[](https://pypi.org/project/pygls/)   [](https://pygls.readthedocs.io/en/latest/)
# pygls: The Generic Language Server Framework
_pygls_ (pronounced like "pie glass") is a pythonic generic implementation of the [Language Server Protocol](https://microsoft.github.io/language-server-protocol/specification) for use as a foundation for writing your own [Language Servers](https://langserver.org/) in just a few lines of code.
## Quickstart
```python
from pygls.server import LanguageServer
from lsprotocol.types import (
TEXT_DOCUMENT_COMPLETION,
CompletionItem,
CompletionList,
CompletionParams,
)
server = LanguageServer("example-server", "v0.1")
@server.feature(TEXT_DOCUMENT_COMPLETION)
def completions(params: CompletionParams):
items = []
document = server.workspace.get_document(params.text_document.uri)
current_line = document.lines[params.position.line].strip()
if current_line.endswith("hello."):
items = [
CompletionItem(label="world"),
CompletionItem(label="friend"),
]
return CompletionList(is_incomplete=False, items=items)
server.start_io()
```
Which might look something like this when you trigger autocompletion in your editor:
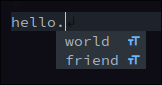
## Docs and Tutorial
The full documentation and a tutorial are available at <https://pygls.readthedocs.io/en/latest/>.
## Projects based on _pygls_
We keep a table of all known _pygls_ [implementations](https://github.com/openlawlibrary/pygls/blob/master/Implementations.md). Please submit a Pull Request with your own or any that you find are missing.
## Alternatives
The main alternative to _pygls_ is Microsoft's [NodeJS-based Generic Language Server Framework](https://github.com/microsoft/vscode-languageserver-node). Being from Microsoft it is focussed on extending VSCode, although in theory it could be used to support any editor. So this is where pygls might be a better choice if you want to support more editors, as pygls is not focussed around VSCode.
There are also other Language Servers with "general" in their descriptons, or at least intentions. They are however only general in the sense of having powerful _configuration_. They achieve generality in so much as configuration is able to, as opposed to what programming (in _pygls'_ case) can achieve.
* https://github.com/iamcco/diagnostic-languageserver
* https://github.com/mattn/efm-langserver
* https://github.com/jose-elias-alvarez/null-ls.nvim (Neovim only)
## Tests
All Pygls sub-tasks require the Poetry `poe` plugin: https://github.com/nat-n/poethepoet
* `poetry install --all-extras`
* `poetry run poe test`
* `poetry run poe test-pyodide`
## Contributing
Your contributions to _pygls_ are most welcome ❤️ Please review the [Contributing](https://github.com/openlawlibrary/pygls/blob/master/CONTRIBUTING.md) and [Code of Conduct](https://github.com/openlawlibrary/pygls/blob/master/CODE_OF_CONDUCT.md) documents for how to get started.
## Donating
[Open Law Library](http://www.openlawlib.org/) is a 501(c)(3) tax exempt organization. Help us maintain our open source projects and open the law to all with [sponsorship](https://github.com/sponsors/openlawlibrary).
### Supporters
We would like to give special thanks to the following supporters:
* [mpourmpoulis](https://github.com/mpourmpoulis)
## License
Apache-2.0
Raw data
{
"_id": null,
"home_page": "https://github.com/openlawlibrary/pygls",
"name": "pygls",
"maintainer": "Tom BH",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "tom@tombh.co.uk",
"keywords": null,
"author": "Open Law Library",
"author_email": "info@openlawlib.org",
"download_url": "https://files.pythonhosted.org/packages/86/b9/41d173dad9eaa9db9c785a85671fc3d68961f08d67706dc2e79011e10b5c/pygls-1.3.1.tar.gz",
"platform": null,
"description": "[](https://pypi.org/project/pygls/)   [](https://pygls.readthedocs.io/en/latest/)\n\n# pygls: The Generic Language Server Framework\n\n_pygls_ (pronounced like \"pie glass\") is a pythonic generic implementation of the [Language Server Protocol](https://microsoft.github.io/language-server-protocol/specification) for use as a foundation for writing your own [Language Servers](https://langserver.org/) in just a few lines of code.\n\n## Quickstart\n```python\nfrom pygls.server import LanguageServer\nfrom lsprotocol.types import (\n TEXT_DOCUMENT_COMPLETION,\n CompletionItem,\n CompletionList,\n CompletionParams,\n)\n\nserver = LanguageServer(\"example-server\", \"v0.1\")\n\n@server.feature(TEXT_DOCUMENT_COMPLETION)\ndef completions(params: CompletionParams):\n items = []\n document = server.workspace.get_document(params.text_document.uri)\n current_line = document.lines[params.position.line].strip()\n if current_line.endswith(\"hello.