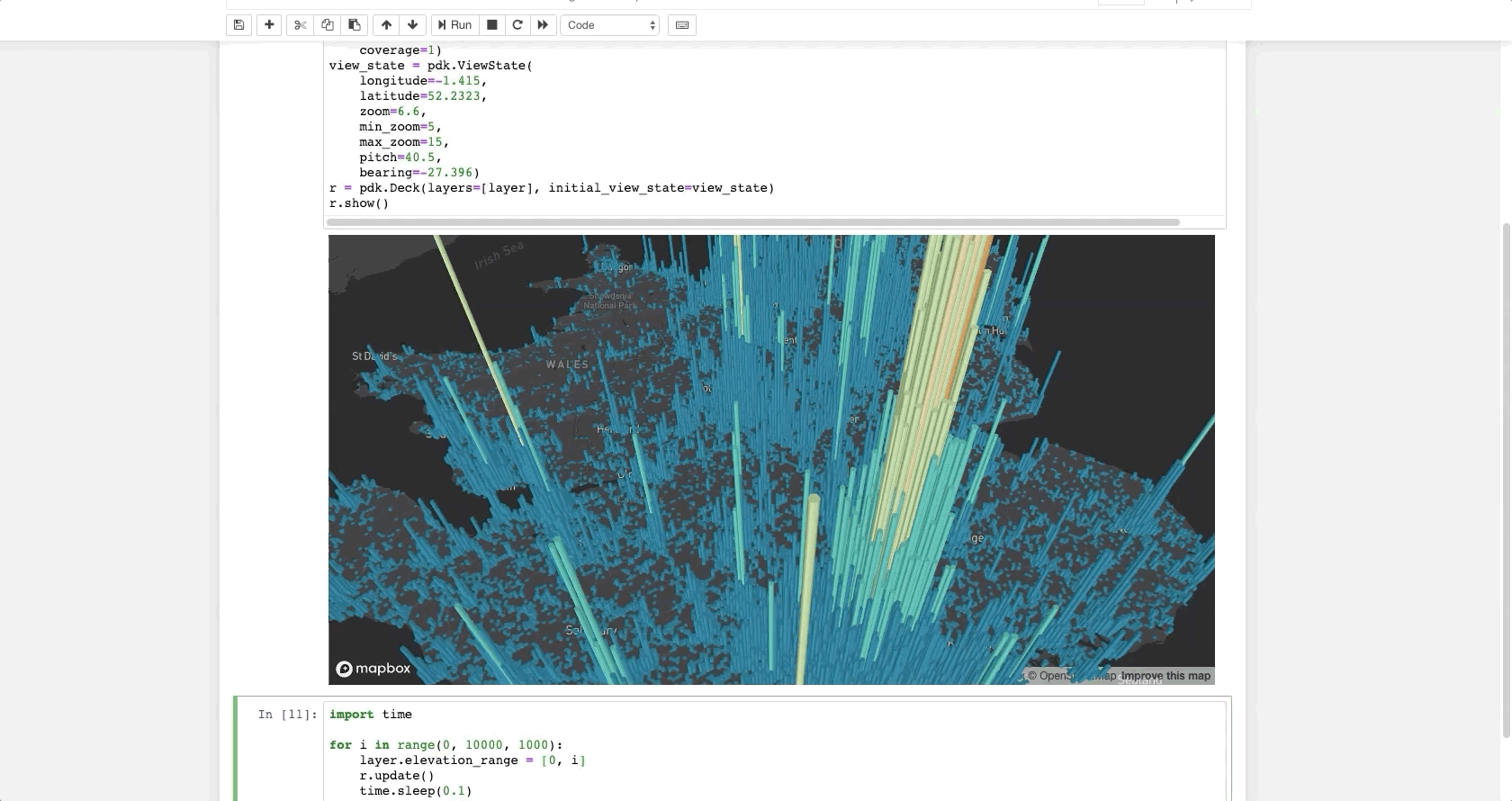](https://pydeck.gl/)\n\nThe pydeck library is a set of Python bindings for making spatial visualizations with [deck.gl](https://deck.gl),\noptimized for a Jupyter environment. To get started, __[see the documentation](https://pydeck.gl/)__.\n\n__[To install pydeck, see the instructions here](https://pydeck.gl/installation.html)__.\n\nFor __interactive demos__, click the binder logo below:\n\n[](https://mybinder.org/v2/gh/uber/deck.gl/binder)\n\n## Sample code\n\nThe following code renders a visualization similar to the one above in a Jupyter notebook:\n\n```python\nimport pydeck as pdk\n\n# 2014 locations of car accidents in the UK\nUK_ACCIDENTS_DATA = ('https://raw.githubusercontent.com/uber-common/'\n 'deck.gl-data/master/examples/3d-heatmap/heatmap-data.csv')\n\n# Define a layer to display on a map\nlayer = pdk.Layer(\n 'HexagonLayer',\n UK_ACCIDENTS_DATA,\n get_position=['lng', 'lat'],\n auto_highlight=True,\n elevation_scale=50,\n pickable=True,\n elevation_range=[0, 3000],\n extruded=True, \n coverage=1)\n\n# Set the viewport location\nview_state = pdk.ViewState(\n longitude=-1.415,\n latitude=52.2323,\n zoom=6,\n min_zoom=5,\n max_zoom=15,\n pitch=40.5,\n bearing=-27.36)\n\n# Render\nr = pdk.Deck(layers=[layer], initial_view_state=view_state)\nr.to_html('demo.html')\n```\n\nIf you're developing outside a Jupyter environment, you can run:\n\n```python\nr.to_html('demo.html', notebook_display=False)\n```\n\n__[See the gallery for more examples.](https://pydeck.gl/#gallery)__\n\n### Issues and contributing\n\nIf you encounter an issue, file it in the [deck.gl issues page](https://github.com/visgl/deck.gl/issues/new?assignees=&labels=question&template=question.md&title=)\nand include your browser's console output, if any.\n\nIf you'd like to contribute to pydeck, please follow the [deck.gl contribution guidelines](https://github.com/visgl/deck.gl/blob/master/CONTRIBUTING.md)\nand the [pydeck development installation instructions](https://pydeck.gl/installation.html#development-notes).\n",
