# Rigol MSO5xxx oscilloscope Python library (unofficial)
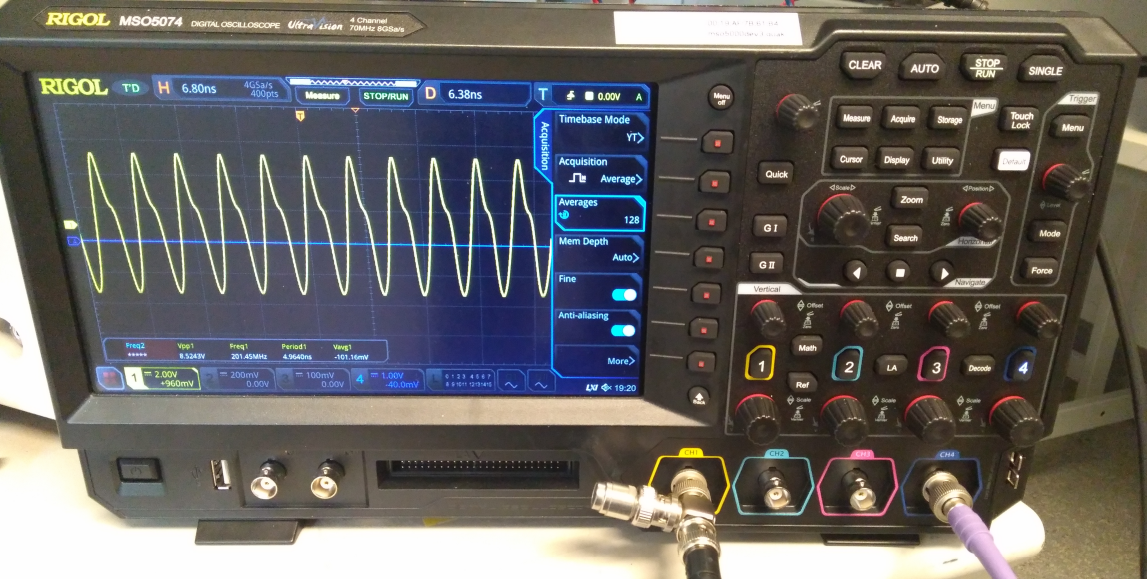
A simple Python library and utility to control and query data from
Rigol MSO5xxx oscilloscopes (not supporting all features of the oscilloscope,
work in progress). This library implements the [Oscilloscope](https://github.com/tspspi/pylabdevs/blob/master/src/labdevices/oscilloscope.py) class from
the [pylabdevs](https://github.com/tspspi/pylabdevs) package which
exposes the public interface.
Patches for raw mode sample query by [MasterJubei](https://github.com/MasterJubei)
## Installing
There is a PyPi package that can be installed using
```
pip install pymso5000-tspspi
```
## Simple example to fetch waveforms:
```
from pymso5000.mso5000 import MSO5000
with MSO5000(address = "10.0.0.123") as mso:
print(f"Identify: {mso.identify()}")
mso.set_channel_enable(0, True)
mso.set_channel_enable(1, True)
data = mso.query_waveform((0, 1))
print(data)
import matplotlib.pyplot as plt
plt.plot(data['x'], data['y0'], label = "Ch1")
plt.plot(data['x'], data['y1'], label = "Ch2")
plt.show()
```
Note that ```numpy``` usage is optional for this implementation.
One can enable numpy support using ```useNumpy = True``` in the
constructor.
## Querying additional statistics
This module allows - via the ```pylabdevs``` base class to query
additional statistics:
* ```mean``` Calculates the mean values and standard deviations
* A single value for each channels mean at ```["means"]["yN_avg"]```
and a single value for each standard deviation at ```["means"]["yN_std"]```
where ```N``` is the channel number
* ```fft``` runs Fourier transform on all queried traces
* The result is stored in ```["fft"]["yN"]``` (complex values) and
in ```["fft"]["yN_real"]``` for the real valued Fourier transform.
Again ```N``` is the channel number
* ```ifft``` runs inverse Fourier transform on all queried traces
* Works as ```fft``` but runs the inverse Fourier transform and stores
its result in ```ifft``` instead of ```fft```
* ```correlate``` calculates the correlation between all queried
waveform pairs.
* The result of the correlations are stored in ```["correlation"]["yNyM"]```
for the correlation between channels ```M``` and ```N```
* ```autocorrelate``` performs calculation of the autocorrelation of each
queried channel.
* The result of the autocorrelation is stored in ```["autocorrelation"]["yN"]```
for channel ```N```
To request calculation of statistics pass the string for the
desired statistic or a list of statistics to the ```stats```
parameter of ```query_waveform```:
```
with MSO5000(address = "10.0.0.123") as mso:
data = mso.query_waveform((1,2), stats = [ 'mean', 'fft' ])
```
## Supported methods
More documentation in progress ...