\"):\n items = [\n CompletionItem(label=\"world\"),\n CompletionItem(label=\"friend\"),\n ]\n return CompletionList(is_incomplete=False, items=items)\n\nserver.start_io()\n```\n\nWhich might look something like this when you trigger autocompletion in your editor:\n\n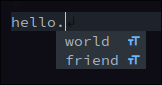\n\n## Docs and Tutorial\n\nThe full documentation and a tutorial are available at <https://pygls.readthedocs.io/en/latest/>.\n\n## Projects based on _pygls_\n\nWe keep a table of all known _pygls_ [implementations](https://github.com/openlawlibrary/pygls/blob/master/Implementations.md). Please submit a Pull Request with your own or any that you find are missing.\n\n## Alternatives\n\nThe main alternative to _pygls_ is Microsoft's [NodeJS-based Generic Language Server Framework](https://github.com/microsoft/vscode-languageserver-node). Being from Microsoft it is focussed on extending VSCode, although in theory it could be used to support any editor. So this is where pygls might be a better choice if you want to support more editors, as pygls is not focussed around VSCode.\n\nThere are also other Language Servers with \"general\" in their descriptons, or at least intentions. They are however only general in the sense of having powerful _configuration_. They achieve generality in so much as configuration is able to, as opposed to what programming (in _pygls'_ case) can achieve.\n * https://github.com/iamcco/diagnostic-languageserver\n * https://github.com/mattn/efm-langserver\n * https://github.com/jose-elias-alvarez/null-ls.nvim (Neovim only)\n\n## Tests\nAll Pygls sub-tasks require the Poetry `poe` plugin: https://github.com/nat-n/poethepoet\n\n* `poetry install --all-extras`\n* `poetry run poe test`\n* `poetry run poe test-pyodide`\n\n\n## Contributing\n\nYour contributions to _pygls_ are most welcome \u2764\ufe0f Please review the [Contributing](https://github.com/openlawlibrary/pygls/blob/master/CONTRIBUTING.md) and [Code of Conduct](https://github.com/openlawlibrary/pygls/blob/master/CODE_OF_CONDUCT.md) documents for how to get started.\n\n## Donating\n\n[Open Law Library](http://www.openlawlib.org/) is a 501(c)(3) tax exempt organization. Help us maintain our open source projects and open the law to all with [sponsorship](https://github.com/sponsors/openlawlibrary).\n\n### Supporters\n\nWe would like to give special thanks to the following supporters:\n* [mpourmpoulis](https://github.com/mpourmpoulis)\n\n## License\n\nApache-2.0\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "A pythonic generic language server (pronounced like 'pie glass')",
"version": "1.3.1",
"project_urls": {
"Documentation": "https://pygls.readthedocs.io/en/latest",
"Homepage": "https://github.com/openlawlibrary/pygls",
"Repository": "https://github.com/openlawlibrary/pygls"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1119b74a10dd24548e96e8c80226cbacb28b021bc3a168a7d2709fb0d0185348",
"md5": "b5d205aa1363104b1eb60880741c8557",
"sha256": "6e00f11efc56321bdeb6eac04f6d86131f654c7d49124344a9ebb968da3dd91e"
},
"downloads": -1,
"filename": "pygls-1.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b5d205aa1363104b1eb60880741c8557",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 56031,
"upload_time": "2024-03-26T18:44:24",
"upload_time_iso_8601": "2024-03-26T18:44:24.249547Z",
"url": "https://files.pythonhosted.org/packages/11/19/b74a10dd24548e96e8c80226cbacb28b021bc3a168a7d2709fb0d0185348/pygls-1.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "86b941d173dad9eaa9db9c785a85671fc3d68961f08d67706dc2e79011e10b5c",
"md5": "aed9156986352710d416de87022d1137",
"sha256": "140edceefa0da0e9b3c533547c892a42a7d2fd9217ae848c330c53d266a55018"
},
"downloads": -1,
"filename": "pygls-1.3.1.tar.gz",
"has_sig": false,
"md5_digest": "aed9156986352710d416de87022d1137",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 45527,
"upload_time": "2024-03-26T18:44:25",
"upload_time_iso_8601": "2024-03-26T18:44:25.679512Z",
"url": "https://files.pythonhosted.org/packages/86/b9/41d173dad9eaa9db9c785a85671fc3d68961f08d67706dc2e79011e10b5c/pygls-1.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-26 18:44:25",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "openlawlibrary",
"github_project": "pygls",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "pygls"
}