### PyKite: A research and development initiative for crafting a Python-centric micro framework.
[//]: # ()
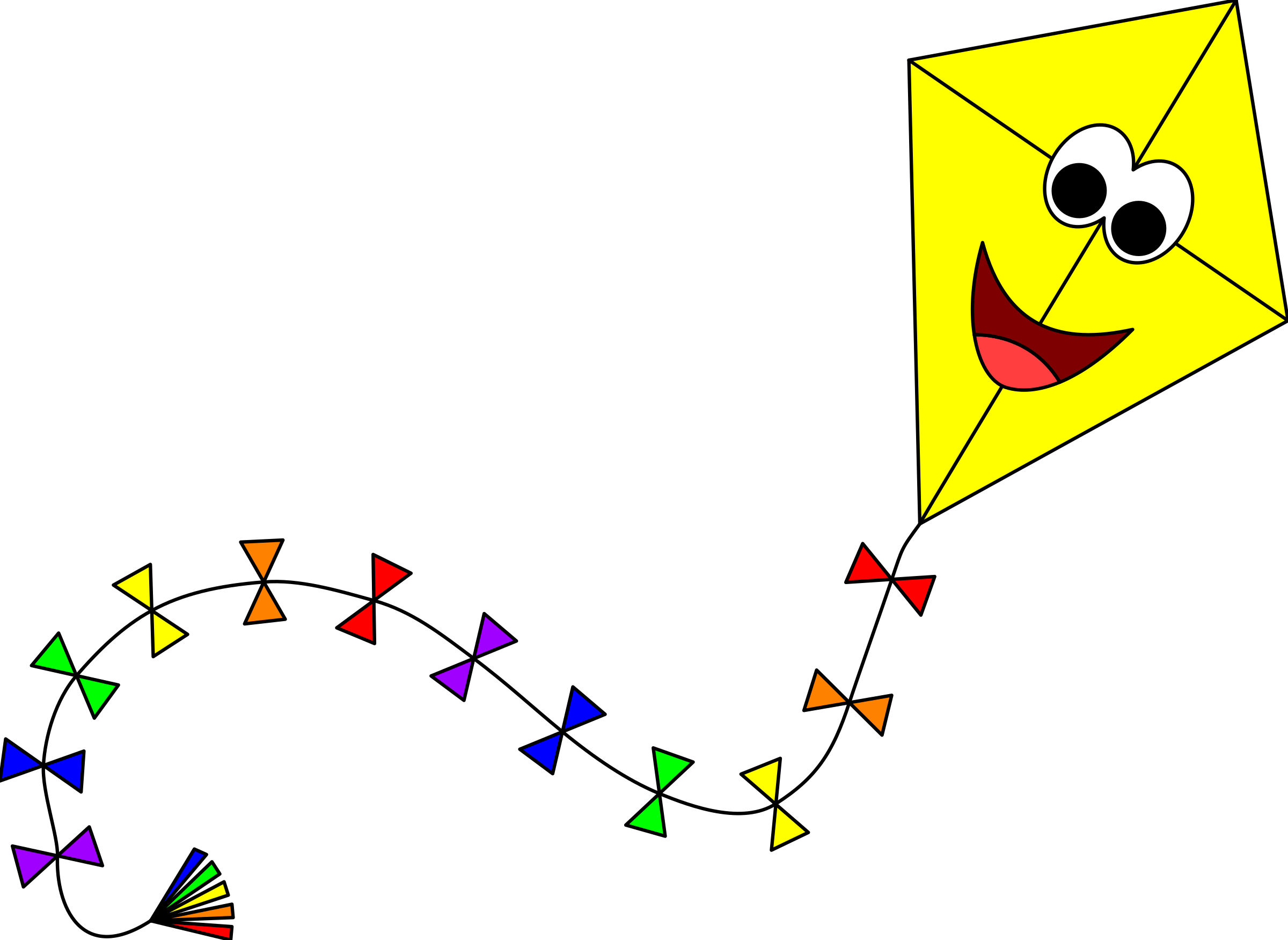
## Features
- **Route Definition:** Define routes using the `@app.route(path)` decorator.
- **HTTP Request Handling:** Create handler functions for each route to process and respond to incoming HTTP requests.
- **Development Server:** Start a development server with a single command, making it easy to test your application locally.
- **Basic Error Handling:** Includes a default 404 response for routes that are not found.