* ```identify()```
* Connection management (when not using ```with``` context management):
* ```connect()```
* ```disconnect()```
* ```set_channel_enable(channel, enabled)```
* ```is_channel_enabled(channel)```
* ```set_sweep_mode(mode)```
* ```get_sweep_mode()```
* ```set_trigger_mode(mode)```
* ```get_trigger_mode()```
* ```force_trigger()```
* ```set_timebase_mode(mode)```
* ```get_timebase_mode()```
* ```set_run_mode(mode)```
* ```get_run_mode()```
* ```set_timebase_scale(secondsPerDivision)```
* ```get_timebase_scale()```
* ```set_channel_coupling(channel, couplingMode)```
* ```get_channel_coupling(channel)```
* ```set_channel_probe_ratio(channel, ratio)```
* ```get_channel_probe_ratio(channel)```
* ```set_channel_scale(channel, scale)```
* ```get_channel_scale(channel)```
* ```query_waveform(channel, stats = None)```
* ```off()```
## CLI fetching utility
This package comes with a ```mso5000fetch``` command line utility. This utility
allows one to simply fetch one or more traces and store them either inside an npz
or a matplotlib plot. In addition it can run all of the ```pylabdevs``` statistics
functions (currently no plot, only stored in the npz) and execute manually assisted
differential scans.
Help for this utility is available via ```mso5000fetch --help```
Raw data
{
"_id": null,
"home_page": "https://github.com/tspspi/pymso5000",
"name": "pymso5000-tspspi",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": null,
"author": "Thomas Spielauer",
"author_email": "pypipackages01@tspi.at",
"download_url": "https://files.pythonhosted.org/packages/5f/08/83c83de3b1ca96a7d890840bbe551926b6946f0e57149c3649126ffe1e68/pymso5000_tspspi-0.0.10.tar.gz",
"platform": null,
"description": "# Rigol MSO5xxx oscilloscope Python library (unofficial)\n\n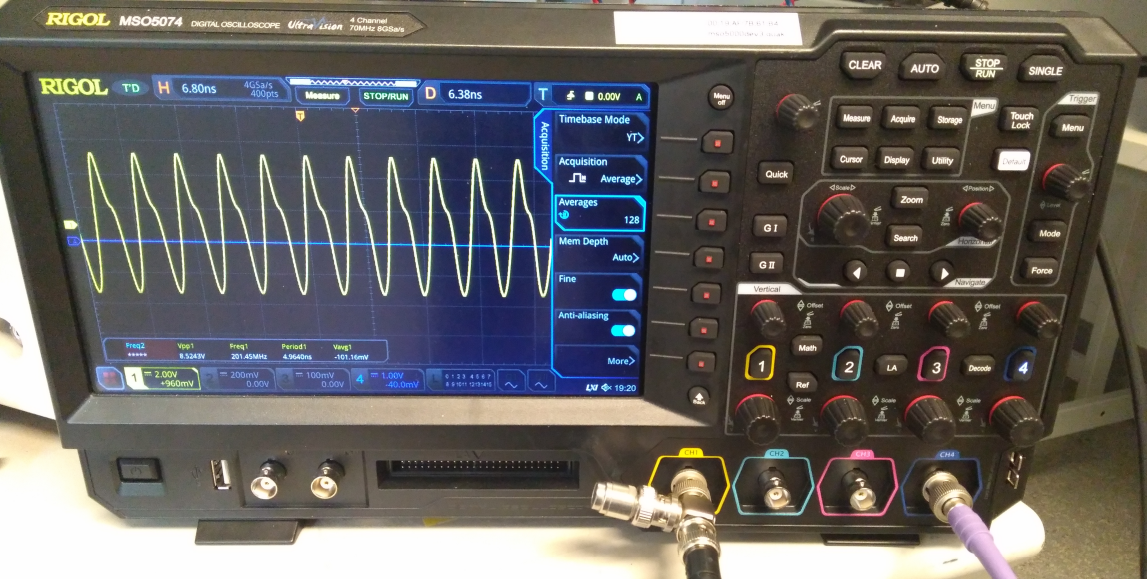\n\nA simple Python library and utility to control and query data from\nRigol MSO5xxx oscilloscopes (not supporting all features of the oscilloscope,\nwork in progress). This library implements the [Oscilloscope](https://github.com/tspspi/pylabdevs/blob/master/src/labdevices/oscilloscope.py) class from\nthe [pylabdevs](https://github.com/tspspi/pylabdevs) package which\nexposes the public interface.\n\nPatches for raw mode sample query by [MasterJubei](https://github.com/MasterJubei)\n\n## Installing \n\nThere is a PyPi package that can be installed using\n\n```\npip install pymso5000-tspspi\n```\n\n## Simple example to fetch waveforms:\n\n```\nfrom pymso5000.mso5000 import MSO5000\n\nwith MSO5000(address = \"10.0.0.123\") as mso:\n print(f\"Identify: {mso.identify()}\")\n\n mso.set_channel_enable(0, True)\n mso.set_channel_enable(1, True)\n\n data = mso.query_waveform((0, 1))\n print(data)\n\n import matplotlib.pyplot as plt\n plt.plot(data['x'], data['y0'], label = \"Ch1\")\n plt.plot(data['x'], data['y1'], label = \"Ch2\")\n plt.show()\n```\n\nNote that ```numpy``` usage is optional for this implementation.\nOne can enable numpy support using ```useNumpy = True``` in the\nconstructor.\n\n## Querying additional statistics\n\nThis module allows - via the ```pylabdevs``` base class to query\nadditional statistics:\n\n* ```mean``` Calculates the mean values and standard deviations\n * A single value for each channels mean at ```[\"means\"][\"yN_avg\"]```\n and a single value for each standard deviation at ```[\"means\"][\"yN_std\"]```\n where ```N``` is the channel number\n* ```fft``` runs Fourier transform on all queried traces\n * The result is stored in ```[\"fft\"][\"yN\"]``` (complex values) and\n in ```[\"fft\"][\"yN_real\"]``` for the real valued Fourier transform.\n Again ```N``` is the channel number\n* ```ifft``` runs inverse Fourier transform on all queried traces\n * Works as ```fft``` but runs the inverse Fourier transform and stores\n its result in ```ifft``` instead of ```fft```\n* ```correlate``` calculates the correlation between all queried\n waveform pairs.