"bugtrack_url": null,
"license": "Apache License 2.0",
"summary": "Widget for deck.gl maps",
"version": "0.9.1",
"project_urls": {
"Homepage": "https://github.com/visgl/deck.gl/tree/master/bindings/pydeck"
},
"split_keywords": [
"data",
" visualization",
" graphics",
" gis",
" maps"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ab4cb888e6cf58bd9db9c93f40d1c6be8283ff49d88919231afe93a6bcf61626",
"md5": "cd04dee9189179ef80d2ec80be8d694e",
"sha256": "b3f75ba0d273fc917094fa61224f3f6076ca8752b93d46faf3bcfd9f9d59b038"
},
"downloads": -1,
"filename": "pydeck-0.9.1-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "cd04dee9189179ef80d2ec80be8d694e",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": ">=3.8",
"size": 6900403,
"upload_time": "2024-05-10T15:36:17",
"upload_time_iso_8601": "2024-05-10T15:36:17.360098Z",
"url": "https://files.pythonhosted.org/packages/ab/4c/b888e6cf58bd9db9c93f40d1c6be8283ff49d88919231afe93a6bcf61626/pydeck-0.9.1-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a1ca40e14e196864a0f61a92abb14d09b3d3da98f94ccb03b49cf51688140dab",
"md5": "b7e8ffdaeeef00e9cbf776512cfb5f27",
"sha256": "f74475ae637951d63f2ee58326757f8d4f9cd9f2a457cf42950715003e2cb605"
},
"downloads": -1,
"filename": "pydeck-0.9.1.tar.gz",
"has_sig": false,
"md5_digest": "b7e8ffdaeeef00e9cbf776512cfb5f27",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 3832240,
"upload_time": "2024-05-10T15:36:21",
"upload_time_iso_8601": "2024-05-10T15:36:21.153633Z",
"url": "https://files.pythonhosted.org/packages/a1/ca/40e14e196864a0f61a92abb14d09b3d3da98f94ccb03b49cf51688140dab/pydeck-0.9.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-05-10 15:36:21",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "visgl",
"github_project": "deck.gl",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pydeck"
}