## Installation
To get started with PyKite, follow these steps:
```bash
pip install pykite
```
## Run the application
1. Create a Python script for your web application using PyKite. Define routes and handlers as needed.
2. Run your application using the `run` method:
```python
from pykite import PyKite
from pykite.http.response import Response
# Create a PyKite application
app = PyKite(debug=True)
# Define a route for the '/' path
@app.route('/')
def index(request, response):
"""Respond with a JSON object containing the message "Hello, World!" to all requests to the '/' path."""
data = {"message": "Hello, World!"}
response = Response(data=data, status=200)
return response
@app.route('/hello/{name}')
def hello(request, response, name):
""" Took a name from the URL and responds with a friendly greeting in JSON."""
data = {"message": f"Hello, {name}"}
response = Response(data=data, status=200)
return response
# Run the application
if __name__ == "__main__":
app.run()
```
3. Access your application in a web browser at http://localhost:8000.
## Middleware
Middleware in web development refers to software components that sit between a web server and an application. They intercept requests and responses, allowing for additional processing or modification before they reach the application or client. Middleware can be used for tasks like authentication, authorization, logging, caching, and more. Let's see [ 👉 how to use middleware in `PyKite`](docs/middleware.md) framework.
## License
This project is licensed under the [MIT License](LICENSE).
Raw data
{
"_id": null,
"home_page": "https://github.com/imamhossainroni/pykite",
"name": "pykite",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "pykite, web framework, micro framework, web applications, Python, HTTP, WSGI",
"author": "Imam Hossain Roni",
"author_email": "imamhossainroni95@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/2c/a8/287b463cb93676e79f3a9c51b0e3ac6e4c563a473d513c1e39a328821a22/pykite-1.0.1.tar.gz",
"platform": null,
"description": "### PyKite: A research and development initiative for crafting a Python-centric micro framework.\n\n[//]: # ()\n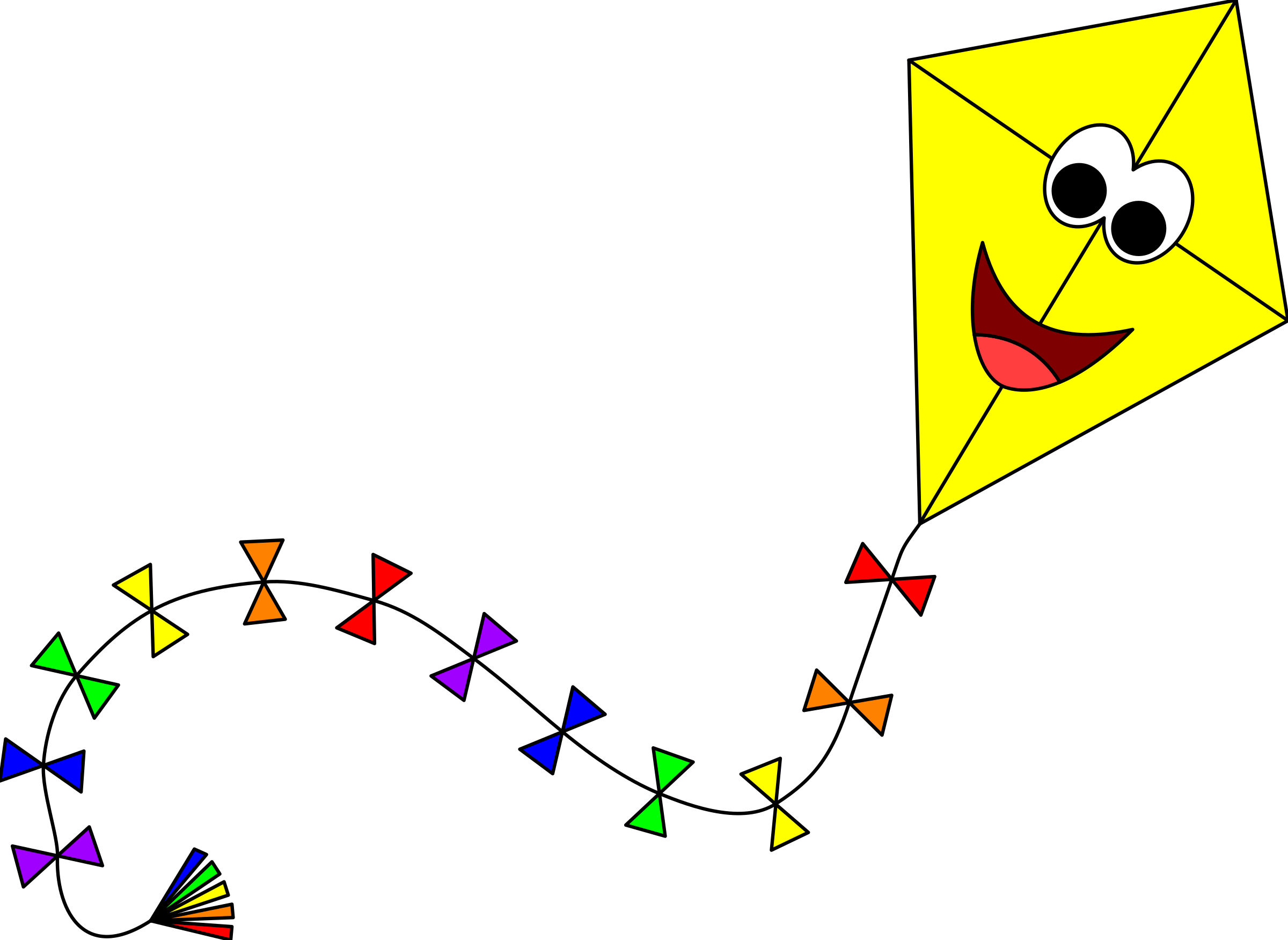\n\n## Features\n\n- **Route Definition:** Define routes using the `@app.route(path)` decorator.\n\n- **HTTP Request Handling:** Create handler functions for each route to process and respond to incoming HTTP requests.\n\n- **Development Server:** Start a development server with a single command, making it easy to test your application locally.\n\n- **Basic Error Handling:** Includes a default 404 response for routes that are not found.\n\n\n## Installation\nTo get started with PyKite, follow these steps:\n```bash\npip install pykite\n```\n## Run the application\n1. Create a Python script for your web application using PyKite. Define routes and handlers as needed.\n2. Run your application using the `run` method:\n\n```python\nfrom pykite import PyKite\nfrom pykite.http.response import Response\n\n# Create a PyKite application\napp = PyKite(debug=True)\n\n\n# Define a route for the '/' path\n@app.route('/')\ndef index(request, response):\n \"\"\"Respond with a JSON object containing the message \"Hello, World!