\n * The result of the correlations are stored in ```[\"correlation\"][\"yNyM\"]```\n for the correlation between channels ```M``` and ```N```\n* ```autocorrelate``` performs calculation of the autocorrelation of each\n queried channel.\n * The result of the autocorrelation is stored in ```[\"autocorrelation\"][\"yN\"]```\n for channel ```N```\n\nTo request calculation of statistics pass the string for the\ndesired statistic or a list of statistics to the ```stats```\nparameter of ```query_waveform```:\n\n```\nwith MSO5000(address = \"10.0.0.123\") as mso:\n\tdata = mso.query_waveform((1,2), stats = [ 'mean', 'fft' ])\n```\n\n## Supported methods\n\nMore documentation in progress ...\n\n* ```identify()```\n* Connection management (when not using ```with``` context management):\n * ```connect()```\n * ```disconnect()```\n* ```set_channel_enable(channel, enabled)```\n* ```is_channel_enabled(channel)```\n* ```set_sweep_mode(mode)```\n* ```get_sweep_mode()```\n* ```set_trigger_mode(mode)```\n* ```get_trigger_mode()```\n* ```force_trigger()```\n* ```set_timebase_mode(mode)```\n* ```get_timebase_mode()```\n* ```set_run_mode(mode)```\n* ```get_run_mode()```\n* ```set_timebase_scale(secondsPerDivision)```\n* ```get_timebase_scale()```\n* ```set_channel_coupling(channel, couplingMode)```\n* ```get_channel_coupling(channel)```\n* ```set_channel_probe_ratio(channel, ratio)```\n* ```get_channel_probe_ratio(channel)```\n* ```set_channel_scale(channel, scale)```\n* ```get_channel_scale(channel)```\n* ```query_waveform(channel, stats = None)```\n* ```off()```\n\n## CLI fetching utility\n\nThis package comes with a ```mso5000fetch``` command line utility. This utility\nallows one to simply fetch one or more traces and store them either inside an npz\nor a matplotlib plot. In addition it can run all of the ```pylabdevs``` statistics\nfunctions (currently no plot, only stored in the npz) and execute manually assisted\ndifferential scans.\n\nHelp for this utility is available via ```mso5000fetch --help```\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Rigol MSO5000 control library (unofficial)",
"version": "0.0.10",
"project_urls": {
"Homepage": "https://github.com/tspspi/pymso5000"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "43ad8f7d5c553dc2ef9b745657d70b8b392e9c6bfbd736aa7bbf37f3675cd64c",
"md5": "e02893c7bc6b8319ad005148cb297722",
"sha256": "bea5210ff249884508855622f39a659a8ebef8e524fe823cd5d30bf20b61cfe9"
},
"downloads": -1,
"filename": "pymso5000_tspspi-0.0.10-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e02893c7bc6b8319ad005148cb297722",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 11577,
"upload_time": "2024-07-25T20:32:31",
"upload_time_iso_8601": "2024-07-25T20:32:31.103935Z",
"url": "https://files.pythonhosted.org/packages/43/ad/8f7d5c553dc2ef9b745657d70b8b392e9c6bfbd736aa7bbf37f3675cd64c/pymso5000_tspspi-0.0.10-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5f0883c83de3b1ca96a7d890840bbe551926b6946f0e57149c3649126ffe1e68",
"md5": "03911ed334affc07d449dcb22e49473a",
"sha256": "7b60da8af60eccaf473e466617c72bd654ab295942f71d9fc375c5bb4e956605"
},
"downloads": -1,
"filename": "pymso5000_tspspi-0.0.10.tar.gz",
"has_sig": false,
"md5_digest": "03911ed334affc07d449dcb22e49473a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 12353,
"upload_time": "2024-07-25T20:32:32",
"upload_time_iso_8601": "2024-07-25T20:32:32.724950Z",
"url": "https://files.pythonhosted.org/packages/5f/08/83c83de3b1ca96a7d890840bbe551926b6946f0e57149c3649126ffe1e68/pymso5000_tspspi-0.0.10.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-25 20:32:32",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tspspi",
"github_project": "pymso5000",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "pymso5000-tspspi"
}