\" to all requests to the '/' path.\"\"\"\n data = {\"message\": \"Hello, World!\"}\n response = Response(data=data, status=200)\n return response\n\n\n@app.route('/hello/{name}')\ndef hello(request, response, name):\n \"\"\" Took a name from the URL and responds with a friendly greeting in JSON.\"\"\"\n data = {\"message\": f\"Hello, {name}\"}\n response = Response(data=data, status=200)\n return response\n\n\n# Run the application\nif __name__ == \"__main__\":\n app.run()\n\n```\n3. Access your application in a web browser at http://localhost:8000.\n\n## Middleware\nMiddleware in web development refers to software components that sit between a web server and an application. They intercept requests and responses, allowing for additional processing or modification before they reach the application or client. Middleware can be used for tasks like authentication, authorization, logging, caching, and more. Let's see [ \ud83d\udc49 how to use middleware in `PyKite`](docs/middleware.md) framework. \n\n\n## License\n\nThis project is licensed under the [MIT License](LICENSE).\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python-centric micro-framework for building web applications",
"version": "1.0.1",
"project_urls": {
"Bug Tracker": "https://github.com/imamhossainroni/pykite/issues",
"Documentation": "https://github.com/imamhossainroni/pykite/blob/main/README.md",
"Homepage": "https://github.com/imamhossainroni/pykite",
"Icon Image": "https://raw.githubusercontent.com/ImamHossainRoni/pykite/main/extras/yellow-kite.png",
"Source Code": "https://github.com/imamhossainroni/pykite"
},
"split_keywords": [
"pykite",
" web framework",
" micro framework",
" web applications",
" python",
" http",
" wsgi"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "69392f31288d698e0d1b2740a972a97b264d23df30397797772651831fca4bb6",
"md5": "6df7cddb08998f67a7389d0cdfc7358d",
"sha256": "312c3f29033450fca39bea28a52276e2af6768ab0200b0408e16a11e6c1b908b"
},
"downloads": -1,
"filename": "pykite-1.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6df7cddb08998f67a7389d0cdfc7358d",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 9764,
"upload_time": "2024-10-12T18:51:34",
"upload_time_iso_8601": "2024-10-12T18:51:34.615543Z",
"url": "https://files.pythonhosted.org/packages/69/39/2f31288d698e0d1b2740a972a97b264d23df30397797772651831fca4bb6/pykite-1.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2ca8287b463cb93676e79f3a9c51b0e3ac6e4c563a473d513c1e39a328821a22",
"md5": "fd6721f97da4cab0a0286512e3d0f352",
"sha256": "322a96fd8214dea9a4fbc77d2196fabe594cd6349855666cd97766af1ad8cd8a"
},
"downloads": -1,
"filename": "pykite-1.0.1.tar.gz",
"has_sig": false,
"md5_digest": "fd6721f97da4cab0a0286512e3d0f352",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 8230,
"upload_time": "2024-10-12T18:51:36",
"upload_time_iso_8601": "2024-10-12T18:51:36.152279Z",
"url": "https://files.pythonhosted.org/packages/2c/a8/287b463cb93676e79f3a9c51b0e3ac6e4c563a473d513c1e39a328821a22/pykite-1.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-12 18:51:36",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "imamhossainroni",
"github_project": "pykite",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "pykite"
}